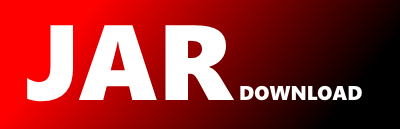
software.amazon.awssdk.services.ecs.model.ClusterSetting Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The settings to use when creating a cluster. This parameter is used to enable CloudWatch Container Insights for a
* cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ClusterSetting implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(ClusterSetting::nameAsString)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField VALUE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("value")
.getter(getter(ClusterSetting::value)).setter(setter(Builder::value))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("value").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, VALUE_FIELD));
private static final long serialVersionUID = 1L;
private final String name;
private final String value;
private ClusterSetting(BuilderImpl builder) {
this.name = builder.name;
this.value = builder.value;
}
/**
*
* The name of the cluster setting. The only supported value is containerInsights
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #name} will return
* {@link ClusterSettingName#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #nameAsString}.
*
*
* @return The name of the cluster setting. The only supported value is containerInsights
.
* @see ClusterSettingName
*/
public final ClusterSettingName name() {
return ClusterSettingName.fromValue(name);
}
/**
*
* The name of the cluster setting. The only supported value is containerInsights
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #name} will return
* {@link ClusterSettingName#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #nameAsString}.
*
*
* @return The name of the cluster setting. The only supported value is containerInsights
.
* @see ClusterSettingName
*/
public final String nameAsString() {
return name;
}
/**
*
* The value to set for the cluster setting. The supported values are enabled
and disabled
* . If enabled
is specified, CloudWatch Container Insights will be enabled for the cluster, otherwise
* it will be disabled unless the containerInsights
account setting is enabled. If a cluster value is
* specified, it will override the containerInsights
value set with PutAccountSetting or
* PutAccountSettingDefault.
*
*
* @return The value to set for the cluster setting. The supported values are enabled
and
* disabled
. If enabled
is specified, CloudWatch Container Insights will be
* enabled for the cluster, otherwise it will be disabled unless the containerInsights
account
* setting is enabled. If a cluster value is specified, it will override the containerInsights
* value set with PutAccountSetting or PutAccountSettingDefault.
*/
public final String value() {
return value;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(nameAsString());
hashCode = 31 * hashCode + Objects.hashCode(value());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ClusterSetting)) {
return false;
}
ClusterSetting other = (ClusterSetting) obj;
return Objects.equals(nameAsString(), other.nameAsString()) && Objects.equals(value(), other.value());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ClusterSetting").add("Name", nameAsString()).add("Value", value()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(nameAsString()));
case "value":
return Optional.ofNullable(clazz.cast(value()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function