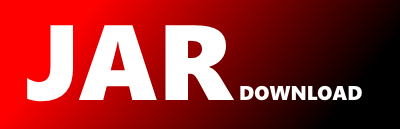
software.amazon.awssdk.services.ecr.model.InitiateLayerUploadResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecr Show documentation
Show all versions of ecr Show documentation
The AWS Java SDK for the Amazon EC2 Container Registry holds the client classes that are used for
communicating with the
Amazon EC2 Container Registry Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class InitiateLayerUploadResponse extends EcrResponse implements
ToCopyableBuilder {
private static final SdkField UPLOAD_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("uploadId").getter(getter(InitiateLayerUploadResponse::uploadId)).setter(setter(Builder::uploadId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("uploadId").build()).build();
private static final SdkField PART_SIZE_FIELD = SdkField. builder(MarshallingType.LONG).memberName("partSize")
.getter(getter(InitiateLayerUploadResponse::partSize)).setter(setter(Builder::partSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("partSize").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(UPLOAD_ID_FIELD,
PART_SIZE_FIELD));
private final String uploadId;
private final Long partSize;
private InitiateLayerUploadResponse(BuilderImpl builder) {
super(builder);
this.uploadId = builder.uploadId;
this.partSize = builder.partSize;
}
/**
*
* The upload ID for the layer upload. This parameter is passed to further UploadLayerPart and
* CompleteLayerUpload operations.
*
*
* @return The upload ID for the layer upload. This parameter is passed to further UploadLayerPart and
* CompleteLayerUpload operations.
*/
public final String uploadId() {
return uploadId;
}
/**
*
* The size, in bytes, that Amazon ECR expects future layer part uploads to be.
*
*
* @return The size, in bytes, that Amazon ECR expects future layer part uploads to be.
*/
public final Long partSize() {
return partSize;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(uploadId());
hashCode = 31 * hashCode + Objects.hashCode(partSize());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof InitiateLayerUploadResponse)) {
return false;
}
InitiateLayerUploadResponse other = (InitiateLayerUploadResponse) obj;
return Objects.equals(uploadId(), other.uploadId()) && Objects.equals(partSize(), other.partSize());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("InitiateLayerUploadResponse").add("UploadId", uploadId()).add("PartSize", partSize()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "uploadId":
return Optional.ofNullable(clazz.cast(uploadId()));
case "partSize":
return Optional.ofNullable(clazz.cast(partSize()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy