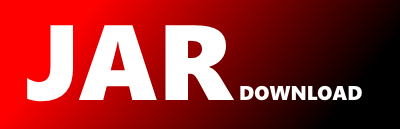
software.amazon.awssdk.services.ecr.EcrAsyncClient Maven / Gradle / Ivy
Show all versions of ecr Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecr;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.ecr.model.BatchCheckLayerAvailabilityRequest;
import software.amazon.awssdk.services.ecr.model.BatchCheckLayerAvailabilityResponse;
import software.amazon.awssdk.services.ecr.model.BatchDeleteImageRequest;
import software.amazon.awssdk.services.ecr.model.BatchDeleteImageResponse;
import software.amazon.awssdk.services.ecr.model.BatchGetImageRequest;
import software.amazon.awssdk.services.ecr.model.BatchGetImageResponse;
import software.amazon.awssdk.services.ecr.model.CompleteLayerUploadRequest;
import software.amazon.awssdk.services.ecr.model.CompleteLayerUploadResponse;
import software.amazon.awssdk.services.ecr.model.CreateRepositoryRequest;
import software.amazon.awssdk.services.ecr.model.CreateRepositoryResponse;
import software.amazon.awssdk.services.ecr.model.DeleteLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.DeleteLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryRequest;
import software.amazon.awssdk.services.ecr.model.DeleteRepositoryResponse;
import software.amazon.awssdk.services.ecr.model.DescribeImagesRequest;
import software.amazon.awssdk.services.ecr.model.DescribeImagesResponse;
import software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest;
import software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse;
import software.amazon.awssdk.services.ecr.model.GetAuthorizationTokenRequest;
import software.amazon.awssdk.services.ecr.model.GetAuthorizationTokenResponse;
import software.amazon.awssdk.services.ecr.model.GetDownloadUrlForLayerRequest;
import software.amazon.awssdk.services.ecr.model.GetDownloadUrlForLayerResponse;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewRequest;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyPreviewResponse;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.GetLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.GetRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.GetRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.InitiateLayerUploadRequest;
import software.amazon.awssdk.services.ecr.model.InitiateLayerUploadResponse;
import software.amazon.awssdk.services.ecr.model.ListImagesRequest;
import software.amazon.awssdk.services.ecr.model.ListImagesResponse;
import software.amazon.awssdk.services.ecr.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.ecr.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.ecr.model.PutImageRequest;
import software.amazon.awssdk.services.ecr.model.PutImageResponse;
import software.amazon.awssdk.services.ecr.model.PutLifecyclePolicyRequest;
import software.amazon.awssdk.services.ecr.model.PutLifecyclePolicyResponse;
import software.amazon.awssdk.services.ecr.model.SetRepositoryPolicyRequest;
import software.amazon.awssdk.services.ecr.model.SetRepositoryPolicyResponse;
import software.amazon.awssdk.services.ecr.model.StartLifecyclePolicyPreviewRequest;
import software.amazon.awssdk.services.ecr.model.StartLifecyclePolicyPreviewResponse;
import software.amazon.awssdk.services.ecr.model.TagResourceRequest;
import software.amazon.awssdk.services.ecr.model.TagResourceResponse;
import software.amazon.awssdk.services.ecr.model.UntagResourceRequest;
import software.amazon.awssdk.services.ecr.model.UntagResourceResponse;
import software.amazon.awssdk.services.ecr.model.UploadLayerPartRequest;
import software.amazon.awssdk.services.ecr.model.UploadLayerPartResponse;
import software.amazon.awssdk.services.ecr.paginators.DescribeImagesPublisher;
import software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher;
import software.amazon.awssdk.services.ecr.paginators.ListImagesPublisher;
/**
* Service client for accessing Amazon ECR asynchronously. This can be created using the static {@link #builder()}
* method.
*
*
* Amazon Elastic Container Registry (Amazon ECR) is a managed Docker registry service. Customers can use the familiar
* Docker CLI to push, pull, and manage images. Amazon ECR provides a secure, scalable, and reliable registry. Amazon
* ECR supports private Docker repositories with resource-based permissions using IAM so that specific users or Amazon
* EC2 instances can access repositories and images. Developers can use the Docker CLI to author and manage images.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface EcrAsyncClient extends SdkClient {
String SERVICE_NAME = "ecr";
/**
* Create a {@link EcrAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static EcrAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link EcrAsyncClient}.
*/
static EcrAsyncClientBuilder builder() {
return new DefaultEcrAsyncClientBuilder();
}
/**
*
* Check the availability of multiple image layers in a specified registry and repository.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param batchCheckLayerAvailabilityRequest
* @return A Java Future containing the result of the BatchCheckLayerAvailability operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchCheckLayerAvailability
* @see AWS API Documentation
*/
default CompletableFuture batchCheckLayerAvailability(
BatchCheckLayerAvailabilityRequest batchCheckLayerAvailabilityRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Check the availability of multiple image layers in a specified registry and repository.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link BatchCheckLayerAvailabilityRequest.Builder}
* avoiding the need to create one manually via {@link BatchCheckLayerAvailabilityRequest#builder()}
*
*
* @param batchCheckLayerAvailabilityRequest
* A {@link Consumer} that will call methods on {@link BatchCheckLayerAvailabilityRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the BatchCheckLayerAvailability operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchCheckLayerAvailability
* @see AWS API Documentation
*/
default CompletableFuture batchCheckLayerAvailability(
Consumer batchCheckLayerAvailabilityRequest) {
return batchCheckLayerAvailability(BatchCheckLayerAvailabilityRequest.builder()
.applyMutation(batchCheckLayerAvailabilityRequest).build());
}
/**
*
* Deletes a list of specified images within a specified repository. Images are specified with either
* imageTag
or imageDigest
.
*
*
* You can remove a tag from an image by specifying the image's tag in your request. When you remove the last tag
* from an image, the image is deleted from your repository.
*
*
* You can completely delete an image (and all of its tags) by specifying the image's digest in your request.
*
*
* @param batchDeleteImageRequest
* Deletes specified images within a specified repository. Images are specified with either the
* imageTag
or imageDigest
.
* @return A Java Future containing the result of the BatchDeleteImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchDeleteImage
* @see AWS API
* Documentation
*/
default CompletableFuture batchDeleteImage(BatchDeleteImageRequest batchDeleteImageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a list of specified images within a specified repository. Images are specified with either
* imageTag
or imageDigest
.
*
*
* You can remove a tag from an image by specifying the image's tag in your request. When you remove the last tag
* from an image, the image is deleted from your repository.
*
*
* You can completely delete an image (and all of its tags) by specifying the image's digest in your request.
*
*
*
* This is a convenience which creates an instance of the {@link BatchDeleteImageRequest.Builder} avoiding the need
* to create one manually via {@link BatchDeleteImageRequest#builder()}
*
*
* @param batchDeleteImageRequest
* A {@link Consumer} that will call methods on {@link BatchDeleteImageRequest.Builder} to create a request.
* Deletes specified images within a specified repository. Images are specified with either the
* imageTag
or imageDigest
.
* @return A Java Future containing the result of the BatchDeleteImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchDeleteImage
* @see AWS API
* Documentation
*/
default CompletableFuture batchDeleteImage(
Consumer batchDeleteImageRequest) {
return batchDeleteImage(BatchDeleteImageRequest.builder().applyMutation(batchDeleteImageRequest).build());
}
/**
*
* Gets detailed information for specified images within a specified repository. Images are specified with either
* imageTag
or imageDigest
.
*
*
* @param batchGetImageRequest
* @return A Java Future containing the result of the BatchGetImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchGetImage
* @see AWS API
* Documentation
*/
default CompletableFuture batchGetImage(BatchGetImageRequest batchGetImageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets detailed information for specified images within a specified repository. Images are specified with either
* imageTag
or imageDigest
.
*
*
*
* This is a convenience which creates an instance of the {@link BatchGetImageRequest.Builder} avoiding the need to
* create one manually via {@link BatchGetImageRequest#builder()}
*
*
* @param batchGetImageRequest
* A {@link Consumer} that will call methods on {@link BatchGetImageRequest.Builder} to create a request.
* @return A Java Future containing the result of the BatchGetImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.BatchGetImage
* @see AWS API
* Documentation
*/
default CompletableFuture batchGetImage(Consumer batchGetImageRequest) {
return batchGetImage(BatchGetImageRequest.builder().applyMutation(batchGetImageRequest).build());
}
/**
*
* Informs Amazon ECR that the image layer upload has completed for a specified registry, repository name, and
* upload ID. You can optionally provide a sha256
digest of the image layer for data validation
* purposes.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param completeLayerUploadRequest
* @return A Java Future containing the result of the CompleteLayerUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - UploadNotFoundException The upload could not be found, or the specified upload id is not valid for
* this repository.
* - InvalidLayerException The layer digest calculation performed by Amazon ECR upon receipt of the image
* layer does not match the digest specified.
* - LayerPartTooSmallException Layer parts must be at least 5 MiB in size.
* - LayerAlreadyExistsException The image layer already exists in the associated repository.
* - EmptyUploadException The specified layer upload does not contain any layer parts.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.CompleteLayerUpload
* @see AWS API
* Documentation
*/
default CompletableFuture completeLayerUpload(
CompleteLayerUploadRequest completeLayerUploadRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Informs Amazon ECR that the image layer upload has completed for a specified registry, repository name, and
* upload ID. You can optionally provide a sha256
digest of the image layer for data validation
* purposes.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link CompleteLayerUploadRequest.Builder} avoiding the
* need to create one manually via {@link CompleteLayerUploadRequest#builder()}
*
*
* @param completeLayerUploadRequest
* A {@link Consumer} that will call methods on {@link CompleteLayerUploadRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CompleteLayerUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - UploadNotFoundException The upload could not be found, or the specified upload id is not valid for
* this repository.
* - InvalidLayerException The layer digest calculation performed by Amazon ECR upon receipt of the image
* layer does not match the digest specified.
* - LayerPartTooSmallException Layer parts must be at least 5 MiB in size.
* - LayerAlreadyExistsException The image layer already exists in the associated repository.
* - EmptyUploadException The specified layer upload does not contain any layer parts.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.CompleteLayerUpload
* @see AWS API
* Documentation
*/
default CompletableFuture completeLayerUpload(
Consumer completeLayerUploadRequest) {
return completeLayerUpload(CompleteLayerUploadRequest.builder().applyMutation(completeLayerUploadRequest).build());
}
/**
*
* Creates an image repository.
*
*
* @param createRepositoryRequest
* @return A Java Future containing the result of the CreateRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryAlreadyExistsException The specified repository already exists in the specified registry.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR Default
* Service Limits in the Amazon Elastic Container Registry User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.CreateRepository
* @see AWS API
* Documentation
*/
default CompletableFuture createRepository(CreateRepositoryRequest createRepositoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an image repository.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRepositoryRequest.Builder} avoiding the need
* to create one manually via {@link CreateRepositoryRequest#builder()}
*
*
* @param createRepositoryRequest
* A {@link Consumer} that will call methods on {@link CreateRepositoryRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryAlreadyExistsException The specified repository already exists in the specified registry.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR Default
* Service Limits in the Amazon Elastic Container Registry User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.CreateRepository
* @see AWS API
* Documentation
*/
default CompletableFuture createRepository(
Consumer createRepositoryRequest) {
return createRepository(CreateRepositoryRequest.builder().applyMutation(createRepositoryRequest).build());
}
/**
*
* Deletes the specified lifecycle policy.
*
*
* @param deleteLifecyclePolicyRequest
* @return A Java Future containing the result of the DeleteLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture deleteLifecyclePolicy(
DeleteLifecyclePolicyRequest deleteLifecyclePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified lifecycle policy.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteLifecyclePolicyRequest#builder()}
*
*
* @param deleteLifecyclePolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteLifecyclePolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture deleteLifecyclePolicy(
Consumer deleteLifecyclePolicyRequest) {
return deleteLifecyclePolicy(DeleteLifecyclePolicyRequest.builder().applyMutation(deleteLifecyclePolicyRequest).build());
}
/**
*
* Deletes an existing image repository. If a repository contains images, you must use the force
option
* to delete it.
*
*
* @param deleteRepositoryRequest
* @return A Java Future containing the result of the DeleteRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryNotEmptyException The specified repository contains images. To delete a repository that
* contains images, you must force the deletion with the
force
parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteRepository
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRepository(DeleteRepositoryRequest deleteRepositoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing image repository. If a repository contains images, you must use the force
option
* to delete it.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRepositoryRequest.Builder} avoiding the need
* to create one manually via {@link DeleteRepositoryRequest#builder()}
*
*
* @param deleteRepositoryRequest
* A {@link Consumer} that will call methods on {@link DeleteRepositoryRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteRepository operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryNotEmptyException The specified repository contains images. To delete a repository that
* contains images, you must force the deletion with the
force
parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteRepository
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRepository(
Consumer deleteRepositoryRequest) {
return deleteRepository(DeleteRepositoryRequest.builder().applyMutation(deleteRepositoryRequest).build());
}
/**
*
* Deletes the repository policy from a specified repository.
*
*
* @param deleteRepositoryPolicyRequest
* @return A Java Future containing the result of the DeleteRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryPolicyNotFoundException The specified repository and registry combination does not have an
* associated repository policy.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteRepositoryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRepositoryPolicy(
DeleteRepositoryPolicyRequest deleteRepositoryPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the repository policy from a specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRepositoryPolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteRepositoryPolicyRequest#builder()}
*
*
* @param deleteRepositoryPolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteRepositoryPolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryPolicyNotFoundException The specified repository and registry combination does not have an
* associated repository policy.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DeleteRepositoryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRepositoryPolicy(
Consumer deleteRepositoryPolicyRequest) {
return deleteRepositoryPolicy(DeleteRepositoryPolicyRequest.builder().applyMutation(deleteRepositoryPolicyRequest)
.build());
}
/**
*
* Returns metadata about the images in a repository, including image size, image tags, and creation date.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* @param describeImagesRequest
* @return A Java Future containing the result of the DescribeImages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default CompletableFuture describeImages(DescribeImagesRequest describeImagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns metadata about the images in a repository, including image size, image tags, and creation date.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImagesRequest.Builder} avoiding the need to
* create one manually via {@link DescribeImagesRequest#builder()}
*
*
* @param describeImagesRequest
* A {@link Consumer} that will call methods on {@link DescribeImagesRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeImages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default CompletableFuture describeImages(Consumer describeImagesRequest) {
return describeImages(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build());
}
/**
*
* Returns metadata about the images in a repository, including image size, image tags, and creation date.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* This is a variant of {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeImagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)} operation.
*
*
* @param describeImagesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default DescribeImagesPublisher describeImagesPaginator(DescribeImagesRequest describeImagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns metadata about the images in a repository, including image size, image tags, and creation date.
*
*
*
* Beginning with Docker version 1.9, the Docker client compresses image layers before pushing them to a V2 Docker
* registry. The output of the docker images
command shows the uncompressed image size, so it may
* return a larger image size than the image sizes returned by DescribeImages.
*
*
*
* This is a variant of {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeImagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImages(software.amazon.awssdk.services.ecr.model.DescribeImagesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeImagesRequest.Builder} avoiding the need to
* create one manually via {@link DescribeImagesRequest#builder()}
*
*
* @param describeImagesRequest
* A {@link Consumer} that will call methods on {@link DescribeImagesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageNotFoundException The image requested does not exist in the specified repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default DescribeImagesPublisher describeImagesPaginator(Consumer describeImagesRequest) {
return describeImagesPaginator(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build());
}
/**
*
* Describes image repositories in a registry.
*
*
* @param describeRepositoriesRequest
* @return A Java Future containing the result of the DescribeRepositories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default CompletableFuture describeRepositories(
DescribeRepositoriesRequest describeRepositoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes image repositories in a registry.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRepositoriesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRepositoriesRequest#builder()}
*
*
* @param describeRepositoriesRequest
* A {@link Consumer} that will call methods on {@link DescribeRepositoriesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeRepositories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default CompletableFuture describeRepositories(
Consumer describeRepositoriesRequest) {
return describeRepositories(DescribeRepositoriesRequest.builder().applyMutation(describeRepositoriesRequest).build());
}
/**
*
* Describes image repositories in a registry.
*
*
* @return A Java Future containing the result of the DescribeRepositories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default CompletableFuture describeRepositories() {
return describeRepositories(DescribeRepositoriesRequest.builder().build());
}
/**
*
* Describes image repositories in a registry.
*
*
*
* This is a variant of
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default DescribeRepositoriesPublisher describeRepositoriesPaginator() {
return describeRepositoriesPaginator(DescribeRepositoriesRequest.builder().build());
}
/**
*
* Describes image repositories in a registry.
*
*
*
* This is a variant of
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)}
* operation.
*
*
* @param describeRepositoriesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default DescribeRepositoriesPublisher describeRepositoriesPaginator(DescribeRepositoriesRequest describeRepositoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes image repositories in a registry.
*
*
*
* This is a variant of
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.DescribeRepositoriesPublisher publisher = client.describeRepositoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRepositories(software.amazon.awssdk.services.ecr.model.DescribeRepositoriesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeRepositoriesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRepositoriesRequest#builder()}
*
*
* @param describeRepositoriesRequest
* A {@link Consumer} that will call methods on {@link DescribeRepositoriesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.DescribeRepositories
* @see AWS API
* Documentation
*/
default DescribeRepositoriesPublisher describeRepositoriesPaginator(
Consumer describeRepositoriesRequest) {
return describeRepositoriesPaginator(DescribeRepositoriesRequest.builder().applyMutation(describeRepositoriesRequest)
.build());
}
/**
*
* Retrieves a token that is valid for a specified registry for 12 hours. This command allows you to use the
* docker
CLI to push and pull images with Amazon ECR. If you do not specify a registry, the default
* registry is assumed.
*
*
* The authorizationToken
returned for each registry specified is a base64 encoded string that can be
* decoded and used in a docker login
command to authenticate to a registry. The AWS CLI offers an
* aws ecr get-login
command that simplifies the login process.
*
*
* @param getAuthorizationTokenRequest
* @return A Java Future containing the result of the GetAuthorizationToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetAuthorizationToken
* @see AWS API
* Documentation
*/
default CompletableFuture getAuthorizationToken(
GetAuthorizationTokenRequest getAuthorizationTokenRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a token that is valid for a specified registry for 12 hours. This command allows you to use the
* docker
CLI to push and pull images with Amazon ECR. If you do not specify a registry, the default
* registry is assumed.
*
*
* The authorizationToken
returned for each registry specified is a base64 encoded string that can be
* decoded and used in a docker login
command to authenticate to a registry. The AWS CLI offers an
* aws ecr get-login
command that simplifies the login process.
*
*
*
* This is a convenience which creates an instance of the {@link GetAuthorizationTokenRequest.Builder} avoiding the
* need to create one manually via {@link GetAuthorizationTokenRequest#builder()}
*
*
* @param getAuthorizationTokenRequest
* A {@link Consumer} that will call methods on {@link GetAuthorizationTokenRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetAuthorizationToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetAuthorizationToken
* @see AWS API
* Documentation
*/
default CompletableFuture getAuthorizationToken(
Consumer getAuthorizationTokenRequest) {
return getAuthorizationToken(GetAuthorizationTokenRequest.builder().applyMutation(getAuthorizationTokenRequest).build());
}
/**
*
* Retrieves a token that is valid for a specified registry for 12 hours. This command allows you to use the
* docker
CLI to push and pull images with Amazon ECR. If you do not specify a registry, the default
* registry is assumed.
*
*
* The authorizationToken
returned for each registry specified is a base64 encoded string that can be
* decoded and used in a docker login
command to authenticate to a registry. The AWS CLI offers an
* aws ecr get-login
command that simplifies the login process.
*
*
* @return A Java Future containing the result of the GetAuthorizationToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetAuthorizationToken
* @see AWS API
* Documentation
*/
default CompletableFuture getAuthorizationToken() {
return getAuthorizationToken(GetAuthorizationTokenRequest.builder().build());
}
/**
*
* Retrieves the pre-signed Amazon S3 download URL corresponding to an image layer. You can only get URLs for image
* layers that are referenced in an image.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param getDownloadUrlForLayerRequest
* @return A Java Future containing the result of the GetDownloadUrlForLayer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - LayersNotFoundException The specified layers could not be found, or the specified layer is not valid
* for this repository.
* - LayerInaccessibleException The specified layer is not available because it is not associated with an
* image. Unassociated image layers may be cleaned up at any time.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetDownloadUrlForLayer
* @see AWS API
* Documentation
*/
default CompletableFuture getDownloadUrlForLayer(
GetDownloadUrlForLayerRequest getDownloadUrlForLayerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the pre-signed Amazon S3 download URL corresponding to an image layer. You can only get URLs for image
* layers that are referenced in an image.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link GetDownloadUrlForLayerRequest.Builder} avoiding the
* need to create one manually via {@link GetDownloadUrlForLayerRequest#builder()}
*
*
* @param getDownloadUrlForLayerRequest
* A {@link Consumer} that will call methods on {@link GetDownloadUrlForLayerRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetDownloadUrlForLayer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - LayersNotFoundException The specified layers could not be found, or the specified layer is not valid
* for this repository.
* - LayerInaccessibleException The specified layer is not available because it is not associated with an
* image. Unassociated image layers may be cleaned up at any time.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetDownloadUrlForLayer
* @see AWS API
* Documentation
*/
default CompletableFuture getDownloadUrlForLayer(
Consumer getDownloadUrlForLayerRequest) {
return getDownloadUrlForLayer(GetDownloadUrlForLayerRequest.builder().applyMutation(getDownloadUrlForLayerRequest)
.build());
}
/**
*
* Retrieves the specified lifecycle policy.
*
*
* @param getLifecyclePolicyRequest
* @return A Java Future containing the result of the GetLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getLifecyclePolicy(GetLifecyclePolicyRequest getLifecyclePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the specified lifecycle policy.
*
*
*
* This is a convenience which creates an instance of the {@link GetLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetLifecyclePolicyRequest#builder()}
*
*
* @param getLifecyclePolicyRequest
* A {@link Consumer} that will call methods on {@link GetLifecyclePolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getLifecyclePolicy(
Consumer getLifecyclePolicyRequest) {
return getLifecyclePolicy(GetLifecyclePolicyRequest.builder().applyMutation(getLifecyclePolicyRequest).build());
}
/**
*
* Retrieves the results of the specified lifecycle policy preview request.
*
*
* @param getLifecyclePolicyPreviewRequest
* @return A Java Future containing the result of the GetLifecyclePolicyPreview operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyPreviewNotFoundException There is no dry run for this repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
default CompletableFuture getLifecyclePolicyPreview(
GetLifecyclePolicyPreviewRequest getLifecyclePolicyPreviewRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the results of the specified lifecycle policy preview request.
*
*
*
* This is a convenience which creates an instance of the {@link GetLifecyclePolicyPreviewRequest.Builder} avoiding
* the need to create one manually via {@link GetLifecyclePolicyPreviewRequest#builder()}
*
*
* @param getLifecyclePolicyPreviewRequest
* A {@link Consumer} that will call methods on {@link GetLifecyclePolicyPreviewRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetLifecyclePolicyPreview operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyPreviewNotFoundException There is no dry run for this repository.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetLifecyclePolicyPreview
* @see AWS
* API Documentation
*/
default CompletableFuture getLifecyclePolicyPreview(
Consumer getLifecyclePolicyPreviewRequest) {
return getLifecyclePolicyPreview(GetLifecyclePolicyPreviewRequest.builder()
.applyMutation(getLifecyclePolicyPreviewRequest).build());
}
/**
*
* Retrieves the repository policy for a specified repository.
*
*
* @param getRepositoryPolicyRequest
* @return A Java Future containing the result of the GetRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryPolicyNotFoundException The specified repository and registry combination does not have an
* associated repository policy.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetRepositoryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getRepositoryPolicy(
GetRepositoryPolicyRequest getRepositoryPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the repository policy for a specified repository.
*
*
*
* This is a convenience which creates an instance of the {@link GetRepositoryPolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetRepositoryPolicyRequest#builder()}
*
*
* @param getRepositoryPolicyRequest
* A {@link Consumer} that will call methods on {@link GetRepositoryPolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - RepositoryPolicyNotFoundException The specified repository and registry combination does not have an
* associated repository policy.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.GetRepositoryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getRepositoryPolicy(
Consumer getRepositoryPolicyRequest) {
return getRepositoryPolicy(GetRepositoryPolicyRequest.builder().applyMutation(getRepositoryPolicyRequest).build());
}
/**
*
* Notify Amazon ECR that you intend to upload an image layer.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param initiateLayerUploadRequest
* @return A Java Future containing the result of the InitiateLayerUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.InitiateLayerUpload
* @see AWS API
* Documentation
*/
default CompletableFuture initiateLayerUpload(
InitiateLayerUploadRequest initiateLayerUploadRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Notify Amazon ECR that you intend to upload an image layer.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link InitiateLayerUploadRequest.Builder} avoiding the
* need to create one manually via {@link InitiateLayerUploadRequest#builder()}
*
*
* @param initiateLayerUploadRequest
* A {@link Consumer} that will call methods on {@link InitiateLayerUploadRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the InitiateLayerUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.InitiateLayerUpload
* @see AWS API
* Documentation
*/
default CompletableFuture initiateLayerUpload(
Consumer initiateLayerUploadRequest) {
return initiateLayerUpload(InitiateLayerUploadRequest.builder().applyMutation(initiateLayerUploadRequest).build());
}
/**
*
* Lists all the image IDs for a given repository.
*
*
* You can filter images based on whether or not they are tagged by setting the tagStatus
parameter to
* TAGGED
or UNTAGGED
. For example, you can filter your results to return only
* UNTAGGED
images and then pipe that result to a BatchDeleteImage operation to delete them. Or,
* you can filter your results to return only TAGGED
images to list all of the tags in your repository.
*
*
* @param listImagesRequest
* @return A Java Future containing the result of the ListImages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListImages
* @see AWS API
* Documentation
*/
default CompletableFuture listImages(ListImagesRequest listImagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the image IDs for a given repository.
*
*
* You can filter images based on whether or not they are tagged by setting the tagStatus
parameter to
* TAGGED
or UNTAGGED
. For example, you can filter your results to return only
* UNTAGGED
images and then pipe that result to a BatchDeleteImage operation to delete them. Or,
* you can filter your results to return only TAGGED
images to list all of the tags in your repository.
*
*
*
* This is a convenience which creates an instance of the {@link ListImagesRequest.Builder} avoiding the need to
* create one manually via {@link ListImagesRequest#builder()}
*
*
* @param listImagesRequest
* A {@link Consumer} that will call methods on {@link ListImagesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListImages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListImages
* @see AWS API
* Documentation
*/
default CompletableFuture listImages(Consumer listImagesRequest) {
return listImages(ListImagesRequest.builder().applyMutation(listImagesRequest).build());
}
/**
*
* Lists all the image IDs for a given repository.
*
*
* You can filter images based on whether or not they are tagged by setting the tagStatus
parameter to
* TAGGED
or UNTAGGED
. For example, you can filter your results to return only
* UNTAGGED
images and then pipe that result to a BatchDeleteImage operation to delete them. Or,
* you can filter your results to return only TAGGED
images to list all of the tags in your repository.
*
*
*
* This is a variant of {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesPublisher publisher = client.listImagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesPublisher publisher = client.listImagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.ListImagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
*
*
* @param listImagesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListImages
* @see AWS API
* Documentation
*/
default ListImagesPublisher listImagesPaginator(ListImagesRequest listImagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the image IDs for a given repository.
*
*
* You can filter images based on whether or not they are tagged by setting the tagStatus
parameter to
* TAGGED
or UNTAGGED
. For example, you can filter your results to return only
* UNTAGGED
images and then pipe that result to a BatchDeleteImage operation to delete them. Or,
* you can filter your results to return only TAGGED
images to list all of the tags in your repository.
*
*
*
* This is a variant of {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesPublisher publisher = client.listImagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecr.paginators.ListImagesPublisher publisher = client.listImagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecr.model.ListImagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImages(software.amazon.awssdk.services.ecr.model.ListImagesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListImagesRequest.Builder} avoiding the need to
* create one manually via {@link ListImagesRequest#builder()}
*
*
* @param listImagesRequest
* A {@link Consumer} that will call methods on {@link ListImagesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListImages
* @see AWS API
* Documentation
*/
default ListImagesPublisher listImagesPaginator(Consumer listImagesRequest) {
return listImagesPaginator(ListImagesRequest.builder().applyMutation(listImagesRequest).build());
}
/**
*
* List the tags for an Amazon ECR resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* List the tags for an Amazon ECR resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Creates or updates the image manifest and tags associated with an image.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param putImageRequest
* @return A Java Future containing the result of the PutImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageAlreadyExistsException The specified image has already been pushed, and there were no changes to
* the manifest or image tag after the last push.
* - LayersNotFoundException The specified layers could not be found, or the specified layer is not valid
* for this repository.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR Default
* Service Limits in the Amazon Elastic Container Registry User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutImage
* @see AWS API
* Documentation
*/
default CompletableFuture putImage(PutImageRequest putImageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the image manifest and tags associated with an image.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link PutImageRequest.Builder} avoiding the need to
* create one manually via {@link PutImageRequest#builder()}
*
*
* @param putImageRequest
* A {@link Consumer} that will call methods on {@link PutImageRequest.Builder} to create a request.
* @return A Java Future containing the result of the PutImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ImageAlreadyExistsException The specified image has already been pushed, and there were no changes to
* the manifest or image tag after the last push.
* - LayersNotFoundException The specified layers could not be found, or the specified layer is not valid
* for this repository.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR Default
* Service Limits in the Amazon Elastic Container Registry User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutImage
* @see AWS API
* Documentation
*/
default CompletableFuture putImage(Consumer putImageRequest) {
return putImage(PutImageRequest.builder().applyMutation(putImageRequest).build());
}
/**
*
* Creates or updates a lifecycle policy. For information about lifecycle policy syntax, see Lifecycle Policy
* Template.
*
*
* @param putLifecyclePolicyRequest
* @return A Java Future containing the result of the PutLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture putLifecyclePolicy(PutLifecyclePolicyRequest putLifecyclePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates a lifecycle policy. For information about lifecycle policy syntax, see Lifecycle Policy
* Template.
*
*
*
* This is a convenience which creates an instance of the {@link PutLifecyclePolicyRequest.Builder} avoiding the
* need to create one manually via {@link PutLifecyclePolicyRequest#builder()}
*
*
* @param putLifecyclePolicyRequest
* A {@link Consumer} that will call methods on {@link PutLifecyclePolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the PutLifecyclePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.PutLifecyclePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture putLifecyclePolicy(
Consumer putLifecyclePolicyRequest) {
return putLifecyclePolicy(PutLifecyclePolicyRequest.builder().applyMutation(putLifecyclePolicyRequest).build());
}
/**
*
* Applies a repository policy on a specified repository to control access permissions.
*
*
* @param setRepositoryPolicyRequest
* @return A Java Future containing the result of the SetRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.SetRepositoryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture setRepositoryPolicy(
SetRepositoryPolicyRequest setRepositoryPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Applies a repository policy on a specified repository to control access permissions.
*
*
*
* This is a convenience which creates an instance of the {@link SetRepositoryPolicyRequest.Builder} avoiding the
* need to create one manually via {@link SetRepositoryPolicyRequest#builder()}
*
*
* @param setRepositoryPolicyRequest
* A {@link Consumer} that will call methods on {@link SetRepositoryPolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the SetRepositoryPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.SetRepositoryPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture setRepositoryPolicy(
Consumer setRepositoryPolicyRequest) {
return setRepositoryPolicy(SetRepositoryPolicyRequest.builder().applyMutation(setRepositoryPolicyRequest).build());
}
/**
*
* Starts a preview of the specified lifecycle policy. This allows you to see the results before creating the
* lifecycle policy.
*
*
* @param startLifecyclePolicyPreviewRequest
* @return A Java Future containing the result of the StartLifecyclePolicyPreview operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - LifecyclePolicyPreviewInProgressException The previous lifecycle policy preview request has not
* completed. Please try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.StartLifecyclePolicyPreview
* @see AWS API Documentation
*/
default CompletableFuture startLifecyclePolicyPreview(
StartLifecyclePolicyPreviewRequest startLifecyclePolicyPreviewRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts a preview of the specified lifecycle policy. This allows you to see the results before creating the
* lifecycle policy.
*
*
*
* This is a convenience which creates an instance of the {@link StartLifecyclePolicyPreviewRequest.Builder}
* avoiding the need to create one manually via {@link StartLifecyclePolicyPreviewRequest#builder()}
*
*
* @param startLifecyclePolicyPreviewRequest
* A {@link Consumer} that will call methods on {@link StartLifecyclePolicyPreviewRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the StartLifecyclePolicyPreview operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - LifecyclePolicyNotFoundException The lifecycle policy could not be found, and no policy is set to the
* repository.
* - LifecyclePolicyPreviewInProgressException The previous lifecycle policy preview request has not
* completed. Please try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.StartLifecyclePolicyPreview
* @see AWS API Documentation
*/
default CompletableFuture startLifecyclePolicyPreview(
Consumer startLifecyclePolicyPreviewRequest) {
return startLifecyclePolicyPreview(StartLifecyclePolicyPreviewRequest.builder()
.applyMutation(startLifecyclePolicyPreviewRequest).build());
}
/**
*
* Adds specified tags to a resource with the specified ARN. Existing tags on a resource are not changed if they are
* not specified in the request parameters.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds specified tags to a resource with the specified ARN. Existing tags on a resource are not changed if they are
* not specified in the request parameters.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes specified tags from a resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidTagParameterException An invalid parameter has been specified. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* - TooManyTagsException The list of tags on the repository is over the limit. The maximum number of tags
* that can be applied to a repository is 50.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - ServerException These errors are usually caused by a server-side issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Uploads an image layer part to Amazon ECR.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* @param uploadLayerPartRequest
* @return A Java Future containing the result of the UploadLayerPart operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidLayerPartException The layer part size is not valid, or the first byte specified is not
* consecutive to the last byte of a previous layer part upload.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - UploadNotFoundException The upload could not be found, or the specified upload id is not valid for
* this repository.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR Default
* Service Limits in the Amazon Elastic Container Registry User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.UploadLayerPart
* @see AWS API
* Documentation
*/
default CompletableFuture uploadLayerPart(UploadLayerPartRequest uploadLayerPartRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Uploads an image layer part to Amazon ECR.
*
*
*
* This operation is used by the Amazon ECR proxy, and it is not intended for general use by customers for pulling
* and pushing images. In most cases, you should use the docker
CLI to pull, tag, and push images.
*
*
*
* This is a convenience which creates an instance of the {@link UploadLayerPartRequest.Builder} avoiding the need
* to create one manually via {@link UploadLayerPartRequest#builder()}
*
*
* @param uploadLayerPartRequest
* A {@link Consumer} that will call methods on {@link UploadLayerPartRequest.Builder} to create a request.
* @return A Java Future containing the result of the UploadLayerPart operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server-side issue.
* - InvalidParameterException The specified parameter is invalid. Review the available parameters for the
* API request.
* - InvalidLayerPartException The layer part size is not valid, or the first byte specified is not
* consecutive to the last byte of a previous layer part upload.
* - RepositoryNotFoundException The specified repository could not be found. Check the spelling of the
* specified repository and ensure that you are performing operations on the correct registry.
* - UploadNotFoundException The upload could not be found, or the specified upload id is not valid for
* this repository.
* - LimitExceededException The operation did not succeed because it would have exceeded a service limit
* for your account. For more information, see Amazon ECR Default
* Service Limits in the Amazon Elastic Container Registry User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcrAsyncClient.UploadLayerPart
* @see AWS API
* Documentation
*/
default CompletableFuture uploadLayerPart(
Consumer uploadLayerPartRequest) {
return uploadLayerPart(UploadLayerPartRequest.builder().applyMutation(uploadLayerPartRequest).build());
}
}