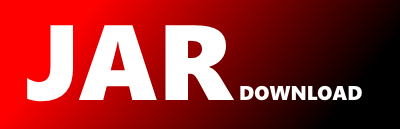
software.amazon.awssdk.services.ecr.model.BatchGetImageRequest Maven / Gradle / Ivy
Show all versions of ecr Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import javax.annotation.Generated;
import software.amazon.awssdk.core.AmazonWebServiceRequest;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public class BatchGetImageRequest extends AmazonWebServiceRequest implements
ToCopyableBuilder {
private final String registryId;
private final String repositoryName;
private final List imageIds;
private final List acceptedMediaTypes;
private BatchGetImageRequest(BuilderImpl builder) {
this.registryId = builder.registryId;
this.repositoryName = builder.repositoryName;
this.imageIds = builder.imageIds;
this.acceptedMediaTypes = builder.acceptedMediaTypes;
}
/**
*
* The AWS account ID associated with the registry that contains the images to describe. If you do not specify a
* registry, the default registry is assumed.
*
*
* @return The AWS account ID associated with the registry that contains the images to describe. If you do not
* specify a registry, the default registry is assumed.
*/
public String registryId() {
return registryId;
}
/**
*
* The repository that contains the images to describe.
*
*
* @return The repository that contains the images to describe.
*/
public String repositoryName() {
return repositoryName;
}
/**
*
* A list of image ID references that correspond to images to describe. The format of the imageIds
* reference is imageTag=tag
or imageDigest=digest
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of image ID references that correspond to images to describe. The format of the
* imageIds
reference is imageTag=tag
or imageDigest=digest
.
*/
public List imageIds() {
return imageIds;
}
/**
*
* The accepted media types for the request.
*
*
* Valid values: application/vnd.docker.distribution.manifest.v1+json
|
* application/vnd.docker.distribution.manifest.v2+json
|
* application/vnd.oci.image.manifest.v1+json
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The accepted media types for the request.
*
* Valid values: application/vnd.docker.distribution.manifest.v1+json
|
* application/vnd.docker.distribution.manifest.v2+json
|
* application/vnd.oci.image.manifest.v1+json
*/
public List acceptedMediaTypes() {
return acceptedMediaTypes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((registryId() == null) ? 0 : registryId().hashCode());
hashCode = 31 * hashCode + ((repositoryName() == null) ? 0 : repositoryName().hashCode());
hashCode = 31 * hashCode + ((imageIds() == null) ? 0 : imageIds().hashCode());
hashCode = 31 * hashCode + ((acceptedMediaTypes() == null) ? 0 : acceptedMediaTypes().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BatchGetImageRequest)) {
return false;
}
BatchGetImageRequest other = (BatchGetImageRequest) obj;
if (other.registryId() == null ^ this.registryId() == null) {
return false;
}
if (other.registryId() != null && !other.registryId().equals(this.registryId())) {
return false;
}
if (other.repositoryName() == null ^ this.repositoryName() == null) {
return false;
}
if (other.repositoryName() != null && !other.repositoryName().equals(this.repositoryName())) {
return false;
}
if (other.imageIds() == null ^ this.imageIds() == null) {
return false;
}
if (other.imageIds() != null && !other.imageIds().equals(this.imageIds())) {
return false;
}
if (other.acceptedMediaTypes() == null ^ this.acceptedMediaTypes() == null) {
return false;
}
if (other.acceptedMediaTypes() != null && !other.acceptedMediaTypes().equals(this.acceptedMediaTypes())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("{");
if (registryId() != null) {
sb.append("RegistryId: ").append(registryId()).append(",");
}
if (repositoryName() != null) {
sb.append("RepositoryName: ").append(repositoryName()).append(",");
}
if (imageIds() != null) {
sb.append("ImageIds: ").append(imageIds()).append(",");
}
if (acceptedMediaTypes() != null) {
sb.append("AcceptedMediaTypes: ").append(acceptedMediaTypes()).append(",");
}
if (sb.length() > 1) {
sb.setLength(sb.length() - 1);
}
sb.append("}");
return sb.toString();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "registryId":
return Optional.of(clazz.cast(registryId()));
case "repositoryName":
return Optional.of(clazz.cast(repositoryName()));
case "imageIds":
return Optional.of(clazz.cast(imageIds()));
case "acceptedMediaTypes":
return Optional.of(clazz.cast(acceptedMediaTypes()));
default:
return Optional.empty();
}
}
public interface Builder extends CopyableBuilder {
/**
*
* The AWS account ID associated with the registry that contains the images to describe. If you do not specify a
* registry, the default registry is assumed.
*
*
* @param registryId
* The AWS account ID associated with the registry that contains the images to describe. If you do not
* specify a registry, the default registry is assumed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder registryId(String registryId);
/**
*
* The repository that contains the images to describe.
*
*
* @param repositoryName
* The repository that contains the images to describe.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder repositoryName(String repositoryName);
/**
*
* A list of image ID references that correspond to images to describe. The format of the imageIds
* reference is imageTag=tag
or imageDigest=digest
.
*
*
* @param imageIds
* A list of image ID references that correspond to images to describe. The format of the
* imageIds
reference is imageTag=tag
or imageDigest=digest
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder imageIds(Collection imageIds);
/**
*
* A list of image ID references that correspond to images to describe. The format of the imageIds
* reference is imageTag=tag
or imageDigest=digest
.
*
*
* @param imageIds
* A list of image ID references that correspond to images to describe. The format of the
* imageIds
reference is imageTag=tag
or imageDigest=digest
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder imageIds(ImageIdentifier... imageIds);
/**
*
* The accepted media types for the request.
*
*
* Valid values: application/vnd.docker.distribution.manifest.v1+json
|
* application/vnd.docker.distribution.manifest.v2+json
|
* application/vnd.oci.image.manifest.v1+json
*
*
* @param acceptedMediaTypes
* The accepted media types for the request.
*
* Valid values: application/vnd.docker.distribution.manifest.v1+json
|
* application/vnd.docker.distribution.manifest.v2+json
|
* application/vnd.oci.image.manifest.v1+json
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder acceptedMediaTypes(Collection acceptedMediaTypes);
/**
*
* The accepted media types for the request.
*
*
* Valid values: application/vnd.docker.distribution.manifest.v1+json
|
* application/vnd.docker.distribution.manifest.v2+json
|
* application/vnd.oci.image.manifest.v1+json
*
*
* @param acceptedMediaTypes
* The accepted media types for the request.
*
* Valid values: application/vnd.docker.distribution.manifest.v1+json
|
* application/vnd.docker.distribution.manifest.v2+json
|
* application/vnd.oci.image.manifest.v1+json
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder acceptedMediaTypes(String... acceptedMediaTypes);
}
static final class BuilderImpl implements Builder {
private String registryId;
private String repositoryName;
private List imageIds;
private List acceptedMediaTypes;
private BuilderImpl() {
}
private BuilderImpl(BatchGetImageRequest model) {
registryId(model.registryId);
repositoryName(model.repositoryName);
imageIds(model.imageIds);
acceptedMediaTypes(model.acceptedMediaTypes);
}
public final String getRegistryId() {
return registryId;
}
@Override
public final Builder registryId(String registryId) {
this.registryId = registryId;
return this;
}
public final void setRegistryId(String registryId) {
this.registryId = registryId;
}
public final String getRepositoryName() {
return repositoryName;
}
@Override
public final Builder repositoryName(String repositoryName) {
this.repositoryName = repositoryName;
return this;
}
public final void setRepositoryName(String repositoryName) {
this.repositoryName = repositoryName;
}
public final Collection getImageIds() {
return imageIds != null ? imageIds.stream().map(ImageIdentifier::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder imageIds(Collection imageIds) {
this.imageIds = ImageIdentifierListCopier.copy(imageIds);
return this;
}
@Override
@SafeVarargs
public final Builder imageIds(ImageIdentifier... imageIds) {
imageIds(Arrays.asList(imageIds));
return this;
}
public final void setImageIds(Collection imageIds) {
this.imageIds = ImageIdentifierListCopier.copyFromBuilder(imageIds);
}
public final Collection getAcceptedMediaTypes() {
return acceptedMediaTypes;
}
@Override
public final Builder acceptedMediaTypes(Collection acceptedMediaTypes) {
this.acceptedMediaTypes = MediaTypeListCopier.copy(acceptedMediaTypes);
return this;
}
@Override
@SafeVarargs
public final Builder acceptedMediaTypes(String... acceptedMediaTypes) {
acceptedMediaTypes(Arrays.asList(acceptedMediaTypes));
return this;
}
public final void setAcceptedMediaTypes(Collection acceptedMediaTypes) {
this.acceptedMediaTypes = MediaTypeListCopier.copy(acceptedMediaTypes);
}
@Override
public BatchGetImageRequest build() {
return new BatchGetImageRequest(this);
}
}
}