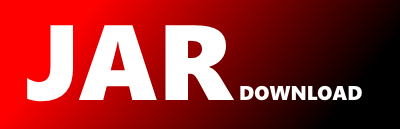
software.amazon.awssdk.services.ec2.model.NatGatewayAddress Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the IP addresses and network interface associated with a NAT gateway.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NatGatewayAddress implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ALLOCATION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AllocationId")
.getter(getter(NatGatewayAddress::allocationId))
.setter(setter(Builder::allocationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocationId")
.unmarshallLocationName("allocationId").build()).build();
private static final SdkField NETWORK_INTERFACE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NetworkInterfaceId")
.getter(getter(NatGatewayAddress::networkInterfaceId))
.setter(setter(Builder::networkInterfaceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkInterfaceId")
.unmarshallLocationName("networkInterfaceId").build()).build();
private static final SdkField PRIVATE_IP_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PrivateIp")
.getter(getter(NatGatewayAddress::privateIp))
.setter(setter(Builder::privateIp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PrivateIp")
.unmarshallLocationName("privateIp").build()).build();
private static final SdkField PUBLIC_IP_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PublicIp")
.getter(getter(NatGatewayAddress::publicIp))
.setter(setter(Builder::publicIp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PublicIp")
.unmarshallLocationName("publicIp").build()).build();
private static final SdkField ASSOCIATION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AssociationId")
.getter(getter(NatGatewayAddress::associationId))
.setter(setter(Builder::associationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssociationId")
.unmarshallLocationName("associationId").build()).build();
private static final SdkField IS_PRIMARY_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IsPrimary")
.getter(getter(NatGatewayAddress::isPrimary))
.setter(setter(Builder::isPrimary))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsPrimary")
.unmarshallLocationName("isPrimary").build()).build();
private static final SdkField FAILURE_MESSAGE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("FailureMessage")
.getter(getter(NatGatewayAddress::failureMessage))
.setter(setter(Builder::failureMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureMessage")
.unmarshallLocationName("failureMessage").build()).build();
private static final SdkField STATUS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Status")
.getter(getter(NatGatewayAddress::statusAsString))
.setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status")
.unmarshallLocationName("status").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ALLOCATION_ID_FIELD,
NETWORK_INTERFACE_ID_FIELD, PRIVATE_IP_FIELD, PUBLIC_IP_FIELD, ASSOCIATION_ID_FIELD, IS_PRIMARY_FIELD,
FAILURE_MESSAGE_FIELD, STATUS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("AllocationId", ALLOCATION_ID_FIELD);
put("NetworkInterfaceId", NETWORK_INTERFACE_ID_FIELD);
put("PrivateIp", PRIVATE_IP_FIELD);
put("PublicIp", PUBLIC_IP_FIELD);
put("AssociationId", ASSOCIATION_ID_FIELD);
put("IsPrimary", IS_PRIMARY_FIELD);
put("FailureMessage", FAILURE_MESSAGE_FIELD);
put("Status", STATUS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String allocationId;
private final String networkInterfaceId;
private final String privateIp;
private final String publicIp;
private final String associationId;
private final Boolean isPrimary;
private final String failureMessage;
private final String status;
private NatGatewayAddress(BuilderImpl builder) {
this.allocationId = builder.allocationId;
this.networkInterfaceId = builder.networkInterfaceId;
this.privateIp = builder.privateIp;
this.publicIp = builder.publicIp;
this.associationId = builder.associationId;
this.isPrimary = builder.isPrimary;
this.failureMessage = builder.failureMessage;
this.status = builder.status;
}
/**
*
* [Public NAT gateway only] The allocation ID of the Elastic IP address that's associated with the NAT gateway.
*
*
* @return [Public NAT gateway only] The allocation ID of the Elastic IP address that's associated with the NAT
* gateway.
*/
public final String allocationId() {
return allocationId;
}
/**
*
* The ID of the network interface associated with the NAT gateway.
*
*
* @return The ID of the network interface associated with the NAT gateway.
*/
public final String networkInterfaceId() {
return networkInterfaceId;
}
/**
*
* The private IP address associated with the NAT gateway.
*
*
* @return The private IP address associated with the NAT gateway.
*/
public final String privateIp() {
return privateIp;
}
/**
*
* [Public NAT gateway only] The Elastic IP address associated with the NAT gateway.
*
*
* @return [Public NAT gateway only] The Elastic IP address associated with the NAT gateway.
*/
public final String publicIp() {
return publicIp;
}
/**
*
* [Public NAT gateway only] The association ID of the Elastic IP address that's associated with the NAT gateway.
*
*
* @return [Public NAT gateway only] The association ID of the Elastic IP address that's associated with the NAT
* gateway.
*/
public final String associationId() {
return associationId;
}
/**
*
* Defines if the IP address is the primary address.
*
*
* @return Defines if the IP address is the primary address.
*/
public final Boolean isPrimary() {
return isPrimary;
}
/**
*
* The address failure message.
*
*
* @return The address failure message.
*/
public final String failureMessage() {
return failureMessage;
}
/**
*
* The address status.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link NatGatewayAddressStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The address status.
* @see NatGatewayAddressStatus
*/
public final NatGatewayAddressStatus status() {
return NatGatewayAddressStatus.fromValue(status);
}
/**
*
* The address status.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link NatGatewayAddressStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The address status.
* @see NatGatewayAddressStatus
*/
public final String statusAsString() {
return status;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(allocationId());
hashCode = 31 * hashCode + Objects.hashCode(networkInterfaceId());
hashCode = 31 * hashCode + Objects.hashCode(privateIp());
hashCode = 31 * hashCode + Objects.hashCode(publicIp());
hashCode = 31 * hashCode + Objects.hashCode(associationId());
hashCode = 31 * hashCode + Objects.hashCode(isPrimary());
hashCode = 31 * hashCode + Objects.hashCode(failureMessage());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NatGatewayAddress)) {
return false;
}
NatGatewayAddress other = (NatGatewayAddress) obj;
return Objects.equals(allocationId(), other.allocationId())
&& Objects.equals(networkInterfaceId(), other.networkInterfaceId())
&& Objects.equals(privateIp(), other.privateIp()) && Objects.equals(publicIp(), other.publicIp())
&& Objects.equals(associationId(), other.associationId()) && Objects.equals(isPrimary(), other.isPrimary())
&& Objects.equals(failureMessage(), other.failureMessage())
&& Objects.equals(statusAsString(), other.statusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NatGatewayAddress").add("AllocationId", allocationId())
.add("NetworkInterfaceId", networkInterfaceId()).add("PrivateIp", privateIp()).add("PublicIp", publicIp())
.add("AssociationId", associationId()).add("IsPrimary", isPrimary()).add("FailureMessage", failureMessage())
.add("Status", statusAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AllocationId":
return Optional.ofNullable(clazz.cast(allocationId()));
case "NetworkInterfaceId":
return Optional.ofNullable(clazz.cast(networkInterfaceId()));
case "PrivateIp":
return Optional.ofNullable(clazz.cast(privateIp()));
case "PublicIp":
return Optional.ofNullable(clazz.cast(publicIp()));
case "AssociationId":
return Optional.ofNullable(clazz.cast(associationId()));
case "IsPrimary":
return Optional.ofNullable(clazz.cast(isPrimary()));
case "FailureMessage":
return Optional.ofNullable(clazz.cast(failureMessage()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function