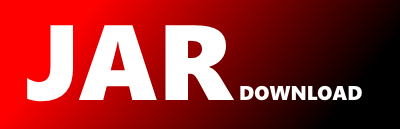
software.amazon.awssdk.services.ecs.model.HealthCheck Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object representing a container health check. Health check parameters that are specified in a container definition
* override any Docker health checks that exist in the container image (such as those specified in a parent image or
* from the image's Dockerfile).
*
*
* You can view the health status of both individual containers and a task with the DescribeTasks API operation or when
* viewing the task details in the console.
*
*
* The following describes the possible healthStatus
values for a container:
*
*
* -
*
* HEALTHY
-The container health check has passed successfully.
*
*
* -
*
* UNHEALTHY
-The container health check has failed.
*
*
* -
*
* UNKNOWN
-The container health check is being evaluated or there is no container health check defined.
*
*
*
*
* The following describes the possible healthStatus
values for a task. The container health check status
* of nonessential containers do not have an effect on the health status of a task.
*
*
* -
*
* HEALTHY
-All essential containers within the task have passed their health checks.
*
*
* -
*
* UNHEALTHY
-One or more essential containers have failed their health check.
*
*
* -
*
* UNKNOWN
-The essential containers within the task are still having their health checks evaluated or there
* are no container health checks defined.
*
*
*
*
* If a task is run manually, and not as part of a service, the task will continue its lifecycle regardless of its
* health status. For tasks that are part of a service, if the task reports as unhealthy then the task will be stopped
* and the service scheduler will replace it.
*
*
* The following are notes about container health check support:
*
*
* -
*
* Container health checks require version 1.17.0 or greater of the Amazon ECS container agent. For more information,
* see Updating the Amazon
* ECS Container Agent.
*
*
* -
*
* Container health checks are supported for Fargate tasks if you are using platform version 1.1.0 or greater. For more
* information, see AWS
* Fargate Platform Versions.
*
*
* -
*
* Container health checks are not supported for tasks that are part of a service that is configured to use a Classic
* Load Balancer.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class HealthCheck implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField> COMMAND_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("command")
.getter(getter(HealthCheck::command))
.setter(setter(Builder::command))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("command").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INTERVAL_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("interval").getter(getter(HealthCheck::interval)).setter(setter(Builder::interval))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("interval").build()).build();
private static final SdkField TIMEOUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("timeout").getter(getter(HealthCheck::timeout)).setter(setter(Builder::timeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timeout").build()).build();
private static final SdkField RETRIES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("retries").getter(getter(HealthCheck::retries)).setter(setter(Builder::retries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("retries").build()).build();
private static final SdkField START_PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("startPeriod").getter(getter(HealthCheck::startPeriod)).setter(setter(Builder::startPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startPeriod").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(COMMAND_FIELD, INTERVAL_FIELD,
TIMEOUT_FIELD, RETRIES_FIELD, START_PERIOD_FIELD));
private static final long serialVersionUID = 1L;
private final List command;
private final Integer interval;
private final Integer timeout;
private final Integer retries;
private final Integer startPeriod;
private HealthCheck(BuilderImpl builder) {
this.command = builder.command;
this.interval = builder.interval;
this.timeout = builder.timeout;
this.retries = builder.retries;
this.startPeriod = builder.startPeriod;
}
/**
* Returns true if the Command property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasCommand() {
return command != null && !(command instanceof SdkAutoConstructList);
}
/**
*
* A string array representing the command that the container runs to determine if it is healthy. The string array
* must start with CMD
to execute the command arguments directly, or CMD-SHELL
to run the
* command with the container's default shell. For example:
*
*
* [ "CMD-SHELL", "curl -f http://localhost/ || exit 1" ]
*
*
* An exit code of 0 indicates success, and non-zero exit code indicates failure. For more information, see
* HealthCheck
in the Create a container section of the
* Docker Remote API.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasCommand()} to see if a value was sent in this field.
*
*
* @return A string array representing the command that the container runs to determine if it is healthy. The string
* array must start with CMD
to execute the command arguments directly, or
* CMD-SHELL
to run the command with the container's default shell. For example:
*
* [ "CMD-SHELL", "curl -f http://localhost/ || exit 1" ]
*
*
* An exit code of 0 indicates success, and non-zero exit code indicates failure. For more information, see
* HealthCheck
in the Create a container section
* of the Docker Remote API.
*/
public List command() {
return command;
}
/**
*
* The time period in seconds between each health check execution. You may specify between 5 and 300 seconds. The
* default value is 30 seconds.
*
*
* @return The time period in seconds between each health check execution. You may specify between 5 and 300
* seconds. The default value is 30 seconds.
*/
public Integer interval() {
return interval;
}
/**
*
* The time period in seconds to wait for a health check to succeed before it is considered a failure. You may
* specify between 2 and 60 seconds. The default value is 5.
*
*
* @return The time period in seconds to wait for a health check to succeed before it is considered a failure. You
* may specify between 2 and 60 seconds. The default value is 5.
*/
public Integer timeout() {
return timeout;
}
/**
*
* The number of times to retry a failed health check before the container is considered unhealthy. You may specify
* between 1 and 10 retries. The default value is 3.
*
*
* @return The number of times to retry a failed health check before the container is considered unhealthy. You may
* specify between 1 and 10 retries. The default value is 3.
*/
public Integer retries() {
return retries;
}
/**
*
* The optional grace period within which to provide containers time to bootstrap before failed health checks count
* towards the maximum number of retries. You may specify between 0 and 300 seconds. The startPeriod
is
* disabled by default.
*
*
*
* If a health check succeeds within the startPeriod
, then the container is considered healthy and any
* subsequent failures count toward the maximum number of retries.
*
*
*
* @return The optional grace period within which to provide containers time to bootstrap before failed health
* checks count towards the maximum number of retries. You may specify between 0 and 300 seconds. The
* startPeriod
is disabled by default.
*
* If a health check succeeds within the startPeriod
, then the container is considered healthy
* and any subsequent failures count toward the maximum number of retries.
*
*/
public Integer startPeriod() {
return startPeriod;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasCommand() ? command() : null);
hashCode = 31 * hashCode + Objects.hashCode(interval());
hashCode = 31 * hashCode + Objects.hashCode(timeout());
hashCode = 31 * hashCode + Objects.hashCode(retries());
hashCode = 31 * hashCode + Objects.hashCode(startPeriod());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof HealthCheck)) {
return false;
}
HealthCheck other = (HealthCheck) obj;
return hasCommand() == other.hasCommand() && Objects.equals(command(), other.command())
&& Objects.equals(interval(), other.interval()) && Objects.equals(timeout(), other.timeout())
&& Objects.equals(retries(), other.retries()) && Objects.equals(startPeriod(), other.startPeriod());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("HealthCheck").add("Command", hasCommand() ? command() : null).add("Interval", interval())
.add("Timeout", timeout()).add("Retries", retries()).add("StartPeriod", startPeriod()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "command":
return Optional.ofNullable(clazz.cast(command()));
case "interval":
return Optional.ofNullable(clazz.cast(interval()));
case "timeout":
return Optional.ofNullable(clazz.cast(timeout()));
case "retries":
return Optional.ofNullable(clazz.cast(retries()));
case "startPeriod":
return Optional.ofNullable(clazz.cast(startPeriod()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function