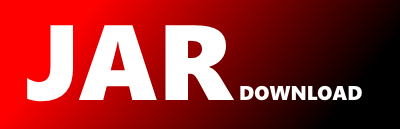
software.amazon.awssdk.services.ecr.model.EnhancedImageScanFinding Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details of an enhanced image scan. This is returned when enhanced scanning is enabled for your private registry.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EnhancedImageScanFinding implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField AWS_ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("awsAccountId").getter(getter(EnhancedImageScanFinding::awsAccountId))
.setter(setter(Builder::awsAccountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("awsAccountId").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("description").getter(getter(EnhancedImageScanFinding::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField FINDING_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("findingArn").getter(getter(EnhancedImageScanFinding::findingArn)).setter(setter(Builder::findingArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("findingArn").build()).build();
private static final SdkField FIRST_OBSERVED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("firstObservedAt").getter(getter(EnhancedImageScanFinding::firstObservedAt))
.setter(setter(Builder::firstObservedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("firstObservedAt").build()).build();
private static final SdkField LAST_OBSERVED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("lastObservedAt").getter(getter(EnhancedImageScanFinding::lastObservedAt))
.setter(setter(Builder::lastObservedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastObservedAt").build()).build();
private static final SdkField PACKAGE_VULNERABILITY_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("packageVulnerabilityDetails")
.getter(getter(EnhancedImageScanFinding::packageVulnerabilityDetails))
.setter(setter(Builder::packageVulnerabilityDetails))
.constructor(PackageVulnerabilityDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("packageVulnerabilityDetails")
.build()).build();
private static final SdkField REMEDIATION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("remediation").getter(getter(EnhancedImageScanFinding::remediation)).setter(setter(Builder::remediation))
.constructor(Remediation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remediation").build()).build();
private static final SdkField> RESOURCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("resources")
.getter(getter(EnhancedImageScanFinding::resources))
.setter(setter(Builder::resources))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resources").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Resource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SCORE_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("score")
.getter(getter(EnhancedImageScanFinding::score)).setter(setter(Builder::score))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("score").build()).build();
private static final SdkField SCORE_DETAILS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("scoreDetails").getter(getter(EnhancedImageScanFinding::scoreDetails))
.setter(setter(Builder::scoreDetails)).constructor(ScoreDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scoreDetails").build()).build();
private static final SdkField SEVERITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("severity").getter(getter(EnhancedImageScanFinding::severity)).setter(setter(Builder::severity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("severity").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(EnhancedImageScanFinding::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField TITLE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("title")
.getter(getter(EnhancedImageScanFinding::title)).setter(setter(Builder::title))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("title").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(EnhancedImageScanFinding::type)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("updatedAt").getter(getter(EnhancedImageScanFinding::updatedAt)).setter(setter(Builder::updatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updatedAt").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AWS_ACCOUNT_ID_FIELD,
DESCRIPTION_FIELD, FINDING_ARN_FIELD, FIRST_OBSERVED_AT_FIELD, LAST_OBSERVED_AT_FIELD,
PACKAGE_VULNERABILITY_DETAILS_FIELD, REMEDIATION_FIELD, RESOURCES_FIELD, SCORE_FIELD, SCORE_DETAILS_FIELD,
SEVERITY_FIELD, STATUS_FIELD, TITLE_FIELD, TYPE_FIELD, UPDATED_AT_FIELD));
private static final long serialVersionUID = 1L;
private final String awsAccountId;
private final String description;
private final String findingArn;
private final Instant firstObservedAt;
private final Instant lastObservedAt;
private final PackageVulnerabilityDetails packageVulnerabilityDetails;
private final Remediation remediation;
private final List resources;
private final Double score;
private final ScoreDetails scoreDetails;
private final String severity;
private final String status;
private final String title;
private final String type;
private final Instant updatedAt;
private EnhancedImageScanFinding(BuilderImpl builder) {
this.awsAccountId = builder.awsAccountId;
this.description = builder.description;
this.findingArn = builder.findingArn;
this.firstObservedAt = builder.firstObservedAt;
this.lastObservedAt = builder.lastObservedAt;
this.packageVulnerabilityDetails = builder.packageVulnerabilityDetails;
this.remediation = builder.remediation;
this.resources = builder.resources;
this.score = builder.score;
this.scoreDetails = builder.scoreDetails;
this.severity = builder.severity;
this.status = builder.status;
this.title = builder.title;
this.type = builder.type;
this.updatedAt = builder.updatedAt;
}
/**
*
* The Amazon Web Services account ID associated with the image.
*
*
* @return The Amazon Web Services account ID associated with the image.
*/
public final String awsAccountId() {
return awsAccountId;
}
/**
*
* The description of the finding.
*
*
* @return The description of the finding.
*/
public final String description() {
return description;
}
/**
*
* The Amazon Resource Number (ARN) of the finding.
*
*
* @return The Amazon Resource Number (ARN) of the finding.
*/
public final String findingArn() {
return findingArn;
}
/**
*
* The date and time that the finding was first observed.
*
*
* @return The date and time that the finding was first observed.
*/
public final Instant firstObservedAt() {
return firstObservedAt;
}
/**
*
* The date and time that the finding was last observed.
*
*
* @return The date and time that the finding was last observed.
*/
public final Instant lastObservedAt() {
return lastObservedAt;
}
/**
*
* An object that contains the details of a package vulnerability finding.
*
*
* @return An object that contains the details of a package vulnerability finding.
*/
public final PackageVulnerabilityDetails packageVulnerabilityDetails() {
return packageVulnerabilityDetails;
}
/**
*
* An object that contains the details about how to remediate a finding.
*
*
* @return An object that contains the details about how to remediate a finding.
*/
public final Remediation remediation() {
return remediation;
}
/**
* For responses, this returns true if the service returned a value for the Resources property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasResources() {
return resources != null && !(resources instanceof SdkAutoConstructList);
}
/**
*
* Contains information on the resources involved in a finding.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasResources} method.
*
*
* @return Contains information on the resources involved in a finding.
*/
public final List resources() {
return resources;
}
/**
*
* The Amazon Inspector score given to the finding.
*
*
* @return The Amazon Inspector score given to the finding.
*/
public final Double score() {
return score;
}
/**
*
* An object that contains details of the Amazon Inspector score.
*
*
* @return An object that contains details of the Amazon Inspector score.
*/
public final ScoreDetails scoreDetails() {
return scoreDetails;
}
/**
*
* The severity of the finding.
*
*
* @return The severity of the finding.
*/
public final String severity() {
return severity;
}
/**
*
* The status of the finding.
*
*
* @return The status of the finding.
*/
public final String status() {
return status;
}
/**
*
* The title of the finding.
*
*
* @return The title of the finding.
*/
public final String title() {
return title;
}
/**
*
* The type of the finding.
*
*
* @return The type of the finding.
*/
public final String type() {
return type;
}
/**
*
* The date and time the finding was last updated at.
*
*
* @return The date and time the finding was last updated at.
*/
public final Instant updatedAt() {
return updatedAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(awsAccountId());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(findingArn());
hashCode = 31 * hashCode + Objects.hashCode(firstObservedAt());
hashCode = 31 * hashCode + Objects.hashCode(lastObservedAt());
hashCode = 31 * hashCode + Objects.hashCode(packageVulnerabilityDetails());
hashCode = 31 * hashCode + Objects.hashCode(remediation());
hashCode = 31 * hashCode + Objects.hashCode(hasResources() ? resources() : null);
hashCode = 31 * hashCode + Objects.hashCode(score());
hashCode = 31 * hashCode + Objects.hashCode(scoreDetails());
hashCode = 31 * hashCode + Objects.hashCode(severity());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(title());
hashCode = 31 * hashCode + Objects.hashCode(type());
hashCode = 31 * hashCode + Objects.hashCode(updatedAt());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EnhancedImageScanFinding)) {
return false;
}
EnhancedImageScanFinding other = (EnhancedImageScanFinding) obj;
return Objects.equals(awsAccountId(), other.awsAccountId()) && Objects.equals(description(), other.description())
&& Objects.equals(findingArn(), other.findingArn()) && Objects.equals(firstObservedAt(), other.firstObservedAt())
&& Objects.equals(lastObservedAt(), other.lastObservedAt())
&& Objects.equals(packageVulnerabilityDetails(), other.packageVulnerabilityDetails())
&& Objects.equals(remediation(), other.remediation()) && hasResources() == other.hasResources()
&& Objects.equals(resources(), other.resources()) && Objects.equals(score(), other.score())
&& Objects.equals(scoreDetails(), other.scoreDetails()) && Objects.equals(severity(), other.severity())
&& Objects.equals(status(), other.status()) && Objects.equals(title(), other.title())
&& Objects.equals(type(), other.type()) && Objects.equals(updatedAt(), other.updatedAt());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EnhancedImageScanFinding").add("AwsAccountId", awsAccountId()).add("Description", description())
.add("FindingArn", findingArn()).add("FirstObservedAt", firstObservedAt())
.add("LastObservedAt", lastObservedAt()).add("PackageVulnerabilityDetails", packageVulnerabilityDetails())
.add("Remediation", remediation()).add("Resources", hasResources() ? resources() : null).add("Score", score())
.add("ScoreDetails", scoreDetails()).add("Severity", severity()).add("Status", status()).add("Title", title())
.add("Type", type()).add("UpdatedAt", updatedAt()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "awsAccountId":
return Optional.ofNullable(clazz.cast(awsAccountId()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "findingArn":
return Optional.ofNullable(clazz.cast(findingArn()));
case "firstObservedAt":
return Optional.ofNullable(clazz.cast(firstObservedAt()));
case "lastObservedAt":
return Optional.ofNullable(clazz.cast(lastObservedAt()));
case "packageVulnerabilityDetails":
return Optional.ofNullable(clazz.cast(packageVulnerabilityDetails()));
case "remediation":
return Optional.ofNullable(clazz.cast(remediation()));
case "resources":
return Optional.ofNullable(clazz.cast(resources()));
case "score":
return Optional.ofNullable(clazz.cast(score()));
case "scoreDetails":
return Optional.ofNullable(clazz.cast(scoreDetails()));
case "severity":
return Optional.ofNullable(clazz.cast(severity()));
case "status":
return Optional.ofNullable(clazz.cast(status()));
case "title":
return Optional.ofNullable(clazz.cast(title()));
case "type":
return Optional.ofNullable(clazz.cast(type()));
case "updatedAt":
return Optional.ofNullable(clazz.cast(updatedAt()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function