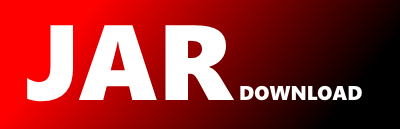
software.amazon.awssdk.services.ec2.model.Ipam Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* IPAM is a VPC feature that you can use to automate your IP address management workflows including assigning,
* tracking, troubleshooting, and auditing IP addresses across Amazon Web Services Regions and accounts throughout your
* Amazon Web Services Organization. For more information, see What is IPAM? in the Amazon VPC IPAM
* User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Ipam implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField OWNER_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OwnerId")
.getter(getter(Ipam::ownerId))
.setter(setter(Builder::ownerId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OwnerId")
.unmarshallLocationName("ownerId").build()).build();
private static final SdkField IPAM_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("IpamId")
.getter(getter(Ipam::ipamId))
.setter(setter(Builder::ipamId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpamId")
.unmarshallLocationName("ipamId").build()).build();
private static final SdkField IPAM_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("IpamArn")
.getter(getter(Ipam::ipamArn))
.setter(setter(Builder::ipamArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpamArn")
.unmarshallLocationName("ipamArn").build()).build();
private static final SdkField IPAM_REGION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("IpamRegion")
.getter(getter(Ipam::ipamRegion))
.setter(setter(Builder::ipamRegion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpamRegion")
.unmarshallLocationName("ipamRegion").build()).build();
private static final SdkField PUBLIC_DEFAULT_SCOPE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PublicDefaultScopeId")
.getter(getter(Ipam::publicDefaultScopeId))
.setter(setter(Builder::publicDefaultScopeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PublicDefaultScopeId")
.unmarshallLocationName("publicDefaultScopeId").build()).build();
private static final SdkField PRIVATE_DEFAULT_SCOPE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PrivateDefaultScopeId")
.getter(getter(Ipam::privateDefaultScopeId))
.setter(setter(Builder::privateDefaultScopeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PrivateDefaultScopeId")
.unmarshallLocationName("privateDefaultScopeId").build()).build();
private static final SdkField SCOPE_COUNT_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ScopeCount")
.getter(getter(Ipam::scopeCount))
.setter(setter(Builder::scopeCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ScopeCount")
.unmarshallLocationName("scopeCount").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Description")
.getter(getter(Ipam::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description")
.unmarshallLocationName("description").build()).build();
private static final SdkField> OPERATING_REGIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OperatingRegions")
.getter(getter(Ipam::operatingRegions))
.setter(setter(Builder::operatingRegions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperatingRegionSet")
.unmarshallLocationName("operatingRegionSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(IpamOperatingRegion::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField STATE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("State")
.getter(getter(Ipam::stateAsString))
.setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State")
.unmarshallLocationName("state").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(Ipam::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagSet")
.unmarshallLocationName("tagSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField DEFAULT_RESOURCE_DISCOVERY_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DefaultResourceDiscoveryId")
.getter(getter(Ipam::defaultResourceDiscoveryId))
.setter(setter(Builder::defaultResourceDiscoveryId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DefaultResourceDiscoveryId")
.unmarshallLocationName("defaultResourceDiscoveryId").build()).build();
private static final SdkField DEFAULT_RESOURCE_DISCOVERY_ASSOCIATION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DefaultResourceDiscoveryAssociationId")
.getter(getter(Ipam::defaultResourceDiscoveryAssociationId))
.setter(setter(Builder::defaultResourceDiscoveryAssociationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("DefaultResourceDiscoveryAssociationId")
.unmarshallLocationName("defaultResourceDiscoveryAssociationId").build()).build();
private static final SdkField RESOURCE_DISCOVERY_ASSOCIATION_COUNT_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ResourceDiscoveryAssociationCount")
.getter(getter(Ipam::resourceDiscoveryAssociationCount))
