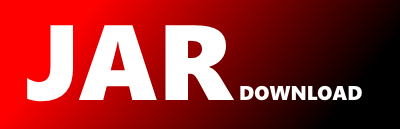
software.amazon.awssdk.services.ecs.model.TaskSet Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a set of Amazon ECS tasks in either an AWS CodeDeploy or an EXTERNAL
deployment. An
* Amazon ECS task set includes details such as the desired number of tasks, how many tasks are running, and whether the
* task set serves production traffic.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TaskSet implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("id")
.getter(getter(TaskSet::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("id").build()).build();
private static final SdkField TASK_SET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskSetArn").getter(getter(TaskSet::taskSetArn)).setter(setter(Builder::taskSetArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskSetArn").build()).build();
private static final SdkField SERVICE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("serviceArn").getter(getter(TaskSet::serviceArn)).setter(setter(Builder::serviceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceArn").build()).build();
private static final SdkField CLUSTER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("clusterArn").getter(getter(TaskSet::clusterArn)).setter(setter(Builder::clusterArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clusterArn").build()).build();
private static final SdkField STARTED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("startedBy").getter(getter(TaskSet::startedBy)).setter(setter(Builder::startedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startedBy").build()).build();
private static final SdkField EXTERNAL_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("externalId").getter(getter(TaskSet::externalId)).setter(setter(Builder::externalId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("externalId").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(TaskSet::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField TASK_DEFINITION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskDefinition").getter(getter(TaskSet::taskDefinition)).setter(setter(Builder::taskDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinition").build()).build();
private static final SdkField COMPUTED_DESIRED_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("computedDesiredCount").getter(getter(TaskSet::computedDesiredCount))
.setter(setter(Builder::computedDesiredCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("computedDesiredCount").build())
.build();
private static final SdkField PENDING_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("pendingCount").getter(getter(TaskSet::pendingCount)).setter(setter(Builder::pendingCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pendingCount").build()).build();
private static final SdkField RUNNING_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("runningCount").getter(getter(TaskSet::runningCount)).setter(setter(Builder::runningCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("runningCount").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("createdAt").getter(getter(TaskSet::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdAt").build()).build();
private static final SdkField UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("updatedAt").getter(getter(TaskSet::updatedAt)).setter(setter(Builder::updatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updatedAt").build()).build();
private static final SdkField LAUNCH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("launchType").getter(getter(TaskSet::launchTypeAsString)).setter(setter(Builder::launchType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("launchType").build()).build();
private static final SdkField> CAPACITY_PROVIDER_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("capacityProviderStrategy")
.getter(getter(TaskSet::capacityProviderStrategy))
.setter(setter(Builder::capacityProviderStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProviderStrategy").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CapacityProviderStrategyItem::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PLATFORM_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformVersion").getter(getter(TaskSet::platformVersion)).setter(setter(Builder::platformVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformVersion").build()).build();
private static final SdkField NETWORK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("networkConfiguration")
.getter(getter(TaskSet::networkConfiguration)).setter(setter(Builder::networkConfiguration))
.constructor(NetworkConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkConfiguration").build())
.build();
private static final SdkField> LOAD_BALANCERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("loadBalancers")
.getter(getter(TaskSet::loadBalancers))
.setter(setter(Builder::loadBalancers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("loadBalancers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LoadBalancer::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SERVICE_REGISTRIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("serviceRegistries")
.getter(getter(TaskSet::serviceRegistries))
.setter(setter(Builder::serviceRegistries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRegistries").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceRegistry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SCALE_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("scale")
.getter(getter(TaskSet::scale)).setter(setter(Builder::scale)).constructor(Scale::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scale").build()).build();
private static final SdkField STABILITY_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("stabilityStatus").getter(getter(TaskSet::stabilityStatusAsString))
.setter(setter(Builder::stabilityStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stabilityStatus").build()).build();
private static final SdkField STABILITY_STATUS_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("stabilityStatusAt").getter(getter(TaskSet::stabilityStatusAt))
.setter(setter(Builder::stabilityStatusAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stabilityStatusAt").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tags")
.getter(getter(TaskSet::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, TASK_SET_ARN_FIELD,
SERVICE_ARN_FIELD, CLUSTER_ARN_FIELD, STARTED_BY_FIELD, EXTERNAL_ID_FIELD, STATUS_FIELD, TASK_DEFINITION_FIELD,
COMPUTED_DESIRED_COUNT_FIELD, PENDING_COUNT_FIELD, RUNNING_COUNT_FIELD, CREATED_AT_FIELD, UPDATED_AT_FIELD,
LAUNCH_TYPE_FIELD, CAPACITY_PROVIDER_STRATEGY_FIELD, PLATFORM_VERSION_FIELD, NETWORK_CONFIGURATION_FIELD,
LOAD_BALANCERS_FIELD, SERVICE_REGISTRIES_FIELD, SCALE_FIELD, STABILITY_STATUS_FIELD, STABILITY_STATUS_AT_FIELD,
TAGS_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String taskSetArn;
private final String serviceArn;
private final String clusterArn;
private final String startedBy;
private final String externalId;
private final String status;
private final String taskDefinition;
private final Integer computedDesiredCount;
private final Integer pendingCount;
private final Integer runningCount;
private final Instant createdAt;
private final Instant updatedAt;
private final String launchType;
private final List capacityProviderStrategy;
private final String platformVersion;
private final NetworkConfiguration networkConfiguration;
private final List loadBalancers;
private final List serviceRegistries;
private final Scale scale;
private final String stabilityStatus;
private final Instant stabilityStatusAt;
private final List tags;
private TaskSet(BuilderImpl builder) {
this.id = builder.id;
this.taskSetArn = builder.taskSetArn;
this.serviceArn = builder.serviceArn;
this.clusterArn = builder.clusterArn;
this.startedBy = builder.startedBy;
this.externalId = builder.externalId;
this.status = builder.status;
this.taskDefinition = builder.taskDefinition;
this.computedDesiredCount = builder.computedDesiredCount;
this.pendingCount = builder.pendingCount;
this.runningCount = builder.runningCount;
this.createdAt = builder.createdAt;
this.updatedAt = builder.updatedAt;
this.launchType = builder.launchType;
this.capacityProviderStrategy = builder.capacityProviderStrategy;
this.platformVersion = builder.platformVersion;
this.networkConfiguration = builder.networkConfiguration;
this.loadBalancers = builder.loadBalancers;
this.serviceRegistries = builder.serviceRegistries;
this.scale = builder.scale;
this.stabilityStatus = builder.stabilityStatus;
this.stabilityStatusAt = builder.stabilityStatusAt;
this.tags = builder.tags;
}
/**
*
* The ID of the task set.
*
*
* @return The ID of the task set.
*/
public final String id() {
return id;
}
/**
*
* The Amazon Resource Name (ARN) of the task set.
*
*
* @return The Amazon Resource Name (ARN) of the task set.
*/
public final String taskSetArn() {
return taskSetArn;
}
/**
*
* The Amazon Resource Name (ARN) of the service the task set exists in.
*
*
* @return The Amazon Resource Name (ARN) of the service the task set exists in.
*/
public final String serviceArn() {
return serviceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster that the service that hosts the task set exists in.
*
*
* @return The Amazon Resource Name (ARN) of the cluster that the service that hosts the task set exists in.
*/
public final String clusterArn() {
return clusterArn;
}
/**
*
* The tag specified when a task set is started. If the task set is created by an AWS CodeDeploy deployment, the
* startedBy
parameter is CODE_DEPLOY
. For a task set created for an external deployment,
* the startedBy field isn't used.
*
*
* @return The tag specified when a task set is started. If the task set is created by an AWS CodeDeploy deployment,
* the startedBy
parameter is CODE_DEPLOY
. For a task set created for an external
* deployment, the startedBy field isn't used.
*/
public final String startedBy() {
return startedBy;
}
/**
*
* The external ID associated with the task set.
*
*
* If a task set is created by an AWS CodeDeploy deployment, the externalId
parameter contains the AWS
* CodeDeploy deployment ID.
*
*
* If a task set is created for an external deployment and is associated with a service discovery registry, the
* externalId
parameter contains the ECS_TASK_SET_EXTERNAL_ID
AWS Cloud Map attribute.
*
*
* @return The external ID associated with the task set.
*
* If a task set is created by an AWS CodeDeploy deployment, the externalId
parameter contains
* the AWS CodeDeploy deployment ID.
*
*
* If a task set is created for an external deployment and is associated with a service discovery registry,
* the externalId
parameter contains the ECS_TASK_SET_EXTERNAL_ID
AWS Cloud Map
* attribute.
*/
public final String externalId() {
return externalId;
}
/**
*
* The status of the task set. The following describes each state:
*
*
* - PRIMARY
* -
*
* The task set is serving production traffic.
*
*
* - ACTIVE
* -
*
* The task set is not serving production traffic.
*
*
* - DRAINING
* -
*
* The tasks in the task set are being stopped and their corresponding targets are being deregistered from their
* target group.
*
*
*
*
* @return The status of the task set. The following describes each state:
*
* - PRIMARY
* -
*
* The task set is serving production traffic.
*
*
* - ACTIVE
* -
*
* The task set is not serving production traffic.
*
*
* - DRAINING
* -
*
* The tasks in the task set are being stopped and their corresponding targets are being deregistered from
* their target group.
*
*
*/
public final String status() {
return status;
}
/**
*
* The task definition the task set is using.
*
*
* @return The task definition the task set is using.
*/
public final String taskDefinition() {
return taskDefinition;
}
/**
*
* The computed desired count for the task set. This is calculated by multiplying the service's
* desiredCount
by the task set's scale
percentage. The result is always rounded up. For
* example, if the computed desired count is 1.2, it rounds up to 2 tasks.
*
*
* @return The computed desired count for the task set. This is calculated by multiplying the service's
* desiredCount
by the task set's scale
percentage. The result is always rounded
* up. For example, if the computed desired count is 1.2, it rounds up to 2 tasks.
*/
public final Integer computedDesiredCount() {
return computedDesiredCount;
}
/**
*
* The number of tasks in the task set that are in the PENDING
status during a deployment. A task in
* the PENDING
state is preparing to enter the RUNNING
state. A task set enters the
* PENDING
status when it launches for the first time or when it is restarted after being in the
* STOPPED
state.
*
*
* @return The number of tasks in the task set that are in the PENDING
status during a deployment. A
* task in the PENDING
state is preparing to enter the RUNNING
state. A task set
* enters the PENDING
status when it launches for the first time or when it is restarted after
* being in the STOPPED
state.
*/
public final Integer pendingCount() {
return pendingCount;
}
/**
*
* The number of tasks in the task set that are in the RUNNING
status during a deployment. A task in
* the RUNNING
state is running and ready for use.
*
*
* @return The number of tasks in the task set that are in the RUNNING
status during a deployment. A
* task in the RUNNING
state is running and ready for use.
*/
public final Integer runningCount() {
return runningCount;
}
/**
*
* The Unix timestamp for when the task set was created.
*
*
* @return The Unix timestamp for when the task set was created.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The Unix timestamp for when the task set was last updated.
*
*
* @return The Unix timestamp for when the task set was last updated.
*/
public final Instant updatedAt() {
return updatedAt;
}
/**
*
* The launch type the tasks in the task set are using. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type the tasks in the task set are using. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public final LaunchType launchType() {
return LaunchType.fromValue(launchType);
}
/**
*
* The launch type the tasks in the task set are using. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type the tasks in the task set are using. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public final String launchTypeAsString() {
return launchType;
}
/**
* Returns true if the CapacityProviderStrategy property was specified by the sender (it may be empty), or false if
* the sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasCapacityProviderStrategy() {
return capacityProviderStrategy != null && !(capacityProviderStrategy instanceof SdkAutoConstructList);
}
/**
*
* The capacity provider strategy associated with the task set.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasCapacityProviderStrategy()} to see if a value was sent in this field.
*
*
* @return The capacity provider strategy associated with the task set.
*/
public final List capacityProviderStrategy() {
return capacityProviderStrategy;
}
/**
*
* The AWS Fargate platform version on which the tasks in the task set are running. A platform version is only
* specified for tasks run on AWS Fargate. For more information, see AWS Fargate platform
* versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The AWS Fargate platform version on which the tasks in the task set are running. A platform version is
* only specified for tasks run on AWS Fargate. For more information, see AWS Fargate
* platform versions in the Amazon Elastic Container Service Developer Guide.
