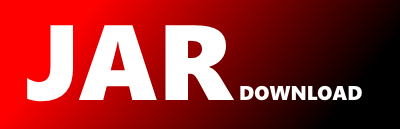
software.amazon.awssdk.services.ec2.waiters.Ec2Waiter Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.waiters;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.Immutable;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.waiters.WaiterOverrideConfiguration;
import software.amazon.awssdk.core.waiters.WaiterResponse;
import software.amazon.awssdk.services.ec2.Ec2Client;
import software.amazon.awssdk.services.ec2.model.DescribeBundleTasksRequest;
import software.amazon.awssdk.services.ec2.model.DescribeBundleTasksResponse;
import software.amazon.awssdk.services.ec2.model.DescribeConversionTasksRequest;
import software.amazon.awssdk.services.ec2.model.DescribeConversionTasksResponse;
import software.amazon.awssdk.services.ec2.model.DescribeCustomerGatewaysRequest;
import software.amazon.awssdk.services.ec2.model.DescribeCustomerGatewaysResponse;
import software.amazon.awssdk.services.ec2.model.DescribeExportTasksRequest;
import software.amazon.awssdk.services.ec2.model.DescribeExportTasksResponse;
import software.amazon.awssdk.services.ec2.model.DescribeImagesRequest;
import software.amazon.awssdk.services.ec2.model.DescribeImagesResponse;
import software.amazon.awssdk.services.ec2.model.DescribeImportSnapshotTasksRequest;
import software.amazon.awssdk.services.ec2.model.DescribeImportSnapshotTasksResponse;
import software.amazon.awssdk.services.ec2.model.DescribeInstanceStatusRequest;
import software.amazon.awssdk.services.ec2.model.DescribeInstanceStatusResponse;
import software.amazon.awssdk.services.ec2.model.DescribeInstancesRequest;
import software.amazon.awssdk.services.ec2.model.DescribeInstancesResponse;
import software.amazon.awssdk.services.ec2.model.DescribeInternetGatewaysRequest;
import software.amazon.awssdk.services.ec2.model.DescribeInternetGatewaysResponse;
import software.amazon.awssdk.services.ec2.model.DescribeKeyPairsRequest;
import software.amazon.awssdk.services.ec2.model.DescribeKeyPairsResponse;
import software.amazon.awssdk.services.ec2.model.DescribeNatGatewaysRequest;
import software.amazon.awssdk.services.ec2.model.DescribeNatGatewaysResponse;
import software.amazon.awssdk.services.ec2.model.DescribeNetworkInterfacesRequest;
import software.amazon.awssdk.services.ec2.model.DescribeNetworkInterfacesResponse;
import software.amazon.awssdk.services.ec2.model.DescribeSecurityGroupsRequest;
import software.amazon.awssdk.services.ec2.model.DescribeSecurityGroupsResponse;
import software.amazon.awssdk.services.ec2.model.DescribeSnapshotsRequest;
import software.amazon.awssdk.services.ec2.model.DescribeSnapshotsResponse;
import software.amazon.awssdk.services.ec2.model.DescribeSpotInstanceRequestsRequest;
import software.amazon.awssdk.services.ec2.model.DescribeSpotInstanceRequestsResponse;
import software.amazon.awssdk.services.ec2.model.DescribeStoreImageTasksRequest;
import software.amazon.awssdk.services.ec2.model.DescribeStoreImageTasksResponse;
import software.amazon.awssdk.services.ec2.model.DescribeSubnetsRequest;
import software.amazon.awssdk.services.ec2.model.DescribeSubnetsResponse;
import software.amazon.awssdk.services.ec2.model.DescribeVolumesRequest;
import software.amazon.awssdk.services.ec2.model.DescribeVolumesResponse;
import software.amazon.awssdk.services.ec2.model.DescribeVpcPeeringConnectionsRequest;
import software.amazon.awssdk.services.ec2.model.DescribeVpcPeeringConnectionsResponse;
import software.amazon.awssdk.services.ec2.model.DescribeVpcsRequest;
import software.amazon.awssdk.services.ec2.model.DescribeVpcsResponse;
import software.amazon.awssdk.services.ec2.model.DescribeVpnConnectionsRequest;
import software.amazon.awssdk.services.ec2.model.DescribeVpnConnectionsResponse;
import software.amazon.awssdk.services.ec2.model.GetPasswordDataRequest;
import software.amazon.awssdk.services.ec2.model.GetPasswordDataResponse;
import software.amazon.awssdk.utils.SdkAutoCloseable;
/**
* Waiter utility class that polls a resource until a desired state is reached or until it is determined that the
* resource will never enter into the desired state. This can be created using the static {@link #builder()} method
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
@Immutable
public interface Ec2Waiter extends SdkAutoCloseable {
/**
* Polls {@link Ec2Client#describeBundleTasks} API until the desired condition {@code BundleTaskComplete} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeBundleTasksRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilBundleTaskComplete(
DescribeBundleTasksRequest describeBundleTasksRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeBundleTasks} API until the desired condition {@code BundleTaskComplete} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeBundleTasksRequest#builder()}
*
* @param describeBundleTasksRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilBundleTaskComplete(
Consumer describeBundleTasksRequest) {
return waitUntilBundleTaskComplete(DescribeBundleTasksRequest.builder().applyMutation(describeBundleTasksRequest).build());
}
/**
* Polls {@link Ec2Client#describeBundleTasks} API until the desired condition {@code BundleTaskComplete} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeBundleTasksRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilBundleTaskComplete(
DescribeBundleTasksRequest describeBundleTasksRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeBundleTasks} API until the desired condition {@code BundleTaskComplete} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeBundleTasksRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilBundleTaskComplete(
Consumer describeBundleTasksRequest,
Consumer overrideConfig) {
return waitUntilBundleTaskComplete(
DescribeBundleTasksRequest.builder().applyMutation(describeBundleTasksRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskCancelled}
* is met, or until it is determined that the resource will never enter into the desired state
*
* @param describeConversionTasksRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskCancelled(
DescribeConversionTasksRequest describeConversionTasksRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskCancelled}
* is met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeConversionTasksRequest#builder()}
*
* @param describeConversionTasksRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskCancelled(
Consumer describeConversionTasksRequest) {
return waitUntilConversionTaskCancelled(DescribeConversionTasksRequest.builder()
.applyMutation(describeConversionTasksRequest).build());
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskCancelled}
* is met, or until it is determined that the resource will never enter into the desired state
*
* @param describeConversionTasksRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskCancelled(
DescribeConversionTasksRequest describeConversionTasksRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskCancelled}
* is met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeConversionTasksRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskCancelled(
Consumer describeConversionTasksRequest,
Consumer overrideConfig) {
return waitUntilConversionTaskCancelled(
DescribeConversionTasksRequest.builder().applyMutation(describeConversionTasksRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskCompleted}
* is met, or until it is determined that the resource will never enter into the desired state
*
* @param describeConversionTasksRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskCompleted(
DescribeConversionTasksRequest describeConversionTasksRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskCompleted}
* is met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeConversionTasksRequest#builder()}
*
* @param describeConversionTasksRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskCompleted(
Consumer describeConversionTasksRequest) {
return waitUntilConversionTaskCompleted(DescribeConversionTasksRequest.builder()
.applyMutation(describeConversionTasksRequest).build());
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskCompleted}
* is met, or until it is determined that the resource will never enter into the desired state
*
* @param describeConversionTasksRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskCompleted(
DescribeConversionTasksRequest describeConversionTasksRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskCompleted}
* is met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeConversionTasksRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskCompleted(
Consumer describeConversionTasksRequest,
Consumer overrideConfig) {
return waitUntilConversionTaskCompleted(
DescribeConversionTasksRequest.builder().applyMutation(describeConversionTasksRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskDeleted} is
* met, or until it is determined that the resource will never enter into the desired state
*
* @param describeConversionTasksRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskDeleted(
DescribeConversionTasksRequest describeConversionTasksRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskDeleted} is
* met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeConversionTasksRequest#builder()}
*
* @param describeConversionTasksRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskDeleted(
Consumer describeConversionTasksRequest) {
return waitUntilConversionTaskDeleted(DescribeConversionTasksRequest.builder()
.applyMutation(describeConversionTasksRequest).build());
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskDeleted} is
* met, or until it is determined that the resource will never enter into the desired state
*
* @param describeConversionTasksRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskDeleted(
DescribeConversionTasksRequest describeConversionTasksRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeConversionTasks} API until the desired condition {@code ConversionTaskDeleted} is
* met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeConversionTasksRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilConversionTaskDeleted(
Consumer describeConversionTasksRequest,
Consumer overrideConfig) {
return waitUntilConversionTaskDeleted(
DescribeConversionTasksRequest.builder().applyMutation(describeConversionTasksRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeCustomerGateways} API until the desired condition {@code CustomerGatewayAvailable}
* is met, or until it is determined that the resource will never enter into the desired state
*
* @param describeCustomerGatewaysRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilCustomerGatewayAvailable(
DescribeCustomerGatewaysRequest describeCustomerGatewaysRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeCustomerGateways} API until the desired condition {@code CustomerGatewayAvailable}
* is met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeCustomerGatewaysRequest#builder()}
*
* @param describeCustomerGatewaysRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilCustomerGatewayAvailable(
Consumer describeCustomerGatewaysRequest) {
return waitUntilCustomerGatewayAvailable(DescribeCustomerGatewaysRequest.builder()
.applyMutation(describeCustomerGatewaysRequest).build());
}
/**
* Polls {@link Ec2Client#describeCustomerGateways} API until the desired condition {@code CustomerGatewayAvailable}
* is met, or until it is determined that the resource will never enter into the desired state
*
* @param describeCustomerGatewaysRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilCustomerGatewayAvailable(
DescribeCustomerGatewaysRequest describeCustomerGatewaysRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeCustomerGateways} API until the desired condition {@code CustomerGatewayAvailable}
* is met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeCustomerGatewaysRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilCustomerGatewayAvailable(
Consumer describeCustomerGatewaysRequest,
Consumer overrideConfig) {
return waitUntilCustomerGatewayAvailable(
DescribeCustomerGatewaysRequest.builder().applyMutation(describeCustomerGatewaysRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeExportTasks} API until the desired condition {@code ExportTaskCancelled} is met,
* or until it is determined that the resource will never enter into the desired state
*
* @param describeExportTasksRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilExportTaskCancelled(
DescribeExportTasksRequest describeExportTasksRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeExportTasks} API until the desired condition {@code ExportTaskCancelled} is met,
* or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeExportTasksRequest#builder()}
*
* @param describeExportTasksRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilExportTaskCancelled(
Consumer describeExportTasksRequest) {
return waitUntilExportTaskCancelled(DescribeExportTasksRequest.builder().applyMutation(describeExportTasksRequest)
.build());
}
/**
* Polls {@link Ec2Client#describeExportTasks} API until the desired condition {@code ExportTaskCancelled} is met,
* or until it is determined that the resource will never enter into the desired state
*
* @param describeExportTasksRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilExportTaskCancelled(
DescribeExportTasksRequest describeExportTasksRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeExportTasks} API until the desired condition {@code ExportTaskCancelled} is met,
* or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeExportTasksRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilExportTaskCancelled(
Consumer describeExportTasksRequest,
Consumer overrideConfig) {
return waitUntilExportTaskCancelled(DescribeExportTasksRequest.builder().applyMutation(describeExportTasksRequest)
.build(), WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeExportTasks} API until the desired condition {@code ExportTaskCompleted} is met,
* or until it is determined that the resource will never enter into the desired state
*
* @param describeExportTasksRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilExportTaskCompleted(
DescribeExportTasksRequest describeExportTasksRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeExportTasks} API until the desired condition {@code ExportTaskCompleted} is met,
* or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeExportTasksRequest#builder()}
*
* @param describeExportTasksRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilExportTaskCompleted(
Consumer describeExportTasksRequest) {
return waitUntilExportTaskCompleted(DescribeExportTasksRequest.builder().applyMutation(describeExportTasksRequest)
.build());
}
/**
* Polls {@link Ec2Client#describeExportTasks} API until the desired condition {@code ExportTaskCompleted} is met,
* or until it is determined that the resource will never enter into the desired state
*
* @param describeExportTasksRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilExportTaskCompleted(
DescribeExportTasksRequest describeExportTasksRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeExportTasks} API until the desired condition {@code ExportTaskCompleted} is met,
* or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeExportTasksRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilExportTaskCompleted(
Consumer describeExportTasksRequest,
Consumer overrideConfig) {
return waitUntilExportTaskCompleted(DescribeExportTasksRequest.builder().applyMutation(describeExportTasksRequest)
.build(), WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeImages} API until the desired condition {@code ImageAvailable} is met, or until it
* is determined that the resource will never enter into the desired state
*
* @param describeImagesRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilImageAvailable(DescribeImagesRequest describeImagesRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeImages} API until the desired condition {@code ImageAvailable} is met, or until it
* is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeImagesRequest#builder()}
*
* @param describeImagesRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilImageAvailable(
Consumer describeImagesRequest) {
return waitUntilImageAvailable(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build());
}
/**
* Polls {@link Ec2Client#describeImages} API until the desired condition {@code ImageAvailable} is met, or until it
* is determined that the resource will never enter into the desired state
*
* @param describeImagesRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilImageAvailable(DescribeImagesRequest describeImagesRequest,
WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeImages} API until the desired condition {@code ImageAvailable} is met, or until it
* is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeImagesRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilImageAvailable(
Consumer describeImagesRequest,
Consumer overrideConfig) {
return waitUntilImageAvailable(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeImages} API until the desired condition {@code ImageExists} is met, or until it is
* determined that the resource will never enter into the desired state
*
* @param describeImagesRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilImageExists(DescribeImagesRequest describeImagesRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeImages} API until the desired condition {@code ImageExists} is met, or until it is
* determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeImagesRequest#builder()}
*
* @param describeImagesRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilImageExists(
Consumer describeImagesRequest) {
return waitUntilImageExists(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build());
}
/**
* Polls {@link Ec2Client#describeImages} API until the desired condition {@code ImageExists} is met, or until it is
* determined that the resource will never enter into the desired state
*
* @param describeImagesRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilImageExists(DescribeImagesRequest describeImagesRequest,
WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeImages} API until the desired condition {@code ImageExists} is met, or until it is
* determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeImagesRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilImageExists(
Consumer describeImagesRequest,
Consumer overrideConfig) {
return waitUntilImageExists(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceExists} is met, or until
* it is determined that the resource will never enter into the desired state
*
* @param describeInstancesRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceExists(DescribeInstancesRequest describeInstancesRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceExists} is met, or until
* it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeInstancesRequest#builder()}
*
* @param describeInstancesRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceExists(
Consumer describeInstancesRequest) {
return waitUntilInstanceExists(DescribeInstancesRequest.builder().applyMutation(describeInstancesRequest).build());
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceExists} is met, or until
* it is determined that the resource will never enter into the desired state
*
* @param describeInstancesRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceExists(DescribeInstancesRequest describeInstancesRequest,
WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceExists} is met, or until
* it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeInstancesRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceExists(
Consumer describeInstancesRequest,
Consumer overrideConfig) {
return waitUntilInstanceExists(DescribeInstancesRequest.builder().applyMutation(describeInstancesRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceRunning} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeInstancesRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceRunning(DescribeInstancesRequest describeInstancesRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceRunning} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeInstancesRequest#builder()}
*
* @param describeInstancesRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceRunning(
Consumer describeInstancesRequest) {
return waitUntilInstanceRunning(DescribeInstancesRequest.builder().applyMutation(describeInstancesRequest).build());
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceRunning} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeInstancesRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceRunning(DescribeInstancesRequest describeInstancesRequest,
WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceRunning} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeInstancesRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceRunning(
Consumer describeInstancesRequest,
Consumer overrideConfig) {
return waitUntilInstanceRunning(DescribeInstancesRequest.builder().applyMutation(describeInstancesRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeInstanceStatus} API until the desired condition {@code InstanceStatusOk} is met,
* or until it is determined that the resource will never enter into the desired state
*
* @param describeInstanceStatusRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceStatusOk(
DescribeInstanceStatusRequest describeInstanceStatusRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstanceStatus} API until the desired condition {@code InstanceStatusOk} is met,
* or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeInstanceStatusRequest#builder()}
*
* @param describeInstanceStatusRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceStatusOk(
Consumer describeInstanceStatusRequest) {
return waitUntilInstanceStatusOk(DescribeInstanceStatusRequest.builder().applyMutation(describeInstanceStatusRequest)
.build());
}
/**
* Polls {@link Ec2Client#describeInstanceStatus} API until the desired condition {@code InstanceStatusOk} is met,
* or until it is determined that the resource will never enter into the desired state
*
* @param describeInstanceStatusRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceStatusOk(
DescribeInstanceStatusRequest describeInstanceStatusRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstanceStatus} API until the desired condition {@code InstanceStatusOk} is met,
* or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeInstanceStatusRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceStatusOk(
Consumer describeInstanceStatusRequest,
Consumer overrideConfig) {
return waitUntilInstanceStatusOk(DescribeInstanceStatusRequest.builder().applyMutation(describeInstanceStatusRequest)
.build(), WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceStopped} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeInstancesRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceStopped(DescribeInstancesRequest describeInstancesRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceStopped} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeInstancesRequest#builder()}
*
* @param describeInstancesRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceStopped(
Consumer describeInstancesRequest) {
return waitUntilInstanceStopped(DescribeInstancesRequest.builder().applyMutation(describeInstancesRequest).build());
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceStopped} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeInstancesRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceStopped(DescribeInstancesRequest describeInstancesRequest,
WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceStopped} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeInstancesRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceStopped(
Consumer describeInstancesRequest,
Consumer overrideConfig) {
return waitUntilInstanceStopped(DescribeInstancesRequest.builder().applyMutation(describeInstancesRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceTerminated} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeInstancesRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceTerminated(
DescribeInstancesRequest describeInstancesRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceTerminated} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeInstancesRequest#builder()}
*
* @param describeInstancesRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceTerminated(
Consumer describeInstancesRequest) {
return waitUntilInstanceTerminated(DescribeInstancesRequest.builder().applyMutation(describeInstancesRequest).build());
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceTerminated} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeInstancesRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceTerminated(
DescribeInstancesRequest describeInstancesRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstances} API until the desired condition {@code InstanceTerminated} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeInstancesRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInstanceTerminated(
Consumer describeInstancesRequest,
Consumer overrideConfig) {
return waitUntilInstanceTerminated(DescribeInstancesRequest.builder().applyMutation(describeInstancesRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeInternetGateways} API until the desired condition {@code InternetGatewayExists} is
* met, or until it is determined that the resource will never enter into the desired state
*
* @param describeInternetGatewaysRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInternetGatewayExists(
DescribeInternetGatewaysRequest describeInternetGatewaysRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInternetGateways} API until the desired condition {@code InternetGatewayExists} is
* met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeInternetGatewaysRequest#builder()}
*
* @param describeInternetGatewaysRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInternetGatewayExists(
Consumer describeInternetGatewaysRequest) {
return waitUntilInternetGatewayExists(DescribeInternetGatewaysRequest.builder()
.applyMutation(describeInternetGatewaysRequest).build());
}
/**
* Polls {@link Ec2Client#describeInternetGateways} API until the desired condition {@code InternetGatewayExists} is
* met, or until it is determined that the resource will never enter into the desired state
*
* @param describeInternetGatewaysRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInternetGatewayExists(
DescribeInternetGatewaysRequest describeInternetGatewaysRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInternetGateways} API until the desired condition {@code InternetGatewayExists} is
* met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeInternetGatewaysRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilInternetGatewayExists(
Consumer describeInternetGatewaysRequest,
Consumer overrideConfig) {
return waitUntilInternetGatewayExists(
DescribeInternetGatewaysRequest.builder().applyMutation(describeInternetGatewaysRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeKeyPairs} API until the desired condition {@code KeyPairExists} is met, or until
* it is determined that the resource will never enter into the desired state
*
* @param describeKeyPairsRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilKeyPairExists(DescribeKeyPairsRequest describeKeyPairsRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeKeyPairs} API until the desired condition {@code KeyPairExists} is met, or until
* it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeKeyPairsRequest#builder()}
*
* @param describeKeyPairsRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilKeyPairExists(
Consumer describeKeyPairsRequest) {
return waitUntilKeyPairExists(DescribeKeyPairsRequest.builder().applyMutation(describeKeyPairsRequest).build());
}
/**
* Polls {@link Ec2Client#describeKeyPairs} API until the desired condition {@code KeyPairExists} is met, or until
* it is determined that the resource will never enter into the desired state
*
* @param describeKeyPairsRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilKeyPairExists(DescribeKeyPairsRequest describeKeyPairsRequest,
WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeKeyPairs} API until the desired condition {@code KeyPairExists} is met, or until
* it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeKeyPairsRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilKeyPairExists(
Consumer describeKeyPairsRequest,
Consumer overrideConfig) {
return waitUntilKeyPairExists(DescribeKeyPairsRequest.builder().applyMutation(describeKeyPairsRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeNatGateways} API until the desired condition {@code NatGatewayAvailable} is met,
* or until it is determined that the resource will never enter into the desired state
*
* @param describeNatGatewaysRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNatGatewayAvailable(
DescribeNatGatewaysRequest describeNatGatewaysRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeNatGateways} API until the desired condition {@code NatGatewayAvailable} is met,
* or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeNatGatewaysRequest#builder()}
*
* @param describeNatGatewaysRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNatGatewayAvailable(
Consumer describeNatGatewaysRequest) {
return waitUntilNatGatewayAvailable(DescribeNatGatewaysRequest.builder().applyMutation(describeNatGatewaysRequest)
.build());
}
/**
* Polls {@link Ec2Client#describeNatGateways} API until the desired condition {@code NatGatewayAvailable} is met,
* or until it is determined that the resource will never enter into the desired state
*
* @param describeNatGatewaysRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNatGatewayAvailable(
DescribeNatGatewaysRequest describeNatGatewaysRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeNatGateways} API until the desired condition {@code NatGatewayAvailable} is met,
* or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeNatGatewaysRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNatGatewayAvailable(
Consumer describeNatGatewaysRequest,
Consumer overrideConfig) {
return waitUntilNatGatewayAvailable(DescribeNatGatewaysRequest.builder().applyMutation(describeNatGatewaysRequest)
.build(), WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeNatGateways} API until the desired condition {@code NatGatewayDeleted} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeNatGatewaysRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNatGatewayDeleted(
DescribeNatGatewaysRequest describeNatGatewaysRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeNatGateways} API until the desired condition {@code NatGatewayDeleted} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeNatGatewaysRequest#builder()}
*
* @param describeNatGatewaysRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNatGatewayDeleted(
Consumer describeNatGatewaysRequest) {
return waitUntilNatGatewayDeleted(DescribeNatGatewaysRequest.builder().applyMutation(describeNatGatewaysRequest).build());
}
/**
* Polls {@link Ec2Client#describeNatGateways} API until the desired condition {@code NatGatewayDeleted} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeNatGatewaysRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNatGatewayDeleted(
DescribeNatGatewaysRequest describeNatGatewaysRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeNatGateways} API until the desired condition {@code NatGatewayDeleted} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeNatGatewaysRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNatGatewayDeleted(
Consumer describeNatGatewaysRequest,
Consumer overrideConfig) {
return waitUntilNatGatewayDeleted(DescribeNatGatewaysRequest.builder().applyMutation(describeNatGatewaysRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeNetworkInterfaces} API until the desired condition
* {@code NetworkInterfaceAvailable} is met, or until it is determined that the resource will never enter into the
* desired state
*
* @param describeNetworkInterfacesRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNetworkInterfaceAvailable(
DescribeNetworkInterfacesRequest describeNetworkInterfacesRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeNetworkInterfaces} API until the desired condition
* {@code NetworkInterfaceAvailable} is met, or until it is determined that the resource will never enter into the
* desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeNetworkInterfacesRequest#builder()}
*
* @param describeNetworkInterfacesRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNetworkInterfaceAvailable(
Consumer describeNetworkInterfacesRequest) {
return waitUntilNetworkInterfaceAvailable(DescribeNetworkInterfacesRequest.builder()
.applyMutation(describeNetworkInterfacesRequest).build());
}
/**
* Polls {@link Ec2Client#describeNetworkInterfaces} API until the desired condition
* {@code NetworkInterfaceAvailable} is met, or until it is determined that the resource will never enter into the
* desired state
*
* @param describeNetworkInterfacesRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNetworkInterfaceAvailable(
DescribeNetworkInterfacesRequest describeNetworkInterfacesRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeNetworkInterfaces} API until the desired condition
* {@code NetworkInterfaceAvailable} is met, or until it is determined that the resource will never enter into the
* desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeNetworkInterfacesRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilNetworkInterfaceAvailable(
Consumer describeNetworkInterfacesRequest,
Consumer overrideConfig) {
return waitUntilNetworkInterfaceAvailable(
DescribeNetworkInterfacesRequest.builder().applyMutation(describeNetworkInterfacesRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#getPasswordData} API until the desired condition {@code PasswordDataAvailable} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param getPasswordDataRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilPasswordDataAvailable(GetPasswordDataRequest getPasswordDataRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#getPasswordData} API until the desired condition {@code PasswordDataAvailable} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link GetPasswordDataRequest#builder()}
*
* @param getPasswordDataRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilPasswordDataAvailable(
Consumer getPasswordDataRequest) {
return waitUntilPasswordDataAvailable(GetPasswordDataRequest.builder().applyMutation(getPasswordDataRequest).build());
}
/**
* Polls {@link Ec2Client#getPasswordData} API until the desired condition {@code PasswordDataAvailable} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param getPasswordDataRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilPasswordDataAvailable(GetPasswordDataRequest getPasswordDataRequest,
WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#getPasswordData} API until the desired condition {@code PasswordDataAvailable} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param getPasswordDataRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilPasswordDataAvailable(
Consumer getPasswordDataRequest,
Consumer overrideConfig) {
return waitUntilPasswordDataAvailable(GetPasswordDataRequest.builder().applyMutation(getPasswordDataRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeSecurityGroups} API until the desired condition {@code SecurityGroupExists} is
* met, or until it is determined that the resource will never enter into the desired state
*
* @param describeSecurityGroupsRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSecurityGroupExists(
DescribeSecurityGroupsRequest describeSecurityGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeSecurityGroups} API until the desired condition {@code SecurityGroupExists} is
* met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeSecurityGroupsRequest#builder()}
*
* @param describeSecurityGroupsRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSecurityGroupExists(
Consumer describeSecurityGroupsRequest) {
return waitUntilSecurityGroupExists(DescribeSecurityGroupsRequest.builder().applyMutation(describeSecurityGroupsRequest)
.build());
}
/**
* Polls {@link Ec2Client#describeSecurityGroups} API until the desired condition {@code SecurityGroupExists} is
* met, or until it is determined that the resource will never enter into the desired state
*
* @param describeSecurityGroupsRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSecurityGroupExists(
DescribeSecurityGroupsRequest describeSecurityGroupsRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeSecurityGroups} API until the desired condition {@code SecurityGroupExists} is
* met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeSecurityGroupsRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSecurityGroupExists(
Consumer describeSecurityGroupsRequest,
Consumer overrideConfig) {
return waitUntilSecurityGroupExists(DescribeSecurityGroupsRequest.builder().applyMutation(describeSecurityGroupsRequest)
.build(), WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeSnapshots} API until the desired condition {@code SnapshotCompleted} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeSnapshotsRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSnapshotCompleted(DescribeSnapshotsRequest describeSnapshotsRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeSnapshots} API until the desired condition {@code SnapshotCompleted} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeSnapshotsRequest#builder()}
*
* @param describeSnapshotsRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSnapshotCompleted(
Consumer describeSnapshotsRequest) {
return waitUntilSnapshotCompleted(DescribeSnapshotsRequest.builder().applyMutation(describeSnapshotsRequest).build());
}
/**
* Polls {@link Ec2Client#describeSnapshots} API until the desired condition {@code SnapshotCompleted} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeSnapshotsRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSnapshotCompleted(
DescribeSnapshotsRequest describeSnapshotsRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeSnapshots} API until the desired condition {@code SnapshotCompleted} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeSnapshotsRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSnapshotCompleted(
Consumer describeSnapshotsRequest,
Consumer overrideConfig) {
return waitUntilSnapshotCompleted(DescribeSnapshotsRequest.builder().applyMutation(describeSnapshotsRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeImportSnapshotTasks} API until the desired condition {@code SnapshotImported} is
* met, or until it is determined that the resource will never enter into the desired state
*
* @param describeImportSnapshotTasksRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSnapshotImported(
DescribeImportSnapshotTasksRequest describeImportSnapshotTasksRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeImportSnapshotTasks} API until the desired condition {@code SnapshotImported} is
* met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeImportSnapshotTasksRequest#builder()}
*
* @param describeImportSnapshotTasksRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSnapshotImported(
Consumer describeImportSnapshotTasksRequest) {
return waitUntilSnapshotImported(DescribeImportSnapshotTasksRequest.builder()
.applyMutation(describeImportSnapshotTasksRequest).build());
}
/**
* Polls {@link Ec2Client#describeImportSnapshotTasks} API until the desired condition {@code SnapshotImported} is
* met, or until it is determined that the resource will never enter into the desired state
*
* @param describeImportSnapshotTasksRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSnapshotImported(
DescribeImportSnapshotTasksRequest describeImportSnapshotTasksRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeImportSnapshotTasks} API until the desired condition {@code SnapshotImported} is
* met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeImportSnapshotTasksRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSnapshotImported(
Consumer describeImportSnapshotTasksRequest,
Consumer overrideConfig) {
return waitUntilSnapshotImported(
DescribeImportSnapshotTasksRequest.builder().applyMutation(describeImportSnapshotTasksRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeSpotInstanceRequests} API until the desired condition
* {@code SpotInstanceRequestFulfilled} is met, or until it is determined that the resource will never enter into
* the desired state
*
* @param describeSpotInstanceRequestsRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSpotInstanceRequestFulfilled(
DescribeSpotInstanceRequestsRequest describeSpotInstanceRequestsRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeSpotInstanceRequests} API until the desired condition
* {@code SpotInstanceRequestFulfilled} is met, or until it is determined that the resource will never enter into
* the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeSpotInstanceRequestsRequest#builder()}
*
* @param describeSpotInstanceRequestsRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSpotInstanceRequestFulfilled(
Consumer describeSpotInstanceRequestsRequest) {
return waitUntilSpotInstanceRequestFulfilled(DescribeSpotInstanceRequestsRequest.builder()
.applyMutation(describeSpotInstanceRequestsRequest).build());
}
/**
* Polls {@link Ec2Client#describeSpotInstanceRequests} API until the desired condition
* {@code SpotInstanceRequestFulfilled} is met, or until it is determined that the resource will never enter into
* the desired state
*
* @param describeSpotInstanceRequestsRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSpotInstanceRequestFulfilled(
DescribeSpotInstanceRequestsRequest describeSpotInstanceRequestsRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeSpotInstanceRequests} API until the desired condition
* {@code SpotInstanceRequestFulfilled} is met, or until it is determined that the resource will never enter into
* the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeSpotInstanceRequestsRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSpotInstanceRequestFulfilled(
Consumer describeSpotInstanceRequestsRequest,
Consumer overrideConfig) {
return waitUntilSpotInstanceRequestFulfilled(
DescribeSpotInstanceRequestsRequest.builder().applyMutation(describeSpotInstanceRequestsRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeStoreImageTasks} API until the desired condition {@code StoreImageTaskComplete} is
* met, or until it is determined that the resource will never enter into the desired state
*
* @param describeStoreImageTasksRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilStoreImageTaskComplete(
DescribeStoreImageTasksRequest describeStoreImageTasksRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeStoreImageTasks} API until the desired condition {@code StoreImageTaskComplete} is
* met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeStoreImageTasksRequest#builder()}
*
* @param describeStoreImageTasksRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilStoreImageTaskComplete(
Consumer describeStoreImageTasksRequest) {
return waitUntilStoreImageTaskComplete(DescribeStoreImageTasksRequest.builder()
.applyMutation(describeStoreImageTasksRequest).build());
}
/**
* Polls {@link Ec2Client#describeStoreImageTasks} API until the desired condition {@code StoreImageTaskComplete} is
* met, or until it is determined that the resource will never enter into the desired state
*
* @param describeStoreImageTasksRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilStoreImageTaskComplete(
DescribeStoreImageTasksRequest describeStoreImageTasksRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeStoreImageTasks} API until the desired condition {@code StoreImageTaskComplete} is
* met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeStoreImageTasksRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilStoreImageTaskComplete(
Consumer describeStoreImageTasksRequest,
Consumer overrideConfig) {
return waitUntilStoreImageTaskComplete(
DescribeStoreImageTasksRequest.builder().applyMutation(describeStoreImageTasksRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeSubnets} API until the desired condition {@code SubnetAvailable} is met, or until
* it is determined that the resource will never enter into the desired state
*
* @param describeSubnetsRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSubnetAvailable(DescribeSubnetsRequest describeSubnetsRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeSubnets} API until the desired condition {@code SubnetAvailable} is met, or until
* it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeSubnetsRequest#builder()}
*
* @param describeSubnetsRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSubnetAvailable(
Consumer describeSubnetsRequest) {
return waitUntilSubnetAvailable(DescribeSubnetsRequest.builder().applyMutation(describeSubnetsRequest).build());
}
/**
* Polls {@link Ec2Client#describeSubnets} API until the desired condition {@code SubnetAvailable} is met, or until
* it is determined that the resource will never enter into the desired state
*
* @param describeSubnetsRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSubnetAvailable(DescribeSubnetsRequest describeSubnetsRequest,
WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeSubnets} API until the desired condition {@code SubnetAvailable} is met, or until
* it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeSubnetsRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSubnetAvailable(
Consumer describeSubnetsRequest,
Consumer overrideConfig) {
return waitUntilSubnetAvailable(DescribeSubnetsRequest.builder().applyMutation(describeSubnetsRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeInstanceStatus} API until the desired condition {@code SystemStatusOk} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeInstanceStatusRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSystemStatusOk(
DescribeInstanceStatusRequest describeInstanceStatusRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstanceStatus} API until the desired condition {@code SystemStatusOk} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeInstanceStatusRequest#builder()}
*
* @param describeInstanceStatusRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSystemStatusOk(
Consumer describeInstanceStatusRequest) {
return waitUntilSystemStatusOk(DescribeInstanceStatusRequest.builder().applyMutation(describeInstanceStatusRequest)
.build());
}
/**
* Polls {@link Ec2Client#describeInstanceStatus} API until the desired condition {@code SystemStatusOk} is met, or
* until it is determined that the resource will never enter into the desired state
*
* @param describeInstanceStatusRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSystemStatusOk(
DescribeInstanceStatusRequest describeInstanceStatusRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeInstanceStatus} API until the desired condition {@code SystemStatusOk} is met, or
* until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeInstanceStatusRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilSystemStatusOk(
Consumer describeInstanceStatusRequest,
Consumer overrideConfig) {
return waitUntilSystemStatusOk(DescribeInstanceStatusRequest.builder().applyMutation(describeInstanceStatusRequest)
.build(), WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link Ec2Client#describeVolumes} API until the desired condition {@code VolumeAvailable} is met, or until
* it is determined that the resource will never enter into the desired state
*
* @param describeVolumesRequest
* the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilVolumeAvailable(DescribeVolumesRequest describeVolumesRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeVolumes} API until the desired condition {@code VolumeAvailable} is met, or until
* it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeVolumesRequest#builder()}
*
* @param describeVolumesRequest
* The consumer that will configure the request to be used for polling
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilVolumeAvailable(
Consumer describeVolumesRequest) {
return waitUntilVolumeAvailable(DescribeVolumesRequest.builder().applyMutation(describeVolumesRequest).build());
}
/**
* Polls {@link Ec2Client#describeVolumes} API until the desired condition {@code VolumeAvailable} is met, or until
* it is determined that the resource will never enter into the desired state
*
* @param describeVolumesRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilVolumeAvailable(DescribeVolumesRequest describeVolumesRequest,
WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link Ec2Client#describeVolumes} API until the desired condition {@code VolumeAvailable} is met, or until
* it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeVolumesRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default WaiterResponse waitUntilVolumeAvailable(
Consumer