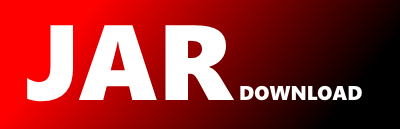
software.amazon.awssdk.services.ecr.EcrClient Maven / Gradle / Ivy
Show all versions of ecr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.ecr.model.BatchCheckLayerAvailabilityRequest;
import software.amazon.awssdk.services.ecr.model.BatchCheckLayerAvailabilityResponse;
import software.amazon.awssdk.services.ecr.model.BatchDeleteImageRequest;
import software.amazon.awssdk.services.ecr.model.BatchDeleteImageResponse;
import software.amazon.awssdk.services.ecr.model.BatchGetImageRequest;
import software.amazon.awssdk.services.ecr.model.BatchGetImageResponse;
import software.amazon.awssdk.services.ecr.model.BatchGetRepositoryScanningConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.BatchGetRepositoryScanningConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.CompleteLayerUploadRequest;
import software.amazon.awssdk.services.ecr.model.CompleteLayerUploadResponse;
import software.amazon.awssdk.services.ecr.model.CreatePullThroughCacheRuleRequest;
import software.amazon.awssdk.services.ecr.model.CreatePullThroughCacheRuleResponse;
import software.amazon.awssdk.services.ecr.model.CreateRepositoryCreationTemplateRequest;
import software.amazon.awssdk.services.ecr.model.CreateRepositoryCreationTemplateResponse;
import software.amazon.awssdk.services.ecr.model.CreateRepositoryRequest;
import software.amazon.awssdk.services.ecr.model.CreateRepositoryResponse;
import software.amazon.awssdk.services.ecr.model.DeleteLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.DeleteLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.DeletePullThroughCacheRuleRequest;
import software.amazon.awssdk.services.ecr.model.DeletePullThroughCacheRuleResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRegistryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRegistryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryCreationTemplateRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryCreationTemplateResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryResponse;
import software.amazon.awssdk.services.ecr.model.DescribeImageReplicationStatusRequest;
import software.amazon.awssdk.services.ecr.model.DescribeImageReplicationStatusResponse;
import software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest;
import software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsResponse;
import software.amazon.awssdk.services.ecr.model.DescribeImagesRequest;
import software.amazon.awssdk.services.ecr.model.DescribeImagesResponse;
import software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest;
import software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesResponse;
import software.amazon.awssdk.services.ecr.model.DescribeRegistryRequest;
import software.amazon.awssdk.services.ecr.model.DescribeRegistryResponse;
import software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest;
import software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse;
import software.amazon.awssdk.services.ecr.model.DescribeRepositoryCreationTemplatesRequest;
import software.amazon.awssdk.services.ecr.model.DescribeRepositoryCreationTemplatesResponse;
import software.amazon.awssdk.services.ecr.model.EcrException;
import software.amazon.awssdk.services.ecr.model.EmptyUploadException;
import software.amazon.awssdk.services.ecr.model.GetAccountSettingRequest;
import software.amazon.awssdk.services.ecr.model.GetAccountSettingResponse;
import software.amazon.awssdk.services.ecr.model.GetAuthorizationTokenRequest;
import software.amazon.awssdk.services.ecr.model.GetAuthorizationTokenResponse;
import software.amazon.awssdk.services.ecr.model.GetDownloadUrlForLayerRequest;
import software.amazon.awssdk.services.ecr.model.GetDownloadUrlForLayerResponse;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewResponse;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.GetRegistryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.GetRegistryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.GetRegistryScanningConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.GetRegistryScanningConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.GetRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.GetRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.ImageAlreadyExistsException;
import software.amazon.awssdk.services.ecr.model.ImageDigestDoesNotMatchException;
import software.amazon.awssdk.services.ecr.model.ImageNotFoundException;
import software.amazon.awssdk.services.ecr.model.ImageTagAlreadyExistsException;
import software.amazon.awssdk.services.ecr.model.InitiateLayerUploadRequest;
import software.amazon.awssdk.services.ecr.model.InitiateLayerUploadResponse;
import software.amazon.awssdk.services.ecr.model.InvalidLayerException;
import software.amazon.awssdk.services.ecr.model.InvalidLayerPartException;
import software.amazon.awssdk.services.ecr.model.InvalidParameterException;
import software.amazon.awssdk.services.ecr.model.InvalidTagParameterException;
import software.amazon.awssdk.services.ecr.model.KmsException;
import software.amazon.awssdk.services.ecr.model.LayerAlreadyExistsException;
import software.amazon.awssdk.services.ecr.model.LayerInaccessibleException;
import software.amazon.awssdk.services.ecr.model.LayerPartTooSmallException;
import software.amazon.awssdk.services.ecr.model.LayersNotFoundException;
import software.amazon.awssdk.services.ecr.model.LifecyclePolicyNotFoundException;
import software.amazon.awssdk.services.ecr.model.LifecyclePolicyPreviewInProgressException;
import software.amazon.awssdk.services.ecr.model.LifecyclePolicyPreviewNotFoundException;
import software.amazon.awssdk.services.ecr.model.LimitExceededException;
import software.amazon.awssdk.services.ecr.model.ListImagesRequest;
import software.amazon.awssdk.services.ecr.model.ListImagesResponse;
import software.amazon.awssdk.services.ecr.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.ecr.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.ecr.model.PullThroughCacheRuleAlreadyExistsException;
import software.amazon.awssdk.services.ecr.model.PullThroughCacheRuleNotFoundException;
import software.amazon.awssdk.services.ecr.model.PutAccountSettingRequest;
import software.amazon.awssdk.services.ecr.model.PutAccountSettingResponse;
import software.amazon.awssdk.services.ecr.model.PutImageRequest;
import software.amazon.awssdk.services.ecr.model.PutImageResponse;
import software.amazon.awssdk.services.ecr.model.PutImageScanningConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.PutImageScanningConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.PutImageTagMutabilityRequest;
import software.amazon.awssdk.services.ecr.model.PutImageTagMutabilityResponse;
import software.amazon.awssdk.services.ecr.model.PutLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.PutLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.PutRegistryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.PutRegistryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.PutRegistryScanningConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.PutRegistryScanningConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.PutReplicationConfigurationRequest;
import software.amazon.awssdk.services.ecr.model.PutReplicationConfigurationResponse;
import software.amazon.awssdk.services.ecr.model.ReferencedImagesNotFoundException;
import software.amazon.awssdk.services.ecr.model.RegistryPolicyNotFoundException;
import software.amazon.awssdk.services.ecr.model.RepositoryAlreadyExistsException;
import software.amazon.awssdk.services.ecr.model.RepositoryNotEmptyException;
import software.amazon.awssdk.services.ecr.model.RepositoryNotFoundException;
import software.amazon.awssdk.services.ecr.model.RepositoryPolicyNotFoundException;
import software.amazon.awssdk.services.ecr.model.ScanNotFoundException;
import software.amazon.awssdk.services.ecr.model.SecretNotFoundException;
import software.amazon.awssdk.services.ecr.model.ServerException;
import software.amazon.awssdk.services.ecr.model.SetRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.SetRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.StartImageScanRequest;
import software.amazon.awssdk.services.ecr.model.StartImageScanResponse;
import software.amazon.awssdk.services.ecr.model.StartLifecyclePolicyPreviewRequest;
import software.amazon.awssdk.services.ecr.model.StartLifecyclePolicyPreviewResponse;
import software.amazon.awssdk.services.ecr.model.TagResourceRequest;
import software.amazon.awssdk.services.ecr.model.TagResourceResponse;
import software.amazon.awssdk.services.ecr.model.TemplateAlreadyExistsException;
import software.amazon.awssdk.services.ecr.model.TemplateNotFoundException;
import software.amazon.awssdk.services.ecr.model.TooManyTagsException;
import software.amazon.awssdk.services.ecr.model.UnableToAccessSecretException;
import software.amazon.awssdk.services.ecr.model.UnableToDecryptSecretValueException;
import software.amazon.awssdk.services.ecr.model.UnableToGetUpstreamImageException;
import software.amazon.awssdk.services.ecr.model.UnableToGetUpstreamLayerException;
import software.amazon.awssdk.services.ecr.model.UnsupportedImageTypeException;
import software.amazon.awssdk.services.ecr.model.UnsupportedUpstreamRegistryException;
import software.amazon.awssdk.services.ecr.model.UntagResourceRequest;
import software.amazon.awssdk.services.ecr.model.UntagResourceResponse;
import software.amazon.awssdk.services.ecr.model.UpdatePullThroughCacheRuleRequest;
import software.amazon.awssdk.services.ecr.model.UpdatePullThroughCacheRuleResponse;
import software.amazon.awssdk.services.ecr.model.UpdateRepositoryCreationTemplateRequest;
import software.amazon.awssdk.services.ecr.model.UpdateRepositoryCreationTemplateResponse;
import software.amazon.awssdk.services.ecr.model.UploadLayerPartRequest;
import software.amazon.awssdk.services.ecr.model.UploadLayerPartResponse;
import software.amazon.awssdk.services.ecr.model.UploadNotFoundException;
import software.amazon.awssdk.services.ecr.model.ValidatePullThroughCacheRuleRequest;
import software.amazon.awssdk.services.ecr.model.ValidatePullThroughCacheRuleResponse;
import software.amazon.awssdk.services.ecr.model.ValidationException;
import software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsIterable;
import software.amazon.awssdk.services.ecr.paginators.DescribeImagesIterable;
import software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesIterable;
import software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable;
import software.amazon.awssdk.services.ecr.paginators.DescribeRepositoryCreationTemplatesIterable;
import software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewIterable;
import software.amazon.awssdk.services.ecr.paginators.ListImagesIterable;
import software.amazon.awssdk.services.ecr.waiters.EcrWaiter;
/**
* Service client for accessing Amazon ECR. This can be created using the static {@link #builder()} method.
*
* Amazon Elastic Container Registry
*
* Amazon Elastic Container Registry (Amazon ECR) is a managed container image registry service. Customers can use the
* familiar Docker CLI, or their preferred client, to push, pull, and manage images. Amazon ECR provides a secure,
* scalable, and reliable registry for your Docker or Open Container Initiative (OCI) images. Amazon ECR supports
* private repositories with resource-based permissions using IAM so that specific users or Amazon EC2 instances can
* access repositories and images.
*
*
* Amazon ECR has service endpoints in each supported Region. For more information, see Amazon ECR endpoints in the Amazon Web Services
* General Reference.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface EcrClient extends AwsClient {
String SERVICE_NAME = "ecr";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "api.ecr";
/**
*
* Checks the availability of one or more image layers in a repository.
*
*
* When an image is pushed to a repository, each image layer is checked to verify if it has been uploaded before. If
* it has been uploaded, then the image layer is skipped.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param batchCheckLayerAvailabilityRequest
* @return Result of the BatchCheckLayerAvailability operation returned by the service.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.BatchCheckLayerAvailability
* @see AWS API Documentation
*/
default BatchCheckLayerAvailabilityResponse batchCheckLayerAvailability(
BatchCheckLayerAvailabilityRequest batchCheckLayerAvailabilityRequest) throws RepositoryNotFoundException,
InvalidParameterException, ServerException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Checks the availability of one or more image layers in a repository.
*
*
* When an image is pushed to a repository, each image layer is checked to verify if it has been uploaded before. If
* it has been uploaded, then the image layer is skipped.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link BatchCheckLayerAvailabilityRequest.Builder}
* avoiding the need to create one manually via {@link BatchCheckLayerAvailabilityRequest#builder()}
*
*
* @param batchCheckLayerAvailabilityRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.BatchCheckLayerAvailabilityRequest.Builder} to create a
* request.
* @return Result of the BatchCheckLayerAvailability operation returned by the service.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.BatchCheckLayerAvailability
* @see AWS API Documentation
*/
default BatchCheckLayerAvailabilityResponse batchCheckLayerAvailability(
Consumer batchCheckLayerAvailabilityRequest)
throws RepositoryNotFoundException, InvalidParameterException, ServerException, AwsServiceException,
SdkClientException, EcrException {
return batchCheckLayerAvailability(BatchCheckLayerAvailabilityRequest.builder()
.applyMutation(batchCheckLayerAvailabilityRequest).build());
}
/**
*
* Deletes a list of specified images within a repository. Images are specified with either an imageTag
* or imageDigest
.
*
*
* You can remove a tag from an image by specifying the image's tag in your request. When you remove the last tag
* from an image, the image is deleted from your repository.
*
*
* You can completely delete an image (and all of its tags) by specifying the image's digest in your request.
*
*
* @param batchDeleteImageRequest
* Deletes specified images within a specified repository. Images are specified with either the
* imageTag
or imageDigest
.
* @return Result of the BatchDeleteImage operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.BatchDeleteImage
* @see AWS API
* Documentation
*/
default BatchDeleteImageResponse batchDeleteImage(BatchDeleteImageRequest batchDeleteImageRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a list of specified images within a repository. Images are specified with either an imageTag
* or imageDigest
.
*
*
* You can remove a tag from an image by specifying the image's tag in your request. When you remove the last tag
* from an image, the image is deleted from your repository.
*
*
* You can completely delete an image (and all of its tags) by specifying the image's digest in your request.
*
*
*
* This is a convenience which creates an instance of the {@link BatchDeleteImageRequest.Builder} avoiding the need
* to create one manually via {@link BatchDeleteImageRequest#builder()}
*
*
* @param batchDeleteImageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.BatchDeleteImageRequest.Builder} to create a request.
* Deletes specified images within a specified repository. Images are specified with either the
* imageTag
or imageDigest
.
* @return Result of the BatchDeleteImage operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.BatchDeleteImage
* @see AWS API
* Documentation
*/
default BatchDeleteImageResponse batchDeleteImage(Consumer batchDeleteImageRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return batchDeleteImage(BatchDeleteImageRequest.builder().applyMutation(batchDeleteImageRequest).build());
}
/**
*
* Gets detailed information for an image. Images are specified with either an imageTag
or
* imageDigest
.
*
*
* When an image is pulled, the BatchGetImage API is called once to retrieve the image manifest.
*
*
* @param batchGetImageRequest
* @return Result of the BatchGetImage operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws UnableToGetUpstreamImageException
* The image or images were unable to be pulled using the pull through cache rule. This is usually caused
* because of an issue with the Secrets Manager secret containing the credentials for the upstream registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.BatchGetImage
* @see AWS API
* Documentation
*/
default BatchGetImageResponse batchGetImage(BatchGetImageRequest batchGetImageRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, LimitExceededException, UnableToGetUpstreamImageException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Gets detailed information for an image. Images are specified with either an imageTag
or
* imageDigest
.
*
*
* When an image is pulled, the BatchGetImage API is called once to retrieve the image manifest.
*
*
*
* This is a convenience which creates an instance of the {@link BatchGetImageRequest.Builder} avoiding the need to
* create one manually via {@link BatchGetImageRequest#builder()}
*
*
* @param batchGetImageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.BatchGetImageRequest.Builder} to create a request.
* @return Result of the BatchGetImage operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws UnableToGetUpstreamImageException
* The image or images were unable to be pulled using the pull through cache rule. This is usually caused
* because of an issue with the Secrets Manager secret containing the credentials for the upstream registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.BatchGetImage
* @see AWS API
* Documentation
*/
default BatchGetImageResponse batchGetImage(Consumer batchGetImageRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, LimitExceededException,
UnableToGetUpstreamImageException, AwsServiceException, SdkClientException, EcrException {
return batchGetImage(BatchGetImageRequest.builder().applyMutation(batchGetImageRequest).build());
}
/**
*
* Gets the scanning configuration for one or more repositories.
*
*
* @param batchGetRepositoryScanningConfigurationRequest
* @return Result of the BatchGetRepositoryScanningConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.BatchGetRepositoryScanningConfiguration
* @see AWS API Documentation
*/
default BatchGetRepositoryScanningConfigurationResponse batchGetRepositoryScanningConfiguration(
BatchGetRepositoryScanningConfigurationRequest batchGetRepositoryScanningConfigurationRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, ValidationException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the scanning configuration for one or more repositories.
*
*
*
* This is a convenience which creates an instance of the
* {@link BatchGetRepositoryScanningConfigurationRequest.Builder} avoiding the need to create one manually via
* {@link BatchGetRepositoryScanningConfigurationRequest#builder()}
*
*
* @param batchGetRepositoryScanningConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.BatchGetRepositoryScanningConfigurationRequest.Builder}
* to create a request.
* @return Result of the BatchGetRepositoryScanningConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.BatchGetRepositoryScanningConfiguration
* @see AWS API Documentation
*/
default BatchGetRepositoryScanningConfigurationResponse batchGetRepositoryScanningConfiguration(
Consumer batchGetRepositoryScanningConfigurationRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, ValidationException,
AwsServiceException, SdkClientException, EcrException {
return batchGetRepositoryScanningConfiguration(BatchGetRepositoryScanningConfigurationRequest.builder()
.applyMutation(batchGetRepositoryScanningConfigurationRequest).build());
}
/**
*
* Informs Amazon ECR that the image layer upload has completed for a specified registry, repository name, and
* upload ID. You can optionally provide a sha256
digest of the image layer for data validation
* purposes.
*
*
* When an image is pushed, the CompleteLayerUpload API is called once per each new image layer to verify that the
* upload has completed.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param completeLayerUploadRequest
* @return Result of the CompleteLayerUpload operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws UploadNotFoundException
* The upload could not be found, or the specified upload ID is not valid for this repository.
* @throws InvalidLayerException
* The layer digest calculation performed by Amazon ECR upon receipt of the image layer does not match the
* digest specified.
* @throws LayerPartTooSmallException
* Layer parts must be at least 5 MiB in size.
* @throws LayerAlreadyExistsException
* The image layer already exists in the associated repository.
* @throws EmptyUploadException
* The specified layer upload does not contain any layer parts.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.CompleteLayerUpload
* @see AWS API
* Documentation
*/
default CompleteLayerUploadResponse completeLayerUpload(CompleteLayerUploadRequest completeLayerUploadRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, UploadNotFoundException,
InvalidLayerException, LayerPartTooSmallException, LayerAlreadyExistsException, EmptyUploadException, KmsException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Informs Amazon ECR that the image layer upload has completed for a specified registry, repository name, and
* upload ID. You can optionally provide a sha256
digest of the image layer for data validation
* purposes.
*
*
* When an image is pushed, the CompleteLayerUpload API is called once per each new image layer to verify that the
* upload has completed.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link CompleteLayerUploadRequest.Builder} avoiding the
* need to create one manually via {@link CompleteLayerUploadRequest#builder()}
*
*
* @param completeLayerUploadRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.CompleteLayerUploadRequest.Builder} to create a request.
* @return Result of the CompleteLayerUpload operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws UploadNotFoundException
* The upload could not be found, or the specified upload ID is not valid for this repository.
* @throws InvalidLayerException
* The layer digest calculation performed by Amazon ECR upon receipt of the image layer does not match the
* digest specified.
* @throws LayerPartTooSmallException
* Layer parts must be at least 5 MiB in size.
* @throws LayerAlreadyExistsException
* The image layer already exists in the associated repository.
* @throws EmptyUploadException
* The specified layer upload does not contain any layer parts.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.CompleteLayerUpload
* @see AWS API
* Documentation
*/
default CompleteLayerUploadResponse completeLayerUpload(
Consumer completeLayerUploadRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, UploadNotFoundException, InvalidLayerException,
LayerPartTooSmallException, LayerAlreadyExistsException, EmptyUploadException, KmsException, AwsServiceException,
SdkClientException, EcrException {
return completeLayerUpload(CompleteLayerUploadRequest.builder().applyMutation(completeLayerUploadRequest).build());
}
/**
*
* Creates a pull through cache rule. A pull through cache rule provides a way to cache images from an upstream
* registry source in your Amazon ECR private registry. For more information, see Using pull through cache
* rules in the Amazon Elastic Container Registry User Guide.
*
*
* @param createPullThroughCacheRuleRequest
* @return Result of the CreatePullThroughCacheRule operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws PullThroughCacheRuleAlreadyExistsException
* A pull through cache rule with these settings already exists for the private registry.
* @throws UnsupportedUpstreamRegistryException
* The specified upstream registry isn't supported.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws UnableToAccessSecretException
* The secret is unable to be accessed. Verify the resource permissions for the secret and try again.
* @throws SecretNotFoundException
* The ARN of the secret specified in the pull through cache rule was not found. Update the pull through
* cache rule with a valid secret ARN and try again.
* @throws UnableToDecryptSecretValueException
* The secret is accessible but is unable to be decrypted. Verify the resource permisisons and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.CreatePullThroughCacheRule
* @see AWS API Documentation
*/
default CreatePullThroughCacheRuleResponse createPullThroughCacheRule(
CreatePullThroughCacheRuleRequest createPullThroughCacheRuleRequest) throws ServerException,
InvalidParameterException, ValidationException, PullThroughCacheRuleAlreadyExistsException,
UnsupportedUpstreamRegistryException, LimitExceededException, UnableToAccessSecretException, SecretNotFoundException,
UnableToDecryptSecretValueException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a pull through cache rule. A pull through cache rule provides a way to cache images from an upstream
* registry source in your Amazon ECR private registry. For more information, see Using pull through cache
* rules in the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreatePullThroughCacheRuleRequest.Builder} avoiding
* the need to create one manually via {@link CreatePullThroughCacheRuleRequest#builder()}
*
*
* @param createPullThroughCacheRuleRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.CreatePullThroughCacheRuleRequest.Builder} to create a
* request.
* @return Result of the CreatePullThroughCacheRule operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws PullThroughCacheRuleAlreadyExistsException
* A pull through cache rule with these settings already exists for the private registry.
* @throws UnsupportedUpstreamRegistryException
* The specified upstream registry isn't supported.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws UnableToAccessSecretException
* The secret is unable to be accessed. Verify the resource permissions for the secret and try again.
* @throws SecretNotFoundException
* The ARN of the secret specified in the pull through cache rule was not found. Update the pull through
* cache rule with a valid secret ARN and try again.
* @throws UnableToDecryptSecretValueException
* The secret is accessible but is unable to be decrypted. Verify the resource permisisons and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.CreatePullThroughCacheRule
* @see AWS API Documentation
*/
default CreatePullThroughCacheRuleResponse createPullThroughCacheRule(
Consumer createPullThroughCacheRuleRequest) throws ServerException,
InvalidParameterException, ValidationException, PullThroughCacheRuleAlreadyExistsException,
UnsupportedUpstreamRegistryException, LimitExceededException, UnableToAccessSecretException, SecretNotFoundException,
UnableToDecryptSecretValueException, AwsServiceException, SdkClientException, EcrException {
return createPullThroughCacheRule(CreatePullThroughCacheRuleRequest.builder()
.applyMutation(createPullThroughCacheRuleRequest).build());
}
/**
*
* Creates a repository. For more information, see Amazon ECR repositories in
* the Amazon Elastic Container Registry User Guide.
*
*
* @param createRepositoryRequest
* @return Result of the CreateRepository operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidTagParameterException
* An invalid parameter has been specified. Tag keys can have a maximum character length of 128 characters,
* and tag values can have a maximum length of 256 characters.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws RepositoryAlreadyExistsException
* The specified repository already exists in the specified registry.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.CreateRepository
* @see AWS API
* Documentation
*/
default CreateRepositoryResponse createRepository(CreateRepositoryRequest createRepositoryRequest) throws ServerException,
InvalidParameterException, InvalidTagParameterException, TooManyTagsException, RepositoryAlreadyExistsException,
LimitExceededException, KmsException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a repository. For more information, see Amazon ECR repositories in
* the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRepositoryRequest.Builder} avoiding the need
* to create one manually via {@link CreateRepositoryRequest#builder()}
*
*
* @param createRepositoryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.CreateRepositoryRequest.Builder} to create a request.
* @return Result of the CreateRepository operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidTagParameterException
* An invalid parameter has been specified. Tag keys can have a maximum character length of 128 characters,
* and tag values can have a maximum length of 256 characters.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws RepositoryAlreadyExistsException
* The specified repository already exists in the specified registry.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.CreateRepository
* @see AWS API
* Documentation
*/
default CreateRepositoryResponse createRepository(Consumer createRepositoryRequest)
throws ServerException, InvalidParameterException, InvalidTagParameterException, TooManyTagsException,
RepositoryAlreadyExistsException, LimitExceededException, KmsException, AwsServiceException, SdkClientException,
EcrException {
return createRepository(CreateRepositoryRequest.builder().applyMutation(createRepositoryRequest).build());
}
/**
*
* Creates a repository creation template. This template is used to define the settings for repositories created by
* Amazon ECR on your behalf. For example, repositories created through pull through cache actions. For more
* information, see Private
* repository creation templates in the Amazon Elastic Container Registry User Guide.
