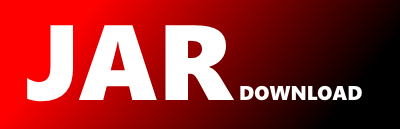
software.amazon.awssdk.services.ec2.model.Subnet Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a subnet.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Subnet implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField AVAILABILITY_ZONE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AvailabilityZoneId")
.getter(getter(Subnet::availabilityZoneId))
.setter(setter(Builder::availabilityZoneId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZoneId")
.unmarshallLocationName("availabilityZoneId").build()).build();
private static final SdkField ENABLE_LNI_AT_DEVICE_INDEX_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("EnableLniAtDeviceIndex")
.getter(getter(Subnet::enableLniAtDeviceIndex))
.setter(setter(Builder::enableLniAtDeviceIndex))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnableLniAtDeviceIndex")
.unmarshallLocationName("enableLniAtDeviceIndex").build()).build();
private static final SdkField MAP_CUSTOMER_OWNED_IP_ON_LAUNCH_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("MapCustomerOwnedIpOnLaunch")
.getter(getter(Subnet::mapCustomerOwnedIpOnLaunch))
.setter(setter(Builder::mapCustomerOwnedIpOnLaunch))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MapCustomerOwnedIpOnLaunch")
.unmarshallLocationName("mapCustomerOwnedIpOnLaunch").build()).build();
private static final SdkField CUSTOMER_OWNED_IPV4_POOL_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CustomerOwnedIpv4Pool")
.getter(getter(Subnet::customerOwnedIpv4Pool))
.setter(setter(Builder::customerOwnedIpv4Pool))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomerOwnedIpv4Pool")
.unmarshallLocationName("customerOwnedIpv4Pool").build()).build();
private static final SdkField OWNER_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OwnerId")
.getter(getter(Subnet::ownerId))
.setter(setter(Builder::ownerId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OwnerId")
.unmarshallLocationName("ownerId").build()).build();
private static final SdkField ASSIGN_IPV6_ADDRESS_ON_CREATION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("AssignIpv6AddressOnCreation")
.getter(getter(Subnet::assignIpv6AddressOnCreation))
.setter(setter(Builder::assignIpv6AddressOnCreation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssignIpv6AddressOnCreation")
.unmarshallLocationName("assignIpv6AddressOnCreation").build()).build();
private static final SdkField> IPV6_CIDR_BLOCK_ASSOCIATION_SET_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Ipv6CidrBlockAssociationSet")
.getter(getter(Subnet::ipv6CidrBlockAssociationSet))
.setter(setter(Builder::ipv6CidrBlockAssociationSet))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ipv6CidrBlockAssociationSet")
.unmarshallLocationName("ipv6CidrBlockAssociationSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SubnetIpv6CidrBlockAssociation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(Subnet::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagSet")
.unmarshallLocationName("tagSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField SUBNET_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SubnetArn")
.getter(getter(Subnet::subnetArn))
.setter(setter(Builder::subnetArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetArn")
.unmarshallLocationName("subnetArn").build()).build();
private static final SdkField OUTPOST_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OutpostArn")
.getter(getter(Subnet::outpostArn))
.setter(setter(Builder::outpostArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutpostArn")
.unmarshallLocationName("outpostArn").build()).build();
private static final SdkField ENABLE_DNS64_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EnableDns64")
.getter(getter(Subnet::enableDns64))
.setter(setter(Builder::enableDns64))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnableDns64")
.unmarshallLocationName("enableDns64").build()).build();
private static final SdkField IPV6_NATIVE_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("Ipv6Native")
.getter(getter(Subnet::ipv6Native))
.setter(setter(Builder::ipv6Native))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ipv6Native")
.unmarshallLocationName("ipv6Native").build()).build();
private static final SdkField PRIVATE_DNS_NAME_OPTIONS_ON_LAUNCH_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("PrivateDnsNameOptionsOnLaunch")
.getter(getter(Subnet::privateDnsNameOptionsOnLaunch))
.setter(setter(Builder::privateDnsNameOptionsOnLaunch))
.constructor(PrivateDnsNameOptionsOnLaunch::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PrivateDnsNameOptionsOnLaunch")
.unmarshallLocationName("privateDnsNameOptionsOnLaunch").build()).build();
private static final SdkField SUBNET_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SubnetId")
.getter(getter(Subnet::subnetId))
.setter(setter(Builder::subnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetId")
.unmarshallLocationName("subnetId").build()).build();
private static final SdkField STATE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("State")
.getter(getter(Subnet::stateAsString))
.setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State")
.unmarshallLocationName("state").build()).build();
private static final SdkField VPC_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VpcId")
.getter(getter(Subnet::vpcId))
.setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId")
.unmarshallLocationName("vpcId").build()).build();
private static final SdkField CIDR_BLOCK_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CidrBlock")
.getter(getter(Subnet::cidrBlock))
.setter(setter(Builder::cidrBlock))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CidrBlock")
.unmarshallLocationName("cidrBlock").build()).build();
private static final SdkField AVAILABLE_IP_ADDRESS_COUNT_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("AvailableIpAddressCount")
.getter(getter(Subnet::availableIpAddressCount))
.setter(setter(Builder::availableIpAddressCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailableIpAddressCount")
.unmarshallLocationName("availableIpAddressCount").build()).build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AvailabilityZone")
.getter(getter(Subnet::availabilityZone))
.setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone")
.unmarshallLocationName("availabilityZone").build()).build();
private static final SdkField DEFAULT_FOR_AZ_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("DefaultForAz")
.getter(getter(Subnet::defaultForAz))
.setter(setter(Builder::defaultForAz))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DefaultForAz")
.unmarshallLocationName("defaultForAz").build()).build();
private static final SdkField MAP_PUBLIC_IP_ON_LAUNCH_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("MapPublicIpOnLaunch")
.getter(getter(Subnet::mapPublicIpOnLaunch))
.setter(setter(Builder::mapPublicIpOnLaunch))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MapPublicIpOnLaunch")
.unmarshallLocationName("mapPublicIpOnLaunch").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AVAILABILITY_ZONE_ID_FIELD,
ENABLE_LNI_AT_DEVICE_INDEX_FIELD, MAP_CUSTOMER_OWNED_IP_ON_LAUNCH_FIELD, CUSTOMER_OWNED_IPV4_POOL_FIELD,
OWNER_ID_FIELD, ASSIGN_IPV6_ADDRESS_ON_CREATION_FIELD, IPV6_CIDR_BLOCK_ASSOCIATION_SET_FIELD, TAGS_FIELD,
SUBNET_ARN_FIELD, OUTPOST_ARN_FIELD, ENABLE_DNS64_FIELD, IPV6_NATIVE_FIELD, PRIVATE_DNS_NAME_OPTIONS_ON_LAUNCH_FIELD,
SUBNET_ID_FIELD, STATE_FIELD, VPC_ID_FIELD, CIDR_BLOCK_FIELD, AVAILABLE_IP_ADDRESS_COUNT_FIELD,
AVAILABILITY_ZONE_FIELD, DEFAULT_FOR_AZ_FIELD, MAP_PUBLIC_IP_ON_LAUNCH_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("AvailabilityZoneId", AVAILABILITY_ZONE_ID_FIELD);
put("EnableLniAtDeviceIndex", ENABLE_LNI_AT_DEVICE_INDEX_FIELD);
put("MapCustomerOwnedIpOnLaunch", MAP_CUSTOMER_OWNED_IP_ON_LAUNCH_FIELD);
put("CustomerOwnedIpv4Pool", CUSTOMER_OWNED_IPV4_POOL_FIELD);
put("OwnerId", OWNER_ID_FIELD);
put("AssignIpv6AddressOnCreation", ASSIGN_IPV6_ADDRESS_ON_CREATION_FIELD);
put("Ipv6CidrBlockAssociationSet", IPV6_CIDR_BLOCK_ASSOCIATION_SET_FIELD);
put("TagSet", TAGS_FIELD);
put("SubnetArn", SUBNET_ARN_FIELD);
put("OutpostArn", OUTPOST_ARN_FIELD);
put("EnableDns64", ENABLE_DNS64_FIELD);
put("Ipv6Native", IPV6_NATIVE_FIELD);
put("PrivateDnsNameOptionsOnLaunch", PRIVATE_DNS_NAME_OPTIONS_ON_LAUNCH_FIELD);
put("SubnetId", SUBNET_ID_FIELD);
put("State", STATE_FIELD);
put("VpcId", VPC_ID_FIELD);
put("CidrBlock", CIDR_BLOCK_FIELD);
put("AvailableIpAddressCount", AVAILABLE_IP_ADDRESS_COUNT_FIELD);
put("AvailabilityZone", AVAILABILITY_ZONE_FIELD);
put("DefaultForAz", DEFAULT_FOR_AZ_FIELD);
put("MapPublicIpOnLaunch", MAP_PUBLIC_IP_ON_LAUNCH_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String availabilityZoneId;
private final Integer enableLniAtDeviceIndex;
private final Boolean mapCustomerOwnedIpOnLaunch;
private final String customerOwnedIpv4Pool;
private final String ownerId;
private final Boolean assignIpv6AddressOnCreation;
private final List ipv6CidrBlockAssociationSet;
private final List tags;
private final String subnetArn;
private final String outpostArn;
private final Boolean enableDns64;
private final Boolean ipv6Native;
private final PrivateDnsNameOptionsOnLaunch privateDnsNameOptionsOnLaunch;
private final String subnetId;
private final String state;
private final String vpcId;
private final String cidrBlock;
private final Integer availableIpAddressCount;
private final String availabilityZone;
private final Boolean defaultForAz;
private final Boolean mapPublicIpOnLaunch;
private Subnet(BuilderImpl builder) {
this.availabilityZoneId = builder.availabilityZoneId;
this.enableLniAtDeviceIndex = builder.enableLniAtDeviceIndex;
this.mapCustomerOwnedIpOnLaunch = builder.mapCustomerOwnedIpOnLaunch;
this.customerOwnedIpv4Pool = builder.customerOwnedIpv4Pool;
this.ownerId = builder.ownerId;
this.assignIpv6AddressOnCreation = builder.assignIpv6AddressOnCreation;
this.ipv6CidrBlockAssociationSet = builder.ipv6CidrBlockAssociationSet;
this.tags = builder.tags;
this.subnetArn = builder.subnetArn;
this.outpostArn = builder.outpostArn;
this.enableDns64 = builder.enableDns64;
this.ipv6Native = builder.ipv6Native;
this.privateDnsNameOptionsOnLaunch = builder.privateDnsNameOptionsOnLaunch;
this.subnetId = builder.subnetId;
this.state = builder.state;
this.vpcId = builder.vpcId;
this.cidrBlock = builder.cidrBlock;
this.availableIpAddressCount = builder.availableIpAddressCount;
this.availabilityZone = builder.availabilityZone;
this.defaultForAz = builder.defaultForAz;
this.mapPublicIpOnLaunch = builder.mapPublicIpOnLaunch;
}
/**
*
* The AZ ID of the subnet.
*
*
* @return The AZ ID of the subnet.
*/
public final String availabilityZoneId() {
return availabilityZoneId;
}
/**
*
* Indicates the device position for local network interfaces in this subnet. For example, 1
indicates
* local network interfaces in this subnet are the secondary network interface (eth1).
*
*
* @return Indicates the device position for local network interfaces in this subnet. For example, 1
* indicates local network interfaces in this subnet are the secondary network interface (eth1).
*/
public final Integer enableLniAtDeviceIndex() {
return enableLniAtDeviceIndex;
}
/**
*
* Indicates whether a network interface created in this subnet (including a network interface created by
* RunInstances) receives a customer-owned IPv4 address.
*
*
* @return Indicates whether a network interface created in this subnet (including a network interface created by
* RunInstances) receives a customer-owned IPv4 address.
*/
public final Boolean mapCustomerOwnedIpOnLaunch() {
return mapCustomerOwnedIpOnLaunch;
}
/**
*
* The customer-owned IPv4 address pool associated with the subnet.
*
*
* @return The customer-owned IPv4 address pool associated with the subnet.
*/
public final String customerOwnedIpv4Pool() {
return customerOwnedIpv4Pool;
}
/**
*
* The ID of the Amazon Web Services account that owns the subnet.
*
*
* @return The ID of the Amazon Web Services account that owns the subnet.
*/
public final String ownerId() {
return ownerId;
}
/**
*
* Indicates whether a network interface created in this subnet (including a network interface created by
* RunInstances) receives an IPv6 address.
*
*
* @return Indicates whether a network interface created in this subnet (including a network interface created by
* RunInstances) receives an IPv6 address.
*/
public final Boolean assignIpv6AddressOnCreation() {
return assignIpv6AddressOnCreation;
}
/**
* For responses, this returns true if the service returned a value for the Ipv6CidrBlockAssociationSet property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasIpv6CidrBlockAssociationSet() {
return ipv6CidrBlockAssociationSet != null && !(ipv6CidrBlockAssociationSet instanceof SdkAutoConstructList);
}
/**
*
* Information about the IPv6 CIDR blocks associated with the subnet.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasIpv6CidrBlockAssociationSet} method.
*
*
* @return Information about the IPv6 CIDR blocks associated with the subnet.
*/
public final List ipv6CidrBlockAssociationSet() {
return ipv6CidrBlockAssociationSet;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* Any tags assigned to the subnet.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return Any tags assigned to the subnet.
*/
public final List tags() {
return tags;
}
/**
*
* The Amazon Resource Name (ARN) of the subnet.
*
*
* @return The Amazon Resource Name (ARN) of the subnet.
*/
public final String subnetArn() {
return subnetArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Outpost.
*
*
* @return The Amazon Resource Name (ARN) of the Outpost.
*/
public final String outpostArn() {
return outpostArn;
}
/**
*
* Indicates whether DNS queries made to the Amazon-provided DNS Resolver in this subnet should return synthetic
* IPv6 addresses for IPv4-only destinations.
*
*
* @return Indicates whether DNS queries made to the Amazon-provided DNS Resolver in this subnet should return
* synthetic IPv6 addresses for IPv4-only destinations.
*/
public final Boolean enableDns64() {
return enableDns64;
}
/**
*
* Indicates whether this is an IPv6 only subnet.
*
*
* @return Indicates whether this is an IPv6 only subnet.
*/
public final Boolean ipv6Native() {
return ipv6Native;
}
/**
*
* The type of hostnames to assign to instances in the subnet at launch. An instance hostname is based on the IPv4
* address or ID of the instance.
*
*
* @return The type of hostnames to assign to instances in the subnet at launch. An instance hostname is based on
* the IPv4 address or ID of the instance.
*/
public final PrivateDnsNameOptionsOnLaunch privateDnsNameOptionsOnLaunch() {
return privateDnsNameOptionsOnLaunch;
}
/**
*
* The ID of the subnet.
*
*
* @return The ID of the subnet.
*/
public final String subnetId() {
return subnetId;
}
/**
*
* The current state of the subnet.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link SubnetState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The current state of the subnet.
* @see SubnetState
*/
public final SubnetState state() {
return SubnetState.fromValue(state);
}
/**
*
* The current state of the subnet.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link SubnetState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The current state of the subnet.
* @see SubnetState
*/
public final String stateAsString() {
return state;
}
/**
*
* The ID of the VPC the subnet is in.
*
*
* @return The ID of the VPC the subnet is in.
*/
public final String vpcId() {
return vpcId;
}
/**
*
* The IPv4 CIDR block assigned to the subnet.
