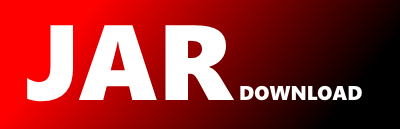
software.amazon.awssdk.services.ecr.waiters.EcrAsyncWaiter Maven / Gradle / Ivy
Show all versions of ecr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.waiters;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.Immutable;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.waiters.WaiterOverrideConfiguration;
import software.amazon.awssdk.core.waiters.WaiterResponse;
import software.amazon.awssdk.services.ecr.EcrAsyncClient;
import software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest;
import software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsResponse;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewResponse;
import software.amazon.awssdk.utils.SdkAutoCloseable;
/**
* Waiter utility class that polls a resource until a desired state is reached or until it is determined that the
* resource will never enter into the desired state. This can be created using the static {@link #builder()} method
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
@Immutable
public interface EcrAsyncWaiter extends SdkAutoCloseable {
/**
* Polls {@link EcrAsyncClient#describeImageScanFindings} API until the desired condition {@code ImageScanComplete}
* is met, or until it is determined that the resource will never enter into the desired state
*
* @param describeImageScanFindingsRequest
* the request to be used for polling
* @return CompletableFuture containing the WaiterResponse. It completes successfully when the resource enters into
* a desired state or exceptionally when it is determined that the resource will never enter into the
* desired state.
*/
default CompletableFuture> waitUntilImageScanComplete(
DescribeImageScanFindingsRequest describeImageScanFindingsRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link EcrAsyncClient#describeImageScanFindings} API until the desired condition {@code ImageScanComplete}
* is met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link DescribeImageScanFindingsRequest#builder()}
*
* @param describeImageScanFindingsRequest
* The consumer that will configure the request to be used for polling
* @return CompletableFuture of the WaiterResponse containing either a response or an exception that has matched
* with the waiter success condition
*/
default CompletableFuture> waitUntilImageScanComplete(
Consumer describeImageScanFindingsRequest) {
return waitUntilImageScanComplete(DescribeImageScanFindingsRequest.builder()
.applyMutation(describeImageScanFindingsRequest).build());
}
/**
* Polls {@link EcrAsyncClient#describeImageScanFindings} API until the desired condition {@code ImageScanComplete}
* is met, or until it is determined that the resource will never enter into the desired state
*
* @param describeImageScanFindingsRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default CompletableFuture> waitUntilImageScanComplete(
DescribeImageScanFindingsRequest describeImageScanFindingsRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link EcrAsyncClient#describeImageScanFindings} API until the desired condition {@code ImageScanComplete}
* is met, or until it is determined that the resource will never enter into the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param describeImageScanFindingsRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default CompletableFuture> waitUntilImageScanComplete(
Consumer describeImageScanFindingsRequest,
Consumer overrideConfig) {
return waitUntilImageScanComplete(
DescribeImageScanFindingsRequest.builder().applyMutation(describeImageScanFindingsRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Polls {@link EcrAsyncClient#getLifecyclePolicyPreview} API until the desired condition
* {@code LifecyclePolicyPreviewComplete} is met, or until it is determined that the resource will never enter into
* the desired state
*
* @param getLifecyclePolicyPreviewRequest
* the request to be used for polling
* @return CompletableFuture containing the WaiterResponse. It completes successfully when the resource enters into
* a desired state or exceptionally when it is determined that the resource will never enter into the
* desired state.
*/
default CompletableFuture> waitUntilLifecyclePolicyPreviewComplete(
GetLifecyclePolicyPreviewRequest getLifecyclePolicyPreviewRequest) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link EcrAsyncClient#getLifecyclePolicyPreview} API until the desired condition
* {@code LifecyclePolicyPreviewComplete} is met, or until it is determined that the resource will never enter into
* the desired state.
*
* This is a convenience method to create an instance of the request builder without the need to create one manually
* using {@link GetLifecyclePolicyPreviewRequest#builder()}
*
* @param getLifecyclePolicyPreviewRequest
* The consumer that will configure the request to be used for polling
* @return CompletableFuture of the WaiterResponse containing either a response or an exception that has matched
* with the waiter success condition
*/
default CompletableFuture> waitUntilLifecyclePolicyPreviewComplete(
Consumer getLifecyclePolicyPreviewRequest) {
return waitUntilLifecyclePolicyPreviewComplete(GetLifecyclePolicyPreviewRequest.builder()
.applyMutation(getLifecyclePolicyPreviewRequest).build());
}
/**
* Polls {@link EcrAsyncClient#getLifecyclePolicyPreview} API until the desired condition
* {@code LifecyclePolicyPreviewComplete} is met, or until it is determined that the resource will never enter into
* the desired state
*
* @param getLifecyclePolicyPreviewRequest
* The request to be used for polling
* @param overrideConfig
* Per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default CompletableFuture> waitUntilLifecyclePolicyPreviewComplete(
GetLifecyclePolicyPreviewRequest getLifecyclePolicyPreviewRequest, WaiterOverrideConfiguration overrideConfig) {
throw new UnsupportedOperationException();
}
/**
* Polls {@link EcrAsyncClient#getLifecyclePolicyPreview} API until the desired condition
* {@code LifecyclePolicyPreviewComplete} is met, or until it is determined that the resource will never enter into
* the desired state.
*
* This is a convenience method to create an instance of the request builder and instance of the override config
* builder
*
* @param getLifecyclePolicyPreviewRequest
* The consumer that will configure the request to be used for polling
* @param overrideConfig
* The consumer that will configure the per request override configuration for waiters
* @return WaiterResponse containing either a response or an exception that has matched with the waiter success
* condition
*/
default CompletableFuture> waitUntilLifecyclePolicyPreviewComplete(
Consumer getLifecyclePolicyPreviewRequest,
Consumer overrideConfig) {
return waitUntilLifecyclePolicyPreviewComplete(
GetLifecyclePolicyPreviewRequest.builder().applyMutation(getLifecyclePolicyPreviewRequest).build(),
WaiterOverrideConfiguration.builder().applyMutation(overrideConfig).build());
}
/**
* Create a builder that can be used to configure and create a {@link EcrAsyncWaiter}.
*
* @return a builder
*/
static Builder builder() {
return DefaultEcrAsyncWaiter.builder();
}
/**
* Create an instance of {@link EcrAsyncWaiter} with the default configuration.
*
* A default {@link EcrAsyncClient} will be created to poll resources. It is recommended to share a single
* instance of the waiter created via this method. If it is not desirable to share a waiter instance, invoke
* {@link #close()} to release the resources once the waiter is not needed.
*
* @return an instance of {@link EcrAsyncWaiter}
*/
static EcrAsyncWaiter create() {
return DefaultEcrAsyncWaiter.builder().build();
}
interface Builder {
/**
* Sets a custom {@link ScheduledExecutorService} that will be used to schedule async polling attempts
*
* This executorService must be closed by the caller when it is ready to be disposed. The SDK will not close the
* executorService when the waiter is closed
*
* @param executorService
* the executorService to set
* @return a reference to this object so that method calls can be chained together.
*/
Builder scheduledExecutorService(ScheduledExecutorService executorService);
/**
* Defines overrides to the default SDK waiter configuration that should be used for waiters created from this
* builder
*
* @param overrideConfiguration
* the override configuration to set
* @return a reference to this object so that method calls can be chained together.
*/
Builder overrideConfiguration(WaiterOverrideConfiguration overrideConfiguration);
/**
* This is a convenient method to pass the override configuration without the need to create an instance
* manually via {@link WaiterOverrideConfiguration#builder()}
*
* @param overrideConfiguration
* The consumer that will configure the overrideConfiguration
* @return a reference to this object so that method calls can be chained together.
* @see #overrideConfiguration(WaiterOverrideConfiguration)
*/
default Builder overrideConfiguration(Consumer overrideConfiguration) {
WaiterOverrideConfiguration.Builder builder = WaiterOverrideConfiguration.builder();
overrideConfiguration.accept(builder);
return overrideConfiguration(builder.build());
}
/**
* Sets a custom {@link EcrAsyncClient} that will be used to poll the resource
*
* This SDK client must be closed by the caller when it is ready to be disposed. The SDK will not close the
* client when the waiter is closed
*
* @param client
* the client to send the request
* @return a reference to this object so that method calls can be chained together.
*/
Builder client(EcrAsyncClient client);
/**
* Builds an instance of {@link EcrAsyncWaiter} based on the configurations supplied to this builder
*
* @return An initialized {@link EcrAsyncWaiter}
*/
EcrAsyncWaiter build();
}
}