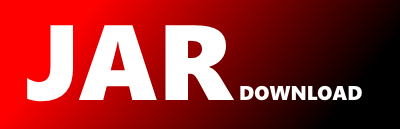
software.amazon.awssdk.services.ec2.model.DescribeInstanceStatusRequest Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeInstanceStatusRequest extends Ec2Request implements
ToCopyableBuilder {
private static final SdkField> INSTANCE_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("InstanceIds")
.getter(getter(DescribeInstanceStatusRequest::instanceIds))
.setter(setter(Builder::instanceIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceId")
.unmarshallLocationName("InstanceId").build(),
ListTrait
.builder()
.memberLocationName("InstanceId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("InstanceId").unmarshallLocationName("InstanceId").build())
.build()).build()).build();
private static final SdkField MAX_RESULTS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("MaxResults")
.getter(getter(DescribeInstanceStatusRequest::maxResults))
.setter(setter(Builder::maxResults))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxResults")
.unmarshallLocationName("MaxResults").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NextToken")
.getter(getter(DescribeInstanceStatusRequest::nextToken))
.setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextToken")
.unmarshallLocationName("NextToken").build()).build();
private static final SdkField DRY_RUN_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("DryRun")
.getter(getter(DescribeInstanceStatusRequest::dryRun))
.setter(setter(Builder::dryRun))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DryRun")
.unmarshallLocationName("dryRun").build()).build();
private static final SdkField> FILTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Filters")
.getter(getter(DescribeInstanceStatusRequest::filters))
.setter(setter(Builder::filters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Filter")
.unmarshallLocationName("Filter").build(),
ListTrait
.builder()
.memberLocationName("Filter")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Filter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Filter").unmarshallLocationName("Filter").build()).build())
.build()).build();
private static final SdkField INCLUDE_ALL_INSTANCES_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IncludeAllInstances")
.getter(getter(DescribeInstanceStatusRequest::includeAllInstances))
.setter(setter(Builder::includeAllInstances))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludeAllInstances")
.unmarshallLocationName("includeAllInstances").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(INSTANCE_IDS_FIELD,
MAX_RESULTS_FIELD, NEXT_TOKEN_FIELD, DRY_RUN_FIELD, FILTERS_FIELD, INCLUDE_ALL_INSTANCES_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("InstanceId", INSTANCE_IDS_FIELD);
put("MaxResults", MAX_RESULTS_FIELD);
put("NextToken", NEXT_TOKEN_FIELD);
put("DryRun", DRY_RUN_FIELD);
put("Filter", FILTERS_FIELD);
put("IncludeAllInstances", INCLUDE_ALL_INSTANCES_FIELD);
}
});
private final List instanceIds;
private final Integer maxResults;
private final String nextToken;
private final Boolean dryRun;
private final List filters;
private final Boolean includeAllInstances;
private DescribeInstanceStatusRequest(BuilderImpl builder) {
super(builder);
this.instanceIds = builder.instanceIds;
this.maxResults = builder.maxResults;
this.nextToken = builder.nextToken;
this.dryRun = builder.dryRun;
this.filters = builder.filters;
this.includeAllInstances = builder.includeAllInstances;
}
/**
* For responses, this returns true if the service returned a value for the InstanceIds property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasInstanceIds() {
return instanceIds != null && !(instanceIds instanceof SdkAutoConstructList);
}
/**
*
* The instance IDs.
*
*
* Default: Describes all your instances.
*
*
* Constraints: Maximum 100 explicitly specified instance IDs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInstanceIds} method.
*
*
* @return The instance IDs.
*
* Default: Describes all your instances.
*
*
* Constraints: Maximum 100 explicitly specified instance IDs.
*/
public final List instanceIds() {
return instanceIds;
}
/**
*
* The maximum number of items to return for this request. To get the next page of items, make another request with
* the token returned in the output. For more information, see Pagination.
*
*
* You cannot specify this parameter and the instance IDs parameter in the same request.
*
*
* @return The maximum number of items to return for this request. To get the next page of items, make another
* request with the token returned in the output. For more information, see Pagination.
*
* You cannot specify this parameter and the instance IDs parameter in the same request.
*/
public final Integer maxResults() {
return maxResults;
}
/**
*
* The token returned from a previous paginated request. Pagination continues from the end of the items returned by
* the previous request.
*
*
* @return The token returned from a previous paginated request. Pagination continues from the end of the items
* returned by the previous request.
*/
public final String nextToken() {
return nextToken;
}
/**
*
* Checks whether you have the required permissions for the operation, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*
*
* @return Checks whether you have the required permissions for the operation, without actually making the request,
* and provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*/
public final Boolean dryRun() {
return dryRun;
}
/**
* For responses, this returns true if the service returned a value for the Filters property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasFilters() {
return filters != null && !(filters instanceof SdkAutoConstructList);
}
/**
*
* The filters.
*
*
* -
*
* availability-zone
- The Availability Zone of the instance.
*
*
* -
*
* event.code
- The code for the scheduled event (instance-reboot
|
* system-reboot
| system-maintenance
| instance-retirement
|
* instance-stop
).
*
*
* -
*
* event.description
- A description of the event.
*
*
* -
*
* event.instance-event-id
- The ID of the event whose date and time you are modifying.
*
*
* -
*
* event.not-after
- The latest end time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before
- The earliest start time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before-deadline
- The deadline for starting the event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* instance-state-code
- The code for the instance state, as a 16-bit unsigned integer. The high byte
* is used for internal purposes and should be ignored. The low byte is set based on the state represented. The
* valid values are 0 (pending), 16 (running), 32 (shutting-down), 48 (terminated), 64 (stopping), and 80 (stopped).
*
*
* -
*
* instance-state-name
- The state of the instance (pending
| running
|
* shutting-down
| terminated
| stopping
| stopped
).
*
*
* -
*
* instance-status.reachability
- Filters on instance status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* instance-status.status
- The status of the instance (ok
| impaired
|
* initializing
| insufficient-data
| not-applicable
).
*
*
* -
*
* system-status.reachability
- Filters on system status where the name is reachability
(
* passed
| failed
| initializing
| insufficient-data
).
*
*
* -
*
* system-status.status
- The system status of the instance (ok
| impaired
|
* initializing
| insufficient-data
| not-applicable
).
*
*
* -
*
* attached-ebs-status.status
- The status of the attached EBS volume for the instance (ok
* | impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasFilters} method.
*
*
* @return The filters.
*
* -
*
* availability-zone
- The Availability Zone of the instance.
*
*
* -
*
* event.code
- The code for the scheduled event (instance-reboot
|
* system-reboot
| system-maintenance
| instance-retirement
|
* instance-stop
).
*
*
* -
*
* event.description
- A description of the event.
*
*
* -
*
* event.instance-event-id
- The ID of the event whose date and time you are modifying.
*
*
* -
*
* event.not-after
- The latest end time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before
- The earliest start time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before-deadline
- The deadline for starting the event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* instance-state-code
- The code for the instance state, as a 16-bit unsigned integer. The
* high byte is used for internal purposes and should be ignored. The low byte is set based on the state
* represented. The valid values are 0 (pending), 16 (running), 32 (shutting-down), 48 (terminated), 64
* (stopping), and 80 (stopped).
*
*
* -
*
* instance-state-name
- The state of the instance (pending
| running
* | shutting-down
| terminated
| stopping
| stopped
).
*
*
* -
*
* instance-status.reachability
- Filters on instance status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* instance-status.status
- The status of the instance (ok
| impaired
* | initializing
| insufficient-data
| not-applicable
).
*
*
* -
*
* system-status.reachability
- Filters on system status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* system-status.status
- The system status of the instance (ok
|
* impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
* -
*
* attached-ebs-status.status
- The status of the attached EBS volume for the instance (
* ok
| impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
*/
public final List filters() {
return filters;
}
/**
*
* When true
, includes the health status for all instances. When false
, includes the
* health status for running instances only.
*
*
* Default: false
*
*
* @return When true
, includes the health status for all instances. When false
, includes
* the health status for running instances only.
*
* Default: false
*/
public final Boolean includeAllInstances() {
return includeAllInstances;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(hasInstanceIds() ? instanceIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(maxResults());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
hashCode = 31 * hashCode + Objects.hashCode(dryRun());
hashCode = 31 * hashCode + Objects.hashCode(hasFilters() ? filters() : null);
hashCode = 31 * hashCode + Objects.hashCode(includeAllInstances());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeInstanceStatusRequest)) {
return false;
}
DescribeInstanceStatusRequest other = (DescribeInstanceStatusRequest) obj;
return hasInstanceIds() == other.hasInstanceIds() && Objects.equals(instanceIds(), other.instanceIds())
&& Objects.equals(maxResults(), other.maxResults()) && Objects.equals(nextToken(), other.nextToken())
&& Objects.equals(dryRun(), other.dryRun()) && hasFilters() == other.hasFilters()
&& Objects.equals(filters(), other.filters())
&& Objects.equals(includeAllInstances(), other.includeAllInstances());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeInstanceStatusRequest").add("InstanceIds", hasInstanceIds() ? instanceIds() : null)
.add("MaxResults", maxResults()).add("NextToken", nextToken()).add("DryRun", dryRun())
.add("Filters", hasFilters() ? filters() : null).add("IncludeAllInstances", includeAllInstances()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "InstanceIds":
return Optional.ofNullable(clazz.cast(instanceIds()));
case "MaxResults":
return Optional.ofNullable(clazz.cast(maxResults()));
case "NextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
case "DryRun":
return Optional.ofNullable(clazz.cast(dryRun()));
case "Filters":
return Optional.ofNullable(clazz.cast(filters()));
case "IncludeAllInstances":
return Optional.ofNullable(clazz.cast(includeAllInstances()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* Default: Describes all your instances.
*
*
* Constraints: Maximum 100 explicitly specified instance IDs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder instanceIds(Collection instanceIds);
/**
*
* The instance IDs.
*
*
* Default: Describes all your instances.
*
*
* Constraints: Maximum 100 explicitly specified instance IDs.
*
*
* @param instanceIds
* The instance IDs.
*
* Default: Describes all your instances.
*
*
* Constraints: Maximum 100 explicitly specified instance IDs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder instanceIds(String... instanceIds);
/**
*
* The maximum number of items to return for this request. To get the next page of items, make another request
* with the token returned in the output. For more information, see Pagination.
*
*
* You cannot specify this parameter and the instance IDs parameter in the same request.
*
*
* @param maxResults
* The maximum number of items to return for this request. To get the next page of items, make another
* request with the token returned in the output. For more information, see Pagination.
*
* You cannot specify this parameter and the instance IDs parameter in the same request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder maxResults(Integer maxResults);
/**
*
* The token returned from a previous paginated request. Pagination continues from the end of the items returned
* by the previous request.
*
*
* @param nextToken
* The token returned from a previous paginated request. Pagination continues from the end of the items
* returned by the previous request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nextToken(String nextToken);
/**
*
* Checks whether you have the required permissions for the operation, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*
*
* @param dryRun
* Checks whether you have the required permissions for the operation, without actually making the
* request, and provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder dryRun(Boolean dryRun);
/**
*
* The filters.
*
*
* -
*
* availability-zone
- The Availability Zone of the instance.
*
*
* -
*
* event.code
- The code for the scheduled event (instance-reboot
|
* system-reboot
| system-maintenance
| instance-retirement
|
* instance-stop
).
*
*
* -
*
* event.description
- A description of the event.
*
*
* -
*
* event.instance-event-id
- The ID of the event whose date and time you are modifying.
*
*
* -
*
* event.not-after
- The latest end time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before
- The earliest start time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before-deadline
- The deadline for starting the event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* instance-state-code
- The code for the instance state, as a 16-bit unsigned integer. The high
* byte is used for internal purposes and should be ignored. The low byte is set based on the state represented.
* The valid values are 0 (pending), 16 (running), 32 (shutting-down), 48 (terminated), 64 (stopping), and 80
* (stopped).
*
*
* -
*
* instance-state-name
- The state of the instance (pending
| running
|
* shutting-down
| terminated
| stopping
| stopped
).
*
*
* -
*
* instance-status.reachability
- Filters on instance status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* instance-status.status
- The status of the instance (ok
| impaired
|
* initializing
| insufficient-data
| not-applicable
).
*
*
* -
*
* system-status.reachability
- Filters on system status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* system-status.status
- The system status of the instance (ok
|
* impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
* -
*
* attached-ebs-status.status
- The status of the attached EBS volume for the instance (
* ok
| impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
*
*
* @param filters
* The filters.
*
* -
*
* availability-zone
- The Availability Zone of the instance.
*
*
* -
*
* event.code
- The code for the scheduled event (instance-reboot
|
* system-reboot
| system-maintenance
| instance-retirement
|
* instance-stop
).
*
*
* -
*
* event.description
- A description of the event.
*
*
* -
*
* event.instance-event-id
- The ID of the event whose date and time you are modifying.
*
*
* -
*
* event.not-after
- The latest end time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before
- The earliest start time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before-deadline
- The deadline for starting the event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* instance-state-code
- The code for the instance state, as a 16-bit unsigned integer. The
* high byte is used for internal purposes and should be ignored. The low byte is set based on the state
* represented. The valid values are 0 (pending), 16 (running), 32 (shutting-down), 48 (terminated), 64
* (stopping), and 80 (stopped).
*
*
* -
*
* instance-state-name
- The state of the instance (pending
|
* running
| shutting-down
| terminated
| stopping
|
* stopped
).
*
*
* -
*
* instance-status.reachability
- Filters on instance status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* instance-status.status
- The status of the instance (ok
|
* impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
* -
*
* system-status.reachability
- Filters on system status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* system-status.status
- The system status of the instance (ok
|
* impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
* -
*
* attached-ebs-status.status
- The status of the attached EBS volume for the instance (
* ok
| impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder filters(Collection filters);
/**
*
* The filters.
*
*
* -
*
* availability-zone
- The Availability Zone of the instance.
*
*
* -
*
* event.code
- The code for the scheduled event (instance-reboot
|
* system-reboot
| system-maintenance
| instance-retirement
|
* instance-stop
).
*
*
* -
*
* event.description
- A description of the event.
*
*
* -
*
* event.instance-event-id
- The ID of the event whose date and time you are modifying.
*
*
* -
*
* event.not-after
- The latest end time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before
- The earliest start time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before-deadline
- The deadline for starting the event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* instance-state-code
- The code for the instance state, as a 16-bit unsigned integer. The high
* byte is used for internal purposes and should be ignored. The low byte is set based on the state represented.
* The valid values are 0 (pending), 16 (running), 32 (shutting-down), 48 (terminated), 64 (stopping), and 80
* (stopped).
*
*
* -
*
* instance-state-name
- The state of the instance (pending
| running
|
* shutting-down
| terminated
| stopping
| stopped
).
*
*
* -
*
* instance-status.reachability
- Filters on instance status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* instance-status.status
- The status of the instance (ok
| impaired
|
* initializing
| insufficient-data
| not-applicable
).
*
*
* -
*
* system-status.reachability
- Filters on system status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* system-status.status
- The system status of the instance (ok
|
* impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
* -
*
* attached-ebs-status.status
- The status of the attached EBS volume for the instance (
* ok
| impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
*
*
* @param filters
* The filters.
*
* -
*
* availability-zone
- The Availability Zone of the instance.
*
*
* -
*
* event.code
- The code for the scheduled event (instance-reboot
|
* system-reboot
| system-maintenance
| instance-retirement
|
* instance-stop
).
*
*
* -
*
* event.description
- A description of the event.
*
*
* -
*
* event.instance-event-id
- The ID of the event whose date and time you are modifying.
*
*
* -
*
* event.not-after
- The latest end time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before
- The earliest start time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before-deadline
- The deadline for starting the event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* instance-state-code
- The code for the instance state, as a 16-bit unsigned integer. The
* high byte is used for internal purposes and should be ignored. The low byte is set based on the state
* represented. The valid values are 0 (pending), 16 (running), 32 (shutting-down), 48 (terminated), 64
* (stopping), and 80 (stopped).
*
*
* -
*
* instance-state-name
- The state of the instance (pending
|
* running
| shutting-down
| terminated
| stopping
|
* stopped
).
*
*
* -
*
* instance-status.reachability
- Filters on instance status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* instance-status.status
- The status of the instance (ok
|
* impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
* -
*
* system-status.reachability
- Filters on system status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* system-status.status
- The system status of the instance (ok
|
* impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
* -
*
* attached-ebs-status.status
- The status of the attached EBS volume for the instance (
* ok
| impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder filters(Filter... filters);
/**
*
* The filters.
*
*
* -
*
* availability-zone
- The Availability Zone of the instance.
*
*
* -
*
* event.code
- The code for the scheduled event (instance-reboot
|
* system-reboot
| system-maintenance
| instance-retirement
|
* instance-stop
).
*
*
* -
*
* event.description
- A description of the event.
*
*
* -
*
* event.instance-event-id
- The ID of the event whose date and time you are modifying.
*
*
* -
*
* event.not-after
- The latest end time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before
- The earliest start time for the scheduled event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* event.not-before-deadline
- The deadline for starting the event (for example,
* 2014-09-15T17:15:20.000Z
).
*
*
* -
*
* instance-state-code
- The code for the instance state, as a 16-bit unsigned integer. The high
* byte is used for internal purposes and should be ignored. The low byte is set based on the state represented.
* The valid values are 0 (pending), 16 (running), 32 (shutting-down), 48 (terminated), 64 (stopping), and 80
* (stopped).
*
*
* -
*
* instance-state-name
- The state of the instance (pending
| running
|
* shutting-down
| terminated
| stopping
| stopped
).
*
*
* -
*
* instance-status.reachability
- Filters on instance status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* instance-status.status
- The status of the instance (ok
| impaired
|
* initializing
| insufficient-data
| not-applicable
).
*
*
* -
*
* system-status.reachability
- Filters on system status where the name is
* reachability
(passed
| failed
| initializing
|
* insufficient-data
).
*
*
* -
*
* system-status.status
- The system status of the instance (ok
|
* impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
* -
*
* attached-ebs-status.status
- The status of the attached EBS volume for the instance (
* ok
| impaired
| initializing
| insufficient-data
|
* not-applicable
).
*
*
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ec2.model.Filter.Builder} avoiding the need to create one manually via
* {@link software.amazon.awssdk.services.ec2.model.Filter#builder()}.
*
*
* When the {@link Consumer} completes, {@link software.amazon.awssdk.services.ec2.model.Filter.Builder#build()}
* is called immediately and its result is passed to {@link #filters(List)}.
*
* @param filters
* a consumer that will call methods on {@link software.amazon.awssdk.services.ec2.model.Filter.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #filters(java.util.Collection)
*/
Builder filters(Consumer... filters);
/**
*
* When true
, includes the health status for all instances. When false
, includes the
* health status for running instances only.
*
*
* Default: false
*
*
* @param includeAllInstances
* When true
, includes the health status for all instances. When false
,
* includes the health status for running instances only.
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder includeAllInstances(Boolean includeAllInstances);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends Ec2Request.BuilderImpl implements Builder {
private List instanceIds = DefaultSdkAutoConstructList.getInstance();
private Integer maxResults;
private String nextToken;
private Boolean dryRun;
private List filters = DefaultSdkAutoConstructList.getInstance();
private Boolean includeAllInstances;
private BuilderImpl() {
}
private BuilderImpl(DescribeInstanceStatusRequest model) {
super(model);
instanceIds(model.instanceIds);
maxResults(model.maxResults);
nextToken(model.nextToken);
dryRun(model.dryRun);
filters(model.filters);
includeAllInstances(model.includeAllInstances);
}
public final Collection getInstanceIds() {
if (instanceIds instanceof SdkAutoConstructList) {
return null;
}
return instanceIds;
}
public final void setInstanceIds(Collection instanceIds) {
this.instanceIds = InstanceIdStringListCopier.copy(instanceIds);
}
@Override
public final Builder instanceIds(Collection instanceIds) {
this.instanceIds = InstanceIdStringListCopier.copy(instanceIds);
return this;
}
@Override
@SafeVarargs
public final Builder instanceIds(String... instanceIds) {
instanceIds(Arrays.asList(instanceIds));
return this;
}
public final Integer getMaxResults() {
return maxResults;
}
public final void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
@Override
public final Builder maxResults(Integer maxResults) {
this.maxResults = maxResults;
return this;
}
public final String getNextToken() {
return nextToken;
}
public final void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
@Override
public final Builder nextToken(String nextToken) {
this.nextToken = nextToken;
return this;
}
public final Boolean getDryRun() {
return dryRun;
}
public final void setDryRun(Boolean dryRun) {
this.dryRun = dryRun;
}
@Override
public final Builder dryRun(Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
public final List getFilters() {
List result = FilterListCopier.copyToBuilder(this.filters);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setFilters(Collection filters) {
this.filters = FilterListCopier.copyFromBuilder(filters);
}
@Override
public final Builder filters(Collection filters) {
this.filters = FilterListCopier.copy(filters);
return this;
}
@Override
@SafeVarargs
public final Builder filters(Filter... filters) {
filters(Arrays.asList(filters));
return this;
}
@Override
@SafeVarargs
public final Builder filters(Consumer... filters) {
filters(Stream.of(filters).map(c -> Filter.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final Boolean getIncludeAllInstances() {
return includeAllInstances;
}
public final void setIncludeAllInstances(Boolean includeAllInstances) {
this.includeAllInstances = includeAllInstances;
}
@Override
public final Builder includeAllInstances(Boolean includeAllInstances) {
this.includeAllInstances = includeAllInstances;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public DescribeInstanceStatusRequest build() {
return new DescribeInstanceStatusRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}