*
*
* @return The IPv4 CIDR block assigned to the subnet.
*/
public final String cidrBlock() {
return cidrBlock;
}
/**
*
* The number of unused private IPv4 addresses in the subnet. The IPv4 addresses for any stopped instances are
* considered unavailable.
*
*
* @return The number of unused private IPv4 addresses in the subnet. The IPv4 addresses for any stopped instances
* are considered unavailable.
*/
public final Integer availableIpAddressCount() {
return availableIpAddressCount;
}
/**
*
* The Availability Zone of the subnet.
*
*
* @return The Availability Zone of the subnet.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* Indicates whether this is the default subnet for the Availability Zone.
*
*
* @return Indicates whether this is the default subnet for the Availability Zone.
*/
public final Boolean defaultForAz() {
return defaultForAz;
}
/**
*
* Indicates whether instances launched in this subnet receive a public IPv4 address.
*
*
* Amazon Web Services charges for all public IPv4 addresses, including public IPv4 addresses associated with
* running instances and Elastic IP addresses. For more information, see the Public IPv4 Address tab on the
* Amazon VPC pricing page.
*
*
* @return Indicates whether instances launched in this subnet receive a public IPv4 address.
*
* Amazon Web Services charges for all public IPv4 addresses, including public IPv4 addresses associated
* with running instances and Elastic IP addresses. For more information, see the Public IPv4 Address
* tab on the Amazon VPC pricing page.
*/
public final Boolean mapPublicIpOnLaunch() {
return mapPublicIpOnLaunch;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(availabilityZoneId());
hashCode = 31 * hashCode + Objects.hashCode(enableLniAtDeviceIndex());
hashCode = 31 * hashCode + Objects.hashCode(mapCustomerOwnedIpOnLaunch());
hashCode = 31 * hashCode + Objects.hashCode(customerOwnedIpv4Pool());
hashCode = 31 * hashCode + Objects.hashCode(ownerId());
hashCode = 31 * hashCode + Objects.hashCode(assignIpv6AddressOnCreation());
hashCode = 31 * hashCode + Objects.hashCode(hasIpv6CidrBlockAssociationSet() ? ipv6CidrBlockAssociationSet() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(subnetArn());
hashCode = 31 * hashCode + Objects.hashCode(outpostArn());
hashCode = 31 * hashCode + Objects.hashCode(enableDns64());
hashCode = 31 * hashCode + Objects.hashCode(ipv6Native());
hashCode = 31 * hashCode + Objects.hashCode(privateDnsNameOptionsOnLaunch());
hashCode = 31 * hashCode + Objects.hashCode(subnetId());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
hashCode = 31 * hashCode + Objects.hashCode(cidrBlock());
hashCode = 31 * hashCode + Objects.hashCode(availableIpAddressCount());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(defaultForAz());
hashCode = 31 * hashCode + Objects.hashCode(mapPublicIpOnLaunch());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Subnet)) {
return false;
}
Subnet other = (Subnet) obj;
return Objects.equals(availabilityZoneId(), other.availabilityZoneId())
&& Objects.equals(enableLniAtDeviceIndex(), other.enableLniAtDeviceIndex())
&& Objects.equals(mapCustomerOwnedIpOnLaunch(), other.mapCustomerOwnedIpOnLaunch())
&& Objects.equals(customerOwnedIpv4Pool(), other.customerOwnedIpv4Pool())
&& Objects.equals(ownerId(), other.ownerId())
&& Objects.equals(assignIpv6AddressOnCreation(), other.assignIpv6AddressOnCreation())
&& hasIpv6CidrBlockAssociationSet() == other.hasIpv6CidrBlockAssociationSet()
&& Objects.equals(ipv6CidrBlockAssociationSet(), other.ipv6CidrBlockAssociationSet())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(subnetArn(), other.subnetArn()) && Objects.equals(outpostArn(), other.outpostArn())
&& Objects.equals(enableDns64(), other.enableDns64()) && Objects.equals(ipv6Native(), other.ipv6Native())
&& Objects.equals(privateDnsNameOptionsOnLaunch(), other.privateDnsNameOptionsOnLaunch())
&& Objects.equals(subnetId(), other.subnetId()) && Objects.equals(stateAsString(), other.stateAsString())
&& Objects.equals(vpcId(), other.vpcId()) && Objects.equals(cidrBlock(), other.cidrBlock())
&& Objects.equals(availableIpAddressCount(), other.availableIpAddressCount())
&& Objects.equals(availabilityZone(), other.availabilityZone())
&& Objects.equals(defaultForAz(), other.defaultForAz())
&& Objects.equals(mapPublicIpOnLaunch(), other.mapPublicIpOnLaunch());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Subnet").add("AvailabilityZoneId", availabilityZoneId())
.add("EnableLniAtDeviceIndex", enableLniAtDeviceIndex())
.add("MapCustomerOwnedIpOnLaunch", mapCustomerOwnedIpOnLaunch())
.add("CustomerOwnedIpv4Pool", customerOwnedIpv4Pool()).add("OwnerId", ownerId())
.add("AssignIpv6AddressOnCreation", assignIpv6AddressOnCreation())
.add("Ipv6CidrBlockAssociationSet", hasIpv6CidrBlockAssociationSet() ? ipv6CidrBlockAssociationSet() : null)
.add("Tags", hasTags() ? tags() : null).add("SubnetArn", subnetArn()).add("OutpostArn", outpostArn())
.add("EnableDns64", enableDns64()).add("Ipv6Native", ipv6Native())
.add("PrivateDnsNameOptionsOnLaunch", privateDnsNameOptionsOnLaunch()).add("SubnetId", subnetId())
.add("State", stateAsString()).add("VpcId", vpcId()).add("CidrBlock", cidrBlock())
.add("AvailableIpAddressCount", availableIpAddressCount()).add("AvailabilityZone", availabilityZone())
.add("DefaultForAz", defaultForAz()).add("MapPublicIpOnLaunch", mapPublicIpOnLaunch()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AvailabilityZoneId":
return Optional.ofNullable(clazz.cast(availabilityZoneId()));
case "EnableLniAtDeviceIndex":
return Optional.ofNullable(clazz.cast(enableLniAtDeviceIndex()));
case "MapCustomerOwnedIpOnLaunch":
return Optional.ofNullable(clazz.cast(mapCustomerOwnedIpOnLaunch()));
case "CustomerOwnedIpv4Pool":
return Optional.ofNullable(clazz.cast(customerOwnedIpv4Pool()));
case "OwnerId":
return Optional.ofNullable(clazz.cast(ownerId()));
case "AssignIpv6AddressOnCreation":
return Optional.ofNullable(clazz.cast(assignIpv6AddressOnCreation()));
case "Ipv6CidrBlockAssociationSet":
return Optional.ofNullable(clazz.cast(ipv6CidrBlockAssociationSet()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "SubnetArn":
return Optional.ofNullable(clazz.cast(subnetArn()));
case "OutpostArn":
return Optional.ofNullable(clazz.cast(outpostArn()));
case "EnableDns64":
return Optional.ofNullable(clazz.cast(enableDns64()));
case "Ipv6Native":
return Optional.ofNullable(clazz.cast(ipv6Native()));
case "PrivateDnsNameOptionsOnLaunch":
return Optional.ofNullable(clazz.cast(privateDnsNameOptionsOnLaunch()));
case "SubnetId":
return Optional.ofNullable(clazz.cast(subnetId()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
case "CidrBlock":
return Optional.ofNullable(clazz.cast(cidrBlock()));
case "AvailableIpAddressCount":
return Optional.ofNullable(clazz.cast(availableIpAddressCount()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "DefaultForAz":
return Optional.ofNullable(clazz.cast(defaultForAz()));
case "MapPublicIpOnLaunch":
return Optional.ofNullable(clazz.cast(mapPublicIpOnLaunch()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function