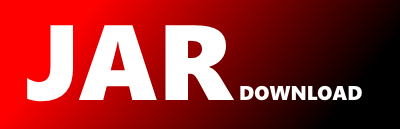
software.amazon.awssdk.services.ecr.model.ImageReplicationStatus Maven / Gradle / Ivy
Show all versions of ecr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The status of the replication process for an image.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ImageReplicationStatus implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField REGION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("region")
.getter(getter(ImageReplicationStatus::region)).setter(setter(Builder::region))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("region").build()).build();
private static final SdkField REGISTRY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("registryId").getter(getter(ImageReplicationStatus::registryId)).setter(setter(Builder::registryId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registryId").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(ImageReplicationStatus::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField FAILURE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("failureCode").getter(getter(ImageReplicationStatus::failureCode)).setter(setter(Builder::failureCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("failureCode").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REGION_FIELD,
REGISTRY_ID_FIELD, STATUS_FIELD, FAILURE_CODE_FIELD));
private static final long serialVersionUID = 1L;
private final String region;
private final String registryId;
private final String status;
private final String failureCode;
private ImageReplicationStatus(BuilderImpl builder) {
this.region = builder.region;
this.registryId = builder.registryId;
this.status = builder.status;
this.failureCode = builder.failureCode;
}
/**
*
* The destination Region for the image replication.
*
*
* @return The destination Region for the image replication.
*/
public final String region() {
return region;
}
/**
*
* The Amazon Web Services account ID associated with the registry to which the image belongs.
*
*
* @return The Amazon Web Services account ID associated with the registry to which the image belongs.
*/
public final String registryId() {
return registryId;
}
/**
*
* The image replication status.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ReplicationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The image replication status.
* @see ReplicationStatus
*/
public final ReplicationStatus status() {
return ReplicationStatus.fromValue(status);
}
/**
*
* The image replication status.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ReplicationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The image replication status.
* @see ReplicationStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The failure code for a replication that has failed.
*
*
* @return The failure code for a replication that has failed.
*/
public final String failureCode() {
return failureCode;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(region());
hashCode = 31 * hashCode + Objects.hashCode(registryId());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(failureCode());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ImageReplicationStatus)) {
return false;
}
ImageReplicationStatus other = (ImageReplicationStatus) obj;
return Objects.equals(region(), other.region()) && Objects.equals(registryId(), other.registryId())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(failureCode(), other.failureCode());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ImageReplicationStatus").add("Region", region()).add("RegistryId", registryId())
.add("Status", statusAsString()).add("FailureCode", failureCode()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "region":
return Optional.ofNullable(clazz.cast(region()));
case "registryId":
return Optional.ofNullable(clazz.cast(registryId()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "failureCode":
return Optional.ofNullable(clazz.cast(failureCode()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function