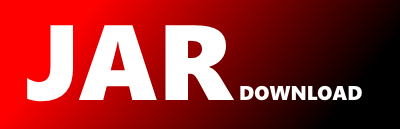
software.amazon.awssdk.services.ec2.model.ModifyIpamPoolRequest Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyIpamPoolRequest extends Ec2Request implements
ToCopyableBuilder {
private static final SdkField DRY_RUN_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("DryRun")
.getter(getter(ModifyIpamPoolRequest::dryRun))
.setter(setter(Builder::dryRun))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DryRun")
.unmarshallLocationName("DryRun").build()).build();
private static final SdkField IPAM_POOL_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("IpamPoolId")
.getter(getter(ModifyIpamPoolRequest::ipamPoolId))
.setter(setter(Builder::ipamPoolId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpamPoolId")
.unmarshallLocationName("IpamPoolId").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Description")
.getter(getter(ModifyIpamPoolRequest::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description")
.unmarshallLocationName("Description").build()).build();
private static final SdkField AUTO_IMPORT_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("AutoImport")
.getter(getter(ModifyIpamPoolRequest::autoImport))
.setter(setter(Builder::autoImport))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoImport")
.unmarshallLocationName("AutoImport").build()).build();
private static final SdkField ALLOCATION_MIN_NETMASK_LENGTH_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("AllocationMinNetmaskLength")
.getter(getter(ModifyIpamPoolRequest::allocationMinNetmaskLength))
.setter(setter(Builder::allocationMinNetmaskLength))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocationMinNetmaskLength")
.unmarshallLocationName("AllocationMinNetmaskLength").build()).build();
private static final SdkField ALLOCATION_MAX_NETMASK_LENGTH_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("AllocationMaxNetmaskLength")
.getter(getter(ModifyIpamPoolRequest::allocationMaxNetmaskLength))
.setter(setter(Builder::allocationMaxNetmaskLength))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocationMaxNetmaskLength")
.unmarshallLocationName("AllocationMaxNetmaskLength").build()).build();
private static final SdkField ALLOCATION_DEFAULT_NETMASK_LENGTH_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("AllocationDefaultNetmaskLength")
.getter(getter(ModifyIpamPoolRequest::allocationDefaultNetmaskLength))
.setter(setter(Builder::allocationDefaultNetmaskLength))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocationDefaultNetmaskLength")
.unmarshallLocationName("AllocationDefaultNetmaskLength").build()).build();
private static final SdkField CLEAR_ALLOCATION_DEFAULT_NETMASK_LENGTH_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ClearAllocationDefaultNetmaskLength")
.getter(getter(ModifyIpamPoolRequest::clearAllocationDefaultNetmaskLength))
.setter(setter(Builder::clearAllocationDefaultNetmaskLength))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ClearAllocationDefaultNetmaskLength")
.unmarshallLocationName("ClearAllocationDefaultNetmaskLength").build()).build();
private static final SdkField> ADD_ALLOCATION_RESOURCE_TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AddAllocationResourceTags")
.getter(getter(ModifyIpamPoolRequest::addAllocationResourceTags))
.setter(setter(Builder::addAllocationResourceTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AddAllocationResourceTag")
.unmarshallLocationName("AddAllocationResourceTag").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(RequestIpamResourceTag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> REMOVE_ALLOCATION_RESOURCE_TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RemoveAllocationResourceTags")
.getter(getter(ModifyIpamPoolRequest::removeAllocationResourceTags))
.setter(setter(Builder::removeAllocationResourceTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RemoveAllocationResourceTag")
.unmarshallLocationName("RemoveAllocationResourceTag").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(RequestIpamResourceTag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DRY_RUN_FIELD,
IPAM_POOL_ID_FIELD, DESCRIPTION_FIELD, AUTO_IMPORT_FIELD, ALLOCATION_MIN_NETMASK_LENGTH_FIELD,
ALLOCATION_MAX_NETMASK_LENGTH_FIELD, ALLOCATION_DEFAULT_NETMASK_LENGTH_FIELD,
CLEAR_ALLOCATION_DEFAULT_NETMASK_LENGTH_FIELD, ADD_ALLOCATION_RESOURCE_TAGS_FIELD,
REMOVE_ALLOCATION_RESOURCE_TAGS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("DryRun", DRY_RUN_FIELD);
put("IpamPoolId", IPAM_POOL_ID_FIELD);
put("Description", DESCRIPTION_FIELD);
put("AutoImport", AUTO_IMPORT_FIELD);
put("AllocationMinNetmaskLength", ALLOCATION_MIN_NETMASK_LENGTH_FIELD);
put("AllocationMaxNetmaskLength", ALLOCATION_MAX_NETMASK_LENGTH_FIELD);
put("AllocationDefaultNetmaskLength", ALLOCATION_DEFAULT_NETMASK_LENGTH_FIELD);
put("ClearAllocationDefaultNetmaskLength", CLEAR_ALLOCATION_DEFAULT_NETMASK_LENGTH_FIELD);
put("AddAllocationResourceTag", ADD_ALLOCATION_RESOURCE_TAGS_FIELD);
put("RemoveAllocationResourceTag", REMOVE_ALLOCATION_RESOURCE_TAGS_FIELD);
}
});
private final Boolean dryRun;
private final String ipamPoolId;
private final String description;
private final Boolean autoImport;
private final Integer allocationMinNetmaskLength;
private final Integer allocationMaxNetmaskLength;
private final Integer allocationDefaultNetmaskLength;
private final Boolean clearAllocationDefaultNetmaskLength;
private final List addAllocationResourceTags;
private final List removeAllocationResourceTags;
private ModifyIpamPoolRequest(BuilderImpl builder) {
super(builder);
this.dryRun = builder.dryRun;
this.ipamPoolId = builder.ipamPoolId;
this.description = builder.description;
this.autoImport = builder.autoImport;
this.allocationMinNetmaskLength = builder.allocationMinNetmaskLength;
this.allocationMaxNetmaskLength = builder.allocationMaxNetmaskLength;
this.allocationDefaultNetmaskLength = builder.allocationDefaultNetmaskLength;
this.clearAllocationDefaultNetmaskLength = builder.clearAllocationDefaultNetmaskLength;
this.addAllocationResourceTags = builder.addAllocationResourceTags;
this.removeAllocationResourceTags = builder.removeAllocationResourceTags;
}
/**
*
* A check for whether you have the required permissions for the action without actually making the request and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*
*
* @return A check for whether you have the required permissions for the action without actually making the request
* and provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*/
public final Boolean dryRun() {
return dryRun;
}
/**
*
* The ID of the IPAM pool you want to modify.
*
*
* @return The ID of the IPAM pool you want to modify.
*/
public final String ipamPoolId() {
return ipamPoolId;
}
/**
*
* The description of the IPAM pool you want to modify.
*
*
* @return The description of the IPAM pool you want to modify.
*/
public final String description() {
return description;
}
/**
*
* If true, IPAM will continuously look for resources within the CIDR range of this pool and automatically import
* them as allocations into your IPAM. The CIDRs that will be allocated for these resources must not already be
* allocated to other resources in order for the import to succeed. IPAM will import a CIDR regardless of its
* compliance with the pool's allocation rules, so a resource might be imported and subsequently marked as
* noncompliant. If IPAM discovers multiple CIDRs that overlap, IPAM will import the largest CIDR only. If IPAM
* discovers multiple CIDRs with matching CIDRs, IPAM will randomly import one of them only.
*
*
* A locale must be set on the pool for this feature to work.
*
*
* @return If true, IPAM will continuously look for resources within the CIDR range of this pool and automatically
* import them as allocations into your IPAM. The CIDRs that will be allocated for these resources must not
* already be allocated to other resources in order for the import to succeed. IPAM will import a CIDR
* regardless of its compliance with the pool's allocation rules, so a resource might be imported and
* subsequently marked as noncompliant. If IPAM discovers multiple CIDRs that overlap, IPAM will import the
* largest CIDR only. If IPAM discovers multiple CIDRs with matching CIDRs, IPAM will randomly import one of
* them only.
*
* A locale must be set on the pool for this feature to work.
*/
public final Boolean autoImport() {
return autoImport;
}
/**
*
* The minimum netmask length required for CIDR allocations in this IPAM pool to be compliant. Possible netmask
* lengths for IPv4 addresses are 0 - 32. Possible netmask lengths for IPv6 addresses are 0 - 128. The minimum
* netmask length must be less than the maximum netmask length.
*
*
* @return The minimum netmask length required for CIDR allocations in this IPAM pool to be compliant. Possible
* netmask lengths for IPv4 addresses are 0 - 32. Possible netmask lengths for IPv6 addresses are 0 - 128.
* The minimum netmask length must be less than the maximum netmask length.
*/
public final Integer allocationMinNetmaskLength() {
return allocationMinNetmaskLength;
}
/**
*
* The maximum netmask length possible for CIDR allocations in this IPAM pool to be compliant. Possible netmask
* lengths for IPv4 addresses are 0 - 32. Possible netmask lengths for IPv6 addresses are 0 - 128.The maximum
* netmask length must be greater than the minimum netmask length.
*
*
* @return The maximum netmask length possible for CIDR allocations in this IPAM pool to be compliant. Possible
* netmask lengths for IPv4 addresses are 0 - 32. Possible netmask lengths for IPv6 addresses are 0 -
* 128.The maximum netmask length must be greater than the minimum netmask length.
*/
public final Integer allocationMaxNetmaskLength() {
return allocationMaxNetmaskLength;
}
/**
*
* The default netmask length for allocations added to this pool. If, for example, the CIDR assigned to this pool is
* 10.0.0.0/8 and you enter 16 here, new allocations will default to 10.0.0.0/16.
*
*
* @return The default netmask length for allocations added to this pool. If, for example, the CIDR assigned to this
* pool is 10.0.0.0/8 and you enter 16 here, new allocations will default to 10.0.0.0/16.
*/
public final Integer allocationDefaultNetmaskLength() {
return allocationDefaultNetmaskLength;
}
/**
*
* Clear the default netmask length allocation rule for this pool.
*
*
* @return Clear the default netmask length allocation rule for this pool.
*/
public final Boolean clearAllocationDefaultNetmaskLength() {
return clearAllocationDefaultNetmaskLength;
}
/**
* For responses, this returns true if the service returned a value for the AddAllocationResourceTags property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasAddAllocationResourceTags() {
return addAllocationResourceTags != null && !(addAllocationResourceTags instanceof SdkAutoConstructList);
}
/**
*
* Add tag allocation rules to a pool. For more information about allocation rules, see Create a top-level pool in the
* Amazon VPC IPAM User Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAddAllocationResourceTags} method.
*
*
* @return Add tag allocation rules to a pool. For more information about allocation rules, see Create a top-level pool in
* the Amazon VPC IPAM User Guide.
*/
public final List addAllocationResourceTags() {
return addAllocationResourceTags;
}
/**
* For responses, this returns true if the service returned a value for the RemoveAllocationResourceTags property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasRemoveAllocationResourceTags() {
return removeAllocationResourceTags != null && !(removeAllocationResourceTags instanceof SdkAutoConstructList);
}
/**
*
* Remove tag allocation rules from a pool.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRemoveAllocationResourceTags} method.
*
*
* @return Remove tag allocation rules from a pool.
*/
public final List removeAllocationResourceTags() {
return removeAllocationResourceTags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dryRun());
hashCode = 31 * hashCode + Objects.hashCode(ipamPoolId());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(autoImport());
hashCode = 31 * hashCode + Objects.hashCode(allocationMinNetmaskLength());
hashCode = 31 * hashCode + Objects.hashCode(allocationMaxNetmaskLength());
hashCode = 31 * hashCode + Objects.hashCode(allocationDefaultNetmaskLength());
hashCode = 31 * hashCode + Objects.hashCode(clearAllocationDefaultNetmaskLength());
hashCode = 31 * hashCode + Objects.hashCode(hasAddAllocationResourceTags() ? addAllocationResourceTags() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasRemoveAllocationResourceTags() ? removeAllocationResourceTags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyIpamPoolRequest)) {
return false;
}
ModifyIpamPoolRequest other = (ModifyIpamPoolRequest) obj;
return Objects.equals(dryRun(), other.dryRun()) && Objects.equals(ipamPoolId(), other.ipamPoolId())
&& Objects.equals(description(), other.description()) && Objects.equals(autoImport(), other.autoImport())
&& Objects.equals(allocationMinNetmaskLength(), other.allocationMinNetmaskLength())
&& Objects.equals(allocationMaxNetmaskLength(), other.allocationMaxNetmaskLength())
&& Objects.equals(allocationDefaultNetmaskLength(), other.allocationDefaultNetmaskLength())
&& Objects.equals(clearAllocationDefaultNetmaskLength(), other.clearAllocationDefaultNetmaskLength())
&& hasAddAllocationResourceTags() == other.hasAddAllocationResourceTags()
&& Objects.equals(addAllocationResourceTags(), other.addAllocationResourceTags())
&& hasRemoveAllocationResourceTags() == other.hasRemoveAllocationResourceTags()
&& Objects.equals(removeAllocationResourceTags(), other.removeAllocationResourceTags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyIpamPoolRequest").add("DryRun", dryRun()).add("IpamPoolId", ipamPoolId())
.add("Description", description()).add("AutoImport", autoImport())
.add("AllocationMinNetmaskLength", allocationMinNetmaskLength())
.add("AllocationMaxNetmaskLength", allocationMaxNetmaskLength())
.add("AllocationDefaultNetmaskLength", allocationDefaultNetmaskLength())
.add("ClearAllocationDefaultNetmaskLength", clearAllocationDefaultNetmaskLength())
.add("AddAllocationResourceTags", hasAddAllocationResourceTags() ? addAllocationResourceTags() : null)
.add("RemoveAllocationResourceTags", hasRemoveAllocationResourceTags() ? removeAllocationResourceTags() : null)
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DryRun":
return Optional.ofNullable(clazz.cast(dryRun()));
case "IpamPoolId":
return Optional.ofNullable(clazz.cast(ipamPoolId()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "AutoImport":
return Optional.ofNullable(clazz.cast(autoImport()));
case "AllocationMinNetmaskLength":
return Optional.ofNullable(clazz.cast(allocationMinNetmaskLength()));
case "AllocationMaxNetmaskLength":
return Optional.ofNullable(clazz.cast(allocationMaxNetmaskLength()));
case "AllocationDefaultNetmaskLength":
return Optional.ofNullable(clazz.cast(allocationDefaultNetmaskLength()));
case "ClearAllocationDefaultNetmaskLength":
return Optional.ofNullable(clazz.cast(clearAllocationDefaultNetmaskLength()));
case "AddAllocationResourceTags":
return Optional.ofNullable(clazz.cast(addAllocationResourceTags()));
case "RemoveAllocationResourceTags":
return Optional.ofNullable(clazz.cast(removeAllocationResourceTags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function