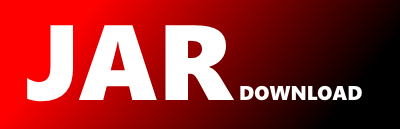
software.amazon.awssdk.services.ec2.model.EbsBlockDevice Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a block device for an EBS volume.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EbsBlockDevice implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField DELETE_ON_TERMINATION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("DeleteOnTermination")
.getter(getter(EbsBlockDevice::deleteOnTermination))
.setter(setter(Builder::deleteOnTermination))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeleteOnTermination")
.unmarshallLocationName("deleteOnTermination").build()).build();
private static final SdkField IOPS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("Iops")
.getter(getter(EbsBlockDevice::iops))
.setter(setter(Builder::iops))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Iops")
.unmarshallLocationName("iops").build()).build();
private static final SdkField SNAPSHOT_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SnapshotId")
.getter(getter(EbsBlockDevice::snapshotId))
.setter(setter(Builder::snapshotId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotId")
.unmarshallLocationName("snapshotId").build()).build();
private static final SdkField VOLUME_SIZE_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("VolumeSize")
.getter(getter(EbsBlockDevice::volumeSize))
.setter(setter(Builder::volumeSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VolumeSize")
.unmarshallLocationName("volumeSize").build()).build();
private static final SdkField VOLUME_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VolumeType")
.getter(getter(EbsBlockDevice::volumeTypeAsString))
.setter(setter(Builder::volumeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VolumeType")
.unmarshallLocationName("volumeType").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("KmsKeyId")
.getter(getter(EbsBlockDevice::kmsKeyId))
.setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId")
.unmarshallLocationName("kmsKeyId").build()).build();
private static final SdkField THROUGHPUT_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("Throughput")
.getter(getter(EbsBlockDevice::throughput))
.setter(setter(Builder::throughput))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Throughput")
.unmarshallLocationName("throughput").build()).build();
private static final SdkField OUTPOST_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OutpostArn")
.getter(getter(EbsBlockDevice::outpostArn))
.setter(setter(Builder::outpostArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutpostArn")
.unmarshallLocationName("outpostArn").build()).build();
private static final SdkField ENCRYPTED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("Encrypted")
.getter(getter(EbsBlockDevice::encrypted))
.setter(setter(Builder::encrypted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Encrypted")
.unmarshallLocationName("encrypted").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DELETE_ON_TERMINATION_FIELD,
IOPS_FIELD, SNAPSHOT_ID_FIELD, VOLUME_SIZE_FIELD, VOLUME_TYPE_FIELD, KMS_KEY_ID_FIELD, THROUGHPUT_FIELD,
OUTPOST_ARN_FIELD, ENCRYPTED_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("DeleteOnTermination", DELETE_ON_TERMINATION_FIELD);
put("Iops", IOPS_FIELD);
put("SnapshotId", SNAPSHOT_ID_FIELD);
put("VolumeSize", VOLUME_SIZE_FIELD);
put("VolumeType", VOLUME_TYPE_FIELD);
put("KmsKeyId", KMS_KEY_ID_FIELD);
put("Throughput", THROUGHPUT_FIELD);
put("OutpostArn", OUTPOST_ARN_FIELD);
put("Encrypted", ENCRYPTED_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final Boolean deleteOnTermination;
private final Integer iops;
private final String snapshotId;
private final Integer volumeSize;
private final String volumeType;
private final String kmsKeyId;
private final Integer throughput;
private final String outpostArn;
private final Boolean encrypted;
private EbsBlockDevice(BuilderImpl builder) {
this.deleteOnTermination = builder.deleteOnTermination;
this.iops = builder.iops;
this.snapshotId = builder.snapshotId;
this.volumeSize = builder.volumeSize;
this.volumeType = builder.volumeType;
this.kmsKeyId = builder.kmsKeyId;
this.throughput = builder.throughput;
this.outpostArn = builder.outpostArn;
this.encrypted = builder.encrypted;
}
/**
*
* Indicates whether the EBS volume is deleted on instance termination. For more information, see Preserving Amazon EBS volumes on instance termination in the Amazon EC2 User Guide.
*
*
* @return Indicates whether the EBS volume is deleted on instance termination. For more information, see Preserving Amazon EBS volumes on instance termination in the Amazon EC2 User Guide.
*/
public final Boolean deleteOnTermination() {
return deleteOnTermination;
}
/**
*
* The number of I/O operations per second (IOPS). For gp3
, io1
, and io2
* volumes, this represents the number of IOPS that are provisioned for the volume. For gp2
volumes,
* this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits
* for bursting.
*
*
* The following are the supported values for each volume type:
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* For io2
volumes, you can achieve up to 256,000 IOPS on instances
* built on the Nitro System. On other instances, you can achieve performance up to 32,000 IOPS.
*
*
* This parameter is required for io1
and io2
volumes. The default for gp3
* volumes is 3,000 IOPS.
*
*
* @return The number of I/O operations per second (IOPS). For gp3
, io1
, and
* io2
volumes, this represents the number of IOPS that are provisioned for the volume. For
* gp2
volumes, this represents the baseline performance of the volume and the rate at which
* the volume accumulates I/O credits for bursting.
*
* The following are the supported values for each volume type:
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* For io2
volumes, you can achieve up to 256,000 IOPS on instances built on the Nitro System. On other instances, you can achieve performance up to 32,000
* IOPS.
*
*
* This parameter is required for io1
and io2
volumes. The default for
* gp3
volumes is 3,000 IOPS.
*/
public final Integer iops() {
return iops;
}
/**
*
* The ID of the snapshot.
*
*
* @return The ID of the snapshot.
*/
public final String snapshotId() {
return snapshotId;
}
/**
*
* The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. If you specify a
* snapshot, the default is the snapshot size. You can specify a volume size that is equal to or larger than the
* snapshot size.
*
*
* The following are the supported sizes for each volume type:
*
*
* -
*
* gp2
and gp3
: 1 - 16,384 GiB
*
*
* -
*
* io1
: 4 - 16,384 GiB
*
*
* -
*
* io2
: 4 - 65,536 GiB
*
*
* -
*
* st1
and sc1
: 125 - 16,384 GiB
*
*
* -
*
* standard
: 1 - 1024 GiB
*
*
*
*
* @return The size of the volume, in GiBs. You must specify either a snapshot ID or a volume size. If you specify a
* snapshot, the default is the snapshot size. You can specify a volume size that is equal to or larger than
* the snapshot size.
*
* The following are the supported sizes for each volume type:
*
*
* -
*
* gp2
and gp3
: 1 - 16,384 GiB
*
*
* -
*
* io1
: 4 - 16,384 GiB
*
*
* -
*
* io2
: 4 - 65,536 GiB
*
*
* -
*
* st1
and sc1
: 125 - 16,384 GiB
*
*
* -
*
* standard
: 1 - 1024 GiB
*
*
*/
public final Integer volumeSize() {
return volumeSize;
}
/**
*
* The volume type. For more information, see Amazon EBS volume types in the
* Amazon EBS User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #volumeType} will
* return {@link VolumeType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #volumeTypeAsString}.
*
*
* @return The volume type. For more information, see Amazon EBS volume types
* in the Amazon EBS User Guide.
* @see VolumeType
*/
public final VolumeType volumeType() {
return VolumeType.fromValue(volumeType);
}
/**
*
* The volume type. For more information, see Amazon EBS volume types in the
* Amazon EBS User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #volumeType} will
* return {@link VolumeType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #volumeTypeAsString}.
*
*
* @return The volume type. For more information, see Amazon EBS volume types
* in the Amazon EBS User Guide.
* @see VolumeType
*/
public final String volumeTypeAsString() {
return volumeType;
}
/**
*
* Identifier (key ID, key alias, key ARN, or alias ARN) of the customer managed KMS key to use for EBS encryption.
*
*
* This parameter is only supported on BlockDeviceMapping
objects called by RunInstances, RequestSpotFleet, and
*
* RequestSpotInstances.
*
*
* @return Identifier (key ID, key alias, key ARN, or alias ARN) of the customer managed KMS key to use for EBS
* encryption.
*
* This parameter is only supported on BlockDeviceMapping
objects called by RunInstances, RequestSpotFleet,
* and
* RequestSpotInstances.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The throughput that the volume supports, in MiB/s.
*
*
* This parameter is valid only for gp3
volumes.
*
*
* Valid Range: Minimum value of 125. Maximum value of 1000.
*
*
* @return The throughput that the volume supports, in MiB/s.
*
* This parameter is valid only for gp3
volumes.
*
*
* Valid Range: Minimum value of 125. Maximum value of 1000.
*/
public final Integer throughput() {
return throughput;
}
/**
*
* The ARN of the Outpost on which the snapshot is stored.
*
*
* This parameter is not supported when using CreateImage.
*
*
* @return The ARN of the Outpost on which the snapshot is stored.
*
* This parameter is not supported when using CreateImage.
*/
public final String outpostArn() {
return outpostArn;
}
/**
*
* Indicates whether the encryption state of an EBS volume is changed while being restored from a backing snapshot.
* The effect of setting the encryption state to true
depends on the volume origin (new or from a
* snapshot), starting encryption state, ownership, and whether encryption by default is enabled. For more
* information, see Amazon EBS
* encryption in the Amazon EBS User Guide.
*
*
* In no case can you remove encryption from an encrypted volume.
*
*
* Encrypted volumes can only be attached to instances that support Amazon EBS encryption. For more information, see
* Supported instance types.
*
*
* This parameter is not returned by DescribeImageAttribute.
*
*
* For CreateImage and RegisterImage, whether you can include this parameter, and the allowed values
* differ depending on the type of block device mapping you are creating.
*
*
* -
*
* If you are creating a block device mapping for a new (empty) volume, you can include this parameter, and
* specify either true
for an encrypted volume, or false
for an unencrypted volume. If you
* omit this parameter, it defaults to false
(unencrypted).
*
*
* -
*
* If you are creating a block device mapping from an existing encrypted or unencrypted snapshot, you must
* omit this parameter. If you include this parameter, the request will fail, regardless of the value that you
* specify.
*
*
* -
*
* If you are creating a block device mapping from an existing unencrypted volume, you can include this
* parameter, but you must specify false
. If you specify true
, the request will fail. In
* this case, we recommend that you omit the parameter.
*
*
* -
*
* If you are creating a block device mapping from an existing encrypted volume, you can include this
* parameter, and specify either true
or false
. However, if you specify false
* , the parameter is ignored and the block device mapping is always encrypted. In this case, we recommend that you
* omit the parameter.
*
*
*
*
* @return Indicates whether the encryption state of an EBS volume is changed while being restored from a backing
* snapshot. The effect of setting the encryption state to true
depends on the volume origin
* (new or from a snapshot), starting encryption state, ownership, and whether encryption by default is
* enabled. For more information, see Amazon
* EBS encryption in the Amazon EBS User Guide.
*
* In no case can you remove encryption from an encrypted volume.
*
*
* Encrypted volumes can only be attached to instances that support Amazon EBS encryption. For more
* information, see Supported instance types.
*
*
* This parameter is not returned by DescribeImageAttribute.
*
*
* For CreateImage and RegisterImage, whether you can include this parameter, and the allowed
* values differ depending on the type of block device mapping you are creating.
*
*
* -
*
* If you are creating a block device mapping for a new (empty) volume, you can include this
* parameter, and specify either true
for an encrypted volume, or false
for an
* unencrypted volume. If you omit this parameter, it defaults to false
(unencrypted).
*
*
* -
*
* If you are creating a block device mapping from an existing encrypted or unencrypted snapshot, you
* must omit this parameter. If you include this parameter, the request will fail, regardless of the value
* that you specify.
*
*
* -
*
* If you are creating a block device mapping from an existing unencrypted volume, you can include
* this parameter, but you must specify false
. If you specify true
, the request
* will fail. In this case, we recommend that you omit the parameter.
*
*
* -
*
* If you are creating a block device mapping from an existing encrypted volume, you can include this
* parameter, and specify either true
or false
. However, if you specify
* false
, the parameter is ignored and the block device mapping is always encrypted. In this
* case, we recommend that you omit the parameter.
*
*
*/
public final Boolean encrypted() {
return encrypted;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(deleteOnTermination());
hashCode = 31 * hashCode + Objects.hashCode(iops());
hashCode = 31 * hashCode + Objects.hashCode(snapshotId());
hashCode = 31 * hashCode + Objects.hashCode(volumeSize());
hashCode = 31 * hashCode + Objects.hashCode(volumeTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(throughput());
hashCode = 31 * hashCode + Objects.hashCode(outpostArn());
hashCode = 31 * hashCode + Objects.hashCode(encrypted());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EbsBlockDevice)) {
return false;
}
EbsBlockDevice other = (EbsBlockDevice) obj;
return Objects.equals(deleteOnTermination(), other.deleteOnTermination()) && Objects.equals(iops(), other.iops())
&& Objects.equals(snapshotId(), other.snapshotId()) && Objects.equals(volumeSize(), other.volumeSize())
&& Objects.equals(volumeTypeAsString(), other.volumeTypeAsString())
&& Objects.equals(kmsKeyId(), other.kmsKeyId()) && Objects.equals(throughput(), other.throughput())
&& Objects.equals(outpostArn(), other.outpostArn()) && Objects.equals(encrypted(), other.encrypted());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EbsBlockDevice").add("DeleteOnTermination", deleteOnTermination()).add("Iops", iops())
.add("SnapshotId", snapshotId()).add("VolumeSize", volumeSize()).add("VolumeType", volumeTypeAsString())
.add("KmsKeyId", kmsKeyId()).add("Throughput", throughput()).add("OutpostArn", outpostArn())
.add("Encrypted", encrypted()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DeleteOnTermination":
return Optional.ofNullable(clazz.cast(deleteOnTermination()));
case "Iops":
return Optional.ofNullable(clazz.cast(iops()));
case "SnapshotId":
return Optional.ofNullable(clazz.cast(snapshotId()));
case "VolumeSize":
return Optional.ofNullable(clazz.cast(volumeSize()));
case "VolumeType":
return Optional.ofNullable(clazz.cast(volumeTypeAsString()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "Throughput":
return Optional.ofNullable(clazz.cast(throughput()));
case "OutpostArn":
return Optional.ofNullable(clazz.cast(outpostArn()));
case "Encrypted":
return Optional.ofNullable(clazz.cast(encrypted()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function