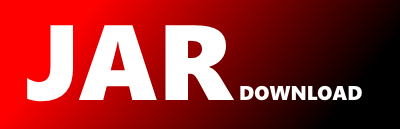
software.amazon.awssdk.services.ec2.model.NetworkInfo Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the networking features of the instance type.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NetworkInfo implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NETWORK_PERFORMANCE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NetworkPerformance")
.getter(getter(NetworkInfo::networkPerformance))
.setter(setter(Builder::networkPerformance))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkPerformance")
.unmarshallLocationName("networkPerformance").build()).build();
private static final SdkField MAXIMUM_NETWORK_INTERFACES_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("MaximumNetworkInterfaces")
.getter(getter(NetworkInfo::maximumNetworkInterfaces))
.setter(setter(Builder::maximumNetworkInterfaces))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaximumNetworkInterfaces")
.unmarshallLocationName("maximumNetworkInterfaces").build()).build();
private static final SdkField MAXIMUM_NETWORK_CARDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("MaximumNetworkCards")
.getter(getter(NetworkInfo::maximumNetworkCards))
.setter(setter(Builder::maximumNetworkCards))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaximumNetworkCards")
.unmarshallLocationName("maximumNetworkCards").build()).build();
private static final SdkField DEFAULT_NETWORK_CARD_INDEX_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("DefaultNetworkCardIndex")
.getter(getter(NetworkInfo::defaultNetworkCardIndex))
.setter(setter(Builder::defaultNetworkCardIndex))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DefaultNetworkCardIndex")
.unmarshallLocationName("defaultNetworkCardIndex").build()).build();
private static final SdkField> NETWORK_CARDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NetworkCards")
.getter(getter(NetworkInfo::networkCards))
.setter(setter(Builder::networkCards))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkCards")
.unmarshallLocationName("networkCards").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NetworkCardInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField IPV4_ADDRESSES_PER_INTERFACE_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("Ipv4AddressesPerInterface")
.getter(getter(NetworkInfo::ipv4AddressesPerInterface))
.setter(setter(Builder::ipv4AddressesPerInterface))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ipv4AddressesPerInterface")
.unmarshallLocationName("ipv4AddressesPerInterface").build()).build();
private static final SdkField IPV6_ADDRESSES_PER_INTERFACE_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("Ipv6AddressesPerInterface")
.getter(getter(NetworkInfo::ipv6AddressesPerInterface))
.setter(setter(Builder::ipv6AddressesPerInterface))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ipv6AddressesPerInterface")
.unmarshallLocationName("ipv6AddressesPerInterface").build()).build();
private static final SdkField IPV6_SUPPORTED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("Ipv6Supported")
.getter(getter(NetworkInfo::ipv6Supported))
.setter(setter(Builder::ipv6Supported))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ipv6Supported")
.unmarshallLocationName("ipv6Supported").build()).build();
private static final SdkField ENA_SUPPORT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EnaSupport")
.getter(getter(NetworkInfo::enaSupportAsString))
.setter(setter(Builder::enaSupport))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnaSupport")
.unmarshallLocationName("enaSupport").build()).build();
private static final SdkField EFA_SUPPORTED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EfaSupported")
.getter(getter(NetworkInfo::efaSupported))
.setter(setter(Builder::efaSupported))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EfaSupported")
.unmarshallLocationName("efaSupported").build()).build();
private static final SdkField EFA_INFO_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("EfaInfo")
.getter(getter(NetworkInfo::efaInfo))
.setter(setter(Builder::efaInfo))
.constructor(EfaInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EfaInfo")
.unmarshallLocationName("efaInfo").build()).build();
private static final SdkField ENCRYPTION_IN_TRANSIT_SUPPORTED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EncryptionInTransitSupported")
.getter(getter(NetworkInfo::encryptionInTransitSupported))
.setter(setter(Builder::encryptionInTransitSupported))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EncryptionInTransitSupported")
.unmarshallLocationName("encryptionInTransitSupported").build()).build();
private static final SdkField ENA_SRD_SUPPORTED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EnaSrdSupported")
.getter(getter(NetworkInfo::enaSrdSupported))
.setter(setter(Builder::enaSrdSupported))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnaSrdSupported")
.unmarshallLocationName("enaSrdSupported").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NETWORK_PERFORMANCE_FIELD,
MAXIMUM_NETWORK_INTERFACES_FIELD, MAXIMUM_NETWORK_CARDS_FIELD, DEFAULT_NETWORK_CARD_INDEX_FIELD, NETWORK_CARDS_FIELD,
IPV4_ADDRESSES_PER_INTERFACE_FIELD, IPV6_ADDRESSES_PER_INTERFACE_FIELD, IPV6_SUPPORTED_FIELD, ENA_SUPPORT_FIELD,
EFA_SUPPORTED_FIELD, EFA_INFO_FIELD, ENCRYPTION_IN_TRANSIT_SUPPORTED_FIELD, ENA_SRD_SUPPORTED_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("NetworkPerformance", NETWORK_PERFORMANCE_FIELD);
put("MaximumNetworkInterfaces", MAXIMUM_NETWORK_INTERFACES_FIELD);
put("MaximumNetworkCards", MAXIMUM_NETWORK_CARDS_FIELD);
put("DefaultNetworkCardIndex", DEFAULT_NETWORK_CARD_INDEX_FIELD);
put("NetworkCards", NETWORK_CARDS_FIELD);
put("Ipv4AddressesPerInterface", IPV4_ADDRESSES_PER_INTERFACE_FIELD);
put("Ipv6AddressesPerInterface", IPV6_ADDRESSES_PER_INTERFACE_FIELD);
put("Ipv6Supported", IPV6_SUPPORTED_FIELD);
put("EnaSupport", ENA_SUPPORT_FIELD);
put("EfaSupported", EFA_SUPPORTED_FIELD);
put("EfaInfo", EFA_INFO_FIELD);
put("EncryptionInTransitSupported", ENCRYPTION_IN_TRANSIT_SUPPORTED_FIELD);
put("EnaSrdSupported", ENA_SRD_SUPPORTED_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String networkPerformance;
private final Integer maximumNetworkInterfaces;
private final Integer maximumNetworkCards;
private final Integer defaultNetworkCardIndex;
private final List networkCards;
private final Integer ipv4AddressesPerInterface;
private final Integer ipv6AddressesPerInterface;
private final Boolean ipv6Supported;
private final String enaSupport;
private final Boolean efaSupported;
private final EfaInfo efaInfo;
private final Boolean encryptionInTransitSupported;
private final Boolean enaSrdSupported;
private NetworkInfo(BuilderImpl builder) {
this.networkPerformance = builder.networkPerformance;
this.maximumNetworkInterfaces = builder.maximumNetworkInterfaces;
this.maximumNetworkCards = builder.maximumNetworkCards;
this.defaultNetworkCardIndex = builder.defaultNetworkCardIndex;
this.networkCards = builder.networkCards;
this.ipv4AddressesPerInterface = builder.ipv4AddressesPerInterface;
this.ipv6AddressesPerInterface = builder.ipv6AddressesPerInterface;
this.ipv6Supported = builder.ipv6Supported;
this.enaSupport = builder.enaSupport;
this.efaSupported = builder.efaSupported;
this.efaInfo = builder.efaInfo;
this.encryptionInTransitSupported = builder.encryptionInTransitSupported;
this.enaSrdSupported = builder.enaSrdSupported;
}
/**
*
* The network performance.
*
*
* @return The network performance.
*/
public final String networkPerformance() {
return networkPerformance;
}
/**
*
* The maximum number of network interfaces for the instance type.
*
*
* @return The maximum number of network interfaces for the instance type.
*/
public final Integer maximumNetworkInterfaces() {
return maximumNetworkInterfaces;
}
/**
*
* The maximum number of physical network cards that can be allocated to the instance.
*
*
* @return The maximum number of physical network cards that can be allocated to the instance.
*/
public final Integer maximumNetworkCards() {
return maximumNetworkCards;
}
/**
*
* The index of the default network card, starting at 0.
*
*
* @return The index of the default network card, starting at 0.
*/
public final Integer defaultNetworkCardIndex() {
return defaultNetworkCardIndex;
}
/**
* For responses, this returns true if the service returned a value for the NetworkCards property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNetworkCards() {
return networkCards != null && !(networkCards instanceof SdkAutoConstructList);
}
/**
*
* Describes the network cards for the instance type.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNetworkCards} method.
*
*
* @return Describes the network cards for the instance type.
*/
public final List networkCards() {
return networkCards;
}
/**
*
* The maximum number of IPv4 addresses per network interface.
*
*
* @return The maximum number of IPv4 addresses per network interface.
*/
public final Integer ipv4AddressesPerInterface() {
return ipv4AddressesPerInterface;
}
/**
*
* The maximum number of IPv6 addresses per network interface.
*
*
* @return The maximum number of IPv6 addresses per network interface.
*/
public final Integer ipv6AddressesPerInterface() {
return ipv6AddressesPerInterface;
}
/**
*
* Indicates whether IPv6 is supported.
*
*
* @return Indicates whether IPv6 is supported.
*/
public final Boolean ipv6Supported() {
return ipv6Supported;
}
/**
*
* Indicates whether Elastic Network Adapter (ENA) is supported.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #enaSupport} will
* return {@link EnaSupport#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #enaSupportAsString}.
*
*
* @return Indicates whether Elastic Network Adapter (ENA) is supported.
* @see EnaSupport
*/
public final EnaSupport enaSupport() {
return EnaSupport.fromValue(enaSupport);
}
/**
*
* Indicates whether Elastic Network Adapter (ENA) is supported.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #enaSupport} will
* return {@link EnaSupport#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #enaSupportAsString}.
*
*
* @return Indicates whether Elastic Network Adapter (ENA) is supported.
* @see EnaSupport
*/
public final String enaSupportAsString() {
return enaSupport;
}
/**
*
* Indicates whether Elastic Fabric Adapter (EFA) is supported.
*
*
* @return Indicates whether Elastic Fabric Adapter (EFA) is supported.
*/
public final Boolean efaSupported() {
return efaSupported;
}
/**
*
* Describes the Elastic Fabric Adapters for the instance type.
*
*
* @return Describes the Elastic Fabric Adapters for the instance type.
*/
public final EfaInfo efaInfo() {
return efaInfo;
}
/**
*
* Indicates whether the instance type automatically encrypts in-transit traffic between instances.
*
*
* @return Indicates whether the instance type automatically encrypts in-transit traffic between instances.
*/
public final Boolean encryptionInTransitSupported() {
return encryptionInTransitSupported;
}
/**
*
* Indicates whether the instance type supports ENA Express. ENA Express uses Amazon Web Services Scalable Reliable
* Datagram (SRD) technology to increase the maximum bandwidth used per stream and minimize tail latency of network
* traffic between EC2 instances.
*
*
* @return Indicates whether the instance type supports ENA Express. ENA Express uses Amazon Web Services Scalable
* Reliable Datagram (SRD) technology to increase the maximum bandwidth used per stream and minimize tail
* latency of network traffic between EC2 instances.
*/
public final Boolean enaSrdSupported() {
return enaSrdSupported;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(networkPerformance());
hashCode = 31 * hashCode + Objects.hashCode(maximumNetworkInterfaces());
hashCode = 31 * hashCode + Objects.hashCode(maximumNetworkCards());
hashCode = 31 * hashCode + Objects.hashCode(defaultNetworkCardIndex());
hashCode = 31 * hashCode + Objects.hashCode(hasNetworkCards() ? networkCards() : null);
hashCode = 31 * hashCode + Objects.hashCode(ipv4AddressesPerInterface());
hashCode = 31 * hashCode + Objects.hashCode(ipv6AddressesPerInterface());
hashCode = 31 * hashCode + Objects.hashCode(ipv6Supported());
hashCode = 31 * hashCode + Objects.hashCode(enaSupportAsString());
hashCode = 31 * hashCode + Objects.hashCode(efaSupported());
hashCode = 31 * hashCode + Objects.hashCode(efaInfo());
hashCode = 31 * hashCode + Objects.hashCode(encryptionInTransitSupported());
hashCode = 31 * hashCode + Objects.hashCode(enaSrdSupported());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NetworkInfo)) {
return false;
}
NetworkInfo other = (NetworkInfo) obj;
return Objects.equals(networkPerformance(), other.networkPerformance())
&& Objects.equals(maximumNetworkInterfaces(), other.maximumNetworkInterfaces())
&& Objects.equals(maximumNetworkCards(), other.maximumNetworkCards())
&& Objects.equals(defaultNetworkCardIndex(), other.defaultNetworkCardIndex())
&& hasNetworkCards() == other.hasNetworkCards() && Objects.equals(networkCards(), other.networkCards())
&& Objects.equals(ipv4AddressesPerInterface(), other.ipv4AddressesPerInterface())
&& Objects.equals(ipv6AddressesPerInterface(), other.ipv6AddressesPerInterface())
&& Objects.equals(ipv6Supported(), other.ipv6Supported())
&& Objects.equals(enaSupportAsString(), other.enaSupportAsString())
&& Objects.equals(efaSupported(), other.efaSupported()) && Objects.equals(efaInfo(), other.efaInfo())
&& Objects.equals(encryptionInTransitSupported(), other.encryptionInTransitSupported())
&& Objects.equals(enaSrdSupported(), other.enaSrdSupported());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NetworkInfo").add("NetworkPerformance", networkPerformance())
.add("MaximumNetworkInterfaces", maximumNetworkInterfaces()).add("MaximumNetworkCards", maximumNetworkCards())
.add("DefaultNetworkCardIndex", defaultNetworkCardIndex())
.add("NetworkCards", hasNetworkCards() ? networkCards() : null)
.add("Ipv4AddressesPerInterface", ipv4AddressesPerInterface())
.add("Ipv6AddressesPerInterface", ipv6AddressesPerInterface()).add("Ipv6Supported", ipv6Supported())
.add("EnaSupport", enaSupportAsString()).add("EfaSupported", efaSupported()).add("EfaInfo", efaInfo())
.add("EncryptionInTransitSupported", encryptionInTransitSupported()).add("EnaSrdSupported", enaSrdSupported())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "NetworkPerformance":
return Optional.ofNullable(clazz.cast(networkPerformance()));
case "MaximumNetworkInterfaces":
return Optional.ofNullable(clazz.cast(maximumNetworkInterfaces()));
case "MaximumNetworkCards":
return Optional.ofNullable(clazz.cast(maximumNetworkCards()));
case "DefaultNetworkCardIndex":
return Optional.ofNullable(clazz.cast(defaultNetworkCardIndex()));
case "NetworkCards":
return Optional.ofNullable(clazz.cast(networkCards()));
case "Ipv4AddressesPerInterface":
return Optional.ofNullable(clazz.cast(ipv4AddressesPerInterface()));
case "Ipv6AddressesPerInterface":
return Optional.ofNullable(clazz.cast(ipv6AddressesPerInterface()));
case "Ipv6Supported":
return Optional.ofNullable(clazz.cast(ipv6Supported()));
case "EnaSupport":
return Optional.ofNullable(clazz.cast(enaSupportAsString()));
case "EfaSupported":
return Optional.ofNullable(clazz.cast(efaSupported()));
case "EfaInfo":
return Optional.ofNullable(clazz.cast(efaInfo()));
case "EncryptionInTransitSupported":
return Optional.ofNullable(clazz.cast(encryptionInTransitSupported()));
case "EnaSrdSupported":
return Optional.ofNullable(clazz.cast(enaSrdSupported()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function