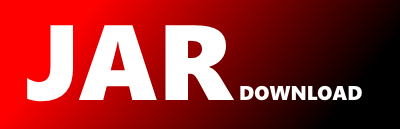
software.amazon.awssdk.services.ecr.model.Repository Maven / Gradle / Ivy
Show all versions of ecr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object representing a repository.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Repository implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField REPOSITORY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Repository::repositoryArn)).setter(setter(Builder::repositoryArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("repositoryArn").build()).build();
private static final SdkField REGISTRY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Repository::registryId)).setter(setter(Builder::registryId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registryId").build()).build();
private static final SdkField REPOSITORY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Repository::repositoryName)).setter(setter(Builder::repositoryName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("repositoryName").build()).build();
private static final SdkField REPOSITORY_URI_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Repository::repositoryUri)).setter(setter(Builder::repositoryUri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("repositoryUri").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(Repository::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdAt").build()).build();
private static final SdkField IMAGE_TAG_MUTABILITY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Repository::imageTagMutabilityAsString)).setter(setter(Builder::imageTagMutability))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("imageTagMutability").build())
.build();
private static final SdkField IMAGE_SCANNING_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(Repository::imageScanningConfiguration))
.setter(setter(Builder::imageScanningConfiguration))
.constructor(ImageScanningConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("imageScanningConfiguration").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REPOSITORY_ARN_FIELD,
REGISTRY_ID_FIELD, REPOSITORY_NAME_FIELD, REPOSITORY_URI_FIELD, CREATED_AT_FIELD, IMAGE_TAG_MUTABILITY_FIELD,
IMAGE_SCANNING_CONFIGURATION_FIELD));
private static final long serialVersionUID = 1L;
private final String repositoryArn;
private final String registryId;
private final String repositoryName;
private final String repositoryUri;
private final Instant createdAt;
private final String imageTagMutability;
private final ImageScanningConfiguration imageScanningConfiguration;
private Repository(BuilderImpl builder) {
this.repositoryArn = builder.repositoryArn;
this.registryId = builder.registryId;
this.repositoryName = builder.repositoryName;
this.repositoryUri = builder.repositoryUri;
this.createdAt = builder.createdAt;
this.imageTagMutability = builder.imageTagMutability;
this.imageScanningConfiguration = builder.imageScanningConfiguration;
}
/**
*
* The Amazon Resource Name (ARN) that identifies the repository. The ARN contains the arn:aws:ecr
* namespace, followed by the region of the repository, AWS account ID of the repository owner, repository
* namespace, and repository name. For example, arn:aws:ecr:region:012345678910:repository/test
.
*
*
* @return The Amazon Resource Name (ARN) that identifies the repository. The ARN contains the
* arn:aws:ecr
namespace, followed by the region of the repository, AWS account ID of the
* repository owner, repository namespace, and repository name. For example,
* arn:aws:ecr:region:012345678910:repository/test
.
*/
public String repositoryArn() {
return repositoryArn;
}
/**
*
* The AWS account ID associated with the registry that contains the repository.
*
*
* @return The AWS account ID associated with the registry that contains the repository.
*/
public String registryId() {
return registryId;
}
/**
*
* The name of the repository.
*
*
* @return The name of the repository.
*/
public String repositoryName() {
return repositoryName;
}
/**
*
* The URI for the repository. You can use this URI for Docker push
or pull
operations.
*
*
* @return The URI for the repository. You can use this URI for Docker push
or pull
* operations.
*/
public String repositoryUri() {
return repositoryUri;
}
/**
*
* The date and time, in JavaScript date format, when the repository was created.
*
*
* @return The date and time, in JavaScript date format, when the repository was created.
*/
public Instant createdAt() {
return createdAt;
}
/**
*
* The tag mutability setting for the repository.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imageTagMutability} will return {@link ImageTagMutability#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #imageTagMutabilityAsString}.
*
*
* @return The tag mutability setting for the repository.
* @see ImageTagMutability
*/
public ImageTagMutability imageTagMutability() {
return ImageTagMutability.fromValue(imageTagMutability);
}
/**
*
* The tag mutability setting for the repository.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imageTagMutability} will return {@link ImageTagMutability#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #imageTagMutabilityAsString}.
*
*
* @return The tag mutability setting for the repository.
* @see ImageTagMutability
*/
public String imageTagMutabilityAsString() {
return imageTagMutability;
}
/**
* Returns the value of the ImageScanningConfiguration property for this object.
*
* @return The value of the ImageScanningConfiguration property for this object.
*/
public ImageScanningConfiguration imageScanningConfiguration() {
return imageScanningConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(repositoryArn());
hashCode = 31 * hashCode + Objects.hashCode(registryId());
hashCode = 31 * hashCode + Objects.hashCode(repositoryName());
hashCode = 31 * hashCode + Objects.hashCode(repositoryUri());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(imageTagMutabilityAsString());
hashCode = 31 * hashCode + Objects.hashCode(imageScanningConfiguration());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Repository)) {
return false;
}
Repository other = (Repository) obj;
return Objects.equals(repositoryArn(), other.repositoryArn()) && Objects.equals(registryId(), other.registryId())
&& Objects.equals(repositoryName(), other.repositoryName())
&& Objects.equals(repositoryUri(), other.repositoryUri()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(imageTagMutabilityAsString(), other.imageTagMutabilityAsString())
&& Objects.equals(imageScanningConfiguration(), other.imageScanningConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("Repository").add("RepositoryArn", repositoryArn()).add("RegistryId", registryId())
.add("RepositoryName", repositoryName()).add("RepositoryUri", repositoryUri()).add("CreatedAt", createdAt())
.add("ImageTagMutability", imageTagMutabilityAsString())
.add("ImageScanningConfiguration", imageScanningConfiguration()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "repositoryArn":
return Optional.ofNullable(clazz.cast(repositoryArn()));
case "registryId":
return Optional.ofNullable(clazz.cast(registryId()));
case "repositoryName":
return Optional.ofNullable(clazz.cast(repositoryName()));
case "repositoryUri":
return Optional.ofNullable(clazz.cast(repositoryUri()));
case "createdAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "imageTagMutability":
return Optional.ofNullable(clazz.cast(imageTagMutabilityAsString()));
case "imageScanningConfiguration":
return Optional.ofNullable(clazz.cast(imageScanningConfiguration()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function