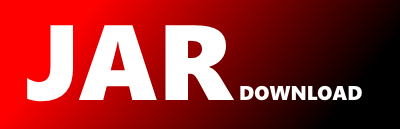
software.amazon.awssdk.services.ec2.model.SnapshotDetail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ec2 Show documentation
Show all versions of ec2 Show documentation
The AWS Java SDK for Amazon EC2 module holds the client classes that are used for communicating with
Amazon EC2 Service
The newest version!
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the snapshot created from the imported disk.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SnapshotDetail implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Description")
.getter(getter(SnapshotDetail::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description")
.unmarshallLocationName("description").build()).build();
private static final SdkField DEVICE_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DeviceName")
.getter(getter(SnapshotDetail::deviceName))
.setter(setter(Builder::deviceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeviceName")
.unmarshallLocationName("deviceName").build()).build();
private static final SdkField DISK_IMAGE_SIZE_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("DiskImageSize")
.getter(getter(SnapshotDetail::diskImageSize))
.setter(setter(Builder::diskImageSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DiskImageSize")
.unmarshallLocationName("diskImageSize").build()).build();
private static final SdkField FORMAT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Format")
.getter(getter(SnapshotDetail::format))
.setter(setter(Builder::format))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Format")
.unmarshallLocationName("format").build()).build();
private static final SdkField PROGRESS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Progress")
.getter(getter(SnapshotDetail::progress))
.setter(setter(Builder::progress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Progress")
.unmarshallLocationName("progress").build()).build();
private static final SdkField SNAPSHOT_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SnapshotId")
.getter(getter(SnapshotDetail::snapshotId))
.setter(setter(Builder::snapshotId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotId")
.unmarshallLocationName("snapshotId").build()).build();
private static final SdkField STATUS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Status")
.getter(getter(SnapshotDetail::status))
.setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status")
.unmarshallLocationName("status").build()).build();
private static final SdkField STATUS_MESSAGE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("StatusMessage")
.getter(getter(SnapshotDetail::statusMessage))
.setter(setter(Builder::statusMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusMessage")
.unmarshallLocationName("statusMessage").build()).build();
private static final SdkField URL_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Url")
.getter(getter(SnapshotDetail::url))
.setter(setter(Builder::url))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Url").unmarshallLocationName("url")
.build()).build();
private static final SdkField USER_BUCKET_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("UserBucket")
.getter(getter(SnapshotDetail::userBucket))
.setter(setter(Builder::userBucket))
.constructor(UserBucketDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserBucket")
.unmarshallLocationName("userBucket").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DESCRIPTION_FIELD,
DEVICE_NAME_FIELD, DISK_IMAGE_SIZE_FIELD, FORMAT_FIELD, PROGRESS_FIELD, SNAPSHOT_ID_FIELD, STATUS_FIELD,
STATUS_MESSAGE_FIELD, URL_FIELD, USER_BUCKET_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("Description", DESCRIPTION_FIELD);
put("DeviceName", DEVICE_NAME_FIELD);
put("DiskImageSize", DISK_IMAGE_SIZE_FIELD);
put("Format", FORMAT_FIELD);
put("Progress", PROGRESS_FIELD);
put("SnapshotId", SNAPSHOT_ID_FIELD);
put("Status", STATUS_FIELD);
put("StatusMessage", STATUS_MESSAGE_FIELD);
put("Url", URL_FIELD);
put("UserBucket", USER_BUCKET_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String description;
private final String deviceName;
private final Double diskImageSize;
private final String format;
private final String progress;
private final String snapshotId;
private final String status;
private final String statusMessage;
private final String url;
private final UserBucketDetails userBucket;
private SnapshotDetail(BuilderImpl builder) {
this.description = builder.description;
this.deviceName = builder.deviceName;
this.diskImageSize = builder.diskImageSize;
this.format = builder.format;
this.progress = builder.progress;
this.snapshotId = builder.snapshotId;
this.status = builder.status;
this.statusMessage = builder.statusMessage;
this.url = builder.url;
this.userBucket = builder.userBucket;
}
/**
*
* A description for the snapshot.
*
*
* @return A description for the snapshot.
*/
public final String description() {
return description;
}
/**
*
* The block device mapping for the snapshot.
*
*
* @return The block device mapping for the snapshot.
*/
public final String deviceName() {
return deviceName;
}
/**
*
* The size of the disk in the snapshot, in GiB.
*
*
* @return The size of the disk in the snapshot, in GiB.
*/
public final Double diskImageSize() {
return diskImageSize;
}
/**
*
* The format of the disk image from which the snapshot is created.
*
*
* @return The format of the disk image from which the snapshot is created.
*/
public final String format() {
return format;
}
/**
*
* The percentage of progress for the task.
*
*
* @return The percentage of progress for the task.
*/
public final String progress() {
return progress;
}
/**
*
* The snapshot ID of the disk being imported.
*
*
* @return The snapshot ID of the disk being imported.
*/
public final String snapshotId() {
return snapshotId;
}
/**
*
* A brief status of the snapshot creation.
*
*
* @return A brief status of the snapshot creation.
*/
public final String status() {
return status;
}
/**
*
* A detailed status message for the snapshot creation.
*
*
* @return A detailed status message for the snapshot creation.
*/
public final String statusMessage() {
return statusMessage;
}
/**
*
* The URL used to access the disk image.
*
*
* @return The URL used to access the disk image.
*/
public final String url() {
return url;
}
/**
*
* The Amazon S3 bucket for the disk image.
*
*
* @return The Amazon S3 bucket for the disk image.
*/
public final UserBucketDetails userBucket() {
return userBucket;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(deviceName());
hashCode = 31 * hashCode + Objects.hashCode(diskImageSize());
hashCode = 31 * hashCode + Objects.hashCode(format());
hashCode = 31 * hashCode + Objects.hashCode(progress());
hashCode = 31 * hashCode + Objects.hashCode(snapshotId());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(statusMessage());
hashCode = 31 * hashCode + Objects.hashCode(url());
hashCode = 31 * hashCode + Objects.hashCode(userBucket());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SnapshotDetail)) {
return false;
}
SnapshotDetail other = (SnapshotDetail) obj;
return Objects.equals(description(), other.description()) && Objects.equals(deviceName(), other.deviceName())
&& Objects.equals(diskImageSize(), other.diskImageSize()) && Objects.equals(format(), other.format())
&& Objects.equals(progress(), other.progress()) && Objects.equals(snapshotId(), other.snapshotId())
&& Objects.equals(status(), other.status()) && Objects.equals(statusMessage(), other.statusMessage())
&& Objects.equals(url(), other.url()) && Objects.equals(userBucket(), other.userBucket());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SnapshotDetail").add("Description", description()).add("DeviceName", deviceName())
.add("DiskImageSize", diskImageSize()).add("Format", format()).add("Progress", progress())
.add("SnapshotId", snapshotId()).add("Status", status()).add("StatusMessage", statusMessage())
.add("Url", url() == null ? null : "*** Sensitive Data Redacted ***").add("UserBucket", userBucket()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "DeviceName":
return Optional.ofNullable(clazz.cast(deviceName()));
case "DiskImageSize":
return Optional.ofNullable(clazz.cast(diskImageSize()));
case "Format":
return Optional.ofNullable(clazz.cast(format()));
case "Progress":
return Optional.ofNullable(clazz.cast(progress()));
case "SnapshotId":
return Optional.ofNullable(clazz.cast(snapshotId()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "StatusMessage":
return Optional.ofNullable(clazz.cast(statusMessage()));
case "Url":
return Optional.ofNullable(clazz.cast(url()));
case "UserBucket":
return Optional.ofNullable(clazz.cast(userBucket()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy