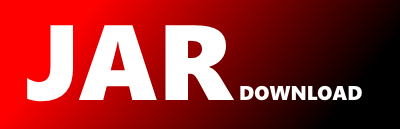
software.amazon.awssdk.services.ec2.model.LocalGatewayVirtualInterface Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a local gateway virtual interface.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LocalGatewayVirtualInterface implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField LOCAL_GATEWAY_VIRTUAL_INTERFACE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LocalGatewayVirtualInterfaceId")
.getter(getter(LocalGatewayVirtualInterface::localGatewayVirtualInterfaceId))
.setter(setter(Builder::localGatewayVirtualInterfaceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LocalGatewayVirtualInterfaceId")
.unmarshallLocationName("localGatewayVirtualInterfaceId").build()).build();
private static final SdkField LOCAL_GATEWAY_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LocalGatewayId")
.getter(getter(LocalGatewayVirtualInterface::localGatewayId))
.setter(setter(Builder::localGatewayId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LocalGatewayId")
.unmarshallLocationName("localGatewayId").build()).build();
private static final SdkField VLAN_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("Vlan")
.getter(getter(LocalGatewayVirtualInterface::vlan))
.setter(setter(Builder::vlan))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Vlan")
.unmarshallLocationName("vlan").build()).build();
private static final SdkField LOCAL_ADDRESS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LocalAddress")
.getter(getter(LocalGatewayVirtualInterface::localAddress))
.setter(setter(Builder::localAddress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LocalAddress")
.unmarshallLocationName("localAddress").build()).build();
private static final SdkField PEER_ADDRESS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PeerAddress")
.getter(getter(LocalGatewayVirtualInterface::peerAddress))
.setter(setter(Builder::peerAddress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PeerAddress")
.unmarshallLocationName("peerAddress").build()).build();
private static final SdkField LOCAL_BGP_ASN_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("LocalBgpAsn")
.getter(getter(LocalGatewayVirtualInterface::localBgpAsn))
.setter(setter(Builder::localBgpAsn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LocalBgpAsn")
.unmarshallLocationName("localBgpAsn").build()).build();
private static final SdkField PEER_BGP_ASN_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("PeerBgpAsn")
.getter(getter(LocalGatewayVirtualInterface::peerBgpAsn))
.setter(setter(Builder::peerBgpAsn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PeerBgpAsn")
.unmarshallLocationName("peerBgpAsn").build()).build();
private static final SdkField OWNER_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OwnerId")
.getter(getter(LocalGatewayVirtualInterface::ownerId))
.setter(setter(Builder::ownerId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OwnerId")
.unmarshallLocationName("ownerId").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(LocalGatewayVirtualInterface::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagSet")
.unmarshallLocationName("tagSet").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
LOCAL_GATEWAY_VIRTUAL_INTERFACE_ID_FIELD, LOCAL_GATEWAY_ID_FIELD, VLAN_FIELD, LOCAL_ADDRESS_FIELD,
PEER_ADDRESS_FIELD, LOCAL_BGP_ASN_FIELD, PEER_BGP_ASN_FIELD, OWNER_ID_FIELD, TAGS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("LocalGatewayVirtualInterfaceId", LOCAL_GATEWAY_VIRTUAL_INTERFACE_ID_FIELD);
put("LocalGatewayId", LOCAL_GATEWAY_ID_FIELD);
put("Vlan", VLAN_FIELD);
put("LocalAddress", LOCAL_ADDRESS_FIELD);
put("PeerAddress", PEER_ADDRESS_FIELD);
put("LocalBgpAsn", LOCAL_BGP_ASN_FIELD);
put("PeerBgpAsn", PEER_BGP_ASN_FIELD);
put("OwnerId", OWNER_ID_FIELD);
put("TagSet", TAGS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String localGatewayVirtualInterfaceId;
private final String localGatewayId;
private final Integer vlan;
private final String localAddress;
private final String peerAddress;
private final Integer localBgpAsn;
private final Integer peerBgpAsn;
private final String ownerId;
private final List tags;
private LocalGatewayVirtualInterface(BuilderImpl builder) {
this.localGatewayVirtualInterfaceId = builder.localGatewayVirtualInterfaceId;
this.localGatewayId = builder.localGatewayId;
this.vlan = builder.vlan;
this.localAddress = builder.localAddress;
this.peerAddress = builder.peerAddress;
this.localBgpAsn = builder.localBgpAsn;
this.peerBgpAsn = builder.peerBgpAsn;
this.ownerId = builder.ownerId;
this.tags = builder.tags;
}
/**
*
* The ID of the virtual interface.
