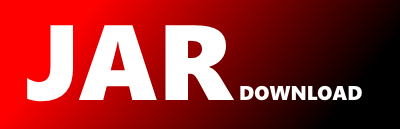
software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewResponse Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetLifecyclePolicyPreviewResponse extends EcrResponse implements
ToCopyableBuilder {
private static final SdkField REGISTRY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("registryId").getter(getter(GetLifecyclePolicyPreviewResponse::registryId))
.setter(setter(Builder::registryId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registryId").build()).build();
private static final SdkField REPOSITORY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("repositoryName").getter(getter(GetLifecyclePolicyPreviewResponse::repositoryName))
.setter(setter(Builder::repositoryName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("repositoryName").build()).build();
private static final SdkField LIFECYCLE_POLICY_TEXT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("lifecyclePolicyText").getter(getter(GetLifecyclePolicyPreviewResponse::lifecyclePolicyText))
.setter(setter(Builder::lifecyclePolicyText))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lifecyclePolicyText").build())
.build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(GetLifecyclePolicyPreviewResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("nextToken").getter(getter(GetLifecyclePolicyPreviewResponse::nextToken))
.setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("nextToken").build()).build();
private static final SdkField> PREVIEW_RESULTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("previewResults")
.getter(getter(GetLifecyclePolicyPreviewResponse::previewResults))
.setter(setter(Builder::previewResults))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("previewResults").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LifecyclePolicyPreviewResult::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SUMMARY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("summary")
.getter(getter(GetLifecyclePolicyPreviewResponse::summary)).setter(setter(Builder::summary))
.constructor(LifecyclePolicyPreviewSummary::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("summary").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REGISTRY_ID_FIELD,
REPOSITORY_NAME_FIELD, LIFECYCLE_POLICY_TEXT_FIELD, STATUS_FIELD, NEXT_TOKEN_FIELD, PREVIEW_RESULTS_FIELD,
SUMMARY_FIELD));
private final String registryId;
private final String repositoryName;
private final String lifecyclePolicyText;
private final String status;
private final String nextToken;
private final List previewResults;
private final LifecyclePolicyPreviewSummary summary;
private GetLifecyclePolicyPreviewResponse(BuilderImpl builder) {
super(builder);
this.registryId = builder.registryId;
this.repositoryName = builder.repositoryName;
this.lifecyclePolicyText = builder.lifecyclePolicyText;
this.status = builder.status;
this.nextToken = builder.nextToken;
this.previewResults = builder.previewResults;
this.summary = builder.summary;
}
/**
*
* The registry ID associated with the request.
*
*
* @return The registry ID associated with the request.
*/
public final String registryId() {
return registryId;
}
/**
*
* The repository name associated with the request.
*
*
* @return The repository name associated with the request.
*/
public final String repositoryName() {
return repositoryName;
}
/**
*
* The JSON lifecycle policy text.
*
*
* @return The JSON lifecycle policy text.
*/
public final String lifecyclePolicyText() {
return lifecyclePolicyText;
}
/**
*
* The status of the lifecycle policy preview request.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link LifecyclePolicyPreviewStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #statusAsString}.
*
*
* @return The status of the lifecycle policy preview request.
* @see LifecyclePolicyPreviewStatus
*/
public final LifecyclePolicyPreviewStatus status() {
return LifecyclePolicyPreviewStatus.fromValue(status);
}
/**
*
* The status of the lifecycle policy preview request.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link LifecyclePolicyPreviewStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #statusAsString}.
*
*
* @return The status of the lifecycle policy preview request.
* @see LifecyclePolicyPreviewStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The nextToken
value to include in a future GetLifecyclePolicyPreview
request. When the
* results of a GetLifecyclePolicyPreview
request exceed maxResults
, this value can be
* used to retrieve the next page of results. This value is null
when there are no more results to
* return.
*
*
* @return The nextToken
value to include in a future GetLifecyclePolicyPreview
request.
* When the results of a GetLifecyclePolicyPreview
request exceed maxResults
, this
* value can be used to retrieve the next page of results. This value is null
when there are no
* more results to return.
*/
public final String nextToken() {
return nextToken;
}
/**
* For responses, this returns true if the service returned a value for the PreviewResults property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPreviewResults() {
return previewResults != null && !(previewResults instanceof SdkAutoConstructList);
}
/**
*
* The results of the lifecycle policy preview request.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPreviewResults} method.
*
*
* @return The results of the lifecycle policy preview request.
*/
public final List previewResults() {
return previewResults;
}
/**
*
* The list of images that is returned as a result of the action.
*
*
* @return The list of images that is returned as a result of the action.
*/
public final LifecyclePolicyPreviewSummary summary() {
return summary;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(registryId());
hashCode = 31 * hashCode + Objects.hashCode(repositoryName());
hashCode = 31 * hashCode + Objects.hashCode(lifecyclePolicyText());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
hashCode = 31 * hashCode + Objects.hashCode(hasPreviewResults() ? previewResults() : null);
hashCode = 31 * hashCode + Objects.hashCode(summary());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetLifecyclePolicyPreviewResponse)) {
return false;
}
GetLifecyclePolicyPreviewResponse other = (GetLifecyclePolicyPreviewResponse) obj;
return Objects.equals(registryId(), other.registryId()) && Objects.equals(repositoryName(), other.repositoryName())
&& Objects.equals(lifecyclePolicyText(), other.lifecyclePolicyText())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(nextToken(), other.nextToken())
&& hasPreviewResults() == other.hasPreviewResults() && Objects.equals(previewResults(), other.previewResults())
&& Objects.equals(summary(), other.summary());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetLifecyclePolicyPreviewResponse").add("RegistryId", registryId())
.add("RepositoryName", repositoryName()).add("LifecyclePolicyText", lifecyclePolicyText())
.add("Status", statusAsString()).add("NextToken", nextToken())
.add("PreviewResults", hasPreviewResults() ? previewResults() : null).add("Summary", summary()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "registryId":
return Optional.ofNullable(clazz.cast(registryId()));
case "repositoryName":
return Optional.ofNullable(clazz.cast(repositoryName()));
case "lifecyclePolicyText":
return Optional.ofNullable(clazz.cast(lifecyclePolicyText()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "nextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
case "previewResults":
return Optional.ofNullable(clazz.cast(previewResults()));
case "summary":
return Optional.ofNullable(clazz.cast(summary()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function