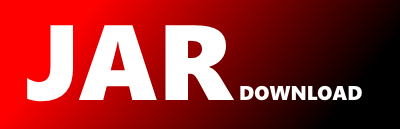
software.amazon.awssdk.services.ecs.model.Failure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecs Show documentation
Show all versions of ecs Show documentation
The AWS Java SDK for the Amazon EC2 Container Service holds the client classes that are used for
communicating with the
Amazon EC2 Container Service
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A failed resource.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Failure implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Failure::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Failure::reason)).setter(setter(Builder::reason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("reason").build()).build();
private static final SdkField DETAIL_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Failure::detail)).setter(setter(Builder::detail))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("detail").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, REASON_FIELD,
DETAIL_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final String reason;
private final String detail;
private Failure(BuilderImpl builder) {
this.arn = builder.arn;
this.reason = builder.reason;
this.detail = builder.detail;
}
/**
*
* The Amazon Resource Name (ARN) of the failed resource.
*
*
* @return The Amazon Resource Name (ARN) of the failed resource.
*/
public String arn() {
return arn;
}
/**
*
* The reason for the failure.
*
*
* @return The reason for the failure.
*/
public String reason() {
return reason;
}
/**
*
* The details of the failure.
*
*
* @return The details of the failure.
*/
public String detail() {
return detail;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(reason());
hashCode = 31 * hashCode + Objects.hashCode(detail());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Failure)) {
return false;
}
Failure other = (Failure) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(reason(), other.reason())
&& Objects.equals(detail(), other.detail());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("Failure").add("Arn", arn()).add("Reason", reason()).add("Detail", detail()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "reason":
return Optional.ofNullable(clazz.cast(reason()));
case "detail":
return Optional.ofNullable(clazz.cast(detail()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy