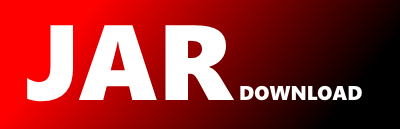
software.amazon.awssdk.services.ecr.model.UploadLayerPartRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecr Show documentation
Show all versions of ecr Show documentation
The AWS Java SDK for the Amazon EC2 Container Registry holds the client classes that are used for
communicating with the
Amazon EC2 Container Registry Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.model;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkBytes;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UploadLayerPartRequest extends EcrRequest implements
ToCopyableBuilder {
private static final SdkField REGISTRY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("registryId").getter(getter(UploadLayerPartRequest::registryId)).setter(setter(Builder::registryId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registryId").build()).build();
private static final SdkField REPOSITORY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("repositoryName").getter(getter(UploadLayerPartRequest::repositoryName))
.setter(setter(Builder::repositoryName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("repositoryName").build()).build();
private static final SdkField UPLOAD_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("uploadId").getter(getter(UploadLayerPartRequest::uploadId)).setter(setter(Builder::uploadId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("uploadId").build()).build();
private static final SdkField PART_FIRST_BYTE_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("partFirstByte").getter(getter(UploadLayerPartRequest::partFirstByte))
.setter(setter(Builder::partFirstByte))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("partFirstByte").build()).build();
private static final SdkField PART_LAST_BYTE_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("partLastByte").getter(getter(UploadLayerPartRequest::partLastByte))
.setter(setter(Builder::partLastByte))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("partLastByte").build()).build();
private static final SdkField LAYER_PART_BLOB_FIELD = SdkField. builder(MarshallingType.SDK_BYTES)
.memberName("layerPartBlob").getter(getter(UploadLayerPartRequest::layerPartBlob))
.setter(setter(Builder::layerPartBlob))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("layerPartBlob").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REGISTRY_ID_FIELD,
REPOSITORY_NAME_FIELD, UPLOAD_ID_FIELD, PART_FIRST_BYTE_FIELD, PART_LAST_BYTE_FIELD, LAYER_PART_BLOB_FIELD));
private final String registryId;
private final String repositoryName;
private final String uploadId;
private final Long partFirstByte;
private final Long partLastByte;
private final SdkBytes layerPartBlob;
private UploadLayerPartRequest(BuilderImpl builder) {
super(builder);
this.registryId = builder.registryId;
this.repositoryName = builder.repositoryName;
this.uploadId = builder.uploadId;
this.partFirstByte = builder.partFirstByte;
this.partLastByte = builder.partLastByte;
this.layerPartBlob = builder.layerPartBlob;
}
/**
*
* The Amazon Web Services account ID associated with the registry to which you are uploading layer parts. If you do
* not specify a registry, the default registry is assumed.
*
*
* @return The Amazon Web Services account ID associated with the registry to which you are uploading layer parts.
* If you do not specify a registry, the default registry is assumed.
*/
public final String registryId() {
return registryId;
}
/**
*
* The name of the repository to which you are uploading layer parts.
*
*
* @return The name of the repository to which you are uploading layer parts.
*/
public final String repositoryName() {
return repositoryName;
}
/**
*
* The upload ID from a previous InitiateLayerUpload operation to associate with the layer part upload.
*
*
* @return The upload ID from a previous InitiateLayerUpload operation to associate with the layer part
* upload.
*/
public final String uploadId() {
return uploadId;
}
/**
*
* The position of the first byte of the layer part witin the overall image layer.
*
*
* @return The position of the first byte of the layer part witin the overall image layer.
*/
public final Long partFirstByte() {
return partFirstByte;
}
/**
*
* The position of the last byte of the layer part within the overall image layer.
*
*
* @return The position of the last byte of the layer part within the overall image layer.
*/
public final Long partLastByte() {
return partLastByte;
}
/**
*
* The base64-encoded layer part payload.
*
*
* @return The base64-encoded layer part payload.
*/
public final SdkBytes layerPartBlob() {
return layerPartBlob;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(registryId());
hashCode = 31 * hashCode + Objects.hashCode(repositoryName());
hashCode = 31 * hashCode + Objects.hashCode(uploadId());
hashCode = 31 * hashCode + Objects.hashCode(partFirstByte());
hashCode = 31 * hashCode + Objects.hashCode(partLastByte());
hashCode = 31 * hashCode + Objects.hashCode(layerPartBlob());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UploadLayerPartRequest)) {
return false;
}
UploadLayerPartRequest other = (UploadLayerPartRequest) obj;
return Objects.equals(registryId(), other.registryId()) && Objects.equals(repositoryName(), other.repositoryName())
&& Objects.equals(uploadId(), other.uploadId()) && Objects.equals(partFirstByte(), other.partFirstByte())
&& Objects.equals(partLastByte(), other.partLastByte()) && Objects.equals(layerPartBlob(), other.layerPartBlob());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UploadLayerPartRequest").add("RegistryId", registryId()).add("RepositoryName", repositoryName())
.add("UploadId", uploadId()).add("PartFirstByte", partFirstByte()).add("PartLastByte", partLastByte())
.add("LayerPartBlob", layerPartBlob()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "registryId":
return Optional.ofNullable(clazz.cast(registryId()));
case "repositoryName":
return Optional.ofNullable(clazz.cast(repositoryName()));
case "uploadId":
return Optional.ofNullable(clazz.cast(uploadId()));
case "partFirstByte":
return Optional.ofNullable(clazz.cast(partFirstByte()));
case "partLastByte":
return Optional.ofNullable(clazz.cast(partLastByte()));
case "layerPartBlob":
return Optional.ofNullable(clazz.cast(layerPartBlob()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy