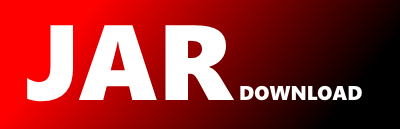
software.amazon.awssdk.services.ecs.model.CreateServiceResponse Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateServiceResponse extends EcsResponse implements
ToCopyableBuilder {
private static final SdkField SERVICE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(CreateServiceResponse::service)).setter(setter(Builder::service)).constructor(Service::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("service").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SERVICE_FIELD));
private final Service service;
private CreateServiceResponse(BuilderImpl builder) {
super(builder);
this.service = builder.service;
}
/**
*
* The full description of your service following the create call.
*
*
* If a service is using the ECS
deployment controller, the deploymentController
and
* taskSets
parameters will not be returned.
*
*
* If the service is using the CODE_DEPLOY
deployment controller, the deploymentController
, taskSets
and deployments
parameters will be returned, however the
* deployments
parameter will be an empty list.
*
*
* @return The full description of your service following the create call.
*
* If a service is using the ECS
deployment controller, the deploymentController
* and taskSets
parameters will not be returned.
*
*
* If the service is using the CODE_DEPLOY
deployment controller, the
* deploymentController
, taskSets
and deployments
parameters will be
* returned, however the deployments
parameter will be an empty list.
*/
public Service service() {
return service;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(service());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateServiceResponse)) {
return false;
}
CreateServiceResponse other = (CreateServiceResponse) obj;
return Objects.equals(service(), other.service());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("CreateServiceResponse").add("Service", service()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "service":
return Optional.ofNullable(clazz.cast(service()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If a service is using the ECS
deployment controller, the
* deploymentController
and taskSets
parameters will not be returned.
*
*
* If the service is using the CODE_DEPLOY
deployment controller, the
* deploymentController
, taskSets
and deployments
parameters will
* be returned, however the deployments
parameter will be an empty list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder service(Service service);
/**
*
* The full description of your service following the create call.
*
*
* If a service is using the ECS
deployment controller, the deploymentController
and
* taskSets
parameters will not be returned.
*
*
* If the service is using the CODE_DEPLOY
deployment controller, the
* deploymentController
, taskSets
and deployments
parameters will be
* returned, however the deployments
parameter will be an empty list.
*
* This is a convenience that creates an instance of the {@link Service.Builder} avoiding the need to create one
* manually via {@link Service#builder()}.
*
* When the {@link Consumer} completes, {@link Service.Builder#build()} is called immediately and its result is
* passed to {@link #service(Service)}.
*
* @param service
* a consumer that will call methods on {@link Service.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #service(Service)
*/
default Builder service(Consumer service) {
return service(Service.builder().applyMutation(service).build());
}
}
static final class BuilderImpl extends EcsResponse.BuilderImpl implements Builder {
private Service service;
private BuilderImpl() {
}
private BuilderImpl(CreateServiceResponse model) {
super(model);
service(model.service);
}
public final Service.Builder getService() {
return service != null ? service.toBuilder() : null;
}
@Override
public final Builder service(Service service) {
this.service = service;
return this;
}
public final void setService(Service.BuilderImpl service) {
this.service = service != null ? service.build() : null;
}
@Override
public CreateServiceResponse build() {
return new CreateServiceResponse(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}