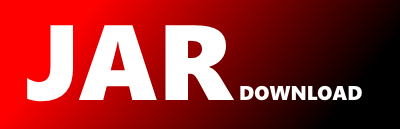
software.amazon.awssdk.services.ec2.model.SpotFleetLaunchSpecification Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the launch specification for one or more Spot Instances. If you include On-Demand capacity in your fleet
* request or want to specify an EFA network device, you can't use SpotFleetLaunchSpecification
; you must
* use LaunchTemplateConfig.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SpotFleetLaunchSpecification implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ADDRESSING_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AddressingType")
.getter(getter(SpotFleetLaunchSpecification::addressingType))
.setter(setter(Builder::addressingType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AddressingType")
.unmarshallLocationName("addressingType").build()).build();
private static final SdkField> BLOCK_DEVICE_MAPPINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("BlockDeviceMappings")
.getter(getter(SpotFleetLaunchSpecification::blockDeviceMappings))
.setter(setter(Builder::blockDeviceMappings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BlockDeviceMapping")
.unmarshallLocationName("blockDeviceMapping").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(BlockDeviceMapping::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField EBS_OPTIMIZED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EbsOptimized")
.getter(getter(SpotFleetLaunchSpecification::ebsOptimized))
.setter(setter(Builder::ebsOptimized))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EbsOptimized")
.unmarshallLocationName("ebsOptimized").build()).build();
private static final SdkField IAM_INSTANCE_PROFILE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("IamInstanceProfile")
.getter(getter(SpotFleetLaunchSpecification::iamInstanceProfile))
.setter(setter(Builder::iamInstanceProfile))
.constructor(IamInstanceProfileSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamInstanceProfile")
.unmarshallLocationName("iamInstanceProfile").build()).build();
private static final SdkField IMAGE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ImageId")
.getter(getter(SpotFleetLaunchSpecification::imageId))
.setter(setter(Builder::imageId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageId")
.unmarshallLocationName("imageId").build()).build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InstanceType")
.getter(getter(SpotFleetLaunchSpecification::instanceTypeAsString))
.setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType")
.unmarshallLocationName("instanceType").build()).build();
private static final SdkField KERNEL_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("KernelId")
.getter(getter(SpotFleetLaunchSpecification::kernelId))
.setter(setter(Builder::kernelId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KernelId")
.unmarshallLocationName("kernelId").build()).build();
private static final SdkField KEY_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("KeyName")
.getter(getter(SpotFleetLaunchSpecification::keyName))
.setter(setter(Builder::keyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeyName")
.unmarshallLocationName("keyName").build()).build();
private static final SdkField MONITORING_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Monitoring")
.getter(getter(SpotFleetLaunchSpecification::monitoring))
.setter(setter(Builder::monitoring))
.constructor(SpotFleetMonitoring::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Monitoring")
.unmarshallLocationName("monitoring").build()).build();
private static final SdkField> NETWORK_INTERFACES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NetworkInterfaces")
.getter(getter(SpotFleetLaunchSpecification::networkInterfaces))
.setter(setter(Builder::networkInterfaces))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkInterfaceSet")
.unmarshallLocationName("networkInterfaceSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(InstanceNetworkInterfaceSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField PLACEMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Placement")
.getter(getter(SpotFleetLaunchSpecification::placement))
.setter(setter(Builder::placement))
.constructor(SpotPlacement::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Placement")
.unmarshallLocationName("placement").build()).build();
private static final SdkField RAMDISK_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RamdiskId")
.getter(getter(SpotFleetLaunchSpecification::ramdiskId))
.setter(setter(Builder::ramdiskId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RamdiskId")
.unmarshallLocationName("ramdiskId").build()).build();
private static final SdkField SPOT_PRICE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SpotPrice")
.getter(getter(SpotFleetLaunchSpecification::spotPrice))
.setter(setter(Builder::spotPrice))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SpotPrice")
.unmarshallLocationName("spotPrice").build()).build();
private static final SdkField SUBNET_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SubnetId")
.getter(getter(SpotFleetLaunchSpecification::subnetId))
.setter(setter(Builder::subnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetId")
.unmarshallLocationName("subnetId").build()).build();
private static final SdkField USER_DATA_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("UserData")
.getter(getter(SpotFleetLaunchSpecification::userData))
.setter(setter(Builder::userData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserData")
.unmarshallLocationName("userData").build()).build();
private static final SdkField WEIGHTED_CAPACITY_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("WeightedCapacity")
.getter(getter(SpotFleetLaunchSpecification::weightedCapacity))
.setter(setter(Builder::weightedCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WeightedCapacity")
.unmarshallLocationName("weightedCapacity").build()).build();
private static final SdkField> TAG_SPECIFICATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TagSpecifications")
.getter(getter(SpotFleetLaunchSpecification::tagSpecifications))
.setter(setter(Builder::tagSpecifications))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagSpecificationSet")
.unmarshallLocationName("tagSpecificationSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SpotFleetTagSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField INSTANCE_REQUIREMENTS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("InstanceRequirements")
.getter(getter(SpotFleetLaunchSpecification::instanceRequirements))
.setter(setter(Builder::instanceRequirements))
.constructor(InstanceRequirements::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceRequirements")
.unmarshallLocationName("instanceRequirements").build()).build();
private static final SdkField> SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SecurityGroups")
.getter(getter(SpotFleetLaunchSpecification::securityGroups))
.setter(setter(Builder::securityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GroupSet")
.unmarshallLocationName("groupSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(GroupIdentifier::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ADDRESSING_TYPE_FIELD,
BLOCK_DEVICE_MAPPINGS_FIELD, EBS_OPTIMIZED_FIELD, IAM_INSTANCE_PROFILE_FIELD, IMAGE_ID_FIELD, INSTANCE_TYPE_FIELD,
KERNEL_ID_FIELD, KEY_NAME_FIELD, MONITORING_FIELD, NETWORK_INTERFACES_FIELD, PLACEMENT_FIELD, RAMDISK_ID_FIELD,
SPOT_PRICE_FIELD, SUBNET_ID_FIELD, USER_DATA_FIELD, WEIGHTED_CAPACITY_FIELD, TAG_SPECIFICATIONS_FIELD,
INSTANCE_REQUIREMENTS_FIELD, SECURITY_GROUPS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("AddressingType", ADDRESSING_TYPE_FIELD);
put("BlockDeviceMapping", BLOCK_DEVICE_MAPPINGS_FIELD);
put("EbsOptimized", EBS_OPTIMIZED_FIELD);
put("IamInstanceProfile", IAM_INSTANCE_PROFILE_FIELD);
put("ImageId", IMAGE_ID_FIELD);
put("InstanceType", INSTANCE_TYPE_FIELD);
put("KernelId", KERNEL_ID_FIELD);
put("KeyName", KEY_NAME_FIELD);
put("Monitoring", MONITORING_FIELD);
put("NetworkInterfaceSet", NETWORK_INTERFACES_FIELD);
put("Placement", PLACEMENT_FIELD);
put("RamdiskId", RAMDISK_ID_FIELD);
put("SpotPrice", SPOT_PRICE_FIELD);
put("SubnetId", SUBNET_ID_FIELD);
put("UserData", USER_DATA_FIELD);
put("WeightedCapacity", WEIGHTED_CAPACITY_FIELD);
put("TagSpecificationSet", TAG_SPECIFICATIONS_FIELD);
put("InstanceRequirements", INSTANCE_REQUIREMENTS_FIELD);
put("GroupSet", SECURITY_GROUPS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String addressingType;
private final List blockDeviceMappings;
private final Boolean ebsOptimized;
private final IamInstanceProfileSpecification iamInstanceProfile;
private final String imageId;
private final String instanceType;
private final String kernelId;
private final String keyName;
private final SpotFleetMonitoring monitoring;
private final List networkInterfaces;
private final SpotPlacement placement;
private final String ramdiskId;
private final String spotPrice;
private final String subnetId;
private final String userData;
private final Double weightedCapacity;
private final List tagSpecifications;
private final InstanceRequirements instanceRequirements;
private final List securityGroups;
private SpotFleetLaunchSpecification(BuilderImpl builder) {
this.addressingType = builder.addressingType;
this.blockDeviceMappings = builder.blockDeviceMappings;
this.ebsOptimized = builder.ebsOptimized;
this.iamInstanceProfile = builder.iamInstanceProfile;
this.imageId = builder.imageId;
this.instanceType = builder.instanceType;
this.kernelId = builder.kernelId;
this.keyName = builder.keyName;
this.monitoring = builder.monitoring;
this.networkInterfaces = builder.networkInterfaces;
this.placement = builder.placement;
this.ramdiskId = builder.ramdiskId;
this.spotPrice = builder.spotPrice;
this.subnetId = builder.subnetId;
this.userData = builder.userData;
this.weightedCapacity = builder.weightedCapacity;
this.tagSpecifications = builder.tagSpecifications;
this.instanceRequirements = builder.instanceRequirements;
this.securityGroups = builder.securityGroups;
}
/**
*
* Deprecated.
*
*
* @return Deprecated.
*/
public final String addressingType() {
return addressingType;
}
/**
* For responses, this returns true if the service returned a value for the BlockDeviceMappings property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasBlockDeviceMappings() {
return blockDeviceMappings != null && !(blockDeviceMappings instanceof SdkAutoConstructList);
}
/**
*
* One or more block devices that are mapped to the Spot Instances. You can't specify both a snapshot ID and an
* encryption value. This is because only blank volumes can be encrypted on creation. If a snapshot is the basis for
* a volume, it is not blank and its encryption status is used for the volume encryption status.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasBlockDeviceMappings} method.
*
*
* @return One or more block devices that are mapped to the Spot Instances. You can't specify both a snapshot ID and
* an encryption value. This is because only blank volumes can be encrypted on creation. If a snapshot is
* the basis for a volume, it is not blank and its encryption status is used for the volume encryption
* status.
*/
public final List blockDeviceMappings() {
return blockDeviceMappings;
}
/**
*
* Indicates whether the instances are optimized for EBS I/O. This optimization provides dedicated throughput to
* Amazon EBS and an optimized configuration stack to provide optimal EBS I/O performance. This optimization isn't
* available with all instance types. Additional usage charges apply when using an EBS Optimized instance.
*
*
* Default: false
*
*
* @return Indicates whether the instances are optimized for EBS I/O. This optimization provides dedicated
* throughput to Amazon EBS and an optimized configuration stack to provide optimal EBS I/O performance.
* This optimization isn't available with all instance types. Additional usage charges apply when using an
* EBS Optimized instance.
*
* Default: false
*/
public final Boolean ebsOptimized() {
return ebsOptimized;
}
/**
*
* The IAM instance profile.
*
*
* @return The IAM instance profile.
*/
public final IamInstanceProfileSpecification iamInstanceProfile() {
return iamInstanceProfile;
}
/**
*
* The ID of the AMI.
*
*
* @return The ID of the AMI.
*/
public final String imageId() {
return imageId;
}
/**
*
* The instance type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #instanceType} will
* return {@link InstanceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #instanceTypeAsString}.
*
*
* @return The instance type.
* @see InstanceType
*/
public final InstanceType instanceType() {
return InstanceType.fromValue(instanceType);
}
/**
*
* The instance type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #instanceType} will
* return {@link InstanceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #instanceTypeAsString}.
*
*
* @return The instance type.
* @see InstanceType
*/
public final String instanceTypeAsString() {
return instanceType;
}
/**
*
* The ID of the kernel.
*
*
* @return The ID of the kernel.
*/
public final String kernelId() {
return kernelId;
}
/**
*
* The name of the key pair.
*
*
* @return The name of the key pair.
*/
public final String keyName() {
return keyName;
}
/**
*
* Enable or disable monitoring for the instances.
*
*
* @return Enable or disable monitoring for the instances.
*/
public final SpotFleetMonitoring monitoring() {
return monitoring;
}
/**
* For responses, this returns true if the service returned a value for the NetworkInterfaces property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNetworkInterfaces() {
return networkInterfaces != null && !(networkInterfaces instanceof SdkAutoConstructList);
}
/**
*
* The network interfaces.
*
*
*
* SpotFleetLaunchSpecification
does not support Elastic Fabric Adapter (EFA). You must use LaunchTemplateConfig instead.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNetworkInterfaces} method.
*
*
* @return The network interfaces.
*
* SpotFleetLaunchSpecification
does not support Elastic Fabric Adapter (EFA). You must use
* LaunchTemplateConfig instead.
*
*/
public final List networkInterfaces() {
return networkInterfaces;
}
/**
*
* The placement information.
*
*
* @return The placement information.
*/
public final SpotPlacement placement() {
return placement;
}
/**
*
* The ID of the RAM disk. Some kernels require additional drivers at launch. Check the kernel requirements for
* information about whether you need to specify a RAM disk. To find kernel requirements, refer to the Amazon Web
* Services Resource Center and search for the kernel ID.
*
*
* @return The ID of the RAM disk. Some kernels require additional drivers at launch. Check the kernel requirements
* for information about whether you need to specify a RAM disk. To find kernel requirements, refer to the
* Amazon Web Services Resource Center and search for the kernel ID.
*/
public final String ramdiskId() {
return ramdiskId;
}
/**
*
* The maximum price per unit hour that you are willing to pay for a Spot Instance. We do not recommend using this
* parameter because it can lead to increased interruptions. If you do not specify this parameter, you will pay the
* current Spot price.
*
*
*
* If you specify a maximum price, your instances will be interrupted more frequently than if you do not specify
* this parameter.
*
*
*
* @return The maximum price per unit hour that you are willing to pay for a Spot Instance. We do not recommend
* using this parameter because it can lead to increased interruptions. If you do not specify this
* parameter, you will pay the current Spot price.
*
* If you specify a maximum price, your instances will be interrupted more frequently than if you do not
* specify this parameter.
*
*/
public final String spotPrice() {
return spotPrice;
}
/**
*
* The IDs of the subnets in which to launch the instances. To specify multiple subnets, separate them using commas;
* for example, "subnet-1234abcdeexample1, subnet-0987cdef6example2".
*
*
* If you specify a network interface, you must specify any subnets as part of the network interface instead of
* using this parameter.
*
*
* @return The IDs of the subnets in which to launch the instances. To specify multiple subnets, separate them using
* commas; for example, "subnet-1234abcdeexample1, subnet-0987cdef6example2".
*
* If you specify a network interface, you must specify any subnets as part of the network interface instead
* of using this parameter.
*/
public final String subnetId() {
return subnetId;
}
/**
*
* The base64-encoded user data that instances use when starting up. User data is limited to 16 KB.
*
*
* @return The base64-encoded user data that instances use when starting up. User data is limited to 16 KB.
*/
public final String userData() {
return userData;
}
/**
*
* The number of units provided by the specified instance type. These are the same units that you chose to set the
* target capacity in terms of instances, or a performance characteristic such as vCPUs, memory, or I/O.
*
*
* If the target capacity divided by this value is not a whole number, Amazon EC2 rounds the number of instances to
* the next whole number. If this value is not specified, the default is 1.
*
*
*
* When specifying weights, the price used in the lowestPrice
and priceCapacityOptimized
* allocation strategies is per unit hour (where the instance price is divided by the specified weight).
* However, if all the specified weights are above the requested TargetCapacity
, resulting in only 1
* instance being launched, the price used is per instance hour.
*
*
*
* @return The number of units provided by the specified instance type. These are the same units that you chose to
* set the target capacity in terms of instances, or a performance characteristic such as vCPUs, memory, or
* I/O.
*
* If the target capacity divided by this value is not a whole number, Amazon EC2 rounds the number of
* instances to the next whole number. If this value is not specified, the default is 1.
*
*
*
* When specifying weights, the price used in the lowestPrice
and
* priceCapacityOptimized
allocation strategies is per unit hour (where the instance
* price is divided by the specified weight). However, if all the specified weights are above the requested
* TargetCapacity
, resulting in only 1 instance being launched, the price used is per
* instance hour.
*
*/
public final Double weightedCapacity() {
return weightedCapacity;
}
/**
* For responses, this returns true if the service returned a value for the TagSpecifications property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTagSpecifications() {
return tagSpecifications != null && !(tagSpecifications instanceof SdkAutoConstructList);
}
/**
*
* The tags to apply during creation.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTagSpecifications} method.
*
*
* @return The tags to apply during creation.
*/
public final List tagSpecifications() {
return tagSpecifications;
}
/**
*
* The attributes for the instance types. When you specify instance attributes, Amazon EC2 will identify instance
* types with those attributes.
*
*
*
* If you specify InstanceRequirements
, you can't specify InstanceType
.
*
*
*
* @return The attributes for the instance types. When you specify instance attributes, Amazon EC2 will identify
* instance types with those attributes.
*
* If you specify InstanceRequirements
, you can't specify InstanceType
.
*
*/
public final InstanceRequirements instanceRequirements() {
return instanceRequirements;
}
/**
* For responses, this returns true if the service returned a value for the SecurityGroups property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecurityGroups() {
return securityGroups != null && !(securityGroups instanceof SdkAutoConstructList);
}
/**
*
* The security groups.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface instead
* of using this parameter.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecurityGroups} method.
*
*
* @return The security groups.
*
* If you specify a network interface, you must specify any security groups as part of the network interface
* instead of using this parameter.
*/
public final List securityGroups() {
return securityGroups;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(addressingType());
hashCode = 31 * hashCode + Objects.hashCode(hasBlockDeviceMappings() ? blockDeviceMappings() : null);
hashCode = 31 * hashCode + Objects.hashCode(ebsOptimized());
hashCode = 31 * hashCode + Objects.hashCode(iamInstanceProfile());
hashCode = 31 * hashCode + Objects.hashCode(imageId());
hashCode = 31 * hashCode + Objects.hashCode(instanceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(kernelId());
hashCode = 31 * hashCode + Objects.hashCode(keyName());
hashCode = 31 * hashCode + Objects.hashCode(monitoring());
hashCode = 31 * hashCode + Objects.hashCode(hasNetworkInterfaces() ? networkInterfaces() : null);
hashCode = 31 * hashCode + Objects.hashCode(placement());
hashCode = 31 * hashCode + Objects.hashCode(ramdiskId());
hashCode = 31 * hashCode + Objects.hashCode(spotPrice());
hashCode = 31 * hashCode + Objects.hashCode(subnetId());
hashCode = 31 * hashCode + Objects.hashCode(userData());
hashCode = 31 * hashCode + Objects.hashCode(weightedCapacity());
hashCode = 31 * hashCode + Objects.hashCode(hasTagSpecifications() ? tagSpecifications() : null);
hashCode = 31 * hashCode + Objects.hashCode(instanceRequirements());
hashCode = 31 * hashCode + Objects.hashCode(hasSecurityGroups() ? securityGroups() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SpotFleetLaunchSpecification)) {
return false;
}
SpotFleetLaunchSpecification other = (SpotFleetLaunchSpecification) obj;
return Objects.equals(addressingType(), other.addressingType())
&& hasBlockDeviceMappings() == other.hasBlockDeviceMappings()
&& Objects.equals(blockDeviceMappings(), other.blockDeviceMappings())
&& Objects.equals(ebsOptimized(), other.ebsOptimized())
&& Objects.equals(iamInstanceProfile(), other.iamInstanceProfile()) && Objects.equals(imageId(), other.imageId())
&& Objects.equals(instanceTypeAsString(), other.instanceTypeAsString())
&& Objects.equals(kernelId(), other.kernelId()) && Objects.equals(keyName(), other.keyName())
&& Objects.equals(monitoring(), other.monitoring()) && hasNetworkInterfaces() == other.hasNetworkInterfaces()
&& Objects.equals(networkInterfaces(), other.networkInterfaces())
&& Objects.equals(placement(), other.placement()) && Objects.equals(ramdiskId(), other.ramdiskId())
&& Objects.equals(spotPrice(), other.spotPrice()) && Objects.equals(subnetId(), other.subnetId())
&& Objects.equals(userData(), other.userData()) && Objects.equals(weightedCapacity(), other.weightedCapacity())
&& hasTagSpecifications() == other.hasTagSpecifications()
&& Objects.equals(tagSpecifications(), other.tagSpecifications())
&& Objects.equals(instanceRequirements(), other.instanceRequirements())
&& hasSecurityGroups() == other.hasSecurityGroups() && Objects.equals(securityGroups(), other.securityGroups());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SpotFleetLaunchSpecification").add("AddressingType", addressingType())
.add("BlockDeviceMappings", hasBlockDeviceMappings() ? blockDeviceMappings() : null)
.add("EbsOptimized", ebsOptimized()).add("IamInstanceProfile", iamInstanceProfile()).add("ImageId", imageId())
.add("InstanceType", instanceTypeAsString()).add("KernelId", kernelId()).add("KeyName", keyName())
.add("Monitoring", monitoring()).add("NetworkInterfaces", hasNetworkInterfaces() ? networkInterfaces() : null)
.add("Placement", placement()).add("RamdiskId", ramdiskId()).add("SpotPrice", spotPrice())
.add("SubnetId", subnetId()).add("UserData", userData() == null ? null : "*** Sensitive Data Redacted ***")
.add("WeightedCapacity", weightedCapacity())
.add("TagSpecifications", hasTagSpecifications() ? tagSpecifications() : null)
.add("InstanceRequirements", instanceRequirements())
.add("SecurityGroups", hasSecurityGroups() ? securityGroups() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AddressingType":
return Optional.ofNullable(clazz.cast(addressingType()));
case "BlockDeviceMappings":
return Optional.ofNullable(clazz.cast(blockDeviceMappings()));
case "EbsOptimized":
return Optional.ofNullable(clazz.cast(ebsOptimized()));
case "IamInstanceProfile":
return Optional.ofNullable(clazz.cast(iamInstanceProfile()));
case "ImageId":
return Optional.ofNullable(clazz.cast(imageId()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceTypeAsString()));
case "KernelId":
return Optional.ofNullable(clazz.cast(kernelId()));
case "KeyName":
return Optional.ofNullable(clazz.cast(keyName()));
case "Monitoring":
return Optional.ofNullable(clazz.cast(monitoring()));
case "NetworkInterfaces":
return Optional.ofNullable(clazz.cast(networkInterfaces()));
case "Placement":
return Optional.ofNullable(clazz.cast(placement()));
case "RamdiskId":
return Optional.ofNullable(clazz.cast(ramdiskId()));
case "SpotPrice":
return Optional.ofNullable(clazz.cast(spotPrice()));
case "SubnetId":
return Optional.ofNullable(clazz.cast(subnetId()));
case "UserData":
return Optional.ofNullable(clazz.cast(userData()));
case "WeightedCapacity":
return Optional.ofNullable(clazz.cast(weightedCapacity()));
case "TagSpecifications":
return Optional.ofNullable(clazz.cast(tagSpecifications()));
case "InstanceRequirements":
return Optional.ofNullable(clazz.cast(instanceRequirements()));
case "SecurityGroups":
return Optional.ofNullable(clazz.cast(securityGroups()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* If the target capacity divided by this value is not a whole number, Amazon EC2 rounds the number of
* instances to the next whole number. If this value is not specified, the default is 1.
*
*
*
* When specifying weights, the price used in the lowestPrice
and
* priceCapacityOptimized
allocation strategies is per unit hour (where the instance
* price is divided by the specified weight). However, if all the specified weights are above the
* requested TargetCapacity
, resulting in only 1 instance being launched, the price used is
* per instance hour.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder weightedCapacity(Double weightedCapacity);
/**
*
* The tags to apply during creation.
*
*
* @param tagSpecifications
* The tags to apply during creation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tagSpecifications(Collection tagSpecifications);
/**
*
* The tags to apply during creation.
*
*
* @param tagSpecifications
* The tags to apply during creation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tagSpecifications(SpotFleetTagSpecification... tagSpecifications);
/**
*
* The tags to apply during creation.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ec2.model.SpotFleetTagSpecification.Builder} avoiding the need to
* create one manually via {@link software.amazon.awssdk.services.ec2.model.SpotFleetTagSpecification#builder()}
* .
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.ec2.model.SpotFleetTagSpecification.Builder#build()} is called
* immediately and its result is passed to {@link #tagSpecifications(List)}.
*
* @param tagSpecifications
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.ec2.model.SpotFleetTagSpecification.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tagSpecifications(java.util.Collection)
*/
Builder tagSpecifications(Consumer... tagSpecifications);
/**
*
* The attributes for the instance types. When you specify instance attributes, Amazon EC2 will identify
* instance types with those attributes.
*
*
*
* If you specify InstanceRequirements
, you can't specify InstanceType
.
*
*
*
* @param instanceRequirements
* The attributes for the instance types. When you specify instance attributes, Amazon EC2 will identify
* instance types with those attributes.
*
* If you specify InstanceRequirements
, you can't specify InstanceType
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder instanceRequirements(InstanceRequirements instanceRequirements);
/**
*
* The attributes for the instance types. When you specify instance attributes, Amazon EC2 will identify
* instance types with those attributes.
*
*
*
* If you specify InstanceRequirements
, you can't specify InstanceType
.
*
* This is a convenience method that creates an instance of the {@link InstanceRequirements.Builder}
* avoiding the need to create one manually via {@link InstanceRequirements#builder()}.
*
*
* When the {@link Consumer} completes, {@link InstanceRequirements.Builder#build()} is called immediately and
* its result is passed to {@link #instanceRequirements(InstanceRequirements)}.
*
* @param instanceRequirements
* a consumer that will call methods on {@link InstanceRequirements.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #instanceRequirements(InstanceRequirements)
*/
default Builder instanceRequirements(Consumer instanceRequirements) {
return instanceRequirements(InstanceRequirements.builder().applyMutation(instanceRequirements).build());
}
/**
*
* The security groups.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface
* instead of using this parameter.
*
*
* @param securityGroups
* The security groups.
*
* If you specify a network interface, you must specify any security groups as part of the network
* interface instead of using this parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder securityGroups(Collection securityGroups);
/**
*
* The security groups.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface
* instead of using this parameter.
*
*
* @param securityGroups
* The security groups.
*
* If you specify a network interface, you must specify any security groups as part of the network
* interface instead of using this parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder securityGroups(GroupIdentifier... securityGroups);
/**
*
* The security groups.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface
* instead of using this parameter.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ec2.model.GroupIdentifier.Builder} avoiding the need to create one
* manually via {@link software.amazon.awssdk.services.ec2.model.GroupIdentifier#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.ec2.model.GroupIdentifier.Builder#build()} is called immediately and
* its result is passed to {@link #securityGroups(List)}.
*
* @param securityGroups
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.ec2.model.GroupIdentifier.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #securityGroups(java.util.Collection)
*/
Builder securityGroups(Consumer... securityGroups);
}
static final class BuilderImpl implements Builder {
private String addressingType;
private List blockDeviceMappings = DefaultSdkAutoConstructList.getInstance();
private Boolean ebsOptimized;
private IamInstanceProfileSpecification iamInstanceProfile;
private String imageId;
private String instanceType;
private String kernelId;
private String keyName;
private SpotFleetMonitoring monitoring;
private List networkInterfaces = DefaultSdkAutoConstructList.getInstance();
private SpotPlacement placement;
private String ramdiskId;
private String spotPrice;
private String subnetId;
private String userData;
private Double weightedCapacity;
private List tagSpecifications = DefaultSdkAutoConstructList.getInstance();
private InstanceRequirements instanceRequirements;
private List securityGroups = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(SpotFleetLaunchSpecification model) {
addressingType(model.addressingType);
blockDeviceMappings(model.blockDeviceMappings);
ebsOptimized(model.ebsOptimized);
iamInstanceProfile(model.iamInstanceProfile);
imageId(model.imageId);
instanceType(model.instanceType);
kernelId(model.kernelId);
keyName(model.keyName);
monitoring(model.monitoring);
networkInterfaces(model.networkInterfaces);
placement(model.placement);
ramdiskId(model.ramdiskId);
spotPrice(model.spotPrice);
subnetId(model.subnetId);
userData(model.userData);
weightedCapacity(model.weightedCapacity);
tagSpecifications(model.tagSpecifications);
instanceRequirements(model.instanceRequirements);
securityGroups(model.securityGroups);
}
public final String getAddressingType() {
return addressingType;
}
public final void setAddressingType(String addressingType) {
this.addressingType = addressingType;
}
@Override
public final Builder addressingType(String addressingType) {
this.addressingType = addressingType;
return this;
}
public final List getBlockDeviceMappings() {
List result = BlockDeviceMappingListCopier.copyToBuilder(this.blockDeviceMappings);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setBlockDeviceMappings(Collection blockDeviceMappings) {
this.blockDeviceMappings = BlockDeviceMappingListCopier.copyFromBuilder(blockDeviceMappings);
}
@Override
public final Builder blockDeviceMappings(Collection blockDeviceMappings) {
this.blockDeviceMappings = BlockDeviceMappingListCopier.copy(blockDeviceMappings);
return this;
}
@Override
@SafeVarargs
public final Builder blockDeviceMappings(BlockDeviceMapping... blockDeviceMappings) {
blockDeviceMappings(Arrays.asList(blockDeviceMappings));
return this;
}
@Override
@SafeVarargs
public final Builder blockDeviceMappings(Consumer... blockDeviceMappings) {
blockDeviceMappings(Stream.of(blockDeviceMappings).map(c -> BlockDeviceMapping.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final Boolean getEbsOptimized() {
return ebsOptimized;
}
public final void setEbsOptimized(Boolean ebsOptimized) {
this.ebsOptimized = ebsOptimized;
}
@Override
public final Builder ebsOptimized(Boolean ebsOptimized) {
this.ebsOptimized = ebsOptimized;
return this;
}
public final IamInstanceProfileSpecification.Builder getIamInstanceProfile() {
return iamInstanceProfile != null ? iamInstanceProfile.toBuilder() : null;
}
public final void setIamInstanceProfile(IamInstanceProfileSpecification.BuilderImpl iamInstanceProfile) {
this.iamInstanceProfile = iamInstanceProfile != null ? iamInstanceProfile.build() : null;
}
@Override
public final Builder iamInstanceProfile(IamInstanceProfileSpecification iamInstanceProfile) {
this.iamInstanceProfile = iamInstanceProfile;
return this;
}
public final String getImageId() {
return imageId;
}
public final void setImageId(String imageId) {
this.imageId = imageId;
}
@Override
public final Builder imageId(String imageId) {
this.imageId = imageId;
return this;
}
public final String getInstanceType() {
return instanceType;
}
public final void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
@Override
public final Builder instanceType(String instanceType) {
this.instanceType = instanceType;
return this;
}
@Override
public final Builder instanceType(InstanceType instanceType) {
this.instanceType(instanceType == null ? null : instanceType.toString());
return this;
}
public final String getKernelId() {
return kernelId;
}
public final void setKernelId(String kernelId) {
this.kernelId = kernelId;
}
@Override
public final Builder kernelId(String kernelId) {
this.kernelId = kernelId;
return this;
}
public final String getKeyName() {
return keyName;
}
public final void setKeyName(String keyName) {
this.keyName = keyName;
}
@Override
public final Builder keyName(String keyName) {
this.keyName = keyName;
return this;
}
public final SpotFleetMonitoring.Builder getMonitoring() {
return monitoring != null ? monitoring.toBuilder() : null;
}
public final void setMonitoring(SpotFleetMonitoring.BuilderImpl monitoring) {
this.monitoring = monitoring != null ? monitoring.build() : null;
}
@Override
public final Builder monitoring(SpotFleetMonitoring monitoring) {
this.monitoring = monitoring;
return this;
}
public final List getNetworkInterfaces() {
List result = InstanceNetworkInterfaceSpecificationListCopier
.copyToBuilder(this.networkInterfaces);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setNetworkInterfaces(Collection networkInterfaces) {
this.networkInterfaces = InstanceNetworkInterfaceSpecificationListCopier.copyFromBuilder(networkInterfaces);
}
@Override
public final Builder networkInterfaces(Collection networkInterfaces) {
this.networkInterfaces = InstanceNetworkInterfaceSpecificationListCopier.copy(networkInterfaces);
return this;
}
@Override
@SafeVarargs
public final Builder networkInterfaces(InstanceNetworkInterfaceSpecification... networkInterfaces) {
networkInterfaces(Arrays.asList(networkInterfaces));
return this;
}
@Override
@SafeVarargs
public final Builder networkInterfaces(Consumer... networkInterfaces) {
networkInterfaces(Stream.of(networkInterfaces)
.map(c -> InstanceNetworkInterfaceSpecification.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final SpotPlacement.Builder getPlacement() {
return placement != null ? placement.toBuilder() : null;
}
public final void setPlacement(SpotPlacement.BuilderImpl placement) {
this.placement = placement != null ? placement.build() : null;
}
@Override
public final Builder placement(SpotPlacement placement) {
this.placement = placement;
return this;
}
public final String getRamdiskId() {
return ramdiskId;
}
public final void setRamdiskId(String ramdiskId) {
this.ramdiskId = ramdiskId;
}
@Override
public final Builder ramdiskId(String ramdiskId) {
this.ramdiskId = ramdiskId;
return this;
}
public final String getSpotPrice() {
return spotPrice;
}
public final void setSpotPrice(String spotPrice) {
this.spotPrice = spotPrice;
}
@Override
public final Builder spotPrice(String spotPrice) {
this.spotPrice = spotPrice;
return this;
}
public final String getSubnetId() {
return subnetId;
}
public final void setSubnetId(String subnetId) {
this.subnetId = subnetId;
}
@Override
public final Builder subnetId(String subnetId) {
this.subnetId = subnetId;
return this;
}
public final String getUserData() {
return userData;
}
public final void setUserData(String userData) {
this.userData = userData;
}
@Override
public final Builder userData(String userData) {
this.userData = userData;
return this;
}
public final Double getWeightedCapacity() {
return weightedCapacity;
}
public final void setWeightedCapacity(Double weightedCapacity) {
this.weightedCapacity = weightedCapacity;
}
@Override
public final Builder weightedCapacity(Double weightedCapacity) {
this.weightedCapacity = weightedCapacity;
return this;
}
public final List getTagSpecifications() {
List result = SpotFleetTagSpecificationListCopier
.copyToBuilder(this.tagSpecifications);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTagSpecifications(Collection tagSpecifications) {
this.tagSpecifications = SpotFleetTagSpecificationListCopier.copyFromBuilder(tagSpecifications);
}
@Override
public final Builder tagSpecifications(Collection tagSpecifications) {
this.tagSpecifications = SpotFleetTagSpecificationListCopier.copy(tagSpecifications);
return this;
}
@Override
@SafeVarargs
public final Builder tagSpecifications(SpotFleetTagSpecification... tagSpecifications) {
tagSpecifications(Arrays.asList(tagSpecifications));
return this;
}
@Override
@SafeVarargs
public final Builder tagSpecifications(Consumer... tagSpecifications) {
tagSpecifications(Stream.of(tagSpecifications).map(c -> SpotFleetTagSpecification.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final InstanceRequirements.Builder getInstanceRequirements() {
return instanceRequirements != null ? instanceRequirements.toBuilder() : null;
}
public final void setInstanceRequirements(InstanceRequirements.BuilderImpl instanceRequirements) {
this.instanceRequirements = instanceRequirements != null ? instanceRequirements.build() : null;
}
@Override
public final Builder instanceRequirements(InstanceRequirements instanceRequirements) {
this.instanceRequirements = instanceRequirements;
return this;
}
public final List getSecurityGroups() {
List result = GroupIdentifierListCopier.copyToBuilder(this.securityGroups);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setSecurityGroups(Collection securityGroups) {
this.securityGroups = GroupIdentifierListCopier.copyFromBuilder(securityGroups);
}
@Override
public final Builder securityGroups(Collection securityGroups) {
this.securityGroups = GroupIdentifierListCopier.copy(securityGroups);
return this;
}
@Override
@SafeVarargs
public final Builder securityGroups(GroupIdentifier... securityGroups) {
securityGroups(Arrays.asList(securityGroups));
return this;
}
@Override
@SafeVarargs
public final Builder securityGroups(Consumer... securityGroups) {
securityGroups(Stream.of(securityGroups).map(c -> GroupIdentifier.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
@Override
public SpotFleetLaunchSpecification build() {
return new SpotFleetLaunchSpecification(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}