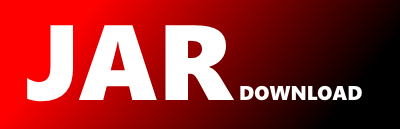
software.amazon.awssdk.services.ecr.model.PackageVulnerabilityDetails Maven / Gradle / Ivy
Show all versions of ecr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a package vulnerability finding.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PackageVulnerabilityDetails implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> CVSS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("cvss")
.getter(getter(PackageVulnerabilityDetails::cvss))
.setter(setter(Builder::cvss))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cvss").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CvssScore::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> REFERENCE_URLS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("referenceUrls")
.getter(getter(PackageVulnerabilityDetails::referenceUrls))
.setter(setter(Builder::referenceUrls))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("referenceUrls").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> RELATED_VULNERABILITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("relatedVulnerabilities")
.getter(getter(PackageVulnerabilityDetails::relatedVulnerabilities))
.setter(setter(Builder::relatedVulnerabilities))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("relatedVulnerabilities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("source")
.getter(getter(PackageVulnerabilityDetails::source)).setter(setter(Builder::source))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("source").build()).build();
private static final SdkField SOURCE_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sourceUrl").getter(getter(PackageVulnerabilityDetails::sourceUrl)).setter(setter(Builder::sourceUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceUrl").build()).build();
private static final SdkField VENDOR_CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("vendorCreatedAt").getter(getter(PackageVulnerabilityDetails::vendorCreatedAt))
.setter(setter(Builder::vendorCreatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vendorCreatedAt").build()).build();
private static final SdkField VENDOR_SEVERITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("vendorSeverity").getter(getter(PackageVulnerabilityDetails::vendorSeverity))
.setter(setter(Builder::vendorSeverity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vendorSeverity").build()).build();
private static final SdkField VENDOR_UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("vendorUpdatedAt").getter(getter(PackageVulnerabilityDetails::vendorUpdatedAt))
.setter(setter(Builder::vendorUpdatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vendorUpdatedAt").build()).build();
private static final SdkField VULNERABILITY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("vulnerabilityId").getter(getter(PackageVulnerabilityDetails::vulnerabilityId))
.setter(setter(Builder::vulnerabilityId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vulnerabilityId").build()).build();
private static final SdkField> VULNERABLE_PACKAGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("vulnerablePackages")
.getter(getter(PackageVulnerabilityDetails::vulnerablePackages))
.setter(setter(Builder::vulnerablePackages))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vulnerablePackages").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(VulnerablePackage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CVSS_FIELD,
REFERENCE_URLS_FIELD, RELATED_VULNERABILITIES_FIELD, SOURCE_FIELD, SOURCE_URL_FIELD, VENDOR_CREATED_AT_FIELD,
VENDOR_SEVERITY_FIELD, VENDOR_UPDATED_AT_FIELD, VULNERABILITY_ID_FIELD, VULNERABLE_PACKAGES_FIELD));
private static final long serialVersionUID = 1L;
private final List cvss;
private final List referenceUrls;
private final List relatedVulnerabilities;
private final String source;
private final String sourceUrl;
private final Instant vendorCreatedAt;
private final String vendorSeverity;
private final Instant vendorUpdatedAt;
private final String vulnerabilityId;
private final List vulnerablePackages;
private PackageVulnerabilityDetails(BuilderImpl builder) {
this.cvss = builder.cvss;
this.referenceUrls = builder.referenceUrls;
this.relatedVulnerabilities = builder.relatedVulnerabilities;
this.source = builder.source;
this.sourceUrl = builder.sourceUrl;
this.vendorCreatedAt = builder.vendorCreatedAt;
this.vendorSeverity = builder.vendorSeverity;
this.vendorUpdatedAt = builder.vendorUpdatedAt;
this.vulnerabilityId = builder.vulnerabilityId;
this.vulnerablePackages = builder.vulnerablePackages;
}
/**
* For responses, this returns true if the service returned a value for the Cvss property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasCvss() {
return cvss != null && !(cvss instanceof SdkAutoConstructList);
}
/**
*
* An object that contains details about the CVSS score of a finding.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCvss} method.
*
*
* @return An object that contains details about the CVSS score of a finding.
*/
public final List cvss() {
return cvss;
}
/**
* For responses, this returns true if the service returned a value for the ReferenceUrls property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasReferenceUrls() {
return referenceUrls != null && !(referenceUrls instanceof SdkAutoConstructList);
}
/**
*
* One or more URLs that contain details about this vulnerability type.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasReferenceUrls} method.
*
*
* @return One or more URLs that contain details about this vulnerability type.
*/
public final List referenceUrls() {
return referenceUrls;
}
/**
* For responses, this returns true if the service returned a value for the RelatedVulnerabilities property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasRelatedVulnerabilities() {
return relatedVulnerabilities != null && !(relatedVulnerabilities instanceof SdkAutoConstructList);
}
/**
*
* One or more vulnerabilities related to the one identified in this finding.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRelatedVulnerabilities} method.
*
*
* @return One or more vulnerabilities related to the one identified in this finding.
*/
public final List relatedVulnerabilities() {
return relatedVulnerabilities;
}
/**
*
* The source of the vulnerability information.
*
*
* @return The source of the vulnerability information.
*/
public final String source() {
return source;
}
/**
*
* A URL to the source of the vulnerability information.
*
*
* @return A URL to the source of the vulnerability information.
*/
public final String sourceUrl() {
return sourceUrl;
}
/**
*
* The date and time that this vulnerability was first added to the vendor's database.
*
*
* @return The date and time that this vulnerability was first added to the vendor's database.
*/
public final Instant vendorCreatedAt() {
return vendorCreatedAt;
}
/**
*
* The severity the vendor has given to this vulnerability type.
*
*
* @return The severity the vendor has given to this vulnerability type.
*/
public final String vendorSeverity() {
return vendorSeverity;
}
/**
*
* The date and time the vendor last updated this vulnerability in their database.
*
*
* @return The date and time the vendor last updated this vulnerability in their database.
*/
public final Instant vendorUpdatedAt() {
return vendorUpdatedAt;
}
/**
*
* The ID given to this vulnerability.
*
*
* @return The ID given to this vulnerability.
*/
public final String vulnerabilityId() {
return vulnerabilityId;
}
/**
* For responses, this returns true if the service returned a value for the VulnerablePackages property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasVulnerablePackages() {
return vulnerablePackages != null && !(vulnerablePackages instanceof SdkAutoConstructList);
}
/**
*
* The packages impacted by this vulnerability.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVulnerablePackages} method.
*
*
* @return The packages impacted by this vulnerability.
*/
public final List vulnerablePackages() {
return vulnerablePackages;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasCvss() ? cvss() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasReferenceUrls() ? referenceUrls() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasRelatedVulnerabilities() ? relatedVulnerabilities() : null);
hashCode = 31 * hashCode + Objects.hashCode(source());
hashCode = 31 * hashCode + Objects.hashCode(sourceUrl());
hashCode = 31 * hashCode + Objects.hashCode(vendorCreatedAt());
hashCode = 31 * hashCode + Objects.hashCode(vendorSeverity());
hashCode = 31 * hashCode + Objects.hashCode(vendorUpdatedAt());
hashCode = 31 * hashCode + Objects.hashCode(vulnerabilityId());
hashCode = 31 * hashCode + Objects.hashCode(hasVulnerablePackages() ? vulnerablePackages() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PackageVulnerabilityDetails)) {
return false;
}
PackageVulnerabilityDetails other = (PackageVulnerabilityDetails) obj;
return hasCvss() == other.hasCvss() && Objects.equals(cvss(), other.cvss())
&& hasReferenceUrls() == other.hasReferenceUrls() && Objects.equals(referenceUrls(), other.referenceUrls())
&& hasRelatedVulnerabilities() == other.hasRelatedVulnerabilities()
&& Objects.equals(relatedVulnerabilities(), other.relatedVulnerabilities())
&& Objects.equals(source(), other.source()) && Objects.equals(sourceUrl(), other.sourceUrl())
&& Objects.equals(vendorCreatedAt(), other.vendorCreatedAt())
&& Objects.equals(vendorSeverity(), other.vendorSeverity())
&& Objects.equals(vendorUpdatedAt(), other.vendorUpdatedAt())
&& Objects.equals(vulnerabilityId(), other.vulnerabilityId())
&& hasVulnerablePackages() == other.hasVulnerablePackages()
&& Objects.equals(vulnerablePackages(), other.vulnerablePackages());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PackageVulnerabilityDetails").add("Cvss", hasCvss() ? cvss() : null)
.add("ReferenceUrls", hasReferenceUrls() ? referenceUrls() : null)
.add("RelatedVulnerabilities", hasRelatedVulnerabilities() ? relatedVulnerabilities() : null)
.add("Source", source()).add("SourceUrl", sourceUrl()).add("VendorCreatedAt", vendorCreatedAt())
.add("VendorSeverity", vendorSeverity()).add("VendorUpdatedAt", vendorUpdatedAt())
.add("VulnerabilityId", vulnerabilityId())
.add("VulnerablePackages", hasVulnerablePackages() ? vulnerablePackages() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cvss":
return Optional.ofNullable(clazz.cast(cvss()));
case "referenceUrls":
return Optional.ofNullable(clazz.cast(referenceUrls()));
case "relatedVulnerabilities":
return Optional.ofNullable(clazz.cast(relatedVulnerabilities()));
case "source":
return Optional.ofNullable(clazz.cast(source()));
case "sourceUrl":
return Optional.ofNullable(clazz.cast(sourceUrl()));
case "vendorCreatedAt":
return Optional.ofNullable(clazz.cast(vendorCreatedAt()));
case "vendorSeverity":
return Optional.ofNullable(clazz.cast(vendorSeverity()));
case "vendorUpdatedAt":
return Optional.ofNullable(clazz.cast(vendorUpdatedAt()));
case "vulnerabilityId":
return Optional.ofNullable(clazz.cast(vulnerabilityId()));
case "vulnerablePackages":
return Optional.ofNullable(clazz.cast(vulnerablePackages()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function