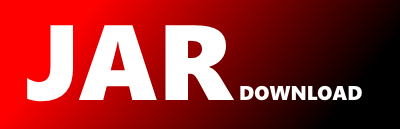
software.amazon.awssdk.services.ecr.model.CreateRepositoryRequest Maven / Gradle / Ivy
Show all versions of ecr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateRepositoryRequest extends EcrRequest implements
ToCopyableBuilder {
private static final SdkField REGISTRY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("registryId").getter(getter(CreateRepositoryRequest::registryId)).setter(setter(Builder::registryId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registryId").build()).build();
private static final SdkField REPOSITORY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("repositoryName").getter(getter(CreateRepositoryRequest::repositoryName))
.setter(setter(Builder::repositoryName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("repositoryName").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tags")
.getter(getter(CreateRepositoryRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField IMAGE_TAG_MUTABILITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("imageTagMutability").getter(getter(CreateRepositoryRequest::imageTagMutabilityAsString))
.setter(setter(Builder::imageTagMutability))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("imageTagMutability").build())
.build();
private static final SdkField IMAGE_SCANNING_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("imageScanningConfiguration")
.getter(getter(CreateRepositoryRequest::imageScanningConfiguration))
.setter(setter(Builder::imageScanningConfiguration))
.constructor(ImageScanningConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("imageScanningConfiguration").build())
.build();
private static final SdkField ENCRYPTION_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("encryptionConfiguration")
.getter(getter(CreateRepositoryRequest::encryptionConfiguration)).setter(setter(Builder::encryptionConfiguration))
.constructor(EncryptionConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryptionConfiguration").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REGISTRY_ID_FIELD,
REPOSITORY_NAME_FIELD, TAGS_FIELD, IMAGE_TAG_MUTABILITY_FIELD, IMAGE_SCANNING_CONFIGURATION_FIELD,
ENCRYPTION_CONFIGURATION_FIELD));
private final String registryId;
private final String repositoryName;
private final List tags;
private final String imageTagMutability;
private final ImageScanningConfiguration imageScanningConfiguration;
private final EncryptionConfiguration encryptionConfiguration;
private CreateRepositoryRequest(BuilderImpl builder) {
super(builder);
this.registryId = builder.registryId;
this.repositoryName = builder.repositoryName;
this.tags = builder.tags;
this.imageTagMutability = builder.imageTagMutability;
this.imageScanningConfiguration = builder.imageScanningConfiguration;
this.encryptionConfiguration = builder.encryptionConfiguration;
}
/**
*
* The Amazon Web Services account ID associated with the registry to create the repository. If you do not specify a
* registry, the default registry is assumed.
*
*
* @return The Amazon Web Services account ID associated with the registry to create the repository. If you do not
* specify a registry, the default registry is assumed.
*/
public final String registryId() {
return registryId;
}
/**
*
* The name to use for the repository. The repository name may be specified on its own (such as
* nginx-web-app
) or it can be prepended with a namespace to group the repository into a category (such
* as project-a/nginx-web-app
).
*
*
* @return The name to use for the repository. The repository name may be specified on its own (such as
* nginx-web-app
) or it can be prepended with a namespace to group the repository into a
* category (such as project-a/nginx-web-app
).
*/
public final String repositoryName() {
return repositoryName;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The metadata that you apply to the repository to help you categorize and organize them. Each tag consists of a
* key and an optional value, both of which you define. Tag keys can have a maximum character length of 128
* characters, and tag values can have a maximum length of 256 characters.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The metadata that you apply to the repository to help you categorize and organize them. Each tag consists
* of a key and an optional value, both of which you define. Tag keys can have a maximum character length of
* 128 characters, and tag values can have a maximum length of 256 characters.
*/
public final List tags() {
return tags;
}
/**
*
* The tag mutability setting for the repository. If this parameter is omitted, the default setting of
* MUTABLE
will be used which will allow image tags to be overwritten. If IMMUTABLE
is
* specified, all image tags within the repository will be immutable which will prevent them from being overwritten.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imageTagMutability} will return {@link ImageTagMutability#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #imageTagMutabilityAsString}.
*
*
* @return The tag mutability setting for the repository. If this parameter is omitted, the default setting of
* MUTABLE
will be used which will allow image tags to be overwritten. If
* IMMUTABLE
is specified, all image tags within the repository will be immutable which will
* prevent them from being overwritten.
* @see ImageTagMutability
*/
public final ImageTagMutability imageTagMutability() {
return ImageTagMutability.fromValue(imageTagMutability);
}
/**
*
* The tag mutability setting for the repository. If this parameter is omitted, the default setting of
* MUTABLE
will be used which will allow image tags to be overwritten. If IMMUTABLE
is
* specified, all image tags within the repository will be immutable which will prevent them from being overwritten.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imageTagMutability} will return {@link ImageTagMutability#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #imageTagMutabilityAsString}.
*
*
* @return The tag mutability setting for the repository. If this parameter is omitted, the default setting of
* MUTABLE
will be used which will allow image tags to be overwritten. If
* IMMUTABLE
is specified, all image tags within the repository will be immutable which will
* prevent them from being overwritten.
* @see ImageTagMutability
*/
public final String imageTagMutabilityAsString() {
return imageTagMutability;
}
/**
*
* The image scanning configuration for the repository. This determines whether images are scanned for known
* vulnerabilities after being pushed to the repository.
*
*
* @return The image scanning configuration for the repository. This determines whether images are scanned for known
* vulnerabilities after being pushed to the repository.
*/
public final ImageScanningConfiguration imageScanningConfiguration() {
return imageScanningConfiguration;
}
/**
*
* The encryption configuration for the repository. This determines how the contents of your repository are
* encrypted at rest.
*
*
* @return The encryption configuration for the repository. This determines how the contents of your repository are
* encrypted at rest.
*/
public final EncryptionConfiguration encryptionConfiguration() {
return encryptionConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(registryId());
hashCode = 31 * hashCode + Objects.hashCode(repositoryName());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(imageTagMutabilityAsString());
hashCode = 31 * hashCode + Objects.hashCode(imageScanningConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(encryptionConfiguration());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateRepositoryRequest)) {
return false;
}
CreateRepositoryRequest other = (CreateRepositoryRequest) obj;
return Objects.equals(registryId(), other.registryId()) && Objects.equals(repositoryName(), other.repositoryName())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(imageTagMutabilityAsString(), other.imageTagMutabilityAsString())
&& Objects.equals(imageScanningConfiguration(), other.imageScanningConfiguration())
&& Objects.equals(encryptionConfiguration(), other.encryptionConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateRepositoryRequest").add("RegistryId", registryId())
.add("RepositoryName", repositoryName()).add("Tags", hasTags() ? tags() : null)
.add("ImageTagMutability", imageTagMutabilityAsString())
.add("ImageScanningConfiguration", imageScanningConfiguration())
.add("EncryptionConfiguration", encryptionConfiguration()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "registryId":
return Optional.ofNullable(clazz.cast(registryId()));
case "repositoryName":
return Optional.ofNullable(clazz.cast(repositoryName()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
case "imageTagMutability":
return Optional.ofNullable(clazz.cast(imageTagMutabilityAsString()));
case "imageScanningConfiguration":
return Optional.ofNullable(clazz.cast(imageScanningConfiguration()));
case "encryptionConfiguration":
return Optional.ofNullable(clazz.cast(encryptionConfiguration()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function