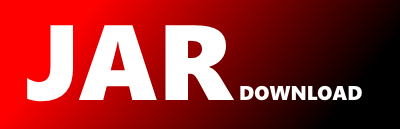
software.amazon.awssdk.services.ec2.model.VpcIpv6CidrBlockAssociation Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes an IPv6 CIDR block associated with a VPC.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class VpcIpv6CidrBlockAssociation implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ASSOCIATION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AssociationId")
.getter(getter(VpcIpv6CidrBlockAssociation::associationId))
.setter(setter(Builder::associationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssociationId")
.unmarshallLocationName("associationId").build()).build();
private static final SdkField IPV6_CIDR_BLOCK_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Ipv6CidrBlock")
.getter(getter(VpcIpv6CidrBlockAssociation::ipv6CidrBlock))
.setter(setter(Builder::ipv6CidrBlock))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ipv6CidrBlock")
.unmarshallLocationName("ipv6CidrBlock").build()).build();
private static final SdkField IPV6_CIDR_BLOCK_STATE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("Ipv6CidrBlockState")
.getter(getter(VpcIpv6CidrBlockAssociation::ipv6CidrBlockState))
.setter(setter(Builder::ipv6CidrBlockState))
.constructor(VpcCidrBlockState::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ipv6CidrBlockState")
.unmarshallLocationName("ipv6CidrBlockState").build()).build();
private static final SdkField NETWORK_BORDER_GROUP_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NetworkBorderGroup")
.getter(getter(VpcIpv6CidrBlockAssociation::networkBorderGroup))
.setter(setter(Builder::networkBorderGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkBorderGroup")
.unmarshallLocationName("networkBorderGroup").build()).build();
private static final SdkField IPV6_POOL_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Ipv6Pool")
.getter(getter(VpcIpv6CidrBlockAssociation::ipv6Pool))
.setter(setter(Builder::ipv6Pool))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ipv6Pool")
.unmarshallLocationName("ipv6Pool").build()).build();
private static final SdkField IPV6_ADDRESS_ATTRIBUTE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Ipv6AddressAttribute")
.getter(getter(VpcIpv6CidrBlockAssociation::ipv6AddressAttributeAsString))
.setter(setter(Builder::ipv6AddressAttribute))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ipv6AddressAttribute")
.unmarshallLocationName("ipv6AddressAttribute").build()).build();
private static final SdkField IP_SOURCE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("IpSource")
.getter(getter(VpcIpv6CidrBlockAssociation::ipSourceAsString))
.setter(setter(Builder::ipSource))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpSource")
.unmarshallLocationName("ipSource").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ASSOCIATION_ID_FIELD,
IPV6_CIDR_BLOCK_FIELD, IPV6_CIDR_BLOCK_STATE_FIELD, NETWORK_BORDER_GROUP_FIELD, IPV6_POOL_FIELD,
IPV6_ADDRESS_ATTRIBUTE_FIELD, IP_SOURCE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("AssociationId", ASSOCIATION_ID_FIELD);
put("Ipv6CidrBlock", IPV6_CIDR_BLOCK_FIELD);
put("Ipv6CidrBlockState", IPV6_CIDR_BLOCK_STATE_FIELD);
put("NetworkBorderGroup", NETWORK_BORDER_GROUP_FIELD);
put("Ipv6Pool", IPV6_POOL_FIELD);
put("Ipv6AddressAttribute", IPV6_ADDRESS_ATTRIBUTE_FIELD);
put("IpSource", IP_SOURCE_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String associationId;
private final String ipv6CidrBlock;
private final VpcCidrBlockState ipv6CidrBlockState;
private final String networkBorderGroup;
private final String ipv6Pool;
private final String ipv6AddressAttribute;
private final String ipSource;
private VpcIpv6CidrBlockAssociation(BuilderImpl builder) {
this.associationId = builder.associationId;
this.ipv6CidrBlock = builder.ipv6CidrBlock;
this.ipv6CidrBlockState = builder.ipv6CidrBlockState;
this.networkBorderGroup = builder.networkBorderGroup;
this.ipv6Pool = builder.ipv6Pool;
this.ipv6AddressAttribute = builder.ipv6AddressAttribute;
this.ipSource = builder.ipSource;
}
/**
*
* The association ID for the IPv6 CIDR block.
*
*
* @return The association ID for the IPv6 CIDR block.
*/
public final String associationId() {
return associationId;
}
/**
*
* The IPv6 CIDR block.
*
*
* @return The IPv6 CIDR block.
*/
public final String ipv6CidrBlock() {
return ipv6CidrBlock;
}
/**
*
* Information about the state of the CIDR block.
*
*
* @return Information about the state of the CIDR block.
*/
public final VpcCidrBlockState ipv6CidrBlockState() {
return ipv6CidrBlockState;
}
/**
*
* The name of the unique set of Availability Zones, Local Zones, or Wavelength Zones from which Amazon Web Services
* advertises IP addresses, for example, us-east-1-wl1-bos-wlz-1
.
*
*
* @return The name of the unique set of Availability Zones, Local Zones, or Wavelength Zones from which Amazon Web
* Services advertises IP addresses, for example, us-east-1-wl1-bos-wlz-1
.
*/
public final String networkBorderGroup() {
return networkBorderGroup;
}
/**
*
* The ID of the IPv6 address pool from which the IPv6 CIDR block is allocated.
*
*
* @return The ID of the IPv6 address pool from which the IPv6 CIDR block is allocated.
*/
public final String ipv6Pool() {
return ipv6Pool;
}
/**
*
* Public IPv6 addresses are those advertised on the internet from Amazon Web Services. Private IP addresses are not
* and cannot be advertised on the internet from Amazon Web Services.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #ipv6AddressAttribute} will return {@link Ipv6AddressAttribute#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #ipv6AddressAttributeAsString}.
*
*
* @return Public IPv6 addresses are those advertised on the internet from Amazon Web Services. Private IP addresses
* are not and cannot be advertised on the internet from Amazon Web Services.
* @see Ipv6AddressAttribute
*/
public final Ipv6AddressAttribute ipv6AddressAttribute() {
return Ipv6AddressAttribute.fromValue(ipv6AddressAttribute);
}
/**
*
* Public IPv6 addresses are those advertised on the internet from Amazon Web Services. Private IP addresses are not
* and cannot be advertised on the internet from Amazon Web Services.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #ipv6AddressAttribute} will return {@link Ipv6AddressAttribute#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #ipv6AddressAttributeAsString}.
*
*
* @return Public IPv6 addresses are those advertised on the internet from Amazon Web Services. Private IP addresses
* are not and cannot be advertised on the internet from Amazon Web Services.
* @see Ipv6AddressAttribute
*/
public final String ipv6AddressAttributeAsString() {
return ipv6AddressAttribute;
}
/**
*
* The source that allocated the IP address space. byoip
or amazon
indicates public IP
* address space allocated by Amazon or space that you have allocated with Bring your own IP (BYOIP).
* none
indicates private space.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipSource} will
* return {@link IpSource#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipSourceAsString}.
*
*
* @return The source that allocated the IP address space. byoip
or amazon
indicates
* public IP address space allocated by Amazon or space that you have allocated with Bring your own IP
* (BYOIP). none
indicates private space.
* @see IpSource
*/
public final IpSource ipSource() {
return IpSource.fromValue(ipSource);
}
/**
*
* The source that allocated the IP address space. byoip
or amazon
indicates public IP
* address space allocated by Amazon or space that you have allocated with Bring your own IP (BYOIP).
* none
indicates private space.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipSource} will
* return {@link IpSource#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipSourceAsString}.
*
*
* @return The source that allocated the IP address space. byoip
or amazon
indicates
* public IP address space allocated by Amazon or space that you have allocated with Bring your own IP
* (BYOIP). none
indicates private space.
* @see IpSource
*/
public final String ipSourceAsString() {
return ipSource;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(associationId());
hashCode = 31 * hashCode + Objects.hashCode(ipv6CidrBlock());
hashCode = 31 * hashCode + Objects.hashCode(ipv6CidrBlockState());
hashCode = 31 * hashCode + Objects.hashCode(networkBorderGroup());
hashCode = 31 * hashCode + Objects.hashCode(ipv6Pool());
hashCode = 31 * hashCode + Objects.hashCode(ipv6AddressAttributeAsString());
hashCode = 31 * hashCode + Objects.hashCode(ipSourceAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof VpcIpv6CidrBlockAssociation)) {
return false;
}
VpcIpv6CidrBlockAssociation other = (VpcIpv6CidrBlockAssociation) obj;
return Objects.equals(associationId(), other.associationId()) && Objects.equals(ipv6CidrBlock(), other.ipv6CidrBlock())
&& Objects.equals(ipv6CidrBlockState(), other.ipv6CidrBlockState())
&& Objects.equals(networkBorderGroup(), other.networkBorderGroup())
&& Objects.equals(ipv6Pool(), other.ipv6Pool())
&& Objects.equals(ipv6AddressAttributeAsString(), other.ipv6AddressAttributeAsString())
&& Objects.equals(ipSourceAsString(), other.ipSourceAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("VpcIpv6CidrBlockAssociation").add("AssociationId", associationId())
.add("Ipv6CidrBlock", ipv6CidrBlock()).add("Ipv6CidrBlockState", ipv6CidrBlockState())
.add("NetworkBorderGroup", networkBorderGroup()).add("Ipv6Pool", ipv6Pool())
.add("Ipv6AddressAttribute", ipv6AddressAttributeAsString()).add("IpSource", ipSourceAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AssociationId":
return Optional.ofNullable(clazz.cast(associationId()));
case "Ipv6CidrBlock":
return Optional.ofNullable(clazz.cast(ipv6CidrBlock()));
case "Ipv6CidrBlockState":
return Optional.ofNullable(clazz.cast(ipv6CidrBlockState()));
case "NetworkBorderGroup":
return Optional.ofNullable(clazz.cast(networkBorderGroup()));
case "Ipv6Pool":
return Optional.ofNullable(clazz.cast(ipv6Pool()));
case "Ipv6AddressAttribute":
return Optional.ofNullable(clazz.cast(ipv6AddressAttributeAsString()));
case "IpSource":
return Optional.ofNullable(clazz.cast(ipSourceAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function