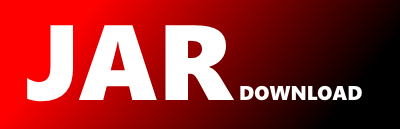
software.amazon.awssdk.services.ecs.model.SubmitTaskStateChangeRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class SubmitTaskStateChangeRequest extends EcsRequest implements
ToCopyableBuilder {
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cluster")
.getter(getter(SubmitTaskStateChangeRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField TASK_FIELD = SdkField. builder(MarshallingType.STRING).memberName("task")
.getter(getter(SubmitTaskStateChangeRequest::task)).setter(setter(Builder::task))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("task").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(SubmitTaskStateChangeRequest::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField REASON_FIELD = SdkField. builder(MarshallingType.STRING).memberName("reason")
.getter(getter(SubmitTaskStateChangeRequest::reason)).setter(setter(Builder::reason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("reason").build()).build();
private static final SdkField> CONTAINERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("containers")
.getter(getter(SubmitTaskStateChangeRequest::containers))
.setter(setter(Builder::containers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ContainerStateChange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ATTACHMENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("attachments")
.getter(getter(SubmitTaskStateChangeRequest::attachments))
.setter(setter(Builder::attachments))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attachments").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AttachmentStateChange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PULL_STARTED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("pullStartedAt").getter(getter(SubmitTaskStateChangeRequest::pullStartedAt))
.setter(setter(Builder::pullStartedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pullStartedAt").build()).build();
private static final SdkField PULL_STOPPED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("pullStoppedAt").getter(getter(SubmitTaskStateChangeRequest::pullStoppedAt))
.setter(setter(Builder::pullStoppedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pullStoppedAt").build()).build();
private static final SdkField EXECUTION_STOPPED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("executionStoppedAt").getter(getter(SubmitTaskStateChangeRequest::executionStoppedAt))
.setter(setter(Builder::executionStoppedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("executionStoppedAt").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_FIELD, TASK_FIELD,
STATUS_FIELD, REASON_FIELD, CONTAINERS_FIELD, ATTACHMENTS_FIELD, PULL_STARTED_AT_FIELD, PULL_STOPPED_AT_FIELD,
EXECUTION_STOPPED_AT_FIELD));
private final String cluster;
private final String task;
private final String status;
private final String reason;
private final List containers;
private final List attachments;
private final Instant pullStartedAt;
private final Instant pullStoppedAt;
private final Instant executionStoppedAt;
private SubmitTaskStateChangeRequest(BuilderImpl builder) {
super(builder);
this.cluster = builder.cluster;
this.task = builder.task;
this.status = builder.status;
this.reason = builder.reason;
this.containers = builder.containers;
this.attachments = builder.attachments;
this.pullStartedAt = builder.pullStartedAt;
this.pullStoppedAt = builder.pullStoppedAt;
this.executionStoppedAt = builder.executionStoppedAt;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster that hosts the task.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster that hosts the task.
*/
public String cluster() {
return cluster;
}
/**
*
* The task ID or full ARN of the task in the state change request.
*
*
* @return The task ID or full ARN of the task in the state change request.
*/
public String task() {
return task;
}
/**
*
* The status of the state change request.
*
*
* @return The status of the state change request.
*/
public String status() {
return status;
}
/**
*
* The reason for the state change request.
*
*
* @return The reason for the state change request.
*/
public String reason() {
return reason;
}
/**
* Returns true if the Containers property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasContainers() {
return containers != null && !(containers instanceof SdkAutoConstructList);
}
/**
*
* Any containers associated with the state change request.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasContainers()} to see if a value was sent in this field.
*
*
* @return Any containers associated with the state change request.
*/
public List containers() {
return containers;
}
/**
* Returns true if the Attachments property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasAttachments() {
return attachments != null && !(attachments instanceof SdkAutoConstructList);
}
/**
*
* Any attachments associated with the state change request.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasAttachments()} to see if a value was sent in this field.
*
*
* @return Any attachments associated with the state change request.
*/
public List attachments() {
return attachments;
}
/**
*
* The Unix timestamp for when the container image pull began.
*
*
* @return The Unix timestamp for when the container image pull began.
*/
public Instant pullStartedAt() {
return pullStartedAt;
}
/**
*
* The Unix timestamp for when the container image pull completed.
*
*
* @return The Unix timestamp for when the container image pull completed.
*/
public Instant pullStoppedAt() {
return pullStoppedAt;
}
/**
*
* The Unix timestamp for when the task execution stopped.
*
*
* @return The Unix timestamp for when the task execution stopped.
*/
public Instant executionStoppedAt() {
return executionStoppedAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(task());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(reason());
hashCode = 31 * hashCode + Objects.hashCode(hasContainers() ? containers() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasAttachments() ? attachments() : null);
hashCode = 31 * hashCode + Objects.hashCode(pullStartedAt());
hashCode = 31 * hashCode + Objects.hashCode(pullStoppedAt());
hashCode = 31 * hashCode + Objects.hashCode(executionStoppedAt());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SubmitTaskStateChangeRequest)) {
return false;
}
SubmitTaskStateChangeRequest other = (SubmitTaskStateChangeRequest) obj;
return Objects.equals(cluster(), other.cluster()) && Objects.equals(task(), other.task())
&& Objects.equals(status(), other.status()) && Objects.equals(reason(), other.reason())
&& hasContainers() == other.hasContainers() && Objects.equals(containers(), other.containers())
&& hasAttachments() == other.hasAttachments() && Objects.equals(attachments(), other.attachments())
&& Objects.equals(pullStartedAt(), other.pullStartedAt())
&& Objects.equals(pullStoppedAt(), other.pullStoppedAt())
&& Objects.equals(executionStoppedAt(), other.executionStoppedAt());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("SubmitTaskStateChangeRequest").add("Cluster", cluster()).add("Task", task())
.add("Status", status()).add("Reason", reason()).add("Containers", hasContainers() ? containers() : null)
.add("Attachments", hasAttachments() ? attachments() : null).add("PullStartedAt", pullStartedAt())
.add("PullStoppedAt", pullStoppedAt()).add("ExecutionStoppedAt", executionStoppedAt()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "task":
return Optional.ofNullable(clazz.cast(task()));
case "status":
return Optional.ofNullable(clazz.cast(status()));
case "reason":
return Optional.ofNullable(clazz.cast(reason()));
case "containers":
return Optional.ofNullable(clazz.cast(containers()));
case "attachments":
return Optional.ofNullable(clazz.cast(attachments()));
case "pullStartedAt":
return Optional.ofNullable(clazz.cast(pullStartedAt()));
case "pullStoppedAt":
return Optional.ofNullable(clazz.cast(pullStoppedAt()));
case "executionStoppedAt":
return Optional.ofNullable(clazz.cast(executionStoppedAt()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function