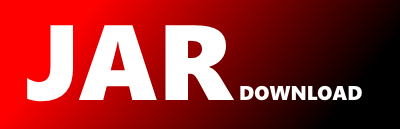
software.amazon.awssdk.services.ec2.model.ModifyImageAttributeRequest Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the parameters for ModifyImageAttribute.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyImageAttributeRequest extends Ec2Request implements
ToCopyableBuilder {
private static final SdkField ATTRIBUTE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Attribute")
.getter(getter(ModifyImageAttributeRequest::attribute))
.setter(setter(Builder::attribute))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Attribute")
.unmarshallLocationName("Attribute").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Description")
.getter(getter(ModifyImageAttributeRequest::description))
.setter(setter(Builder::description))
.constructor(AttributeValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description")
.unmarshallLocationName("Description").build()).build();
private static final SdkField IMAGE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ImageId")
.getter(getter(ModifyImageAttributeRequest::imageId))
.setter(setter(Builder::imageId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageId")
.unmarshallLocationName("ImageId").build()).build();
private static final SdkField LAUNCH_PERMISSION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("LaunchPermission")
.getter(getter(ModifyImageAttributeRequest::launchPermission))
.setter(setter(Builder::launchPermission))
.constructor(LaunchPermissionModifications::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchPermission")
.unmarshallLocationName("LaunchPermission").build()).build();
private static final SdkField OPERATION_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OperationType")
.getter(getter(ModifyImageAttributeRequest::operationTypeAsString))
.setter(setter(Builder::operationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperationType")
.unmarshallLocationName("OperationType").build()).build();
private static final SdkField> PRODUCT_CODES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ProductCodes")
.getter(getter(ModifyImageAttributeRequest::productCodes))
.setter(setter(Builder::productCodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProductCode")
.unmarshallLocationName("ProductCode").build(),
ListTrait
.builder()
.memberLocationName("ProductCode")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ProductCode").unmarshallLocationName("ProductCode").build())
.build()).build()).build();
private static final SdkField> USER_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("UserGroups")
.getter(getter(ModifyImageAttributeRequest::userGroups))
.setter(setter(Builder::userGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserGroup")
.unmarshallLocationName("UserGroup").build(),
ListTrait
.builder()
.memberLocationName("UserGroup")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("UserGroup").unmarshallLocationName("UserGroup").build())
.build()).build()).build();
private static final SdkField> USER_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("UserIds")
.getter(getter(ModifyImageAttributeRequest::userIds))
.setter(setter(Builder::userIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserId")
.unmarshallLocationName("UserId").build(),
ListTrait
.builder()
.memberLocationName("UserId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("UserId").unmarshallLocationName("UserId").build()).build())
.build()).build();
private static final SdkField VALUE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Value")
.getter(getter(ModifyImageAttributeRequest::value))
.setter(setter(Builder::value))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Value")
.unmarshallLocationName("Value").build()).build();
private static final SdkField> ORGANIZATION_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OrganizationArns")
.getter(getter(ModifyImageAttributeRequest::organizationArns))
.setter(setter(Builder::organizationArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OrganizationArn")
.unmarshallLocationName("OrganizationArn").build(),
ListTrait
.builder()
.memberLocationName("OrganizationArn")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("OrganizationArn").unmarshallLocationName("OrganizationArn")
.build()).build()).build()).build();
private static final SdkField> ORGANIZATIONAL_UNIT_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OrganizationalUnitArns")
.getter(getter(ModifyImageAttributeRequest::organizationalUnitArns))
.setter(setter(Builder::organizationalUnitArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OrganizationalUnitArn")
.unmarshallLocationName("OrganizationalUnitArn").build(),
ListTrait
.builder()
.memberLocationName("OrganizationalUnitArn")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("OrganizationalUnitArn")
.unmarshallLocationName("OrganizationalUnitArn").build()).build()).build())
.build();
private static final SdkField IMDS_SUPPORT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ImdsSupport")
.getter(getter(ModifyImageAttributeRequest::imdsSupport))
.setter(setter(Builder::imdsSupport))
.constructor(AttributeValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImdsSupport")
.unmarshallLocationName("ImdsSupport").build()).build();
private static final SdkField DRY_RUN_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("DryRun")
.getter(getter(ModifyImageAttributeRequest::dryRun))
.setter(setter(Builder::dryRun))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DryRun")
.unmarshallLocationName("dryRun").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ATTRIBUTE_FIELD,
DESCRIPTION_FIELD, IMAGE_ID_FIELD, LAUNCH_PERMISSION_FIELD, OPERATION_TYPE_FIELD, PRODUCT_CODES_FIELD,
USER_GROUPS_FIELD, USER_IDS_FIELD, VALUE_FIELD, ORGANIZATION_ARNS_FIELD, ORGANIZATIONAL_UNIT_ARNS_FIELD,
IMDS_SUPPORT_FIELD, DRY_RUN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("Attribute", ATTRIBUTE_FIELD);
put("Description", DESCRIPTION_FIELD);
put("ImageId", IMAGE_ID_FIELD);
put("LaunchPermission", LAUNCH_PERMISSION_FIELD);
put("OperationType", OPERATION_TYPE_FIELD);
put("ProductCode", PRODUCT_CODES_FIELD);
put("UserGroup", USER_GROUPS_FIELD);
put("UserId", USER_IDS_FIELD);
put("Value", VALUE_FIELD);
put("OrganizationArn", ORGANIZATION_ARNS_FIELD);
put("OrganizationalUnitArn", ORGANIZATIONAL_UNIT_ARNS_FIELD);
put("ImdsSupport", IMDS_SUPPORT_FIELD);
put("DryRun", DRY_RUN_FIELD);
}
});
private final String attribute;
private final AttributeValue description;
private final String imageId;
private final LaunchPermissionModifications launchPermission;
private final String operationType;
private final List productCodes;
private final List userGroups;
private final List userIds;
private final String value;
private final List organizationArns;
private final List organizationalUnitArns;
private final AttributeValue imdsSupport;
private final Boolean dryRun;
private ModifyImageAttributeRequest(BuilderImpl builder) {
super(builder);
this.attribute = builder.attribute;
this.description = builder.description;
this.imageId = builder.imageId;
this.launchPermission = builder.launchPermission;
this.operationType = builder.operationType;
this.productCodes = builder.productCodes;
this.userGroups = builder.userGroups;
this.userIds = builder.userIds;
this.value = builder.value;
this.organizationArns = builder.organizationArns;
this.organizationalUnitArns = builder.organizationalUnitArns;
this.imdsSupport = builder.imdsSupport;
this.dryRun = builder.dryRun;
}
/**
*
* The name of the attribute to modify.
*
*
* Valid values: description
| imdsSupport
| launchPermission
*
*
* @return The name of the attribute to modify.
*
* Valid values: description
| imdsSupport
| launchPermission
*/
public final String attribute() {
return attribute;
}
/**
*
* A new description for the AMI.
*
*
* @return A new description for the AMI.
*/
public final AttributeValue description() {
return description;
}
/**
*
* The ID of the AMI.
*
*
* @return The ID of the AMI.
*/
public final String imageId() {
return imageId;
}
/**
*
* A new launch permission for the AMI.
*
*
* @return A new launch permission for the AMI.
*/
public final LaunchPermissionModifications launchPermission() {
return launchPermission;
}
/**
*
* The operation type. This parameter can be used only when the Attribute
parameter is
* launchPermission
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #operationType}
* will return {@link OperationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #operationTypeAsString}.
*
*
* @return The operation type. This parameter can be used only when the Attribute
parameter is
* launchPermission
.
* @see OperationType
*/
public final OperationType operationType() {
return OperationType.fromValue(operationType);
}
/**
*
* The operation type. This parameter can be used only when the Attribute
parameter is
* launchPermission
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #operationType}
* will return {@link OperationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #operationTypeAsString}.
*
*
* @return The operation type. This parameter can be used only when the Attribute
parameter is
* launchPermission
.
* @see OperationType
*/
public final String operationTypeAsString() {
return operationType;
}
/**
* For responses, this returns true if the service returned a value for the ProductCodes property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasProductCodes() {
return productCodes != null && !(productCodes instanceof SdkAutoConstructList);
}
/**
*
* Not supported.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasProductCodes} method.
*
*
* @return Not supported.
*/
public final List productCodes() {
return productCodes;
}
/**
* For responses, this returns true if the service returned a value for the UserGroups property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasUserGroups() {
return userGroups != null && !(userGroups instanceof SdkAutoConstructList);
}
/**
*
* The user groups. This parameter can be used only when the Attribute
parameter is
* launchPermission
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasUserGroups} method.
*
*
* @return The user groups. This parameter can be used only when the Attribute
parameter is
* launchPermission
.
*/
public final List userGroups() {
return userGroups;
}
/**
* For responses, this returns true if the service returned a value for the UserIds property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasUserIds() {
return userIds != null && !(userIds instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Web Services account IDs. This parameter can be used only when the Attribute
parameter is
* launchPermission
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasUserIds} method.
*
*
* @return The Amazon Web Services account IDs. This parameter can be used only when the Attribute
* parameter is launchPermission
.
*/
public final List userIds() {
return userIds;
}
/**
*
* The value of the attribute being modified. This parameter can be used only when the Attribute
* parameter is description
or imdsSupport
.
*
*
* @return The value of the attribute being modified. This parameter can be used only when the
* Attribute
parameter is description
or imdsSupport
.
*/
public final String value() {
return value;
}
/**
* For responses, this returns true if the service returned a value for the OrganizationArns property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasOrganizationArns() {
return organizationArns != null && !(organizationArns instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Resource Name (ARN) of an organization. This parameter can be used only when the
* Attribute
parameter is launchPermission
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOrganizationArns} method.
*
*
* @return The Amazon Resource Name (ARN) of an organization. This parameter can be used only when the
* Attribute
parameter is launchPermission
.
*/
public final List organizationArns() {
return organizationArns;
}
/**
* For responses, this returns true if the service returned a value for the OrganizationalUnitArns property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasOrganizationalUnitArns() {
return organizationalUnitArns != null && !(organizationalUnitArns instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Resource Name (ARN) of an organizational unit (OU). This parameter can be used only when the
* Attribute
parameter is launchPermission
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOrganizationalUnitArns} method.
*
*
* @return The Amazon Resource Name (ARN) of an organizational unit (OU). This parameter can be used only when the
* Attribute
parameter is launchPermission
.
*/
public final List organizationalUnitArns() {
return organizationalUnitArns;
}
/**
*
* Set to v2.0
to indicate that IMDSv2 is specified in the AMI. Instances launched from this AMI will
* have HttpTokens
automatically set to required
so that, by default, the instance
* requires that IMDSv2 is used when requesting instance metadata. In addition, HttpPutResponseHopLimit
* is set to 2
. For more information, see Configure the AMI in the Amazon EC2 User Guide.
*
*
*
* Do not use this parameter unless your AMI software supports IMDSv2. After you set the value to v2.0
,
* you can't undo it. The only way to “reset” your AMI is to create a new AMI from the underlying snapshot.
*
*
*
* @return Set to v2.0
to indicate that IMDSv2 is specified in the AMI. Instances launched from this
* AMI will have HttpTokens
automatically set to required
so that, by default, the
* instance requires that IMDSv2 is used when requesting instance metadata. In addition,
* HttpPutResponseHopLimit
is set to 2
. For more information, see Configure the AMI in the Amazon EC2 User Guide.
*
* Do not use this parameter unless your AMI software supports IMDSv2. After you set the value to
* v2.0
, you can't undo it. The only way to “reset” your AMI is to create a new AMI from the
* underlying snapshot.
*
*/
public final AttributeValue imdsSupport() {
return imdsSupport;
}
/**
*
* Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*
*
* @return Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*/
public final Boolean dryRun() {
return dryRun;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(attribute());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(imageId());
hashCode = 31 * hashCode + Objects.hashCode(launchPermission());
hashCode = 31 * hashCode + Objects.hashCode(operationTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasProductCodes() ? productCodes() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasUserGroups() ? userGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasUserIds() ? userIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(value());
hashCode = 31 * hashCode + Objects.hashCode(hasOrganizationArns() ? organizationArns() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasOrganizationalUnitArns() ? organizationalUnitArns() : null);
hashCode = 31 * hashCode + Objects.hashCode(imdsSupport());
hashCode = 31 * hashCode + Objects.hashCode(dryRun());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyImageAttributeRequest)) {
return false;
}
ModifyImageAttributeRequest other = (ModifyImageAttributeRequest) obj;
return Objects.equals(attribute(), other.attribute()) && Objects.equals(description(), other.description())
&& Objects.equals(imageId(), other.imageId()) && Objects.equals(launchPermission(), other.launchPermission())
&& Objects.equals(operationTypeAsString(), other.operationTypeAsString())
&& hasProductCodes() == other.hasProductCodes() && Objects.equals(productCodes(), other.productCodes())
&& hasUserGroups() == other.hasUserGroups() && Objects.equals(userGroups(), other.userGroups())
&& hasUserIds() == other.hasUserIds() && Objects.equals(userIds(), other.userIds())
&& Objects.equals(value(), other.value()) && hasOrganizationArns() == other.hasOrganizationArns()
&& Objects.equals(organizationArns(), other.organizationArns())
&& hasOrganizationalUnitArns() == other.hasOrganizationalUnitArns()
&& Objects.equals(organizationalUnitArns(), other.organizationalUnitArns())
&& Objects.equals(imdsSupport(), other.imdsSupport()) && Objects.equals(dryRun(), other.dryRun());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyImageAttributeRequest").add("Attribute", attribute()).add("Description", description())
.add("ImageId", imageId()).add("LaunchPermission", launchPermission())
.add("OperationType", operationTypeAsString()).add("ProductCodes", hasProductCodes() ? productCodes() : null)
.add("UserGroups", hasUserGroups() ? userGroups() : null).add("UserIds", hasUserIds() ? userIds() : null)
.add("Value", value()).add("OrganizationArns", hasOrganizationArns() ? organizationArns() : null)
.add("OrganizationalUnitArns", hasOrganizationalUnitArns() ? organizationalUnitArns() : null)
.add("ImdsSupport", imdsSupport()).add("DryRun", dryRun()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Attribute":
return Optional.ofNullable(clazz.cast(attribute()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "ImageId":
return Optional.ofNullable(clazz.cast(imageId()));
case "LaunchPermission":
return Optional.ofNullable(clazz.cast(launchPermission()));
case "OperationType":
return Optional.ofNullable(clazz.cast(operationTypeAsString()));
case "ProductCodes":
return Optional.ofNullable(clazz.cast(productCodes()));
case "UserGroups":
return Optional.ofNullable(clazz.cast(userGroups()));
case "UserIds":
return Optional.ofNullable(clazz.cast(userIds()));
case "Value":
return Optional.ofNullable(clazz.cast(value()));
case "OrganizationArns":
return Optional.ofNullable(clazz.cast(organizationArns()));
case "OrganizationalUnitArns":
return Optional.ofNullable(clazz.cast(organizationalUnitArns()));
case "ImdsSupport":
return Optional.ofNullable(clazz.cast(imdsSupport()));
case "DryRun":
return Optional.ofNullable(clazz.cast(dryRun()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function