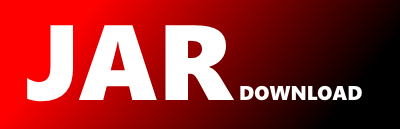
software.amazon.awssdk.services.ec2.model.InstanceMetadataOptionsResponse Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The metadata options for the instance.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class InstanceMetadataOptionsResponse implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField STATE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("State")
.getter(getter(InstanceMetadataOptionsResponse::stateAsString))
.setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State")
.unmarshallLocationName("state").build()).build();
private static final SdkField HTTP_TOKENS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("HttpTokens")
.getter(getter(InstanceMetadataOptionsResponse::httpTokensAsString))
.setter(setter(Builder::httpTokens))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HttpTokens")
.unmarshallLocationName("httpTokens").build()).build();
private static final SdkField HTTP_PUT_RESPONSE_HOP_LIMIT_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("HttpPutResponseHopLimit")
.getter(getter(InstanceMetadataOptionsResponse::httpPutResponseHopLimit))
.setter(setter(Builder::httpPutResponseHopLimit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HttpPutResponseHopLimit")
.unmarshallLocationName("httpPutResponseHopLimit").build()).build();
private static final SdkField HTTP_ENDPOINT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("HttpEndpoint")
.getter(getter(InstanceMetadataOptionsResponse::httpEndpointAsString))
.setter(setter(Builder::httpEndpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HttpEndpoint")
.unmarshallLocationName("httpEndpoint").build()).build();
private static final SdkField HTTP_PROTOCOL_IPV6_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("HttpProtocolIpv6")
.getter(getter(InstanceMetadataOptionsResponse::httpProtocolIpv6AsString))
.setter(setter(Builder::httpProtocolIpv6))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HttpProtocolIpv6")
.unmarshallLocationName("httpProtocolIpv6").build()).build();
private static final SdkField INSTANCE_METADATA_TAGS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InstanceMetadataTags")
.getter(getter(InstanceMetadataOptionsResponse::instanceMetadataTagsAsString))
.setter(setter(Builder::instanceMetadataTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceMetadataTags")
.unmarshallLocationName("instanceMetadataTags").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STATE_FIELD,
HTTP_TOKENS_FIELD, HTTP_PUT_RESPONSE_HOP_LIMIT_FIELD, HTTP_ENDPOINT_FIELD, HTTP_PROTOCOL_IPV6_FIELD,
INSTANCE_METADATA_TAGS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("State", STATE_FIELD);
put("HttpTokens", HTTP_TOKENS_FIELD);
put("HttpPutResponseHopLimit", HTTP_PUT_RESPONSE_HOP_LIMIT_FIELD);
put("HttpEndpoint", HTTP_ENDPOINT_FIELD);
put("HttpProtocolIpv6", HTTP_PROTOCOL_IPV6_FIELD);
put("InstanceMetadataTags", INSTANCE_METADATA_TAGS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String state;
private final String httpTokens;
private final Integer httpPutResponseHopLimit;
private final String httpEndpoint;
private final String httpProtocolIpv6;
private final String instanceMetadataTags;
private InstanceMetadataOptionsResponse(BuilderImpl builder) {
this.state = builder.state;
this.httpTokens = builder.httpTokens;
this.httpPutResponseHopLimit = builder.httpPutResponseHopLimit;
this.httpEndpoint = builder.httpEndpoint;
this.httpProtocolIpv6 = builder.httpProtocolIpv6;
this.instanceMetadataTags = builder.instanceMetadataTags;
}
/**
*
* The state of the metadata option changes.
*
*
* pending
- The metadata options are being updated and the instance is not ready to process metadata
* traffic with the new selection.
*
*
* applied
- The metadata options have been successfully applied on the instance.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link InstanceMetadataOptionsState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #stateAsString}.
*
*
* @return The state of the metadata option changes.
*
* pending
- The metadata options are being updated and the instance is not ready to process
* metadata traffic with the new selection.
*
*
* applied
- The metadata options have been successfully applied on the instance.
* @see InstanceMetadataOptionsState
*/
public final InstanceMetadataOptionsState state() {
return InstanceMetadataOptionsState.fromValue(state);
}
/**
*
* The state of the metadata option changes.
*
*
* pending
- The metadata options are being updated and the instance is not ready to process metadata
* traffic with the new selection.
*
*
* applied
- The metadata options have been successfully applied on the instance.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link InstanceMetadataOptionsState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #stateAsString}.
*
*
* @return The state of the metadata option changes.
*
* pending
- The metadata options are being updated and the instance is not ready to process
* metadata traffic with the new selection.
*
*
* applied
- The metadata options have been successfully applied on the instance.
* @see InstanceMetadataOptionsState
*/
public final String stateAsString() {
return state;
}
/**
*
* Indicates whether IMDSv2 is required.
*
*
* -
*
* optional
- IMDSv2 is optional, which means that you can use either IMDSv2 or IMDSv1.
*
*
* -
*
* required
- IMDSv2 is required, which means that IMDSv1 is disabled, and you must use IMDSv2.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #httpTokens} will
* return {@link HttpTokensState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #httpTokensAsString}.
*
*
* @return Indicates whether IMDSv2 is required.
*
* -
*
* optional
- IMDSv2 is optional, which means that you can use either IMDSv2 or IMDSv1.
*
*
* -
*
* required
- IMDSv2 is required, which means that IMDSv1 is disabled, and you must use IMDSv2.
*
*
* @see HttpTokensState
*/
public final HttpTokensState httpTokens() {
return HttpTokensState.fromValue(httpTokens);
}
/**
*
* Indicates whether IMDSv2 is required.
*
*
* -
*
* optional
- IMDSv2 is optional, which means that you can use either IMDSv2 or IMDSv1.
*
*
* -
*
* required
- IMDSv2 is required, which means that IMDSv1 is disabled, and you must use IMDSv2.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #httpTokens} will
* return {@link HttpTokensState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #httpTokensAsString}.
*
*
* @return Indicates whether IMDSv2 is required.
*
* -
*
* optional
- IMDSv2 is optional, which means that you can use either IMDSv2 or IMDSv1.
*
*
* -
*
* required
- IMDSv2 is required, which means that IMDSv1 is disabled, and you must use IMDSv2.
*
*
* @see HttpTokensState
*/
public final String httpTokensAsString() {
return httpTokens;
}
/**
*
* The maximum number of hops that the metadata token can travel.
*
*
* Possible values: Integers from 1
to 64
*
*
* @return The maximum number of hops that the metadata token can travel.
*
* Possible values: Integers from 1
to 64
*/
public final Integer httpPutResponseHopLimit() {
return httpPutResponseHopLimit;
}
/**
*
* Indicates whether the HTTP metadata endpoint on your instances is enabled or disabled.
*
*
* If the value is disabled
, you cannot access your instance metadata.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #httpEndpoint} will
* return {@link InstanceMetadataEndpointState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #httpEndpointAsString}.
*
*
* @return Indicates whether the HTTP metadata endpoint on your instances is enabled or disabled.
*
* If the value is disabled
, you cannot access your instance metadata.
* @see InstanceMetadataEndpointState
*/
public final InstanceMetadataEndpointState httpEndpoint() {
return InstanceMetadataEndpointState.fromValue(httpEndpoint);
}
/**
*
* Indicates whether the HTTP metadata endpoint on your instances is enabled or disabled.
*
*
* If the value is disabled
, you cannot access your instance metadata.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #httpEndpoint} will
* return {@link InstanceMetadataEndpointState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #httpEndpointAsString}.
*
*
* @return Indicates whether the HTTP metadata endpoint on your instances is enabled or disabled.
*
* If the value is disabled
, you cannot access your instance metadata.
* @see InstanceMetadataEndpointState
*/
public final String httpEndpointAsString() {
return httpEndpoint;
}
/**
*
* Indicates whether the IPv6 endpoint for the instance metadata service is enabled or disabled.
*
*
* Default: disabled
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #httpProtocolIpv6}
* will return {@link InstanceMetadataProtocolState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service
* is available from {@link #httpProtocolIpv6AsString}.
*
*
* @return Indicates whether the IPv6 endpoint for the instance metadata service is enabled or disabled.
*
* Default: disabled
* @see InstanceMetadataProtocolState
*/
public final InstanceMetadataProtocolState httpProtocolIpv6() {
return InstanceMetadataProtocolState.fromValue(httpProtocolIpv6);
}
/**
*
* Indicates whether the IPv6 endpoint for the instance metadata service is enabled or disabled.
*
*
* Default: disabled
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #httpProtocolIpv6}
* will return {@link InstanceMetadataProtocolState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service
* is available from {@link #httpProtocolIpv6AsString}.
*
*
* @return Indicates whether the IPv6 endpoint for the instance metadata service is enabled or disabled.
*
* Default: disabled
* @see InstanceMetadataProtocolState
*/
public final String httpProtocolIpv6AsString() {
return httpProtocolIpv6;
}
/**
*
* Indicates whether access to instance tags from the instance metadata is enabled or disabled. For more
* information, see Work with
* instance tags using the instance metadata.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #instanceMetadataTags} will return {@link InstanceMetadataTagsState#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #instanceMetadataTagsAsString}.
*
*
* @return Indicates whether access to instance tags from the instance metadata is enabled or disabled. For more
* information, see Work
* with instance tags using the instance metadata.
* @see InstanceMetadataTagsState
*/
public final InstanceMetadataTagsState instanceMetadataTags() {
return InstanceMetadataTagsState.fromValue(instanceMetadataTags);
}
/**
*
* Indicates whether access to instance tags from the instance metadata is enabled or disabled. For more
* information, see Work with
* instance tags using the instance metadata.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #instanceMetadataTags} will return {@link InstanceMetadataTagsState#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #instanceMetadataTagsAsString}.
*
*
* @return Indicates whether access to instance tags from the instance metadata is enabled or disabled. For more
* information, see Work
* with instance tags using the instance metadata.
* @see InstanceMetadataTagsState
*/
public final String instanceMetadataTagsAsString() {
return instanceMetadataTags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(httpTokensAsString());
hashCode = 31 * hashCode + Objects.hashCode(httpPutResponseHopLimit());
hashCode = 31 * hashCode + Objects.hashCode(httpEndpointAsString());
hashCode = 31 * hashCode + Objects.hashCode(httpProtocolIpv6AsString());
hashCode = 31 * hashCode + Objects.hashCode(instanceMetadataTagsAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof InstanceMetadataOptionsResponse)) {
return false;
}
InstanceMetadataOptionsResponse other = (InstanceMetadataOptionsResponse) obj;
return Objects.equals(stateAsString(), other.stateAsString())
&& Objects.equals(httpTokensAsString(), other.httpTokensAsString())
&& Objects.equals(httpPutResponseHopLimit(), other.httpPutResponseHopLimit())
&& Objects.equals(httpEndpointAsString(), other.httpEndpointAsString())
&& Objects.equals(httpProtocolIpv6AsString(), other.httpProtocolIpv6AsString())
&& Objects.equals(instanceMetadataTagsAsString(), other.instanceMetadataTagsAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("InstanceMetadataOptionsResponse").add("State", stateAsString())
.add("HttpTokens", httpTokensAsString()).add("HttpPutResponseHopLimit", httpPutResponseHopLimit())
.add("HttpEndpoint", httpEndpointAsString()).add("HttpProtocolIpv6", httpProtocolIpv6AsString())
.add("InstanceMetadataTags", instanceMetadataTagsAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "HttpTokens":
return Optional.ofNullable(clazz.cast(httpTokensAsString()));
case "HttpPutResponseHopLimit":
return Optional.ofNullable(clazz.cast(httpPutResponseHopLimit()));
case "HttpEndpoint":
return Optional.ofNullable(clazz.cast(httpEndpointAsString()));
case "HttpProtocolIpv6":
return Optional.ofNullable(clazz.cast(httpProtocolIpv6AsString()));
case "InstanceMetadataTags":
return Optional.ofNullable(clazz.cast(instanceMetadataTagsAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function