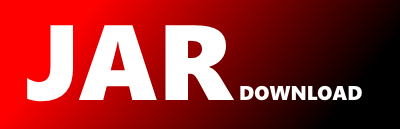
software.amazon.awssdk.services.ec2.model.FirewallStatefulRule Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a stateful rule.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class FirewallStatefulRule implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField RULE_GROUP_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RuleGroupArn")
.getter(getter(FirewallStatefulRule::ruleGroupArn))
.setter(setter(Builder::ruleGroupArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RuleGroupArn")
.unmarshallLocationName("ruleGroupArn").build()).build();
private static final SdkField> SOURCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Sources")
.getter(getter(FirewallStatefulRule::sources))
.setter(setter(Builder::sources))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceSet")
.unmarshallLocationName("sourceSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> DESTINATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Destinations")
.getter(getter(FirewallStatefulRule::destinations))
.setter(setter(Builder::destinations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DestinationSet")
.unmarshallLocationName("destinationSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> SOURCE_PORTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SourcePorts")
.getter(getter(FirewallStatefulRule::sourcePorts))
.setter(setter(Builder::sourcePorts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourcePortSet")
.unmarshallLocationName("sourcePortSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PortRange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> DESTINATION_PORTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DestinationPorts")
.getter(getter(FirewallStatefulRule::destinationPorts))
.setter(setter(Builder::destinationPorts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DestinationPortSet")
.unmarshallLocationName("destinationPortSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PortRange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField PROTOCOL_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Protocol")
.getter(getter(FirewallStatefulRule::protocol))
.setter(setter(Builder::protocol))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Protocol")
.unmarshallLocationName("protocol").build()).build();
private static final SdkField RULE_ACTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RuleAction")
.getter(getter(FirewallStatefulRule::ruleAction))
.setter(setter(Builder::ruleAction))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RuleAction")
.unmarshallLocationName("ruleAction").build()).build();
private static final SdkField DIRECTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Direction")
.getter(getter(FirewallStatefulRule::direction))
.setter(setter(Builder::direction))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Direction")
.unmarshallLocationName("direction").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(RULE_GROUP_ARN_FIELD,
SOURCES_FIELD, DESTINATIONS_FIELD, SOURCE_PORTS_FIELD, DESTINATION_PORTS_FIELD, PROTOCOL_FIELD, RULE_ACTION_FIELD,
DIRECTION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("RuleGroupArn", RULE_GROUP_ARN_FIELD);
put("SourceSet", SOURCES_FIELD);
put("DestinationSet", DESTINATIONS_FIELD);
put("SourcePortSet", SOURCE_PORTS_FIELD);
put("DestinationPortSet", DESTINATION_PORTS_FIELD);
put("Protocol", PROTOCOL_FIELD);
put("RuleAction", RULE_ACTION_FIELD);
put("Direction", DIRECTION_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String ruleGroupArn;
private final List sources;
private final List destinations;
private final List sourcePorts;
private final List destinationPorts;
private final String protocol;
private final String ruleAction;
private final String direction;
private FirewallStatefulRule(BuilderImpl builder) {
this.ruleGroupArn = builder.ruleGroupArn;
this.sources = builder.sources;
this.destinations = builder.destinations;
this.sourcePorts = builder.sourcePorts;
this.destinationPorts = builder.destinationPorts;
this.protocol = builder.protocol;
this.ruleAction = builder.ruleAction;
this.direction = builder.direction;
}
/**
*
* The ARN of the stateful rule group.
*
*
* @return The ARN of the stateful rule group.
*/
public final String ruleGroupArn() {
return ruleGroupArn;
}
/**
* For responses, this returns true if the service returned a value for the Sources property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasSources() {
return sources != null && !(sources instanceof SdkAutoConstructList);
}
/**
*
* The source IP addresses, in CIDR notation.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSources} method.
*
*
* @return The source IP addresses, in CIDR notation.
*/
public final List sources() {
return sources;
}
/**
* For responses, this returns true if the service returned a value for the Destinations property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDestinations() {
return destinations != null && !(destinations instanceof SdkAutoConstructList);
}
/**
*
* The destination IP addresses, in CIDR notation.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDestinations} method.
*
*
* @return The destination IP addresses, in CIDR notation.
*/
public final List destinations() {
return destinations;
}
/**
* For responses, this returns true if the service returned a value for the SourcePorts property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSourcePorts() {
return sourcePorts != null && !(sourcePorts instanceof SdkAutoConstructList);
}
/**
*
* The source ports.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSourcePorts} method.
*
*
* @return The source ports.
*/
public final List sourcePorts() {
return sourcePorts;
}
/**
* For responses, this returns true if the service returned a value for the DestinationPorts property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDestinationPorts() {
return destinationPorts != null && !(destinationPorts instanceof SdkAutoConstructList);
}
/**
*
* The destination ports.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDestinationPorts} method.
*
*
* @return The destination ports.
*/
public final List destinationPorts() {
return destinationPorts;
}
/**
*
* The protocol.
*
*
* @return The protocol.
*/
public final String protocol() {
return protocol;
}
/**
*
* The rule action. The possible values are pass
, drop
, and alert
.
*
*
* @return The rule action. The possible values are pass
, drop
, and alert
.
*/
public final String ruleAction() {
return ruleAction;
}
/**
*
* The direction. The possible values are FORWARD
and ANY
.
*
*
* @return The direction. The possible values are FORWARD
and ANY
.
*/
public final String direction() {
return direction;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(ruleGroupArn());
hashCode = 31 * hashCode + Objects.hashCode(hasSources() ? sources() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasDestinations() ? destinations() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSourcePorts() ? sourcePorts() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasDestinationPorts() ? destinationPorts() : null);
hashCode = 31 * hashCode + Objects.hashCode(protocol());
hashCode = 31 * hashCode + Objects.hashCode(ruleAction());
hashCode = 31 * hashCode + Objects.hashCode(direction());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof FirewallStatefulRule)) {
return false;
}
FirewallStatefulRule other = (FirewallStatefulRule) obj;
return Objects.equals(ruleGroupArn(), other.ruleGroupArn()) && hasSources() == other.hasSources()
&& Objects.equals(sources(), other.sources()) && hasDestinations() == other.hasDestinations()
&& Objects.equals(destinations(), other.destinations()) && hasSourcePorts() == other.hasSourcePorts()
&& Objects.equals(sourcePorts(), other.sourcePorts()) && hasDestinationPorts() == other.hasDestinationPorts()
&& Objects.equals(destinationPorts(), other.destinationPorts()) && Objects.equals(protocol(), other.protocol())
&& Objects.equals(ruleAction(), other.ruleAction()) && Objects.equals(direction(), other.direction());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("FirewallStatefulRule").add("RuleGroupArn", ruleGroupArn())
.add("Sources", hasSources() ? sources() : null).add("Destinations", hasDestinations() ? destinations() : null)
.add("SourcePorts", hasSourcePorts() ? sourcePorts() : null)
.add("DestinationPorts", hasDestinationPorts() ? destinationPorts() : null).add("Protocol", protocol())
.add("RuleAction", ruleAction()).add("Direction", direction()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RuleGroupArn":
return Optional.ofNullable(clazz.cast(ruleGroupArn()));
case "Sources":
return Optional.ofNullable(clazz.cast(sources()));
case "Destinations":
return Optional.ofNullable(clazz.cast(destinations()));
case "SourcePorts":
return Optional.ofNullable(clazz.cast(sourcePorts()));
case "DestinationPorts":
return Optional.ofNullable(clazz.cast(destinationPorts()));
case "Protocol":
return Optional.ofNullable(clazz.cast(protocol()));
case "RuleAction":
return Optional.ofNullable(clazz.cast(ruleAction()));
case "Direction":
return Optional.ofNullable(clazz.cast(direction()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function