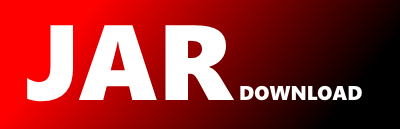
software.amazon.awssdk.services.ec2.model.SpotFleetRequestConfigData Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the configuration of a Spot Fleet request.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SpotFleetRequestConfigData implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ALLOCATION_STRATEGY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AllocationStrategy")
.getter(getter(SpotFleetRequestConfigData::allocationStrategyAsString))
.setter(setter(Builder::allocationStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocationStrategy")
.unmarshallLocationName("allocationStrategy").build()).build();
private static final SdkField ON_DEMAND_ALLOCATION_STRATEGY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OnDemandAllocationStrategy")
.getter(getter(SpotFleetRequestConfigData::onDemandAllocationStrategyAsString))
.setter(setter(Builder::onDemandAllocationStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OnDemandAllocationStrategy")
.unmarshallLocationName("onDemandAllocationStrategy").build()).build();
private static final SdkField SPOT_MAINTENANCE_STRATEGIES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("SpotMaintenanceStrategies")
.getter(getter(SpotFleetRequestConfigData::spotMaintenanceStrategies))
.setter(setter(Builder::spotMaintenanceStrategies))
.constructor(SpotMaintenanceStrategies::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SpotMaintenanceStrategies")
.unmarshallLocationName("spotMaintenanceStrategies").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ClientToken")
.getter(getter(SpotFleetRequestConfigData::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientToken")
.unmarshallLocationName("clientToken").build()).build();
private static final SdkField EXCESS_CAPACITY_TERMINATION_POLICY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ExcessCapacityTerminationPolicy")
.getter(getter(SpotFleetRequestConfigData::excessCapacityTerminationPolicyAsString))
.setter(setter(Builder::excessCapacityTerminationPolicy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExcessCapacityTerminationPolicy")
.unmarshallLocationName("excessCapacityTerminationPolicy").build()).build();
private static final SdkField FULFILLED_CAPACITY_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("FulfilledCapacity")
.getter(getter(SpotFleetRequestConfigData::fulfilledCapacity))
.setter(setter(Builder::fulfilledCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FulfilledCapacity")
.unmarshallLocationName("fulfilledCapacity").build()).build();
private static final SdkField ON_DEMAND_FULFILLED_CAPACITY_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("OnDemandFulfilledCapacity")
.getter(getter(SpotFleetRequestConfigData::onDemandFulfilledCapacity))
.setter(setter(Builder::onDemandFulfilledCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OnDemandFulfilledCapacity")
.unmarshallLocationName("onDemandFulfilledCapacity").build()).build();
private static final SdkField IAM_FLEET_ROLE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("IamFleetRole")
.getter(getter(SpotFleetRequestConfigData::iamFleetRole))
.setter(setter(Builder::iamFleetRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamFleetRole")
.unmarshallLocationName("iamFleetRole").build()).build();
private static final SdkField> LAUNCH_SPECIFICATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LaunchSpecifications")
.getter(getter(SpotFleetRequestConfigData::launchSpecifications))
.setter(setter(Builder::launchSpecifications))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchSpecifications")
.unmarshallLocationName("launchSpecifications").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SpotFleetLaunchSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> LAUNCH_TEMPLATE_CONFIGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LaunchTemplateConfigs")
.getter(getter(SpotFleetRequestConfigData::launchTemplateConfigs))
.setter(setter(Builder::launchTemplateConfigs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchTemplateConfigs")
.unmarshallLocationName("launchTemplateConfigs").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LaunchTemplateConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField SPOT_PRICE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SpotPrice")
.getter(getter(SpotFleetRequestConfigData::spotPrice))
.setter(setter(Builder::spotPrice))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SpotPrice")
.unmarshallLocationName("spotPrice").build()).build();
private static final SdkField TARGET_CAPACITY_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("TargetCapacity")
.getter(getter(SpotFleetRequestConfigData::targetCapacity))
.setter(setter(Builder::targetCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetCapacity")
.unmarshallLocationName("targetCapacity").build()).build();
private static final SdkField ON_DEMAND_TARGET_CAPACITY_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("OnDemandTargetCapacity")
.getter(getter(SpotFleetRequestConfigData::onDemandTargetCapacity))
.setter(setter(Builder::onDemandTargetCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OnDemandTargetCapacity")
.unmarshallLocationName("onDemandTargetCapacity").build()).build();
private static final SdkField ON_DEMAND_MAX_TOTAL_PRICE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OnDemandMaxTotalPrice")
.getter(getter(SpotFleetRequestConfigData::onDemandMaxTotalPrice))
.setter(setter(Builder::onDemandMaxTotalPrice))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OnDemandMaxTotalPrice")
.unmarshallLocationName("onDemandMaxTotalPrice").build()).build();
private static final SdkField SPOT_MAX_TOTAL_PRICE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SpotMaxTotalPrice")
.getter(getter(SpotFleetRequestConfigData::spotMaxTotalPrice))
.setter(setter(Builder::spotMaxTotalPrice))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SpotMaxTotalPrice")
.unmarshallLocationName("spotMaxTotalPrice").build()).build();
private static final SdkField TERMINATE_INSTANCES_WITH_EXPIRATION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("TerminateInstancesWithExpiration")
.getter(getter(SpotFleetRequestConfigData::terminateInstancesWithExpiration))
.setter(setter(Builder::terminateInstancesWithExpiration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TerminateInstancesWithExpiration")
.unmarshallLocationName("terminateInstancesWithExpiration").build()).build();
private static final SdkField TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Type")
.getter(getter(SpotFleetRequestConfigData::typeAsString))
.setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type")
.unmarshallLocationName("type").build()).build();
private static final SdkField VALID_FROM_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("ValidFrom")
.getter(getter(SpotFleetRequestConfigData::validFrom))
.setter(setter(Builder::validFrom))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ValidFrom")
.unmarshallLocationName("validFrom").build()).build();
private static final SdkField VALID_UNTIL_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("ValidUntil")
.getter(getter(SpotFleetRequestConfigData::validUntil))
.setter(setter(Builder::validUntil))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ValidUntil")
.unmarshallLocationName("validUntil").build()).build();
private static final SdkField REPLACE_UNHEALTHY_INSTANCES_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ReplaceUnhealthyInstances")
.getter(getter(SpotFleetRequestConfigData::replaceUnhealthyInstances))
.setter(setter(Builder::replaceUnhealthyInstances))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplaceUnhealthyInstances")
.unmarshallLocationName("replaceUnhealthyInstances").build()).build();
private static final SdkField INSTANCE_INTERRUPTION_BEHAVIOR_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InstanceInterruptionBehavior")
.getter(getter(SpotFleetRequestConfigData::instanceInterruptionBehaviorAsString))
.setter(setter(Builder::instanceInterruptionBehavior))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceInterruptionBehavior")
.unmarshallLocationName("instanceInterruptionBehavior").build()).build();
private static final SdkField LOAD_BALANCERS_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("LoadBalancersConfig")
.getter(getter(SpotFleetRequestConfigData::loadBalancersConfig))
.setter(setter(Builder::loadBalancersConfig))
.constructor(LoadBalancersConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LoadBalancersConfig")
.unmarshallLocationName("loadBalancersConfig").build()).build();
private static final SdkField INSTANCE_POOLS_TO_USE_COUNT_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("InstancePoolsToUseCount")
.getter(getter(SpotFleetRequestConfigData::instancePoolsToUseCount))
.setter(setter(Builder::instancePoolsToUseCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstancePoolsToUseCount")
.unmarshallLocationName("instancePoolsToUseCount").build()).build();
private static final SdkField CONTEXT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Context")
.getter(getter(SpotFleetRequestConfigData::context))
.setter(setter(Builder::context))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Context")
.unmarshallLocationName("context").build()).build();
private static final SdkField TARGET_CAPACITY_UNIT_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("TargetCapacityUnitType")
.getter(getter(SpotFleetRequestConfigData::targetCapacityUnitTypeAsString))
.setter(setter(Builder::targetCapacityUnitType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetCapacityUnitType")
.unmarshallLocationName("targetCapacityUnitType").build()).build();
private static final SdkField> TAG_SPECIFICATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TagSpecifications")
.getter(getter(SpotFleetRequestConfigData::tagSpecifications))
.setter(setter(Builder::tagSpecifications))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagSpecification")
.unmarshallLocationName("TagSpecification").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TagSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ALLOCATION_STRATEGY_FIELD,
ON_DEMAND_ALLOCATION_STRATEGY_FIELD, SPOT_MAINTENANCE_STRATEGIES_FIELD, CLIENT_TOKEN_FIELD,
EXCESS_CAPACITY_TERMINATION_POLICY_FIELD, FULFILLED_CAPACITY_FIELD, ON_DEMAND_FULFILLED_CAPACITY_FIELD,
IAM_FLEET_ROLE_FIELD, LAUNCH_SPECIFICATIONS_FIELD, LAUNCH_TEMPLATE_CONFIGS_FIELD, SPOT_PRICE_FIELD,
TARGET_CAPACITY_FIELD, ON_DEMAND_TARGET_CAPACITY_FIELD, ON_DEMAND_MAX_TOTAL_PRICE_FIELD, SPOT_MAX_TOTAL_PRICE_FIELD,
TERMINATE_INSTANCES_WITH_EXPIRATION_FIELD, TYPE_FIELD, VALID_FROM_FIELD, VALID_UNTIL_FIELD,
REPLACE_UNHEALTHY_INSTANCES_FIELD, INSTANCE_INTERRUPTION_BEHAVIOR_FIELD, LOAD_BALANCERS_CONFIG_FIELD,
INSTANCE_POOLS_TO_USE_COUNT_FIELD, CONTEXT_FIELD, TARGET_CAPACITY_UNIT_TYPE_FIELD, TAG_SPECIFICATIONS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("AllocationStrategy", ALLOCATION_STRATEGY_FIELD);
put("OnDemandAllocationStrategy", ON_DEMAND_ALLOCATION_STRATEGY_FIELD);
put("SpotMaintenanceStrategies", SPOT_MAINTENANCE_STRATEGIES_FIELD);
put("ClientToken", CLIENT_TOKEN_FIELD);
put("ExcessCapacityTerminationPolicy", EXCESS_CAPACITY_TERMINATION_POLICY_FIELD);
put("FulfilledCapacity", FULFILLED_CAPACITY_FIELD);
put("OnDemandFulfilledCapacity", ON_DEMAND_FULFILLED_CAPACITY_FIELD);
put("IamFleetRole", IAM_FLEET_ROLE_FIELD);
put("LaunchSpecifications", LAUNCH_SPECIFICATIONS_FIELD);
put("LaunchTemplateConfigs", LAUNCH_TEMPLATE_CONFIGS_FIELD);
put("SpotPrice", SPOT_PRICE_FIELD);
put("TargetCapacity", TARGET_CAPACITY_FIELD);
put("OnDemandTargetCapacity", ON_DEMAND_TARGET_CAPACITY_FIELD);
put("OnDemandMaxTotalPrice", ON_DEMAND_MAX_TOTAL_PRICE_FIELD);
put("SpotMaxTotalPrice", SPOT_MAX_TOTAL_PRICE_FIELD);
put("TerminateInstancesWithExpiration", TERMINATE_INSTANCES_WITH_EXPIRATION_FIELD);
put("Type", TYPE_FIELD);
put("ValidFrom", VALID_FROM_FIELD);
put("ValidUntil", VALID_UNTIL_FIELD);
put("ReplaceUnhealthyInstances", REPLACE_UNHEALTHY_INSTANCES_FIELD);
put("InstanceInterruptionBehavior", INSTANCE_INTERRUPTION_BEHAVIOR_FIELD);
put("LoadBalancersConfig", LOAD_BALANCERS_CONFIG_FIELD);
put("InstancePoolsToUseCount", INSTANCE_POOLS_TO_USE_COUNT_FIELD);
put("Context", CONTEXT_FIELD);
put("TargetCapacityUnitType", TARGET_CAPACITY_UNIT_TYPE_FIELD);
put("TagSpecification", TAG_SPECIFICATIONS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String allocationStrategy;
private final String onDemandAllocationStrategy;
private final SpotMaintenanceStrategies spotMaintenanceStrategies;
private final String clientToken;
private final String excessCapacityTerminationPolicy;
private final Double fulfilledCapacity;
private final Double onDemandFulfilledCapacity;
private final String iamFleetRole;
private final List launchSpecifications;
private final List launchTemplateConfigs;
private final String spotPrice;
private final Integer targetCapacity;
private final Integer onDemandTargetCapacity;
private final String onDemandMaxTotalPrice;
private final String spotMaxTotalPrice;
private final Boolean terminateInstancesWithExpiration;
private final String type;
private final Instant validFrom;
private final Instant validUntil;
private final Boolean replaceUnhealthyInstances;
private final String instanceInterruptionBehavior;
private final LoadBalancersConfig loadBalancersConfig;
private final Integer instancePoolsToUseCount;
private final String context;
private final String targetCapacityUnitType;
private final List tagSpecifications;
private SpotFleetRequestConfigData(BuilderImpl builder) {
this.allocationStrategy = builder.allocationStrategy;
this.onDemandAllocationStrategy = builder.onDemandAllocationStrategy;
this.spotMaintenanceStrategies = builder.spotMaintenanceStrategies;
this.clientToken = builder.clientToken;
this.excessCapacityTerminationPolicy = builder.excessCapacityTerminationPolicy;
this.fulfilledCapacity = builder.fulfilledCapacity;
this.onDemandFulfilledCapacity = builder.onDemandFulfilledCapacity;
this.iamFleetRole = builder.iamFleetRole;
this.launchSpecifications = builder.launchSpecifications;
this.launchTemplateConfigs = builder.launchTemplateConfigs;
this.spotPrice = builder.spotPrice;
this.targetCapacity = builder.targetCapacity;
this.onDemandTargetCapacity = builder.onDemandTargetCapacity;
this.onDemandMaxTotalPrice = builder.onDemandMaxTotalPrice;
this.spotMaxTotalPrice = builder.spotMaxTotalPrice;
this.terminateInstancesWithExpiration = builder.terminateInstancesWithExpiration;
this.type = builder.type;
this.validFrom = builder.validFrom;
this.validUntil = builder.validUntil;
this.replaceUnhealthyInstances = builder.replaceUnhealthyInstances;
this.instanceInterruptionBehavior = builder.instanceInterruptionBehavior;
this.loadBalancersConfig = builder.loadBalancersConfig;
this.instancePoolsToUseCount = builder.instancePoolsToUseCount;
this.context = builder.context;
this.targetCapacityUnitType = builder.targetCapacityUnitType;
this.tagSpecifications = builder.tagSpecifications;
}
/**
*
* The strategy that determines how to allocate the target Spot Instance capacity across the Spot Instance pools
* specified by the Spot Fleet launch configuration. For more information, see Allocation
* strategies for Spot Instances in the Amazon EC2 User Guide.
*
*
* - priceCapacityOptimized (recommended)
* -
*
* Spot Fleet identifies the pools with the highest capacity availability for the number of instances that are
* launching. This means that we will request Spot Instances from the pools that we believe have the lowest chance
* of interruption in the near term. Spot Fleet then requests Spot Instances from the lowest priced of these pools.
*
*
* - capacityOptimized
* -
*
* Spot Fleet identifies the pools with the highest capacity availability for the number of instances that are
* launching. This means that we will request Spot Instances from the pools that we believe have the lowest chance
* of interruption in the near term. To give certain instance types a higher chance of launching first, use
* capacityOptimizedPrioritized
. Set a priority for each instance type by using the
* Priority
parameter for LaunchTemplateOverrides
. You can assign the same priority to
* different LaunchTemplateOverrides
. EC2 implements the priorities on a best-effort basis, but
* optimizes for capacity first. capacityOptimizedPrioritized
is supported only if your Spot Fleet uses
* a launch template. Note that if the OnDemandAllocationStrategy
is set to prioritized
,
* the same priority is applied when fulfilling On-Demand capacity.
*
*
* - diversified
* -
*
* Spot Fleet requests instances from all of the Spot Instance pools that you specify.
*
*
* - lowestPrice (not recommended)
*
*
* We don't recommend the lowestPrice
allocation strategy because it has the highest risk of
* interruption for your Spot Instances.
*
*
*
* Spot Fleet requests instances from the lowest priced Spot Instance pool that has available capacity. If the
* lowest priced pool doesn't have available capacity, the Spot Instances come from the next lowest priced pool that
* has available capacity. If a pool runs out of capacity before fulfilling your desired capacity, Spot Fleet will
* continue to fulfill your request by drawing from the next lowest priced pool. To ensure that your desired
* capacity is met, you might receive Spot Instances from several pools. Because this strategy only considers
* instance price and not capacity availability, it might lead to high interruption rates.
*
*
*
*
* Default: lowestPrice
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #allocationStrategy} will return {@link AllocationStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #allocationStrategyAsString}.
*
*
* @return The strategy that determines how to allocate the target Spot Instance capacity across the Spot Instance
* pools specified by the Spot Fleet launch configuration. For more information, see Allocation
* strategies for Spot Instances in the Amazon EC2 User Guide.
*
* - priceCapacityOptimized (recommended)
* -
*
* Spot Fleet identifies the pools with the highest capacity availability for the number of instances that
* are launching. This means that we will request Spot Instances from the pools that we believe have the
* lowest chance of interruption in the near term. Spot Fleet then requests Spot Instances from the lowest
* priced of these pools.
*
*
* - capacityOptimized
* -
*
* Spot Fleet identifies the pools with the highest capacity availability for the number of instances that
* are launching. This means that we will request Spot Instances from the pools that we believe have the
* lowest chance of interruption in the near term. To give certain instance types a higher chance of
* launching first, use capacityOptimizedPrioritized
. Set a priority for each instance type by
* using the Priority
parameter for LaunchTemplateOverrides
. You can assign the
* same priority to different LaunchTemplateOverrides
. EC2 implements the priorities on a
* best-effort basis, but optimizes for capacity first. capacityOptimizedPrioritized
is
* supported only if your Spot Fleet uses a launch template. Note that if the
* OnDemandAllocationStrategy
is set to prioritized
, the same priority is applied
* when fulfilling On-Demand capacity.
*
*
* - diversified
* -
*
* Spot Fleet requests instances from all of the Spot Instance pools that you specify.
*
*
* - lowestPrice (not recommended)
*
*
* We don't recommend the lowestPrice
allocation strategy because it has the highest risk of
* interruption for your Spot Instances.
*
*
*
* Spot Fleet requests instances from the lowest priced Spot Instance pool that has available capacity. If
* the lowest priced pool doesn't have available capacity, the Spot Instances come from the next lowest
* priced pool that has available capacity. If a pool runs out of capacity before fulfilling your desired
* capacity, Spot Fleet will continue to fulfill your request by drawing from the next lowest priced pool.
* To ensure that your desired capacity is met, you might receive Spot Instances from several pools. Because
* this strategy only considers instance price and not capacity availability, it might lead to high
* interruption rates.
*
*
*
*
* Default: lowestPrice
* @see AllocationStrategy
*/
public final AllocationStrategy allocationStrategy() {
return AllocationStrategy.fromValue(allocationStrategy);
}
/**
*
* The strategy that determines how to allocate the target Spot Instance capacity across the Spot Instance pools
* specified by the Spot Fleet launch configuration. For more information, see Allocation
* strategies for Spot Instances in the Amazon EC2 User Guide.
*
*
* - priceCapacityOptimized (recommended)
* -
*
* Spot Fleet identifies the pools with the highest capacity availability for the number of instances that are
* launching. This means that we will request Spot Instances from the pools that we believe have the lowest chance
* of interruption in the near term. Spot Fleet then requests Spot Instances from the lowest priced of these pools.
*
*
* - capacityOptimized
* -
*
* Spot Fleet identifies the pools with the highest capacity availability for the number of instances that are
* launching. This means that we will request Spot Instances from the pools that we believe have the lowest chance
* of interruption in the near term. To give certain instance types a higher chance of launching first, use
* capacityOptimizedPrioritized
. Set a priority for each instance type by using the
* Priority
parameter for LaunchTemplateOverrides
. You can assign the same priority to
* different LaunchTemplateOverrides
. EC2 implements the priorities on a best-effort basis, but
* optimizes for capacity first. capacityOptimizedPrioritized
is supported only if your Spot Fleet uses
* a launch template. Note that if the OnDemandAllocationStrategy
is set to prioritized
,
* the same priority is applied when fulfilling On-Demand capacity.
*
*
* - diversified
* -
*
* Spot Fleet requests instances from all of the Spot Instance pools that you specify.
*
*
* - lowestPrice (not recommended)
*
*
* We don't recommend the lowestPrice
allocation strategy because it has the highest risk of
* interruption for your Spot Instances.
*
*
*
* Spot Fleet requests instances from the lowest priced Spot Instance pool that has available capacity. If the
* lowest priced pool doesn't have available capacity, the Spot Instances come from the next lowest priced pool that
* has available capacity. If a pool runs out of capacity before fulfilling your desired capacity, Spot Fleet will
* continue to fulfill your request by drawing from the next lowest priced pool. To ensure that your desired
* capacity is met, you might receive Spot Instances from several pools. Because this strategy only considers
* instance price and not capacity availability, it might lead to high interruption rates.
*
*
*
*
* Default: lowestPrice
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #allocationStrategy} will return {@link AllocationStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #allocationStrategyAsString}.
*
*
* @return The strategy that determines how to allocate the target Spot Instance capacity across the Spot Instance
* pools specified by the Spot Fleet launch configuration. For more information, see Allocation
* strategies for Spot Instances in the Amazon EC2 User Guide.
*
* - priceCapacityOptimized (recommended)
* -
*
* Spot Fleet identifies the pools with the highest capacity availability for the number of instances that
* are launching. This means that we will request Spot Instances from the pools that we believe have the
* lowest chance of interruption in the near term. Spot Fleet then requests Spot Instances from the lowest
* priced of these pools.
*
*
* - capacityOptimized
* -
*
* Spot Fleet identifies the pools with the highest capacity availability for the number of instances that
* are launching. This means that we will request Spot Instances from the pools that we believe have the
* lowest chance of interruption in the near term. To give certain instance types a higher chance of
* launching first, use capacityOptimizedPrioritized
. Set a priority for each instance type by
* using the Priority
parameter for LaunchTemplateOverrides
. You can assign the
* same priority to different LaunchTemplateOverrides
. EC2 implements the priorities on a
* best-effort basis, but optimizes for capacity first. capacityOptimizedPrioritized
is
* supported only if your Spot Fleet uses a launch template. Note that if the
* OnDemandAllocationStrategy
is set to prioritized
, the same priority is applied
* when fulfilling On-Demand capacity.
*
*
* - diversified
* -
*
* Spot Fleet requests instances from all of the Spot Instance pools that you specify.
*
*
* - lowestPrice (not recommended)
*
*
* We don't recommend the lowestPrice
allocation strategy because it has the highest risk of
* interruption for your Spot Instances.
*
*
*
* Spot Fleet requests instances from the lowest priced Spot Instance pool that has available capacity. If
* the lowest priced pool doesn't have available capacity, the Spot Instances come from the next lowest
* priced pool that has available capacity. If a pool runs out of capacity before fulfilling your desired
* capacity, Spot Fleet will continue to fulfill your request by drawing from the next lowest priced pool.
* To ensure that your desired capacity is met, you might receive Spot Instances from several pools. Because
* this strategy only considers instance price and not capacity availability, it might lead to high
* interruption rates.
*
*
*
*
* Default: lowestPrice
* @see AllocationStrategy
*/
public final String allocationStrategyAsString() {
return allocationStrategy;
}
/**
*
* The order of the launch template overrides to use in fulfilling On-Demand capacity. If you specify
* lowestPrice
, Spot Fleet uses price to determine the order, launching the lowest price first. If you
* specify prioritized
, Spot Fleet uses the priority that you assign to each Spot Fleet launch template
* override, launching the highest priority first. If you do not specify a value, Spot Fleet defaults to
* lowestPrice
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #onDemandAllocationStrategy} will return {@link OnDemandAllocationStrategy#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #onDemandAllocationStrategyAsString}.
*
*
* @return The order of the launch template overrides to use in fulfilling On-Demand capacity. If you specify
* lowestPrice
, Spot Fleet uses price to determine the order, launching the lowest price first.
* If you specify prioritized
, Spot Fleet uses the priority that you assign to each Spot Fleet
* launch template override, launching the highest priority first. If you do not specify a value, Spot Fleet
* defaults to lowestPrice
.
* @see OnDemandAllocationStrategy
*/
public final OnDemandAllocationStrategy onDemandAllocationStrategy() {
return OnDemandAllocationStrategy.fromValue(onDemandAllocationStrategy);
}
/**
*
* The order of the launch template overrides to use in fulfilling On-Demand capacity. If you specify
* lowestPrice
, Spot Fleet uses price to determine the order, launching the lowest price first. If you
* specify prioritized
, Spot Fleet uses the priority that you assign to each Spot Fleet launch template
* override, launching the highest priority first. If you do not specify a value, Spot Fleet defaults to
* lowestPrice
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #onDemandAllocationStrategy} will return {@link OnDemandAllocationStrategy#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #onDemandAllocationStrategyAsString}.
*
*
* @return The order of the launch template overrides to use in fulfilling On-Demand capacity. If you specify
* lowestPrice
, Spot Fleet uses price to determine the order, launching the lowest price first.
* If you specify prioritized
, Spot Fleet uses the priority that you assign to each Spot Fleet
* launch template override, launching the highest priority first. If you do not specify a value, Spot Fleet
* defaults to lowestPrice
.
* @see OnDemandAllocationStrategy
*/
public final String onDemandAllocationStrategyAsString() {
return onDemandAllocationStrategy;
}
/**
*
* The strategies for managing your Spot Instances that are at an elevated risk of being interrupted.
*
*
* @return The strategies for managing your Spot Instances that are at an elevated risk of being interrupted.
*/
public final SpotMaintenanceStrategies spotMaintenanceStrategies() {
return spotMaintenanceStrategies;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of your listings. This helps to
* avoid duplicate listings. For more information, see Ensuring
* Idempotency.
*
*
* @return A unique, case-sensitive identifier that you provide to ensure the idempotency of your listings. This
* helps to avoid duplicate listings. For more information, see Ensuring
* Idempotency.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* Indicates whether running instances should be terminated if you decrease the target capacity of the Spot Fleet
* request below the current size of the Spot Fleet.
*
*
* Supported only for fleets of type maintain
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #excessCapacityTerminationPolicy} will return
* {@link ExcessCapacityTerminationPolicy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #excessCapacityTerminationPolicyAsString}.
*
*
* @return Indicates whether running instances should be terminated if you decrease the target capacity of the Spot
* Fleet request below the current size of the Spot Fleet.
*
* Supported only for fleets of type maintain
.
* @see ExcessCapacityTerminationPolicy
*/
public final ExcessCapacityTerminationPolicy excessCapacityTerminationPolicy() {
return ExcessCapacityTerminationPolicy.fromValue(excessCapacityTerminationPolicy);
}
/**
*
* Indicates whether running instances should be terminated if you decrease the target capacity of the Spot Fleet
* request below the current size of the Spot Fleet.
*
*
* Supported only for fleets of type maintain
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #excessCapacityTerminationPolicy} will return
* {@link ExcessCapacityTerminationPolicy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #excessCapacityTerminationPolicyAsString}.
*
*
* @return Indicates whether running instances should be terminated if you decrease the target capacity of the Spot
* Fleet request below the current size of the Spot Fleet.
*
* Supported only for fleets of type maintain
.
* @see ExcessCapacityTerminationPolicy
*/
public final String excessCapacityTerminationPolicyAsString() {
return excessCapacityTerminationPolicy;
}
/**
*
* The number of units fulfilled by this request compared to the set target capacity. You cannot set this value.
*
*
* @return The number of units fulfilled by this request compared to the set target capacity. You cannot set this
* value.
*/
public final Double fulfilledCapacity() {
return fulfilledCapacity;
}
/**
*
* The number of On-Demand units fulfilled by this request compared to the set target On-Demand capacity.
*
*
* @return The number of On-Demand units fulfilled by this request compared to the set target On-Demand capacity.
*/
public final Double onDemandFulfilledCapacity() {
return onDemandFulfilledCapacity;
}
/**
*
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that grants the Spot Fleet the
* permission to request, launch, terminate, and tag instances on your behalf. For more information, see Spot
* Fleet prerequisites in the Amazon EC2 User Guide. Spot Fleet can terminate Spot Instances on your
* behalf when you cancel its Spot Fleet request using CancelSpotFleetRequests or when the Spot Fleet request expires, if you set
* TerminateInstancesWithExpiration
.
*
*
* @return The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that grants the Spot Fleet
* the permission to request, launch, terminate, and tag instances on your behalf. For more information, see
* Spot Fleet prerequisites in the Amazon EC2 User Guide. Spot Fleet can terminate Spot
* Instances on your behalf when you cancel its Spot Fleet request using CancelSpotFleetRequests or when the Spot Fleet request expires, if you set
* TerminateInstancesWithExpiration
.
*/
public final String iamFleetRole() {
return iamFleetRole;
}
/**
* For responses, this returns true if the service returned a value for the LaunchSpecifications property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLaunchSpecifications() {
return launchSpecifications != null && !(launchSpecifications instanceof SdkAutoConstructList);
}
/**
*
* The launch specifications for the Spot Fleet request. If you specify LaunchSpecifications
, you can't
* specify LaunchTemplateConfigs
. If you include On-Demand capacity in your request, you must use
* LaunchTemplateConfigs
.
*
*
*
* If an AMI specified in a launch specification is deregistered or disabled, no new instances can be launched from
* the AMI. For fleets of type maintain
, the target capacity will not be maintained.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLaunchSpecifications} method.
*
*
* @return The launch specifications for the Spot Fleet request. If you specify LaunchSpecifications
,
* you can't specify LaunchTemplateConfigs
. If you include On-Demand capacity in your request,
* you must use LaunchTemplateConfigs
.
*
* If an AMI specified in a launch specification is deregistered or disabled, no new instances can be
* launched from the AMI. For fleets of type maintain
, the target capacity will not be
* maintained.
*
*/
public final List launchSpecifications() {
return launchSpecifications;
}
/**
* For responses, this returns true if the service returned a value for the LaunchTemplateConfigs property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasLaunchTemplateConfigs() {
return launchTemplateConfigs != null && !(launchTemplateConfigs instanceof SdkAutoConstructList);
}
/**
*
* The launch template and overrides. If you specify LaunchTemplateConfigs
, you can't specify
* LaunchSpecifications
. If you include On-Demand capacity in your request, you must use
* LaunchTemplateConfigs
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLaunchTemplateConfigs} method.
*
*
* @return The launch template and overrides. If you specify LaunchTemplateConfigs
, you can't specify
* LaunchSpecifications
. If you include On-Demand capacity in your request, you must use
* LaunchTemplateConfigs
.
*/
public final List launchTemplateConfigs() {
return launchTemplateConfigs;
}
/**
*
* The maximum price per unit hour that you are willing to pay for a Spot Instance. We do not recommend using this
* parameter because it can lead to increased interruptions. If you do not specify this parameter, you will pay the
* current Spot price.
*
*
*
* If you specify a maximum price, your instances will be interrupted more frequently than if you do not specify
* this parameter.
*
*
*
* @return The maximum price per unit hour that you are willing to pay for a Spot Instance. We do not recommend
* using this parameter because it can lead to increased interruptions. If you do not specify this
* parameter, you will pay the current Spot price.
*
* If you specify a maximum price, your instances will be interrupted more frequently than if you do not
* specify this parameter.
*
*/
public final String spotPrice() {
return spotPrice;
}
/**
*
* The number of units to request for the Spot Fleet. You can choose to set the target capacity in terms of
* instances or a performance characteristic that is important to your application workload, such as vCPUs, memory,
* or I/O. If the request type is maintain
, you can specify a target capacity of 0 and add capacity
* later.
*
*
* @return The number of units to request for the Spot Fleet. You can choose to set the target capacity in terms of
* instances or a performance characteristic that is important to your application workload, such as vCPUs,
* memory, or I/O. If the request type is maintain
, you can specify a target capacity of 0 and
* add capacity later.
*/
public final Integer targetCapacity() {
return targetCapacity;
}
/**
*
* The number of On-Demand units to request. You can choose to set the target capacity in terms of instances or a
* performance characteristic that is important to your application workload, such as vCPUs, memory, or I/O. If the
* request type is maintain
, you can specify a target capacity of 0 and add capacity later.
*
*
* @return The number of On-Demand units to request. You can choose to set the target capacity in terms of instances
* or a performance characteristic that is important to your application workload, such as vCPUs, memory, or
* I/O. If the request type is maintain
, you can specify a target capacity of 0 and add
* capacity later.
*/
public final Integer onDemandTargetCapacity() {
return onDemandTargetCapacity;
}
/**
*
* The maximum amount per hour for On-Demand Instances that you're willing to pay. You can use the
* onDemandMaxTotalPrice
parameter, the spotMaxTotalPrice
parameter, or both parameters to
* ensure that your fleet cost does not exceed your budget. If you set a maximum price per hour for the On-Demand
* Instances and Spot Instances in your request, Spot Fleet will launch instances until it reaches the maximum
* amount you're willing to pay. When the maximum amount you're willing to pay is reached, the fleet stops launching
* instances even if it hasn’t met the target capacity.
*
*
*
* If your fleet includes T instances that are configured as unlimited
, and if their average CPU usage
* exceeds the baseline utilization, you will incur a charge for surplus credits. The
* onDemandMaxTotalPrice
does not account for surplus credits, and, if you use surplus credits, your
* final cost might be higher than what you specified for onDemandMaxTotalPrice
. For more information,
* see Surplus credits can incur charges in the Amazon EC2 User Guide.
*
*
*
* @return The maximum amount per hour for On-Demand Instances that you're willing to pay. You can use the
* onDemandMaxTotalPrice
parameter, the spotMaxTotalPrice
parameter, or both
* parameters to ensure that your fleet cost does not exceed your budget. If you set a maximum price per
* hour for the On-Demand Instances and Spot Instances in your request, Spot Fleet will launch instances
* until it reaches the maximum amount you're willing to pay. When the maximum amount you're willing to pay
* is reached, the fleet stops launching instances even if it hasn’t met the target capacity.
*
* If your fleet includes T instances that are configured as unlimited
, and if their average
* CPU usage exceeds the baseline utilization, you will incur a charge for surplus credits. The
* onDemandMaxTotalPrice
does not account for surplus credits, and, if you use surplus credits,
* your final cost might be higher than what you specified for onDemandMaxTotalPrice
. For more
* information, see Surplus credits can incur charges in the Amazon EC2 User Guide.
*
*/
public final String onDemandMaxTotalPrice() {
return onDemandMaxTotalPrice;
}
/**
*
* The maximum amount per hour for Spot Instances that you're willing to pay. You can use the
* spotMaxTotalPrice
parameter, the onDemandMaxTotalPrice
parameter, or both parameters to
* ensure that your fleet cost does not exceed your budget. If you set a maximum price per hour for the On-Demand
* Instances and Spot Instances in your request, Spot Fleet will launch instances until it reaches the maximum
* amount you're willing to pay. When the maximum amount you're willing to pay is reached, the fleet stops launching
* instances even if it hasn’t met the target capacity.
*
*
*
* If your fleet includes T instances that are configured as unlimited
, and if their average CPU usage
* exceeds the baseline utilization, you will incur a charge for surplus credits. The spotMaxTotalPrice
* does not account for surplus credits, and, if you use surplus credits, your final cost might be higher than what
* you specified for spotMaxTotalPrice
. For more information, see Surplus credits can incur charges in the Amazon EC2 User Guide.
*
*
*
* @return The maximum amount per hour for Spot Instances that you're willing to pay. You can use the
* spotMaxTotalPrice
parameter, the onDemandMaxTotalPrice
parameter, or both
* parameters to ensure that your fleet cost does not exceed your budget. If you set a maximum price per
* hour for the On-Demand Instances and Spot Instances in your request, Spot Fleet will launch instances
* until it reaches the maximum amount you're willing to pay. When the maximum amount you're willing to pay
* is reached, the fleet stops launching instances even if it hasn’t met the target capacity.
*
* If your fleet includes T instances that are configured as unlimited
, and if their average
* CPU usage exceeds the baseline utilization, you will incur a charge for surplus credits. The
* spotMaxTotalPrice
does not account for surplus credits, and, if you use surplus credits,
* your final cost might be higher than what you specified for spotMaxTotalPrice
. For more
* information, see Surplus credits can incur charges in the Amazon EC2 User Guide.
*
*/
public final String spotMaxTotalPrice() {
return spotMaxTotalPrice;
}
/**
*
* Indicates whether running Spot Instances are terminated when the Spot Fleet request expires.
*
*
* @return Indicates whether running Spot Instances are terminated when the Spot Fleet request expires.
*/
public final Boolean terminateInstancesWithExpiration() {
return terminateInstancesWithExpiration;
}
/**
*
* The type of request. Indicates whether the Spot Fleet only requests the target capacity or also attempts to
* maintain it. When this value is request
, the Spot Fleet only places the required requests. It does
* not attempt to replenish Spot Instances if capacity is diminished, nor does it submit requests in alternative
* Spot pools if capacity is not available. When this value is maintain
, the Spot Fleet maintains the
* target capacity. The Spot Fleet places the required requests to meet capacity and automatically replenishes any
* interrupted instances. Default: maintain
. instant
is listed but is not used by Spot
* Fleet.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link FleetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of request. Indicates whether the Spot Fleet only requests the target capacity or also attempts
* to maintain it. When this value is request
, the Spot Fleet only places the required
* requests. It does not attempt to replenish Spot Instances if capacity is diminished, nor does it submit
* requests in alternative Spot pools if capacity is not available. When this value is maintain
* , the Spot Fleet maintains the target capacity. The Spot Fleet places the required requests to meet
* capacity and automatically replenishes any interrupted instances. Default: maintain
.
* instant
is listed but is not used by Spot Fleet.
* @see FleetType
*/
public final FleetType type() {
return FleetType.fromValue(type);
}
/**
*
* The type of request. Indicates whether the Spot Fleet only requests the target capacity or also attempts to
* maintain it. When this value is request
, the Spot Fleet only places the required requests. It does
* not attempt to replenish Spot Instances if capacity is diminished, nor does it submit requests in alternative
* Spot pools if capacity is not available. When this value is maintain
, the Spot Fleet maintains the
* target capacity. The Spot Fleet places the required requests to meet capacity and automatically replenishes any
* interrupted instances. Default: maintain
. instant
is listed but is not used by Spot
* Fleet.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link FleetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of request. Indicates whether the Spot Fleet only requests the target capacity or also attempts
* to maintain it. When this value is request
, the Spot Fleet only places the required
* requests. It does not attempt to replenish Spot Instances if capacity is diminished, nor does it submit
* requests in alternative Spot pools if capacity is not available. When this value is maintain
* , the Spot Fleet maintains the target capacity. The Spot Fleet places the required requests to meet
* capacity and automatically replenishes any interrupted instances. Default: maintain
.
* instant
is listed but is not used by Spot Fleet.
* @see FleetType
*/
public final String typeAsString() {
return type;
}
/**
*
* The start date and time of the request, in UTC format
* (YYYY-MM-DDTHH:MM:SSZ). By default, Amazon EC2 starts fulfilling the
* request immediately.
*
*
* @return The start date and time of the request, in UTC format
* (YYYY-MM-DDTHH:MM:SSZ). By default, Amazon EC2 starts
* fulfilling the request immediately.
*/
public final Instant validFrom() {
return validFrom;
}
/**
*
* The end date and time of the request, in UTC format
* (YYYY-MM-DDTHH:MM:SSZ). After the end date and time, no new Spot
* Instance requests are placed or able to fulfill the request. If no value is specified, the Spot Fleet request
* remains until you cancel it.
*
*
* @return The end date and time of the request, in UTC format
* (YYYY-MM-DDTHH:MM:SSZ). After the end date and time, no new
* Spot Instance requests are placed or able to fulfill the request. If no value is specified, the Spot
* Fleet request remains until you cancel it.
*/
public final Instant validUntil() {
return validUntil;
}
/**
*
* Indicates whether Spot Fleet should replace unhealthy instances.
*
*
* @return Indicates whether Spot Fleet should replace unhealthy instances.
*/
public final Boolean replaceUnhealthyInstances() {
return replaceUnhealthyInstances;
}
/**
*
* The behavior when a Spot Instance is interrupted. The default is terminate
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #instanceInterruptionBehavior} will return {@link InstanceInterruptionBehavior#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #instanceInterruptionBehaviorAsString}.
*
*
* @return The behavior when a Spot Instance is interrupted. The default is terminate
.
* @see InstanceInterruptionBehavior
*/
public final InstanceInterruptionBehavior instanceInterruptionBehavior() {
return InstanceInterruptionBehavior.fromValue(instanceInterruptionBehavior);
}
/**
*
* The behavior when a Spot Instance is interrupted. The default is terminate
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #instanceInterruptionBehavior} will return {@link InstanceInterruptionBehavior#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #instanceInterruptionBehaviorAsString}.
*
*
* @return The behavior when a Spot Instance is interrupted. The default is terminate
.
* @see InstanceInterruptionBehavior
*/
public final String instanceInterruptionBehaviorAsString() {
return instanceInterruptionBehavior;
}
/**
*
* One or more Classic Load Balancers and target groups to attach to the Spot Fleet request. Spot Fleet registers
* the running Spot Instances with the specified Classic Load Balancers and target groups.
*
*
* With Network Load Balancers, Spot Fleet cannot register instances that have the following instance types: C1,
* CC1, CC2, CG1, CG2, CR1, CS1, G1, G2, HI1, HS1, M1, M2, M3, and T1.
*
*
* @return One or more Classic Load Balancers and target groups to attach to the Spot Fleet request. Spot Fleet
* registers the running Spot Instances with the specified Classic Load Balancers and target groups.
*
* With Network Load Balancers, Spot Fleet cannot register instances that have the following instance types:
* C1, CC1, CC2, CG1, CG2, CR1, CS1, G1, G2, HI1, HS1, M1, M2, M3, and T1.
*/
public final LoadBalancersConfig loadBalancersConfig() {
return loadBalancersConfig;
}
/**
*
* The number of Spot pools across which to allocate your target Spot capacity. Valid only when Spot
* AllocationStrategy is set to lowest-price
. Spot Fleet selects the cheapest Spot pools and
* evenly allocates your target Spot capacity across the number of Spot pools that you specify.
*
*
* Note that Spot Fleet attempts to draw Spot Instances from the number of pools that you specify on a best effort
* basis. If a pool runs out of Spot capacity before fulfilling your target capacity, Spot Fleet will continue to
* fulfill your request by drawing from the next cheapest pool. To ensure that your target capacity is met, you
* might receive Spot Instances from more than the number of pools that you specified. Similarly, if most of the
* pools have no Spot capacity, you might receive your full target capacity from fewer than the number of pools that
* you specified.
*
*
* @return The number of Spot pools across which to allocate your target Spot capacity. Valid only when Spot
* AllocationStrategy is set to lowest-price
. Spot Fleet selects the cheapest Spot pools
* and evenly allocates your target Spot capacity across the number of Spot pools that you specify.
*
* Note that Spot Fleet attempts to draw Spot Instances from the number of pools that you specify on a best
* effort basis. If a pool runs out of Spot capacity before fulfilling your target capacity, Spot Fleet will
* continue to fulfill your request by drawing from the next cheapest pool. To ensure that your target
* capacity is met, you might receive Spot Instances from more than the number of pools that you specified.
* Similarly, if most of the pools have no Spot capacity, you might receive your full target capacity from
* fewer than the number of pools that you specified.
*/
public final Integer instancePoolsToUseCount() {
return instancePoolsToUseCount;
}
/**
*
* Reserved.
*
*
* @return Reserved.
*/
public final String context() {
return context;
}
/**
*
* The unit for the target capacity. You can specify this parameter only when using attribute-based instance type
* selection.
*
*
* Default: units
(the number of instances)
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #targetCapacityUnitType} will return {@link TargetCapacityUnitType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #targetCapacityUnitTypeAsString}.
*
*
* @return The unit for the target capacity. You can specify this parameter only when using attribute-based instance
* type selection.
*
* Default: units
(the number of instances)
* @see TargetCapacityUnitType
*/
public final TargetCapacityUnitType targetCapacityUnitType() {
return TargetCapacityUnitType.fromValue(targetCapacityUnitType);
}
/**
*
* The unit for the target capacity. You can specify this parameter only when using attribute-based instance type
* selection.
*
*
* Default: units
(the number of instances)
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #targetCapacityUnitType} will return {@link TargetCapacityUnitType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #targetCapacityUnitTypeAsString}.
*
*
* @return The unit for the target capacity. You can specify this parameter only when using attribute-based instance
* type selection.
*
* Default: units
(the number of instances)
* @see TargetCapacityUnitType
*/
public final String targetCapacityUnitTypeAsString() {
return targetCapacityUnitType;
}
/**
* For responses, this returns true if the service returned a value for the TagSpecifications property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTagSpecifications() {
return tagSpecifications != null && !(tagSpecifications instanceof SdkAutoConstructList);
}
/**
*
* The key-value pair for tagging the Spot Fleet request on creation. The value for ResourceType
must
* be spot-fleet-request
, otherwise the Spot Fleet request fails. To tag instances at launch, specify
* the tags in the launch template (valid only if you use LaunchTemplateConfigs
) or in the
* SpotFleetTagSpecification
* (valid only if you use LaunchSpecifications
). For information about tagging after launch, see Tag your resources.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTagSpecifications} method.
*
*
* @return The key-value pair for tagging the Spot Fleet request on creation. The value for
* ResourceType
must be spot-fleet-request
, otherwise the Spot Fleet request
* fails. To tag instances at launch, specify the tags in the launch template (valid only if you use LaunchTemplateConfigs
) or in the
* SpotFleetTagSpecification
* (valid only if you use LaunchSpecifications
). For information about tagging after launch,
* see Tag your
* resources.
*/
public final List tagSpecifications() {
return tagSpecifications;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(allocationStrategyAsString());
hashCode = 31 * hashCode + Objects.hashCode(onDemandAllocationStrategyAsString());
hashCode = 31 * hashCode + Objects.hashCode(spotMaintenanceStrategies());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(excessCapacityTerminationPolicyAsString());
hashCode = 31 * hashCode + Objects.hashCode(fulfilledCapacity());
hashCode = 31 * hashCode + Objects.hashCode(onDemandFulfilledCapacity());
hashCode = 31 * hashCode + Objects.hashCode(iamFleetRole());
hashCode = 31 * hashCode + Objects.hashCode(hasLaunchSpecifications() ? launchSpecifications() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLaunchTemplateConfigs() ? launchTemplateConfigs() : null);
hashCode = 31 * hashCode + Objects.hashCode(spotPrice());
hashCode = 31 * hashCode + Objects.hashCode(targetCapacity());
hashCode = 31 * hashCode + Objects.hashCode(onDemandTargetCapacity());
hashCode = 31 * hashCode + Objects.hashCode(onDemandMaxTotalPrice());
hashCode = 31 * hashCode + Objects.hashCode(spotMaxTotalPrice());
hashCode = 31 * hashCode + Objects.hashCode(terminateInstancesWithExpiration());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(validFrom());
hashCode = 31 * hashCode + Objects.hashCode(validUntil());
hashCode = 31 * hashCode + Objects.hashCode(replaceUnhealthyInstances());
hashCode = 31 * hashCode + Objects.hashCode(instanceInterruptionBehaviorAsString());
hashCode = 31 * hashCode + Objects.hashCode(loadBalancersConfig());
hashCode = 31 * hashCode + Objects.hashCode(instancePoolsToUseCount());
hashCode = 31 * hashCode + Objects.hashCode(context());
hashCode = 31 * hashCode + Objects.hashCode(targetCapacityUnitTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasTagSpecifications() ? tagSpecifications() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SpotFleetRequestConfigData)) {
return false;
}
SpotFleetRequestConfigData other = (SpotFleetRequestConfigData) obj;
return Objects.equals(allocationStrategyAsString(), other.allocationStrategyAsString())
&& Objects.equals(onDemandAllocationStrategyAsString(), other.onDemandAllocationStrategyAsString())
&& Objects.equals(spotMaintenanceStrategies(), other.spotMaintenanceStrategies())
&& Objects.equals(clientToken(), other.clientToken())
&& Objects.equals(excessCapacityTerminationPolicyAsString(), other.excessCapacityTerminationPolicyAsString())
&& Objects.equals(fulfilledCapacity(), other.fulfilledCapacity())
&& Objects.equals(onDemandFulfilledCapacity(), other.onDemandFulfilledCapacity())
&& Objects.equals(iamFleetRole(), other.iamFleetRole())
&& hasLaunchSpecifications() == other.hasLaunchSpecifications()
&& Objects.equals(launchSpecifications(), other.launchSpecifications())
&& hasLaunchTemplateConfigs() == other.hasLaunchTemplateConfigs()
&& Objects.equals(launchTemplateConfigs(), other.launchTemplateConfigs())
&& Objects.equals(spotPrice(), other.spotPrice()) && Objects.equals(targetCapacity(), other.targetCapacity())
&& Objects.equals(onDemandTargetCapacity(), other.onDemandTargetCapacity())
&& Objects.equals(onDemandMaxTotalPrice(), other.onDemandMaxTotalPrice())
&& Objects.equals(spotMaxTotalPrice(), other.spotMaxTotalPrice())
&& Objects.equals(terminateInstancesWithExpiration(), other.terminateInstancesWithExpiration())
&& Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(validFrom(), other.validFrom())
&& Objects.equals(validUntil(), other.validUntil())
&& Objects.equals(replaceUnhealthyInstances(), other.replaceUnhealthyInstances())
&& Objects.equals(instanceInterruptionBehaviorAsString(), other.instanceInterruptionBehaviorAsString())
&& Objects.equals(loadBalancersConfig(), other.loadBalancersConfig())
&& Objects.equals(instancePoolsToUseCount(), other.instancePoolsToUseCount())
&& Objects.equals(context(), other.context())
&& Objects.equals(targetCapacityUnitTypeAsString(), other.targetCapacityUnitTypeAsString())
&& hasTagSpecifications() == other.hasTagSpecifications()
&& Objects.equals(tagSpecifications(), other.tagSpecifications());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SpotFleetRequestConfigData").add("AllocationStrategy", allocationStrategyAsString())
.add("OnDemandAllocationStrategy", onDemandAllocationStrategyAsString())
.add("SpotMaintenanceStrategies", spotMaintenanceStrategies()).add("ClientToken", clientToken())
.add("ExcessCapacityTerminationPolicy", excessCapacityTerminationPolicyAsString())
.add("FulfilledCapacity", fulfilledCapacity()).add("OnDemandFulfilledCapacity", onDemandFulfilledCapacity())
.add("IamFleetRole", iamFleetRole())
.add("LaunchSpecifications", hasLaunchSpecifications() ? launchSpecifications() : null)
.add("LaunchTemplateConfigs", hasLaunchTemplateConfigs() ? launchTemplateConfigs() : null)
.add("SpotPrice", spotPrice()).add("TargetCapacity", targetCapacity())
.add("OnDemandTargetCapacity", onDemandTargetCapacity()).add("OnDemandMaxTotalPrice", onDemandMaxTotalPrice())
.add("SpotMaxTotalPrice", spotMaxTotalPrice())
.add("TerminateInstancesWithExpiration", terminateInstancesWithExpiration()).add("Type", typeAsString())
.add("ValidFrom", validFrom()).add("ValidUntil", validUntil())
.add("ReplaceUnhealthyInstances", replaceUnhealthyInstances())
.add("InstanceInterruptionBehavior", instanceInterruptionBehaviorAsString())
.add("LoadBalancersConfig", loadBalancersConfig()).add("InstancePoolsToUseCount", instancePoolsToUseCount())
.add("Context", context()).add("TargetCapacityUnitType", targetCapacityUnitTypeAsString())
.add("TagSpecifications", hasTagSpecifications() ? tagSpecifications() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AllocationStrategy":
return Optional.ofNullable(clazz.cast(allocationStrategyAsString()));
case "OnDemandAllocationStrategy":
return Optional.ofNullable(clazz.cast(onDemandAllocationStrategyAsString()));
case "SpotMaintenanceStrategies":
return Optional.ofNullable(clazz.cast(spotMaintenanceStrategies()));
case "ClientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "ExcessCapacityTerminationPolicy":
return Optional.ofNullable(clazz.cast(excessCapacityTerminationPolicyAsString()));
case "FulfilledCapacity":
return Optional.ofNullable(clazz.cast(fulfilledCapacity()));
case "OnDemandFulfilledCapacity":
return Optional.ofNullable(clazz.cast(onDemandFulfilledCapacity()));
case "IamFleetRole":
return Optional.ofNullable(clazz.cast(iamFleetRole()));
case "LaunchSpecifications":
return Optional.ofNullable(clazz.cast(launchSpecifications()));
case "LaunchTemplateConfigs":
return Optional.ofNullable(clazz.cast(launchTemplateConfigs()));
case "SpotPrice":
return Optional.ofNullable(clazz.cast(spotPrice()));
case "TargetCapacity":
return Optional.ofNullable(clazz.cast(targetCapacity()));
case "OnDemandTargetCapacity":
return Optional.ofNullable(clazz.cast(onDemandTargetCapacity()));
case "OnDemandMaxTotalPrice":
return Optional.ofNullable(clazz.cast(onDemandMaxTotalPrice()));
case "SpotMaxTotalPrice":
return Optional.ofNullable(clazz.cast(spotMaxTotalPrice()));
case "TerminateInstancesWithExpiration":
return Optional.ofNullable(clazz.cast(terminateInstancesWithExpiration()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "ValidFrom":
return Optional.ofNullable(clazz.cast(validFrom()));
case "ValidUntil":
return Optional.ofNullable(clazz.cast(validUntil()));
case "ReplaceUnhealthyInstances":
return Optional.ofNullable(clazz.cast(replaceUnhealthyInstances()));
case "InstanceInterruptionBehavior":
return Optional.ofNullable(clazz.cast(instanceInterruptionBehaviorAsString()));
case "LoadBalancersConfig":
return Optional.ofNullable(clazz.cast(loadBalancersConfig()));
case "InstancePoolsToUseCount":
return Optional.ofNullable(clazz.cast(instancePoolsToUseCount()));
case "Context":
return Optional.ofNullable(clazz.cast(context()));
case "TargetCapacityUnitType":
return Optional.ofNullable(clazz.cast(targetCapacityUnitTypeAsString()));
case "TagSpecifications":
return Optional.ofNullable(clazz.cast(tagSpecifications()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function