*/
public final String platformVersion() {
return platformVersion;
}
/**
*
* The network configuration for the task set.
*
*
* @return The network configuration for the task set.
*/
public final NetworkConfiguration networkConfiguration() {
return networkConfiguration;
}
/**
* Returns true if the LoadBalancers property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasLoadBalancers() {
return loadBalancers != null && !(loadBalancers instanceof SdkAutoConstructList);
}
/**
*
* Details on a load balancer that is used with a task set.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasLoadBalancers()} to see if a value was sent in this field.
*
*
* @return Details on a load balancer that is used with a task set.
*/
public final List loadBalancers() {
return loadBalancers;
}
/**
* Returns true if the ServiceRegistries property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasServiceRegistries() {
return serviceRegistries != null && !(serviceRegistries instanceof SdkAutoConstructList);
}
/**
*
* The details of the service discovery registries to assign to this task set. For more information, see Service discovery.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasServiceRegistries()} to see if a value was sent in this field.
*
*
* @return The details of the service discovery registries to assign to this task set. For more information, see Service
* discovery.
*/
public final List serviceRegistries() {
return serviceRegistries;
}
/**
*
* A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*
*
* @return A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*/
public final Scale scale() {
return scale;
}
/**
*
* The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stabilityStatus}
* will return {@link StabilityStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #stabilityStatusAsString}.
*
*
* @return The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health
* checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
* @see StabilityStatus
*/
public final StabilityStatus stabilityStatus() {
return StabilityStatus.fromValue(stabilityStatus);
}
/**
*
* The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stabilityStatus}
* will return {@link StabilityStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #stabilityStatusAsString}.
*
*
* @return The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health
* checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
* @see StabilityStatus
*/
public final String stabilityStatusAsString() {
return stabilityStatus;
}
/**
*
* The Unix timestamp for when the task set stability status was retrieved.
*
*
* @return The Unix timestamp for when the task set stability status was retrieved.
*/
public final Instant stabilityStatusAt() {
return stabilityStatusAt;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The metadata that you apply to the task set to help you categorize and organize them. Each tag consists of a key
* and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return The metadata that you apply to the task set to help you categorize and organize them. Each tag consists
* of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or
* values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(taskSetArn());
hashCode = 31 * hashCode + Objects.hashCode(serviceArn());
hashCode = 31 * hashCode + Objects.hashCode(clusterArn());
hashCode = 31 * hashCode + Objects.hashCode(startedBy());
hashCode = 31 * hashCode + Objects.hashCode(externalId());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(taskDefinition());
hashCode = 31 * hashCode + Objects.hashCode(computedDesiredCount());
hashCode = 31 * hashCode + Objects.hashCode(pendingCount());
hashCode = 31 * hashCode + Objects.hashCode(runningCount());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(updatedAt());
hashCode = 31 * hashCode + Objects.hashCode(launchTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasCapacityProviderStrategy() ? capacityProviderStrategy() : null);
hashCode = 31 * hashCode + Objects.hashCode(platformVersion());
hashCode = 31 * hashCode + Objects.hashCode(networkConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasLoadBalancers() ? loadBalancers() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasServiceRegistries() ? serviceRegistries() : null);
hashCode = 31 * hashCode + Objects.hashCode(scale());
hashCode = 31 * hashCode + Objects.hashCode(stabilityStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(stabilityStatusAt());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TaskSet)) {
return false;
}
TaskSet other = (TaskSet) obj;
return Objects.equals(id(), other.id()) && Objects.equals(taskSetArn(), other.taskSetArn())
&& Objects.equals(serviceArn(), other.serviceArn()) && Objects.equals(clusterArn(), other.clusterArn())
&& Objects.equals(startedBy(), other.startedBy()) && Objects.equals(externalId(), other.externalId())
&& Objects.equals(status(), other.status()) && Objects.equals(taskDefinition(), other.taskDefinition())
&& Objects.equals(computedDesiredCount(), other.computedDesiredCount())
&& Objects.equals(pendingCount(), other.pendingCount()) && Objects.equals(runningCount(), other.runningCount())
&& Objects.equals(createdAt(), other.createdAt()) && Objects.equals(updatedAt(), other.updatedAt())
&& Objects.equals(launchTypeAsString(), other.launchTypeAsString())
&& hasCapacityProviderStrategy() == other.hasCapacityProviderStrategy()
&& Objects.equals(capacityProviderStrategy(), other.capacityProviderStrategy())
&& Objects.equals(platformVersion(), other.platformVersion())
&& Objects.equals(networkConfiguration(), other.networkConfiguration())
&& hasLoadBalancers() == other.hasLoadBalancers() && Objects.equals(loadBalancers(), other.loadBalancers())
&& hasServiceRegistries() == other.hasServiceRegistries()
&& Objects.equals(serviceRegistries(), other.serviceRegistries()) && Objects.equals(scale(), other.scale())
&& Objects.equals(stabilityStatusAsString(), other.stabilityStatusAsString())
&& Objects.equals(stabilityStatusAt(), other.stabilityStatusAt()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TaskSet").add("Id", id()).add("TaskSetArn", taskSetArn()).add("ServiceArn", serviceArn())
.add("ClusterArn", clusterArn()).add("StartedBy", startedBy()).add("ExternalId", externalId())
.add("Status", status()).add("TaskDefinition", taskDefinition())
.add("ComputedDesiredCount", computedDesiredCount()).add("PendingCount", pendingCount())
.add("RunningCount", runningCount()).add("CreatedAt", createdAt()).add("UpdatedAt", updatedAt())
.add("LaunchType", launchTypeAsString())
.add("CapacityProviderStrategy", hasCapacityProviderStrategy() ? capacityProviderStrategy() : null)
.add("PlatformVersion", platformVersion()).add("NetworkConfiguration", networkConfiguration())
.add("LoadBalancers", hasLoadBalancers() ? loadBalancers() : null)
.add("ServiceRegistries", hasServiceRegistries() ? serviceRegistries() : null).add("Scale", scale())
.add("StabilityStatus", stabilityStatusAsString()).add("StabilityStatusAt", stabilityStatusAt())
.add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "id":
return Optional.ofNullable(clazz.cast(id()));
case "taskSetArn":
return Optional.ofNullable(clazz.cast(taskSetArn()));
case "serviceArn":
return Optional.ofNullable(clazz.cast(serviceArn()));
case "clusterArn":
return Optional.ofNullable(clazz.cast(clusterArn()));
case "startedBy":
return Optional.ofNullable(clazz.cast(startedBy()));
case "externalId":
return Optional.ofNullable(clazz.cast(externalId()));
case "status":
return Optional.ofNullable(clazz.cast(status()));
case "taskDefinition":
return Optional.ofNullable(clazz.cast(taskDefinition()));
case "computedDesiredCount":
return Optional.ofNullable(clazz.cast(computedDesiredCount()));
case "pendingCount":
return Optional.ofNullable(clazz.cast(pendingCount()));
case "runningCount":
return Optional.ofNullable(clazz.cast(runningCount()));
case "createdAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "updatedAt":
return Optional.ofNullable(clazz.cast(updatedAt()));
case "launchType":
return Optional.ofNullable(clazz.cast(launchTypeAsString()));
case "capacityProviderStrategy":
return Optional.ofNullable(clazz.cast(capacityProviderStrategy()));
case "platformVersion":
return Optional.ofNullable(clazz.cast(platformVersion()));
case "networkConfiguration":
return Optional.ofNullable(clazz.cast(networkConfiguration()));
case "loadBalancers":
return Optional.ofNullable(clazz.cast(loadBalancers()));
case "serviceRegistries":
return Optional.ofNullable(clazz.cast(serviceRegistries()));
case "scale":
return Optional.ofNullable(clazz.cast(scale()));
case "stabilityStatus":
return Optional.ofNullable(clazz.cast(stabilityStatusAsString()));
case "stabilityStatusAt":
return Optional.ofNullable(clazz.cast(stabilityStatusAt()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function