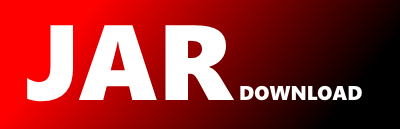
software.amazon.awssdk.services.ecr.EcrAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.ecr.model.BatchCheckLayerAvailabilityRequest;
import software.amazon.awssdk.services.ecr.model.BatchCheckLayerAvailabilityResponse;
import software.amazon.awssdk.services.ecr.model.BatchDeleteImageRequest;
import software.amazon.awssdk.services.ecr.model.BatchDeleteImageResponse;
import software.amazon.awssdk.services.ecr.model.BatchGetImageRequest;
import software.amazon.awssdk.services.ecr.model.BatchGetImageResponse;
import software.amazon.awssdk.services.ecr.model.BatchGetRepositoryScanningConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.BatchGetRepositoryScanningConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.CompleteLayerUploadRequest;
import software.amazon.awssdk.services.ecr.model.CompleteLayerUploadResponse;
import software.amazon.awssdk.services.ecr.model.CreatePullThroughCacheRuleRequest;
import software.amazon.awssdk.services.ecr.model.CreatePullThroughCacheRuleResponse;
import software.amazon.awssdk.services.ecr.model.CreateRepositoryRequest;
import software.amazon.awssdk.services.ecr.model.CreateRepositoryResponse;
import software.amazon.awssdk.services.ecr.model.DeleteLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.DeleteLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.DeletePullThroughCacheRuleRequest;
import software.amazon.awssdk.services.ecr.model.DeletePullThroughCacheRuleResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRegistryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRegistryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryResponse;
import software.amazon.awssdk.services.ecr.model.DescribeImageReplicationStatusRequest;
import software.amazon.awssdk.services.ecr.model.DescribeImageReplicationStatusResponse;
import software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest;
import software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsResponse;
import software.amazon.awssdk.services.ecr.model.DescribeImagesRequest;
import software.amazon.awssdk.services.ecr.model.DescribeImagesResponse;
import software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest;
import software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesResponse;
import software.amazon.awssdk.services.ecr.model.DescribeRegistryRequest;
import software.amazon.awssdk.services.ecr.model.DescribeRegistryResponse;
import software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest;
import software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse;
import software.amazon.awssdk.services.ecr.model.GetAuthorizationTokenRequest;
import software.amazon.awssdk.services.ecr.model.GetAuthorizationTokenResponse;
import software.amazon.awssdk.services.ecr.model.GetDownloadUrlForLayerRequest;
import software.amazon.awssdk.services.ecr.model.GetDownloadUrlForLayerResponse;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewResponse;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.GetRegistryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.GetRegistryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.GetRegistryScanningConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.GetRegistryScanningConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.GetRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.GetRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.InitiateLayerUploadRequest;
import software.amazon.awssdk.services.ecr.model.InitiateLayerUploadResponse;
import software.amazon.awssdk.services.ecr.model.ListImagesRequest;
import software.amazon.awssdk.services.ecr.model.ListImagesResponse;
import software.amazon.awssdk.services.ecr.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.ecr.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.ecr.model.PutImageRequest;
import software.amazon.awssdk.services.ecr.model.PutImageResponse;
import software.amazon.awssdk.services.ecr.model.PutImageScanningConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.PutImageScanningConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.PutImageTagMutabilityRequest;
import software.amazon.awssdk.services.ecr.model.PutImageTagMutabilityResponse;
import software.amazon.awssdk.services.ecr.model.PutLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.PutLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.PutRegistryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.PutRegistryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.PutRegistryScanningConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.PutRegistryScanningConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.PutReplicationConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.PutReplicationConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.SetRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.SetRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.StartImageScanRequest;
import software.amazon.awssdk.services.ecr.model.StartImageScanResponse;
import software.amazon.awssdk.services.ecr.model.StartLifecyclePolicyPreviewRequest;
import software.amazon.awssdk.services.ecr.model.StartLifecyclePolicyPreviewResponse;
import software.amazon.awssdk.services.ecr.model.TagResourceRequest;
import software.amazon.awssdk.services.ecr.model.TagResourceResponse;
import software.amazon.awssdk.services.ecr.model.UntagResourceRequest;
import software.amazon.awssdk.services.ecr.model.UntagResourceResponse;
import software.amazon.awssdk.services.ecr.model.UploadLayerPartRequest;
import software.amazon.awssdk.services.ecr.model.UploadLayerPartResponse;
import software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsPublisher;
import software.amazon.awssdk.services.ecr.paginators.DescribeImagesPublisher;
import software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesPublisher;
import software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher;
import software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewPublisher;
import software.amazon.awssdk.services.ecr.paginators.ListImagesPublisher;
import software.amazon.awssdk.services.ecr.waiters.EcrAsyncWaiter;
/**
* Service client for accessing Amazon ECR asynchronously. This can be created using the static {@link #builder()}
* method.
*
* Amazon Elastic Container Registry
*
* Amazon Elastic Container Registry (Amazon ECR) is a managed container image registry service. Customers can use the
* familiar Docker CLI, or their preferred client, to push, pull, and manage images. Amazon ECR provides a secure,
* scalable, and reliable registry for your Docker or Open Container Initiative (OCI) images. Amazon ECR supports
* private repositories with resource-based permissions using IAM so that specific users or Amazon EC2 instances can
* access repositories and images.
*
*
* Amazon ECR has service endpoints in each supported Region. For more information, see Amazon ECR endpoints in the Amazon Web Services
* General Reference.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface EcrAsyncClient extends SdkClient {
String SERVICE_NAME = "ecr";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "api.ecr";
/**
* Create a {@link EcrAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static EcrAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link EcrAsyncClient}.
*/
static EcrAsyncClientBuilder builder() {
return new DefaultEcrAsyncClientBuilder();
}
/**
*
* Checks the availability of one or more image layers in a repository.
*
*
* When an image is pushed to a repository, each image layer is checked to verify if it has been uploaded before. If
* it has been uploaded, then the image layer is skipped.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param batchCheckLayerAvailabilityRequest
* @return A Java Future containing the result of the BatchCheckLayerAvailability operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchCheckLayerAvailability
* @see AWS API Documentation
*/
default CompletableFuture batchCheckLayerAvailability(
BatchCheckLayerAvailabilityRequest batchCheckLayerAvailabilityRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Checks the availability of one or more image layers in a repository.
*
*
* When an image is pushed to a repository, each image layer is checked to verify if it has been uploaded before. If
* it has been uploaded, then the image layer is skipped.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link BatchCheckLayerAvailabilityRequest.Builder}
* avoiding the need to create one manually via {@link BatchCheckLayerAvailabilityRequest#builder()}
*
*
* @param batchCheckLayerAvailabilityRequest
* A {@link Consumer} that will call methods on {@link BatchCheckLayerAvailabilityRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the BatchCheckLayerAvailability operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchCheckLayerAvailability
* @see AWS API Documentation
*/
default CompletableFuture batchCheckLayerAvailability(
Consumer batchCheckLayerAvailabilityRequest) {
return batchCheckLayerAvailability(BatchCheckLayerAvailabilityRequest.builder()
.applyMutation(batchCheckLayerAvailabilityRequest).build());
}
/**
*
* Deletes a list of specified images within a repository. Images are specified with either an imageTag
* or imageDigest
.
*
*
* You can remove a tag from an image by specifying the image's tag in your request. When you remove the last tag
* from an image, the image is deleted from your repository.
*
*
* You can completely delete an image (and all of its tags) by specifying the image's digest in your request.
*
*
* @param batchDeleteImageRequest
* Deletes specified images within a specified repository. Images are specified with either the
* imageTag
or imageDigest
.
* @return A Java Future containing the result of the BatchDeleteImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchDeleteImage
* @see AWS API
* Documentation
*/
default CompletableFuture batchDeleteImage(BatchDeleteImageRequest batchDeleteImageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a list of specified images within a repository. Images are specified with either an imageTag
* or imageDigest
.
*
*
* You can remove a tag from an image by specifying the image's tag in your request. When you remove the last tag
* from an image, the image is deleted from your repository.
*
*
* You can completely delete an image (and all of its tags) by specifying the image's digest in your request.
*
*
*
* This is a convenience which creates an instance of the {@link BatchDeleteImageRequest.Builder} avoiding the need
* to create one manually via {@link BatchDeleteImageRequest#builder()}
*
*
* @param batchDeleteImageRequest
* A {@link Consumer} that will call methods on {@link BatchDeleteImageRequest.Builder} to create a request.
* Deletes specified images within a specified repository. Images are specified with either the
* imageTag
or imageDigest
.
* @return A Java Future containing the result of the BatchDeleteImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchDeleteImage
* @see AWS API
* Documentation
*/
default CompletableFuture batchDeleteImage(
Consumer batchDeleteImageRequest) {
return batchDeleteImage(BatchDeleteImageRequest.builder().applyMutation(batchDeleteImageRequest).build());
}
/**
*
* Gets detailed information for an image. Images are specified with either an imageTag
or
* imageDigest
.
*
*
* When an image is pulled, the BatchGetImage API is called once to retrieve the image manifest.
*
*
* @param batchGetImageRequest
* @return A Java Future containing the result of the BatchGetImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchGetImage
* @see AWS API
* Documentation
*/
default CompletableFuture batchGetImage(BatchGetImageRequest batchGetImageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets detailed information for an image. Images are specified with either an imageTag
or
* imageDigest
.
*
*
* When an image is pulled, the BatchGetImage API is called once to retrieve the image manifest.
*
*
*
* This is a convenience which creates an instance of the {@link BatchGetImageRequest.Builder} avoiding the need to
* create one manually via {@link BatchGetImageRequest#builder()}
*
*
* @param batchGetImageRequest
* A {@link Consumer} that will call methods on {@link BatchGetImageRequest.Builder} to create a request.
* @return A Java Future containing the result of the BatchGetImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchGetImage
* @see AWS API
* Documentation
*/
default CompletableFuture batchGetImage(Consumer batchGetImageRequest) {
return batchGetImage(BatchGetImageRequest.builder().applyMutation(batchGetImageRequest).build());
}
/**
*
* Gets the scanning configuration for one or more repositories.
*
*
* @param batchGetRepositoryScanningConfigurationRequest
* @return A Java Future containing the result of the BatchGetRepositoryScanningConfiguration operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchGetRepositoryScanningConfiguration
* @see AWS API Documentation
*/
default CompletableFuture batchGetRepositoryScanningConfiguration(
BatchGetRepositoryScanningConfigurationRequest batchGetRepositoryScanningConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the scanning configuration for one or more repositories.
*
*
*
* This is a convenience which creates an instance of the
* {@link BatchGetRepositoryScanningConfigurationRequest.Builder} avoiding the need to create one manually via
* {@link BatchGetRepositoryScanningConfigurationRequest#builder()}
*
*
* @param batchGetRepositoryScanningConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link BatchGetRepositoryScanningConfigurationRequest.Builder} to create a request.
* @return A Java Future containing the result of the BatchGetRepositoryScanningConfiguration operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchGetRepositoryScanningConfiguration
* @see AWS API Documentation
*/
default CompletableFuture batchGetRepositoryScanningConfiguration(
Consumer batchGetRepositoryScanningConfigurationRequest) {
return batchGetRepositoryScanningConfiguration(BatchGetRepositoryScanningConfigurationRequest.builder()
.applyMutation(batchGetRepositoryScanningConfigurationRequest).build());
}
/**
*
* Informs Amazon ECR that the image layer upload has completed for a specified registry, repository name, and
* upload ID. You can optionally provide a sha256
digest of the image layer for data validation
* purposes.
*
*
* When an image is pushed, the CompleteLayerUpload API is called once per each new image layer to verify that the
* upload has completed.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param completeLayerUploadRequest
* @return A Java Future containing the result of the CompleteLayerUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - UploadNotFoundException The upload could not be found, or the specified upload ID is not valid for
* this repository.
* - InvalidLayerException The layer digest calculation performed by Amazon ECR upon receipt of the image
* layer does not match the digest specified.
* - LayerPartTooSmallException Layer parts must be at least 5 MiB in size.
* - LayerAlreadyExistsException The image layer already exists in the associated repository.
* - EmptyUploadException The specified layer upload does not contain any layer parts.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.CompleteLayerUpload
* @see AWS API
* Documentation
*/
default CompletableFuture completeLayerUpload(
CompleteLayerUploadRequest completeLayerUploadRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Informs Amazon ECR that the image layer upload has completed for a specified registry, repository name, and
* upload ID. You can optionally provide a sha256
digest of the image layer for data validation
* purposes.
*
*
* When an image is pushed, the CompleteLayerUpload API is called once per each new image layer to verify that the
* upload has completed.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link CompleteLayerUploadRequest.Builder} avoiding the
* need to create one manually via {@link CompleteLayerUploadRequest#builder()}
*
*
* @param completeLayerUploadRequest
* A {@link Consumer} that will call methods on {@link CompleteLayerUploadRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CompleteLayerUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - UploadNotFoundException The upload could not be found, or the specified upload ID is not valid for
* this repository.
* - InvalidLayerException The layer digest calculation performed by Amazon ECR upon receipt of the image
* layer does not match the digest specified.
* - LayerPartTooSmallException Layer parts must be at least 5 MiB in size.
* - LayerAlreadyExistsException The image layer already exists in the associated repository.
* - EmptyUploadException The specified layer upload does not contain any layer parts.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.CompleteLayerUpload
* @see AWS API
* Documentation
*/
default CompletableFuture completeLayerUpload(
Consumer completeLayerUploadRequest) {
return completeLayerUpload(CompleteLayerUploadRequest.builder().applyMutation(completeLayerUploadRequest).build());
}
/**
*
* Creates a pull through cache rule. A pull through cache rule provides a way to cache images from an external
* public registry in your Amazon ECR private registry.
*
*
* @param createPullThroughCacheRuleRequest
* @return A Java Future containing the result of the CreatePullThroughCacheRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - PullThroughCacheRuleAlreadyExistsException A pull through cache rule with these settings already
* exists for the private registry.
* - UnsupportedUpstreamRegistryException The specified upstream registry isn't supported.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.CreatePullThroughCacheRule
* @see AWS API Documentation
*/
default CompletableFuture createPullThroughCacheRule(
CreatePullThroughCacheRuleRequest createPullThroughCacheRuleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a pull through cache rule. A pull through cache rule provides a way to cache images from an external
* public registry in your Amazon ECR private registry.
*
*
*
* This is a convenience which creates an instance of the {@link CreatePullThroughCacheRuleRequest.Builder} avoiding
* the need to create one manually via {@link CreatePullThroughCacheRuleRequest#builder()}
*
*
* @param createPullThroughCacheRuleRequest
* A {@link Consumer} that will call methods on {@link CreatePullThroughCacheRuleRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreatePullThroughCacheRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - PullThroughCacheRuleAlreadyExistsException A pull through cache rule with these settings already
* exists for the private registry.
* - UnsupportedUpstreamRegistryException The specified upstream registry isn't supported.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.CreatePullThroughCacheRule
* @see AWS API Documentation
*/
default CompletableFuture createPullThroughCacheRule(
Consumer createPullThroughCacheRuleRequest) {
return createPullThroughCacheRule(CreatePullThroughCacheRuleRequest.builder()
.applyMutation(createPullThroughCacheRuleRequest).build());
}
/**
*
* Creates a repository. For more information, see Amazon ECR repositories in
* the Amazon Elastic Container Registry User Guide.
*
*
* @param createRepositoryRequest
* @return A Java Future containing the result of the CreateRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryAlreadyExistsException The specified repository already exists in the specified registry.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.CreateRepository
* @see AWS API
* Documentation
*/
default CompletableFuture createRepository(CreateRepositoryRequest createRepositoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a repository. For more information, see Amazon ECR repositories in
* the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRepositoryRequest.Builder} avoiding the need
* to create one manually via {@link CreateRepositoryRequest#builder()}
*
*
* @param createRepositoryRequest
* A {@link Consumer} that will call methods on {@link CreateRepositoryRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryAlreadyExistsException The specified repository already exists in the specified registry.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.CreateRepository
* @see AWS API
* Documentation
*/
default CompletableFuture createRepository(
Consumer createRepositoryRequest) {
return createRepository(CreateRepositoryRequest.builder().applyMutation(createRepositoryRequest).build());
}
/**
*
* Deletes the lifecycle policy associated with the specified repository.
*
*
* @param deleteLifecyclePolicyRequest
* @return A Java Future containing the result of the DeleteLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture deleteLifecyclePolicy(
DeleteLifecyclePolicyRequest deleteLifecyclePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the lifecycle policy associated with the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteLifecyclePolicyRequest#builder()}
*
*
* @param deleteLifecyclePolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteLifecyclePolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture deleteLifecyclePolicy(
Consumer deleteLifecyclePolicyRequest) {
return deleteLifecyclePolicy(DeleteLifecyclePolicyRequest.builder().applyMutation(deleteLifecyclePolicyRequest).build());
}
/**
*
* Deletes a pull through cache rule.
*
*
* @param deletePullThroughCacheRuleRequest
* @return A Java Future containing the result of the DeletePullThroughCacheRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - PullThroughCacheRuleNotFoundException The pull through cache rule was not found. Specify a valid pull
* through cache rule and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeletePullThroughCacheRule
* @see AWS API Documentation
*/
default CompletableFuture deletePullThroughCacheRule(
DeletePullThroughCacheRuleRequest deletePullThroughCacheRuleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a pull through cache rule.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePullThroughCacheRuleRequest.Builder} avoiding
* the need to create one manually via {@link DeletePullThroughCacheRuleRequest#builder()}
*
*
* @param deletePullThroughCacheRuleRequest
* A {@link Consumer} that will call methods on {@link DeletePullThroughCacheRuleRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeletePullThroughCacheRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - PullThroughCacheRuleNotFoundException The pull through cache rule was not found. Specify a valid pull
* through cache rule and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeletePullThroughCacheRule
* @see AWS API Documentation
*/
default CompletableFuture deletePullThroughCacheRule(
Consumer deletePullThroughCacheRuleRequest) {
return deletePullThroughCacheRule(DeletePullThroughCacheRuleRequest.builder()
.applyMutation(deletePullThroughCacheRuleRequest).build());
}
/**
*
* Deletes the registry permissions policy.
*
*
* @param deleteRegistryPolicyRequest
* @return A Java Future containing the result of the DeleteRegistryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RegistryPolicyNotFoundException The registry doesn't have an associated registry policy.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteRegistryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRegistryPolicy(
DeleteRegistryPolicyRequest deleteRegistryPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the registry permissions policy.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRegistryPolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteRegistryPolicyRequest#builder()}
*
*
* @param deleteRegistryPolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteRegistryPolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteRegistryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RegistryPolicyNotFoundException The registry doesn't have an associated registry policy.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteRegistryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRegistryPolicy(
Consumer deleteRegistryPolicyRequest) {
return deleteRegistryPolicy(DeleteRegistryPolicyRequest.builder().applyMutation(deleteRegistryPolicyRequest).build());
}
/**
*
* Deletes a repository. If the repository contains images, you must either delete all images in the repository or
* use the force
option to delete the repository.
*
*
* @param deleteRepositoryRequest
* @return A Java Future containing the result of the DeleteRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryNotEmptyException The specified repository contains images. To delete a repository that
* contains images, you must force the deletion with the
force
parameter.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteRepository
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRepository(DeleteRepositoryRequest deleteRepositoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a repository. If the repository contains images, you must either delete all images in the repository or
* use the force
option to delete the repository.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRepositoryRequest.Builder} avoiding the need
* to create one manually via {@link DeleteRepositoryRequest#builder()}
*
*
* @param deleteRepositoryRequest
* A {@link Consumer} that will call methods on {@link DeleteRepositoryRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryNotEmptyException The specified repository contains images. To delete a repository that
* contains images, you must force the deletion with the
force
parameter.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteRepository
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRepository(
Consumer deleteRepositoryRequest) {
return deleteRepository(DeleteRepositoryRequest.builder().applyMutation(deleteRepositoryRequest).build());
}
/**
*
* Deletes the repository policy associated with the specified repository.
*
*
* @param deleteRepositoryPolicyRequest
* @return A Java Future containing the result of the DeleteRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryPolicyNotFoundException The specified repository and registry combination does not have an
* associated repository policy.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteRepositoryPolicy
* @see AWS
* API Documentation
*/
default CompletableFuture deleteRepositoryPolicy(
DeleteRepositoryPolicyRequest deleteRepositoryPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the repository policy associated with the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRepositoryPolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteRepositoryPolicyRequest#builder()}
*
*
* @param deleteRepositoryPolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteRepositoryPolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryPolicyNotFoundException The specified repository and registry combination does not have an
* associated repository policy.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteRepositoryPolicy
* @see AWS
* API Documentation
*/
default CompletableFuture deleteRepositoryPolicy(
Consumer deleteRepositoryPolicyRequest) {
return deleteRepositoryPolicy(DeleteRepositoryPolicyRequest.builder().applyMutation(deleteRepositoryPolicyRequest)
.build());
}
/**
*
* Returns the replication status for a specified image.
*
*
* @param describeImageReplicationStatusRequest
* @return A Java Future containing the result of the DescribeImageReplicationStatus operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImageReplicationStatus
* @see AWS API Documentation
*/
default CompletableFuture describeImageReplicationStatus(
DescribeImageReplicationStatusRequest describeImageReplicationStatusRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the replication status for a specified image.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImageReplicationStatusRequest.Builder}
* avoiding the need to create one manually via {@link DescribeImageReplicationStatusRequest#builder()}
*
*
* @param describeImageReplicationStatusRequest
* A {@link Consumer} that will call methods on {@link DescribeImageReplicationStatusRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeImageReplicationStatus operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImageReplicationStatus
* @see AWS API Documentation
*/
default CompletableFuture describeImageReplicationStatus(
Consumer describeImageReplicationStatusRequest) {
return describeImageReplicationStatus(DescribeImageReplicationStatusRequest.builder()
.applyMutation(describeImageReplicationStatusRequest).build());
}
/**
*
* Returns the scan findings for the specified image.
*
*
* @param describeImageScanFindingsRequest
* @return A Java Future containing the result of the DescribeImageScanFindings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - ScanNotFoundException The specified image scan could not be found. Ensure that image scanning is
* enabled on the repository and try again.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImageScanFindings
* @see AWS
* API Documentation
*/
default CompletableFuture describeImageScanFindings(
DescribeImageScanFindingsRequest describeImageScanFindingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the scan findings for the specified image.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImageScanFindingsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeImageScanFindingsRequest#builder()}
*
*
* @param describeImageScanFindingsRequest
* A {@link Consumer} that will call methods on {@link DescribeImageScanFindingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeImageScanFindings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - ScanNotFoundException The specified image scan could not be found. Ensure that image scanning is
* enabled on the repository and try again.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImageScanFindings
* @see AWS
* API Documentation
*/
default CompletableFuture describeImageScanFindings(
Consumer describeImageScanFindingsRequest) {
return describeImageScanFindings(DescribeImageScanFindingsRequest.builder()
.applyMutation(describeImageScanFindingsRequest).build());
}
/**
*
* Returns the scan findings for the specified image.
*
*
*
* This is a variant of
* {@link #describeImageScanFindings(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsPublisher publisher = client.describeImageScanFindingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsPublisher publisher = client.describeImageScanFindingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImageScanFindings(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest)}
* operation.
*
*
* @param describeImageScanFindingsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - ScanNotFoundException The specified image scan could not be found. Ensure that image scanning is
* enabled on the repository and try again.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImageScanFindings
* @see AWS
* API Documentation
*/
default DescribeImageScanFindingsPublisher describeImageScanFindingsPaginator(
DescribeImageScanFindingsRequest describeImageScanFindingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the scan findings for the specified image.
*
*
*
* This is a variant of
* {@link #describeImageScanFindings(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsPublisher publisher = client.describeImageScanFindingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsPublisher publisher = client.describeImageScanFindingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImageScanFindings(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeImageScanFindingsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeImageScanFindingsRequest#builder()}
*
*
* @param describeImageScanFindingsRequest
* A {@link Consumer} that will call methods on {@link DescribeImageScanFindingsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - ScanNotFoundException The specified image scan could not be found. Ensure that image scanning is
* enabled on the repository and try again.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImageScanFindings
* @see AWS
* API Documentation
*/
default DescribeImageScanFindingsPublisher describeImageScanFindingsPaginator(
Consumer describeImageScanFindingsRequest) {
return describeImageScanFindingsPaginator(DescribeImageScanFindingsRequest.builder()
.applyMutation(describeImageScanFindingsRequest).build());
}
/**
*
* Returns metadata about the images in a repository.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* @param describeImagesRequest
* @return A Java Future containing the result of the DescribeImages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default CompletableFuture describeImages(DescribeImagesRequest describeImagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns metadata about the images in a repository.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImagesRequest.Builder} avoiding the need to
* create one manually via {@link DescribeImagesRequest#builder()}
*
*
* @param describeImagesRequest
* A {@link Consumer} that will call methods on {@link DescribeImagesRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeImages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default CompletableFuture describeImages(Consumer describeImagesRequest) {
return describeImages(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build());
}
/**
*
* Returns metadata about the images in a repository.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* This is a variant of {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeImagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)} operation.
*
*
* @param describeImagesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default DescribeImagesPublisher describeImagesPaginator(DescribeImagesRequest describeImagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns metadata about the images in a repository.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* This is a variant of {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeImagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeImagesRequest.Builder} avoiding the need to
* create one manually via {@link DescribeImagesRequest#builder()}
*
*
* @param describeImagesRequest
* A {@link Consumer} that will call methods on {@link DescribeImagesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default DescribeImagesPublisher describeImagesPaginator(Consumer describeImagesRequest) {
return describeImagesPaginator(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build());
}
/**
*
* Returns the pull through cache rules for a registry.
*
*
* @param describePullThroughCacheRulesRequest
* @return A Java Future containing the result of the DescribePullThroughCacheRules operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - PullThroughCacheRuleNotFoundException The pull through cache rule was not found. Specify a valid pull
* through cache rule and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribePullThroughCacheRules
* @see AWS API Documentation
*/
default CompletableFuture describePullThroughCacheRules(
DescribePullThroughCacheRulesRequest describePullThroughCacheRulesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the pull through cache rules for a registry.
*
*
*
* This is a convenience which creates an instance of the {@link DescribePullThroughCacheRulesRequest.Builder}
* avoiding the need to create one manually via {@link DescribePullThroughCacheRulesRequest#builder()}
*
*
* @param describePullThroughCacheRulesRequest
* A {@link Consumer} that will call methods on {@link DescribePullThroughCacheRulesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribePullThroughCacheRules operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - PullThroughCacheRuleNotFoundException The pull through cache rule was not found. Specify a valid pull
* through cache rule and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribePullThroughCacheRules
* @see AWS API Documentation
*/
default CompletableFuture describePullThroughCacheRules(
Consumer describePullThroughCacheRulesRequest) {
return describePullThroughCacheRules(DescribePullThroughCacheRulesRequest.builder()
.applyMutation(describePullThroughCacheRulesRequest).build());
}
/**
*
* Returns the pull through cache rules for a registry.
*
*
*
* This is a variant of
* {@link #describePullThroughCacheRules(software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesPublisher publisher = client.describePullThroughCacheRulesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesPublisher publisher = client.describePullThroughCacheRulesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describePullThroughCacheRules(software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest)}
* operation.
*
*
* @param describePullThroughCacheRulesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - PullThroughCacheRuleNotFoundException The pull through cache rule was not found. Specify a valid pull
* through cache rule and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribePullThroughCacheRules
* @see AWS API Documentation
*/
default DescribePullThroughCacheRulesPublisher describePullThroughCacheRulesPaginator(
DescribePullThroughCacheRulesRequest describePullThroughCacheRulesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the pull through cache rules for a registry.
*
*
*
* This is a variant of
* {@link #describePullThroughCacheRules(software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesPublisher publisher = client.describePullThroughCacheRulesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesPublisher publisher = client.describePullThroughCacheRulesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describePullThroughCacheRules(software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribePullThroughCacheRulesRequest.Builder}
* avoiding the need to create one manually via {@link DescribePullThroughCacheRulesRequest#builder()}
*
*
* @param describePullThroughCacheRulesRequest
* A {@link Consumer} that will call methods on {@link DescribePullThroughCacheRulesRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - PullThroughCacheRuleNotFoundException The pull through cache rule was not found. Specify a valid pull
* through cache rule and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribePullThroughCacheRules
* @see AWS API Documentation
*/
default DescribePullThroughCacheRulesPublisher describePullThroughCacheRulesPaginator(
Consumer describePullThroughCacheRulesRequest) {
return describePullThroughCacheRulesPaginator(DescribePullThroughCacheRulesRequest.builder()
.applyMutation(describePullThroughCacheRulesRequest).build());
}
/**
*
* Describes the settings for a registry. The replication configuration for a repository can be created or updated
* with the PutReplicationConfiguration API action.
*
*
* @param describeRegistryRequest
* @return A Java Future containing the result of the DescribeRegistry operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRegistry
* @see AWS API
* Documentation
*/
default CompletableFuture describeRegistry(DescribeRegistryRequest describeRegistryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the settings for a registry. The replication configuration for a repository can be created or updated
* with the PutReplicationConfiguration API action.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRegistryRequest.Builder} avoiding the need
* to create one manually via {@link DescribeRegistryRequest#builder()}
*
*
* @param describeRegistryRequest
* A {@link Consumer} that will call methods on {@link DescribeRegistryRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeRegistry operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRegistry
* @see AWS API
* Documentation
*/
default CompletableFuture describeRegistry(
Consumer describeRegistryRequest) {
return describeRegistry(DescribeRegistryRequest.builder().applyMutation(describeRegistryRequest).build());
}
/**
*
* Describes image repositories in a registry.
*
*
* @param describeRepositoriesRequest
* @return A Java Future containing the result of the DescribeRepositories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default CompletableFuture describeRepositories(
DescribeRepositoriesRequest describeRepositoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes image repositories in a registry.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRepositoriesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRepositoriesRequest#builder()}
*
*
* @param describeRepositoriesRequest
* A {@link Consumer} that will call methods on {@link DescribeRepositoriesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeRepositories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default CompletableFuture describeRepositories(
Consumer describeRepositoriesRequest) {
return describeRepositories(DescribeRepositoriesRequest.builder().applyMutation(describeRepositoriesRequest).build());
}
/**
*
* Describes image repositories in a registry.
*
*
* @return A Java Future containing the result of the DescribeRepositories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default CompletableFuture describeRepositories() {
return describeRepositories(DescribeRepositoriesRequest.builder().build());
}
/**
*
* Describes image repositories in a registry.
*
*
*
* This is a variant of
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default DescribeRepositoriesPublisher describeRepositoriesPaginator() {
return describeRepositoriesPaginator(DescribeRepositoriesRequest.builder().build());
}
/**
*
* Describes image repositories in a registry.
*
*
*
* This is a variant of
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)}
* operation.
*
*
* @param describeRepositoriesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default DescribeRepositoriesPublisher describeRepositoriesPaginator(DescribeRepositoriesRequest describeRepositoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes image repositories in a registry.
*
*
*
* This is a variant of
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeRepositoriesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRepositoriesRequest#builder()}
*
*
* @param describeRepositoriesRequest
* A {@link Consumer} that will call methods on {@link DescribeRepositoriesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default DescribeRepositoriesPublisher describeRepositoriesPaginator(
Consumer describeRepositoriesRequest) {
return describeRepositoriesPaginator(DescribeRepositoriesRequest.builder().applyMutation(describeRepositoriesRequest)
.build());
}
/**
*
* Retrieves an authorization token. An authorization token represents your IAM authentication credentials and can
* be used to access any Amazon ECR registry that your IAM principal has access to. The authorization token is valid
* for 12 hours.
*
*
* The authorizationToken
returned is a base64 encoded string that can be decoded and used in a
* docker login
command to authenticate to a registry. The CLI offers an
* get-login-password
command that simplifies the login process. For more information, see Registry
* authentication in the Amazon Elastic Container Registry User Guide.
*
*
* @param getAuthorizationTokenRequest
* @return A Java Future containing the result of the GetAuthorizationToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetAuthorizationToken
* @see AWS API
* Documentation
*/
default CompletableFuture getAuthorizationToken(
GetAuthorizationTokenRequest getAuthorizationTokenRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves an authorization token. An authorization token represents your IAM authentication credentials and can
* be used to access any Amazon ECR registry that your IAM principal has access to. The authorization token is valid
* for 12 hours.
*
*
* The authorizationToken
returned is a base64 encoded string that can be decoded and used in a
* docker login
command to authenticate to a registry. The CLI offers an
* get-login-password
command that simplifies the login process. For more information, see Registry
* authentication in the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetAuthorizationTokenRequest.Builder} avoiding the
* need to create one manually via {@link GetAuthorizationTokenRequest#builder()}
*
*
* @param getAuthorizationTokenRequest
* A {@link Consumer} that will call methods on {@link GetAuthorizationTokenRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetAuthorizationToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetAuthorizationToken
* @see AWS API
* Documentation
*/
default CompletableFuture getAuthorizationToken(
Consumer getAuthorizationTokenRequest) {
return getAuthorizationToken(GetAuthorizationTokenRequest.builder().applyMutation(getAuthorizationTokenRequest).build());
}
/**
*
* Retrieves an authorization token. An authorization token represents your IAM authentication credentials and can
* be used to access any Amazon ECR registry that your IAM principal has access to. The authorization token is valid
* for 12 hours.
*
*
* The authorizationToken
returned is a base64 encoded string that can be decoded and used in a
* docker login
command to authenticate to a registry. The CLI offers an
* get-login-password
command that simplifies the login process. For more information, see Registry
* authentication in the Amazon Elastic Container Registry User Guide.
*
*
* @return A Java Future containing the result of the GetAuthorizationToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetAuthorizationToken
* @see AWS API
* Documentation
*/
default CompletableFuture getAuthorizationToken() {
return getAuthorizationToken(GetAuthorizationTokenRequest.builder().build());
}
/**
*
* Retrieves the pre-signed Amazon S3 download URL corresponding to an image layer. You can only get URLs for image
* layers that are referenced in an image.
*
*
* When an image is pulled, the GetDownloadUrlForLayer API is called once per image layer that is not already
* cached.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param getDownloadUrlForLayerRequest
* @return A Java Future containing the result of the GetDownloadUrlForLayer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - LayersNotFoundException The specified layers could not be found, or the specified layer is not valid
* for this repository.
* - LayerInaccessibleException The specified layer is not available because it is not associated with an
* image. Unassociated image layers may be cleaned up at any time.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetDownloadUrlForLayer
* @see AWS
* API Documentation
*/
default CompletableFuture getDownloadUrlForLayer(
GetDownloadUrlForLayerRequest getDownloadUrlForLayerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the pre-signed Amazon S3 download URL corresponding to an image layer. You can only get URLs for image
* layers that are referenced in an image.
*
*
* When an image is pulled, the GetDownloadUrlForLayer API is called once per image layer that is not already
* cached.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link GetDownloadUrlForLayerRequest.Builder} avoiding the
* need to create one manually via {@link GetDownloadUrlForLayerRequest#builder()}
*
*
* @param getDownloadUrlForLayerRequest
* A {@link Consumer} that will call methods on {@link GetDownloadUrlForLayerRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetDownloadUrlForLayer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - LayersNotFoundException The specified layers could not be found, or the specified layer is not valid
* for this repository.
* - LayerInaccessibleException The specified layer is not available because it is not associated with an
* image. Unassociated image layers may be cleaned up at any time.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetDownloadUrlForLayer
* @see AWS
* API Documentation
*/
default CompletableFuture getDownloadUrlForLayer(
Consumer getDownloadUrlForLayerRequest) {
return getDownloadUrlForLayer(GetDownloadUrlForLayerRequest.builder().applyMutation(getDownloadUrlForLayerRequest)
.build());
}
/**
*
* Retrieves the lifecycle policy for the specified repository.
*
*
* @param getLifecyclePolicyRequest
* @return A Java Future containing the result of the GetLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getLifecyclePolicy(GetLifecyclePolicyRequest getLifecyclePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the lifecycle policy for the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link GetLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetLifecyclePolicyRequest#builder()}
*
*
* @param getLifecyclePolicyRequest
* A {@link Consumer} that will call methods on {@link GetLifecyclePolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getLifecyclePolicy(
Consumer getLifecyclePolicyRequest) {
return getLifecyclePolicy(GetLifecyclePolicyRequest.builder().applyMutation(getLifecyclePolicyRequest).build());
}
/**
*
* Retrieves the results of the lifecycle policy preview request for the specified repository.
*
*
* @param getLifecyclePolicyPreviewRequest
* @return A Java Future containing the result of the GetLifecyclePolicyPreview operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyPreviewNotFoundException There is no dry run for this repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
default CompletableFuture getLifecyclePolicyPreview(
GetLifecyclePolicyPreviewRequest getLifecyclePolicyPreviewRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the results of the lifecycle policy preview request for the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link GetLifecyclePolicyPreviewRequest.Builder} avoiding
* the need to create one manually via {@link GetLifecyclePolicyPreviewRequest#builder()}
*
*
* @param getLifecyclePolicyPreviewRequest
* A {@link Consumer} that will call methods on {@link GetLifecyclePolicyPreviewRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetLifecyclePolicyPreview operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyPreviewNotFoundException There is no dry run for this repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
default CompletableFuture getLifecyclePolicyPreview(
Consumer getLifecyclePolicyPreviewRequest) {
return getLifecyclePolicyPreview(GetLifecyclePolicyPreviewRequest.builder()
.applyMutation(getLifecyclePolicyPreviewRequest).build());
}
/**
*
* Retrieves the results of the lifecycle policy preview request for the specified repository.
*
*
*
* This is a variant of
* {@link #getLifecyclePolicyPreview(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewPublisher publisher = client.getLifecyclePolicyPreviewPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewPublisher publisher = client.getLifecyclePolicyPreviewPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLifecyclePolicyPreview(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest)}
* operation.
*
*
* @param getLifecyclePolicyPreviewRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyPreviewNotFoundException There is no dry run for this repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
default GetLifecyclePolicyPreviewPublisher getLifecyclePolicyPreviewPaginator(
GetLifecyclePolicyPreviewRequest getLifecyclePolicyPreviewRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the results of the lifecycle policy preview request for the specified repository.
*
*
*
* This is a variant of
* {@link #getLifecyclePolicyPreview(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewPublisher publisher = client.getLifecyclePolicyPreviewPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewPublisher publisher = client.getLifecyclePolicyPreviewPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLifecyclePolicyPreview(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetLifecyclePolicyPreviewRequest.Builder} avoiding
* the need to create one manually via {@link GetLifecyclePolicyPreviewRequest#builder()}
*
*
* @param getLifecyclePolicyPreviewRequest
* A {@link Consumer} that will call methods on {@link GetLifecyclePolicyPreviewRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyPreviewNotFoundException There is no dry run for this repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
default GetLifecyclePolicyPreviewPublisher getLifecyclePolicyPreviewPaginator(
Consumer getLifecyclePolicyPreviewRequest) {
return getLifecyclePolicyPreviewPaginator(GetLifecyclePolicyPreviewRequest.builder()
.applyMutation(getLifecyclePolicyPreviewRequest).build());
}
/**
*
* Retrieves the permissions policy for a registry.
*
*
* @param getRegistryPolicyRequest
* @return A Java Future containing the result of the GetRegistryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RegistryPolicyNotFoundException The registry doesn't have an associated registry policy.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetRegistryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getRegistryPolicy(GetRegistryPolicyRequest getRegistryPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the permissions policy for a registry.
*
*
*
* This is a convenience which creates an instance of the {@link GetRegistryPolicyRequest.Builder} avoiding the need
* to create one manually via {@link GetRegistryPolicyRequest#builder()}
*
*
* @param getRegistryPolicyRequest
* A {@link Consumer} that will call methods on {@link GetRegistryPolicyRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetRegistryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RegistryPolicyNotFoundException The registry doesn't have an associated registry policy.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetRegistryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getRegistryPolicy(
Consumer getRegistryPolicyRequest) {
return getRegistryPolicy(GetRegistryPolicyRequest.builder().applyMutation(getRegistryPolicyRequest).build());
}
/**
*
* Retrieves the scanning configuration for a registry.
*
*
* @param getRegistryScanningConfigurationRequest
* @return A Java Future containing the result of the GetRegistryScanningConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetRegistryScanningConfiguration
* @see AWS API Documentation
*/
default CompletableFuture getRegistryScanningConfiguration(
GetRegistryScanningConfigurationRequest getRegistryScanningConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the scanning configuration for a registry.
*
*
*
* This is a convenience which creates an instance of the {@link GetRegistryScanningConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link GetRegistryScanningConfigurationRequest#builder()}
*
*
* @param getRegistryScanningConfigurationRequest
* A {@link Consumer} that will call methods on {@link GetRegistryScanningConfigurationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetRegistryScanningConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetRegistryScanningConfiguration
* @see AWS API Documentation
*/
default CompletableFuture getRegistryScanningConfiguration(
Consumer getRegistryScanningConfigurationRequest) {
return getRegistryScanningConfiguration(GetRegistryScanningConfigurationRequest.builder()
.applyMutation(getRegistryScanningConfigurationRequest).build());
}
/**
*
* Retrieves the repository policy for the specified repository.
*
*
* @param getRepositoryPolicyRequest
* @return A Java Future containing the result of the GetRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryPolicyNotFoundException The specified repository and registry combination does not have an
* associated repository policy.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetRepositoryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getRepositoryPolicy(
GetRepositoryPolicyRequest getRepositoryPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the repository policy for the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link GetRepositoryPolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetRepositoryPolicyRequest#builder()}
*
*
* @param getRepositoryPolicyRequest
* A {@link Consumer} that will call methods on {@link GetRepositoryPolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryPolicyNotFoundException The specified repository and registry combination does not have an
* associated repository policy.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetRepositoryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getRepositoryPolicy(
Consumer getRepositoryPolicyRequest) {
return getRepositoryPolicy(GetRepositoryPolicyRequest.builder().applyMutation(getRepositoryPolicyRequest).build());
}
/**
*
* Notifies Amazon ECR that you intend to upload an image layer.
*
*
* When an image is pushed, the InitiateLayerUpload API is called once per image layer that has not already been
* uploaded. Whether or not an image layer has been uploaded is determined by the BatchCheckLayerAvailability API
* action.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param initiateLayerUploadRequest
* @return A Java Future containing the result of the InitiateLayerUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.InitiateLayerUpload
* @see AWS API
* Documentation
*/
default CompletableFuture initiateLayerUpload(
InitiateLayerUploadRequest initiateLayerUploadRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Notifies Amazon ECR that you intend to upload an image layer.
*
*
* When an image is pushed, the InitiateLayerUpload API is called once per image layer that has not already been
* uploaded. Whether or not an image layer has been uploaded is determined by the BatchCheckLayerAvailability API
* action.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link InitiateLayerUploadRequest.Builder} avoiding the
* need to create one manually via {@link InitiateLayerUploadRequest#builder()}
*
*
* @param initiateLayerUploadRequest
* A {@link Consumer} that will call methods on {@link InitiateLayerUploadRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the InitiateLayerUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.InitiateLayerUpload
* @see AWS API
* Documentation
*/
default CompletableFuture initiateLayerUpload(
Consumer initiateLayerUploadRequest) {
return initiateLayerUpload(InitiateLayerUploadRequest.builder().applyMutation(initiateLayerUploadRequest).build());
}
/**
*
* Lists all the image IDs for the specified repository.
*
*
* You can filter images based on whether or not they are tagged by using the tagStatus
filter and
* specifying either TAGGED
, UNTAGGED
or ANY
. For example, you can filter
* your results to return only UNTAGGED
images and then pipe that result to a BatchDeleteImage
* operation to delete them. Or, you can filter your results to return only TAGGED
images to list all
* of the tags in your repository.
*
*
* @param listImagesRequest
* @return A Java Future containing the result of the ListImages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListImages
* @see AWS API
* Documentation
*/
default CompletableFuture listImages(ListImagesRequest listImagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the image IDs for the specified repository.
*
*
* You can filter images based on whether or not they are tagged by using the tagStatus
filter and
* specifying either TAGGED
, UNTAGGED
or ANY
. For example, you can filter
* your results to return only UNTAGGED
images and then pipe that result to a BatchDeleteImage
* operation to delete them. Or, you can filter your results to return only TAGGED
images to list all
* of the tags in your repository.
*
*
*
* This is a convenience which creates an instance of the {@link ListImagesRequest.Builder} avoiding the need to
* create one manually via {@link ListImagesRequest#builder()}
*
*
* @param listImagesRequest
* A {@link Consumer} that will call methods on {@link ListImagesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListImages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListImages
* @see AWS API
* Documentation
*/
default CompletableFuture listImages(Consumer listImagesRequest) {
return listImages(ListImagesRequest.builder().applyMutation(listImagesRequest).build());
}
/**
*
* Lists all the image IDs for the specified repository.
*
*
* You can filter images based on whether or not they are tagged by using the tagStatus
filter and
* specifying either TAGGED
, UNTAGGED
or ANY
. For example, you can filter
* your results to return only UNTAGGED
images and then pipe that result to a BatchDeleteImage
* operation to delete them. Or, you can filter your results to return only TAGGED
images to list all
* of the tags in your repository.
*
*
*
* This is a variant of {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesPublisher publisher = client.listImagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesPublisher publisher = client.listImagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.ListImagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
*
*
* @param listImagesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListImages
* @see AWS API
* Documentation
*/
default ListImagesPublisher listImagesPaginator(ListImagesRequest listImagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the image IDs for the specified repository.
*
*
* You can filter images based on whether or not they are tagged by using the tagStatus
filter and
* specifying either TAGGED
, UNTAGGED
or ANY
. For example, you can filter
* your results to return only UNTAGGED
images and then pipe that result to a BatchDeleteImage
* operation to delete them. Or, you can filter your results to return only TAGGED
images to list all
* of the tags in your repository.
*
*
*
* This is a variant of {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesPublisher publisher = client.listImagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesPublisher publisher = client.listImagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.ListImagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListImagesRequest.Builder} avoiding the need to
* create one manually via {@link ListImagesRequest#builder()}
*
*
* @param listImagesRequest
* A {@link Consumer} that will call methods on {@link ListImagesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListImages
* @see AWS API
* Documentation
*/
default ListImagesPublisher listImagesPaginator(Consumer listImagesRequest) {
return listImagesPaginator(ListImagesRequest.builder().applyMutation(listImagesRequest).build());
}
/**
*
* List the tags for an Amazon ECR resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* List the tags for an Amazon ECR resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Creates or updates the image manifest and tags associated with an image.
*
*
* When an image is pushed and all new image layers have been uploaded, the PutImage API is called once to create or
* update the image manifest and the tags associated with the image.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param putImageRequest
* @return A Java Future containing the result of the PutImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageAlreadyExistsException The specified image has already been pushed, and there were no changes to
* the manifest or image tag after the last push.
* - LayersNotFoundException The specified layers could not be found, or the specified layer is not valid
* for this repository.
* - ReferencedImagesNotFoundException The manifest list is referencing an image that does not exist.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* - ImageTagAlreadyExistsException The specified image is tagged with a tag that already exists. The
* repository is configured for tag immutability.
* - ImageDigestDoesNotMatchException The specified image digest does not match the digest that Amazon ECR
* calculated for the image.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutImage
* @see AWS API
* Documentation
*/
default CompletableFuture putImage(PutImageRequest putImageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the image manifest and tags associated with an image.
*
*
* When an image is pushed and all new image layers have been uploaded, the PutImage API is called once to create or
* update the image manifest and the tags associated with the image.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link PutImageRequest.Builder} avoiding the need to
* create one manually via {@link PutImageRequest#builder()}
*
*
* @param putImageRequest
* A {@link Consumer} that will call methods on {@link PutImageRequest.Builder} to create a request.
* @return A Java Future containing the result of the PutImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageAlreadyExistsException The specified image has already been pushed, and there were no changes to
* the manifest or image tag after the last push.
* - LayersNotFoundException The specified layers could not be found, or the specified layer is not valid
* for this repository.
* - ReferencedImagesNotFoundException The manifest list is referencing an image that does not exist.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* - ImageTagAlreadyExistsException The specified image is tagged with a tag that already exists. The
* repository is configured for tag immutability.
* - ImageDigestDoesNotMatchException The specified image digest does not match the digest that Amazon ECR
* calculated for the image.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutImage
* @see AWS API
* Documentation
*/
default CompletableFuture putImage(Consumer putImageRequest) {
return putImage(PutImageRequest.builder().applyMutation(putImageRequest).build());
}
/**
*
* Updates the image scanning configuration for the specified repository.
*
*
* @param putImageScanningConfigurationRequest
* @return A Java Future containing the result of the PutImageScanningConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutImageScanningConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putImageScanningConfiguration(
PutImageScanningConfigurationRequest putImageScanningConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the image scanning configuration for the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link PutImageScanningConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link PutImageScanningConfigurationRequest#builder()}
*
*
* @param putImageScanningConfigurationRequest
* A {@link Consumer} that will call methods on {@link PutImageScanningConfigurationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the PutImageScanningConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutImageScanningConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putImageScanningConfiguration(
Consumer putImageScanningConfigurationRequest) {
return putImageScanningConfiguration(PutImageScanningConfigurationRequest.builder()
.applyMutation(putImageScanningConfigurationRequest).build());
}
/**
*
* Updates the image tag mutability settings for the specified repository. For more information, see Image tag mutability
* in the Amazon Elastic Container Registry User Guide.
*
*
* @param putImageTagMutabilityRequest
* @return A Java Future containing the result of the PutImageTagMutability operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutImageTagMutability
* @see AWS API
* Documentation
*/
default CompletableFuture putImageTagMutability(
PutImageTagMutabilityRequest putImageTagMutabilityRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the image tag mutability settings for the specified repository. For more information, see Image tag mutability
* in the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutImageTagMutabilityRequest.Builder} avoiding the
* need to create one manually via {@link PutImageTagMutabilityRequest#builder()}
*
*
* @param putImageTagMutabilityRequest
* A {@link Consumer} that will call methods on {@link PutImageTagMutabilityRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the PutImageTagMutability operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutImageTagMutability
* @see AWS API
* Documentation
*/
default CompletableFuture putImageTagMutability(
Consumer putImageTagMutabilityRequest) {
return putImageTagMutability(PutImageTagMutabilityRequest.builder().applyMutation(putImageTagMutabilityRequest).build());
}
/**
*
* Creates or updates the lifecycle policy for the specified repository. For more information, see Lifecycle policy
* template.
*
*
* @param putLifecyclePolicyRequest
* @return A Java Future containing the result of the PutLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture putLifecyclePolicy(PutLifecyclePolicyRequest putLifecyclePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the lifecycle policy for the specified repository. For more information, see Lifecycle policy
* template.
*
*
*
* This is a convenience which creates an instance of the {@link PutLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link PutLifecyclePolicyRequest#builder()}
*
*
* @param putLifecyclePolicyRequest
* A {@link Consumer} that will call methods on {@link PutLifecyclePolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the PutLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture putLifecyclePolicy(
Consumer putLifecyclePolicyRequest) {
return putLifecyclePolicy(PutLifecyclePolicyRequest.builder().applyMutation(putLifecyclePolicyRequest).build());
}
/**
*
* Creates or updates the permissions policy for your registry.
*
*
* A registry policy is used to specify permissions for another Amazon Web Services account and is used when
* configuring cross-account replication. For more information, see Registry permissions
* in the Amazon Elastic Container Registry User Guide.
*
*
* @param putRegistryPolicyRequest
* @return A Java Future containing the result of the PutRegistryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutRegistryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture putRegistryPolicy(PutRegistryPolicyRequest putRegistryPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the permissions policy for your registry.
*
*
* A registry policy is used to specify permissions for another Amazon Web Services account and is used when
* configuring cross-account replication. For more information, see Registry permissions
* in the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutRegistryPolicyRequest.Builder} avoiding the need
* to create one manually via {@link PutRegistryPolicyRequest#builder()}
*
*
* @param putRegistryPolicyRequest
* A {@link Consumer} that will call methods on {@link PutRegistryPolicyRequest.Builder} to create a request.
* @return A Java Future containing the result of the PutRegistryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutRegistryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture putRegistryPolicy(
Consumer putRegistryPolicyRequest) {
return putRegistryPolicy(PutRegistryPolicyRequest.builder().applyMutation(putRegistryPolicyRequest).build());
}
/**
*
* Creates or updates the scanning configuration for your private registry.
*
*
* @param putRegistryScanningConfigurationRequest
* @return A Java Future containing the result of the PutRegistryScanningConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutRegistryScanningConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putRegistryScanningConfiguration(
PutRegistryScanningConfigurationRequest putRegistryScanningConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the scanning configuration for your private registry.
*
*
*
* This is a convenience which creates an instance of the {@link PutRegistryScanningConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link PutRegistryScanningConfigurationRequest#builder()}
*
*
* @param putRegistryScanningConfigurationRequest
* A {@link Consumer} that will call methods on {@link PutRegistryScanningConfigurationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the PutRegistryScanningConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutRegistryScanningConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putRegistryScanningConfiguration(
Consumer putRegistryScanningConfigurationRequest) {
return putRegistryScanningConfiguration(PutRegistryScanningConfigurationRequest.builder()
.applyMutation(putRegistryScanningConfigurationRequest).build());
}
/**
*
* Creates or updates the replication configuration for a registry. The existing replication configuration for a
* repository can be retrieved with the DescribeRegistry API action. The first time the
* PutReplicationConfiguration API is called, a service-linked IAM role is created in your account for the
* replication process. For more information, see Using
* service-linked roles for Amazon ECR in the Amazon Elastic Container Registry User Guide.
