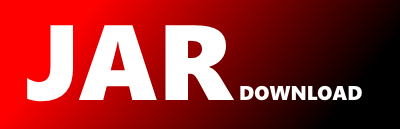
software.amazon.awssdk.services.ecs.model.ListTasksRequest Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListTasksRequest extends EcsRequest implements ToCopyableBuilder {
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cluster")
.getter(getter(ListTasksRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField CONTAINER_INSTANCE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("containerInstance").getter(getter(ListTasksRequest::containerInstance))
.setter(setter(Builder::containerInstance))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerInstance").build()).build();
private static final SdkField FAMILY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("family")
.getter(getter(ListTasksRequest::family)).setter(setter(Builder::family))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("family").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("nextToken").getter(getter(ListTasksRequest::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("nextToken").build()).build();
private static final SdkField MAX_RESULTS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("maxResults").getter(getter(ListTasksRequest::maxResults)).setter(setter(Builder::maxResults))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("maxResults").build()).build();
private static final SdkField STARTED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("startedBy").getter(getter(ListTasksRequest::startedBy)).setter(setter(Builder::startedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startedBy").build()).build();
private static final SdkField SERVICE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("serviceName").getter(getter(ListTasksRequest::serviceName)).setter(setter(Builder::serviceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceName").build()).build();
private static final SdkField DESIRED_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("desiredStatus").getter(getter(ListTasksRequest::desiredStatusAsString))
.setter(setter(Builder::desiredStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("desiredStatus").build()).build();
private static final SdkField LAUNCH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("launchType").getter(getter(ListTasksRequest::launchTypeAsString)).setter(setter(Builder::launchType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("launchType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_FIELD,
CONTAINER_INSTANCE_FIELD, FAMILY_FIELD, NEXT_TOKEN_FIELD, MAX_RESULTS_FIELD, STARTED_BY_FIELD, SERVICE_NAME_FIELD,
DESIRED_STATUS_FIELD, LAUNCH_TYPE_FIELD));
private final String cluster;
private final String containerInstance;
private final String family;
private final String nextToken;
private final Integer maxResults;
private final String startedBy;
private final String serviceName;
private final String desiredStatus;
private final String launchType;
private ListTasksRequest(BuilderImpl builder) {
super(builder);
this.cluster = builder.cluster;
this.containerInstance = builder.containerInstance;
this.family = builder.family;
this.nextToken = builder.nextToken;
this.maxResults = builder.maxResults;
this.startedBy = builder.startedBy;
this.serviceName = builder.serviceName;
this.desiredStatus = builder.desiredStatus;
this.launchType = builder.launchType;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster that hosts the tasks to list. If you do not
* specify a cluster, the default cluster is assumed.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster that hosts the tasks to list. If you do
* not specify a cluster, the default cluster is assumed.
*/
public final String cluster() {
return cluster;
}
/**
*
* The container instance ID or full ARN of the container instance with which to filter the ListTasks
* results. Specifying a containerInstance
limits the results to tasks that belong to that container
* instance.
*
*
* @return The container instance ID or full ARN of the container instance with which to filter the
* ListTasks
results. Specifying a containerInstance
limits the results to tasks
* that belong to that container instance.
*/
public final String containerInstance() {
return containerInstance;
}
/**
*
* The name of the family with which to filter the ListTasks
results. Specifying a family
* limits the results to tasks that belong to that family.
*
*
* @return The name of the family with which to filter the ListTasks
results. Specifying a
* family
limits the results to tasks that belong to that family.
*/
public final String family() {
return family;
}
/**
*
* The nextToken
value returned from a ListTasks
request indicating that more results are
* available to fulfill the request and further calls will be needed. If maxResults
was provided, it is
* possible the number of results to be fewer than maxResults
.
*
*
*
* This token should be treated as an opaque identifier that is only used to retrieve the next items in a list and
* not for other programmatic purposes.
*
*
*
* @return The nextToken
value returned from a ListTasks
request indicating that more
* results are available to fulfill the request and further calls will be needed. If maxResults
* was provided, it is possible the number of results to be fewer than maxResults
.
*
* This token should be treated as an opaque identifier that is only used to retrieve the next items in a
* list and not for other programmatic purposes.
*
*/
public final String nextToken() {
return nextToken;
}
/**
*
* The maximum number of task results returned by ListTasks
in paginated output. When this parameter is
* used, ListTasks
only returns maxResults
results in a single page along with a
* nextToken
response element. The remaining results of the initial request can be seen by sending
* another ListTasks
request with the returned nextToken
value. This value can be between
* 1 and 100. If this parameter is not used, then ListTasks
returns up to 100 results and a
* nextToken
value if applicable.
*
*
* @return The maximum number of task results returned by ListTasks
in paginated output. When this
* parameter is used, ListTasks
only returns maxResults
results in a single page
* along with a nextToken
response element. The remaining results of the initial request can be
* seen by sending another ListTasks
request with the returned nextToken
value.
* This value can be between 1 and 100. If this parameter is not used, then ListTasks
returns
* up to 100 results and a nextToken
value if applicable.
*/
public final Integer maxResults() {
return maxResults;
}
/**
*
* The startedBy
value with which to filter the task results. Specifying a startedBy
value
* limits the results to tasks that were started with that value.
*
*
* @return The startedBy
value with which to filter the task results. Specifying a
* startedBy
value limits the results to tasks that were started with that value.
*/
public final String startedBy() {
return startedBy;
}
/**
*
* The name of the service with which to filter the ListTasks
results. Specifying a
* serviceName
limits the results to tasks that belong to that service.
*
*
* @return The name of the service with which to filter the ListTasks
results. Specifying a
* serviceName
limits the results to tasks that belong to that service.
*/
public final String serviceName() {
return serviceName;
}
/**
*
* The task desired status with which to filter the ListTasks
results. Specifying a
* desiredStatus
of STOPPED
limits the results to tasks that Amazon ECS has set the
* desired status to STOPPED
. This can be useful for debugging tasks that are not starting properly or
* have died or finished. The default status filter is RUNNING
, which shows tasks that Amazon ECS has
* set the desired status to RUNNING
.
*
*
*
* Although you can filter results based on a desired status of PENDING
, this does not return any
* results. Amazon ECS never sets the desired status of a task to that value (only a task's lastStatus
* may have a value of PENDING
).
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #desiredStatus}
* will return {@link DesiredStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #desiredStatusAsString}.
*
*
* @return The task desired status with which to filter the ListTasks
results. Specifying a
* desiredStatus
of STOPPED
limits the results to tasks that Amazon ECS has set
* the desired status to STOPPED
. This can be useful for debugging tasks that are not starting
* properly or have died or finished. The default status filter is RUNNING
, which shows tasks
* that Amazon ECS has set the desired status to RUNNING
.
*
* Although you can filter results based on a desired status of PENDING
, this does not return
* any results. Amazon ECS never sets the desired status of a task to that value (only a task's
* lastStatus
may have a value of PENDING
).
*
* @see DesiredStatus
*/
public final DesiredStatus desiredStatus() {
return DesiredStatus.fromValue(desiredStatus);
}
/**
*
* The task desired status with which to filter the ListTasks
results. Specifying a
* desiredStatus
of STOPPED
limits the results to tasks that Amazon ECS has set the
* desired status to STOPPED
. This can be useful for debugging tasks that are not starting properly or
* have died or finished. The default status filter is RUNNING
, which shows tasks that Amazon ECS has
* set the desired status to RUNNING
.
*
*
*
* Although you can filter results based on a desired status of PENDING
, this does not return any
* results. Amazon ECS never sets the desired status of a task to that value (only a task's lastStatus
* may have a value of PENDING
).
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #desiredStatus}
* will return {@link DesiredStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #desiredStatusAsString}.
*
*
* @return The task desired status with which to filter the ListTasks
results. Specifying a
* desiredStatus
of STOPPED
limits the results to tasks that Amazon ECS has set
* the desired status to STOPPED
. This can be useful for debugging tasks that are not starting
* properly or have died or finished. The default status filter is RUNNING
, which shows tasks
* that Amazon ECS has set the desired status to RUNNING
.
*
* Although you can filter results based on a desired status of PENDING
, this does not return
* any results. Amazon ECS never sets the desired status of a task to that value (only a task's
* lastStatus
may have a value of PENDING
).
*
* @see DesiredStatus
*/
public final String desiredStatusAsString() {
return desiredStatus;
}
/**
*
* The launch type for services to list.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type for services to list.
* @see LaunchType
*/
public final LaunchType launchType() {
return LaunchType.fromValue(launchType);
}
/**
*
* The launch type for services to list.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type for services to list.
* @see LaunchType
*/
public final String launchTypeAsString() {
return launchType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(containerInstance());
hashCode = 31 * hashCode + Objects.hashCode(family());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
hashCode = 31 * hashCode + Objects.hashCode(maxResults());
hashCode = 31 * hashCode + Objects.hashCode(startedBy());
hashCode = 31 * hashCode + Objects.hashCode(serviceName());
hashCode = 31 * hashCode + Objects.hashCode(desiredStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(launchTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListTasksRequest)) {
return false;
}
ListTasksRequest other = (ListTasksRequest) obj;
return Objects.equals(cluster(), other.cluster()) && Objects.equals(containerInstance(), other.containerInstance())
&& Objects.equals(family(), other.family()) && Objects.equals(nextToken(), other.nextToken())
&& Objects.equals(maxResults(), other.maxResults()) && Objects.equals(startedBy(), other.startedBy())
&& Objects.equals(serviceName(), other.serviceName())
&& Objects.equals(desiredStatusAsString(), other.desiredStatusAsString())
&& Objects.equals(launchTypeAsString(), other.launchTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ListTasksRequest").add("Cluster", cluster()).add("ContainerInstance", containerInstance())
.add("Family", family()).add("NextToken", nextToken()).add("MaxResults", maxResults())
.add("StartedBy", startedBy()).add("ServiceName", serviceName()).add("DesiredStatus", desiredStatusAsString())
.add("LaunchType", launchTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "containerInstance":
return Optional.ofNullable(clazz.cast(containerInstance()));
case "family":
return Optional.ofNullable(clazz.cast(family()));
case "nextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
case "maxResults":
return Optional.ofNullable(clazz.cast(maxResults()));
case "startedBy":
return Optional.ofNullable(clazz.cast(startedBy()));
case "serviceName":
return Optional.ofNullable(clazz.cast(serviceName()));
case "desiredStatus":
return Optional.ofNullable(clazz.cast(desiredStatusAsString()));
case "launchType":
return Optional.ofNullable(clazz.cast(launchTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function