*
*
* @return The ID of the virtual interface.
*/
public final String localGatewayVirtualInterfaceId() {
return localGatewayVirtualInterfaceId;
}
/**
*
* The ID of the local gateway.
*
*
* @return The ID of the local gateway.
*/
public final String localGatewayId() {
return localGatewayId;
}
/**
*
* The ID of the VLAN.
*
*
* @return The ID of the VLAN.
*/
public final Integer vlan() {
return vlan;
}
/**
*
* The local address.
*
*
* @return The local address.
*/
public final String localAddress() {
return localAddress;
}
/**
*
* The peer address.
*
*
* @return The peer address.
*/
public final String peerAddress() {
return peerAddress;
}
/**
*
* The Border Gateway Protocol (BGP) Autonomous System Number (ASN) of the local gateway.
*
*
* @return The Border Gateway Protocol (BGP) Autonomous System Number (ASN) of the local gateway.
*/
public final Integer localBgpAsn() {
return localBgpAsn;
}
/**
*
* The peer BGP ASN.
*
*
* @return The peer BGP ASN.
*/
public final Integer peerBgpAsn() {
return peerBgpAsn;
}
/**
*
* The ID of the Amazon Web Services account that owns the local gateway virtual interface.
*
*
* @return The ID of the Amazon Web Services account that owns the local gateway virtual interface.
*/
public final String ownerId() {
return ownerId;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The tags assigned to the virtual interface.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The tags assigned to the virtual interface.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(localGatewayVirtualInterfaceId());
hashCode = 31 * hashCode + Objects.hashCode(localGatewayId());
hashCode = 31 * hashCode + Objects.hashCode(vlan());
hashCode = 31 * hashCode + Objects.hashCode(localAddress());
hashCode = 31 * hashCode + Objects.hashCode(peerAddress());
hashCode = 31 * hashCode + Objects.hashCode(localBgpAsn());
hashCode = 31 * hashCode + Objects.hashCode(peerBgpAsn());
hashCode = 31 * hashCode + Objects.hashCode(ownerId());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LocalGatewayVirtualInterface)) {
return false;
}
LocalGatewayVirtualInterface other = (LocalGatewayVirtualInterface) obj;
return Objects.equals(localGatewayVirtualInterfaceId(), other.localGatewayVirtualInterfaceId())
&& Objects.equals(localGatewayId(), other.localGatewayId()) && Objects.equals(vlan(), other.vlan())
&& Objects.equals(localAddress(), other.localAddress()) && Objects.equals(peerAddress(), other.peerAddress())
&& Objects.equals(localBgpAsn(), other.localBgpAsn()) && Objects.equals(peerBgpAsn(), other.peerBgpAsn())
&& Objects.equals(ownerId(), other.ownerId()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LocalGatewayVirtualInterface")
.add("LocalGatewayVirtualInterfaceId", localGatewayVirtualInterfaceId()).add("LocalGatewayId", localGatewayId())
.add("Vlan", vlan()).add("LocalAddress", localAddress()).add("PeerAddress", peerAddress())
.add("LocalBgpAsn", localBgpAsn()).add("PeerBgpAsn", peerBgpAsn()).add("OwnerId", ownerId())
.add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "LocalGatewayVirtualInterfaceId":
return Optional.ofNullable(clazz.cast(localGatewayVirtualInterfaceId()));
case "LocalGatewayId":
return Optional.ofNullable(clazz.cast(localGatewayId()));
case "Vlan":
return Optional.ofNullable(clazz.cast(vlan()));
case "LocalAddress":
return Optional.ofNullable(clazz.cast(localAddress()));
case "PeerAddress":
return Optional.ofNullable(clazz.cast(peerAddress()));
case "LocalBgpAsn":
return Optional.ofNullable(clazz.cast(localBgpAsn()));
case "PeerBgpAsn":
return Optional.ofNullable(clazz.cast(peerBgpAsn()));
case "OwnerId":
return Optional.ofNullable(clazz.cast(ownerId()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function