*
*
*
* When configuring cross-account replication, the destination account must grant the source account permission to
* replicate. This permission is controlled using a registry permissions policy. For more information, see
* PutRegistryPolicy.
*
*
*
* @param putReplicationConfigurationRequest
* @return A Java Future containing the result of the PutReplicationConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutReplicationConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putReplicationConfiguration(
PutReplicationConfigurationRequest putReplicationConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the replication configuration for a registry. The existing replication configuration for a
* repository can be retrieved with the DescribeRegistry API action. The first time the
* PutReplicationConfiguration API is called, a service-linked IAM role is created in your account for the
* replication process. For more information, see Using
* service-linked roles for Amazon ECR in the Amazon Elastic Container Registry User Guide.
*
*
*
* When configuring cross-account replication, the destination account must grant the source account permission to
* replicate. This permission is controlled using a registry permissions policy. For more information, see
* PutRegistryPolicy.
*
*
*
* This is a convenience which creates an instance of the {@link PutReplicationConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link PutReplicationConfigurationRequest#builder()}
*
*
* @param putReplicationConfigurationRequest
* A {@link Consumer} that will call methods on {@link PutReplicationConfigurationRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the PutReplicationConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutReplicationConfiguration
* @see AWS API Documentation
*/
default CompletableFuture putReplicationConfiguration(
Consumer putReplicationConfigurationRequest) {
return putReplicationConfiguration(PutReplicationConfigurationRequest.builder()
.applyMutation(putReplicationConfigurationRequest).build());
}
/**
*
* Applies a repository policy to the specified repository to control access permissions. For more information, see
* Amazon ECR Repository
* policies in the Amazon Elastic Container Registry User Guide.
*
*
* @param setRepositoryPolicyRequest
* @return A Java Future containing the result of the SetRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.SetRepositoryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture setRepositoryPolicy(
SetRepositoryPolicyRequest setRepositoryPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Applies a repository policy to the specified repository to control access permissions. For more information, see
* Amazon ECR Repository
* policies in the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link SetRepositoryPolicyRequest.Builder} avoiding the
* need to create one manually via {@link SetRepositoryPolicyRequest#builder()}
*
*
* @param setRepositoryPolicyRequest
* A {@link Consumer} that will call methods on {@link SetRepositoryPolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the SetRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.SetRepositoryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture setRepositoryPolicy(
Consumer setRepositoryPolicyRequest) {
return setRepositoryPolicy(SetRepositoryPolicyRequest.builder().applyMutation(setRepositoryPolicyRequest).build());
}
/**
*
* Starts an image vulnerability scan. An image scan can only be started once per 24 hours on an individual image.
* This limit includes if an image was scanned on initial push. For more information, see Image scanning in the
* Amazon Elastic Container Registry User Guide.
*
*
* @param startImageScanRequest
* @return A Java Future containing the result of the StartImageScan operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - UnsupportedImageTypeException The image is of a type that cannot be scanned.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.StartImageScan
* @see AWS API
* Documentation
*/
default CompletableFuture startImageScan(StartImageScanRequest startImageScanRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts an image vulnerability scan. An image scan can only be started once per 24 hours on an individual image.
* This limit includes if an image was scanned on initial push. For more information, see Image scanning in the
* Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link StartImageScanRequest.Builder} avoiding the need to
* create one manually via {@link StartImageScanRequest#builder()}
*
*
* @param startImageScanRequest
* A {@link Consumer} that will call methods on {@link StartImageScanRequest.Builder} to create a request.
* @return A Java Future containing the result of the StartImageScan operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - UnsupportedImageTypeException The image is of a type that cannot be scanned.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - ValidationException There was an exception validating this request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.StartImageScan
* @see AWS API
* Documentation
*/
default CompletableFuture startImageScan(Consumer startImageScanRequest) {
return startImageScan(StartImageScanRequest.builder().applyMutation(startImageScanRequest).build());
}
/**
*
* Starts a preview of a lifecycle policy for the specified repository. This allows you to see the results before
* associating the lifecycle policy with the repository.
*
*
* @param startLifecyclePolicyPreviewRequest
* @return A Java Future containing the result of the StartLifecyclePolicyPreview operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - LifecyclePolicyPreviewInProgressException The previous lifecycle policy preview request has not
* completed. Wait and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.StartLifecyclePolicyPreview
* @see AWS API Documentation
*/
default CompletableFuture startLifecyclePolicyPreview(
StartLifecyclePolicyPreviewRequest startLifecyclePolicyPreviewRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts a preview of a lifecycle policy for the specified repository. This allows you to see the results before
* associating the lifecycle policy with the repository.
*
*
*
* This is a convenience which creates an instance of the {@link StartLifecyclePolicyPreviewRequest.Builder}
* avoiding the need to create one manually via {@link StartLifecyclePolicyPreviewRequest#builder()}
*
*
* @param startLifecyclePolicyPreviewRequest
* A {@link Consumer} that will call methods on {@link StartLifecyclePolicyPreviewRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the StartLifecyclePolicyPreview operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - LifecyclePolicyPreviewInProgressException The previous lifecycle policy preview request has not
* completed. Wait and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.StartLifecyclePolicyPreview
* @see AWS API Documentation
*/
default CompletableFuture startLifecyclePolicyPreview(
Consumer startLifecyclePolicyPreviewRequest) {
return startLifecyclePolicyPreview(StartLifecyclePolicyPreviewRequest.builder()
.applyMutation(startLifecyclePolicyPreviewRequest).build());
}
/**
*
* Adds specified tags to a resource with the specified ARN. Existing tags on a resource are not changed if they are
* not specified in the request parameters.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds specified tags to a resource with the specified ARN. Existing tags on a resource are not changed if they are
* not specified in the request parameters.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes specified tags from a resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Uploads an image layer part to Amazon ECR.
*
*
* When an image is pushed, each new image layer is uploaded in parts. The maximum size of each image layer part can
* be 20971520 bytes (or about 20MB). The UploadLayerPart API is called once per each new image layer part.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param uploadLayerPartRequest
* @return A Java Future containing the result of the UploadLayerPart operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidLayerPartException The layer part size is not valid, or the first byte specified is not
* consecutive to the last byte of a previous layer part upload.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - UploadNotFoundException The upload could not be found, or the specified upload ID is not valid for
* this repository.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.UploadLayerPart
* @see AWS API
* Documentation
*/
default CompletableFuture uploadLayerPart(UploadLayerPartRequest uploadLayerPartRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Uploads an image layer part to Amazon ECR.
*
*
* When an image is pushed, each new image layer is uploaded in parts. The maximum size of each image layer part can
* be 20971520 bytes (or about 20MB). The UploadLayerPart API is called once per each new image layer part.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link UploadLayerPartRequest.Builder} avoiding the need
* to create one manually via {@link UploadLayerPartRequest#builder()}
*
*
* @param uploadLayerPartRequest
* A {@link Consumer} that will call methods on {@link UploadLayerPartRequest.Builder} to create a request.
* @return A Java Future containing the result of the UploadLayerPart operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidLayerPartException The layer part size is not valid, or the first byte specified is not
* consecutive to the last byte of a previous layer part upload.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - UploadNotFoundException The upload could not be found, or the specified upload ID is not valid for
* this repository.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* - KmsException The operation failed due to a KMS exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.UploadLayerPart
* @see AWS API
* Documentation
*/
default CompletableFuture uploadLayerPart(
Consumer uploadLayerPartRequest) {
return uploadLayerPart(UploadLayerPartRequest.builder().applyMutation(uploadLayerPartRequest).build());
}
/**
* Create an instance of {@link EcrAsyncWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link EcrAsyncWaiter}
*/
default EcrAsyncWaiter waiter() {
throw new UnsupportedOperationException();
}
}