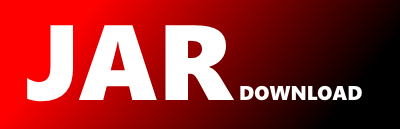
software.amazon.awssdk.services.ec2.model.NetworkInsightsAnalysis Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a network insights analysis.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NetworkInsightsAnalysis implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField NETWORK_INSIGHTS_ANALYSIS_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NetworkInsightsAnalysisId")
.getter(getter(NetworkInsightsAnalysis::networkInsightsAnalysisId))
.setter(setter(Builder::networkInsightsAnalysisId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkInsightsAnalysisId")
.unmarshallLocationName("networkInsightsAnalysisId").build()).build();
private static final SdkField NETWORK_INSIGHTS_ANALYSIS_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NetworkInsightsAnalysisArn")
.getter(getter(NetworkInsightsAnalysis::networkInsightsAnalysisArn))
.setter(setter(Builder::networkInsightsAnalysisArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkInsightsAnalysisArn")
.unmarshallLocationName("networkInsightsAnalysisArn").build()).build();
private static final SdkField NETWORK_INSIGHTS_PATH_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NetworkInsightsPathId")
.getter(getter(NetworkInsightsAnalysis::networkInsightsPathId))
.setter(setter(Builder::networkInsightsPathId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkInsightsPathId")
.unmarshallLocationName("networkInsightsPathId").build()).build();
private static final SdkField> ADDITIONAL_ACCOUNTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AdditionalAccounts")
.getter(getter(NetworkInsightsAnalysis::additionalAccounts))
.setter(setter(Builder::additionalAccounts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdditionalAccountSet")
.unmarshallLocationName("additionalAccountSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> FILTER_IN_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("FilterInArns")
.getter(getter(NetworkInsightsAnalysis::filterInArns))
.setter(setter(Builder::filterInArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FilterInArnSet")
.unmarshallLocationName("filterInArnSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField START_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("StartDate")
.getter(getter(NetworkInsightsAnalysis::startDate))
.setter(setter(Builder::startDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartDate")
.unmarshallLocationName("startDate").build()).build();
private static final SdkField STATUS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Status")
.getter(getter(NetworkInsightsAnalysis::statusAsString))
.setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status")
.unmarshallLocationName("status").build()).build();
private static final SdkField STATUS_MESSAGE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("StatusMessage")
.getter(getter(NetworkInsightsAnalysis::statusMessage))
.setter(setter(Builder::statusMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusMessage")
.unmarshallLocationName("statusMessage").build()).build();
private static final SdkField WARNING_MESSAGE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("WarningMessage")
.getter(getter(NetworkInsightsAnalysis::warningMessage))
.setter(setter(Builder::warningMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WarningMessage")
.unmarshallLocationName("warningMessage").build()).build();
private static final SdkField NETWORK_PATH_FOUND_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("NetworkPathFound")
.getter(getter(NetworkInsightsAnalysis::networkPathFound))
.setter(setter(Builder::networkPathFound))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkPathFound")
.unmarshallLocationName("networkPathFound").build()).build();
private static final SdkField> FORWARD_PATH_COMPONENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ForwardPathComponents")
.getter(getter(NetworkInsightsAnalysis::forwardPathComponents))
.setter(setter(Builder::forwardPathComponents))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ForwardPathComponentSet")
.unmarshallLocationName("forwardPathComponentSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PathComponent::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> RETURN_PATH_COMPONENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ReturnPathComponents")
.getter(getter(NetworkInsightsAnalysis::returnPathComponents))
.setter(setter(Builder::returnPathComponents))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReturnPathComponentSet")
.unmarshallLocationName("returnPathComponentSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PathComponent::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> EXPLANATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Explanations")
.getter(getter(NetworkInsightsAnalysis::explanations))
.setter(setter(Builder::explanations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExplanationSet")
.unmarshallLocationName("explanationSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Explanation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> ALTERNATE_PATH_HINTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AlternatePathHints")
.getter(getter(NetworkInsightsAnalysis::alternatePathHints))
.setter(setter(Builder::alternatePathHints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AlternatePathHintSet")
.unmarshallLocationName("alternatePathHintSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AlternatePathHint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> SUGGESTED_ACCOUNTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SuggestedAccounts")
.getter(getter(NetworkInsightsAnalysis::suggestedAccounts))
.setter(setter(Builder::suggestedAccounts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SuggestedAccountSet")
.unmarshallLocationName("suggestedAccountSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(NetworkInsightsAnalysis::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagSet")
.unmarshallLocationName("tagSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
NETWORK_INSIGHTS_ANALYSIS_ID_FIELD, NETWORK_INSIGHTS_ANALYSIS_ARN_FIELD, NETWORK_INSIGHTS_PATH_ID_FIELD,
ADDITIONAL_ACCOUNTS_FIELD, FILTER_IN_ARNS_FIELD, START_DATE_FIELD, STATUS_FIELD, STATUS_MESSAGE_FIELD,
WARNING_MESSAGE_FIELD, NETWORK_PATH_FOUND_FIELD, FORWARD_PATH_COMPONENTS_FIELD, RETURN_PATH_COMPONENTS_FIELD,
EXPLANATIONS_FIELD, ALTERNATE_PATH_HINTS_FIELD, SUGGESTED_ACCOUNTS_FIELD, TAGS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("NetworkInsightsAnalysisId", NETWORK_INSIGHTS_ANALYSIS_ID_FIELD);
put("NetworkInsightsAnalysisArn", NETWORK_INSIGHTS_ANALYSIS_ARN_FIELD);
put("NetworkInsightsPathId", NETWORK_INSIGHTS_PATH_ID_FIELD);
put("AdditionalAccountSet", ADDITIONAL_ACCOUNTS_FIELD);
put("FilterInArnSet", FILTER_IN_ARNS_FIELD);
put("StartDate", START_DATE_FIELD);
put("Status", STATUS_FIELD);
put("StatusMessage", STATUS_MESSAGE_FIELD);
put("WarningMessage", WARNING_MESSAGE_FIELD);
put("NetworkPathFound", NETWORK_PATH_FOUND_FIELD);
put("ForwardPathComponentSet", FORWARD_PATH_COMPONENTS_FIELD);
put("ReturnPathComponentSet", RETURN_PATH_COMPONENTS_FIELD);
put("ExplanationSet", EXPLANATIONS_FIELD);
put("AlternatePathHintSet", ALTERNATE_PATH_HINTS_FIELD);
put("SuggestedAccountSet", SUGGESTED_ACCOUNTS_FIELD);
put("TagSet", TAGS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String networkInsightsAnalysisId;
private final String networkInsightsAnalysisArn;
private final String networkInsightsPathId;
private final List additionalAccounts;
private final List filterInArns;
private final Instant startDate;
private final String status;
private final String statusMessage;
private final String warningMessage;
private final Boolean networkPathFound;
private final List forwardPathComponents;
private final List returnPathComponents;
private final List explanations;
private final List alternatePathHints;
private final List suggestedAccounts;
private final List tags;
private NetworkInsightsAnalysis(BuilderImpl builder) {
this.networkInsightsAnalysisId = builder.networkInsightsAnalysisId;
this.networkInsightsAnalysisArn = builder.networkInsightsAnalysisArn;
this.networkInsightsPathId = builder.networkInsightsPathId;
this.additionalAccounts = builder.additionalAccounts;
this.filterInArns = builder.filterInArns;
this.startDate = builder.startDate;
this.status = builder.status;
this.statusMessage = builder.statusMessage;
this.warningMessage = builder.warningMessage;
this.networkPathFound = builder.networkPathFound;
this.forwardPathComponents = builder.forwardPathComponents;
this.returnPathComponents = builder.returnPathComponents;
this.explanations = builder.explanations;
this.alternatePathHints = builder.alternatePathHints;
this.suggestedAccounts = builder.suggestedAccounts;
this.tags = builder.tags;
}
/**
*
* The ID of the network insights analysis.
*
*
* @return The ID of the network insights analysis.
*/
public final String networkInsightsAnalysisId() {
return networkInsightsAnalysisId;
}
/**
*
* The Amazon Resource Name (ARN) of the network insights analysis.
*
*
* @return The Amazon Resource Name (ARN) of the network insights analysis.
*/
public final String networkInsightsAnalysisArn() {
return networkInsightsAnalysisArn;
}
/**
*
* The ID of the path.
*
*
* @return The ID of the path.
*/
public final String networkInsightsPathId() {
return networkInsightsPathId;
}
/**
* For responses, this returns true if the service returned a value for the AdditionalAccounts property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAdditionalAccounts() {
return additionalAccounts != null && !(additionalAccounts instanceof SdkAutoConstructList);
}
/**
*
* The member accounts that contain resources that the path can traverse.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAdditionalAccounts} method.
*
*
* @return The member accounts that contain resources that the path can traverse.
*/
public final List additionalAccounts() {
return additionalAccounts;
}
/**
* For responses, this returns true if the service returned a value for the FilterInArns property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasFilterInArns() {
return filterInArns != null && !(filterInArns instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Resource Names (ARN) of the resources that the path must traverse.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasFilterInArns} method.
*
*
* @return The Amazon Resource Names (ARN) of the resources that the path must traverse.
*/
public final List filterInArns() {
return filterInArns;
}
/**
*
* The time the analysis started.
*
*
* @return The time the analysis started.
*/
public final Instant startDate() {
return startDate;
}
/**
*
* The status of the network insights analysis.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link AnalysisStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the network insights analysis.
* @see AnalysisStatus
*/
public final AnalysisStatus status() {
return AnalysisStatus.fromValue(status);
}
/**
*
* The status of the network insights analysis.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link AnalysisStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the network insights analysis.
* @see AnalysisStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The status message, if the status is failed
.
*
*
* @return The status message, if the status is failed
.
*/
public final String statusMessage() {
return statusMessage;
}
/**
*
* The warning message.
*
*
* @return The warning message.
*/
public final String warningMessage() {
return warningMessage;
}
/**
*
* Indicates whether the destination is reachable from the source.
*
*
* @return Indicates whether the destination is reachable from the source.
*/
public final Boolean networkPathFound() {
return networkPathFound;
}
/**
* For responses, this returns true if the service returned a value for the ForwardPathComponents property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasForwardPathComponents() {
return forwardPathComponents != null && !(forwardPathComponents instanceof SdkAutoConstructList);
}
/**
*
* The components in the path from source to destination.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasForwardPathComponents} method.
*
*
* @return The components in the path from source to destination.
*/
public final List forwardPathComponents() {
return forwardPathComponents;
}
/**
* For responses, this returns true if the service returned a value for the ReturnPathComponents property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasReturnPathComponents() {
return returnPathComponents != null && !(returnPathComponents instanceof SdkAutoConstructList);
}
/**
*
* The components in the path from destination to source.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasReturnPathComponents} method.
*
*
* @return The components in the path from destination to source.
*/
public final List returnPathComponents() {
return returnPathComponents;
}
/**
* For responses, this returns true if the service returned a value for the Explanations property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasExplanations() {
return explanations != null && !(explanations instanceof SdkAutoConstructList);
}
/**
*
* The explanations. For more information, see Reachability Analyzer
* explanation codes.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExplanations} method.
*
*
* @return The explanations. For more information, see Reachability Analyzer
* explanation codes.
*/
public final List explanations() {
return explanations;
}
/**
* For responses, this returns true if the service returned a value for the AlternatePathHints property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAlternatePathHints() {
return alternatePathHints != null && !(alternatePathHints instanceof SdkAutoConstructList);
}
/**
*
* Potential intermediate components.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAlternatePathHints} method.
*
*
* @return Potential intermediate components.
*/
public final List alternatePathHints() {
return alternatePathHints;
}
/**
* For responses, this returns true if the service returned a value for the SuggestedAccounts property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSuggestedAccounts() {
return suggestedAccounts != null && !(suggestedAccounts instanceof SdkAutoConstructList);
}
/**
*
* Potential intermediate accounts.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSuggestedAccounts} method.
*
*
* @return Potential intermediate accounts.
*/
public final List suggestedAccounts() {
return suggestedAccounts;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The tags.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The tags.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(networkInsightsAnalysisId());
hashCode = 31 * hashCode + Objects.hashCode(networkInsightsAnalysisArn());
hashCode = 31 * hashCode + Objects.hashCode(networkInsightsPathId());
hashCode = 31 * hashCode + Objects.hashCode(hasAdditionalAccounts() ? additionalAccounts() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasFilterInArns() ? filterInArns() : null);
hashCode = 31 * hashCode + Objects.hashCode(startDate());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusMessage());
hashCode = 31 * hashCode + Objects.hashCode(warningMessage());
hashCode = 31 * hashCode + Objects.hashCode(networkPathFound());
hashCode = 31 * hashCode + Objects.hashCode(hasForwardPathComponents() ? forwardPathComponents() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasReturnPathComponents() ? returnPathComponents() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasExplanations() ? explanations() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasAlternatePathHints() ? alternatePathHints() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSuggestedAccounts() ? suggestedAccounts() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NetworkInsightsAnalysis)) {
return false;
}
NetworkInsightsAnalysis other = (NetworkInsightsAnalysis) obj;
return Objects.equals(networkInsightsAnalysisId(), other.networkInsightsAnalysisId())
&& Objects.equals(networkInsightsAnalysisArn(), other.networkInsightsAnalysisArn())
&& Objects.equals(networkInsightsPathId(), other.networkInsightsPathId())
&& hasAdditionalAccounts() == other.hasAdditionalAccounts()
&& Objects.equals(additionalAccounts(), other.additionalAccounts())
&& hasFilterInArns() == other.hasFilterInArns() && Objects.equals(filterInArns(), other.filterInArns())
&& Objects.equals(startDate(), other.startDate()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(statusMessage(), other.statusMessage())
&& Objects.equals(warningMessage(), other.warningMessage())
&& Objects.equals(networkPathFound(), other.networkPathFound())
&& hasForwardPathComponents() == other.hasForwardPathComponents()
&& Objects.equals(forwardPathComponents(), other.forwardPathComponents())
&& hasReturnPathComponents() == other.hasReturnPathComponents()
&& Objects.equals(returnPathComponents(), other.returnPathComponents())
&& hasExplanations() == other.hasExplanations() && Objects.equals(explanations(), other.explanations())
&& hasAlternatePathHints() == other.hasAlternatePathHints()
&& Objects.equals(alternatePathHints(), other.alternatePathHints())
&& hasSuggestedAccounts() == other.hasSuggestedAccounts()
&& Objects.equals(suggestedAccounts(), other.suggestedAccounts()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NetworkInsightsAnalysis").add("NetworkInsightsAnalysisId", networkInsightsAnalysisId())
.add("NetworkInsightsAnalysisArn", networkInsightsAnalysisArn())
.add("NetworkInsightsPathId", networkInsightsPathId())
.add("AdditionalAccounts", hasAdditionalAccounts() ? additionalAccounts() : null)
.add("FilterInArns", hasFilterInArns() ? filterInArns() : null).add("StartDate", startDate())
.add("Status", statusAsString()).add("StatusMessage", statusMessage()).add("WarningMessage", warningMessage())
.add("NetworkPathFound", networkPathFound())
.add("ForwardPathComponents", hasForwardPathComponents() ? forwardPathComponents() : null)
.add("ReturnPathComponents", hasReturnPathComponents() ? returnPathComponents() : null)
.add("Explanations", hasExplanations() ? explanations() : null)
.add("AlternatePathHints", hasAlternatePathHints() ? alternatePathHints() : null)
.add("SuggestedAccounts", hasSuggestedAccounts() ? suggestedAccounts() : null)
.add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "NetworkInsightsAnalysisId":
return Optional.ofNullable(clazz.cast(networkInsightsAnalysisId()));
case "NetworkInsightsAnalysisArn":
return Optional.ofNullable(clazz.cast(networkInsightsAnalysisArn()));
case "NetworkInsightsPathId":
return Optional.ofNullable(clazz.cast(networkInsightsPathId()));
case "AdditionalAccounts":
return Optional.ofNullable(clazz.cast(additionalAccounts()));
case "FilterInArns":
return Optional.ofNullable(clazz.cast(filterInArns()));
case "StartDate":
return Optional.ofNullable(clazz.cast(startDate()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "StatusMessage":
return Optional.ofNullable(clazz.cast(statusMessage()));
case "WarningMessage":
return Optional.ofNullable(clazz.cast(warningMessage()));
case "NetworkPathFound":
return Optional.ofNullable(clazz.cast(networkPathFound()));
case "ForwardPathComponents":
return Optional.ofNullable(clazz.cast(forwardPathComponents()));
case "ReturnPathComponents":
return Optional.ofNullable(clazz.cast(returnPathComponents()));
case "Explanations":
return Optional.ofNullable(clazz.cast(explanations()));
case "AlternatePathHints":
return Optional.ofNullable(clazz.cast(alternatePathHints()));
case "SuggestedAccounts":
return Optional.ofNullable(clazz.cast(suggestedAccounts()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function