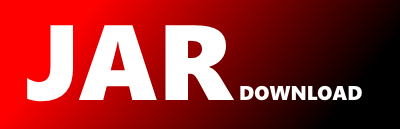
software.amazon.awssdk.services.ecs.model.CreateServiceRequest Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateServiceRequest extends EcsRequest implements
ToCopyableBuilder {
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cluster")
.getter(getter(CreateServiceRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField SERVICE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("serviceName").getter(getter(CreateServiceRequest::serviceName)).setter(setter(Builder::serviceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceName").build()).build();
private static final SdkField TASK_DEFINITION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskDefinition").getter(getter(CreateServiceRequest::taskDefinition))
.setter(setter(Builder::taskDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinition").build()).build();
private static final SdkField> LOAD_BALANCERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("loadBalancers")
.getter(getter(CreateServiceRequest::loadBalancers))
.setter(setter(Builder::loadBalancers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("loadBalancers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LoadBalancer::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SERVICE_REGISTRIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("serviceRegistries")
.getter(getter(CreateServiceRequest::serviceRegistries))
.setter(setter(Builder::serviceRegistries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRegistries").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceRegistry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DESIRED_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("desiredCount").getter(getter(CreateServiceRequest::desiredCount)).setter(setter(Builder::desiredCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("desiredCount").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("clientToken").getter(getter(CreateServiceRequest::clientToken)).setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clientToken").build()).build();
private static final SdkField LAUNCH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("launchType").getter(getter(CreateServiceRequest::launchTypeAsString))
.setter(setter(Builder::launchType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("launchType").build()).build();
private static final SdkField> CAPACITY_PROVIDER_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("capacityProviderStrategy")
.getter(getter(CreateServiceRequest::capacityProviderStrategy))
.setter(setter(Builder::capacityProviderStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProviderStrategy").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CapacityProviderStrategyItem::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PLATFORM_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformVersion").getter(getter(CreateServiceRequest::platformVersion))
.setter(setter(Builder::platformVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformVersion").build()).build();
private static final SdkField ROLE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("role")
.getter(getter(CreateServiceRequest::role)).setter(setter(Builder::role))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("role").build()).build();
private static final SdkField DEPLOYMENT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("deploymentConfiguration")
.getter(getter(CreateServiceRequest::deploymentConfiguration)).setter(setter(Builder::deploymentConfiguration))
.constructor(DeploymentConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentConfiguration").build())
.build();
private static final SdkField> PLACEMENT_CONSTRAINTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("placementConstraints")
.getter(getter(CreateServiceRequest::placementConstraints))
.setter(setter(Builder::placementConstraints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("placementConstraints").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PlacementConstraint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> PLACEMENT_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("placementStrategy")
.getter(getter(CreateServiceRequest::placementStrategy))
.setter(setter(Builder::placementStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("placementStrategy").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PlacementStrategy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField NETWORK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("networkConfiguration")
.getter(getter(CreateServiceRequest::networkConfiguration)).setter(setter(Builder::networkConfiguration))
.constructor(NetworkConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkConfiguration").build())
.build();
private static final SdkField HEALTH_CHECK_GRACE_PERIOD_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("healthCheckGracePeriodSeconds")
.getter(getter(CreateServiceRequest::healthCheckGracePeriodSeconds))
.setter(setter(Builder::healthCheckGracePeriodSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("healthCheckGracePeriodSeconds")
.build()).build();
private static final SdkField SCHEDULING_STRATEGY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("schedulingStrategy").getter(getter(CreateServiceRequest::schedulingStrategyAsString))
.setter(setter(Builder::schedulingStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("schedulingStrategy").build())
.build();
private static final SdkField DEPLOYMENT_CONTROLLER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("deploymentController")
.getter(getter(CreateServiceRequest::deploymentController)).setter(setter(Builder::deploymentController))
.constructor(DeploymentController::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentController").build())
.build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tags")
.getter(getter(CreateServiceRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ENABLE_ECS_MANAGED_TAGS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("enableECSManagedTags").getter(getter(CreateServiceRequest::enableECSManagedTags))
.setter(setter(Builder::enableECSManagedTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enableECSManagedTags").build())
.build();
private static final SdkField PROPAGATE_TAGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("propagateTags").getter(getter(CreateServiceRequest::propagateTagsAsString))
.setter(setter(Builder::propagateTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("propagateTags").build()).build();
private static final SdkField ENABLE_EXECUTE_COMMAND_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("enableExecuteCommand").getter(getter(CreateServiceRequest::enableExecuteCommand))
.setter(setter(Builder::enableExecuteCommand))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enableExecuteCommand").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_FIELD,
SERVICE_NAME_FIELD, TASK_DEFINITION_FIELD, LOAD_BALANCERS_FIELD, SERVICE_REGISTRIES_FIELD, DESIRED_COUNT_FIELD,
CLIENT_TOKEN_FIELD, LAUNCH_TYPE_FIELD, CAPACITY_PROVIDER_STRATEGY_FIELD, PLATFORM_VERSION_FIELD, ROLE_FIELD,
DEPLOYMENT_CONFIGURATION_FIELD, PLACEMENT_CONSTRAINTS_FIELD, PLACEMENT_STRATEGY_FIELD, NETWORK_CONFIGURATION_FIELD,
HEALTH_CHECK_GRACE_PERIOD_SECONDS_FIELD, SCHEDULING_STRATEGY_FIELD, DEPLOYMENT_CONTROLLER_FIELD, TAGS_FIELD,
ENABLE_ECS_MANAGED_TAGS_FIELD, PROPAGATE_TAGS_FIELD, ENABLE_EXECUTE_COMMAND_FIELD));
private final String cluster;
private final String serviceName;
private final String taskDefinition;
private final List loadBalancers;
private final List serviceRegistries;
private final Integer desiredCount;
private final String clientToken;
private final String launchType;
private final List capacityProviderStrategy;
private final String platformVersion;
private final String role;
private final DeploymentConfiguration deploymentConfiguration;
private final List placementConstraints;
private final List placementStrategy;
private final NetworkConfiguration networkConfiguration;
private final Integer healthCheckGracePeriodSeconds;
private final String schedulingStrategy;
private final DeploymentController deploymentController;
private final List tags;
private final Boolean enableECSManagedTags;
private final String propagateTags;
private final Boolean enableExecuteCommand;
private CreateServiceRequest(BuilderImpl builder) {
super(builder);
this.cluster = builder.cluster;
this.serviceName = builder.serviceName;
this.taskDefinition = builder.taskDefinition;
this.loadBalancers = builder.loadBalancers;
this.serviceRegistries = builder.serviceRegistries;
this.desiredCount = builder.desiredCount;
this.clientToken = builder.clientToken;
this.launchType = builder.launchType;
this.capacityProviderStrategy = builder.capacityProviderStrategy;
this.platformVersion = builder.platformVersion;
this.role = builder.role;
this.deploymentConfiguration = builder.deploymentConfiguration;
this.placementConstraints = builder.placementConstraints;
this.placementStrategy = builder.placementStrategy;
this.networkConfiguration = builder.networkConfiguration;
this.healthCheckGracePeriodSeconds = builder.healthCheckGracePeriodSeconds;
this.schedulingStrategy = builder.schedulingStrategy;
this.deploymentController = builder.deploymentController;
this.tags = builder.tags;
this.enableECSManagedTags = builder.enableECSManagedTags;
this.propagateTags = builder.propagateTags;
this.enableExecuteCommand = builder.enableExecuteCommand;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster on which to run your service. If you do not
* specify a cluster, the default cluster is assumed.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster on which to run your service. If you do
* not specify a cluster, the default cluster is assumed.
*/
public final String cluster() {
return cluster;
}
/**
*
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, and hyphens are allowed. Service
* names must be unique within a cluster, but you can have similarly named services in multiple clusters within a
* Region or across multiple Regions.
*
*
* @return The name of your service. Up to 255 letters (uppercase and lowercase), numbers, and hyphens are allowed.
* Service names must be unique within a cluster, but you can have similarly named services in multiple
* clusters within a Region or across multiple Regions.
*/
public final String serviceName() {
return serviceName;
}
/**
*
* The family
and revision
(family:revision
) or full ARN of the task
* definition to run in your service. If a revision
is not specified, the latest ACTIVE
* revision is used.
*
*
* A task definition must be specified if the service is using either the ECS
or
* CODE_DEPLOY
deployment controllers.
*
*
* @return The family
and revision
(family:revision
) or full ARN of the task
* definition to run in your service. If a revision
is not specified, the latest
* ACTIVE
revision is used.
*
* A task definition must be specified if the service is using either the ECS
or
* CODE_DEPLOY
deployment controllers.
*/
public final String taskDefinition() {
return taskDefinition;
}
/**
* Returns true if the LoadBalancers property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasLoadBalancers() {
return loadBalancers != null && !(loadBalancers instanceof SdkAutoConstructList);
}
/**
*
* A load balancer object representing the load balancers to use with your service. For more information, see Service Load
* Balancing in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service is using the rolling update (ECS
) deployment controller and using either an
* Application Load Balancer or Network Load Balancer, you must specify one or more target group ARNs to attach to
* the service. The service-linked role is required for services that make use of multiple target groups. For more
* information, see Using
* service-linked roles for Amazon ECS in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service is using the CODE_DEPLOY
deployment controller, the service is required to use either
* an Application Load Balancer or Network Load Balancer. When creating an AWS CodeDeploy deployment group, you
* specify two target groups (referred to as a targetGroupPair
). During a deployment, AWS CodeDeploy
* determines which task set in your service has the status PRIMARY
and associates one target group
* with it, and then associates the other target group with the replacement task set. The load balancer can also
* have up to two listeners: a required listener for production traffic and an optional listener that allows you
* perform validation tests with Lambda functions before routing production traffic to it.
*
*
* After you create a service using the ECS
deployment controller, the load balancer name or target
* group ARN, container name, and container port specified in the service definition are immutable. If you are using
* the CODE_DEPLOY
deployment controller, these values can be changed when updating the service.
*
*
* For Application Load Balancers and Network Load Balancers, this object must contain the load balancer target
* group ARN, the container name (as it appears in a container definition), and the container port to access from
* the load balancer. The load balancer name parameter must be omitted. When a task from this service is placed on a
* container instance, the container instance and port combination is registered as a target in the target group
* specified here.
*
*
* For Classic Load Balancers, this object must contain the load balancer name, the container name (as it appears in
* a container definition), and the container port to access from the load balancer. The target group ARN parameter
* must be omitted. When a task from this service is placed on a container instance, the container instance is
* registered with the load balancer specified here.
*
*
* Services with tasks that use the awsvpc
network mode (for example, those with the Fargate launch
* type) only support Application Load Balancers and Network Load Balancers. Classic Load Balancers are not
* supported. Also, when you create any target groups for these services, you must choose ip
as the
* target type, not instance
, because tasks that use the awsvpc
network mode are
* associated with an elastic network interface, not an Amazon EC2 instance.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasLoadBalancers()} to see if a value was sent in this field.
*
*
* @return A load balancer object representing the load balancers to use with your service. For more information,
* see Service
* Load Balancing in the Amazon Elastic Container Service Developer Guide.
*
* If the service is using the rolling update (ECS
) deployment controller and using either an
* Application Load Balancer or Network Load Balancer, you must specify one or more target group ARNs to
* attach to the service. The service-linked role is required for services that make use of multiple target
* groups. For more information, see Using
* service-linked roles for Amazon ECS in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service is using the CODE_DEPLOY
deployment controller, the service is required to
* use either an Application Load Balancer or Network Load Balancer. When creating an AWS CodeDeploy
* deployment group, you specify two target groups (referred to as a targetGroupPair
). During a
* deployment, AWS CodeDeploy determines which task set in your service has the status PRIMARY
* and associates one target group with it, and then associates the other target group with the replacement
* task set. The load balancer can also have up to two listeners: a required listener for production traffic
* and an optional listener that allows you perform validation tests with Lambda functions before routing
* production traffic to it.
*
*
* After you create a service using the ECS
deployment controller, the load balancer name or
* target group ARN, container name, and container port specified in the service definition are immutable.
* If you are using the CODE_DEPLOY
deployment controller, these values can be changed when
* updating the service.
*
*
* For Application Load Balancers and Network Load Balancers, this object must contain the load balancer
* target group ARN, the container name (as it appears in a container definition), and the container port to
* access from the load balancer. The load balancer name parameter must be omitted. When a task from this
* service is placed on a container instance, the container instance and port combination is registered as a
* target in the target group specified here.
*
*
* For Classic Load Balancers, this object must contain the load balancer name, the container name (as it
* appears in a container definition), and the container port to access from the load balancer. The target
* group ARN parameter must be omitted. When a task from this service is placed on a container instance, the
* container instance is registered with the load balancer specified here.
*
*
* Services with tasks that use the awsvpc
network mode (for example, those with the Fargate
* launch type) only support Application Load Balancers and Network Load Balancers. Classic Load Balancers
* are not supported. Also, when you create any target groups for these services, you must choose
* ip
as the target type, not instance
, because tasks that use the
* awsvpc
network mode are associated with an elastic network interface, not an Amazon EC2
* instance.
*/
public final List loadBalancers() {
return loadBalancers;
}
/**
* Returns true if the ServiceRegistries property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasServiceRegistries() {
return serviceRegistries != null && !(serviceRegistries instanceof SdkAutoConstructList);
}
/**
*
* The details of the service discovery registry to associate with this service. For more information, see Service discovery.
*
*
*
* Each service may be associated with one service registry. Multiple service registries per service isn't
* supported.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasServiceRegistries()} to see if a value was sent in this field.
*
*
* @return The details of the service discovery registry to associate with this service. For more information, see
* Service
* discovery.
*
* Each service may be associated with one service registry. Multiple service registries per service isn't
* supported.
*
*/
public final List serviceRegistries() {
return serviceRegistries;
}
/**
*
* The number of instantiations of the specified task definition to place and keep running on your cluster.
*
*
* This is required if schedulingStrategy
is REPLICA
or is not specified. If
* schedulingStrategy
is DAEMON
then this is not required.
*
*
* @return The number of instantiations of the specified task definition to place and keep running on your
* cluster.
*
* This is required if schedulingStrategy
is REPLICA
or is not specified. If
* schedulingStrategy
is DAEMON
then this is not required.
*/
public final Integer desiredCount() {
return desiredCount;
}
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Up to 32 ASCII
* characters are allowed.
*
*
* @return Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Up to 32
* ASCII characters are allowed.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* The launch type on which to run your service. The accepted values are FARGATE
and EC2
.
* For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the FARGATE_SPOT
* capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered to your
* cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be omitted.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type on which to run your service. The accepted values are FARGATE
and
* EC2
. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the
* FARGATE_SPOT
capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered
* to your cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be
* omitted.
* @see LaunchType
*/
public final LaunchType launchType() {
return LaunchType.fromValue(launchType);
}
/**
*
* The launch type on which to run your service. The accepted values are FARGATE
and EC2
.
* For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the FARGATE_SPOT
* capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered to your
* cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be omitted.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type on which to run your service. The accepted values are FARGATE
and
* EC2
. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the
* FARGATE_SPOT
capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered
* to your cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be
* omitted.
* @see LaunchType
*/
public final String launchTypeAsString() {
return launchType;
}
/**
* Returns true if the CapacityProviderStrategy property was specified by the sender (it may be empty), or false if
* the sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasCapacityProviderStrategy() {
return capacityProviderStrategy != null && !(capacityProviderStrategy instanceof SdkAutoConstructList);
}
/**
*
* The capacity provider strategy to use for the service.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasCapacityProviderStrategy()} to see if a value was sent in this field.
*
*
* @return The capacity provider strategy to use for the service.
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be
* omitted. If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*/
public final List capacityProviderStrategy() {
return capacityProviderStrategy;
}
/**
*
* The platform version that your tasks in the service are running on. A platform version is specified only for
* tasks using the Fargate launch type. If one isn't specified, the LATEST
platform version is used by
* default. For more information, see AWS Fargate platform
* versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The platform version that your tasks in the service are running on. A platform version is specified only
* for tasks using the Fargate launch type. If one isn't specified, the LATEST
platform version
* is used by default. For more information, see AWS Fargate
* platform versions in the Amazon Elastic Container Service Developer Guide.
*/
public final String platformVersion() {
return platformVersion;
}
/**
*
* The name or full Amazon Resource Name (ARN) of the IAM role that allows Amazon ECS to make calls to your load
* balancer on your behalf. This parameter is only permitted if you are using a load balancer with your service and
* your task definition does not use the awsvpc
network mode. If you specify the role
* parameter, you must also specify a load balancer object with the loadBalancers
parameter.
*
*
*
* If your account has already created the Amazon ECS service-linked role, that role is used by default for your
* service unless you specify a role here. The service-linked role is required if your task definition uses the
* awsvpc
network mode or if the service is configured to use service discovery, an external deployment
* controller, multiple target groups, or Elastic Inference accelerators in which case you should not specify a role
* here. For more information, see Using
* service-linked roles for Amazon ECS in the Amazon Elastic Container Service Developer Guide.
*
*
*
* If your specified role has a path other than /
, then you must either specify the full role ARN (this
* is recommended) or prefix the role name with the path. For example, if a role with the name bar
has
* a path of /foo/
then you would specify /foo/bar
as the role name. For more information,
* see Friendly names and paths in the IAM User Guide.
*
*
* @return The name or full Amazon Resource Name (ARN) of the IAM role that allows Amazon ECS to make calls to your
* load balancer on your behalf. This parameter is only permitted if you are using a load balancer with your
* service and your task definition does not use the awsvpc
network mode. If you specify the
* role
parameter, you must also specify a load balancer object with the
* loadBalancers
parameter.
*
* If your account has already created the Amazon ECS service-linked role, that role is used by default for
* your service unless you specify a role here. The service-linked role is required if your task definition
* uses the awsvpc
network mode or if the service is configured to use service discovery, an
* external deployment controller, multiple target groups, or Elastic Inference accelerators in which case
* you should not specify a role here. For more information, see Using
* service-linked roles for Amazon ECS in the Amazon Elastic Container Service Developer Guide.
*
*
*
* If your specified role has a path other than /
, then you must either specify the full role
* ARN (this is recommended) or prefix the role name with the path. For example, if a role with the name
* bar
has a path of /foo/
then you would specify /foo/bar
as the
* role name. For more information, see Friendly names and paths in the IAM User Guide.
*/
public final String role() {
return role;
}
/**
*
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of stopping
* and starting tasks.
*
*
* @return Optional deployment parameters that control how many tasks run during the deployment and the ordering of
* stopping and starting tasks.
*/
public final DeploymentConfiguration deploymentConfiguration() {
return deploymentConfiguration;
}
/**
* Returns true if the PlacementConstraints property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasPlacementConstraints() {
return placementConstraints != null && !(placementConstraints instanceof SdkAutoConstructList);
}
/**
*
* An array of placement constraint objects to use for tasks in your service. You can specify a maximum of 10
* constraints per task (this limit includes constraints in the task definition and those specified at runtime).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasPlacementConstraints()} to see if a value was sent in this field.
*
*
* @return An array of placement constraint objects to use for tasks in your service. You can specify a maximum of
* 10 constraints per task (this limit includes constraints in the task definition and those specified at
* runtime).
*/
public final List placementConstraints() {
return placementConstraints;
}
/**
* Returns true if the PlacementStrategy property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasPlacementStrategy() {
return placementStrategy != null && !(placementStrategy instanceof SdkAutoConstructList);
}
/**
*
* The placement strategy objects to use for tasks in your service. You can specify a maximum of five strategy rules
* per service.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasPlacementStrategy()} to see if a value was sent in this field.
*
*
* @return The placement strategy objects to use for tasks in your service. You can specify a maximum of five
* strategy rules per service.
*/
public final List placementStrategy() {
return placementStrategy;
}
/**
*
* The network configuration for the service. This parameter is required for task definitions that use the
* awsvpc
network mode to receive their own elastic network interface, and it is not supported for
* other network modes. For more information, see Task networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return The network configuration for the service. This parameter is required for task definitions that use the
* awsvpc
network mode to receive their own elastic network interface, and it is not supported
* for other network modes. For more information, see Task
* networking in the Amazon Elastic Container Service Developer Guide.
*/
public final NetworkConfiguration networkConfiguration() {
return networkConfiguration;
}
/**
*
* The period of time, in seconds, that the Amazon ECS service scheduler should ignore unhealthy Elastic Load
* Balancing target health checks after a task has first started. This is only used when your service is configured
* to use a load balancer. If your service has a load balancer defined and you don't specify a health check grace
* period value, the default value of 0
is used.
*
*
* If your service's tasks take a while to start and respond to Elastic Load Balancing health checks, you can
* specify a health check grace period of up to 2,147,483,647 seconds. During that time, the Amazon ECS service
* scheduler ignores health check status. This grace period can prevent the service scheduler from marking tasks as
* unhealthy and stopping them before they have time to come up.
*
*
* @return The period of time, in seconds, that the Amazon ECS service scheduler should ignore unhealthy Elastic
* Load Balancing target health checks after a task has first started. This is only used when your service
* is configured to use a load balancer. If your service has a load balancer defined and you don't specify a
* health check grace period value, the default value of 0
is used.
*
* If your service's tasks take a while to start and respond to Elastic Load Balancing health checks, you
* can specify a health check grace period of up to 2,147,483,647 seconds. During that time, the Amazon ECS
* service scheduler ignores health check status. This grace period can prevent the service scheduler from
* marking tasks as unhealthy and stopping them before they have time to come up.
*/
public final Integer healthCheckGracePeriodSeconds() {
return healthCheckGracePeriodSeconds;
}
/**
*
* The scheduling strategy to use for the service. For more information, see Services.
*
*
* There are two service scheduler strategies available:
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks across your
* cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task placement
* strategies and constraints to customize task placement decisions. This scheduler strategy is required if the
* service is using the CODE_DEPLOY
or EXTERNAL
deployment controller types.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container instance
* that meets all of the task placement constraints that you specify in your cluster. The service scheduler also
* evaluates the task placement constraints for running tasks and will stop tasks that do not meet the placement
* constraints. When you're using this strategy, you don't need to specify a desired number of tasks, a task
* placement strategy, or use Service Auto Scaling policies.
*
*
*
* Tasks using the Fargate launch type or the CODE_DEPLOY
or EXTERNAL
deployment
* controller types don't support the DAEMON
scheduling strategy.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #schedulingStrategy} will return {@link SchedulingStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #schedulingStrategyAsString}.
*
*
* @return The scheduling strategy to use for the service. For more information, see Services.
*
* There are two service scheduler strategies available:
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks
* across your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can
* use task placement strategies and constraints to customize task placement decisions. This scheduler
* strategy is required if the service is using the CODE_DEPLOY
or EXTERNAL
* deployment controller types.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container
* instance that meets all of the task placement constraints that you specify in your cluster. The service
* scheduler also evaluates the task placement constraints for running tasks and will stop tasks that do not
* meet the placement constraints. When you're using this strategy, you don't need to specify a desired
* number of tasks, a task placement strategy, or use Service Auto Scaling policies.
*
*
*
* Tasks using the Fargate launch type or the CODE_DEPLOY
or EXTERNAL
deployment
* controller types don't support the DAEMON
scheduling strategy.
*
*
* @see SchedulingStrategy
*/
public final SchedulingStrategy schedulingStrategy() {
return SchedulingStrategy.fromValue(schedulingStrategy);
}
/**
*
* The scheduling strategy to use for the service. For more information, see Services.
*
*
* There are two service scheduler strategies available:
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks across your
* cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task placement
* strategies and constraints to customize task placement decisions. This scheduler strategy is required if the
* service is using the CODE_DEPLOY
or EXTERNAL
deployment controller types.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container instance
* that meets all of the task placement constraints that you specify in your cluster. The service scheduler also
* evaluates the task placement constraints for running tasks and will stop tasks that do not meet the placement
* constraints. When you're using this strategy, you don't need to specify a desired number of tasks, a task
* placement strategy, or use Service Auto Scaling policies.
*
*
*
* Tasks using the Fargate launch type or the CODE_DEPLOY
or EXTERNAL
deployment
* controller types don't support the DAEMON
scheduling strategy.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #schedulingStrategy} will return {@link SchedulingStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #schedulingStrategyAsString}.
*
*
* @return The scheduling strategy to use for the service. For more information, see Services.
*
* There are two service scheduler strategies available:
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks
* across your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can
* use task placement strategies and constraints to customize task placement decisions. This scheduler
* strategy is required if the service is using the CODE_DEPLOY
or EXTERNAL
* deployment controller types.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container
* instance that meets all of the task placement constraints that you specify in your cluster. The service
* scheduler also evaluates the task placement constraints for running tasks and will stop tasks that do not
* meet the placement constraints. When you're using this strategy, you don't need to specify a desired
* number of tasks, a task placement strategy, or use Service Auto Scaling policies.
*
*
*
* Tasks using the Fargate launch type or the CODE_DEPLOY
or EXTERNAL
deployment
* controller types don't support the DAEMON
scheduling strategy.
*
*
* @see SchedulingStrategy
*/
public final String schedulingStrategyAsString() {
return schedulingStrategy;
}
/**
*
* The deployment controller to use for the service. If no deployment controller is specified, the default value of
* ECS
is used.
*
*
* @return The deployment controller to use for the service. If no deployment controller is specified, the default
* value of ECS
is used.
*/
public final DeploymentController deploymentController() {
return deploymentController;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The metadata that you apply to the service to help you categorize and organize them. Each tag consists of a key
* and an optional value, both of which you define. When a service is deleted, the tags are deleted as well.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return The metadata that you apply to the service to help you categorize and organize them. Each tag consists of
* a key and an optional value, both of which you define. When a service is deleted, the tags are deleted as
* well.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or
* values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*/
public final List tags() {
return tags;
}
/**
*
* Specifies whether to enable Amazon ECS managed tags for the tasks within the service. For more information, see
* Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Specifies whether to enable Amazon ECS managed tags for the tasks within the service. For more
* information, see Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public final Boolean enableECSManagedTags() {
return enableECSManagedTags;
}
/**
*
* Specifies whether to propagate the tags from the task definition or the service to the tasks in the service. If
* no value is specified, the tags are not propagated. Tags can only be propagated to the tasks within the service
* during service creation. To add tags to a task after service creation, use the TagResource API action.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #propagateTags}
* will return {@link PropagateTags#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #propagateTagsAsString}.
*
*
* @return Specifies whether to propagate the tags from the task definition or the service to the tasks in the
* service. If no value is specified, the tags are not propagated. Tags can only be propagated to the tasks
* within the service during service creation. To add tags to a task after service creation, use the
* TagResource API action.
* @see PropagateTags
*/
public final PropagateTags propagateTags() {
return PropagateTags.fromValue(propagateTags);
}
/**
*
* Specifies whether to propagate the tags from the task definition or the service to the tasks in the service. If
* no value is specified, the tags are not propagated. Tags can only be propagated to the tasks within the service
* during service creation. To add tags to a task after service creation, use the TagResource API action.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #propagateTags}
* will return {@link PropagateTags#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #propagateTagsAsString}.
*
*
* @return Specifies whether to propagate the tags from the task definition or the service to the tasks in the
* service. If no value is specified, the tags are not propagated. Tags can only be propagated to the tasks
* within the service during service creation. To add tags to a task after service creation, use the
* TagResource API action.
* @see PropagateTags
*/
public final String propagateTagsAsString() {
return propagateTags;
}
/**
*
* Whether or not the execute command functionality is enabled for the service. If true
, this enables
* execute command functionality on all containers in the service tasks.
*
*
* @return Whether or not the execute command functionality is enabled for the service. If true
, this
* enables execute command functionality on all containers in the service tasks.
*/
public final Boolean enableExecuteCommand() {
return enableExecuteCommand;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(serviceName());
hashCode = 31 * hashCode + Objects.hashCode(taskDefinition());
hashCode = 31 * hashCode + Objects.hashCode(hasLoadBalancers() ? loadBalancers() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasServiceRegistries() ? serviceRegistries() : null);
hashCode = 31 * hashCode + Objects.hashCode(desiredCount());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(launchTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasCapacityProviderStrategy() ? capacityProviderStrategy() : null);
hashCode = 31 * hashCode + Objects.hashCode(platformVersion());
hashCode = 31 * hashCode + Objects.hashCode(role());
hashCode = 31 * hashCode + Objects.hashCode(deploymentConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasPlacementConstraints() ? placementConstraints() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasPlacementStrategy() ? placementStrategy() : null);
hashCode = 31 * hashCode + Objects.hashCode(networkConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckGracePeriodSeconds());
hashCode = 31 * hashCode + Objects.hashCode(schedulingStrategyAsString());
hashCode = 31 * hashCode + Objects.hashCode(deploymentController());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(enableECSManagedTags());
hashCode = 31 * hashCode + Objects.hashCode(propagateTagsAsString());
hashCode = 31 * hashCode + Objects.hashCode(enableExecuteCommand());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateServiceRequest)) {
return false;
}
CreateServiceRequest other = (CreateServiceRequest) obj;
return Objects.equals(cluster(), other.cluster()) && Objects.equals(serviceName(), other.serviceName())
&& Objects.equals(taskDefinition(), other.taskDefinition()) && hasLoadBalancers() == other.hasLoadBalancers()
&& Objects.equals(loadBalancers(), other.loadBalancers())
&& hasServiceRegistries() == other.hasServiceRegistries()
&& Objects.equals(serviceRegistries(), other.serviceRegistries())
&& Objects.equals(desiredCount(), other.desiredCount()) && Objects.equals(clientToken(), other.clientToken())
&& Objects.equals(launchTypeAsString(), other.launchTypeAsString())
&& hasCapacityProviderStrategy() == other.hasCapacityProviderStrategy()
&& Objects.equals(capacityProviderStrategy(), other.capacityProviderStrategy())
&& Objects.equals(platformVersion(), other.platformVersion()) && Objects.equals(role(), other.role())
&& Objects.equals(deploymentConfiguration(), other.deploymentConfiguration())
&& hasPlacementConstraints() == other.hasPlacementConstraints()
&& Objects.equals(placementConstraints(), other.placementConstraints())
&& hasPlacementStrategy() == other.hasPlacementStrategy()
&& Objects.equals(placementStrategy(), other.placementStrategy())
&& Objects.equals(networkConfiguration(), other.networkConfiguration())
&& Objects.equals(healthCheckGracePeriodSeconds(), other.healthCheckGracePeriodSeconds())
&& Objects.equals(schedulingStrategyAsString(), other.schedulingStrategyAsString())
&& Objects.equals(deploymentController(), other.deploymentController()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(enableECSManagedTags(), other.enableECSManagedTags())
&& Objects.equals(propagateTagsAsString(), other.propagateTagsAsString())
&& Objects.equals(enableExecuteCommand(), other.enableExecuteCommand());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateServiceRequest").add("Cluster", cluster()).add("ServiceName", serviceName())
.add("TaskDefinition", taskDefinition()).add("LoadBalancers", hasLoadBalancers() ? loadBalancers() : null)
.add("ServiceRegistries", hasServiceRegistries() ? serviceRegistries() : null)
.add("DesiredCount", desiredCount()).add("ClientToken", clientToken()).add("LaunchType", launchTypeAsString())
.add("CapacityProviderStrategy", hasCapacityProviderStrategy() ? capacityProviderStrategy() : null)
.add("PlatformVersion", platformVersion()).add("Role", role())
.add("DeploymentConfiguration", deploymentConfiguration())
.add("PlacementConstraints", hasPlacementConstraints() ? placementConstraints() : null)
.add("PlacementStrategy", hasPlacementStrategy() ? placementStrategy() : null)
.add("NetworkConfiguration", networkConfiguration())
.add("HealthCheckGracePeriodSeconds", healthCheckGracePeriodSeconds())
.add("SchedulingStrategy", schedulingStrategyAsString()).add("DeploymentController", deploymentController())
.add("Tags", hasTags() ? tags() : null).add("EnableECSManagedTags", enableECSManagedTags())
.add("PropagateTags", propagateTagsAsString()).add("EnableExecuteCommand", enableExecuteCommand()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "serviceName":
return Optional.ofNullable(clazz.cast(serviceName()));
case "taskDefinition":
return Optional.ofNullable(clazz.cast(taskDefinition()));
case "loadBalancers":
return Optional.ofNullable(clazz.cast(loadBalancers()));
case "serviceRegistries":
return Optional.ofNullable(clazz.cast(serviceRegistries()));
case "desiredCount":
return Optional.ofNullable(clazz.cast(desiredCount()));
case "clientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "launchType":
return Optional.ofNullable(clazz.cast(launchTypeAsString()));
case "capacityProviderStrategy":
return Optional.ofNullable(clazz.cast(capacityProviderStrategy()));
case "platformVersion":
return Optional.ofNullable(clazz.cast(platformVersion()));
case "role":
return Optional.ofNullable(clazz.cast(role()));
case "deploymentConfiguration":
return Optional.ofNullable(clazz.cast(deploymentConfiguration()));
case "placementConstraints":
return Optional.ofNullable(clazz.cast(placementConstraints()));
case "placementStrategy":
return Optional.ofNullable(clazz.cast(placementStrategy()));
case "networkConfiguration":
return Optional.ofNullable(clazz.cast(networkConfiguration()));
case "healthCheckGracePeriodSeconds":
return Optional.ofNullable(clazz.cast(healthCheckGracePeriodSeconds()));
case "schedulingStrategy":
return Optional.ofNullable(clazz.cast(schedulingStrategyAsString()));
case "deploymentController":
return Optional.ofNullable(clazz.cast(deploymentController()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
case "enableECSManagedTags":
return Optional.ofNullable(clazz.cast(enableECSManagedTags()));
case "propagateTags":
return Optional.ofNullable(clazz.cast(propagateTagsAsString()));
case "enableExecuteCommand":
return Optional.ofNullable(clazz.cast(enableExecuteCommand()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function