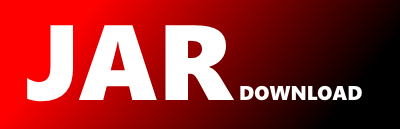
software.amazon.awssdk.services.ec2.model.TransitGatewayRequestOptions Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the options for a transit gateway.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TransitGatewayRequestOptions implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField AMAZON_SIDE_ASN_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("AmazonSideAsn")
.getter(getter(TransitGatewayRequestOptions::amazonSideAsn))
.setter(setter(Builder::amazonSideAsn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AmazonSideAsn")
.unmarshallLocationName("AmazonSideAsn").build()).build();
private static final SdkField AUTO_ACCEPT_SHARED_ATTACHMENTS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AutoAcceptSharedAttachments")
.getter(getter(TransitGatewayRequestOptions::autoAcceptSharedAttachmentsAsString))
.setter(setter(Builder::autoAcceptSharedAttachments))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoAcceptSharedAttachments")
.unmarshallLocationName("AutoAcceptSharedAttachments").build()).build();
private static final SdkField DEFAULT_ROUTE_TABLE_ASSOCIATION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DefaultRouteTableAssociation")
.getter(getter(TransitGatewayRequestOptions::defaultRouteTableAssociationAsString))
.setter(setter(Builder::defaultRouteTableAssociation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DefaultRouteTableAssociation")
.unmarshallLocationName("DefaultRouteTableAssociation").build()).build();
private static final SdkField DEFAULT_ROUTE_TABLE_PROPAGATION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DefaultRouteTablePropagation")
.getter(getter(TransitGatewayRequestOptions::defaultRouteTablePropagationAsString))
.setter(setter(Builder::defaultRouteTablePropagation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DefaultRouteTablePropagation")
.unmarshallLocationName("DefaultRouteTablePropagation").build()).build();
private static final SdkField VPN_ECMP_SUPPORT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VpnEcmpSupport")
.getter(getter(TransitGatewayRequestOptions::vpnEcmpSupportAsString))
.setter(setter(Builder::vpnEcmpSupport))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpnEcmpSupport")
.unmarshallLocationName("VpnEcmpSupport").build()).build();
private static final SdkField DNS_SUPPORT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DnsSupport")
.getter(getter(TransitGatewayRequestOptions::dnsSupportAsString))
.setter(setter(Builder::dnsSupport))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DnsSupport")
.unmarshallLocationName("DnsSupport").build()).build();
private static final SdkField SECURITY_GROUP_REFERENCING_SUPPORT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SecurityGroupReferencingSupport")
.getter(getter(TransitGatewayRequestOptions::securityGroupReferencingSupportAsString))
.setter(setter(Builder::securityGroupReferencingSupport))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroupReferencingSupport")
.unmarshallLocationName("SecurityGroupReferencingSupport").build()).build();
private static final SdkField MULTICAST_SUPPORT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MulticastSupport")
.getter(getter(TransitGatewayRequestOptions::multicastSupportAsString))
.setter(setter(Builder::multicastSupport))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MulticastSupport")
.unmarshallLocationName("MulticastSupport").build()).build();
private static final SdkField> TRANSIT_GATEWAY_CIDR_BLOCKS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TransitGatewayCidrBlocks")
.getter(getter(TransitGatewayRequestOptions::transitGatewayCidrBlocks))
.setter(setter(Builder::transitGatewayCidrBlocks))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransitGatewayCidrBlocks")
.unmarshallLocationName("TransitGatewayCidrBlocks").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AMAZON_SIDE_ASN_FIELD,
AUTO_ACCEPT_SHARED_ATTACHMENTS_FIELD, DEFAULT_ROUTE_TABLE_ASSOCIATION_FIELD, DEFAULT_ROUTE_TABLE_PROPAGATION_FIELD,
VPN_ECMP_SUPPORT_FIELD, DNS_SUPPORT_FIELD, SECURITY_GROUP_REFERENCING_SUPPORT_FIELD, MULTICAST_SUPPORT_FIELD,
TRANSIT_GATEWAY_CIDR_BLOCKS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("AmazonSideAsn", AMAZON_SIDE_ASN_FIELD);
put("AutoAcceptSharedAttachments", AUTO_ACCEPT_SHARED_ATTACHMENTS_FIELD);
put("DefaultRouteTableAssociation", DEFAULT_ROUTE_TABLE_ASSOCIATION_FIELD);
put("DefaultRouteTablePropagation", DEFAULT_ROUTE_TABLE_PROPAGATION_FIELD);
put("VpnEcmpSupport", VPN_ECMP_SUPPORT_FIELD);
put("DnsSupport", DNS_SUPPORT_FIELD);
put("SecurityGroupReferencingSupport", SECURITY_GROUP_REFERENCING_SUPPORT_FIELD);
put("MulticastSupport", MULTICAST_SUPPORT_FIELD);
put("TransitGatewayCidrBlocks", TRANSIT_GATEWAY_CIDR_BLOCKS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final Long amazonSideAsn;
private final String autoAcceptSharedAttachments;
private final String defaultRouteTableAssociation;
private final String defaultRouteTablePropagation;
private final String vpnEcmpSupport;
private final String dnsSupport;
private final String securityGroupReferencingSupport;
private final String multicastSupport;
private final List transitGatewayCidrBlocks;
private TransitGatewayRequestOptions(BuilderImpl builder) {
this.amazonSideAsn = builder.amazonSideAsn;
this.autoAcceptSharedAttachments = builder.autoAcceptSharedAttachments;
this.defaultRouteTableAssociation = builder.defaultRouteTableAssociation;
this.defaultRouteTablePropagation = builder.defaultRouteTablePropagation;
this.vpnEcmpSupport = builder.vpnEcmpSupport;
this.dnsSupport = builder.dnsSupport;
this.securityGroupReferencingSupport = builder.securityGroupReferencingSupport;
this.multicastSupport = builder.multicastSupport;
this.transitGatewayCidrBlocks = builder.transitGatewayCidrBlocks;
}
/**
*
* A private Autonomous System Number (ASN) for the Amazon side of a BGP session. The range is 64512 to 65534 for
* 16-bit ASNs and 4200000000 to 4294967294 for 32-bit ASNs. The default is 64512
.
*
*
* @return A private Autonomous System Number (ASN) for the Amazon side of a BGP session. The range is 64512 to
* 65534 for 16-bit ASNs and 4200000000 to 4294967294 for 32-bit ASNs. The default is 64512
.
*/
public final Long amazonSideAsn() {
return amazonSideAsn;
}
/**
*
* Enable or disable automatic acceptance of attachment requests. Disabled by default.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #autoAcceptSharedAttachments} will return {@link AutoAcceptSharedAttachmentsValue#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #autoAcceptSharedAttachmentsAsString}.
*
*
* @return Enable or disable automatic acceptance of attachment requests. Disabled by default.
* @see AutoAcceptSharedAttachmentsValue
*/
public final AutoAcceptSharedAttachmentsValue autoAcceptSharedAttachments() {
return AutoAcceptSharedAttachmentsValue.fromValue(autoAcceptSharedAttachments);
}
/**
*
* Enable or disable automatic acceptance of attachment requests. Disabled by default.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #autoAcceptSharedAttachments} will return {@link AutoAcceptSharedAttachmentsValue#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #autoAcceptSharedAttachmentsAsString}.
*
*
* @return Enable or disable automatic acceptance of attachment requests. Disabled by default.
* @see AutoAcceptSharedAttachmentsValue
*/
public final String autoAcceptSharedAttachmentsAsString() {
return autoAcceptSharedAttachments;
}
/**
*
* Enable or disable automatic association with the default association route table. Enabled by default.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #defaultRouteTableAssociation} will return
* {@link DefaultRouteTableAssociationValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #defaultRouteTableAssociationAsString}.
*
*
* @return Enable or disable automatic association with the default association route table. Enabled by default.
* @see DefaultRouteTableAssociationValue
*/
public final DefaultRouteTableAssociationValue defaultRouteTableAssociation() {
return DefaultRouteTableAssociationValue.fromValue(defaultRouteTableAssociation);
}
/**
*
* Enable or disable automatic association with the default association route table. Enabled by default.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #defaultRouteTableAssociation} will return
* {@link DefaultRouteTableAssociationValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #defaultRouteTableAssociationAsString}.
*
*
* @return Enable or disable automatic association with the default association route table. Enabled by default.
* @see DefaultRouteTableAssociationValue
*/
public final String defaultRouteTableAssociationAsString() {
return defaultRouteTableAssociation;
}
/**
*
* Enable or disable automatic propagation of routes to the default propagation route table. Enabled by default.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #defaultRouteTablePropagation} will return
* {@link DefaultRouteTablePropagationValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #defaultRouteTablePropagationAsString}.
*
*
* @return Enable or disable automatic propagation of routes to the default propagation route table. Enabled by
* default.
* @see DefaultRouteTablePropagationValue
*/
public final DefaultRouteTablePropagationValue defaultRouteTablePropagation() {
return DefaultRouteTablePropagationValue.fromValue(defaultRouteTablePropagation);
}
/**
*
* Enable or disable automatic propagation of routes to the default propagation route table. Enabled by default.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #defaultRouteTablePropagation} will return
* {@link DefaultRouteTablePropagationValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #defaultRouteTablePropagationAsString}.
*
*
* @return Enable or disable automatic propagation of routes to the default propagation route table. Enabled by
* default.
* @see DefaultRouteTablePropagationValue
*/
public final String defaultRouteTablePropagationAsString() {
return defaultRouteTablePropagation;
}
/**
*
* Enable or disable Equal Cost Multipath Protocol support. Enabled by default.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #vpnEcmpSupport}
* will return {@link VpnEcmpSupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #vpnEcmpSupportAsString}.
*
*
* @return Enable or disable Equal Cost Multipath Protocol support. Enabled by default.
* @see VpnEcmpSupportValue
*/
public final VpnEcmpSupportValue vpnEcmpSupport() {
return VpnEcmpSupportValue.fromValue(vpnEcmpSupport);
}
/**
*
* Enable or disable Equal Cost Multipath Protocol support. Enabled by default.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #vpnEcmpSupport}
* will return {@link VpnEcmpSupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #vpnEcmpSupportAsString}.
*
*
* @return Enable or disable Equal Cost Multipath Protocol support. Enabled by default.
* @see VpnEcmpSupportValue
*/
public final String vpnEcmpSupportAsString() {
return vpnEcmpSupport;
}
/**
*
* Enable or disable DNS support. Enabled by default.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dnsSupport} will
* return {@link DnsSupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dnsSupportAsString}.
*
*
* @return Enable or disable DNS support. Enabled by default.
* @see DnsSupportValue
*/
public final DnsSupportValue dnsSupport() {
return DnsSupportValue.fromValue(dnsSupport);
}
/**
*
* Enable or disable DNS support. Enabled by default.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dnsSupport} will
* return {@link DnsSupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dnsSupportAsString}.
*
*
* @return Enable or disable DNS support. Enabled by default.
* @see DnsSupportValue
*/
public final String dnsSupportAsString() {
return dnsSupport;
}
/**
*
* Enables you to reference a security group across VPCs attached to a transit gateway to simplify security group
* management.
*
*
* This option is disabled by default.
*
*
* For more information about security group referencing, see Security group
* referencing in the Amazon Web Services Transit Gateways Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #securityGroupReferencingSupport} will return
* {@link SecurityGroupReferencingSupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #securityGroupReferencingSupportAsString}.
*
*
* @return Enables you to reference a security group across VPCs attached to a transit gateway to simplify security
* group management.
*
* This option is disabled by default.
*
*
* For more information about security group referencing, see Security group referencing in the Amazon Web Services Transit Gateways Guide.
* @see SecurityGroupReferencingSupportValue
*/
public final SecurityGroupReferencingSupportValue securityGroupReferencingSupport() {
return SecurityGroupReferencingSupportValue.fromValue(securityGroupReferencingSupport);
}
/**
*
* Enables you to reference a security group across VPCs attached to a transit gateway to simplify security group
* management.
*
*
* This option is disabled by default.
*
*
* For more information about security group referencing, see Security group
* referencing in the Amazon Web Services Transit Gateways Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #securityGroupReferencingSupport} will return
* {@link SecurityGroupReferencingSupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #securityGroupReferencingSupportAsString}.
*
*
* @return Enables you to reference a security group across VPCs attached to a transit gateway to simplify security
* group management.
*
* This option is disabled by default.
*
*
* For more information about security group referencing, see Security group referencing in the Amazon Web Services Transit Gateways Guide.
* @see SecurityGroupReferencingSupportValue
*/
public final String securityGroupReferencingSupportAsString() {
return securityGroupReferencingSupport;
}
/**
*
* Indicates whether multicast is enabled on the transit gateway
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #multicastSupport}
* will return {@link MulticastSupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #multicastSupportAsString}.
*
*
* @return Indicates whether multicast is enabled on the transit gateway
* @see MulticastSupportValue
*/
public final MulticastSupportValue multicastSupport() {
return MulticastSupportValue.fromValue(multicastSupport);
}
/**
*
* Indicates whether multicast is enabled on the transit gateway
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #multicastSupport}
* will return {@link MulticastSupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #multicastSupportAsString}.
*
*
* @return Indicates whether multicast is enabled on the transit gateway
* @see MulticastSupportValue
*/
public final String multicastSupportAsString() {
return multicastSupport;
}
/**
* For responses, this returns true if the service returned a value for the TransitGatewayCidrBlocks property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasTransitGatewayCidrBlocks() {
return transitGatewayCidrBlocks != null && !(transitGatewayCidrBlocks instanceof SdkAutoConstructList);
}
/**
*
* One or more IPv4 or IPv6 CIDR blocks for the transit gateway. Must be a size /24 CIDR block or larger for IPv4,
* or a size /64 CIDR block or larger for IPv6.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTransitGatewayCidrBlocks} method.
*
*
* @return One or more IPv4 or IPv6 CIDR blocks for the transit gateway. Must be a size /24 CIDR block or larger for
* IPv4, or a size /64 CIDR block or larger for IPv6.
*/
public final List transitGatewayCidrBlocks() {
return transitGatewayCidrBlocks;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(amazonSideAsn());
hashCode = 31 * hashCode + Objects.hashCode(autoAcceptSharedAttachmentsAsString());
hashCode = 31 * hashCode + Objects.hashCode(defaultRouteTableAssociationAsString());
hashCode = 31 * hashCode + Objects.hashCode(defaultRouteTablePropagationAsString());
hashCode = 31 * hashCode + Objects.hashCode(vpnEcmpSupportAsString());
hashCode = 31 * hashCode + Objects.hashCode(dnsSupportAsString());
hashCode = 31 * hashCode + Objects.hashCode(securityGroupReferencingSupportAsString());
hashCode = 31 * hashCode + Objects.hashCode(multicastSupportAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasTransitGatewayCidrBlocks() ? transitGatewayCidrBlocks() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TransitGatewayRequestOptions)) {
return false;
}
TransitGatewayRequestOptions other = (TransitGatewayRequestOptions) obj;
return Objects.equals(amazonSideAsn(), other.amazonSideAsn())
&& Objects.equals(autoAcceptSharedAttachmentsAsString(), other.autoAcceptSharedAttachmentsAsString())
&& Objects.equals(defaultRouteTableAssociationAsString(), other.defaultRouteTableAssociationAsString())
&& Objects.equals(defaultRouteTablePropagationAsString(), other.defaultRouteTablePropagationAsString())
&& Objects.equals(vpnEcmpSupportAsString(), other.vpnEcmpSupportAsString())
&& Objects.equals(dnsSupportAsString(), other.dnsSupportAsString())
&& Objects.equals(securityGroupReferencingSupportAsString(), other.securityGroupReferencingSupportAsString())
&& Objects.equals(multicastSupportAsString(), other.multicastSupportAsString())
&& hasTransitGatewayCidrBlocks() == other.hasTransitGatewayCidrBlocks()
&& Objects.equals(transitGatewayCidrBlocks(), other.transitGatewayCidrBlocks());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TransitGatewayRequestOptions").add("AmazonSideAsn", amazonSideAsn())
.add("AutoAcceptSharedAttachments", autoAcceptSharedAttachmentsAsString())
.add("DefaultRouteTableAssociation", defaultRouteTableAssociationAsString())
.add("DefaultRouteTablePropagation", defaultRouteTablePropagationAsString())
.add("VpnEcmpSupport", vpnEcmpSupportAsString()).add("DnsSupport", dnsSupportAsString())
.add("SecurityGroupReferencingSupport", securityGroupReferencingSupportAsString())
.add("MulticastSupport", multicastSupportAsString())
.add("TransitGatewayCidrBlocks", hasTransitGatewayCidrBlocks() ? transitGatewayCidrBlocks() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AmazonSideAsn":
return Optional.ofNullable(clazz.cast(amazonSideAsn()));
case "AutoAcceptSharedAttachments":
return Optional.ofNullable(clazz.cast(autoAcceptSharedAttachmentsAsString()));
case "DefaultRouteTableAssociation":
return Optional.ofNullable(clazz.cast(defaultRouteTableAssociationAsString()));
case "DefaultRouteTablePropagation":
return Optional.ofNullable(clazz.cast(defaultRouteTablePropagationAsString()));
case "VpnEcmpSupport":
return Optional.ofNullable(clazz.cast(vpnEcmpSupportAsString()));
case "DnsSupport":
return Optional.ofNullable(clazz.cast(dnsSupportAsString()));
case "SecurityGroupReferencingSupport":
return Optional.ofNullable(clazz.cast(securityGroupReferencingSupportAsString()));
case "MulticastSupport":
return Optional.ofNullable(clazz.cast(multicastSupportAsString()));
case "TransitGatewayCidrBlocks":
return Optional.ofNullable(clazz.cast(transitGatewayCidrBlocks()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function