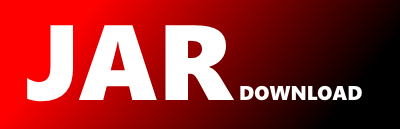
software.amazon.awssdk.services.ec2.model.ModifySubnetAttributeRequest Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifySubnetAttributeRequest extends Ec2Request implements
ToCopyableBuilder {
private static final SdkField ASSIGN_IPV6_ADDRESS_ON_CREATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("AssignIpv6AddressOnCreation")
.getter(getter(ModifySubnetAttributeRequest::assignIpv6AddressOnCreation))
.setter(setter(Builder::assignIpv6AddressOnCreation))
.constructor(AttributeBooleanValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssignIpv6AddressOnCreation")
.unmarshallLocationName("AssignIpv6AddressOnCreation").build()).build();
private static final SdkField MAP_PUBLIC_IP_ON_LAUNCH_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("MapPublicIpOnLaunch")
.getter(getter(ModifySubnetAttributeRequest::mapPublicIpOnLaunch))
.setter(setter(Builder::mapPublicIpOnLaunch))
.constructor(AttributeBooleanValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MapPublicIpOnLaunch")
.unmarshallLocationName("MapPublicIpOnLaunch").build()).build();
private static final SdkField SUBNET_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SubnetId")
.getter(getter(ModifySubnetAttributeRequest::subnetId))
.setter(setter(Builder::subnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetId")
.unmarshallLocationName("subnetId").build()).build();
private static final SdkField MAP_CUSTOMER_OWNED_IP_ON_LAUNCH_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("MapCustomerOwnedIpOnLaunch")
.getter(getter(ModifySubnetAttributeRequest::mapCustomerOwnedIpOnLaunch))
.setter(setter(Builder::mapCustomerOwnedIpOnLaunch))
.constructor(AttributeBooleanValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MapCustomerOwnedIpOnLaunch")
.unmarshallLocationName("MapCustomerOwnedIpOnLaunch").build()).build();
private static final SdkField CUSTOMER_OWNED_IPV4_POOL_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CustomerOwnedIpv4Pool")
.getter(getter(ModifySubnetAttributeRequest::customerOwnedIpv4Pool))
.setter(setter(Builder::customerOwnedIpv4Pool))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomerOwnedIpv4Pool")
.unmarshallLocationName("CustomerOwnedIpv4Pool").build()).build();
private static final SdkField ENABLE_DNS64_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("EnableDns64")
.getter(getter(ModifySubnetAttributeRequest::enableDns64))
.setter(setter(Builder::enableDns64))
.constructor(AttributeBooleanValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnableDns64")
.unmarshallLocationName("EnableDns64").build()).build();
private static final SdkField PRIVATE_DNS_HOSTNAME_TYPE_ON_LAUNCH_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PrivateDnsHostnameTypeOnLaunch")
.getter(getter(ModifySubnetAttributeRequest::privateDnsHostnameTypeOnLaunchAsString))
.setter(setter(Builder::privateDnsHostnameTypeOnLaunch))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PrivateDnsHostnameTypeOnLaunch")
.unmarshallLocationName("PrivateDnsHostnameTypeOnLaunch").build()).build();
private static final SdkField ENABLE_RESOURCE_NAME_DNS_A_RECORD_ON_LAUNCH_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("EnableResourceNameDnsARecordOnLaunch")
.getter(getter(ModifySubnetAttributeRequest::enableResourceNameDnsARecordOnLaunch))
.setter(setter(Builder::enableResourceNameDnsARecordOnLaunch))
.constructor(AttributeBooleanValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("EnableResourceNameDnsARecordOnLaunch")
.unmarshallLocationName("EnableResourceNameDnsARecordOnLaunch").build()).build();
private static final SdkField ENABLE_RESOURCE_NAME_DNS_AAAA_RECORD_ON_LAUNCH_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("EnableResourceNameDnsAAAARecordOnLaunch")
.getter(getter(ModifySubnetAttributeRequest::enableResourceNameDnsAAAARecordOnLaunch))
.setter(setter(Builder::enableResourceNameDnsAAAARecordOnLaunch))