*
*
* @param createRepositoryCreationTemplateRequest
* @return Result of the CreateRepositoryCreationTemplate operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws TemplateAlreadyExistsException
* The repository creation template already exists. Specify a unique prefix and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.CreateRepositoryCreationTemplate
* @see AWS API Documentation
*/
default CreateRepositoryCreationTemplateResponse createRepositoryCreationTemplate(
CreateRepositoryCreationTemplateRequest createRepositoryCreationTemplateRequest) throws ServerException,
ValidationException, InvalidParameterException, LimitExceededException, TemplateAlreadyExistsException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a repository creation template. This template is used to define the settings for repositories created by
* Amazon ECR on your behalf. For example, repositories created through pull through cache actions. For more
* information, see Private
* repository creation templates in the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRepositoryCreationTemplateRequest.Builder}
* avoiding the need to create one manually via {@link CreateRepositoryCreationTemplateRequest#builder()}
*
*
* @param createRepositoryCreationTemplateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.CreateRepositoryCreationTemplateRequest.Builder} to
* create a request.
* @return Result of the CreateRepositoryCreationTemplate operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws TemplateAlreadyExistsException
* The repository creation template already exists. Specify a unique prefix and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.CreateRepositoryCreationTemplate
* @see AWS API Documentation
*/
default CreateRepositoryCreationTemplateResponse createRepositoryCreationTemplate(
Consumer createRepositoryCreationTemplateRequest)
throws ServerException, ValidationException, InvalidParameterException, LimitExceededException,
TemplateAlreadyExistsException, AwsServiceException, SdkClientException, EcrException {
return createRepositoryCreationTemplate(CreateRepositoryCreationTemplateRequest.builder()
.applyMutation(createRepositoryCreationTemplateRequest).build());
}
/**
*
* Deletes the lifecycle policy associated with the specified repository.
*
*
* @param deleteLifecyclePolicyRequest
* @return Result of the DeleteLifecyclePolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyNotFoundException
* The lifecycle policy could not be found, and no policy is set to the repository.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteLifecyclePolicy
* @see AWS API
* Documentation
*/
default DeleteLifecyclePolicyResponse deleteLifecyclePolicy(DeleteLifecyclePolicyRequest deleteLifecyclePolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, LifecyclePolicyNotFoundException,
ValidationException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the lifecycle policy associated with the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteLifecyclePolicyRequest#builder()}
*
*
* @param deleteLifecyclePolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DeleteLifecyclePolicyRequest.Builder} to create a
* request.
* @return Result of the DeleteLifecyclePolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyNotFoundException
* The lifecycle policy could not be found, and no policy is set to the repository.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteLifecyclePolicy
* @see AWS API
* Documentation
*/
default DeleteLifecyclePolicyResponse deleteLifecyclePolicy(
Consumer deleteLifecyclePolicyRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, LifecyclePolicyNotFoundException, ValidationException,
AwsServiceException, SdkClientException, EcrException {
return deleteLifecyclePolicy(DeleteLifecyclePolicyRequest.builder().applyMutation(deleteLifecyclePolicyRequest).build());
}
/**
*
* Deletes a pull through cache rule.
*
*
* @param deletePullThroughCacheRuleRequest
* @return Result of the DeletePullThroughCacheRule operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws PullThroughCacheRuleNotFoundException
* The pull through cache rule was not found. Specify a valid pull through cache rule and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeletePullThroughCacheRule
* @see AWS API Documentation
*/
default DeletePullThroughCacheRuleResponse deletePullThroughCacheRule(
DeletePullThroughCacheRuleRequest deletePullThroughCacheRuleRequest) throws ServerException,
InvalidParameterException, ValidationException, PullThroughCacheRuleNotFoundException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a pull through cache rule.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePullThroughCacheRuleRequest.Builder} avoiding
* the need to create one manually via {@link DeletePullThroughCacheRuleRequest#builder()}
*
*
* @param deletePullThroughCacheRuleRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DeletePullThroughCacheRuleRequest.Builder} to create a
* request.
* @return Result of the DeletePullThroughCacheRule operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws PullThroughCacheRuleNotFoundException
* The pull through cache rule was not found. Specify a valid pull through cache rule and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeletePullThroughCacheRule
* @see AWS API Documentation
*/
default DeletePullThroughCacheRuleResponse deletePullThroughCacheRule(
Consumer deletePullThroughCacheRuleRequest) throws ServerException,
InvalidParameterException, ValidationException, PullThroughCacheRuleNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return deletePullThroughCacheRule(DeletePullThroughCacheRuleRequest.builder()
.applyMutation(deletePullThroughCacheRuleRequest).build());
}
/**
*
* Deletes the registry permissions policy.
*
*
* @param deleteRegistryPolicyRequest
* @return Result of the DeleteRegistryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RegistryPolicyNotFoundException
* The registry doesn't have an associated registry policy.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteRegistryPolicy
* @see AWS API
* Documentation
*/
default DeleteRegistryPolicyResponse deleteRegistryPolicy(DeleteRegistryPolicyRequest deleteRegistryPolicyRequest)
throws ServerException, InvalidParameterException, RegistryPolicyNotFoundException, ValidationException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the registry permissions policy.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRegistryPolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteRegistryPolicyRequest#builder()}
*
*
* @param deleteRegistryPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DeleteRegistryPolicyRequest.Builder} to create a request.
* @return Result of the DeleteRegistryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RegistryPolicyNotFoundException
* The registry doesn't have an associated registry policy.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteRegistryPolicy
* @see AWS API
* Documentation
*/
default DeleteRegistryPolicyResponse deleteRegistryPolicy(
Consumer deleteRegistryPolicyRequest) throws ServerException,
InvalidParameterException, RegistryPolicyNotFoundException, ValidationException, AwsServiceException,
SdkClientException, EcrException {
return deleteRegistryPolicy(DeleteRegistryPolicyRequest.builder().applyMutation(deleteRegistryPolicyRequest).build());
}
/**
*
* Deletes a repository. If the repository isn't empty, you must either delete the contents of the repository or use
* the force
option to delete the repository and have Amazon ECR delete all of its contents on your
* behalf.
*
*
* @param deleteRepositoryRequest
* @return Result of the DeleteRepository operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws RepositoryNotEmptyException
* The specified repository contains images. To delete a repository that contains images, you must force the
* deletion with the force
parameter.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteRepository
* @see AWS API
* Documentation
*/
default DeleteRepositoryResponse deleteRepository(DeleteRepositoryRequest deleteRepositoryRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, RepositoryNotEmptyException, KmsException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a repository. If the repository isn't empty, you must either delete the contents of the repository or use
* the force
option to delete the repository and have Amazon ECR delete all of its contents on your
* behalf.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRepositoryRequest.Builder} avoiding the need
* to create one manually via {@link DeleteRepositoryRequest#builder()}
*
*
* @param deleteRepositoryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DeleteRepositoryRequest.Builder} to create a request.
* @return Result of the DeleteRepository operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws RepositoryNotEmptyException
* The specified repository contains images. To delete a repository that contains images, you must force the
* deletion with the force
parameter.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteRepository
* @see AWS API
* Documentation
*/
default DeleteRepositoryResponse deleteRepository(Consumer deleteRepositoryRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, RepositoryNotEmptyException,
KmsException, AwsServiceException, SdkClientException, EcrException {
return deleteRepository(DeleteRepositoryRequest.builder().applyMutation(deleteRepositoryRequest).build());
}
/**
*
* Deletes a repository creation template.
*
*
* @param deleteRepositoryCreationTemplateRequest
* @return Result of the DeleteRepositoryCreationTemplate operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws TemplateNotFoundException
* The specified repository creation template can't be found. Verify the registry ID and prefix and try
* again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteRepositoryCreationTemplate
* @see AWS API Documentation
*/
default DeleteRepositoryCreationTemplateResponse deleteRepositoryCreationTemplate(
DeleteRepositoryCreationTemplateRequest deleteRepositoryCreationTemplateRequest) throws ServerException,
ValidationException, InvalidParameterException, TemplateNotFoundException, AwsServiceException, SdkClientException,
EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a repository creation template.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRepositoryCreationTemplateRequest.Builder}
* avoiding the need to create one manually via {@link DeleteRepositoryCreationTemplateRequest#builder()}
*
*
* @param deleteRepositoryCreationTemplateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DeleteRepositoryCreationTemplateRequest.Builder} to
* create a request.
* @return Result of the DeleteRepositoryCreationTemplate operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws TemplateNotFoundException
* The specified repository creation template can't be found. Verify the registry ID and prefix and try
* again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteRepositoryCreationTemplate
* @see AWS API Documentation
*/
default DeleteRepositoryCreationTemplateResponse deleteRepositoryCreationTemplate(
Consumer deleteRepositoryCreationTemplateRequest)
throws ServerException, ValidationException, InvalidParameterException, TemplateNotFoundException,
AwsServiceException, SdkClientException, EcrException {
return deleteRepositoryCreationTemplate(DeleteRepositoryCreationTemplateRequest.builder()
.applyMutation(deleteRepositoryCreationTemplateRequest).build());
}
/**
*
* Deletes the repository policy associated with the specified repository.
*
*
* @param deleteRepositoryPolicyRequest
* @return Result of the DeleteRepositoryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws RepositoryPolicyNotFoundException
* The specified repository and registry combination does not have an associated repository policy.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteRepositoryPolicy
* @see AWS
* API Documentation
*/
default DeleteRepositoryPolicyResponse deleteRepositoryPolicy(DeleteRepositoryPolicyRequest deleteRepositoryPolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, RepositoryPolicyNotFoundException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the repository policy associated with the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRepositoryPolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteRepositoryPolicyRequest#builder()}
*
*
* @param deleteRepositoryPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DeleteRepositoryPolicyRequest.Builder} to create a
* request.
* @return Result of the DeleteRepositoryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws RepositoryPolicyNotFoundException
* The specified repository and registry combination does not have an associated repository policy.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DeleteRepositoryPolicy
* @see AWS
* API Documentation
*/
default DeleteRepositoryPolicyResponse deleteRepositoryPolicy(
Consumer deleteRepositoryPolicyRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, RepositoryPolicyNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return deleteRepositoryPolicy(DeleteRepositoryPolicyRequest.builder().applyMutation(deleteRepositoryPolicyRequest)
.build());
}
/**
*
* Returns the replication status for a specified image.
*
*
* @param describeImageReplicationStatusRequest
* @return Result of the DescribeImageReplicationStatus operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImageReplicationStatus
* @see AWS API Documentation
*/
default DescribeImageReplicationStatusResponse describeImageReplicationStatus(
DescribeImageReplicationStatusRequest describeImageReplicationStatusRequest) throws ServerException,
InvalidParameterException, ImageNotFoundException, RepositoryNotFoundException, ValidationException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the replication status for a specified image.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImageReplicationStatusRequest.Builder}
* avoiding the need to create one manually via {@link DescribeImageReplicationStatusRequest#builder()}
*
*
* @param describeImageReplicationStatusRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribeImageReplicationStatusRequest.Builder} to create
* a request.
* @return Result of the DescribeImageReplicationStatus operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImageReplicationStatus
* @see AWS API Documentation
*/
default DescribeImageReplicationStatusResponse describeImageReplicationStatus(
Consumer describeImageReplicationStatusRequest)
throws ServerException, InvalidParameterException, ImageNotFoundException, RepositoryNotFoundException,
ValidationException, AwsServiceException, SdkClientException, EcrException {
return describeImageReplicationStatus(DescribeImageReplicationStatusRequest.builder()
.applyMutation(describeImageReplicationStatusRequest).build());
}
/**
*
* Returns the scan findings for the specified image.
*
*
* @param describeImageScanFindingsRequest
* @return Result of the DescribeImageScanFindings operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws ScanNotFoundException
* The specified image scan could not be found. Ensure that image scanning is enabled on the repository and
* try again.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImageScanFindings
* @see AWS
* API Documentation
*/
default DescribeImageScanFindingsResponse describeImageScanFindings(
DescribeImageScanFindingsRequest describeImageScanFindingsRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, ImageNotFoundException, ScanNotFoundException, ValidationException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the scan findings for the specified image.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImageScanFindingsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeImageScanFindingsRequest#builder()}
*
*
* @param describeImageScanFindingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest.Builder} to create a
* request.
* @return Result of the DescribeImageScanFindings operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws ScanNotFoundException
* The specified image scan could not be found. Ensure that image scanning is enabled on the repository and
* try again.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImageScanFindings
* @see AWS
* API Documentation
*/
default DescribeImageScanFindingsResponse describeImageScanFindings(
Consumer describeImageScanFindingsRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, ImageNotFoundException, ScanNotFoundException,
ValidationException, AwsServiceException, SdkClientException, EcrException {
return describeImageScanFindings(DescribeImageScanFindingsRequest.builder()
.applyMutation(describeImageScanFindingsRequest).build());
}
/**
*
* This is a variant of
* {@link #describeImageScanFindings(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsIterable responses = client.describeImageScanFindingsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsIterable responses = client
* .describeImageScanFindingsPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsIterable responses = client.describeImageScanFindingsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImageScanFindings(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest)}
* operation.
*
*
* @param describeImageScanFindingsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws ScanNotFoundException
* The specified image scan could not be found. Ensure that image scanning is enabled on the repository and
* try again.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImageScanFindings
* @see AWS
* API Documentation
*/
default DescribeImageScanFindingsIterable describeImageScanFindingsPaginator(
DescribeImageScanFindingsRequest describeImageScanFindingsRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, ImageNotFoundException, ScanNotFoundException, ValidationException, AwsServiceException,
SdkClientException, EcrException {
return new DescribeImageScanFindingsIterable(this, describeImageScanFindingsRequest);
}
/**
*
* This is a variant of
* {@link #describeImageScanFindings(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsIterable responses = client.describeImageScanFindingsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsIterable responses = client
* .describeImageScanFindingsPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImageScanFindingsIterable responses = client.describeImageScanFindingsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImageScanFindings(software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImageScanFindingsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeImageScanFindingsRequest#builder()}
*
*
* @param describeImageScanFindingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribeImageScanFindingsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws ScanNotFoundException
* The specified image scan could not be found. Ensure that image scanning is enabled on the repository and
* try again.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImageScanFindings
* @see AWS
* API Documentation
*/
default DescribeImageScanFindingsIterable describeImageScanFindingsPaginator(
Consumer describeImageScanFindingsRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, ImageNotFoundException, ScanNotFoundException,
ValidationException, AwsServiceException, SdkClientException, EcrException {
return describeImageScanFindingsPaginator(DescribeImageScanFindingsRequest.builder()
.applyMutation(describeImageScanFindingsRequest).build());
}
/**
*
* Returns metadata about the images in a repository.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* @param describeImagesRequest
* @return Result of the DescribeImages operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImages
* @see AWS API
* Documentation
*/
default DescribeImagesResponse describeImages(DescribeImagesRequest describeImagesRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, ImageNotFoundException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Returns metadata about the images in a repository.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImagesRequest.Builder} avoiding the need to
* create one manually via {@link DescribeImagesRequest#builder()}
*
*
* @param describeImagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribeImagesRequest.Builder} to create a request.
* @return Result of the DescribeImages operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImages
* @see AWS API
* Documentation
*/
default DescribeImagesResponse describeImages(Consumer describeImagesRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, ImageNotFoundException,
AwsServiceException, SdkClientException, EcrException {
return describeImages(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build());
}
/**
*
* This is a variant of {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesIterable responses = client.describeImagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesIterable responses = client.describeImagesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeImagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesIterable responses = client.describeImagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)} operation.
*
*
* @param describeImagesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImages
* @see AWS API
* Documentation
*/
default DescribeImagesIterable describeImagesPaginator(DescribeImagesRequest describeImagesRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, ImageNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return new DescribeImagesIterable(this, describeImagesRequest);
}
/**
*
* This is a variant of {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesIterable responses = client.describeImagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesIterable responses = client.describeImagesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeImagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesIterable responses = client.describeImagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImagesRequest.Builder} avoiding the need to
* create one manually via {@link DescribeImagesRequest#builder()}
*
*
* @param describeImagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribeImagesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeImages
* @see AWS API
* Documentation
*/
default DescribeImagesIterable describeImagesPaginator(Consumer describeImagesRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, ImageNotFoundException,
AwsServiceException, SdkClientException, EcrException {
return describeImagesPaginator(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build());
}
/**
*
* Returns the pull through cache rules for a registry.
*
*
* @param describePullThroughCacheRulesRequest
* @return Result of the DescribePullThroughCacheRules operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws PullThroughCacheRuleNotFoundException
* The pull through cache rule was not found. Specify a valid pull through cache rule and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribePullThroughCacheRules
* @see AWS API Documentation
*/
default DescribePullThroughCacheRulesResponse describePullThroughCacheRules(
DescribePullThroughCacheRulesRequest describePullThroughCacheRulesRequest) throws ServerException,
InvalidParameterException, ValidationException, PullThroughCacheRuleNotFoundException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the pull through cache rules for a registry.
*
*
*
* This is a convenience which creates an instance of the {@link DescribePullThroughCacheRulesRequest.Builder}
* avoiding the need to create one manually via {@link DescribePullThroughCacheRulesRequest#builder()}
*
*
* @param describePullThroughCacheRulesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest.Builder} to create a
* request.
* @return Result of the DescribePullThroughCacheRules operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws PullThroughCacheRuleNotFoundException
* The pull through cache rule was not found. Specify a valid pull through cache rule and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribePullThroughCacheRules
* @see AWS API Documentation
*/
default DescribePullThroughCacheRulesResponse describePullThroughCacheRules(
Consumer describePullThroughCacheRulesRequest) throws ServerException,
InvalidParameterException, ValidationException, PullThroughCacheRuleNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return describePullThroughCacheRules(DescribePullThroughCacheRulesRequest.builder()
.applyMutation(describePullThroughCacheRulesRequest).build());
}
/**
*
* This is a variant of
* {@link #describePullThroughCacheRules(software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesIterable responses = client.describePullThroughCacheRulesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesIterable responses = client
* .describePullThroughCacheRulesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesIterable responses = client.describePullThroughCacheRulesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describePullThroughCacheRules(software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest)}
* operation.
*
*
* @param describePullThroughCacheRulesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws PullThroughCacheRuleNotFoundException
* The pull through cache rule was not found. Specify a valid pull through cache rule and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribePullThroughCacheRules
* @see AWS API Documentation
*/
default DescribePullThroughCacheRulesIterable describePullThroughCacheRulesPaginator(
DescribePullThroughCacheRulesRequest describePullThroughCacheRulesRequest) throws ServerException,
InvalidParameterException, ValidationException, PullThroughCacheRuleNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return new DescribePullThroughCacheRulesIterable(this, describePullThroughCacheRulesRequest);
}
/**
*
* This is a variant of
* {@link #describePullThroughCacheRules(software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesIterable responses = client.describePullThroughCacheRulesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesIterable responses = client
* .describePullThroughCacheRulesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribePullThroughCacheRulesIterable responses = client.describePullThroughCacheRulesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describePullThroughCacheRules(software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribePullThroughCacheRulesRequest.Builder}
* avoiding the need to create one manually via {@link DescribePullThroughCacheRulesRequest#builder()}
*
*
* @param describePullThroughCacheRulesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribePullThroughCacheRulesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws PullThroughCacheRuleNotFoundException
* The pull through cache rule was not found. Specify a valid pull through cache rule and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribePullThroughCacheRules
* @see AWS API Documentation
*/
default DescribePullThroughCacheRulesIterable describePullThroughCacheRulesPaginator(
Consumer describePullThroughCacheRulesRequest) throws ServerException,
InvalidParameterException, ValidationException, PullThroughCacheRuleNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return describePullThroughCacheRulesPaginator(DescribePullThroughCacheRulesRequest.builder()
.applyMutation(describePullThroughCacheRulesRequest).build());
}
/**
*
* Describes the settings for a registry. The replication configuration for a repository can be created or updated
* with the PutReplicationConfiguration API action.
*
*
* @param describeRegistryRequest
* @return Result of the DescribeRegistry operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRegistry
* @see AWS API
* Documentation
*/
default DescribeRegistryResponse describeRegistry(DescribeRegistryRequest describeRegistryRequest) throws ServerException,
InvalidParameterException, ValidationException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the settings for a registry. The replication configuration for a repository can be created or updated
* with the PutReplicationConfiguration API action.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRegistryRequest.Builder} avoiding the need
* to create one manually via {@link DescribeRegistryRequest#builder()}
*
*
* @param describeRegistryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribeRegistryRequest.Builder} to create a request.
* @return Result of the DescribeRegistry operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRegistry
* @see AWS API
* Documentation
*/
default DescribeRegistryResponse describeRegistry(Consumer describeRegistryRequest)
throws ServerException, InvalidParameterException, ValidationException, AwsServiceException, SdkClientException,
EcrException {
return describeRegistry(DescribeRegistryRequest.builder().applyMutation(describeRegistryRequest).build());
}
/**
*
* Describes image repositories in a registry.
*
*
* @param describeRepositoriesRequest
* @return Result of the DescribeRepositories operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default DescribeRepositoriesResponse describeRepositories(DescribeRepositoriesRequest describeRepositoriesRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Describes image repositories in a registry.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRepositoriesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRepositoriesRequest#builder()}
*
*
* @param describeRepositoriesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest.Builder} to create a request.
* @return Result of the DescribeRepositories operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default DescribeRepositoriesResponse describeRepositories(
Consumer describeRepositoriesRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
return describeRepositories(DescribeRepositoriesRequest.builder().applyMutation(describeRepositoriesRequest).build());
}
/**
*
* Describes image repositories in a registry.
*
*
* @return Result of the DescribeRepositories operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositories
* @see #describeRepositories(DescribeRepositoriesRequest)
* @see AWS API
* Documentation
*/
default DescribeRepositoriesResponse describeRepositories() throws ServerException, InvalidParameterException,
RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
return describeRepositories(DescribeRepositoriesRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client.describeRepositoriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client
* .describeRepositoriesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client.describeRepositoriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositories
* @see #describeRepositoriesPaginator(DescribeRepositoriesRequest)
* @see AWS API
* Documentation
*/
default DescribeRepositoriesIterable describeRepositoriesPaginator() throws ServerException, InvalidParameterException,
RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
return describeRepositoriesPaginator(DescribeRepositoriesRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client.describeRepositoriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client
* .describeRepositoriesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client.describeRepositoriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)}
* operation.
*
*
* @param describeRepositoriesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default DescribeRepositoriesIterable describeRepositoriesPaginator(DescribeRepositoriesRequest describeRepositoriesRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return new DescribeRepositoriesIterable(this, describeRepositoriesRequest);
}
/**
*
* This is a variant of
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client.describeRepositoriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client
* .describeRepositoriesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesIterable responses = client.describeRepositoriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRepositoriesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRepositoriesRequest#builder()}
*
*
* @param describeRepositoriesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default DescribeRepositoriesIterable describeRepositoriesPaginator(
Consumer describeRepositoriesRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
return describeRepositoriesPaginator(DescribeRepositoriesRequest.builder().applyMutation(describeRepositoriesRequest)
.build());
}
/**
*
* Returns details about the repository creation templates in a registry. The prefixes
request
* parameter can be used to return the details for a specific repository creation template.
*
*
* @param describeRepositoryCreationTemplatesRequest
* @return Result of the DescribeRepositoryCreationTemplates operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositoryCreationTemplates
* @see AWS API Documentation
*/
default DescribeRepositoryCreationTemplatesResponse describeRepositoryCreationTemplates(
DescribeRepositoryCreationTemplatesRequest describeRepositoryCreationTemplatesRequest) throws ServerException,
ValidationException, InvalidParameterException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Returns details about the repository creation templates in a registry. The prefixes
request
* parameter can be used to return the details for a specific repository creation template.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRepositoryCreationTemplatesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeRepositoryCreationTemplatesRequest#builder()}
*
*
* @param describeRepositoryCreationTemplatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribeRepositoryCreationTemplatesRequest.Builder} to
* create a request.
* @return Result of the DescribeRepositoryCreationTemplates operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositoryCreationTemplates
* @see AWS API Documentation
*/
default DescribeRepositoryCreationTemplatesResponse describeRepositoryCreationTemplates(
Consumer describeRepositoryCreationTemplatesRequest)
throws ServerException, ValidationException, InvalidParameterException, AwsServiceException, SdkClientException,
EcrException {
return describeRepositoryCreationTemplates(DescribeRepositoryCreationTemplatesRequest.builder()
.applyMutation(describeRepositoryCreationTemplatesRequest).build());
}
/**
*
* This is a variant of
* {@link #describeRepositoryCreationTemplates(software.amazon.awssdk.services.ecr.model.DescribeRepositoryCreationTemplatesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoryCreationTemplatesIterable responses = client.describeRepositoryCreationTemplatesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoryCreationTemplatesIterable responses = client
* .describeRepositoryCreationTemplatesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeRepositoryCreationTemplatesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoryCreationTemplatesIterable responses = client.describeRepositoryCreationTemplatesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositoryCreationTemplates(software.amazon.awssdk.services.ecr.model.DescribeRepositoryCreationTemplatesRequest)}
* operation.
*
*
* @param describeRepositoryCreationTemplatesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositoryCreationTemplates
* @see AWS API Documentation
*/
default DescribeRepositoryCreationTemplatesIterable describeRepositoryCreationTemplatesPaginator(
DescribeRepositoryCreationTemplatesRequest describeRepositoryCreationTemplatesRequest) throws ServerException,
ValidationException, InvalidParameterException, AwsServiceException, SdkClientException, EcrException {
return new DescribeRepositoryCreationTemplatesIterable(this, describeRepositoryCreationTemplatesRequest);
}
/**
*
* This is a variant of
* {@link #describeRepositoryCreationTemplates(software.amazon.awssdk.services.ecr.model.DescribeRepositoryCreationTemplatesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoryCreationTemplatesIterable responses = client.describeRepositoryCreationTemplatesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoryCreationTemplatesIterable responses = client
* .describeRepositoryCreationTemplatesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.DescribeRepositoryCreationTemplatesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoryCreationTemplatesIterable responses = client.describeRepositoryCreationTemplatesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositoryCreationTemplates(software.amazon.awssdk.services.ecr.model.DescribeRepositoryCreationTemplatesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRepositoryCreationTemplatesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeRepositoryCreationTemplatesRequest#builder()}
*
*
* @param describeRepositoryCreationTemplatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.DescribeRepositoryCreationTemplatesRequest.Builder} to
* create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.DescribeRepositoryCreationTemplates
* @see AWS API Documentation
*/
default DescribeRepositoryCreationTemplatesIterable describeRepositoryCreationTemplatesPaginator(
Consumer describeRepositoryCreationTemplatesRequest)
throws ServerException, ValidationException, InvalidParameterException, AwsServiceException, SdkClientException,
EcrException {
return describeRepositoryCreationTemplatesPaginator(DescribeRepositoryCreationTemplatesRequest.builder()
.applyMutation(describeRepositoryCreationTemplatesRequest).build());
}
/**
*
* Retrieves the basic scan type version name.
*
*
* @param getAccountSettingRequest
* @return Result of the GetAccountSetting operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetAccountSetting
* @see AWS API
* Documentation
*/
default GetAccountSettingResponse getAccountSetting(GetAccountSettingRequest getAccountSettingRequest)
throws ServerException, ValidationException, InvalidParameterException, AwsServiceException, SdkClientException,
EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the basic scan type version name.
*
*
*
* This is a convenience which creates an instance of the {@link GetAccountSettingRequest.Builder} avoiding the need
* to create one manually via {@link GetAccountSettingRequest#builder()}
*
*
* @param getAccountSettingRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.GetAccountSettingRequest.Builder} to create a request.
* @return Result of the GetAccountSetting operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetAccountSetting
* @see AWS API
* Documentation
*/
default GetAccountSettingResponse getAccountSetting(Consumer getAccountSettingRequest)
throws ServerException, ValidationException, InvalidParameterException, AwsServiceException, SdkClientException,
EcrException {
return getAccountSetting(GetAccountSettingRequest.builder().applyMutation(getAccountSettingRequest).build());
}
/**
*
* Retrieves an authorization token. An authorization token represents your IAM authentication credentials and can
* be used to access any Amazon ECR registry that your IAM principal has access to. The authorization token is valid
* for 12 hours.
*
*
* The authorizationToken
returned is a base64 encoded string that can be decoded and used in a
* docker login
command to authenticate to a registry. The CLI offers an
* get-login-password
command that simplifies the login process. For more information, see Registry
* authentication in the Amazon Elastic Container Registry User Guide.
*
*
* @param getAuthorizationTokenRequest
* @return Result of the GetAuthorizationToken operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetAuthorizationToken
* @see AWS API
* Documentation
*/
default GetAuthorizationTokenResponse getAuthorizationToken(GetAuthorizationTokenRequest getAuthorizationTokenRequest)
throws ServerException, InvalidParameterException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves an authorization token. An authorization token represents your IAM authentication credentials and can
* be used to access any Amazon ECR registry that your IAM principal has access to. The authorization token is valid
* for 12 hours.
*
*
* The authorizationToken
returned is a base64 encoded string that can be decoded and used in a
* docker login
command to authenticate to a registry. The CLI offers an
* get-login-password
command that simplifies the login process. For more information, see Registry
* authentication in the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetAuthorizationTokenRequest.Builder} avoiding the
* need to create one manually via {@link GetAuthorizationTokenRequest#builder()}
*
*
* @param getAuthorizationTokenRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.GetAuthorizationTokenRequest.Builder} to create a
* request.
* @return Result of the GetAuthorizationToken operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetAuthorizationToken
* @see AWS API
* Documentation
*/
default GetAuthorizationTokenResponse getAuthorizationToken(
Consumer getAuthorizationTokenRequest) throws ServerException,
InvalidParameterException, AwsServiceException, SdkClientException, EcrException {
return getAuthorizationToken(GetAuthorizationTokenRequest.builder().applyMutation(getAuthorizationTokenRequest).build());
}
/**
*
* Retrieves an authorization token. An authorization token represents your IAM authentication credentials and can
* be used to access any Amazon ECR registry that your IAM principal has access to. The authorization token is valid
* for 12 hours.
*
*
* The authorizationToken
returned is a base64 encoded string that can be decoded and used in a
* docker login
command to authenticate to a registry. The CLI offers an
* get-login-password
command that simplifies the login process. For more information, see Registry
* authentication in the Amazon Elastic Container Registry User Guide.
*
*
* @return Result of the GetAuthorizationToken operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetAuthorizationToken
* @see #getAuthorizationToken(GetAuthorizationTokenRequest)
* @see AWS API
* Documentation
*/
default GetAuthorizationTokenResponse getAuthorizationToken() throws ServerException, InvalidParameterException,
AwsServiceException, SdkClientException, EcrException {
return getAuthorizationToken(GetAuthorizationTokenRequest.builder().build());
}
/**
*
* Retrieves the pre-signed Amazon S3 download URL corresponding to an image layer. You can only get URLs for image
* layers that are referenced in an image.
*
*
* When an image is pulled, the GetDownloadUrlForLayer API is called once per image layer that is not already
* cached.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param getDownloadUrlForLayerRequest
* @return Result of the GetDownloadUrlForLayer operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws LayersNotFoundException
* The specified layers could not be found, or the specified layer is not valid for this repository.
* @throws LayerInaccessibleException
* The specified layer is not available because it is not associated with an image. Unassociated image
* layers may be cleaned up at any time.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws UnableToGetUpstreamLayerException
* There was an issue getting the upstream layer matching the pull through cache rule.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetDownloadUrlForLayer
* @see AWS
* API Documentation
*/
default GetDownloadUrlForLayerResponse getDownloadUrlForLayer(GetDownloadUrlForLayerRequest getDownloadUrlForLayerRequest)
throws ServerException, InvalidParameterException, LayersNotFoundException, LayerInaccessibleException,
RepositoryNotFoundException, UnableToGetUpstreamLayerException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the pre-signed Amazon S3 download URL corresponding to an image layer. You can only get URLs for image
* layers that are referenced in an image.
*
*
* When an image is pulled, the GetDownloadUrlForLayer API is called once per image layer that is not already
* cached.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link GetDownloadUrlForLayerRequest.Builder} avoiding the
* need to create one manually via {@link GetDownloadUrlForLayerRequest#builder()}
*
*
* @param getDownloadUrlForLayerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.GetDownloadUrlForLayerRequest.Builder} to create a
* request.
* @return Result of the GetDownloadUrlForLayer operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws LayersNotFoundException
* The specified layers could not be found, or the specified layer is not valid for this repository.
* @throws LayerInaccessibleException
* The specified layer is not available because it is not associated with an image. Unassociated image
* layers may be cleaned up at any time.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws UnableToGetUpstreamLayerException
* There was an issue getting the upstream layer matching the pull through cache rule.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetDownloadUrlForLayer
* @see AWS
* API Documentation
*/
default GetDownloadUrlForLayerResponse getDownloadUrlForLayer(
Consumer getDownloadUrlForLayerRequest) throws ServerException,
InvalidParameterException, LayersNotFoundException, LayerInaccessibleException, RepositoryNotFoundException,
UnableToGetUpstreamLayerException, AwsServiceException, SdkClientException, EcrException {
return getDownloadUrlForLayer(GetDownloadUrlForLayerRequest.builder().applyMutation(getDownloadUrlForLayerRequest)
.build());
}
/**
*
* Retrieves the lifecycle policy for the specified repository.
*
*
* @param getLifecyclePolicyRequest
* @return Result of the GetLifecyclePolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyNotFoundException
* The lifecycle policy could not be found, and no policy is set to the repository.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetLifecyclePolicy
* @see AWS API
* Documentation
*/
default GetLifecyclePolicyResponse getLifecyclePolicy(GetLifecyclePolicyRequest getLifecyclePolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, LifecyclePolicyNotFoundException,
ValidationException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the lifecycle policy for the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link GetLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetLifecyclePolicyRequest#builder()}
*
*
* @param getLifecyclePolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyRequest.Builder} to create a request.
* @return Result of the GetLifecyclePolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyNotFoundException
* The lifecycle policy could not be found, and no policy is set to the repository.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetLifecyclePolicy
* @see AWS API
* Documentation
*/
default GetLifecyclePolicyResponse getLifecyclePolicy(Consumer getLifecyclePolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, LifecyclePolicyNotFoundException,
ValidationException, AwsServiceException, SdkClientException, EcrException {
return getLifecyclePolicy(GetLifecyclePolicyRequest.builder().applyMutation(getLifecyclePolicyRequest).build());
}
/**
*
* Retrieves the results of the lifecycle policy preview request for the specified repository.
*
*
* @param getLifecyclePolicyPreviewRequest
* @return Result of the GetLifecyclePolicyPreview operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyPreviewNotFoundException
* There is no dry run for this repository.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
default GetLifecyclePolicyPreviewResponse getLifecyclePolicyPreview(
GetLifecyclePolicyPreviewRequest getLifecyclePolicyPreviewRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, LifecyclePolicyPreviewNotFoundException, ValidationException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the results of the lifecycle policy preview request for the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link GetLifecyclePolicyPreviewRequest.Builder} avoiding
* the need to create one manually via {@link GetLifecyclePolicyPreviewRequest#builder()}
*
*
* @param getLifecyclePolicyPreviewRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest.Builder} to create a
* request.
* @return Result of the GetLifecyclePolicyPreview operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyPreviewNotFoundException
* There is no dry run for this repository.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
default GetLifecyclePolicyPreviewResponse getLifecyclePolicyPreview(
Consumer getLifecyclePolicyPreviewRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, LifecyclePolicyPreviewNotFoundException, ValidationException,
AwsServiceException, SdkClientException, EcrException {
return getLifecyclePolicyPreview(GetLifecyclePolicyPreviewRequest.builder()
.applyMutation(getLifecyclePolicyPreviewRequest).build());
}
/**
*
* This is a variant of
* {@link #getLifecyclePolicyPreview(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewIterable responses = client.getLifecyclePolicyPreviewPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewIterable responses = client
* .getLifecyclePolicyPreviewPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewIterable responses = client.getLifecyclePolicyPreviewPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLifecyclePolicyPreview(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest)}
* operation.
*
*
* @param getLifecyclePolicyPreviewRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyPreviewNotFoundException
* There is no dry run for this repository.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
default GetLifecyclePolicyPreviewIterable getLifecyclePolicyPreviewPaginator(
GetLifecyclePolicyPreviewRequest getLifecyclePolicyPreviewRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, LifecyclePolicyPreviewNotFoundException, ValidationException, AwsServiceException,
SdkClientException, EcrException {
return new GetLifecyclePolicyPreviewIterable(this, getLifecyclePolicyPreviewRequest);
}
/**
*
* This is a variant of
* {@link #getLifecyclePolicyPreview(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewIterable responses = client.getLifecyclePolicyPreviewPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewIterable responses = client
* .getLifecyclePolicyPreviewPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.GetLifecyclePolicyPreviewIterable responses = client.getLifecyclePolicyPreviewPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLifecyclePolicyPreview(software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetLifecyclePolicyPreviewRequest.Builder} avoiding
* the need to create one manually via {@link GetLifecyclePolicyPreviewRequest#builder()}
*
*
* @param getLifecyclePolicyPreviewRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyPreviewNotFoundException
* There is no dry run for this repository.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
default GetLifecyclePolicyPreviewIterable getLifecyclePolicyPreviewPaginator(
Consumer getLifecyclePolicyPreviewRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, LifecyclePolicyPreviewNotFoundException, ValidationException,
AwsServiceException, SdkClientException, EcrException {
return getLifecyclePolicyPreviewPaginator(GetLifecyclePolicyPreviewRequest.builder()
.applyMutation(getLifecyclePolicyPreviewRequest).build());
}
/**
*
* Retrieves the permissions policy for a registry.
*
*
* @param getRegistryPolicyRequest
* @return Result of the GetRegistryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RegistryPolicyNotFoundException
* The registry doesn't have an associated registry policy.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetRegistryPolicy
* @see AWS API
* Documentation
*/
default GetRegistryPolicyResponse getRegistryPolicy(GetRegistryPolicyRequest getRegistryPolicyRequest)
throws ServerException, InvalidParameterException, RegistryPolicyNotFoundException, ValidationException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the permissions policy for a registry.
*
*
*
* This is a convenience which creates an instance of the {@link GetRegistryPolicyRequest.Builder} avoiding the need
* to create one manually via {@link GetRegistryPolicyRequest#builder()}
*
*
* @param getRegistryPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.GetRegistryPolicyRequest.Builder} to create a request.
* @return Result of the GetRegistryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RegistryPolicyNotFoundException
* The registry doesn't have an associated registry policy.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetRegistryPolicy
* @see AWS API
* Documentation
*/
default GetRegistryPolicyResponse getRegistryPolicy(Consumer getRegistryPolicyRequest)
throws ServerException, InvalidParameterException, RegistryPolicyNotFoundException, ValidationException,
AwsServiceException, SdkClientException, EcrException {
return getRegistryPolicy(GetRegistryPolicyRequest.builder().applyMutation(getRegistryPolicyRequest).build());
}
/**
*
* Retrieves the scanning configuration for a registry.
*
*
* @param getRegistryScanningConfigurationRequest
* @return Result of the GetRegistryScanningConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetRegistryScanningConfiguration
* @see AWS API Documentation
*/
default GetRegistryScanningConfigurationResponse getRegistryScanningConfiguration(
GetRegistryScanningConfigurationRequest getRegistryScanningConfigurationRequest) throws ServerException,
InvalidParameterException, ValidationException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the scanning configuration for a registry.
*
*
*
* This is a convenience which creates an instance of the {@link GetRegistryScanningConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link GetRegistryScanningConfigurationRequest#builder()}
*
*
* @param getRegistryScanningConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.GetRegistryScanningConfigurationRequest.Builder} to
* create a request.
* @return Result of the GetRegistryScanningConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetRegistryScanningConfiguration
* @see AWS API Documentation
*/
default GetRegistryScanningConfigurationResponse getRegistryScanningConfiguration(
Consumer getRegistryScanningConfigurationRequest)
throws ServerException, InvalidParameterException, ValidationException, AwsServiceException, SdkClientException,
EcrException {
return getRegistryScanningConfiguration(GetRegistryScanningConfigurationRequest.builder()
.applyMutation(getRegistryScanningConfigurationRequest).build());
}
/**
*
* Retrieves the repository policy for the specified repository.
*
*
* @param getRepositoryPolicyRequest
* @return Result of the GetRepositoryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws RepositoryPolicyNotFoundException
* The specified repository and registry combination does not have an associated repository policy.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetRepositoryPolicy
* @see AWS API
* Documentation
*/
default GetRepositoryPolicyResponse getRepositoryPolicy(GetRepositoryPolicyRequest getRepositoryPolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, RepositoryPolicyNotFoundException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the repository policy for the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link GetRepositoryPolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetRepositoryPolicyRequest#builder()}
*
*
* @param getRepositoryPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.GetRepositoryPolicyRequest.Builder} to create a request.
* @return Result of the GetRepositoryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws RepositoryPolicyNotFoundException
* The specified repository and registry combination does not have an associated repository policy.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.GetRepositoryPolicy
* @see AWS API
* Documentation
*/
default GetRepositoryPolicyResponse getRepositoryPolicy(
Consumer getRepositoryPolicyRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, RepositoryPolicyNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return getRepositoryPolicy(GetRepositoryPolicyRequest.builder().applyMutation(getRepositoryPolicyRequest).build());
}
/**
*
* Notifies Amazon ECR that you intend to upload an image layer.
*
*
* When an image is pushed, the InitiateLayerUpload API is called once per image layer that has not already been
* uploaded. Whether or not an image layer has been uploaded is determined by the BatchCheckLayerAvailability API
* action.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param initiateLayerUploadRequest
* @return Result of the InitiateLayerUpload operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.InitiateLayerUpload
* @see AWS API
* Documentation
*/
default InitiateLayerUploadResponse initiateLayerUpload(InitiateLayerUploadRequest initiateLayerUploadRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, KmsException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Notifies Amazon ECR that you intend to upload an image layer.
*
*
* When an image is pushed, the InitiateLayerUpload API is called once per image layer that has not already been
* uploaded. Whether or not an image layer has been uploaded is determined by the BatchCheckLayerAvailability API
* action.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link InitiateLayerUploadRequest.Builder} avoiding the
* need to create one manually via {@link InitiateLayerUploadRequest#builder()}
*
*
* @param initiateLayerUploadRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.InitiateLayerUploadRequest.Builder} to create a request.
* @return Result of the InitiateLayerUpload operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.InitiateLayerUpload
* @see AWS API
* Documentation
*/
default InitiateLayerUploadResponse initiateLayerUpload(
Consumer initiateLayerUploadRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, KmsException, AwsServiceException, SdkClientException,
EcrException {
return initiateLayerUpload(InitiateLayerUploadRequest.builder().applyMutation(initiateLayerUploadRequest).build());
}
/**
*
* Lists all the image IDs for the specified repository.
*
*
* You can filter images based on whether or not they are tagged by using the tagStatus
filter and
* specifying either TAGGED
, UNTAGGED
or ANY
. For example, you can filter
* your results to return only UNTAGGED
images and then pipe that result to a BatchDeleteImage
* operation to delete them. Or, you can filter your results to return only TAGGED
images to list all
* of the tags in your repository.
*
*
* @param listImagesRequest
* @return Result of the ListImages operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.ListImages
* @see AWS API
* Documentation
*/
default ListImagesResponse listImages(ListImagesRequest listImagesRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the image IDs for the specified repository.
*
*
* You can filter images based on whether or not they are tagged by using the tagStatus
filter and
* specifying either TAGGED
, UNTAGGED
or ANY
. For example, you can filter
* your results to return only UNTAGGED
images and then pipe that result to a BatchDeleteImage
* operation to delete them. Or, you can filter your results to return only TAGGED
images to list all
* of the tags in your repository.
*
*
*
* This is a convenience which creates an instance of the {@link ListImagesRequest.Builder} avoiding the need to
* create one manually via {@link ListImagesRequest#builder()}
*
*
* @param listImagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.ListImagesRequest.Builder} to create a request.
* @return Result of the ListImages operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.ListImages
* @see AWS API
* Documentation
*/
default ListImagesResponse listImages(Consumer listImagesRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
return listImages(ListImagesRequest.builder().applyMutation(listImagesRequest).build());
}
/**
*
* This is a variant of {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesIterable responses = client.listImagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.ListImagesIterable responses = client.listImagesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.ListImagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesIterable responses = client.listImagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
*
*
* @param listImagesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.ListImages
* @see AWS API
* Documentation
*/
default ListImagesIterable listImagesPaginator(ListImagesRequest listImagesRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
return new ListImagesIterable(this, listImagesRequest);
}
/**
*
* This is a variant of {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesIterable responses = client.listImagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ecr.paginators.ListImagesIterable responses = client.listImagesPaginator(request);
* for (software.amazon.awssdk.services.ecr.model.ListImagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesIterable responses = client.listImagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListImagesRequest.Builder} avoiding the need to
* create one manually via {@link ListImagesRequest#builder()}
*
*
* @param listImagesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.ListImagesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.ListImages
* @see AWS API
* Documentation
*/
default ListImagesIterable listImagesPaginator(Consumer listImagesRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
return listImagesPaginator(ListImagesRequest.builder().applyMutation(listImagesRequest).build());
}
/**
*
* List the tags for an Amazon ECR resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws InvalidParameterException, RepositoryNotFoundException, ServerException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* List the tags for an Amazon ECR resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.ListTagsForResourceRequest.Builder} to create a request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws InvalidParameterException,
RepositoryNotFoundException, ServerException, AwsServiceException, SdkClientException, EcrException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Allows you to change the basic scan type version by setting the name
parameter to either
* CLAIR
to AWS_NATIVE
.
*
*
* @param putAccountSettingRequest
* @return Result of the PutAccountSetting operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutAccountSetting
* @see AWS API
* Documentation
*/
default PutAccountSettingResponse putAccountSetting(PutAccountSettingRequest putAccountSettingRequest)
throws ServerException, ValidationException, InvalidParameterException, LimitExceededException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Allows you to change the basic scan type version by setting the name
parameter to either
* CLAIR
to AWS_NATIVE
.
*
*
*
* This is a convenience which creates an instance of the {@link PutAccountSettingRequest.Builder} avoiding the need
* to create one manually via {@link PutAccountSettingRequest#builder()}
*
*
* @param putAccountSettingRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.PutAccountSettingRequest.Builder} to create a request.
* @return Result of the PutAccountSetting operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutAccountSetting
* @see AWS API
* Documentation
*/
default PutAccountSettingResponse putAccountSetting(Consumer putAccountSettingRequest)
throws ServerException, ValidationException, InvalidParameterException, LimitExceededException, AwsServiceException,
SdkClientException, EcrException {
return putAccountSetting(PutAccountSettingRequest.builder().applyMutation(putAccountSettingRequest).build());
}
/**
*
* Creates or updates the image manifest and tags associated with an image.
*
*
* When an image is pushed and all new image layers have been uploaded, the PutImage API is called once to create or
* update the image manifest and the tags associated with the image.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param putImageRequest
* @return Result of the PutImage operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageAlreadyExistsException
* The specified image has already been pushed, and there were no changes to the manifest or image tag after
* the last push.
* @throws LayersNotFoundException
* The specified layers could not be found, or the specified layer is not valid for this repository.
* @throws ReferencedImagesNotFoundException
* The manifest list is referencing an image that does not exist.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws ImageTagAlreadyExistsException
* The specified image is tagged with a tag that already exists. The repository is configured for tag
* immutability.
* @throws ImageDigestDoesNotMatchException
* The specified image digest does not match the digest that Amazon ECR calculated for the image.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutImage
* @see AWS API
* Documentation
*/
default PutImageResponse putImage(PutImageRequest putImageRequest) throws ServerException, InvalidParameterException,
RepositoryNotFoundException, ImageAlreadyExistsException, LayersNotFoundException, ReferencedImagesNotFoundException,
LimitExceededException, ImageTagAlreadyExistsException, ImageDigestDoesNotMatchException, KmsException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the image manifest and tags associated with an image.
*
*
* When an image is pushed and all new image layers have been uploaded, the PutImage API is called once to create or
* update the image manifest and the tags associated with the image.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link PutImageRequest.Builder} avoiding the need to
* create one manually via {@link PutImageRequest#builder()}
*
*
* @param putImageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.PutImageRequest.Builder} to create a request.
* @return Result of the PutImage operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageAlreadyExistsException
* The specified image has already been pushed, and there were no changes to the manifest or image tag after
* the last push.
* @throws LayersNotFoundException
* The specified layers could not be found, or the specified layer is not valid for this repository.
* @throws ReferencedImagesNotFoundException
* The manifest list is referencing an image that does not exist.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws ImageTagAlreadyExistsException
* The specified image is tagged with a tag that already exists. The repository is configured for tag
* immutability.
* @throws ImageDigestDoesNotMatchException
* The specified image digest does not match the digest that Amazon ECR calculated for the image.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutImage
* @see AWS API
* Documentation
*/
default PutImageResponse putImage(Consumer putImageRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, ImageAlreadyExistsException, LayersNotFoundException,
ReferencedImagesNotFoundException, LimitExceededException, ImageTagAlreadyExistsException,
ImageDigestDoesNotMatchException, KmsException, AwsServiceException, SdkClientException, EcrException {
return putImage(PutImageRequest.builder().applyMutation(putImageRequest).build());
}
/**
*
*
* The PutImageScanningConfiguration
API is being deprecated, in favor of specifying the image scanning
* configuration at the registry level. For more information, see PutRegistryScanningConfiguration.
*
*
*
* Updates the image scanning configuration for the specified repository.
*
*
* @param putImageScanningConfigurationRequest
* @return Result of the PutImageScanningConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutImageScanningConfiguration
* @see AWS API Documentation
*/
default PutImageScanningConfigurationResponse putImageScanningConfiguration(
PutImageScanningConfigurationRequest putImageScanningConfigurationRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, ValidationException, AwsServiceException, SdkClientException,
EcrException {
throw new UnsupportedOperationException();
}
/**
*
*
* The PutImageScanningConfiguration
API is being deprecated, in favor of specifying the image scanning
* configuration at the registry level. For more information, see PutRegistryScanningConfiguration.
*
*
*
* Updates the image scanning configuration for the specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link PutImageScanningConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link PutImageScanningConfigurationRequest#builder()}
*
*
* @param putImageScanningConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.PutImageScanningConfigurationRequest.Builder} to create a
* request.
* @return Result of the PutImageScanningConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutImageScanningConfiguration
* @see AWS API Documentation
*/
default PutImageScanningConfigurationResponse putImageScanningConfiguration(
Consumer putImageScanningConfigurationRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, ValidationException, AwsServiceException, SdkClientException,
EcrException {
return putImageScanningConfiguration(PutImageScanningConfigurationRequest.builder()
.applyMutation(putImageScanningConfigurationRequest).build());
}
/**
*
* Updates the image tag mutability settings for the specified repository. For more information, see Image tag mutability
* in the Amazon Elastic Container Registry User Guide.
*
*
* @param putImageTagMutabilityRequest
* @return Result of the PutImageTagMutability operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutImageTagMutability
* @see AWS API
* Documentation
*/
default PutImageTagMutabilityResponse putImageTagMutability(PutImageTagMutabilityRequest putImageTagMutabilityRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the image tag mutability settings for the specified repository. For more information, see Image tag mutability
* in the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutImageTagMutabilityRequest.Builder} avoiding the
* need to create one manually via {@link PutImageTagMutabilityRequest#builder()}
*
*
* @param putImageTagMutabilityRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.PutImageTagMutabilityRequest.Builder} to create a
* request.
* @return Result of the PutImageTagMutability operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutImageTagMutability
* @see AWS API
* Documentation
*/
default PutImageTagMutabilityResponse putImageTagMutability(
Consumer putImageTagMutabilityRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
return putImageTagMutability(PutImageTagMutabilityRequest.builder().applyMutation(putImageTagMutabilityRequest).build());
}
/**
*
* Creates or updates the lifecycle policy for the specified repository. For more information, see Lifecycle policy
* template.
*
*
* @param putLifecyclePolicyRequest
* @return Result of the PutLifecyclePolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutLifecyclePolicy
* @see AWS API
* Documentation
*/
default PutLifecyclePolicyResponse putLifecyclePolicy(PutLifecyclePolicyRequest putLifecyclePolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, ValidationException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the lifecycle policy for the specified repository. For more information, see Lifecycle policy
* template.
*
*
*
* This is a convenience which creates an instance of the {@link PutLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link PutLifecyclePolicyRequest#builder()}
*
*
* @param putLifecyclePolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.PutLifecyclePolicyRequest.Builder} to create a request.
* @return Result of the PutLifecyclePolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutLifecyclePolicy
* @see AWS API
* Documentation
*/
default PutLifecyclePolicyResponse putLifecyclePolicy(Consumer putLifecyclePolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, ValidationException,
AwsServiceException, SdkClientException, EcrException {
return putLifecyclePolicy(PutLifecyclePolicyRequest.builder().applyMutation(putLifecyclePolicyRequest).build());
}
/**
*
* Creates or updates the permissions policy for your registry.
*
*
* A registry policy is used to specify permissions for another Amazon Web Services account and is used when
* configuring cross-account replication. For more information, see Registry permissions
* in the Amazon Elastic Container Registry User Guide.
*
*
* @param putRegistryPolicyRequest
* @return Result of the PutRegistryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutRegistryPolicy
* @see AWS API
* Documentation
*/
default PutRegistryPolicyResponse putRegistryPolicy(PutRegistryPolicyRequest putRegistryPolicyRequest)
throws ServerException, InvalidParameterException, ValidationException, AwsServiceException, SdkClientException,
EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the permissions policy for your registry.
*
*
* A registry policy is used to specify permissions for another Amazon Web Services account and is used when
* configuring cross-account replication. For more information, see Registry permissions
* in the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutRegistryPolicyRequest.Builder} avoiding the need
* to create one manually via {@link PutRegistryPolicyRequest#builder()}
*
*
* @param putRegistryPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.PutRegistryPolicyRequest.Builder} to create a request.
* @return Result of the PutRegistryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutRegistryPolicy
* @see AWS API
* Documentation
*/
default PutRegistryPolicyResponse putRegistryPolicy(Consumer putRegistryPolicyRequest)
throws ServerException, InvalidParameterException, ValidationException, AwsServiceException, SdkClientException,
EcrException {
return putRegistryPolicy(PutRegistryPolicyRequest.builder().applyMutation(putRegistryPolicyRequest).build());
}
/**
*
* Creates or updates the scanning configuration for your private registry.
*
*
* @param putRegistryScanningConfigurationRequest
* @return Result of the PutRegistryScanningConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutRegistryScanningConfiguration
* @see AWS API Documentation
*/
default PutRegistryScanningConfigurationResponse putRegistryScanningConfiguration(
PutRegistryScanningConfigurationRequest putRegistryScanningConfigurationRequest) throws ServerException,
InvalidParameterException, ValidationException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the scanning configuration for your private registry.
*
*
*
* This is a convenience which creates an instance of the {@link PutRegistryScanningConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link PutRegistryScanningConfigurationRequest#builder()}
*
*
* @param putRegistryScanningConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.PutRegistryScanningConfigurationRequest.Builder} to
* create a request.
* @return Result of the PutRegistryScanningConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutRegistryScanningConfiguration
* @see AWS API Documentation
*/
default PutRegistryScanningConfigurationResponse putRegistryScanningConfiguration(
Consumer putRegistryScanningConfigurationRequest)
throws ServerException, InvalidParameterException, ValidationException, AwsServiceException, SdkClientException,
EcrException {
return putRegistryScanningConfiguration(PutRegistryScanningConfigurationRequest.builder()
.applyMutation(putRegistryScanningConfigurationRequest).build());
}
/**
*
* Creates or updates the replication configuration for a registry. The existing replication configuration for a
* repository can be retrieved with the DescribeRegistry API action. The first time the
* PutReplicationConfiguration API is called, a service-linked IAM role is created in your account for the
* replication process. For more information, see Using
* service-linked roles for Amazon ECR in the Amazon Elastic Container Registry User Guide. For more
* information on the custom role for replication, see Creating an IAM role for replication.
*
*
*
* When configuring cross-account replication, the destination account must grant the source account permission to
* replicate. This permission is controlled using a registry permissions policy. For more information, see
* PutRegistryPolicy.
*
*
*
* @param putReplicationConfigurationRequest
* @return Result of the PutReplicationConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutReplicationConfiguration
* @see AWS API Documentation
*/
default PutReplicationConfigurationResponse putReplicationConfiguration(
PutReplicationConfigurationRequest putReplicationConfigurationRequest) throws ServerException,
InvalidParameterException, ValidationException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the replication configuration for a registry. The existing replication configuration for a
* repository can be retrieved with the DescribeRegistry API action. The first time the
* PutReplicationConfiguration API is called, a service-linked IAM role is created in your account for the
* replication process. For more information, see Using
* service-linked roles for Amazon ECR in the Amazon Elastic Container Registry User Guide. For more
* information on the custom role for replication, see Creating an IAM role for replication.
*
*
*
* When configuring cross-account replication, the destination account must grant the source account permission to
* replicate. This permission is controlled using a registry permissions policy. For more information, see
* PutRegistryPolicy.
*
*
*
* This is a convenience which creates an instance of the {@link PutReplicationConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link PutReplicationConfigurationRequest#builder()}
*
*
* @param putReplicationConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.PutReplicationConfigurationRequest.Builder} to create a
* request.
* @return Result of the PutReplicationConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.PutReplicationConfiguration
* @see AWS API Documentation
*/
default PutReplicationConfigurationResponse putReplicationConfiguration(
Consumer putReplicationConfigurationRequest) throws ServerException,
InvalidParameterException, ValidationException, AwsServiceException, SdkClientException, EcrException {
return putReplicationConfiguration(PutReplicationConfigurationRequest.builder()
.applyMutation(putReplicationConfigurationRequest).build());
}
/**
*
* Applies a repository policy to the specified repository to control access permissions. For more information, see
* Amazon ECR Repository
* policies in the Amazon Elastic Container Registry User Guide.
*
*
* @param setRepositoryPolicyRequest
* @return Result of the SetRepositoryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.SetRepositoryPolicy
* @see AWS API
* Documentation
*/
default SetRepositoryPolicyResponse setRepositoryPolicy(SetRepositoryPolicyRequest setRepositoryPolicyRequest)
throws ServerException, InvalidParameterException, RepositoryNotFoundException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Applies a repository policy to the specified repository to control access permissions. For more information, see
* Amazon ECR Repository
* policies in the Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link SetRepositoryPolicyRequest.Builder} avoiding the
* need to create one manually via {@link SetRepositoryPolicyRequest#builder()}
*
*
* @param setRepositoryPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.SetRepositoryPolicyRequest.Builder} to create a request.
* @return Result of the SetRepositoryPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.SetRepositoryPolicy
* @see AWS API
* Documentation
*/
default SetRepositoryPolicyResponse setRepositoryPolicy(
Consumer setRepositoryPolicyRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, AwsServiceException, SdkClientException, EcrException {
return setRepositoryPolicy(SetRepositoryPolicyRequest.builder().applyMutation(setRepositoryPolicyRequest).build());
}
/**
*
* Starts an image vulnerability scan. An image scan can only be started once per 24 hours on an individual image.
* This limit includes if an image was scanned on initial push. For more information, see Image scanning in the
* Amazon Elastic Container Registry User Guide.
*
*
* @param startImageScanRequest
* @return Result of the StartImageScan operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws UnsupportedImageTypeException
* The image is of a type that cannot be scanned.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.StartImageScan
* @see AWS API
* Documentation
*/
default StartImageScanResponse startImageScan(StartImageScanRequest startImageScanRequest) throws ServerException,
InvalidParameterException, UnsupportedImageTypeException, LimitExceededException, RepositoryNotFoundException,
ImageNotFoundException, ValidationException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Starts an image vulnerability scan. An image scan can only be started once per 24 hours on an individual image.
* This limit includes if an image was scanned on initial push. For more information, see Image scanning in the
* Amazon Elastic Container Registry User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link StartImageScanRequest.Builder} avoiding the need to
* create one manually via {@link StartImageScanRequest#builder()}
*
*
* @param startImageScanRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.StartImageScanRequest.Builder} to create a request.
* @return Result of the StartImageScan operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws UnsupportedImageTypeException
* The image is of a type that cannot be scanned.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ImageNotFoundException
* The image requested does not exist in the specified repository.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.StartImageScan
* @see AWS API
* Documentation
*/
default StartImageScanResponse startImageScan(Consumer startImageScanRequest)
throws ServerException, InvalidParameterException, UnsupportedImageTypeException, LimitExceededException,
RepositoryNotFoundException, ImageNotFoundException, ValidationException, AwsServiceException, SdkClientException,
EcrException {
return startImageScan(StartImageScanRequest.builder().applyMutation(startImageScanRequest).build());
}
/**
*
* Starts a preview of a lifecycle policy for the specified repository. This allows you to see the results before
* associating the lifecycle policy with the repository.
*
*
* @param startLifecyclePolicyPreviewRequest
* @return Result of the StartLifecyclePolicyPreview operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyNotFoundException
* The lifecycle policy could not be found, and no policy is set to the repository.
* @throws LifecyclePolicyPreviewInProgressException
* The previous lifecycle policy preview request has not completed. Wait and try again.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.StartLifecyclePolicyPreview
* @see AWS API Documentation
*/
default StartLifecyclePolicyPreviewResponse startLifecyclePolicyPreview(
StartLifecyclePolicyPreviewRequest startLifecyclePolicyPreviewRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, LifecyclePolicyNotFoundException,
LifecyclePolicyPreviewInProgressException, ValidationException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Starts a preview of a lifecycle policy for the specified repository. This allows you to see the results before
* associating the lifecycle policy with the repository.
*
*
*
* This is a convenience which creates an instance of the {@link StartLifecyclePolicyPreviewRequest.Builder}
* avoiding the need to create one manually via {@link StartLifecyclePolicyPreviewRequest#builder()}
*
*
* @param startLifecyclePolicyPreviewRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.StartLifecyclePolicyPreviewRequest.Builder} to create a
* request.
* @return Result of the StartLifecyclePolicyPreview operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws LifecyclePolicyNotFoundException
* The lifecycle policy could not be found, and no policy is set to the repository.
* @throws LifecyclePolicyPreviewInProgressException
* The previous lifecycle policy preview request has not completed. Wait and try again.
* @throws ValidationException
* There was an exception validating this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.StartLifecyclePolicyPreview
* @see AWS API Documentation
*/
default StartLifecyclePolicyPreviewResponse startLifecyclePolicyPreview(
Consumer startLifecyclePolicyPreviewRequest) throws ServerException,
InvalidParameterException, RepositoryNotFoundException, LifecyclePolicyNotFoundException,
LifecyclePolicyPreviewInProgressException, ValidationException, AwsServiceException, SdkClientException, EcrException {
return startLifecyclePolicyPreview(StartLifecyclePolicyPreviewRequest.builder()
.applyMutation(startLifecyclePolicyPreviewRequest).build());
}
/**
*
* Adds specified tags to a resource with the specified ARN. Existing tags on a resource are not changed if they are
* not specified in the request parameters.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidTagParameterException
* An invalid parameter has been specified. Tag keys can have a maximum character length of 128 characters,
* and tag values can have a maximum length of 256 characters.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws InvalidParameterException,
InvalidTagParameterException, TooManyTagsException, RepositoryNotFoundException, ServerException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Adds specified tags to a resource with the specified ARN. Existing tags on a resource are not changed if they are
* not specified in the request parameters.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidTagParameterException
* An invalid parameter has been specified. Tag keys can have a maximum character length of 128 characters,
* and tag values can have a maximum length of 256 characters.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest)
throws InvalidParameterException, InvalidTagParameterException, TooManyTagsException, RepositoryNotFoundException,
ServerException, AwsServiceException, SdkClientException, EcrException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidTagParameterException
* An invalid parameter has been specified. Tag keys can have a maximum character length of 128 characters,
* and tag values can have a maximum length of 256 characters.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws InvalidParameterException,
InvalidTagParameterException, TooManyTagsException, RepositoryNotFoundException, ServerException,
AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes specified tags from a resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidTagParameterException
* An invalid parameter has been specified. Tag keys can have a maximum character length of 128 characters,
* and tag values can have a maximum length of 256 characters.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws InvalidParameterException, InvalidTagParameterException, TooManyTagsException, RepositoryNotFoundException,
ServerException, AwsServiceException, SdkClientException, EcrException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates an existing pull through cache rule.
*
*
* @param updatePullThroughCacheRuleRequest
* @return Result of the UpdatePullThroughCacheRule operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws UnableToAccessSecretException
* The secret is unable to be accessed. Verify the resource permissions for the secret and try again.
* @throws PullThroughCacheRuleNotFoundException
* The pull through cache rule was not found. Specify a valid pull through cache rule and try again.
* @throws SecretNotFoundException
* The ARN of the secret specified in the pull through cache rule was not found. Update the pull through
* cache rule with a valid secret ARN and try again.
* @throws UnableToDecryptSecretValueException
* The secret is accessible but is unable to be decrypted. Verify the resource permisisons and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.UpdatePullThroughCacheRule
* @see AWS API Documentation
*/
default UpdatePullThroughCacheRuleResponse updatePullThroughCacheRule(
UpdatePullThroughCacheRuleRequest updatePullThroughCacheRuleRequest) throws ServerException,
InvalidParameterException, ValidationException, UnableToAccessSecretException, PullThroughCacheRuleNotFoundException,
SecretNotFoundException, UnableToDecryptSecretValueException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing pull through cache rule.
*
*
*
* This is a convenience which creates an instance of the {@link UpdatePullThroughCacheRuleRequest.Builder} avoiding
* the need to create one manually via {@link UpdatePullThroughCacheRuleRequest#builder()}
*
*
* @param updatePullThroughCacheRuleRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.UpdatePullThroughCacheRuleRequest.Builder} to create a
* request.
* @return Result of the UpdatePullThroughCacheRule operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws UnableToAccessSecretException
* The secret is unable to be accessed. Verify the resource permissions for the secret and try again.
* @throws PullThroughCacheRuleNotFoundException
* The pull through cache rule was not found. Specify a valid pull through cache rule and try again.
* @throws SecretNotFoundException
* The ARN of the secret specified in the pull through cache rule was not found. Update the pull through
* cache rule with a valid secret ARN and try again.
* @throws UnableToDecryptSecretValueException
* The secret is accessible but is unable to be decrypted. Verify the resource permisisons and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.UpdatePullThroughCacheRule
* @see AWS API Documentation
*/
default UpdatePullThroughCacheRuleResponse updatePullThroughCacheRule(
Consumer updatePullThroughCacheRuleRequest) throws ServerException,
InvalidParameterException, ValidationException, UnableToAccessSecretException, PullThroughCacheRuleNotFoundException,
SecretNotFoundException, UnableToDecryptSecretValueException, AwsServiceException, SdkClientException, EcrException {
return updatePullThroughCacheRule(UpdatePullThroughCacheRuleRequest.builder()
.applyMutation(updatePullThroughCacheRuleRequest).build());
}
/**
*
* Updates an existing repository creation template.
*
*
* @param updateRepositoryCreationTemplateRequest
* @return Result of the UpdateRepositoryCreationTemplate operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws TemplateNotFoundException
* The specified repository creation template can't be found. Verify the registry ID and prefix and try
* again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.UpdateRepositoryCreationTemplate
* @see AWS API Documentation
*/
default UpdateRepositoryCreationTemplateResponse updateRepositoryCreationTemplate(
UpdateRepositoryCreationTemplateRequest updateRepositoryCreationTemplateRequest) throws ServerException,
ValidationException, InvalidParameterException, TemplateNotFoundException, AwsServiceException, SdkClientException,
EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing repository creation template.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateRepositoryCreationTemplateRequest.Builder}
* avoiding the need to create one manually via {@link UpdateRepositoryCreationTemplateRequest#builder()}
*
*
* @param updateRepositoryCreationTemplateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.UpdateRepositoryCreationTemplateRequest.Builder} to
* create a request.
* @return Result of the UpdateRepositoryCreationTemplate operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ValidationException
* There was an exception validating this request.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws TemplateNotFoundException
* The specified repository creation template can't be found. Verify the registry ID and prefix and try
* again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.UpdateRepositoryCreationTemplate
* @see AWS API Documentation
*/
default UpdateRepositoryCreationTemplateResponse updateRepositoryCreationTemplate(
Consumer updateRepositoryCreationTemplateRequest)
throws ServerException, ValidationException, InvalidParameterException, TemplateNotFoundException,
AwsServiceException, SdkClientException, EcrException {
return updateRepositoryCreationTemplate(UpdateRepositoryCreationTemplateRequest.builder()
.applyMutation(updateRepositoryCreationTemplateRequest).build());
}
/**
*
* Uploads an image layer part to Amazon ECR.
*
*
* When an image is pushed, each new image layer is uploaded in parts. The maximum size of each image layer part can
* be 20971520 bytes (or about 20MB). The UploadLayerPart API is called once per each new image layer part.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param uploadLayerPartRequest
* @return Result of the UploadLayerPart operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidLayerPartException
* The layer part size is not valid, or the first byte specified is not consecutive to the last byte of a
* previous layer part upload.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws UploadNotFoundException
* The upload could not be found, or the specified upload ID is not valid for this repository.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.UploadLayerPart
* @see AWS API
* Documentation
*/
default UploadLayerPartResponse uploadLayerPart(UploadLayerPartRequest uploadLayerPartRequest) throws ServerException,
InvalidParameterException, InvalidLayerPartException, RepositoryNotFoundException, UploadNotFoundException,
LimitExceededException, KmsException, AwsServiceException, SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Uploads an image layer part to Amazon ECR.
*
*
* When an image is pushed, each new image layer is uploaded in parts. The maximum size of each image layer part can
* be 20971520 bytes (or about 20MB). The UploadLayerPart API is called once per each new image layer part.
*
*
*
* This operation is used by the Amazon ECR proxy and is not generally used by customers for pulling and pushing
* images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link UploadLayerPartRequest.Builder} avoiding the need
* to create one manually via {@link UploadLayerPartRequest#builder()}
*
*
* @param uploadLayerPartRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.UploadLayerPartRequest.Builder} to create a request.
* @return Result of the UploadLayerPart operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidLayerPartException
* The layer part size is not valid, or the first byte specified is not consecutive to the last byte of a
* previous layer part upload.
* @throws RepositoryNotFoundException
* The specified repository could not be found. Check the spelling of the specified repository and ensure
* that you are performing operations on the correct registry.
* @throws UploadNotFoundException
* The upload could not be found, or the specified upload ID is not valid for this repository.
* @throws LimitExceededException
* The operation did not succeed because it would have exceeded a service limit for your account. For more
* information, see Amazon ECR service
* quotas in the Amazon Elastic Container Registry User Guide.
* @throws KmsException
* The operation failed due to a KMS exception.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.UploadLayerPart
* @see AWS API
* Documentation
*/
default UploadLayerPartResponse uploadLayerPart(Consumer uploadLayerPartRequest)
throws ServerException, InvalidParameterException, InvalidLayerPartException, RepositoryNotFoundException,
UploadNotFoundException, LimitExceededException, KmsException, AwsServiceException, SdkClientException, EcrException {
return uploadLayerPart(UploadLayerPartRequest.builder().applyMutation(uploadLayerPartRequest).build());
}
/**
*
* Validates an existing pull through cache rule for an upstream registry that requires authentication. This will
* retrieve the contents of the Amazon Web Services Secrets Manager secret, verify the syntax, and then validate
* that authentication to the upstream registry is successful.
*
*
* @param validatePullThroughCacheRuleRequest
* @return Result of the ValidatePullThroughCacheRule operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws PullThroughCacheRuleNotFoundException
* The pull through cache rule was not found. Specify a valid pull through cache rule and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.ValidatePullThroughCacheRule
* @see AWS API Documentation
*/
default ValidatePullThroughCacheRuleResponse validatePullThroughCacheRule(
ValidatePullThroughCacheRuleRequest validatePullThroughCacheRuleRequest) throws ServerException,
InvalidParameterException, ValidationException, PullThroughCacheRuleNotFoundException, AwsServiceException,
SdkClientException, EcrException {
throw new UnsupportedOperationException();
}
/**
*
* Validates an existing pull through cache rule for an upstream registry that requires authentication. This will
* retrieve the contents of the Amazon Web Services Secrets Manager secret, verify the syntax, and then validate
* that authentication to the upstream registry is successful.
*
*
*
* This is a convenience which creates an instance of the {@link ValidatePullThroughCacheRuleRequest.Builder}
* avoiding the need to create one manually via {@link ValidatePullThroughCacheRuleRequest#builder()}
*
*
* @param validatePullThroughCacheRuleRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ecr.model.ValidatePullThroughCacheRuleRequest.Builder} to create a
* request.
* @return Result of the ValidatePullThroughCacheRule operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ValidationException
* There was an exception validating this request.
* @throws PullThroughCacheRuleNotFoundException
* The pull through cache rule was not found. Specify a valid pull through cache rule and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EcrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EcrClient.ValidatePullThroughCacheRule
* @see AWS API Documentation
*/
default ValidatePullThroughCacheRuleResponse validatePullThroughCacheRule(
Consumer validatePullThroughCacheRuleRequest) throws ServerException,
InvalidParameterException, ValidationException, PullThroughCacheRuleNotFoundException, AwsServiceException,
SdkClientException, EcrException {
return validatePullThroughCacheRule(ValidatePullThroughCacheRuleRequest.builder()
.applyMutation(validatePullThroughCacheRuleRequest).build());
}
/**
* Create an instance of {@link EcrWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link EcrWaiter}
*/
default EcrWaiter waiter() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link EcrClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static EcrClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link EcrClient}.
*/
static EcrClientBuilder builder() {
return new DefaultEcrClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default EcrServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}