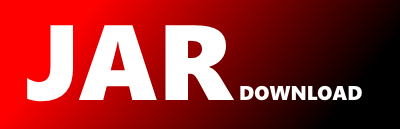
software.amazon.awssdk.services.ec2.model.NatGateway Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a NAT gateway.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NatGateway implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CREATE_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("CreateTime")
.getter(getter(NatGateway::createTime))
.setter(setter(Builder::createTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateTime")
.unmarshallLocationName("createTime").build()).build();
private static final SdkField DELETE_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("DeleteTime")
.getter(getter(NatGateway::deleteTime))
.setter(setter(Builder::deleteTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeleteTime")
.unmarshallLocationName("deleteTime").build()).build();
private static final SdkField FAILURE_CODE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("FailureCode")
.getter(getter(NatGateway::failureCode))
.setter(setter(Builder::failureCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureCode")
.unmarshallLocationName("failureCode").build()).build();
private static final SdkField FAILURE_MESSAGE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("FailureMessage")
.getter(getter(NatGateway::failureMessage))
.setter(setter(Builder::failureMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureMessage")
.unmarshallLocationName("failureMessage").build()).build();
private static final SdkField> NAT_GATEWAY_ADDRESSES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NatGatewayAddresses")
.getter(getter(NatGateway::natGatewayAddresses))
.setter(setter(Builder::natGatewayAddresses))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NatGatewayAddressSet")
.unmarshallLocationName("natGatewayAddressSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NatGatewayAddress::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField NAT_GATEWAY_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NatGatewayId")
.getter(getter(NatGateway::natGatewayId))
.setter(setter(Builder::natGatewayId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NatGatewayId")
.unmarshallLocationName("natGatewayId").build()).build();
private static final SdkField PROVISIONED_BANDWIDTH_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ProvisionedBandwidth")
.getter(getter(NatGateway::provisionedBandwidth))
.setter(setter(Builder::provisionedBandwidth))
.constructor(ProvisionedBandwidth::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProvisionedBandwidth")
.unmarshallLocationName("provisionedBandwidth").build()).build();
private static final SdkField STATE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("State")
.getter(getter(NatGateway::stateAsString))
.setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State")
.unmarshallLocationName("state").build()).build();
private static final SdkField SUBNET_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SubnetId")
.getter(getter(NatGateway::subnetId))
.setter(setter(Builder::subnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetId")
.unmarshallLocationName("subnetId").build()).build();
private static final SdkField VPC_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VpcId")
.getter(getter(NatGateway::vpcId))
.setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId")
.unmarshallLocationName("vpcId").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(NatGateway::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagSet")
.unmarshallLocationName("tagSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField CONNECTIVITY_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ConnectivityType")
.getter(getter(NatGateway::connectivityTypeAsString))
.setter(setter(Builder::connectivityType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConnectivityType")
.unmarshallLocationName("connectivityType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CREATE_TIME_FIELD,
DELETE_TIME_FIELD, FAILURE_CODE_FIELD, FAILURE_MESSAGE_FIELD, NAT_GATEWAY_ADDRESSES_FIELD, NAT_GATEWAY_ID_FIELD,
PROVISIONED_BANDWIDTH_FIELD, STATE_FIELD, SUBNET_ID_FIELD, VPC_ID_FIELD, TAGS_FIELD, CONNECTIVITY_TYPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("CreateTime", CREATE_TIME_FIELD);
put("DeleteTime", DELETE_TIME_FIELD);
put("FailureCode", FAILURE_CODE_FIELD);
put("FailureMessage", FAILURE_MESSAGE_FIELD);
put("NatGatewayAddressSet", NAT_GATEWAY_ADDRESSES_FIELD);
put("NatGatewayId", NAT_GATEWAY_ID_FIELD);
put("ProvisionedBandwidth", PROVISIONED_BANDWIDTH_FIELD);
put("State", STATE_FIELD);
put("SubnetId", SUBNET_ID_FIELD);
put("VpcId", VPC_ID_FIELD);
put("TagSet", TAGS_FIELD);
put("ConnectivityType", CONNECTIVITY_TYPE_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final Instant createTime;
private final Instant deleteTime;
private final String failureCode;
private final String failureMessage;
private final List natGatewayAddresses;
private final String natGatewayId;
private final ProvisionedBandwidth provisionedBandwidth;
private final String state;
private final String subnetId;
private final String vpcId;
private final List tags;
private final String connectivityType;
private NatGateway(BuilderImpl builder) {
this.createTime = builder.createTime;
this.deleteTime = builder.deleteTime;
this.failureCode = builder.failureCode;
this.failureMessage = builder.failureMessage;
this.natGatewayAddresses = builder.natGatewayAddresses;
this.natGatewayId = builder.natGatewayId;
this.provisionedBandwidth = builder.provisionedBandwidth;
this.state = builder.state;
this.subnetId = builder.subnetId;
this.vpcId = builder.vpcId;
this.tags = builder.tags;
this.connectivityType = builder.connectivityType;
}
/**
*
* The date and time the NAT gateway was created.
*
*
* @return The date and time the NAT gateway was created.
*/
public final Instant createTime() {
return createTime;
}
/**
*
* The date and time the NAT gateway was deleted, if applicable.
*
*
* @return The date and time the NAT gateway was deleted, if applicable.
*/
public final Instant deleteTime() {
return deleteTime;
}
/**
*
* If the NAT gateway could not be created, specifies the error code for the failure. (
* InsufficientFreeAddressesInSubnet
| Gateway.NotAttached
|
* InvalidAllocationID.NotFound
| Resource.AlreadyAssociated
| InternalError
* | InvalidSubnetID.NotFound
)
*
*
* @return If the NAT gateway could not be created, specifies the error code for the failure. (
* InsufficientFreeAddressesInSubnet
| Gateway.NotAttached
|
* InvalidAllocationID.NotFound
| Resource.AlreadyAssociated
|
* InternalError
| InvalidSubnetID.NotFound
)
*/
public final String failureCode() {
return failureCode;
}
/**
*
* If the NAT gateway could not be created, specifies the error message for the failure, that corresponds to the
* error code.
*
*
* -
*
* For InsufficientFreeAddressesInSubnet: "Subnet has insufficient free addresses to create this NAT gateway"
*
*
* -
*
* For Gateway.NotAttached: "Network vpc-xxxxxxxx has no Internet gateway attached"
*
*
* -
*
* For InvalidAllocationID.NotFound:
* "Elastic IP address eipalloc-xxxxxxxx could not be associated with this NAT gateway"
*
*
* -
*
* For Resource.AlreadyAssociated: "Elastic IP address eipalloc-xxxxxxxx is already associated"
*
*
* -
*
* For InternalError:
* "Network interface eni-xxxxxxxx, created and used internally by this NAT gateway is in an invalid state. Please try again."
*
*
* -
*
* For InvalidSubnetID.NotFound: "The specified subnet subnet-xxxxxxxx does not exist or could not be found."
*
*
*
*
* @return If the NAT gateway could not be created, specifies the error message for the failure, that corresponds to
* the error code.
*
* -
*
* For InsufficientFreeAddressesInSubnet:
* "Subnet has insufficient free addresses to create this NAT gateway"
*
*
* -
*
* For Gateway.NotAttached: "Network vpc-xxxxxxxx has no Internet gateway attached"
*
*
* -
*
* For InvalidAllocationID.NotFound:
* "Elastic IP address eipalloc-xxxxxxxx could not be associated with this NAT gateway"
*
*
* -
*
* For Resource.AlreadyAssociated: "Elastic IP address eipalloc-xxxxxxxx is already associated"
*
*
* -
*
* For InternalError:
* "Network interface eni-xxxxxxxx, created and used internally by this NAT gateway is in an invalid state. Please try again."
*
*
* -
*
* For InvalidSubnetID.NotFound:
* "The specified subnet subnet-xxxxxxxx does not exist or could not be found."
*
*
*/
public final String failureMessage() {
return failureMessage;
}
/**
* For responses, this returns true if the service returned a value for the NatGatewayAddresses property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNatGatewayAddresses() {
return natGatewayAddresses != null && !(natGatewayAddresses instanceof SdkAutoConstructList);
}
/**
*
* Information about the IP addresses and network interface associated with the NAT gateway.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNatGatewayAddresses} method.
*
*
* @return Information about the IP addresses and network interface associated with the NAT gateway.
*/
public final List natGatewayAddresses() {
return natGatewayAddresses;
}
/**
*
* The ID of the NAT gateway.
*
*
* @return The ID of the NAT gateway.
*/
public final String natGatewayId() {
return natGatewayId;
}
/**
*
* Reserved. If you need to sustain traffic greater than the documented
* limits, contact Amazon Web Services Support.
*
*
* @return Reserved. If you need to sustain traffic greater than the documented limits, contact Amazon Web Services Support.
*/
public final ProvisionedBandwidth provisionedBandwidth() {
return provisionedBandwidth;
}
/**
*
* The state of the NAT gateway.
*
*
* -
*
* pending
: The NAT gateway is being created and is not ready to process traffic.
*
*
* -
*
* failed
: The NAT gateway could not be created. Check the failureCode
and
* failureMessage
fields for the reason.
*
*
* -
*
* available
: The NAT gateway is able to process traffic. This status remains until you delete the NAT
* gateway, and does not indicate the health of the NAT gateway.
*
*
* -
*
* deleting
: The NAT gateway is in the process of being terminated and may still be processing traffic.
*
*
* -
*
* deleted
: The NAT gateway has been terminated and is no longer processing traffic.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link NatGatewayState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the NAT gateway.
*
* -
*
* pending
: The NAT gateway is being created and is not ready to process traffic.
*
*
* -
*
* failed
: The NAT gateway could not be created. Check the failureCode
and
* failureMessage
fields for the reason.
*
*
* -
*
* available
: The NAT gateway is able to process traffic. This status remains until you delete
* the NAT gateway, and does not indicate the health of the NAT gateway.
*
*
* -
*
* deleting
: The NAT gateway is in the process of being terminated and may still be processing
* traffic.
*
*
* -
*
* deleted
: The NAT gateway has been terminated and is no longer processing traffic.
*
*
* @see NatGatewayState
*/
public final NatGatewayState state() {
return NatGatewayState.fromValue(state);
}
/**
*
* The state of the NAT gateway.
*
*
* -
*
* pending
: The NAT gateway is being created and is not ready to process traffic.
*
*
* -
*
* failed
: The NAT gateway could not be created. Check the failureCode
and
* failureMessage
fields for the reason.
*
*
* -
*
* available
: The NAT gateway is able to process traffic. This status remains until you delete the NAT
* gateway, and does not indicate the health of the NAT gateway.
*
*
* -
*
* deleting
: The NAT gateway is in the process of being terminated and may still be processing traffic.
*
*
* -
*
* deleted
: The NAT gateway has been terminated and is no longer processing traffic.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link NatGatewayState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the NAT gateway.
*
* -
*
* pending
: The NAT gateway is being created and is not ready to process traffic.
*
*
* -
*
* failed
: The NAT gateway could not be created. Check the failureCode
and
* failureMessage
fields for the reason.
*
*
* -
*
* available
: The NAT gateway is able to process traffic. This status remains until you delete
* the NAT gateway, and does not indicate the health of the NAT gateway.
*
*
* -
*
* deleting
: The NAT gateway is in the process of being terminated and may still be processing
* traffic.
*
*
* -
*
* deleted
: The NAT gateway has been terminated and is no longer processing traffic.
*
*
* @see NatGatewayState
*/
public final String stateAsString() {
return state;
}
/**
*
* The ID of the subnet in which the NAT gateway is located.
*
*
* @return The ID of the subnet in which the NAT gateway is located.
*/
public final String subnetId() {
return subnetId;
}
/**
*
* The ID of the VPC in which the NAT gateway is located.
*
*
* @return The ID of the VPC in which the NAT gateway is located.
*/
public final String vpcId() {
return vpcId;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The tags for the NAT gateway.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The tags for the NAT gateway.
*/
public final List tags() {
return tags;
}
/**
*
* Indicates whether the NAT gateway supports public or private connectivity.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #connectivityType}
* will return {@link ConnectivityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #connectivityTypeAsString}.
*
*
* @return Indicates whether the NAT gateway supports public or private connectivity.
* @see ConnectivityType
*/
public final ConnectivityType connectivityType() {
return ConnectivityType.fromValue(connectivityType);
}
/**
*
* Indicates whether the NAT gateway supports public or private connectivity.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #connectivityType}
* will return {@link ConnectivityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #connectivityTypeAsString}.
*
*
* @return Indicates whether the NAT gateway supports public or private connectivity.
* @see ConnectivityType
*/
public final String connectivityTypeAsString() {
return connectivityType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(createTime());
hashCode = 31 * hashCode + Objects.hashCode(deleteTime());
hashCode = 31 * hashCode + Objects.hashCode(failureCode());
hashCode = 31 * hashCode + Objects.hashCode(failureMessage());
hashCode = 31 * hashCode + Objects.hashCode(hasNatGatewayAddresses() ? natGatewayAddresses() : null);
hashCode = 31 * hashCode + Objects.hashCode(natGatewayId());
hashCode = 31 * hashCode + Objects.hashCode(provisionedBandwidth());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(subnetId());
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(connectivityTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NatGateway)) {
return false;
}
NatGateway other = (NatGateway) obj;
return Objects.equals(createTime(), other.createTime()) && Objects.equals(deleteTime(), other.deleteTime())
&& Objects.equals(failureCode(), other.failureCode()) && Objects.equals(failureMessage(), other.failureMessage())
&& hasNatGatewayAddresses() == other.hasNatGatewayAddresses()
&& Objects.equals(natGatewayAddresses(), other.natGatewayAddresses())
&& Objects.equals(natGatewayId(), other.natGatewayId())
&& Objects.equals(provisionedBandwidth(), other.provisionedBandwidth())
&& Objects.equals(stateAsString(), other.stateAsString()) && Objects.equals(subnetId(), other.subnetId())
&& Objects.equals(vpcId(), other.vpcId()) && hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(connectivityTypeAsString(), other.connectivityTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NatGateway").add("CreateTime", createTime()).add("DeleteTime", deleteTime())
.add("FailureCode", failureCode()).add("FailureMessage", failureMessage())
.add("NatGatewayAddresses", hasNatGatewayAddresses() ? natGatewayAddresses() : null)
.add("NatGatewayId", natGatewayId()).add("ProvisionedBandwidth", provisionedBandwidth())
.add("State", stateAsString()).add("SubnetId", subnetId()).add("VpcId", vpcId())
.add("Tags", hasTags() ? tags() : null).add("ConnectivityType", connectivityTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CreateTime":
return Optional.ofNullable(clazz.cast(createTime()));
case "DeleteTime":
return Optional.ofNullable(clazz.cast(deleteTime()));
case "FailureCode":
return Optional.ofNullable(clazz.cast(failureCode()));
case "FailureMessage":
return Optional.ofNullable(clazz.cast(failureMessage()));
case "NatGatewayAddresses":
return Optional.ofNullable(clazz.cast(natGatewayAddresses()));
case "NatGatewayId":
return Optional.ofNullable(clazz.cast(natGatewayId()));
case "ProvisionedBandwidth":
return Optional.ofNullable(clazz.cast(provisionedBandwidth()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "SubnetId":
return Optional.ofNullable(clazz.cast(subnetId()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "ConnectivityType":
return Optional.ofNullable(clazz.cast(connectivityTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function