.setter(setter(Builder::resourceDiscoveryAssociationCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceDiscoveryAssociationCount")
.unmarshallLocationName("resourceDiscoveryAssociationCount").build()).build();
private static final SdkField STATE_MESSAGE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("StateMessage")
.getter(getter(Ipam::stateMessage))
.setter(setter(Builder::stateMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateMessage")
.unmarshallLocationName("stateMessage").build()).build();
private static final SdkField TIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Tier")
.getter(getter(Ipam::tierAsString))
.setter(setter(Builder::tier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tier")
.unmarshallLocationName("tier").build()).build();
private static final SdkField ENABLE_PRIVATE_GUA_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EnablePrivateGua")
.getter(getter(Ipam::enablePrivateGua))
.setter(setter(Builder::enablePrivateGua))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnablePrivateGua")
.unmarshallLocationName("enablePrivateGua").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(OWNER_ID_FIELD, IPAM_ID_FIELD,
IPAM_ARN_FIELD, IPAM_REGION_FIELD, PUBLIC_DEFAULT_SCOPE_ID_FIELD, PRIVATE_DEFAULT_SCOPE_ID_FIELD, SCOPE_COUNT_FIELD,
DESCRIPTION_FIELD, OPERATING_REGIONS_FIELD, STATE_FIELD, TAGS_FIELD, DEFAULT_RESOURCE_DISCOVERY_ID_FIELD,
DEFAULT_RESOURCE_DISCOVERY_ASSOCIATION_ID_FIELD, RESOURCE_DISCOVERY_ASSOCIATION_COUNT_FIELD, STATE_MESSAGE_FIELD,
TIER_FIELD, ENABLE_PRIVATE_GUA_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("OwnerId", OWNER_ID_FIELD);
put("IpamId", IPAM_ID_FIELD);
put("IpamArn", IPAM_ARN_FIELD);
put("IpamRegion", IPAM_REGION_FIELD);
put("PublicDefaultScopeId", PUBLIC_DEFAULT_SCOPE_ID_FIELD);
put("PrivateDefaultScopeId", PRIVATE_DEFAULT_SCOPE_ID_FIELD);
put("ScopeCount", SCOPE_COUNT_FIELD);
put("Description", DESCRIPTION_FIELD);
put("OperatingRegionSet", OPERATING_REGIONS_FIELD);
put("State", STATE_FIELD);
put("TagSet", TAGS_FIELD);
put("DefaultResourceDiscoveryId", DEFAULT_RESOURCE_DISCOVERY_ID_FIELD);
put("DefaultResourceDiscoveryAssociationId", DEFAULT_RESOURCE_DISCOVERY_ASSOCIATION_ID_FIELD);
put("ResourceDiscoveryAssociationCount", RESOURCE_DISCOVERY_ASSOCIATION_COUNT_FIELD);
put("StateMessage", STATE_MESSAGE_FIELD);
put("Tier", TIER_FIELD);
put("EnablePrivateGua", ENABLE_PRIVATE_GUA_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String ownerId;
private final String ipamId;
private final String ipamArn;
private final String ipamRegion;
private final String publicDefaultScopeId;
private final String privateDefaultScopeId;
private final Integer scopeCount;
private final String description;
private final List operatingRegions;
private final String state;
private final List tags;
private final String defaultResourceDiscoveryId;
private final String defaultResourceDiscoveryAssociationId;
private final Integer resourceDiscoveryAssociationCount;
private final String stateMessage;
private final String tier;
private final Boolean enablePrivateGua;
private Ipam(BuilderImpl builder) {
this.ownerId = builder.ownerId;
this.ipamId = builder.ipamId;
this.ipamArn = builder.ipamArn;
this.ipamRegion = builder.ipamRegion;
this.publicDefaultScopeId = builder.publicDefaultScopeId;
this.privateDefaultScopeId = builder.privateDefaultScopeId;
this.scopeCount = builder.scopeCount;
this.description = builder.description;
this.operatingRegions = builder.operatingRegions;
this.state = builder.state;
this.tags = builder.tags;
this.defaultResourceDiscoveryId = builder.defaultResourceDiscoveryId;
this.defaultResourceDiscoveryAssociationId = builder.defaultResourceDiscoveryAssociationId;
this.resourceDiscoveryAssociationCount = builder.resourceDiscoveryAssociationCount;
this.stateMessage = builder.stateMessage;
this.tier = builder.tier;
this.enablePrivateGua = builder.enablePrivateGua;
}
/**
*
* The Amazon Web Services account ID of the owner of the IPAM.
*
*
* @return The Amazon Web Services account ID of the owner of the IPAM.
*/
public final String ownerId() {
return ownerId;
}
/**
*
* The ID of the IPAM.
*
*
* @return The ID of the IPAM.
*/
public final String ipamId() {
return ipamId;
}
/**
*
* The Amazon Resource Name (ARN) of the IPAM.
*
*
* @return The Amazon Resource Name (ARN) of the IPAM.
*/
public final String ipamArn() {
return ipamArn;
}
/**
*
* The Amazon Web Services Region of the IPAM.
*
*
* @return The Amazon Web Services Region of the IPAM.
*/
public final String ipamRegion() {
return ipamRegion;
}
/**
*
* The ID of the IPAM's default public scope.
*
*
* @return The ID of the IPAM's default public scope.
*/
public final String publicDefaultScopeId() {
return publicDefaultScopeId;
}
/**
*
* The ID of the IPAM's default private scope.
*
*
* @return The ID of the IPAM's default private scope.
*/
public final String privateDefaultScopeId() {
return privateDefaultScopeId;
}
/**
*
* The number of scopes in the IPAM. The scope quota is 5. For more information on quotas, see Quotas in IPAM in the Amazon VPC IPAM
* User Guide.
*
*
* @return The number of scopes in the IPAM. The scope quota is 5. For more information on quotas, see Quotas in IPAM in the Amazon
* VPC IPAM User Guide.
*/
public final Integer scopeCount() {
return scopeCount;
}
/**
*
* The description for the IPAM.
*
*
* @return The description for the IPAM.
*/
public final String description() {
return description;
}
/**
* For responses, this returns true if the service returned a value for the OperatingRegions property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasOperatingRegions() {
return operatingRegions != null && !(operatingRegions instanceof SdkAutoConstructList);
}
/**
*
* The operating Regions for an IPAM. Operating Regions are Amazon Web Services Regions where the IPAM is allowed to
* manage IP address CIDRs. IPAM only discovers and monitors resources in the Amazon Web Services Regions you select
* as operating Regions.
*
*
* For more information about operating Regions, see Create an IPAM in the Amazon VPC IPAM
* User Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOperatingRegions} method.
*
*
* @return The operating Regions for an IPAM. Operating Regions are Amazon Web Services Regions where the IPAM is
* allowed to manage IP address CIDRs. IPAM only discovers and monitors resources in the Amazon Web Services
* Regions you select as operating Regions.
*
* For more information about operating Regions, see Create an IPAM in the Amazon
* VPC IPAM User Guide.
*/
public final List operatingRegions() {
return operatingRegions;
}
/**
*
* The state of the IPAM.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link IpamState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the IPAM.
* @see IpamState
*/
public final IpamState state() {
return IpamState.fromValue(state);
}
/**
*
* The state of the IPAM.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link IpamState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the IPAM.
* @see IpamState
*/
public final String stateAsString() {
return state;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The key/value combination of a tag assigned to the resource. Use the tag key in the filter name and the tag value
* as the filter value. For example, to find all resources that have a tag with the key Owner
and the
* value TeamA
, specify tag:Owner
for the filter name and TeamA
for the
* filter value.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The key/value combination of a tag assigned to the resource. Use the tag key in the filter name and the
* tag value as the filter value. For example, to find all resources that have a tag with the key
* Owner
and the value TeamA
, specify tag:Owner
for the filter name
* and TeamA
for the filter value.
*/
public final List tags() {
return tags;
}
/**
*
* The IPAM's default resource discovery ID.
*
*
* @return The IPAM's default resource discovery ID.
*/
public final String defaultResourceDiscoveryId() {
return defaultResourceDiscoveryId;
}
/**
*
* The IPAM's default resource discovery association ID.
*
*
* @return The IPAM's default resource discovery association ID.
*/
public final String defaultResourceDiscoveryAssociationId() {
return defaultResourceDiscoveryAssociationId;
}
/**
*
* The IPAM's resource discovery association count.
*
*
* @return The IPAM's resource discovery association count.
*/
public final Integer resourceDiscoveryAssociationCount() {
return resourceDiscoveryAssociationCount;
}
/**
*
* The state message.
*
*
* @return The state message.
*/
public final String stateMessage() {
return stateMessage;
}
/**
*
* IPAM is offered in a Free Tier and an Advanced Tier. For more information about the features available in each
* tier and the costs associated with the tiers, see Amazon VPC pricing
* > IPAM tab.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tier} will return
* {@link IpamTier#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #tierAsString}.
*
*
* @return IPAM is offered in a Free Tier and an Advanced Tier. For more information about the features available in
* each tier and the costs associated with the tiers, see Amazon VPC pricing > IPAM tab.
* @see IpamTier
*/
public final IpamTier tier() {
return IpamTier.fromValue(tier);
}
/**
*
* IPAM is offered in a Free Tier and an Advanced Tier. For more information about the features available in each
* tier and the costs associated with the tiers, see Amazon VPC pricing
* > IPAM tab.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tier} will return
* {@link IpamTier#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #tierAsString}.
*
*
* @return IPAM is offered in a Free Tier and an Advanced Tier. For more information about the features available in
* each tier and the costs associated with the tiers, see Amazon VPC pricing > IPAM tab.
* @see IpamTier
*/
public final String tierAsString() {
return tier;
}
/**
*
* Enable this option to use your own GUA ranges as private IPv6 addresses. This option is disabled by default.
*
*
* @return Enable this option to use your own GUA ranges as private IPv6 addresses. This option is disabled by
* default.
*/
public final Boolean enablePrivateGua() {
return enablePrivateGua;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(ownerId());
hashCode = 31 * hashCode + Objects.hashCode(ipamId());
hashCode = 31 * hashCode + Objects.hashCode(ipamArn());
hashCode = 31 * hashCode + Objects.hashCode(ipamRegion());
hashCode = 31 * hashCode + Objects.hashCode(publicDefaultScopeId());
hashCode = 31 * hashCode + Objects.hashCode(privateDefaultScopeId());
hashCode = 31 * hashCode + Objects.hashCode(scopeCount());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(hasOperatingRegions() ? operatingRegions() : null);
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(defaultResourceDiscoveryId());
hashCode = 31 * hashCode + Objects.hashCode(defaultResourceDiscoveryAssociationId());
hashCode = 31 * hashCode + Objects.hashCode(resourceDiscoveryAssociationCount());
hashCode = 31 * hashCode + Objects.hashCode(stateMessage());
hashCode = 31 * hashCode + Objects.hashCode(tierAsString());
hashCode = 31 * hashCode + Objects.hashCode(enablePrivateGua());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Ipam)) {
return false;
}
Ipam other = (Ipam) obj;
return Objects.equals(ownerId(), other.ownerId()) && Objects.equals(ipamId(), other.ipamId())
&& Objects.equals(ipamArn(), other.ipamArn()) && Objects.equals(ipamRegion(), other.ipamRegion())
&& Objects.equals(publicDefaultScopeId(), other.publicDefaultScopeId())
&& Objects.equals(privateDefaultScopeId(), other.privateDefaultScopeId())
&& Objects.equals(scopeCount(), other.scopeCount()) && Objects.equals(description(), other.description())
&& hasOperatingRegions() == other.hasOperatingRegions()
&& Objects.equals(operatingRegions(), other.operatingRegions())
&& Objects.equals(stateAsString(), other.stateAsString()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags())
&& Objects.equals(defaultResourceDiscoveryId(), other.defaultResourceDiscoveryId())
&& Objects.equals(defaultResourceDiscoveryAssociationId(), other.defaultResourceDiscoveryAssociationId())
&& Objects.equals(resourceDiscoveryAssociationCount(), other.resourceDiscoveryAssociationCount())
&& Objects.equals(stateMessage(), other.stateMessage()) && Objects.equals(tierAsString(), other.tierAsString())
&& Objects.equals(enablePrivateGua(), other.enablePrivateGua());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Ipam").add("OwnerId", ownerId()).add("IpamId", ipamId()).add("IpamArn", ipamArn())
.add("IpamRegion", ipamRegion()).add("PublicDefaultScopeId", publicDefaultScopeId())
.add("PrivateDefaultScopeId", privateDefaultScopeId()).add("ScopeCount", scopeCount())
.add("Description", description()).add("OperatingRegions", hasOperatingRegions() ? operatingRegions() : null)
.add("State", stateAsString()).add("Tags", hasTags() ? tags() : null)
.add("DefaultResourceDiscoveryId", defaultResourceDiscoveryId())
.add("DefaultResourceDiscoveryAssociationId", defaultResourceDiscoveryAssociationId())
.add("ResourceDiscoveryAssociationCount", resourceDiscoveryAssociationCount())
.add("StateMessage", stateMessage()).add("Tier", tierAsString()).add("EnablePrivateGua", enablePrivateGua())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "OwnerId":
return Optional.ofNullable(clazz.cast(ownerId()));
case "IpamId":
return Optional.ofNullable(clazz.cast(ipamId()));
case "IpamArn":
return Optional.ofNullable(clazz.cast(ipamArn()));
case "IpamRegion":
return Optional.ofNullable(clazz.cast(ipamRegion()));
case "PublicDefaultScopeId":
return Optional.ofNullable(clazz.cast(publicDefaultScopeId()));
case "PrivateDefaultScopeId":
return Optional.ofNullable(clazz.cast(privateDefaultScopeId()));
case "ScopeCount":
return Optional.ofNullable(clazz.cast(scopeCount()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "OperatingRegions":
return Optional.ofNullable(clazz.cast(operatingRegions()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "DefaultResourceDiscoveryId":
return Optional.ofNullable(clazz.cast(defaultResourceDiscoveryId()));
case "DefaultResourceDiscoveryAssociationId":
return Optional.ofNullable(clazz.cast(defaultResourceDiscoveryAssociationId()));
case "ResourceDiscoveryAssociationCount":
return Optional.ofNullable(clazz.cast(resourceDiscoveryAssociationCount()));
case "StateMessage":
return Optional.ofNullable(clazz.cast(stateMessage()));
case "Tier":
return Optional.ofNullable(clazz.cast(tierAsString()));
case "EnablePrivateGua":
return Optional.ofNullable(clazz.cast(enablePrivateGua()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function