.constructor(AttributeBooleanValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("EnableResourceNameDnsAAAARecordOnLaunch")
.unmarshallLocationName("EnableResourceNameDnsAAAARecordOnLaunch").build()).build();
private static final SdkField ENABLE_LNI_AT_DEVICE_INDEX_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("EnableLniAtDeviceIndex")
.getter(getter(ModifySubnetAttributeRequest::enableLniAtDeviceIndex))
.setter(setter(Builder::enableLniAtDeviceIndex))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnableLniAtDeviceIndex")
.unmarshallLocationName("EnableLniAtDeviceIndex").build()).build();
private static final SdkField DISABLE_LNI_AT_DEVICE_INDEX_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("DisableLniAtDeviceIndex")
.getter(getter(ModifySubnetAttributeRequest::disableLniAtDeviceIndex))
.setter(setter(Builder::disableLniAtDeviceIndex))
.constructor(AttributeBooleanValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DisableLniAtDeviceIndex")
.unmarshallLocationName("DisableLniAtDeviceIndex").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
ASSIGN_IPV6_ADDRESS_ON_CREATION_FIELD, MAP_PUBLIC_IP_ON_LAUNCH_FIELD, SUBNET_ID_FIELD,
MAP_CUSTOMER_OWNED_IP_ON_LAUNCH_FIELD, CUSTOMER_OWNED_IPV4_POOL_FIELD, ENABLE_DNS64_FIELD,
PRIVATE_DNS_HOSTNAME_TYPE_ON_LAUNCH_FIELD, ENABLE_RESOURCE_NAME_DNS_A_RECORD_ON_LAUNCH_FIELD,
ENABLE_RESOURCE_NAME_DNS_AAAA_RECORD_ON_LAUNCH_FIELD, ENABLE_LNI_AT_DEVICE_INDEX_FIELD,
DISABLE_LNI_AT_DEVICE_INDEX_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("AssignIpv6AddressOnCreation", ASSIGN_IPV6_ADDRESS_ON_CREATION_FIELD);
put("MapPublicIpOnLaunch", MAP_PUBLIC_IP_ON_LAUNCH_FIELD);
put("SubnetId", SUBNET_ID_FIELD);
put("MapCustomerOwnedIpOnLaunch", MAP_CUSTOMER_OWNED_IP_ON_LAUNCH_FIELD);
put("CustomerOwnedIpv4Pool", CUSTOMER_OWNED_IPV4_POOL_FIELD);
put("EnableDns64", ENABLE_DNS64_FIELD);
put("PrivateDnsHostnameTypeOnLaunch", PRIVATE_DNS_HOSTNAME_TYPE_ON_LAUNCH_FIELD);
put("EnableResourceNameDnsARecordOnLaunch", ENABLE_RESOURCE_NAME_DNS_A_RECORD_ON_LAUNCH_FIELD);
put("EnableResourceNameDnsAAAARecordOnLaunch", ENABLE_RESOURCE_NAME_DNS_AAAA_RECORD_ON_LAUNCH_FIELD);
put("EnableLniAtDeviceIndex", ENABLE_LNI_AT_DEVICE_INDEX_FIELD);
put("DisableLniAtDeviceIndex", DISABLE_LNI_AT_DEVICE_INDEX_FIELD);
}
});
private final AttributeBooleanValue assignIpv6AddressOnCreation;
private final AttributeBooleanValue mapPublicIpOnLaunch;
private final String subnetId;
private final AttributeBooleanValue mapCustomerOwnedIpOnLaunch;
private final String customerOwnedIpv4Pool;
private final AttributeBooleanValue enableDns64;
private final String privateDnsHostnameTypeOnLaunch;
private final AttributeBooleanValue enableResourceNameDnsARecordOnLaunch;
private final AttributeBooleanValue enableResourceNameDnsAAAARecordOnLaunch;
private final Integer enableLniAtDeviceIndex;
private final AttributeBooleanValue disableLniAtDeviceIndex;
private ModifySubnetAttributeRequest(BuilderImpl builder) {
super(builder);
this.assignIpv6AddressOnCreation = builder.assignIpv6AddressOnCreation;
this.mapPublicIpOnLaunch = builder.mapPublicIpOnLaunch;
this.subnetId = builder.subnetId;
this.mapCustomerOwnedIpOnLaunch = builder.mapCustomerOwnedIpOnLaunch;
this.customerOwnedIpv4Pool = builder.customerOwnedIpv4Pool;
this.enableDns64 = builder.enableDns64;
this.privateDnsHostnameTypeOnLaunch = builder.privateDnsHostnameTypeOnLaunch;
this.enableResourceNameDnsARecordOnLaunch = builder.enableResourceNameDnsARecordOnLaunch;
this.enableResourceNameDnsAAAARecordOnLaunch = builder.enableResourceNameDnsAAAARecordOnLaunch;
this.enableLniAtDeviceIndex = builder.enableLniAtDeviceIndex;
this.disableLniAtDeviceIndex = builder.disableLniAtDeviceIndex;
}
/**
*
* Specify true
to indicate that network interfaces created in the specified subnet should be assigned
* an IPv6 address. This includes a network interface that's created when launching an instance into the subnet (the
* instance therefore receives an IPv6 address).
*
*
* If you enable the IPv6 addressing feature for your subnet, your network interface or instance only receives an
* IPv6 address if it's created using version 2016-11-15
or later of the Amazon EC2 API.
*
*
* @return Specify true
to indicate that network interfaces created in the specified subnet should be
* assigned an IPv6 address. This includes a network interface that's created when launching an instance
* into the subnet (the instance therefore receives an IPv6 address).
*
* If you enable the IPv6 addressing feature for your subnet, your network interface or instance only
* receives an IPv6 address if it's created using version 2016-11-15
or later of the Amazon EC2
* API.
*/
public final AttributeBooleanValue assignIpv6AddressOnCreation() {
return assignIpv6AddressOnCreation;
}
/**
*
* Specify true
to indicate that network interfaces attached to instances created in the specified
* subnet should be assigned a public IPv4 address.
*
*
* Amazon Web Services charges for all public IPv4 addresses, including public IPv4 addresses associated with
* running instances and Elastic IP addresses. For more information, see the Public IPv4 Address tab on the
* Amazon VPC pricing page.
*
*
* @return Specify true
to indicate that network interfaces attached to instances created in the
* specified subnet should be assigned a public IPv4 address.
*
* Amazon Web Services charges for all public IPv4 addresses, including public IPv4 addresses associated
* with running instances and Elastic IP addresses. For more information, see the Public IPv4 Address
* tab on the Amazon VPC pricing page.
*/
public final AttributeBooleanValue mapPublicIpOnLaunch() {
return mapPublicIpOnLaunch;
}
/**
*
* The ID of the subnet.
*
*
* @return The ID of the subnet.
*/
public final String subnetId() {
return subnetId;
}
/**
*
* Specify true
to indicate that network interfaces attached to instances created in the specified
* subnet should be assigned a customer-owned IPv4 address.
*
*
* When this value is true
, you must specify the customer-owned IP pool using
* CustomerOwnedIpv4Pool
.
*
*
* @return Specify true
to indicate that network interfaces attached to instances created in the
* specified subnet should be assigned a customer-owned IPv4 address.
*
* When this value is true
, you must specify the customer-owned IP pool using
* CustomerOwnedIpv4Pool
.
*/
public final AttributeBooleanValue mapCustomerOwnedIpOnLaunch() {
return mapCustomerOwnedIpOnLaunch;
}
/**
*
* The customer-owned IPv4 address pool associated with the subnet.
*
*
* You must set this value when you specify true
for MapCustomerOwnedIpOnLaunch
.
*
*
* @return The customer-owned IPv4 address pool associated with the subnet.
*
* You must set this value when you specify true
for MapCustomerOwnedIpOnLaunch
.
*/
public final String customerOwnedIpv4Pool() {
return customerOwnedIpv4Pool;
}
/**
*
* Indicates whether DNS queries made to the Amazon-provided DNS Resolver in this subnet should return synthetic
* IPv6 addresses for IPv4-only destinations.
*
*
* You must first configure a NAT gateway in a public subnet (separate from the subnet containing the IPv6-only
* workloads). For example, the subnet containing the NAT gateway should have a 0.0.0.0/0
route
* pointing to the internet gateway. For more information, see Configure DNS64 and NAT64 in the Amazon VPC User Guide.
*
*
* @return Indicates whether DNS queries made to the Amazon-provided DNS Resolver in this subnet should return
* synthetic IPv6 addresses for IPv4-only destinations.
*
* You must first configure a NAT gateway in a public subnet (separate from the subnet containing the
* IPv6-only workloads). For example, the subnet containing the NAT gateway should have a
* 0.0.0.0/0
route pointing to the internet gateway. For more information, see Configure DNS64 and NAT64 in the Amazon VPC User Guide.
*/
public final AttributeBooleanValue enableDns64() {
return enableDns64;
}
/**
*
* The type of hostname to assign to instances in the subnet at launch. For IPv4-only and dual-stack (IPv4 and IPv6)
* subnets, an instance DNS name can be based on the instance IPv4 address (ip-name) or the instance ID
* (resource-name). For IPv6 only subnets, an instance DNS name must be based on the instance ID (resource-name).
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #privateDnsHostnameTypeOnLaunch} will return {@link HostnameType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #privateDnsHostnameTypeOnLaunchAsString}.
*
*
* @return The type of hostname to assign to instances in the subnet at launch. For IPv4-only and dual-stack (IPv4
* and IPv6) subnets, an instance DNS name can be based on the instance IPv4 address (ip-name) or the
* instance ID (resource-name). For IPv6 only subnets, an instance DNS name must be based on the instance ID
* (resource-name).
* @see HostnameType
*/
public final HostnameType privateDnsHostnameTypeOnLaunch() {
return HostnameType.fromValue(privateDnsHostnameTypeOnLaunch);
}
/**
*
* The type of hostname to assign to instances in the subnet at launch. For IPv4-only and dual-stack (IPv4 and IPv6)
* subnets, an instance DNS name can be based on the instance IPv4 address (ip-name) or the instance ID
* (resource-name). For IPv6 only subnets, an instance DNS name must be based on the instance ID (resource-name).
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #privateDnsHostnameTypeOnLaunch} will return {@link HostnameType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #privateDnsHostnameTypeOnLaunchAsString}.
*
*
* @return The type of hostname to assign to instances in the subnet at launch. For IPv4-only and dual-stack (IPv4
* and IPv6) subnets, an instance DNS name can be based on the instance IPv4 address (ip-name) or the
* instance ID (resource-name). For IPv6 only subnets, an instance DNS name must be based on the instance ID
* (resource-name).
* @see HostnameType
*/
public final String privateDnsHostnameTypeOnLaunchAsString() {
return privateDnsHostnameTypeOnLaunch;
}
/**
*
* Indicates whether to respond to DNS queries for instance hostnames with DNS A records.
*
*
* @return Indicates whether to respond to DNS queries for instance hostnames with DNS A records.
*/
public final AttributeBooleanValue enableResourceNameDnsARecordOnLaunch() {
return enableResourceNameDnsARecordOnLaunch;
}
/**
*
* Indicates whether to respond to DNS queries for instance hostnames with DNS AAAA records.
*
*
* @return Indicates whether to respond to DNS queries for instance hostnames with DNS AAAA records.
*/
public final AttributeBooleanValue enableResourceNameDnsAAAARecordOnLaunch() {
return enableResourceNameDnsAAAARecordOnLaunch;
}
/**
*
* Indicates the device position for local network interfaces in this subnet. For example, 1
indicates
* local network interfaces in this subnet are the secondary network interface (eth1). A local network interface
* cannot be the primary network interface (eth0).
*
*
* @return Indicates the device position for local network interfaces in this subnet. For example, 1
* indicates local network interfaces in this subnet are the secondary network interface (eth1). A local
* network interface cannot be the primary network interface (eth0).
*/
public final Integer enableLniAtDeviceIndex() {
return enableLniAtDeviceIndex;
}
/**
*
* Specify true
to indicate that local network interfaces at the current position should be disabled.
*
*
* @return Specify true
to indicate that local network interfaces at the current position should be
* disabled.
*/
public final AttributeBooleanValue disableLniAtDeviceIndex() {
return disableLniAtDeviceIndex;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(assignIpv6AddressOnCreation());
hashCode = 31 * hashCode + Objects.hashCode(mapPublicIpOnLaunch());
hashCode = 31 * hashCode + Objects.hashCode(subnetId());
hashCode = 31 * hashCode + Objects.hashCode(mapCustomerOwnedIpOnLaunch());
hashCode = 31 * hashCode + Objects.hashCode(customerOwnedIpv4Pool());
hashCode = 31 * hashCode + Objects.hashCode(enableDns64());
hashCode = 31 * hashCode + Objects.hashCode(privateDnsHostnameTypeOnLaunchAsString());
hashCode = 31 * hashCode + Objects.hashCode(enableResourceNameDnsARecordOnLaunch());
hashCode = 31 * hashCode + Objects.hashCode(enableResourceNameDnsAAAARecordOnLaunch());
hashCode = 31 * hashCode + Objects.hashCode(enableLniAtDeviceIndex());
hashCode = 31 * hashCode + Objects.hashCode(disableLniAtDeviceIndex());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifySubnetAttributeRequest)) {
return false;
}
ModifySubnetAttributeRequest other = (ModifySubnetAttributeRequest) obj;
return Objects.equals(assignIpv6AddressOnCreation(), other.assignIpv6AddressOnCreation())
&& Objects.equals(mapPublicIpOnLaunch(), other.mapPublicIpOnLaunch())
&& Objects.equals(subnetId(), other.subnetId())
&& Objects.equals(mapCustomerOwnedIpOnLaunch(), other.mapCustomerOwnedIpOnLaunch())
&& Objects.equals(customerOwnedIpv4Pool(), other.customerOwnedIpv4Pool())
&& Objects.equals(enableDns64(), other.enableDns64())
&& Objects.equals(privateDnsHostnameTypeOnLaunchAsString(), other.privateDnsHostnameTypeOnLaunchAsString())
&& Objects.equals(enableResourceNameDnsARecordOnLaunch(), other.enableResourceNameDnsARecordOnLaunch())
&& Objects.equals(enableResourceNameDnsAAAARecordOnLaunch(), other.enableResourceNameDnsAAAARecordOnLaunch())
&& Objects.equals(enableLniAtDeviceIndex(), other.enableLniAtDeviceIndex())
&& Objects.equals(disableLniAtDeviceIndex(), other.disableLniAtDeviceIndex());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifySubnetAttributeRequest").add("AssignIpv6AddressOnCreation", assignIpv6AddressOnCreation())
.add("MapPublicIpOnLaunch", mapPublicIpOnLaunch()).add("SubnetId", subnetId())
.add("MapCustomerOwnedIpOnLaunch", mapCustomerOwnedIpOnLaunch())
.add("CustomerOwnedIpv4Pool", customerOwnedIpv4Pool()).add("EnableDns64", enableDns64())
.add("PrivateDnsHostnameTypeOnLaunch", privateDnsHostnameTypeOnLaunchAsString())
.add("EnableResourceNameDnsARecordOnLaunch", enableResourceNameDnsARecordOnLaunch())
.add("EnableResourceNameDnsAAAARecordOnLaunch", enableResourceNameDnsAAAARecordOnLaunch())
.add("EnableLniAtDeviceIndex", enableLniAtDeviceIndex())
.add("DisableLniAtDeviceIndex", disableLniAtDeviceIndex()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AssignIpv6AddressOnCreation":
return Optional.ofNullable(clazz.cast(assignIpv6AddressOnCreation()));
case "MapPublicIpOnLaunch":
return Optional.ofNullable(clazz.cast(mapPublicIpOnLaunch()));
case "SubnetId":
return Optional.ofNullable(clazz.cast(subnetId()));
case "MapCustomerOwnedIpOnLaunch":
return Optional.ofNullable(clazz.cast(mapCustomerOwnedIpOnLaunch()));
case "CustomerOwnedIpv4Pool":
return Optional.ofNullable(clazz.cast(customerOwnedIpv4Pool()));
case "EnableDns64":
return Optional.ofNullable(clazz.cast(enableDns64()));
case "PrivateDnsHostnameTypeOnLaunch":
return Optional.ofNullable(clazz.cast(privateDnsHostnameTypeOnLaunchAsString()));
case "EnableResourceNameDnsARecordOnLaunch":
return Optional.ofNullable(clazz.cast(enableResourceNameDnsARecordOnLaunch()));
case "EnableResourceNameDnsAAAARecordOnLaunch":
return Optional.ofNullable(clazz.cast(enableResourceNameDnsAAAARecordOnLaunch()));
case "EnableLniAtDeviceIndex":
return Optional.ofNullable(clazz.cast(enableLniAtDeviceIndex()));
case "DisableLniAtDeviceIndex":
return Optional.ofNullable(clazz.cast(disableLniAtDeviceIndex()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function