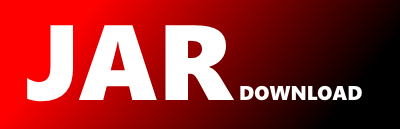
software.amazon.awssdk.services.ecs.model.NetworkInterface Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecs Show documentation
Show all versions of ecs Show documentation
The AWS Java SDK for the Amazon EC2 Container Service holds the client classes that are used for
communicating with the
Amazon EC2 Container Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object representing the elastic network interface for tasks that use the awsvpc
network mode.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NetworkInterface implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ATTACHMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("attachmentId").getter(getter(NetworkInterface::attachmentId)).setter(setter(Builder::attachmentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attachmentId").build()).build();
private static final SdkField PRIVATE_IPV4_ADDRESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("privateIpv4Address").getter(getter(NetworkInterface::privateIpv4Address))
.setter(setter(Builder::privateIpv4Address))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("privateIpv4Address").build())
.build();
private static final SdkField IPV6_ADDRESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ipv6Address").getter(getter(NetworkInterface::ipv6Address)).setter(setter(Builder::ipv6Address))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ipv6Address").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ATTACHMENT_ID_FIELD,
PRIVATE_IPV4_ADDRESS_FIELD, IPV6_ADDRESS_FIELD));
private static final long serialVersionUID = 1L;
private final String attachmentId;
private final String privateIpv4Address;
private final String ipv6Address;
private NetworkInterface(BuilderImpl builder) {
this.attachmentId = builder.attachmentId;
this.privateIpv4Address = builder.privateIpv4Address;
this.ipv6Address = builder.ipv6Address;
}
/**
*
* The attachment ID for the network interface.
*
*
* @return The attachment ID for the network interface.
*/
public final String attachmentId() {
return attachmentId;
}
/**
*
* The private IPv4 address for the network interface.
*
*
* @return The private IPv4 address for the network interface.
*/
public final String privateIpv4Address() {
return privateIpv4Address;
}
/**
*
* The private IPv6 address for the network interface.
*
*
* @return The private IPv6 address for the network interface.
*/
public final String ipv6Address() {
return ipv6Address;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(attachmentId());
hashCode = 31 * hashCode + Objects.hashCode(privateIpv4Address());
hashCode = 31 * hashCode + Objects.hashCode(ipv6Address());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NetworkInterface)) {
return false;
}
NetworkInterface other = (NetworkInterface) obj;
return Objects.equals(attachmentId(), other.attachmentId())
&& Objects.equals(privateIpv4Address(), other.privateIpv4Address())
&& Objects.equals(ipv6Address(), other.ipv6Address());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NetworkInterface").add("AttachmentId", attachmentId())
.add("PrivateIpv4Address", privateIpv4Address()).add("Ipv6Address", ipv6Address()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "attachmentId":
return Optional.ofNullable(clazz.cast(attachmentId()));
case "privateIpv4Address":
return Optional.ofNullable(clazz.cast(privateIpv4Address()));
case "ipv6Address":
return Optional.ofNullable(clazz.cast(ipv6Address()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy