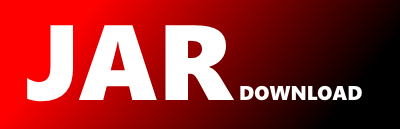
software.amazon.awssdk.services.ecr.DefaultEcrClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.ecr.model.BatchCheckLayerAvailabilityRequest;
import software.amazon.awssdk.services.ecr.model.BatchCheckLayerAvailabilityResponse;
import software.amazon.awssdk.services.ecr.model.BatchDeleteImageRequest;
import software.amazon.awssdk.services.ecr.model.BatchDeleteImageResponse;
import software.amazon.awssdk.services.ecr.model.BatchGetImageRequest;
import software.amazon.awssdk.services.ecr.model.BatchGetImageResponse;
import software.amazon.awssdk.services.ecr.model.CompleteLayerUploadRequest;
import software.amazon.awssdk.services.ecr.model.CompleteLayerUploadResponse;
import software.amazon.awssdk.services.ecr.model.CreateRepositoryRequest;
import software.amazon.awssdk.services.ecr.model.CreateRepositoryResponse;
import software.amazon.awssdk.services.ecr.model.DeleteLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.DeleteLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRegistryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRegistryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryResponse;
import software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest;
import software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsResponse;
import software.amazon.awssdk.services.ecr.model.DescribeImagesRequest;
import software.amazon.awssdk.services.ecr.model.DescribeImagesResponse;
import software.amazon.awssdk.services.ecr.model.DescribeRegistryRequest;
import software.amazon.awssdk.services.ecr.model.DescribeRegistryResponse;
import software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest;
import software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse;
import software.amazon.awssdk.services.ecr.model.EcrException;
import software.amazon.awssdk.services.ecr.model.EcrRequest;
import software.amazon.awssdk.services.ecr.model.EmptyUploadException;
import software.amazon.awssdk.services.ecr.model.GetAuthorizationTokenRequest;
import software.amazon.awssdk.services.ecr.model.GetAuthorizationTokenResponse;
import software.amazon.awssdk.services.ecr.model.GetDownloadUrlForLayerRequest;
import software.amazon.awssdk.services.ecr.model.GetDownloadUrlForLayerResponse;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewResponse;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.GetRegistryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.GetRegistryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.GetRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.GetRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.ImageAlreadyExistsException;
import software.amazon.awssdk.services.ecr.model.ImageDigestDoesNotMatchException;
import software.amazon.awssdk.services.ecr.model.ImageNotFoundException;
import software.amazon.awssdk.services.ecr.model.ImageTagAlreadyExistsException;
import software.amazon.awssdk.services.ecr.model.InitiateLayerUploadRequest;
import software.amazon.awssdk.services.ecr.model.InitiateLayerUploadResponse;
import software.amazon.awssdk.services.ecr.model.InvalidLayerException;
import software.amazon.awssdk.services.ecr.model.InvalidLayerPartException;
import software.amazon.awssdk.services.ecr.model.InvalidParameterException;
import software.amazon.awssdk.services.ecr.model.InvalidTagParameterException;
import software.amazon.awssdk.services.ecr.model.KmsException;
import software.amazon.awssdk.services.ecr.model.LayerAlreadyExistsException;
import software.amazon.awssdk.services.ecr.model.LayerInaccessibleException;
import software.amazon.awssdk.services.ecr.model.LayerPartTooSmallException;
import software.amazon.awssdk.services.ecr.model.LayersNotFoundException;
import software.amazon.awssdk.services.ecr.model.LifecyclePolicyNotFoundException;
import software.amazon.awssdk.services.ecr.model.LifecyclePolicyPreviewInProgressException;
import software.amazon.awssdk.services.ecr.model.LifecyclePolicyPreviewNotFoundException;
import software.amazon.awssdk.services.ecr.model.LimitExceededException;
import software.amazon.awssdk.services.ecr.model.ListImagesRequest;
import software.amazon.awssdk.services.ecr.model.ListImagesResponse;
import software.amazon.awssdk.services.ecr.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.ecr.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.ecr.model.PutImageRequest;
import software.amazon.awssdk.services.ecr.model.PutImageResponse;
import software.amazon.awssdk.services.ecr.model.PutImageScanningConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.PutImageScanningConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.PutImageTagMutabilityRequest;
import software.amazon.awssdk.services.ecr.model.PutImageTagMutabilityResponse;
import software.amazon.awssdk.services.ecr.model.PutLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.PutLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.PutRegistryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.PutRegistryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.PutReplicationConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.PutReplicationConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.ReferencedImagesNotFoundException;
import software.amazon.awssdk.services.ecr.model.RegistryPolicyNotFoundException;
import software.amazon.awssdk.services.ecr.model.RepositoryAlreadyExistsException;
import software.amazon.awssdk.services.ecr.model.RepositoryNotEmptyException;
import software.amazon.awssdk.services.ecr.model.RepositoryNotFoundException;
import software.amazon.awssdk.services.ecr.model.RepositoryPolicyNotFoundException;
import software.amazon.awssdk.services.ecr.model.ScanNotFoundException;
import software.amazon.awssdk.services.ecr.model.ServerException;
import software.amazon.awssdk.services.ecr.model.SetRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.SetRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.StartImageScanRequest;
import software.amazon.awssdk.services.ecr.model.StartImageScanResponse;
import software.amazon.awssdk.services.ecr.model.StartLifecyclePolicyPreviewRequest;
import software.amazon.awssdk.services.ecr.model.StartLifecyclePolicyPreviewResponse;
import software.amazon.awssdk.services.ecr.model.TagResourceRequest;
import software.amazon.awssdk.services.ecr.model.TagResourceResponse;
import software.amazon.awssdk.services.ecr.model.TooManyTagsException;
import software.amazon.awssdk.services.ecr.model.UnsupportedImageTypeException;
import software.amazon.awssdk.services.ecr.model.UntagResourceRequest;
import software.amazon.awssdk.services.ecr.model.UntagResourceResponse;
import software.amazon.awssdk.services.ecr.model.UploadLayerPartRequest;
import software.amazon.awssdk.services.ecr.model.UploadLayerPartResponse;
import software.amazon.awssdk.services.ecr.model.UploadNotFoundException;
import software.amazon.awssdk.services.ecr.model.ValidationException;
import software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsIterable;
import software.amazon.awssdk.services.ecr.paginators.DescribeImagesIterable;
import software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable;
import software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewIterable;
import software.amazon.awssdk.services.ecr.paginators.ListImagesIterable;
import software.amazon.awssdk.services.ecr.transform.BatchCheckLayerAvailabilityRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.BatchDeleteImageRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.BatchGetImageRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.CompleteLayerUploadRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.CreateRepositoryRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.DeleteLifecyclePolicyRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.DeleteRegistryPolicyRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.DeleteRepositoryPolicyRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.DeleteRepositoryRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.DescribeImageScanFindingsRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.DescribeImagesRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.DescribeRegistryRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.DescribeRepositoriesRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.GetAuthorizationTokenRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.GetDownloadUrlForLayerRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.GetLifecyclePolicyPreviewRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.GetLifecyclePolicyRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.GetRegistryPolicyRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.GetRepositoryPolicyRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.InitiateLayerUploadRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.ListImagesRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.PutImageRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.PutImageScanningConfigurationRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.PutImageTagMutabilityRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.PutLifecyclePolicyRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.PutRegistryPolicyRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.PutReplicationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.SetRepositoryPolicyRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.StartImageScanRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.StartLifecyclePolicyPreviewRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.ecr.transform.UploadLayerPartRequestMarshaller;
import software.amazon.awssdk.services.ecr.waiters.EcrWaiter;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link EcrClient}.
*
* @see EcrClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultEcrClient implements EcrClient {
private static final Logger log = Logger.loggerFor(DefaultEcrClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultEcrClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Checks the availability of one or more image layers in a repository.
*
*
* When an image is pushed to a repository, each image layer is checked to verify if it has been uploaded before. If
* it has been uploaded, then the image layer is skipped.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param batchCheckLayerAvailabilityRequest
* @return Result of the BatchCheckLayerAvailability operation returned by the service.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.BatchCheckLayerAvailability
* @see AWS API Documentation
*/
@Override
public BatchCheckLayerAvailabilityResponse batchCheckLayerAvailability(
BatchCheckLayerAvailabilityRequest batchCheckLayerAvailabilityRequest) throws RepositoryNotFoundException,
InvalidParameterException, ServerException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchCheckLayerAvailabilityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchCheckLayerAvailabilityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchCheckLayerAvailability");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchCheckLayerAvailability").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(batchCheckLayerAvailabilityRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchCheckLayerAvailabilityRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a list of specified images within a repository. Images are specified with either an imageTag
* or imageDigest
.
*
*
* You can remove a tag from an image by specifying the image's tag in your request. When you remove the last tag
* from an image, the image is deleted from your repository.
*
*
* You can completely delete an image (and all of its tags) by specifying the image's digest in your request.
*
*
* @param batchDeleteImageRequest
* Deletes specified images within a specified repository. Images are specified with either the
* imageTag
or imageDigest
.
* @return Result of the BatchDeleteImage operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.BatchDeleteImage
* @see AWS API
* Documentation
*/
@Override
public BatchDeleteImageResponse batchDeleteImage(BatchDeleteImageRequest batchDeleteImageRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
BatchDeleteImageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDeleteImageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeleteImage");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("BatchDeleteImage").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(batchDeleteImageRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchDeleteImageRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets detailed information for an image. Images are specified with either an imageTag
or
* imageDigest
.
*
*
* When an image is pulled, the BatchGetImage API is called once to retrieve the image manifest.
*
*
* @param batchGetImageRequest
* @return Result of the BatchGetImage operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.BatchGetImage
* @see AWS API
* Documentation
*/
@Override
public BatchGetImageResponse batchGetImage(BatchGetImageRequest batchGetImageRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
BatchGetImageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchGetImageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchGetImage");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("BatchGetImage").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(batchGetImageRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchGetImageRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Informs Amazon ECR that the image layer upload has completed for a specified registry, repository name, and
* upload ID. You can optionally provide a sha256
digest of the image layer for data validation
* purposes.
*
*
* When an image is pushed, the CompleteLayerUpload API is called once per each new image layer to verify that the
* upload has completed.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param completeLayerUploadRequest
* @return Result of the CompleteLayerUpload operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws UploadNotFoundException
* The upload could not be found, or the specified upload ID is not valid for this repository.
* @throws InvalidLayerException
* The layer digest calculation performed by Amazon ECR upon receipt of the image layer does not match the
* digest specified.
* @throws LayerPartTooSmallException
* Layer parts must be at least 5 MiB in size.
* @throws LayerAlreadyExistsException
* The image layer already exists in the associated repository.
* @throws EmptyUploadException
* The specified layer upload does not contain any layer parts.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.CompleteLayerUpload
* @see AWS API
* Documentation
*/
@Override
public CompleteLayerUploadResponse completeLayerUpload(CompleteLayerUploadRequest completeLayerUploadRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, UploadNotFoundException,
InvalidLayerException, LayerPartTooSmallException, LayerAlreadyExistsException, EmptyUploadException, KmsException,
AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CompleteLayerUploadResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, completeLayerUploadRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CompleteLayerUpload");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CompleteLayerUpload").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(completeLayerUploadRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CompleteLayerUploadRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a repository. For more information, see Amazon ECR Repositories in
* the Amazon Elastic Container Registry User Guide.
*
*
* @param createRepositoryRequest
* @return Result of the CreateRepository operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidTagParameterException
* An invalid parameter has been specified. Tag keys can have a maximum character length of 128 characters,
* and tag values can have a maximum length of 256 characters.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws RepositoryAlreadyExistsException
* The specified repository already exists in the specified registry.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR Service
* Quotas in the Amazon Elastic Container Registry User Guide.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.CreateRepository
* @see AWS API
* Documentation
*/
@Override
public CreateRepositoryResponse createRepository(CreateRepositoryRequest createRepositoryRequest) throws ServerException,
InvalidParameterException, InvalidTagParameterException, TooManyTagsException, RepositoryAlreadyExistsException,
LimitExceededException, KmsException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateRepositoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createRepositoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRepository");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateRepository").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createRepositoryRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateRepositoryRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the lifecycle policy associated with the specified repository.
*
*
* @param deleteLifecyclePolicyRequest
* @return Result of the DeleteLifecyclePolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyNotFoundException
* The lifecycle policy could not be found, and no policy is set to the repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteLifecyclePolicy
* @see AWS API
* Documentation
*/
@Override
public DeleteLifecyclePolicyResponse deleteLifecyclePolicy(DeleteLifecyclePolicyRequest deleteLifecyclePolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, LifecyclePolicyNotFoundException,
AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteLifecyclePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteLifecyclePolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteLifecyclePolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteLifecyclePolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteLifecyclePolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteLifecyclePolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the registry permissions policy.
*
*
* @param deleteRegistryPolicyRequest
* @return Result of the DeleteRegistryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RegistryPolicyNotFoundException
* The registry doesn't have an associated registry policy.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteRegistryPolicy
* @see AWS API
* Documentation
*/
@Override
public DeleteRegistryPolicyResponse deleteRegistryPolicy(DeleteRegistryPolicyRequest deleteRegistryPolicyRequest)
throws ServerException, InvalidParameterException, RegistryPolicyNotFoundException, AwsServiceException,
SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteRegistryPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRegistryPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRegistryPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteRegistryPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteRegistryPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteRegistryPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a repository. If the repository contains images, you must either delete all images in the repository or
* use the force
option to delete the repository.
*
*
* @param deleteRepositoryRequest
* @return Result of the DeleteRepository operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws RepositoryNotEmptyException
* The specified repository contains images. To delete a repository that contains images, you must force the
* deletion with the force
parameter.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteRepository
* @see AWS API
* Documentation
*/
@Override
public DeleteRepositoryResponse deleteRepository(DeleteRepositoryRequest deleteRepositoryRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, RepositoryNotEmptyException, KmsException,
AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteRepositoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRepositoryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRepository");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteRepository").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteRepositoryRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteRepositoryRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the repository policy associated with the specified repository.
*
*
* @param deleteRepositoryPolicyRequest
* @return Result of the DeleteRepositoryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws RepositoryPolicyNotFoundException
* The specified repository and registry combination does not have an associated repository policy.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteRepositoryPolicy
* @see AWS API
* Documentation
*/
@Override
public DeleteRepositoryPolicyResponse deleteRepositoryPolicy(DeleteRepositoryPolicyRequest deleteRepositoryPolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, RepositoryPolicyNotFoundException,
AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteRepositoryPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRepositoryPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRepositoryPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteRepositoryPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteRepositoryPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteRepositoryPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the scan findings for the specified image.
*
*
* @param describeImageScanFindingsRequest
* @return Result of the DescribeImageScanFindings operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws ScanNotFoundException
* The specified image scan could not be found. Ensure that image scanning is enabled on the repository and
* try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImageScanFindings
* @see AWS
* API Documentation
*/
@Override
public DescribeImageScanFindingsResponse describeImageScanFindings(
DescribeImageScanFindingsRequest describeImageScanFindingsRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, ImageNotFoundException, ScanNotFoundException, AwsServiceException, SdkClientException,
EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeImageScanFindingsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeImageScanFindingsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeImageScanFindings");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeImageScanFindings").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeImageScanFindingsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeImageScanFindingsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the scan findings for the specified image.
*
*
*
* This is a variant of
* {@link #describeImageScanFindings(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsIterable responses = client.describeImageScanFindingsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsIterable responses = client
* .describeImageScanFindingsPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsIterable responses = client.describeImageScanFindingsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImageScanFindings(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest)}
* operation.
*
*
* @param describeImageScanFindingsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws ScanNotFoundException
* The specified image scan could not be found. Ensure that image scanning is enabled on the repository and
* try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImageScanFindings
* @see AWS
* API Documentation
*/
@Override
public DescribeImageScanFindingsIterable describeImageScanFindingsPaginator(
DescribeImageScanFindingsRequest describeImageScanFindingsRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, ImageNotFoundException, ScanNotFoundException, AwsServiceException, SdkClientException,
EcrException {
return new DescribeImageScanFindingsIterable(this, applyPaginatorUserAgent(describeImageScanFindingsRequest));
}
/**
*
* Returns metadata about the images in a repository.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* @param describeImagesRequest
* @return Result of the DescribeImages operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImages
* @see AWS API
* Documentation
*/
@Override
public DescribeImagesResponse describeImages(DescribeImagesRequest describeImagesRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, ImageNotFoundException, AwsServiceException,
SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeImagesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeImagesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeImages");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeImages").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeImagesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeImagesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns metadata about the images in a repository.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* This is a variant of {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesIterable responses = client.describeImagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesIterable responses = client.describeImagesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeImagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesIterable responses = client.describeImagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)} operation.
*
*
* @param describeImagesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImages
* @see AWS API
* Documentation
*/
@Override
public DescribeImagesIterable describeImagesPaginator(DescribeImagesRequest describeImagesRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, ImageNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return new DescribeImagesIterable(this, applyPaginatorUserAgent(describeImagesRequest));
}
/**
*
* Describes the settings for a registry. The replication configuration for a repository can be created or updated
* with the PutReplicationConfiguration API action.
*
*
* @param describeRegistryRequest
* @return Result of the DescribeRegistry operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRegistry
* @see AWS API
* Documentation
*/
@Override
public DescribeRegistryResponse describeRegistry(DescribeRegistryRequest describeRegistryRequest) throws ServerException,
InvalidParameterException, ValidationException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeRegistryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRegistryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRegistry");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeRegistry").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeRegistryRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeRegistryRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes image repositories in a registry.
*
*
* @param describeRepositoriesRequest
* @return Result of the DescribeRepositories operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositories
* @see AWS API
* Documentation
*/
@Override
public DescribeRepositoriesResponse describeRepositories(DescribeRepositoriesRequest describeRepositoriesRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, AwsServiceException,
SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeRepositoriesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRepositoriesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRepositories");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeRepositories").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeRepositoriesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeRepositoriesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes image repositories in a registry.
*
*
*
* This is a variant of
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client.describeRepositoriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client
* .describeRepositoriesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client.describeRepositoriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)}
* operation.
*
*
* @param describeRepositoriesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositories
* @see AWS API
* Documentation
*/
@Override
public DescribeRepositoriesIterable describeRepositoriesPaginator(DescribeRepositoriesRequest describeRepositoriesRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return new DescribeRepositoriesIterable(this, applyPaginatorUserAgent(describeRepositoriesRequest));
}
/**
*
* Retrieves an authorization token. An authorization token represents your IAM authentication credentials and can
* be used to access any Amazon ECR registry that your IAM principal has access to. The authorization token is valid
* for 12 hours.
*
*
* The authorizationToken
returned is a base64 encoded string that can be decoded and used in a
* docker login
command to authenticate to a registry. The AWS CLI offers an
* get-login-password
command that simplifies the login process. For more information, see Registry
* Authentication in the Amazon Elastic Container Registry User Guide.
*
*
* @param getAuthorizationTokenRequest
* @return Result of the GetAuthorizationToken operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetAuthorizationToken
* @see AWS API
* Documentation
*/
@Override
public GetAuthorizationTokenResponse getAuthorizationToken(GetAuthorizationTokenRequest getAuthorizationTokenRequest)
throws ServerException, InvalidParameterException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAuthorizationTokenResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAuthorizationTokenRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAuthorizationToken");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetAuthorizationToken").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getAuthorizationTokenRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetAuthorizationTokenRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the pre-signed Amazon S3 download URL corresponding to an image layer. You can only get URLs for image
* layers that are referenced in an image.
*
*
* When an image is pulled, the GetDownloadUrlForLayer API is called once per image layer that is not already
* cached.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param getDownloadUrlForLayerRequest
* @return Result of the GetDownloadUrlForLayer operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws LayersNotFoundException
* The specified layers could not be found, or the specified layer is not valid for this repository.
* @throws LayerInaccessibleException
* The specified layer is not available because it is not associated with an image. Unassociated image
* layers may be cleaned up at any time.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetDownloadUrlForLayer
* @see AWS API
* Documentation
*/
@Override
public GetDownloadUrlForLayerResponse getDownloadUrlForLayer(GetDownloadUrlForLayerRequest getDownloadUrlForLayerRequest)
throws ServerException, InvalidParameterException, LayersNotFoundException, LayerInaccessibleException,
RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDownloadUrlForLayerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDownloadUrlForLayerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDownloadUrlForLayer");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDownloadUrlForLayer").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getDownloadUrlForLayerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDownloadUrlForLayerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the lifecycle policy for the specified repository.
*
*
* @param getLifecyclePolicyRequest
* @return Result of the GetLifecyclePolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyNotFoundException
* The lifecycle policy could not be found, and no policy is set to the repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetLifecyclePolicy
* @see AWS API
* Documentation
*/
@Override
public GetLifecyclePolicyResponse getLifecyclePolicy(GetLifecyclePolicyRequest getLifecyclePolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, LifecyclePolicyNotFoundException,
AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetLifecyclePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getLifecyclePolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetLifecyclePolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetLifecyclePolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getLifecyclePolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetLifecyclePolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the results of the lifecycle policy preview request for the specified repository.
*
*
* @param getLifecyclePolicyPreviewRequest
* @return Result of the GetLifecyclePolicyPreview operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyPreviewNotFoundException
* There is no dry run for this repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
@Override
public GetLifecyclePolicyPreviewResponse getLifecyclePolicyPreview(
GetLifecyclePolicyPreviewRequest getLifecyclePolicyPreviewRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, LifecyclePolicyPreviewNotFoundException, AwsServiceException, SdkClientException,
EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetLifecyclePolicyPreviewResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getLifecyclePolicyPreviewRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetLifecyclePolicyPreview");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetLifecyclePolicyPreview").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getLifecyclePolicyPreviewRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetLifecyclePolicyPreviewRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the results of the lifecycle policy preview request for the specified repository.
*
*
*
* This is a variant of
* {@link #getLifecyclePolicyPreview(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewIterable responses = client.getLifecyclePolicyPreviewPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewIterable responses = client
* .getLifecyclePolicyPreviewPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewIterable responses = client.getLifecyclePolicyPreviewPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLifecyclePolicyPreview(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest)}
* operation.
*
*
* @param getLifecyclePolicyPreviewRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyPreviewNotFoundException
* There is no dry run for this repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
@Override
public GetLifecyclePolicyPreviewIterable getLifecyclePolicyPreviewPaginator(
GetLifecyclePolicyPreviewRequest getLifecyclePolicyPreviewRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, LifecyclePolicyPreviewNotFoundException, AwsServiceException, SdkClientException,
EcrException {
return new GetLifecyclePolicyPreviewIterable(this, applyPaginatorUserAgent(getLifecyclePolicyPreviewRequest));
}
/**
*
* Retrieves the permissions policy for a registry.
*
*
* @param getRegistryPolicyRequest
* @return Result of the GetRegistryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RegistryPolicyNotFoundException
* The registry doesn't have an associated registry policy.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetRegistryPolicy
* @see AWS API
* Documentation
*/
@Override
public GetRegistryPolicyResponse getRegistryPolicy(GetRegistryPolicyRequest getRegistryPolicyRequest) throws ServerException,
InvalidParameterException, RegistryPolicyNotFoundException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetRegistryPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRegistryPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRegistryPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetRegistryPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getRegistryPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetRegistryPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the repository policy for the specified repository.
*
*
* @param getRepositoryPolicyRequest
* @return Result of the GetRepositoryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws RepositoryPolicyNotFoundException
* The specified repository and registry combination does not have an associated repository policy.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetRepositoryPolicy
* @see AWS API
* Documentation
*/
@Override
public GetRepositoryPolicyResponse getRepositoryPolicy(GetRepositoryPolicyRequest getRepositoryPolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, RepositoryPolicyNotFoundException,
AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetRepositoryPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRepositoryPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRepositoryPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetRepositoryPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getRepositoryPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetRepositoryPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Notifies Amazon ECR that you intend to upload an image layer.
*
*
* When an image is pushed, the InitiateLayerUpload API is called once per image layer that has not already been
* uploaded. Whether or not an image layer has been uploaded is determined by the BatchCheckLayerAvailability API
* action.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param initiateLayerUploadRequest
* @return Result of the InitiateLayerUpload operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.InitiateLayerUpload
* @see AWS API
* Documentation
*/
@Override
public InitiateLayerUploadResponse initiateLayerUpload(InitiateLayerUploadRequest initiateLayerUploadRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, KmsException, AwsServiceException,
SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, InitiateLayerUploadResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, initiateLayerUploadRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "InitiateLayerUpload");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("InitiateLayerUpload").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(initiateLayerUploadRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new InitiateLayerUploadRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all the image IDs for the specified repository.
*
*
* You can filter images based on whether or not they are tagged by using the tagStatus
filter and
* specifying either TAGGED
, UNTAGGED
or ANY
. For example, you can filter
* your results to return only UNTAGGED
images and then pipe that result to a BatchDeleteImage
* operation to delete them. Or, you can filter your results to return only TAGGED
images to list all
* of the tags in your repository.
*
*
* @param listImagesRequest
* @return Result of the ListImages operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.ListImages
* @see AWS API
* Documentation
*/
@Override
public ListImagesResponse listImages(ListImagesRequest listImagesRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListImagesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listImagesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListImages");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("ListImages")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listImagesRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListImagesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all the image IDs for the specified repository.
*
*
* You can filter images based on whether or not they are tagged by using the tagStatus
filter and
* specifying either TAGGED
, UNTAGGED
or ANY
. For example, you can filter
* your results to return only UNTAGGED
images and then pipe that result to a BatchDeleteImage
* operation to delete them. Or, you can filter your results to return only TAGGED
images to list all
* of the tags in your repository.
*
*
*
* This is a variant of {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesIterable responses = client.listImagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.ListImagesIterable responses = client.listImagesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.ListImagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesIterable responses = client.listImagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
*
*
* @param listImagesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.ListImages
* @see AWS API
* Documentation
*/
@Override
public ListImagesIterable listImagesPaginator(ListImagesRequest listImagesRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
return new ListImagesIterable(this, applyPaginatorUserAgent(listImagesRequest));
}
/**
*
* List the tags for an Amazon ECR resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.ListTagsForResource
* @see AWS API
* Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws InvalidParameterException, RepositoryNotFoundException, ServerException, AwsServiceException,
SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listTagsForResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or updates the image manifest and tags associated with an image.
*
*
* When an image is pushed and all new image layers have been uploaded, the PutImage API is called once to create or
* update the image manifest and the tags associated with the image.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param putImageRequest
* @return Result of the PutImage operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageAlreadyExistsException
* The specified image has already been pushed, and there were no changes to the manifest or image tag after
* the last push.
* @throws LayersNotFoundException
* The specified layers could not be found, or the specified layer is not valid for this repository.
* @throws ReferencedImagesNotFoundException
* The manifest list is referencing an image that does not exist.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR Service
* Quotas in the Amazon Elastic Container Registry User Guide.
* @throws ImageTagAlreadyExistsException
* The specified image is tagged with a tag that already exists. The repository is configured for tag
* immutability.
* @throws ImageDigestDoesNotMatchException
* The specified image digest does not match the digest that Amazon ECR calculated for the image.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutImage
* @see AWS API
* Documentation
*/
@Override
public PutImageResponse putImage(PutImageRequest putImageRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, ImageAlreadyExistsException, LayersNotFoundException, ReferencedImagesNotFoundException,
LimitExceededException, ImageTagAlreadyExistsException, ImageDigestDoesNotMatchException, KmsException,
AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutImageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putImageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutImage");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutImage").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putImageRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new PutImageRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the image scanning configuration for the specified repository.
*
*
* @param putImageScanningConfigurationRequest
* @return Result of the PutImageScanningConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutImageScanningConfiguration
* @see AWS API Documentation
*/
@Override
public PutImageScanningConfigurationResponse putImageScanningConfiguration(
PutImageScanningConfigurationRequest putImageScanningConfigurationRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutImageScanningConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putImageScanningConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutImageScanningConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutImageScanningConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putImageScanningConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutImageScanningConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the image tag mutability settings for the specified repository. For more information, see Image Tag Mutability
* in the Amazon Elastic Container Registry User Guide.
*
*
* @param putImageTagMutabilityRequest
* @return Result of the PutImageTagMutability operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutImageTagMutability
* @see AWS API
* Documentation
*/
@Override
public PutImageTagMutabilityResponse putImageTagMutability(PutImageTagMutabilityRequest putImageTagMutabilityRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, AwsServiceException,
SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutImageTagMutabilityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putImageTagMutabilityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutImageTagMutability");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutImageTagMutability").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putImageTagMutabilityRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutImageTagMutabilityRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or updates the lifecycle policy for the specified repository. For more information, see Lifecycle Policy
* Template.
*
*
* @param putLifecyclePolicyRequest
* @return Result of the PutLifecyclePolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutLifecyclePolicy
* @see AWS API
* Documentation
*/
@Override
public PutLifecyclePolicyResponse putLifecyclePolicy(PutLifecyclePolicyRequest putLifecyclePolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, AwsServiceException,
SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutLifecyclePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putLifecyclePolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutLifecyclePolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutLifecyclePolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putLifecyclePolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutLifecyclePolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or updates the permissions policy for your registry.
*
*
* A registry policy is used to specify permissions for another AWS account and is used when configuring
* cross-account replication. For more information, see Registry permissions
* in the Amazon Elastic Container Registry User Guide.
*
*
* @param putRegistryPolicyRequest
* @return Result of the PutRegistryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutRegistryPolicy
* @see AWS API
* Documentation
*/
@Override
public PutRegistryPolicyResponse putRegistryPolicy(PutRegistryPolicyRequest putRegistryPolicyRequest) throws ServerException,
InvalidParameterException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutRegistryPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putRegistryPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutRegistryPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutRegistryPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putRegistryPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutRegistryPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or updates the replication configuration for a registry. The existing replication configuration for a
* repository can be retrieved with the DescribeRegistry API action. The first time the
* PutReplicationConfiguration API is called, a service-linked IAM role is created in your account for the
* replication process. For more information, see Using
* Service-Linked Roles for Amazon ECR in the Amazon Elastic Container Registry User Guide.
*
*
*
* When configuring cross-account replication, the destination account must grant the source account permission to
* replicate. This permission is controlled using a registry permissions policy. For more information, see
* PutRegistryPolicy.
*
*
*
* @param putReplicationConfigurationRequest
* @return Result of the PutReplicationConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutReplicationConfiguration
* @see AWS API Documentation
*/
@Override
public PutReplicationConfigurationResponse putReplicationConfiguration(
PutReplicationConfigurationRequest putReplicationConfigurationRequest) throws ServerException,
InvalidParameterException, ValidationException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutReplicationConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putReplicationConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutReplicationConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutReplicationConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putReplicationConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutReplicationConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Applies a repository policy to the specified repository to control access permissions. For more information, see
* Amazon ECR Repository
* Policies in the Amazon Elastic Container Registry User Guide.
*
*
* @param setRepositoryPolicyRequest
* @return Result of the SetRepositoryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.SetRepositoryPolicy
* @see AWS API
* Documentation
*/
@Override
public SetRepositoryPolicyResponse setRepositoryPolicy(SetRepositoryPolicyRequest setRepositoryPolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, AwsServiceException,
SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetRepositoryPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, setRepositoryPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetRepositoryPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("SetRepositoryPolicy").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(setRepositoryPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetRepositoryPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Starts an image vulnerability scan. An image scan can only be started once per day on an individual image. This
* limit includes if an image was scanned on initial push. For more information, see Image Scanning in the
* Amazon Elastic Container Registry User Guide.
*
*
* @param startImageScanRequest
* @return Result of the StartImageScan operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws UnsupportedImageTypeException
* The image is of a type that cannot be scanned.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR Service
* Quotas in the Amazon Elastic Container Registry User Guide.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.StartImageScan
* @see AWS API
* Documentation
*/
@Override
public StartImageScanResponse startImageScan(StartImageScanRequest startImageScanRequest) throws ServerException,
InvalidParameterException, UnsupportedImageTypeException, LimitExceededException, RepositoryNotFoundException,
ImageNotFoundException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartImageScanResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startImageScanRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartImageScan");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StartImageScan").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(startImageScanRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartImageScanRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Starts a preview of a lifecycle policy for the specified repository. This allows you to see the results before
* associating the lifecycle policy with the repository.
*
*
* @param startLifecyclePolicyPreviewRequest
* @return Result of the StartLifecyclePolicyPreview operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyNotFoundException
* The lifecycle policy could not be found, and no policy is set to the repository.
* @throws LifecyclePolicyPreviewInProgressException
* The previous lifecycle policy preview request has not completed. Wait and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.StartLifecyclePolicyPreview
* @see AWS API Documentation
*/
@Override
public StartLifecyclePolicyPreviewResponse startLifecyclePolicyPreview(
StartLifecyclePolicyPreviewRequest startLifecyclePolicyPreviewRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, LifecyclePolicyNotFoundException,
LifecyclePolicyPreviewInProgressException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartLifecyclePolicyPreviewResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startLifecyclePolicyPreviewRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartLifecyclePolicyPreview");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartLifecyclePolicyPreview").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(startLifecyclePolicyPreviewRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartLifecyclePolicyPreviewRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds specified tags to a resource with the specified ARN. Existing tags on a resource are not changed if they are
* not specified in the request parameters.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidTagParameterException
* An invalid parameter has been specified. Tag keys can have a maximum character length of 128 characters,
* and tag values can have a maximum length of 256 characters.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws InvalidParameterException,
InvalidTagParameterException, TooManyTagsException, RepositoryNotFoundException, ServerException,
AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(tagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidTagParameterException
* An invalid parameter has been specified. Tag keys can have a maximum character length of 128 characters,
* and tag values can have a maximum length of 256 characters.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws InvalidParameterException,
InvalidTagParameterException, TooManyTagsException, RepositoryNotFoundException, ServerException,
AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(untagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Uploads an image layer part to Amazon ECR.
*
*
* When an image is pushed, each new image layer is uploaded in parts. The maximum size of each image layer part can
* be 20971520 bytes (or about 20MB). The UploadLayerPart API is called once per each new image layer part.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param uploadLayerPartRequest
* @return Result of the UploadLayerPart operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidLayerPartException
* The layer part size is not valid, or the first byte specified is not consecutive to the last byte of a
* previous layer part upload.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws UploadNotFoundException
* The upload could not be found, or the specified upload ID is not valid for this repository.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR Service
* Quotas in the Amazon Elastic Container Registry User Guide.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.UploadLayerPart
* @see AWS API
* Documentation
*/
@Override
public UploadLayerPartResponse uploadLayerPart(UploadLayerPartRequest uploadLayerPartRequest) throws ServerException,
InvalidParameterException, InvalidLayerPartException, RepositoryNotFoundException, UploadNotFoundException,
LimitExceededException, KmsException, AwsServiceException, SdkClientException, EcrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UploadLayerPartResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, uploadLayerPartRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UploadLayerPart");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UploadLayerPart").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(uploadLayerPartRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UploadLayerPartRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(EcrException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("LayerPartTooSmallException")
.exceptionBuilderSupplier(LayerPartTooSmallException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidParameterException")
.exceptionBuilderSupplier(InvalidParameterException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RepositoryNotEmptyException")
.exceptionBuilderSupplier(RepositoryNotEmptyException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("KmsException").exceptionBuilderSupplier(KmsException::builder)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LayerAlreadyExistsException")
.exceptionBuilderSupplier(LayerAlreadyExistsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("EmptyUploadException")
.exceptionBuilderSupplier(EmptyUploadException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ReferencedImagesNotFoundException")
.exceptionBuilderSupplier(ReferencedImagesNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RepositoryAlreadyExistsException")
.exceptionBuilderSupplier(RepositoryAlreadyExistsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RepositoryPolicyNotFoundException")
.exceptionBuilderSupplier(RepositoryPolicyNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ImageAlreadyExistsException")
.exceptionBuilderSupplier(ImageAlreadyExistsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LayerInaccessibleException")
.exceptionBuilderSupplier(LayerInaccessibleException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LimitExceededException")
.exceptionBuilderSupplier(LimitExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("UploadNotFoundException")
.exceptionBuilderSupplier(UploadNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyTagsException")
.exceptionBuilderSupplier(TooManyTagsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidLayerPartException")
.exceptionBuilderSupplier(InvalidLayerPartException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ImageTagAlreadyExistsException")
.exceptionBuilderSupplier(ImageTagAlreadyExistsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LifecyclePolicyPreviewNotFoundException")
.exceptionBuilderSupplier(LifecyclePolicyPreviewNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServerException")
.exceptionBuilderSupplier(ServerException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ScanNotFoundException")
.exceptionBuilderSupplier(ScanNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LifecyclePolicyNotFoundException")
.exceptionBuilderSupplier(LifecyclePolicyNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ImageDigestDoesNotMatchException")
.exceptionBuilderSupplier(ImageDigestDoesNotMatchException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RegistryPolicyNotFoundException")
.exceptionBuilderSupplier(RegistryPolicyNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTagParameterException")
.exceptionBuilderSupplier(InvalidTagParameterException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ImageNotFoundException")
.exceptionBuilderSupplier(ImageNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LayersNotFoundException")
.exceptionBuilderSupplier(LayersNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidLayerException")
.exceptionBuilderSupplier(InvalidLayerException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RepositoryNotFoundException")
.exceptionBuilderSupplier(RepositoryNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnsupportedImageTypeException")
.exceptionBuilderSupplier(UnsupportedImageTypeException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LifecyclePolicyPreviewInProgressException")
.exceptionBuilderSupplier(LifecyclePolicyPreviewInProgressException::builder).build());
}
@Override
public void close() {
clientHandler.close();
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
@Override
public EcrWaiter waiter() {
return EcrWaiter.builder().client(this).build();
}
}