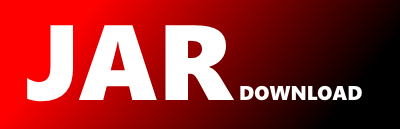
software.amazon.awssdk.services.ecs.model.UpdateTaskSetRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecs Show documentation
Show all versions of ecs Show documentation
The AWS Java SDK for the Amazon EC2 Container Service holds the client classes that are used for
communicating with the
Amazon EC2 Container Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateTaskSetRequest extends EcsRequest implements
ToCopyableBuilder {
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cluster")
.getter(getter(UpdateTaskSetRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField SERVICE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("service")
.getter(getter(UpdateTaskSetRequest::service)).setter(setter(Builder::service))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("service").build()).build();
private static final SdkField TASK_SET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskSet").getter(getter(UpdateTaskSetRequest::taskSet)).setter(setter(Builder::taskSet))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskSet").build()).build();
private static final SdkField SCALE_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("scale")
.getter(getter(UpdateTaskSetRequest::scale)).setter(setter(Builder::scale)).constructor(Scale::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scale").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_FIELD, SERVICE_FIELD,
TASK_SET_FIELD, SCALE_FIELD));
private final String cluster;
private final String service;
private final String taskSet;
private final Scale scale;
private UpdateTaskSetRequest(BuilderImpl builder) {
super(builder);
this.cluster = builder.cluster;
this.service = builder.service;
this.taskSet = builder.taskSet;
this.scale = builder.scale;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster that hosts the service that the task set is
* found in.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster that hosts the service that the task set
* is found in.
*/
public final String cluster() {
return cluster;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the service that the task set is found in.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the service that the task set is found in.
*/
public final String service() {
return service;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the task set to update.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the task set to update.
*/
public final String taskSet() {
return taskSet;
}
/**
*
* A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*
*
* @return A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*/
public final Scale scale() {
return scale;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(service());
hashCode = 31 * hashCode + Objects.hashCode(taskSet());
hashCode = 31 * hashCode + Objects.hashCode(scale());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateTaskSetRequest)) {
return false;
}
UpdateTaskSetRequest other = (UpdateTaskSetRequest) obj;
return Objects.equals(cluster(), other.cluster()) && Objects.equals(service(), other.service())
&& Objects.equals(taskSet(), other.taskSet()) && Objects.equals(scale(), other.scale());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateTaskSetRequest").add("Cluster", cluster()).add("Service", service())
.add("TaskSet", taskSet()).add("Scale", scale()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "service":
return Optional.ofNullable(clazz.cast(service()));
case "taskSet":
return Optional.ofNullable(clazz.cast(taskSet()));
case "scale":
return Optional.ofNullable(clazz.cast(scale()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy