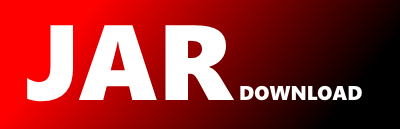
software.amazon.awssdk.services.ec2.model.DescribeLaunchTemplateVersionsRequest Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeLaunchTemplateVersionsRequest extends Ec2Request implements
ToCopyableBuilder {
private static final SdkField DRY_RUN_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("DryRun")
.getter(getter(DescribeLaunchTemplateVersionsRequest::dryRun))
.setter(setter(Builder::dryRun))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DryRun")
.unmarshallLocationName("DryRun").build()).build();
private static final SdkField LAUNCH_TEMPLATE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LaunchTemplateId")
.getter(getter(DescribeLaunchTemplateVersionsRequest::launchTemplateId))
.setter(setter(Builder::launchTemplateId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchTemplateId")
.unmarshallLocationName("LaunchTemplateId").build()).build();
private static final SdkField LAUNCH_TEMPLATE_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LaunchTemplateName")
.getter(getter(DescribeLaunchTemplateVersionsRequest::launchTemplateName))
.setter(setter(Builder::launchTemplateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchTemplateName")
.unmarshallLocationName("LaunchTemplateName").build()).build();
private static final SdkField> VERSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Versions")
.getter(getter(DescribeLaunchTemplateVersionsRequest::versions))
.setter(setter(Builder::versions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchTemplateVersion")
.unmarshallLocationName("LaunchTemplateVersion").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField MIN_VERSION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MinVersion")
.getter(getter(DescribeLaunchTemplateVersionsRequest::minVersion))
.setter(setter(Builder::minVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MinVersion")
.unmarshallLocationName("MinVersion").build()).build();
private static final SdkField MAX_VERSION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MaxVersion")
.getter(getter(DescribeLaunchTemplateVersionsRequest::maxVersion))
.setter(setter(Builder::maxVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxVersion")
.unmarshallLocationName("MaxVersion").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NextToken")
.getter(getter(DescribeLaunchTemplateVersionsRequest::nextToken))
.setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextToken")
.unmarshallLocationName("NextToken").build()).build();
private static final SdkField MAX_RESULTS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("MaxResults")
.getter(getter(DescribeLaunchTemplateVersionsRequest::maxResults))
.setter(setter(Builder::maxResults))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxResults")
.unmarshallLocationName("MaxResults").build()).build();
private static final SdkField> FILTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Filters")
.getter(getter(DescribeLaunchTemplateVersionsRequest::filters))
.setter(setter(Builder::filters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Filter")
.unmarshallLocationName("Filter").build(),
ListTrait
.builder()
.memberLocationName("Filter")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Filter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Filter").unmarshallLocationName("Filter").build()).build())
.build()).build();
private static final SdkField RESOLVE_ALIAS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ResolveAlias")
.getter(getter(DescribeLaunchTemplateVersionsRequest::resolveAlias))
.setter(setter(Builder::resolveAlias))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResolveAlias")
.unmarshallLocationName("ResolveAlias").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DRY_RUN_FIELD,
LAUNCH_TEMPLATE_ID_FIELD, LAUNCH_TEMPLATE_NAME_FIELD, VERSIONS_FIELD, MIN_VERSION_FIELD, MAX_VERSION_FIELD,
NEXT_TOKEN_FIELD, MAX_RESULTS_FIELD, FILTERS_FIELD, RESOLVE_ALIAS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("DryRun", DRY_RUN_FIELD);
put("LaunchTemplateId", LAUNCH_TEMPLATE_ID_FIELD);
put("LaunchTemplateName", LAUNCH_TEMPLATE_NAME_FIELD);
put("LaunchTemplateVersion", VERSIONS_FIELD);
put("MinVersion", MIN_VERSION_FIELD);
put("MaxVersion", MAX_VERSION_FIELD);
put("NextToken", NEXT_TOKEN_FIELD);
put("MaxResults", MAX_RESULTS_FIELD);
put("Filter", FILTERS_FIELD);
put("ResolveAlias", RESOLVE_ALIAS_FIELD);
}
});
private final Boolean dryRun;
private final String launchTemplateId;
private final String launchTemplateName;
private final List versions;
private final String minVersion;
private final String maxVersion;
private final String nextToken;
private final Integer maxResults;
private final List filters;
private final Boolean resolveAlias;
private DescribeLaunchTemplateVersionsRequest(BuilderImpl builder) {
super(builder);
this.dryRun = builder.dryRun;
this.launchTemplateId = builder.launchTemplateId;
this.launchTemplateName = builder.launchTemplateName;
this.versions = builder.versions;
this.minVersion = builder.minVersion;
this.maxVersion = builder.maxVersion;
this.nextToken = builder.nextToken;
this.maxResults = builder.maxResults;
this.filters = builder.filters;
this.resolveAlias = builder.resolveAlias;
}
/**
*
* Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*
*
* @return Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*/
public final Boolean dryRun() {
return dryRun;
}
/**
*
* The ID of the launch template.
*
*
* To describe one or more versions of a specified launch template, you must specify either the launch template ID
* or the launch template name, but not both.
*
*
* To describe all the latest or default launch template versions in your account, you must omit this parameter.
*
*
* @return The ID of the launch template.
*
* To describe one or more versions of a specified launch template, you must specify either the launch
* template ID or the launch template name, but not both.
*
*
* To describe all the latest or default launch template versions in your account, you must omit this
* parameter.
*/
public final String launchTemplateId() {
return launchTemplateId;
}
/**
*
* The name of the launch template.
*
*
* To describe one or more versions of a specified launch template, you must specify either the launch template name
* or the launch template ID, but not both.
*
*
* To describe all the latest or default launch template versions in your account, you must omit this parameter.
*
*
* @return The name of the launch template.
*
* To describe one or more versions of a specified launch template, you must specify either the launch
* template name or the launch template ID, but not both.
*
*
* To describe all the latest or default launch template versions in your account, you must omit this
* parameter.
*/
public final String launchTemplateName() {
return launchTemplateName;
}
/**
* For responses, this returns true if the service returned a value for the Versions property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasVersions() {
return versions != null && !(versions instanceof SdkAutoConstructList);
}
/**
*
* One or more versions of the launch template. Valid values depend on whether you are describing a specified launch
* template (by ID or name) or all launch templates in your account.
*
*
* To describe one or more versions of a specified launch template, valid values are $Latest
,
* $Default
, and numbers.
*
*
* To describe all launch templates in your account that are defined as the latest version, the valid value is
* $Latest
. To describe all launch templates in your account that are defined as the default version,
* the valid value is $Default
. You can specify $Latest
and $Default
in the
* same request. You cannot specify numbers.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVersions} method.
*
*
* @return One or more versions of the launch template. Valid values depend on whether you are describing a
* specified launch template (by ID or name) or all launch templates in your account.
*
* To describe one or more versions of a specified launch template, valid values are $Latest
,
* $Default
, and numbers.
*
*
* To describe all launch templates in your account that are defined as the latest version, the valid value
* is $Latest
. To describe all launch templates in your account that are defined as the default
* version, the valid value is $Default
. You can specify $Latest
and
* $Default
in the same request. You cannot specify numbers.
*/
public final List versions() {
return versions;
}
/**
*
* The version number after which to describe launch template versions.
*
*
* @return The version number after which to describe launch template versions.
*/
public final String minVersion() {
return minVersion;
}
/**
*
* The version number up to which to describe launch template versions.
*
*
* @return The version number up to which to describe launch template versions.
*/
public final String maxVersion() {
return maxVersion;
}
/**
*
* The token to request the next page of results.
*
*
* @return The token to request the next page of results.
*/
public final String nextToken() {
return nextToken;
}
/**
*
* The maximum number of results to return in a single call. To retrieve the remaining results, make another call
* with the returned NextToken
value. This value can be between 1 and 200.
*
*
* @return The maximum number of results to return in a single call. To retrieve the remaining results, make another
* call with the returned NextToken
value. This value can be between 1 and 200.
*/
public final Integer maxResults() {
return maxResults;
}
/**
* For responses, this returns true if the service returned a value for the Filters property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasFilters() {
return filters != null && !(filters instanceof SdkAutoConstructList);
}
/**
*
* One or more filters.
*
*
* -
*
* create-time
- The time the launch template version was created.
*
*
* -
*
* ebs-optimized
- A boolean that indicates whether the instance is optimized for Amazon EBS I/O.
*
*
* -
*
* http-endpoint
- Indicates whether the HTTP metadata endpoint on your instances is enabled (
* enabled
| disabled
).
*
*
* -
*
* http-protocol-ipv4
- Indicates whether the IPv4 endpoint for the instance metadata service is
* enabled (enabled
| disabled
).
*
*
* -
*
* host-resource-group-arn
- The ARN of the host resource group in which to launch the instances.
*
*
* -
*
* http-tokens
- The state of token usage for your instance metadata requests (optional
|
* required
).
*
*
* -
*
* iam-instance-profile
- The ARN of the IAM instance profile.
*
*
* -
*
* image-id
- The ID of the AMI.
*
*
* -
*
* instance-type
- The instance type.
*
*
* -
*
* is-default-version
- A boolean that indicates whether the launch template version is the default
* version.
*
*
* -
*
* kernel-id
- The kernel ID.
*
*
* -
*
* license-configuration-arn
- The ARN of the license configuration.
*
*
* -
*
* network-card-index
- The index of the network card.
*
*
* -
*
* ram-disk-id
- The RAM disk ID.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasFilters} method.
*
*
* @return One or more filters.
*
* -
*
* create-time
- The time the launch template version was created.
*
*
* -
*
* ebs-optimized
- A boolean that indicates whether the instance is optimized for Amazon EBS
* I/O.
*
*
* -
*
* http-endpoint
- Indicates whether the HTTP metadata endpoint on your instances is enabled (
* enabled
| disabled
).
*
*
* -
*
* http-protocol-ipv4
- Indicates whether the IPv4 endpoint for the instance metadata service
* is enabled (enabled
| disabled
).
*
*
* -
*
* host-resource-group-arn
- The ARN of the host resource group in which to launch the
* instances.
*
*
* -
*
* http-tokens
- The state of token usage for your instance metadata requests (
* optional
| required
).
*
*
* -
*
* iam-instance-profile
- The ARN of the IAM instance profile.
*
*
* -
*
* image-id
- The ID of the AMI.
*
*
* -
*
* instance-type
- The instance type.
*
*
* -
*
* is-default-version
- A boolean that indicates whether the launch template version is the
* default version.
*
*
* -
*
* kernel-id
- The kernel ID.
*
*
* -
*
* license-configuration-arn
- The ARN of the license configuration.
*
*
* -
*
* network-card-index
- The index of the network card.
*
*
* -
*
* ram-disk-id
- The RAM disk ID.
*
*
*/
public final List filters() {
return filters;
}
/**
*
* If true
, and if a Systems Manager parameter is specified for ImageId
, the AMI ID is
* displayed in the response for imageId
.
*
*
* If false
, and if a Systems Manager parameter is specified for ImageId
, the parameter is
* displayed in the response for imageId
.
*
*
* For more information, see Use a Systems Manager parameter instead of an AMI ID in the Amazon EC2 User Guide.
*
*
* Default: false
*
*
* @return If true
, and if a Systems Manager parameter is specified for ImageId
, the AMI
* ID is displayed in the response for imageId
.
*
* If false
, and if a Systems Manager parameter is specified for ImageId
, the
* parameter is displayed in the response for imageId
.
*
*
* For more information, see Use a Systems Manager parameter instead of an AMI ID in the Amazon EC2 User Guide.
*
*
* Default: false
*/
public final Boolean resolveAlias() {
return resolveAlias;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dryRun());
hashCode = 31 * hashCode + Objects.hashCode(launchTemplateId());
hashCode = 31 * hashCode + Objects.hashCode(launchTemplateName());
hashCode = 31 * hashCode + Objects.hashCode(hasVersions() ? versions() : null);
hashCode = 31 * hashCode + Objects.hashCode(minVersion());
hashCode = 31 * hashCode + Objects.hashCode(maxVersion());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
hashCode = 31 * hashCode + Objects.hashCode(maxResults());
hashCode = 31 * hashCode + Objects.hashCode(hasFilters() ? filters() : null);
hashCode = 31 * hashCode + Objects.hashCode(resolveAlias());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeLaunchTemplateVersionsRequest)) {
return false;
}
DescribeLaunchTemplateVersionsRequest other = (DescribeLaunchTemplateVersionsRequest) obj;
return Objects.equals(dryRun(), other.dryRun()) && Objects.equals(launchTemplateId(), other.launchTemplateId())
&& Objects.equals(launchTemplateName(), other.launchTemplateName()) && hasVersions() == other.hasVersions()
&& Objects.equals(versions(), other.versions()) && Objects.equals(minVersion(), other.minVersion())
&& Objects.equals(maxVersion(), other.maxVersion()) && Objects.equals(nextToken(), other.nextToken())
&& Objects.equals(maxResults(), other.maxResults()) && hasFilters() == other.hasFilters()
&& Objects.equals(filters(), other.filters()) && Objects.equals(resolveAlias(), other.resolveAlias());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeLaunchTemplateVersionsRequest").add("DryRun", dryRun())
.add("LaunchTemplateId", launchTemplateId()).add("LaunchTemplateName", launchTemplateName())
.add("Versions", hasVersions() ? versions() : null).add("MinVersion", minVersion())
.add("MaxVersion", maxVersion()).add("NextToken", nextToken()).add("MaxResults", maxResults())
.add("Filters", hasFilters() ? filters() : null).add("ResolveAlias", resolveAlias()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DryRun":
return Optional.ofNullable(clazz.cast(dryRun()));
case "LaunchTemplateId":
return Optional.ofNullable(clazz.cast(launchTemplateId()));
case "LaunchTemplateName":
return Optional.ofNullable(clazz.cast(launchTemplateName()));
case "Versions":
return Optional.ofNullable(clazz.cast(versions()));
case "MinVersion":
return Optional.ofNullable(clazz.cast(minVersion()));
case "MaxVersion":
return Optional.ofNullable(clazz.cast(maxVersion()));
case "NextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
case "MaxResults":
return Optional.ofNullable(clazz.cast(maxResults()));
case "Filters":
return Optional.ofNullable(clazz.cast(filters()));
case "ResolveAlias":
return Optional.ofNullable(clazz.cast(resolveAlias()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* To describe one or more versions of a specified launch template, you must specify either the launch
* template ID or the launch template name, but not both.
*
*
* To describe all the latest or default launch template versions in your account, you must omit this
* parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder launchTemplateId(String launchTemplateId);
/**
*
* The name of the launch template.
*
*
* To describe one or more versions of a specified launch template, you must specify either the launch template
* name or the launch template ID, but not both.
*
*
* To describe all the latest or default launch template versions in your account, you must omit this parameter.
*
*
* @param launchTemplateName
* The name of the launch template.
*
* To describe one or more versions of a specified launch template, you must specify either the launch
* template name or the launch template ID, but not both.
*
*
* To describe all the latest or default launch template versions in your account, you must omit this
* parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder launchTemplateName(String launchTemplateName);
/**
*
* One or more versions of the launch template. Valid values depend on whether you are describing a specified
* launch template (by ID or name) or all launch templates in your account.
*
*
* To describe one or more versions of a specified launch template, valid values are $Latest
,
* $Default
, and numbers.
*
*
* To describe all launch templates in your account that are defined as the latest version, the valid value is
* $Latest
. To describe all launch templates in your account that are defined as the default
* version, the valid value is $Default
. You can specify $Latest
and
* $Default
in the same request. You cannot specify numbers.
*
*
* @param versions
* One or more versions of the launch template. Valid values depend on whether you are describing a
* specified launch template (by ID or name) or all launch templates in your account.
*
* To describe one or more versions of a specified launch template, valid values are $Latest
, $Default
, and numbers.
*
*
* To describe all launch templates in your account that are defined as the latest version, the valid
* value is $Latest
. To describe all launch templates in your account that are defined as
* the default version, the valid value is $Default
. You can specify $Latest
* and $Default
in the same request. You cannot specify numbers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder versions(Collection versions);
/**
*
* One or more versions of the launch template. Valid values depend on whether you are describing a specified
* launch template (by ID or name) or all launch templates in your account.
*
*
* To describe one or more versions of a specified launch template, valid values are $Latest
,
* $Default
, and numbers.
*
*
* To describe all launch templates in your account that are defined as the latest version, the valid value is
* $Latest
. To describe all launch templates in your account that are defined as the default
* version, the valid value is $Default
. You can specify $Latest
and
* $Default
in the same request. You cannot specify numbers.
*
*
* @param versions
* One or more versions of the launch template. Valid values depend on whether you are describing a
* specified launch template (by ID or name) or all launch templates in your account.
*
* To describe one or more versions of a specified launch template, valid values are $Latest
, $Default
, and numbers.
*
*
* To describe all launch templates in your account that are defined as the latest version, the valid
* value is $Latest
. To describe all launch templates in your account that are defined as
* the default version, the valid value is $Default
. You can specify $Latest
* and $Default
in the same request. You cannot specify numbers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder versions(String... versions);
/**
*
* The version number after which to describe launch template versions.
*
*
* @param minVersion
* The version number after which to describe launch template versions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder minVersion(String minVersion);
/**
*
* The version number up to which to describe launch template versions.
*
*
* @param maxVersion
* The version number up to which to describe launch template versions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder maxVersion(String maxVersion);
/**
*
* The token to request the next page of results.
*
*
* @param nextToken
* The token to request the next page of results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nextToken(String nextToken);
/**
*
* The maximum number of results to return in a single call. To retrieve the remaining results, make another
* call with the returned NextToken
value. This value can be between 1 and 200.
*
*
* @param maxResults
* The maximum number of results to return in a single call. To retrieve the remaining results, make
* another call with the returned NextToken
value. This value can be between 1 and 200.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder maxResults(Integer maxResults);
/**
*
* One or more filters.
*
*
* -
*
* create-time
- The time the launch template version was created.
*
*
* -
*
* ebs-optimized
- A boolean that indicates whether the instance is optimized for Amazon EBS I/O.
*
*
* -
*
* http-endpoint
- Indicates whether the HTTP metadata endpoint on your instances is enabled (
* enabled
| disabled
).
*
*
* -
*
* http-protocol-ipv4
- Indicates whether the IPv4 endpoint for the instance metadata service is
* enabled (enabled
| disabled
).
*
*
* -
*
* host-resource-group-arn
- The ARN of the host resource group in which to launch the instances.
*
*
* -
*
* http-tokens
- The state of token usage for your instance metadata requests (
* optional
| required
).
*
*
* -
*
* iam-instance-profile
- The ARN of the IAM instance profile.
*
*
* -
*
* image-id
- The ID of the AMI.
*
*
* -
*
* instance-type
- The instance type.
*
*
* -
*
* is-default-version
- A boolean that indicates whether the launch template version is the default
* version.
*
*
* -
*
* kernel-id
- The kernel ID.
*
*
* -
*
* license-configuration-arn
- The ARN of the license configuration.
*
*
* -
*
* network-card-index
- The index of the network card.
*
*
* -
*
* ram-disk-id
- The RAM disk ID.
*
*
*
*
* @param filters
* One or more filters.
*
* -
*
* create-time
- The time the launch template version was created.
*
*
* -
*
* ebs-optimized
- A boolean that indicates whether the instance is optimized for Amazon EBS
* I/O.
*
*
* -
*
* http-endpoint
- Indicates whether the HTTP metadata endpoint on your instances is enabled
* (enabled
| disabled
).
*
*
* -
*
* http-protocol-ipv4
- Indicates whether the IPv4 endpoint for the instance metadata
* service is enabled (enabled
| disabled
).
*
*
* -
*
* host-resource-group-arn
- The ARN of the host resource group in which to launch the
* instances.
*
*
* -
*
* http-tokens
- The state of token usage for your instance metadata requests (
* optional
| required
).
*
*
* -
*
* iam-instance-profile
- The ARN of the IAM instance profile.
*
*
* -
*
* image-id
- The ID of the AMI.
*
*
* -
*
* instance-type
- The instance type.
*
*
* -
*
* is-default-version
- A boolean that indicates whether the launch template version is the
* default version.
*
*
* -
*
* kernel-id
- The kernel ID.
*
*
* -
*
* license-configuration-arn
- The ARN of the license configuration.
*
*
* -
*
* network-card-index
- The index of the network card.
*
*
* -
*
* ram-disk-id
- The RAM disk ID.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder filters(Collection filters);
/**
*
* One or more filters.
*
*
* -
*
* create-time
- The time the launch template version was created.
*
*
* -
*
* ebs-optimized
- A boolean that indicates whether the instance is optimized for Amazon EBS I/O.
*
*
* -
*
* http-endpoint
- Indicates whether the HTTP metadata endpoint on your instances is enabled (
* enabled
| disabled
).
*
*
* -
*
* http-protocol-ipv4
- Indicates whether the IPv4 endpoint for the instance metadata service is
* enabled (enabled
| disabled
).
*
*
* -
*
* host-resource-group-arn
- The ARN of the host resource group in which to launch the instances.
*
*
* -
*
* http-tokens
- The state of token usage for your instance metadata requests (
* optional
| required
).
*
*
* -
*
* iam-instance-profile
- The ARN of the IAM instance profile.
*
*
* -
*
* image-id
- The ID of the AMI.
*
*
* -
*
* instance-type
- The instance type.
*
*
* -
*
* is-default-version
- A boolean that indicates whether the launch template version is the default
* version.
*
*
* -
*
* kernel-id
- The kernel ID.
*
*
* -
*
* license-configuration-arn
- The ARN of the license configuration.
*
*
* -
*
* network-card-index
- The index of the network card.
*
*
* -
*
* ram-disk-id
- The RAM disk ID.
*
*
*
*
* @param filters
* One or more filters.
*
* -
*
* create-time
- The time the launch template version was created.
*
*
* -
*
* ebs-optimized
- A boolean that indicates whether the instance is optimized for Amazon EBS
* I/O.
*
*
* -
*
* http-endpoint
- Indicates whether the HTTP metadata endpoint on your instances is enabled
* (enabled
| disabled
).
*
*
* -
*
* http-protocol-ipv4
- Indicates whether the IPv4 endpoint for the instance metadata
* service is enabled (enabled
| disabled
).
*
*
* -
*
* host-resource-group-arn
- The ARN of the host resource group in which to launch the
* instances.
*
*
* -
*
* http-tokens
- The state of token usage for your instance metadata requests (
* optional
| required
).
*
*
* -
*
* iam-instance-profile
- The ARN of the IAM instance profile.
*
*
* -
*
* image-id
- The ID of the AMI.
*
*
* -
*
* instance-type
- The instance type.
*
*
* -
*
* is-default-version
- A boolean that indicates whether the launch template version is the
* default version.
*
*
* -
*
* kernel-id
- The kernel ID.
*
*
* -
*
* license-configuration-arn
- The ARN of the license configuration.
*
*
* -
*
* network-card-index
- The index of the network card.
*
*
* -
*
* ram-disk-id
- The RAM disk ID.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder filters(Filter... filters);
/**
*
* One or more filters.
*
*
* -
*
* create-time
- The time the launch template version was created.
*
*
* -
*
* ebs-optimized
- A boolean that indicates whether the instance is optimized for Amazon EBS I/O.
*
*
* -
*
* http-endpoint
- Indicates whether the HTTP metadata endpoint on your instances is enabled (
* enabled
| disabled
).
*
*
* -
*
* http-protocol-ipv4
- Indicates whether the IPv4 endpoint for the instance metadata service is
* enabled (enabled
| disabled
).
*
*
* -
*
* host-resource-group-arn
- The ARN of the host resource group in which to launch the instances.
*
*
* -
*
* http-tokens
- The state of token usage for your instance metadata requests (
* optional
| required
).
*
*
* -
*
* iam-instance-profile
- The ARN of the IAM instance profile.
*
*
* -
*
* image-id
- The ID of the AMI.
*
*
* -
*
* instance-type
- The instance type.
*
*
* -
*
* is-default-version
- A boolean that indicates whether the launch template version is the default
* version.
*
*
* -
*
* kernel-id
- The kernel ID.
*
*
* -
*
* license-configuration-arn
- The ARN of the license configuration.
*
*
* -
*
* network-card-index
- The index of the network card.
*
*
* -
*
* ram-disk-id
- The RAM disk ID.
*
*
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ec2.model.Filter.Builder} avoiding the need to create one manually via
* {@link software.amazon.awssdk.services.ec2.model.Filter#builder()}.
*
*
* When the {@link Consumer} completes, {@link software.amazon.awssdk.services.ec2.model.Filter.Builder#build()}
* is called immediately and its result is passed to {@link #filters(List)}.
*
* @param filters
* a consumer that will call methods on {@link software.amazon.awssdk.services.ec2.model.Filter.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #filters(java.util.Collection)
*/
Builder filters(Consumer... filters);
/**
*
* If true
, and if a Systems Manager parameter is specified for ImageId
, the AMI ID is
* displayed in the response for imageId
.
*
*
* If false
, and if a Systems Manager parameter is specified for ImageId
, the
* parameter is displayed in the response for imageId
.
*
*
* For more information, see Use a Systems Manager parameter instead of an AMI ID in the Amazon EC2 User Guide.
*
*
* Default: false
*
*
* @param resolveAlias
* If true
, and if a Systems Manager parameter is specified for ImageId
, the
* AMI ID is displayed in the response for imageId
.
*
* If false
, and if a Systems Manager parameter is specified for ImageId
, the
* parameter is displayed in the response for imageId
.
*
*
* For more information, see Use a Systems Manager parameter instead of an AMI ID in the Amazon EC2 User Guide.
*
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder resolveAlias(Boolean resolveAlias);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends Ec2Request.BuilderImpl implements Builder {
private Boolean dryRun;
private String launchTemplateId;
private String launchTemplateName;
private List versions = DefaultSdkAutoConstructList.getInstance();
private String minVersion;
private String maxVersion;
private String nextToken;
private Integer maxResults;
private List filters = DefaultSdkAutoConstructList.getInstance();
private Boolean resolveAlias;
private BuilderImpl() {
}
private BuilderImpl(DescribeLaunchTemplateVersionsRequest model) {
super(model);
dryRun(model.dryRun);
launchTemplateId(model.launchTemplateId);
launchTemplateName(model.launchTemplateName);
versions(model.versions);
minVersion(model.minVersion);
maxVersion(model.maxVersion);
nextToken(model.nextToken);
maxResults(model.maxResults);
filters(model.filters);
resolveAlias(model.resolveAlias);
}
public final Boolean getDryRun() {
return dryRun;
}
public final void setDryRun(Boolean dryRun) {
this.dryRun = dryRun;
}
@Override
public final Builder dryRun(Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
public final String getLaunchTemplateId() {
return launchTemplateId;
}
public final void setLaunchTemplateId(String launchTemplateId) {
this.launchTemplateId = launchTemplateId;
}
@Override
public final Builder launchTemplateId(String launchTemplateId) {
this.launchTemplateId = launchTemplateId;
return this;
}
public final String getLaunchTemplateName() {
return launchTemplateName;
}
public final void setLaunchTemplateName(String launchTemplateName) {
this.launchTemplateName = launchTemplateName;
}
@Override
public final Builder launchTemplateName(String launchTemplateName) {
this.launchTemplateName = launchTemplateName;
return this;
}
public final Collection getVersions() {
if (versions instanceof SdkAutoConstructList) {
return null;
}
return versions;
}
public final void setVersions(Collection versions) {
this.versions = VersionStringListCopier.copy(versions);
}
@Override
public final Builder versions(Collection versions) {
this.versions = VersionStringListCopier.copy(versions);
return this;
}
@Override
@SafeVarargs
public final Builder versions(String... versions) {
versions(Arrays.asList(versions));
return this;
}
public final String getMinVersion() {
return minVersion;
}
public final void setMinVersion(String minVersion) {
this.minVersion = minVersion;
}
@Override
public final Builder minVersion(String minVersion) {
this.minVersion = minVersion;
return this;
}
public final String getMaxVersion() {
return maxVersion;
}
public final void setMaxVersion(String maxVersion) {
this.maxVersion = maxVersion;
}
@Override
public final Builder maxVersion(String maxVersion) {
this.maxVersion = maxVersion;
return this;
}
public final String getNextToken() {
return nextToken;
}
public final void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
@Override
public final Builder nextToken(String nextToken) {
this.nextToken = nextToken;
return this;
}
public final Integer getMaxResults() {
return maxResults;
}
public final void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
@Override
public final Builder maxResults(Integer maxResults) {
this.maxResults = maxResults;
return this;
}
public final List getFilters() {
List result = FilterListCopier.copyToBuilder(this.filters);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setFilters(Collection filters) {
this.filters = FilterListCopier.copyFromBuilder(filters);
}
@Override
public final Builder filters(Collection filters) {
this.filters = FilterListCopier.copy(filters);
return this;
}
@Override
@SafeVarargs
public final Builder filters(Filter... filters) {
filters(Arrays.asList(filters));
return this;
}
@Override
@SafeVarargs
public final Builder filters(Consumer... filters) {
filters(Stream.of(filters).map(c -> Filter.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final Boolean getResolveAlias() {
return resolveAlias;
}
public final void setResolveAlias(Boolean resolveAlias) {
this.resolveAlias = resolveAlias;
}
@Override
public final Builder resolveAlias(Boolean resolveAlias) {
this.resolveAlias = resolveAlias;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public DescribeLaunchTemplateVersionsRequest build() {
return new DescribeLaunchTemplateVersionsRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}