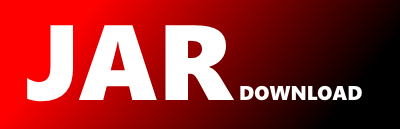
software.amazon.awssdk.services.ec2.model.Ec2InstanceConnectEndpoint Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The EC2 Instance Connect Endpoint.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Ec2InstanceConnectEndpoint implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField OWNER_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OwnerId")
.getter(getter(Ec2InstanceConnectEndpoint::ownerId))
.setter(setter(Builder::ownerId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OwnerId")
.unmarshallLocationName("ownerId").build()).build();
private static final SdkField INSTANCE_CONNECT_ENDPOINT_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InstanceConnectEndpointId")
.getter(getter(Ec2InstanceConnectEndpoint::instanceConnectEndpointId))
.setter(setter(Builder::instanceConnectEndpointId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceConnectEndpointId")
.unmarshallLocationName("instanceConnectEndpointId").build()).build();
private static final SdkField INSTANCE_CONNECT_ENDPOINT_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InstanceConnectEndpointArn")
.getter(getter(Ec2InstanceConnectEndpoint::instanceConnectEndpointArn))
.setter(setter(Builder::instanceConnectEndpointArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceConnectEndpointArn")
.unmarshallLocationName("instanceConnectEndpointArn").build()).build();
private static final SdkField STATE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("State")
.getter(getter(Ec2InstanceConnectEndpoint::stateAsString))
.setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State")
.unmarshallLocationName("state").build()).build();
private static final SdkField STATE_MESSAGE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("StateMessage")
.getter(getter(Ec2InstanceConnectEndpoint::stateMessage))
.setter(setter(Builder::stateMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateMessage")
.unmarshallLocationName("stateMessage").build()).build();
private static final SdkField DNS_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DnsName")
.getter(getter(Ec2InstanceConnectEndpoint::dnsName))
.setter(setter(Builder::dnsName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DnsName")
.unmarshallLocationName("dnsName").build()).build();
private static final SdkField FIPS_DNS_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("FipsDnsName")
.getter(getter(Ec2InstanceConnectEndpoint::fipsDnsName))
.setter(setter(Builder::fipsDnsName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FipsDnsName")
.unmarshallLocationName("fipsDnsName").build()).build();
private static final SdkField> NETWORK_INTERFACE_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NetworkInterfaceIds")
.getter(getter(Ec2InstanceConnectEndpoint::networkInterfaceIds))
.setter(setter(Builder::networkInterfaceIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkInterfaceIdSet")
.unmarshallLocationName("networkInterfaceIdSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField VPC_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VpcId")
.getter(getter(Ec2InstanceConnectEndpoint::vpcId))
.setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId")
.unmarshallLocationName("vpcId").build()).build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AvailabilityZone")
.getter(getter(Ec2InstanceConnectEndpoint::availabilityZone))
.setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone")
.unmarshallLocationName("availabilityZone").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("CreatedAt")
.getter(getter(Ec2InstanceConnectEndpoint::createdAt))
.setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedAt")
.unmarshallLocationName("createdAt").build()).build();
private static final SdkField SUBNET_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SubnetId")
.getter(getter(Ec2InstanceConnectEndpoint::subnetId))
.setter(setter(Builder::subnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetId")
.unmarshallLocationName("subnetId").build()).build();
private static final SdkField PRESERVE_CLIENT_IP_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("PreserveClientIp")
.getter(getter(Ec2InstanceConnectEndpoint::preserveClientIp))
.setter(setter(Builder::preserveClientIp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreserveClientIp")
.unmarshallLocationName("preserveClientIp").build()).build();
private static final SdkField> SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SecurityGroupIds")
.getter(getter(Ec2InstanceConnectEndpoint::securityGroupIds))
.setter(setter(Builder::securityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroupIdSet")
.unmarshallLocationName("securityGroupIdSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(Ec2InstanceConnectEndpoint::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagSet")
.unmarshallLocationName("tagSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(OWNER_ID_FIELD,
INSTANCE_CONNECT_ENDPOINT_ID_FIELD, INSTANCE_CONNECT_ENDPOINT_ARN_FIELD, STATE_FIELD, STATE_MESSAGE_FIELD,
DNS_NAME_FIELD, FIPS_DNS_NAME_FIELD, NETWORK_INTERFACE_IDS_FIELD, VPC_ID_FIELD, AVAILABILITY_ZONE_FIELD,
CREATED_AT_FIELD, SUBNET_ID_FIELD, PRESERVE_CLIENT_IP_FIELD, SECURITY_GROUP_IDS_FIELD, TAGS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("OwnerId", OWNER_ID_FIELD);
put("InstanceConnectEndpointId", INSTANCE_CONNECT_ENDPOINT_ID_FIELD);
put("InstanceConnectEndpointArn", INSTANCE_CONNECT_ENDPOINT_ARN_FIELD);
put("State", STATE_FIELD);
put("StateMessage", STATE_MESSAGE_FIELD);
put("DnsName", DNS_NAME_FIELD);
put("FipsDnsName", FIPS_DNS_NAME_FIELD);
put("NetworkInterfaceIdSet", NETWORK_INTERFACE_IDS_FIELD);
put("VpcId", VPC_ID_FIELD);
put("AvailabilityZone", AVAILABILITY_ZONE_FIELD);
put("CreatedAt", CREATED_AT_FIELD);
put("SubnetId", SUBNET_ID_FIELD);
put("PreserveClientIp", PRESERVE_CLIENT_IP_FIELD);
put("SecurityGroupIdSet", SECURITY_GROUP_IDS_FIELD);
put("TagSet", TAGS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String ownerId;
private final String instanceConnectEndpointId;
private final String instanceConnectEndpointArn;
private final String state;
private final String stateMessage;
private final String dnsName;
private final String fipsDnsName;
private final List networkInterfaceIds;
private final String vpcId;
private final String availabilityZone;
private final Instant createdAt;
private final String subnetId;
private final Boolean preserveClientIp;
private final List securityGroupIds;
private final List tags;
private Ec2InstanceConnectEndpoint(BuilderImpl builder) {
this.ownerId = builder.ownerId;
this.instanceConnectEndpointId = builder.instanceConnectEndpointId;
this.instanceConnectEndpointArn = builder.instanceConnectEndpointArn;
this.state = builder.state;
this.stateMessage = builder.stateMessage;
this.dnsName = builder.dnsName;
this.fipsDnsName = builder.fipsDnsName;
this.networkInterfaceIds = builder.networkInterfaceIds;
this.vpcId = builder.vpcId;
this.availabilityZone = builder.availabilityZone;
this.createdAt = builder.createdAt;
this.subnetId = builder.subnetId;
this.preserveClientIp = builder.preserveClientIp;
this.securityGroupIds = builder.securityGroupIds;
this.tags = builder.tags;
}
/**
*
* The ID of the Amazon Web Services account that created the EC2 Instance Connect Endpoint.
*
*
* @return The ID of the Amazon Web Services account that created the EC2 Instance Connect Endpoint.
*/
public final String ownerId() {
return ownerId;
}
/**
*
* The ID of the EC2 Instance Connect Endpoint.
*
*
* @return The ID of the EC2 Instance Connect Endpoint.
*/
public final String instanceConnectEndpointId() {
return instanceConnectEndpointId;
}
/**
*
* The Amazon Resource Name (ARN) of the EC2 Instance Connect Endpoint.
*
*
* @return The Amazon Resource Name (ARN) of the EC2 Instance Connect Endpoint.
*/
public final String instanceConnectEndpointArn() {
return instanceConnectEndpointArn;
}
/**
*
* The current state of the EC2 Instance Connect Endpoint.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link Ec2InstanceConnectEndpointState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #stateAsString}.
*
*
* @return The current state of the EC2 Instance Connect Endpoint.
* @see Ec2InstanceConnectEndpointState
*/
public final Ec2InstanceConnectEndpointState state() {
return Ec2InstanceConnectEndpointState.fromValue(state);
}
/**
*
* The current state of the EC2 Instance Connect Endpoint.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link Ec2InstanceConnectEndpointState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #stateAsString}.
*
*
* @return The current state of the EC2 Instance Connect Endpoint.
* @see Ec2InstanceConnectEndpointState
*/
public final String stateAsString() {
return state;
}
/**
*
* The message for the current state of the EC2 Instance Connect Endpoint. Can include a failure message.
*
*
* @return The message for the current state of the EC2 Instance Connect Endpoint. Can include a failure message.
*/
public final String stateMessage() {
return stateMessage;
}
/**
*
* The DNS name of the EC2 Instance Connect Endpoint.
*
*
* @return The DNS name of the EC2 Instance Connect Endpoint.
*/
public final String dnsName() {
return dnsName;
}
/**
*
*
* @return
*/
public final String fipsDnsName() {
return fipsDnsName;
}
/**
* For responses, this returns true if the service returned a value for the NetworkInterfaceIds property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNetworkInterfaceIds() {
return networkInterfaceIds != null && !(networkInterfaceIds instanceof SdkAutoConstructList);
}
/**
*
* The ID of the elastic network interface that Amazon EC2 automatically created when creating the EC2 Instance
* Connect Endpoint.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNetworkInterfaceIds} method.
*
*
* @return The ID of the elastic network interface that Amazon EC2 automatically created when creating the EC2
* Instance Connect Endpoint.
*/
public final List networkInterfaceIds() {
return networkInterfaceIds;
}
/**
*
* The ID of the VPC in which the EC2 Instance Connect Endpoint was created.
*
*
* @return The ID of the VPC in which the EC2 Instance Connect Endpoint was created.
*/
public final String vpcId() {
return vpcId;
}
/**
*
* The Availability Zone of the EC2 Instance Connect Endpoint.
*
*
* @return The Availability Zone of the EC2 Instance Connect Endpoint.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* The date and time that the EC2 Instance Connect Endpoint was created.
*
*
* @return The date and time that the EC2 Instance Connect Endpoint was created.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The ID of the subnet in which the EC2 Instance Connect Endpoint was created.
*
*
* @return The ID of the subnet in which the EC2 Instance Connect Endpoint was created.
*/
public final String subnetId() {
return subnetId;
}
/**
*
* Indicates whether your client's IP address is preserved as the source. The value is true
or
* false
.
*
*
* -
*
* If true
, your client's IP address is used when you connect to a resource.
*
*
* -
*
* If false
, the elastic network interface IP address is used when you connect to a resource.
*
*
*
*
* Default: true
*
*
* @return Indicates whether your client's IP address is preserved as the source. The value is true
or
* false
.
*
* -
*
* If true
, your client's IP address is used when you connect to a resource.
*
*
* -
*
* If false
, the elastic network interface IP address is used when you connect to a resource.
*
*
*
*
* Default: true
*/
public final Boolean preserveClientIp() {
return preserveClientIp;
}
/**
* For responses, this returns true if the service returned a value for the SecurityGroupIds property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecurityGroupIds() {
return securityGroupIds != null && !(securityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* The security groups associated with the endpoint. If you didn't specify a security group, the default security
* group for your VPC is associated with the endpoint.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecurityGroupIds} method.
*
*
* @return The security groups associated with the endpoint. If you didn't specify a security group, the default
* security group for your VPC is associated with the endpoint.
*/
public final List securityGroupIds() {
return securityGroupIds;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The tags assigned to the EC2 Instance Connect Endpoint.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The tags assigned to the EC2 Instance Connect Endpoint.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(ownerId());
hashCode = 31 * hashCode + Objects.hashCode(instanceConnectEndpointId());
hashCode = 31 * hashCode + Objects.hashCode(instanceConnectEndpointArn());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(stateMessage());
hashCode = 31 * hashCode + Objects.hashCode(dnsName());
hashCode = 31 * hashCode + Objects.hashCode(fipsDnsName());
hashCode = 31 * hashCode + Objects.hashCode(hasNetworkInterfaceIds() ? networkInterfaceIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(subnetId());
hashCode = 31 * hashCode + Objects.hashCode(preserveClientIp());
hashCode = 31 * hashCode + Objects.hashCode(hasSecurityGroupIds() ? securityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Ec2InstanceConnectEndpoint)) {
return false;
}
Ec2InstanceConnectEndpoint other = (Ec2InstanceConnectEndpoint) obj;
return Objects.equals(ownerId(), other.ownerId())
&& Objects.equals(instanceConnectEndpointId(), other.instanceConnectEndpointId())
&& Objects.equals(instanceConnectEndpointArn(), other.instanceConnectEndpointArn())
&& Objects.equals(stateAsString(), other.stateAsString()) && Objects.equals(stateMessage(), other.stateMessage())
&& Objects.equals(dnsName(), other.dnsName()) && Objects.equals(fipsDnsName(), other.fipsDnsName())
&& hasNetworkInterfaceIds() == other.hasNetworkInterfaceIds()
&& Objects.equals(networkInterfaceIds(), other.networkInterfaceIds()) && Objects.equals(vpcId(), other.vpcId())
&& Objects.equals(availabilityZone(), other.availabilityZone()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(subnetId(), other.subnetId()) && Objects.equals(preserveClientIp(), other.preserveClientIp())
&& hasSecurityGroupIds() == other.hasSecurityGroupIds()
&& Objects.equals(securityGroupIds(), other.securityGroupIds()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Ec2InstanceConnectEndpoint").add("OwnerId", ownerId())
.add("InstanceConnectEndpointId", instanceConnectEndpointId())
.add("InstanceConnectEndpointArn", instanceConnectEndpointArn()).add("State", stateAsString())
.add("StateMessage", stateMessage()).add("DnsName", dnsName()).add("FipsDnsName", fipsDnsName())
.add("NetworkInterfaceIds", hasNetworkInterfaceIds() ? networkInterfaceIds() : null).add("VpcId", vpcId())
.add("AvailabilityZone", availabilityZone()).add("CreatedAt", createdAt()).add("SubnetId", subnetId())
.add("PreserveClientIp", preserveClientIp())
.add("SecurityGroupIds", hasSecurityGroupIds() ? securityGroupIds() : null)
.add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "OwnerId":
return Optional.ofNullable(clazz.cast(ownerId()));
case "InstanceConnectEndpointId":
return Optional.ofNullable(clazz.cast(instanceConnectEndpointId()));
case "InstanceConnectEndpointArn":
return Optional.ofNullable(clazz.cast(instanceConnectEndpointArn()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "StateMessage":
return Optional.ofNullable(clazz.cast(stateMessage()));
case "DnsName":
return Optional.ofNullable(clazz.cast(dnsName()));
case "FipsDnsName":
return Optional.ofNullable(clazz.cast(fipsDnsName()));
case "NetworkInterfaceIds":
return Optional.ofNullable(clazz.cast(networkInterfaceIds()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "SubnetId":
return Optional.ofNullable(clazz.cast(subnetId()));
case "PreserveClientIp":
return Optional.ofNullable(clazz.cast(preserveClientIp()));
case "SecurityGroupIds":
return Optional.ofNullable(clazz.cast(securityGroupIds()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function