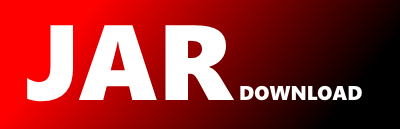
software.amazon.awssdk.services.ecr.model.CompleteLayerUploadResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecr Show documentation
Show all versions of ecr Show documentation
The AWS Java SDK for the Amazon EC2 Container Registry holds the client classes that are used for
communicating with the
Amazon EC2 Container Registry Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CompleteLayerUploadResponse extends EcrResponse implements
ToCopyableBuilder {
private static final SdkField REGISTRY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("registryId").getter(getter(CompleteLayerUploadResponse::registryId)).setter(setter(Builder::registryId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registryId").build()).build();
private static final SdkField REPOSITORY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("repositoryName").getter(getter(CompleteLayerUploadResponse::repositoryName))
.setter(setter(Builder::repositoryName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("repositoryName").build()).build();
private static final SdkField UPLOAD_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("uploadId").getter(getter(CompleteLayerUploadResponse::uploadId)).setter(setter(Builder::uploadId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("uploadId").build()).build();
private static final SdkField LAYER_DIGEST_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("layerDigest").getter(getter(CompleteLayerUploadResponse::layerDigest))
.setter(setter(Builder::layerDigest))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("layerDigest").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REGISTRY_ID_FIELD,
REPOSITORY_NAME_FIELD, UPLOAD_ID_FIELD, LAYER_DIGEST_FIELD));
private final String registryId;
private final String repositoryName;
private final String uploadId;
private final String layerDigest;
private CompleteLayerUploadResponse(BuilderImpl builder) {
super(builder);
this.registryId = builder.registryId;
this.repositoryName = builder.repositoryName;
this.uploadId = builder.uploadId;
this.layerDigest = builder.layerDigest;
}
/**
*
* The registry ID associated with the request.
*
*
* @return The registry ID associated with the request.
*/
public final String registryId() {
return registryId;
}
/**
*
* The repository name associated with the request.
*
*
* @return The repository name associated with the request.
*/
public final String repositoryName() {
return repositoryName;
}
/**
*
* The upload ID associated with the layer.
*
*
* @return The upload ID associated with the layer.
*/
public final String uploadId() {
return uploadId;
}
/**
*
* The sha256
digest of the image layer.
*
*
* @return The sha256
digest of the image layer.
*/
public final String layerDigest() {
return layerDigest;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(registryId());
hashCode = 31 * hashCode + Objects.hashCode(repositoryName());
hashCode = 31 * hashCode + Objects.hashCode(uploadId());
hashCode = 31 * hashCode + Objects.hashCode(layerDigest());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CompleteLayerUploadResponse)) {
return false;
}
CompleteLayerUploadResponse other = (CompleteLayerUploadResponse) obj;
return Objects.equals(registryId(), other.registryId()) && Objects.equals(repositoryName(), other.repositoryName())
&& Objects.equals(uploadId(), other.uploadId()) && Objects.equals(layerDigest(), other.layerDigest());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CompleteLayerUploadResponse").add("RegistryId", registryId())
.add("RepositoryName", repositoryName()).add("UploadId", uploadId()).add("LayerDigest", layerDigest()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "registryId":
return Optional.ofNullable(clazz.cast(registryId()));
case "repositoryName":
return Optional.ofNullable(clazz.cast(repositoryName()));
case "uploadId":
return Optional.ofNullable(clazz.cast(uploadId()));
case "layerDigest":
return Optional.ofNullable(clazz.cast(layerDigest()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy