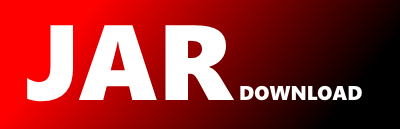
software.amazon.awssdk.services.ec2.model.VpcPeeringConnectionVpcInfo Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a VPC in a VPC peering connection.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class VpcPeeringConnectionVpcInfo implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CIDR_BLOCK_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CidrBlock")
.getter(getter(VpcPeeringConnectionVpcInfo::cidrBlock))
.setter(setter(Builder::cidrBlock))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CidrBlock")
.unmarshallLocationName("cidrBlock").build()).build();
private static final SdkField> IPV6_CIDR_BLOCK_SET_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Ipv6CidrBlockSet")
.getter(getter(VpcPeeringConnectionVpcInfo::ipv6CidrBlockSet))
.setter(setter(Builder::ipv6CidrBlockSet))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ipv6CidrBlockSet")
.unmarshallLocationName("ipv6CidrBlockSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Ipv6CidrBlock::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField> CIDR_BLOCK_SET_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CidrBlockSet")
.getter(getter(VpcPeeringConnectionVpcInfo::cidrBlockSet))
.setter(setter(Builder::cidrBlockSet))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CidrBlockSet")
.unmarshallLocationName("cidrBlockSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CidrBlock::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField OWNER_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OwnerId")
.getter(getter(VpcPeeringConnectionVpcInfo::ownerId))
.setter(setter(Builder::ownerId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OwnerId")
.unmarshallLocationName("ownerId").build()).build();
private static final SdkField PEERING_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("PeeringOptions")
.getter(getter(VpcPeeringConnectionVpcInfo::peeringOptions))
.setter(setter(Builder::peeringOptions))
.constructor(VpcPeeringConnectionOptionsDescription::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PeeringOptions")
.unmarshallLocationName("peeringOptions").build()).build();
private static final SdkField VPC_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VpcId")
.getter(getter(VpcPeeringConnectionVpcInfo::vpcId))
.setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId")
.unmarshallLocationName("vpcId").build()).build();
private static final SdkField REGION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Region")
.getter(getter(VpcPeeringConnectionVpcInfo::region))
.setter(setter(Builder::region))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Region")
.unmarshallLocationName("region").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CIDR_BLOCK_FIELD,
IPV6_CIDR_BLOCK_SET_FIELD, CIDR_BLOCK_SET_FIELD, OWNER_ID_FIELD, PEERING_OPTIONS_FIELD, VPC_ID_FIELD, REGION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("CidrBlock", CIDR_BLOCK_FIELD);
put("Ipv6CidrBlockSet", IPV6_CIDR_BLOCK_SET_FIELD);
put("CidrBlockSet", CIDR_BLOCK_SET_FIELD);
put("OwnerId", OWNER_ID_FIELD);
put("PeeringOptions", PEERING_OPTIONS_FIELD);
put("VpcId", VPC_ID_FIELD);
put("Region", REGION_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String cidrBlock;
private final List ipv6CidrBlockSet;
private final List cidrBlockSet;
private final String ownerId;
private final VpcPeeringConnectionOptionsDescription peeringOptions;
private final String vpcId;
private final String region;
private VpcPeeringConnectionVpcInfo(BuilderImpl builder) {
this.cidrBlock = builder.cidrBlock;
this.ipv6CidrBlockSet = builder.ipv6CidrBlockSet;
this.cidrBlockSet = builder.cidrBlockSet;
this.ownerId = builder.ownerId;
this.peeringOptions = builder.peeringOptions;
this.vpcId = builder.vpcId;
this.region = builder.region;
}
/**
*
* The IPv4 CIDR block for the VPC.
*
*
* @return The IPv4 CIDR block for the VPC.
*/
public final String cidrBlock() {
return cidrBlock;
}
/**
* For responses, this returns true if the service returned a value for the Ipv6CidrBlockSet property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasIpv6CidrBlockSet() {
return ipv6CidrBlockSet != null && !(ipv6CidrBlockSet instanceof SdkAutoConstructList);
}
/**
*
* The IPv6 CIDR block for the VPC.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasIpv6CidrBlockSet} method.
*
*
* @return The IPv6 CIDR block for the VPC.
*/
public final List ipv6CidrBlockSet() {
return ipv6CidrBlockSet;
}
/**
* For responses, this returns true if the service returned a value for the CidrBlockSet property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCidrBlockSet() {
return cidrBlockSet != null && !(cidrBlockSet instanceof SdkAutoConstructList);
}
/**
*
* Information about the IPv4 CIDR blocks for the VPC.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCidrBlockSet} method.
*
*
* @return Information about the IPv4 CIDR blocks for the VPC.
*/
public final List cidrBlockSet() {
return cidrBlockSet;
}
/**
*
* The ID of the Amazon Web Services account that owns the VPC.
*
*
* @return The ID of the Amazon Web Services account that owns the VPC.
*/
public final String ownerId() {
return ownerId;
}
/**
*
* Information about the VPC peering connection options for the accepter or requester VPC.
*
*
* @return Information about the VPC peering connection options for the accepter or requester VPC.
*/
public final VpcPeeringConnectionOptionsDescription peeringOptions() {
return peeringOptions;
}
/**
*
* The ID of the VPC.
*
*
* @return The ID of the VPC.
*/
public final String vpcId() {
return vpcId;
}
/**
*
* The Region in which the VPC is located.
*
*
* @return The Region in which the VPC is located.
*/
public final String region() {
return region;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(cidrBlock());
hashCode = 31 * hashCode + Objects.hashCode(hasIpv6CidrBlockSet() ? ipv6CidrBlockSet() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCidrBlockSet() ? cidrBlockSet() : null);
hashCode = 31 * hashCode + Objects.hashCode(ownerId());
hashCode = 31 * hashCode + Objects.hashCode(peeringOptions());
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
hashCode = 31 * hashCode + Objects.hashCode(region());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof VpcPeeringConnectionVpcInfo)) {
return false;
}
VpcPeeringConnectionVpcInfo other = (VpcPeeringConnectionVpcInfo) obj;
return Objects.equals(cidrBlock(), other.cidrBlock()) && hasIpv6CidrBlockSet() == other.hasIpv6CidrBlockSet()
&& Objects.equals(ipv6CidrBlockSet(), other.ipv6CidrBlockSet()) && hasCidrBlockSet() == other.hasCidrBlockSet()
&& Objects.equals(cidrBlockSet(), other.cidrBlockSet()) && Objects.equals(ownerId(), other.ownerId())
&& Objects.equals(peeringOptions(), other.peeringOptions()) && Objects.equals(vpcId(), other.vpcId())
&& Objects.equals(region(), other.region());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("VpcPeeringConnectionVpcInfo").add("CidrBlock", cidrBlock())
.add("Ipv6CidrBlockSet", hasIpv6CidrBlockSet() ? ipv6CidrBlockSet() : null)
.add("CidrBlockSet", hasCidrBlockSet() ? cidrBlockSet() : null).add("OwnerId", ownerId())
.add("PeeringOptions", peeringOptions()).add("VpcId", vpcId()).add("Region", region()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CidrBlock":
return Optional.ofNullable(clazz.cast(cidrBlock()));
case "Ipv6CidrBlockSet":
return Optional.ofNullable(clazz.cast(ipv6CidrBlockSet()));
case "CidrBlockSet":
return Optional.ofNullable(clazz.cast(cidrBlockSet()));
case "OwnerId":
return Optional.ofNullable(clazz.cast(ownerId()));
case "PeeringOptions":
return Optional.ofNullable(clazz.cast(peeringOptions()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
case "Region":
return Optional.ofNullable(clazz.cast(region()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function