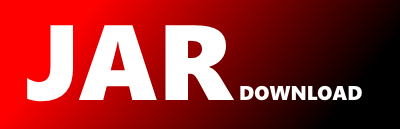
software.amazon.awssdk.services.ecr.model.RepositoryCreationTemplate Maven / Gradle / Ivy
Show all versions of ecr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details of the repository creation template associated with the request.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RepositoryCreationTemplate implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField PREFIX_FIELD = SdkField. builder(MarshallingType.STRING).memberName("prefix")
.getter(getter(RepositoryCreationTemplate::prefix)).setter(setter(Builder::prefix))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("prefix").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("description").getter(getter(RepositoryCreationTemplate::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField ENCRYPTION_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("encryptionConfiguration").getter(getter(RepositoryCreationTemplate::encryptionConfiguration))
.setter(setter(Builder::encryptionConfiguration))
.constructor(EncryptionConfigurationForRepositoryCreationTemplate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encryptionConfiguration").build())
.build();
private static final SdkField> RESOURCE_TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("resourceTags")
.getter(getter(RepositoryCreationTemplate::resourceTags))
.setter(setter(Builder::resourceTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceTags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField IMAGE_TAG_MUTABILITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("imageTagMutability").getter(getter(RepositoryCreationTemplate::imageTagMutabilityAsString))
.setter(setter(Builder::imageTagMutability))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("imageTagMutability").build())
.build();
private static final SdkField REPOSITORY_POLICY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("repositoryPolicy").getter(getter(RepositoryCreationTemplate::repositoryPolicy))
.setter(setter(Builder::repositoryPolicy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("repositoryPolicy").build()).build();
private static final SdkField LIFECYCLE_POLICY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("lifecyclePolicy").getter(getter(RepositoryCreationTemplate::lifecyclePolicy))
.setter(setter(Builder::lifecyclePolicy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lifecyclePolicy").build()).build();
private static final SdkField> APPLIED_FOR_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("appliedFor")
.getter(getter(RepositoryCreationTemplate::appliedForAsStrings))
.setter(setter(Builder::appliedForWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("appliedFor").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CUSTOM_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("customRoleArn").getter(getter(RepositoryCreationTemplate::customRoleArn))
.setter(setter(Builder::customRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("customRoleArn").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("createdAt").getter(getter(RepositoryCreationTemplate::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdAt").build()).build();
private static final SdkField UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("updatedAt").getter(getter(RepositoryCreationTemplate::updatedAt)).setter(setter(Builder::updatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updatedAt").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PREFIX_FIELD,
DESCRIPTION_FIELD, ENCRYPTION_CONFIGURATION_FIELD, RESOURCE_TAGS_FIELD, IMAGE_TAG_MUTABILITY_FIELD,
REPOSITORY_POLICY_FIELD, LIFECYCLE_POLICY_FIELD, APPLIED_FOR_FIELD, CUSTOM_ROLE_ARN_FIELD, CREATED_AT_FIELD,
UPDATED_AT_FIELD));
private static final long serialVersionUID = 1L;
private final String prefix;
private final String description;
private final EncryptionConfigurationForRepositoryCreationTemplate encryptionConfiguration;
private final List resourceTags;
private final String imageTagMutability;
private final String repositoryPolicy;
private final String lifecyclePolicy;
private final List appliedFor;
private final String customRoleArn;
private final Instant createdAt;
private final Instant updatedAt;
private RepositoryCreationTemplate(BuilderImpl builder) {
this.prefix = builder.prefix;
this.description = builder.description;
this.encryptionConfiguration = builder.encryptionConfiguration;
this.resourceTags = builder.resourceTags;
this.imageTagMutability = builder.imageTagMutability;
this.repositoryPolicy = builder.repositoryPolicy;
this.lifecyclePolicy = builder.lifecyclePolicy;
this.appliedFor = builder.appliedFor;
this.customRoleArn = builder.customRoleArn;
this.createdAt = builder.createdAt;
this.updatedAt = builder.updatedAt;
}
/**
*
* The repository namespace prefix associated with the repository creation template.
*
*
* @return The repository namespace prefix associated with the repository creation template.
*/
public final String prefix() {
return prefix;
}
/**
*
* The description associated with the repository creation template.
*
*
* @return The description associated with the repository creation template.
*/
public final String description() {
return description;
}
/**
*
* The encryption configuration associated with the repository creation template.
*
*
* @return The encryption configuration associated with the repository creation template.
*/
public final EncryptionConfigurationForRepositoryCreationTemplate encryptionConfiguration() {
return encryptionConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the ResourceTags property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasResourceTags() {
return resourceTags != null && !(resourceTags instanceof SdkAutoConstructList);
}
/**
*
* The metadata to apply to the repository to help you categorize and organize. Each tag consists of a key and an
* optional value, both of which you define. Tag keys can have a maximum character length of 128 characters, and tag
* values can have a maximum length of 256 characters.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasResourceTags} method.
*
*
* @return The metadata to apply to the repository to help you categorize and organize. Each tag consists of a key
* and an optional value, both of which you define. Tag keys can have a maximum character length of 128
* characters, and tag values can have a maximum length of 256 characters.
*/
public final List resourceTags() {
return resourceTags;
}
/**
*
* The tag mutability setting for the repository. If this parameter is omitted, the default setting of MUTABLE will
* be used which will allow image tags to be overwritten. If IMMUTABLE is specified, all image tags within the
* repository will be immutable which will prevent them from being overwritten.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imageTagMutability} will return {@link ImageTagMutability#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #imageTagMutabilityAsString}.
*
*
* @return The tag mutability setting for the repository. If this parameter is omitted, the default setting of
* MUTABLE will be used which will allow image tags to be overwritten. If IMMUTABLE is specified, all image
* tags within the repository will be immutable which will prevent them from being overwritten.
* @see ImageTagMutability
*/
public final ImageTagMutability imageTagMutability() {
return ImageTagMutability.fromValue(imageTagMutability);
}
/**
*
* The tag mutability setting for the repository. If this parameter is omitted, the default setting of MUTABLE will
* be used which will allow image tags to be overwritten. If IMMUTABLE is specified, all image tags within the
* repository will be immutable which will prevent them from being overwritten.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #imageTagMutability} will return {@link ImageTagMutability#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #imageTagMutabilityAsString}.
*
*
* @return The tag mutability setting for the repository. If this parameter is omitted, the default setting of
* MUTABLE will be used which will allow image tags to be overwritten. If IMMUTABLE is specified, all image
* tags within the repository will be immutable which will prevent them from being overwritten.
* @see ImageTagMutability
*/
public final String imageTagMutabilityAsString() {
return imageTagMutability;
}
/**
*
* he repository policy to apply to repositories created using the template. A repository policy is a permissions
* policy associated with a repository to control access permissions.
*
*
* @return he repository policy to apply to repositories created using the template. A repository policy is a
* permissions policy associated with a repository to control access permissions.
*/
public final String repositoryPolicy() {
return repositoryPolicy;
}
/**
*
* The lifecycle policy to use for repositories created using the template.
*
*
* @return The lifecycle policy to use for repositories created using the template.
*/
public final String lifecyclePolicy() {
return lifecyclePolicy;
}
/**
*
* A list of enumerable Strings representing the repository creation scenarios that this template will apply
* towards. The two supported scenarios are PULL_THROUGH_CACHE and REPLICATION
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAppliedFor} method.
*
*
* @return A list of enumerable Strings representing the repository creation scenarios that this template will apply
* towards. The two supported scenarios are PULL_THROUGH_CACHE and REPLICATION
*/
public final List appliedFor() {
return RCTAppliedForListCopier.copyStringToEnum(appliedFor);
}
/**
* For responses, this returns true if the service returned a value for the AppliedFor property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAppliedFor() {
return appliedFor != null && !(appliedFor instanceof SdkAutoConstructList);
}
/**
*
* A list of enumerable Strings representing the repository creation scenarios that this template will apply
* towards. The two supported scenarios are PULL_THROUGH_CACHE and REPLICATION
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAppliedFor} method.
*
*
* @return A list of enumerable Strings representing the repository creation scenarios that this template will apply
* towards. The two supported scenarios are PULL_THROUGH_CACHE and REPLICATION
*/
public final List appliedForAsStrings() {
return appliedFor;
}
/**
*
* The ARN of the role to be assumed by Amazon ECR.
*
*
* @return The ARN of the role to be assumed by Amazon ECR.
*/
public final String customRoleArn() {
return customRoleArn;
}
/**
*
* The date and time, in JavaScript date format, when the repository creation template was created.
*
*
* @return The date and time, in JavaScript date format, when the repository creation template was created.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The date and time, in JavaScript date format, when the repository creation template was last updated.
*
*
* @return The date and time, in JavaScript date format, when the repository creation template was last updated.
*/
public final Instant updatedAt() {
return updatedAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(prefix());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(encryptionConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasResourceTags() ? resourceTags() : null);
hashCode = 31 * hashCode + Objects.hashCode(imageTagMutabilityAsString());
hashCode = 31 * hashCode + Objects.hashCode(repositoryPolicy());
hashCode = 31 * hashCode + Objects.hashCode(lifecyclePolicy());
hashCode = 31 * hashCode + Objects.hashCode(hasAppliedFor() ? appliedForAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(customRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(updatedAt());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RepositoryCreationTemplate)) {
return false;
}
RepositoryCreationTemplate other = (RepositoryCreationTemplate) obj;
return Objects.equals(prefix(), other.prefix()) && Objects.equals(description(), other.description())
&& Objects.equals(encryptionConfiguration(), other.encryptionConfiguration())
&& hasResourceTags() == other.hasResourceTags() && Objects.equals(resourceTags(), other.resourceTags())
&& Objects.equals(imageTagMutabilityAsString(), other.imageTagMutabilityAsString())
&& Objects.equals(repositoryPolicy(), other.repositoryPolicy())
&& Objects.equals(lifecyclePolicy(), other.lifecyclePolicy()) && hasAppliedFor() == other.hasAppliedFor()
&& Objects.equals(appliedForAsStrings(), other.appliedForAsStrings())
&& Objects.equals(customRoleArn(), other.customRoleArn()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(updatedAt(), other.updatedAt());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RepositoryCreationTemplate").add("Prefix", prefix()).add("Description", description())
.add("EncryptionConfiguration", encryptionConfiguration())
.add("ResourceTags", hasResourceTags() ? resourceTags() : null)
.add("ImageTagMutability", imageTagMutabilityAsString()).add("RepositoryPolicy", repositoryPolicy())
.add("LifecyclePolicy", lifecyclePolicy()).add("AppliedFor", hasAppliedFor() ? appliedForAsStrings() : null)
.add("CustomRoleArn", customRoleArn()).add("CreatedAt", createdAt()).add("UpdatedAt", updatedAt()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "prefix":
return Optional.ofNullable(clazz.cast(prefix()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "encryptionConfiguration":
return Optional.ofNullable(clazz.cast(encryptionConfiguration()));
case "resourceTags":
return Optional.ofNullable(clazz.cast(resourceTags()));
case "imageTagMutability":
return Optional.ofNullable(clazz.cast(imageTagMutabilityAsString()));
case "repositoryPolicy":
return Optional.ofNullable(clazz.cast(repositoryPolicy()));
case "lifecyclePolicy":
return Optional.ofNullable(clazz.cast(lifecyclePolicy()));
case "appliedFor":
return Optional.ofNullable(clazz.cast(appliedForAsStrings()));
case "customRoleArn":
return Optional.ofNullable(clazz.cast(customRoleArn()));
case "createdAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "updatedAt":
return Optional.ofNullable(clazz.cast(updatedAt()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function