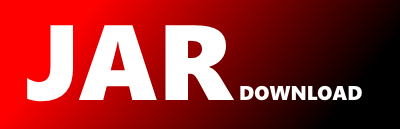
software.amazon.awssdk.services.ecs.model.ExecuteCommandLogConfiguration Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The log configuration for the results of the execute command actions. The logs can be sent to CloudWatch Logs or an
* Amazon S3 bucket.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ExecuteCommandLogConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CLOUD_WATCH_LOG_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("cloudWatchLogGroupName").getter(getter(ExecuteCommandLogConfiguration::cloudWatchLogGroupName))
.setter(setter(Builder::cloudWatchLogGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cloudWatchLogGroupName").build())
.build();
private static final SdkField CLOUD_WATCH_ENCRYPTION_ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("cloudWatchEncryptionEnabled")
.getter(getter(ExecuteCommandLogConfiguration::cloudWatchEncryptionEnabled))
.setter(setter(Builder::cloudWatchEncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cloudWatchEncryptionEnabled")
.build()).build();
private static final SdkField S3_BUCKET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("s3BucketName").getter(getter(ExecuteCommandLogConfiguration::s3BucketName))
.setter(setter(Builder::s3BucketName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("s3BucketName").build()).build();
private static final SdkField S3_ENCRYPTION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("s3EncryptionEnabled").getter(getter(ExecuteCommandLogConfiguration::s3EncryptionEnabled))
.setter(setter(Builder::s3EncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("s3EncryptionEnabled").build())
.build();
private static final SdkField S3_KEY_PREFIX_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("s3KeyPrefix").getter(getter(ExecuteCommandLogConfiguration::s3KeyPrefix))
.setter(setter(Builder::s3KeyPrefix))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("s3KeyPrefix").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
CLOUD_WATCH_LOG_GROUP_NAME_FIELD, CLOUD_WATCH_ENCRYPTION_ENABLED_FIELD, S3_BUCKET_NAME_FIELD,
S3_ENCRYPTION_ENABLED_FIELD, S3_KEY_PREFIX_FIELD));
private static final long serialVersionUID = 1L;
private final String cloudWatchLogGroupName;
private final Boolean cloudWatchEncryptionEnabled;
private final String s3BucketName;
private final Boolean s3EncryptionEnabled;
private final String s3KeyPrefix;
private ExecuteCommandLogConfiguration(BuilderImpl builder) {
this.cloudWatchLogGroupName = builder.cloudWatchLogGroupName;
this.cloudWatchEncryptionEnabled = builder.cloudWatchEncryptionEnabled;
this.s3BucketName = builder.s3BucketName;
this.s3EncryptionEnabled = builder.s3EncryptionEnabled;
this.s3KeyPrefix = builder.s3KeyPrefix;
}
/**
*
* The name of the CloudWatch log group to send logs to.
*
*
*
* The CloudWatch log group must already be created.
*
*
*
* @return The name of the CloudWatch log group to send logs to.
*
* The CloudWatch log group must already be created.
*
*/
public final String cloudWatchLogGroupName() {
return cloudWatchLogGroupName;
}
/**
*
* Determines whether to enable encryption on the CloudWatch logs. If not specified, encryption will be disabled.
*
*
* @return Determines whether to enable encryption on the CloudWatch logs. If not specified, encryption will be
* disabled.
*/
public final Boolean cloudWatchEncryptionEnabled() {
return cloudWatchEncryptionEnabled;
}
/**
*
* The name of the S3 bucket to send logs to.
*
*
*
* The S3 bucket must already be created.
*
*
*
* @return The name of the S3 bucket to send logs to.
*
* The S3 bucket must already be created.
*
*/
public final String s3BucketName() {
return s3BucketName;
}
/**
*
* Determines whether to use encryption on the S3 logs. If not specified, encryption is not used.
*
*
* @return Determines whether to use encryption on the S3 logs. If not specified, encryption is not used.
*/
public final Boolean s3EncryptionEnabled() {
return s3EncryptionEnabled;
}
/**
*
* An optional folder in the S3 bucket to place logs in.
*
*
* @return An optional folder in the S3 bucket to place logs in.
*/
public final String s3KeyPrefix() {
return s3KeyPrefix;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(cloudWatchLogGroupName());
hashCode = 31 * hashCode + Objects.hashCode(cloudWatchEncryptionEnabled());
hashCode = 31 * hashCode + Objects.hashCode(s3BucketName());
hashCode = 31 * hashCode + Objects.hashCode(s3EncryptionEnabled());
hashCode = 31 * hashCode + Objects.hashCode(s3KeyPrefix());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ExecuteCommandLogConfiguration)) {
return false;
}
ExecuteCommandLogConfiguration other = (ExecuteCommandLogConfiguration) obj;
return Objects.equals(cloudWatchLogGroupName(), other.cloudWatchLogGroupName())
&& Objects.equals(cloudWatchEncryptionEnabled(), other.cloudWatchEncryptionEnabled())
&& Objects.equals(s3BucketName(), other.s3BucketName())
&& Objects.equals(s3EncryptionEnabled(), other.s3EncryptionEnabled())
&& Objects.equals(s3KeyPrefix(), other.s3KeyPrefix());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ExecuteCommandLogConfiguration").add("CloudWatchLogGroupName", cloudWatchLogGroupName())
.add("CloudWatchEncryptionEnabled", cloudWatchEncryptionEnabled()).add("S3BucketName", s3BucketName())
.add("S3EncryptionEnabled", s3EncryptionEnabled()).add("S3KeyPrefix", s3KeyPrefix()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cloudWatchLogGroupName":
return Optional.ofNullable(clazz.cast(cloudWatchLogGroupName()));
case "cloudWatchEncryptionEnabled":
return Optional.ofNullable(clazz.cast(cloudWatchEncryptionEnabled()));
case "s3BucketName":
return Optional.ofNullable(clazz.cast(s3BucketName()));
case "s3EncryptionEnabled":
return Optional.ofNullable(clazz.cast(s3EncryptionEnabled()));
case "s3KeyPrefix":
return Optional.ofNullable(clazz.cast(s3KeyPrefix()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function